Raspberry Pi Pico - OLED 128x64
This tutorial instructs you how to use a Raspberry Pi Pico with an OLED 128x64 I2C display. We will cover:
- How to connect a 128x64 OLED screen with a Raspberry Pi Pico.
- How to program the Raspberry Pi Pico to display text, integer and float number on an OLED.
- How to automatically vertical and horizontal center align text/number on OLED.
- How to program the Raspberry Pi Pico to draw shape on an OLED.
- How to program the Raspberry Pi Pico to display image on an OLED.
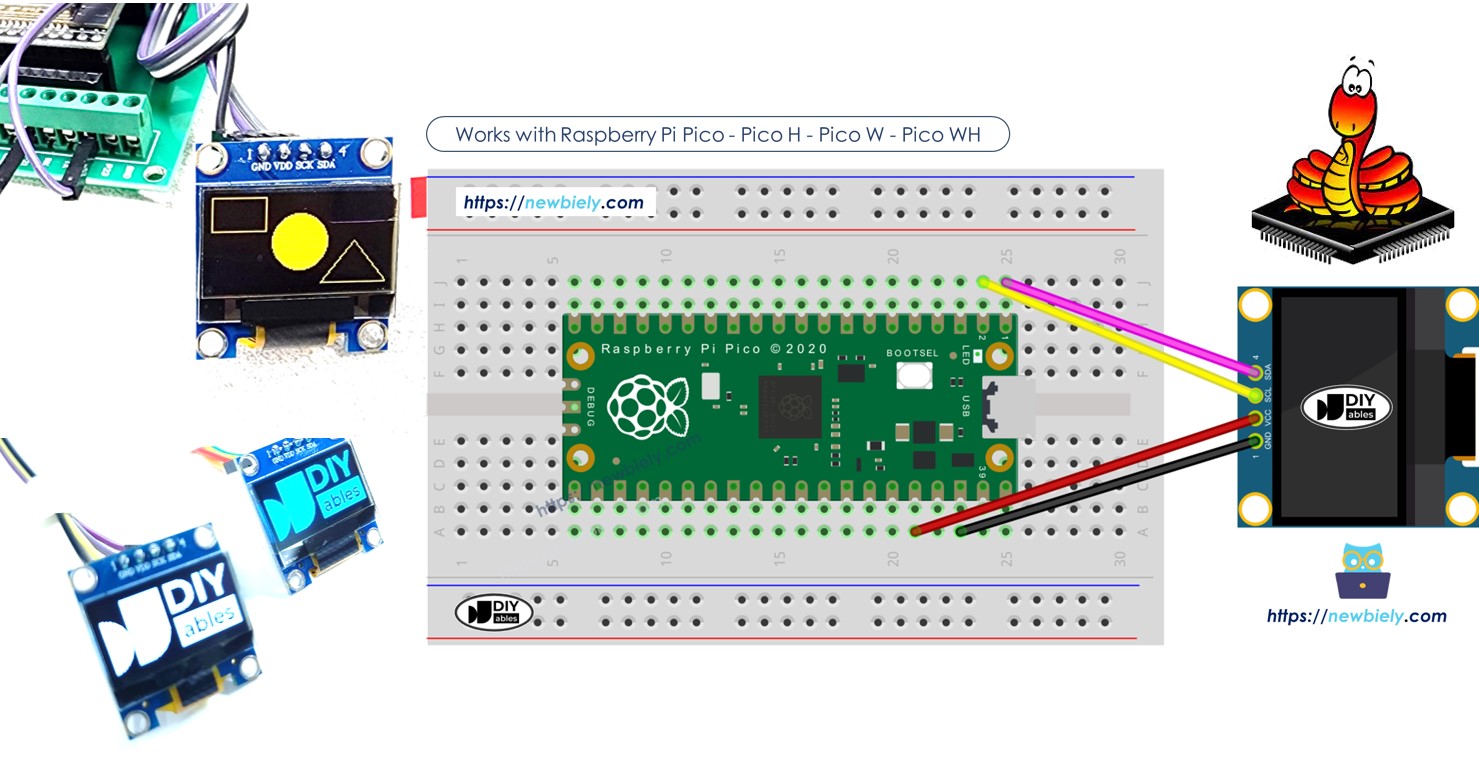
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of OLED Display
OLED displays come in various types, each differing in communication protocols, sizes, and color options.
- Communication protocols: I2C, SPI
- Sizes: 128x64, 128x32
- Available colors: white, blue, yellow, and more
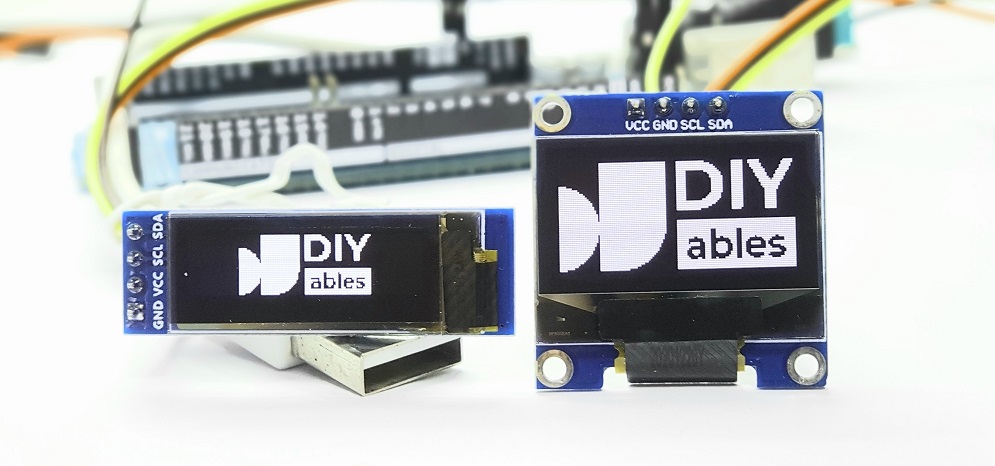
SPI vs I2C for OLED Displays
- SPI: Faster but requires more pins on the Raspberry Pi Pico.
- I2C: Uses fewer pins and allows connection to multiple devices.
Choose SPI for faster data transfer or I2C for fewer pins. This guide uses the 128x64 SSD1306 I2C OLED Display.
I2C OLED Display Pinout
- GND pin: Connect to Raspberry Pi Pico ground.
- VCC pin: Connect to 5V or 3.3V on Raspberry Pi Pico.
- SCL pin: Clock pin for I2C interface.
- SDA pin: Data pin for I2C interface.
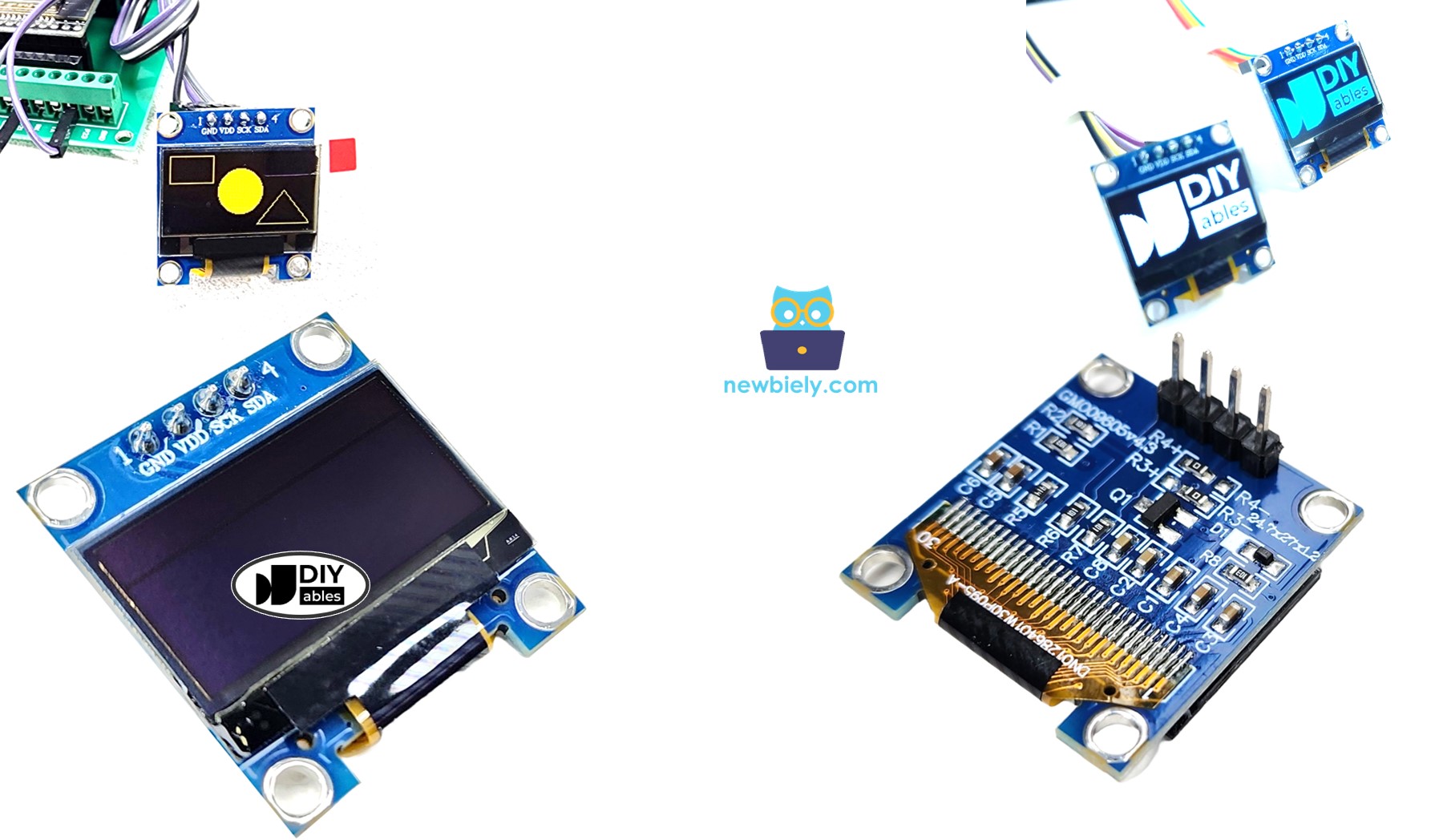
※ NOTE THAT:
This guide is for using an OLED display with the SSD1306 I2C driver. We tested it with an OLED display from DIYables, and it worked flawlessly.
Wiring Diagram
- How to Connect Raspberry Pi Pico to OLED 128x64 Screen
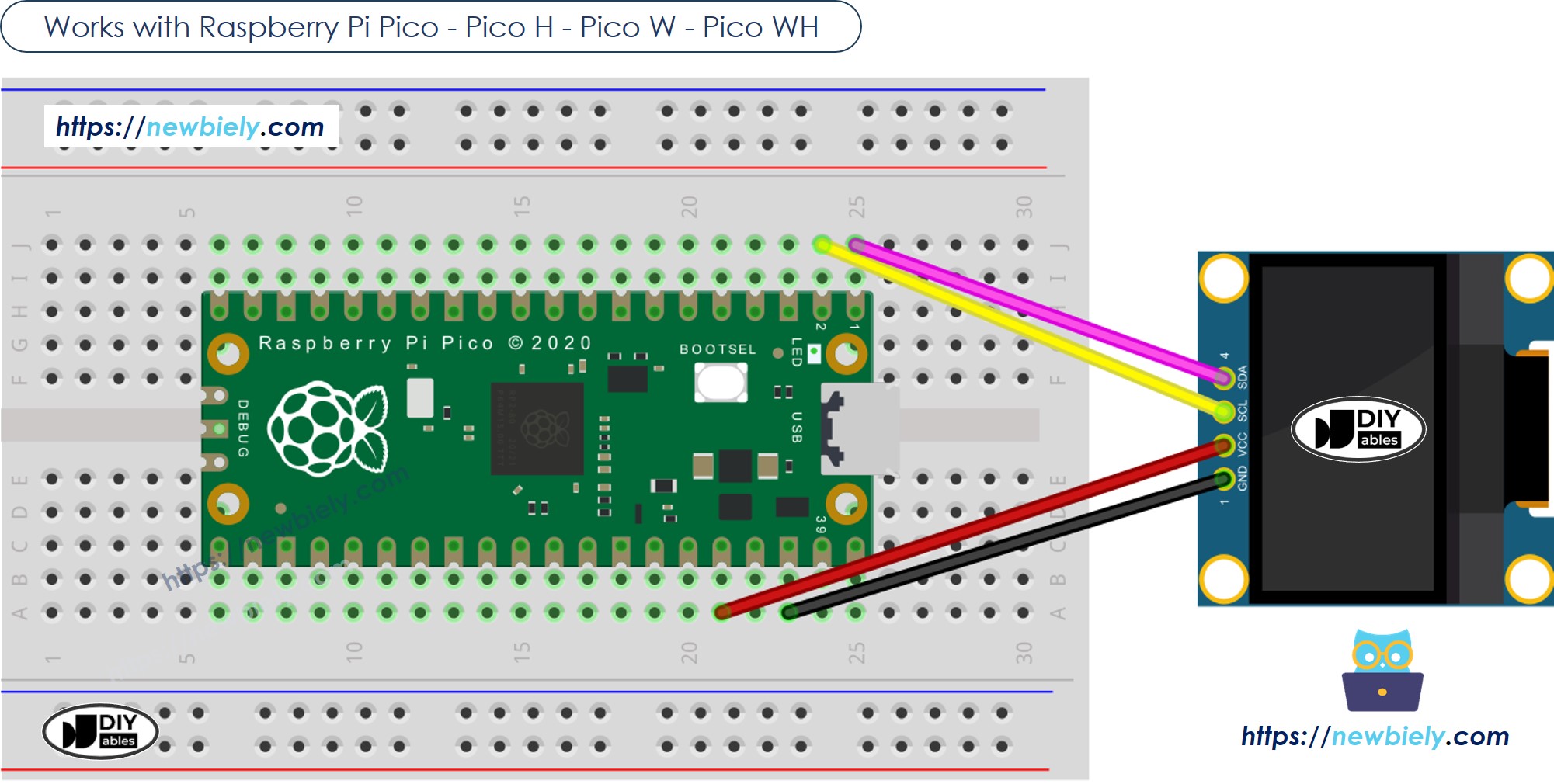
This image is created using Fritzing. Click to enlarge image
Check the table below for details on other Raspberry Pi Pico models.
OLED Module | Raspberry Pi Pico |
---|---|
VCC | 3.3V |
GND | GND |
SDA | GP0 |
SCL | GP1 |
Raspberry Pi Pico Code - Display Text, Integer and Float Number on OLED
Detailed Instructions
Please follow these instructions step by step:
- Ensure that Thonny IDE is installed on your computer.
- Ensure that MicroPython firmware is installed on your Raspberry Pi Pico.
- If this is your first time using a Raspberry Pico, refer to the Raspberry Pi Pico - Getting Started tutorial for detailed instructions.
- Connect the OLED display to the Raspberry Pi Pico according to the provided diagram.
- Connect the Raspberry Pi Pico to your computer using a USB cable.
- Launch the Thonny IDE on your computer.
- On Thonny IDE, select MicroPython (Raspberry Pi Pico) Interpreter by navigating to Tools Options.
- In the Interpreter tab, select MicroPython (Raspberry Pi Pico) from the drop-down menu.
- Ensure the correct port is selected. Thonny IDE should automatically detect the port, but you may need to select it manually (e.g., COM3 on Windows or /dev/ttyACM0 on Linux).
- Navigate to the Tools Manage packages on the Thonny IDE.
- Search “DIYables-MicroPython-OLED”, then find the OLED library created by DIYables.
- Click on DIYables-MicroPython-OLED, then click Install button to install OLED library.
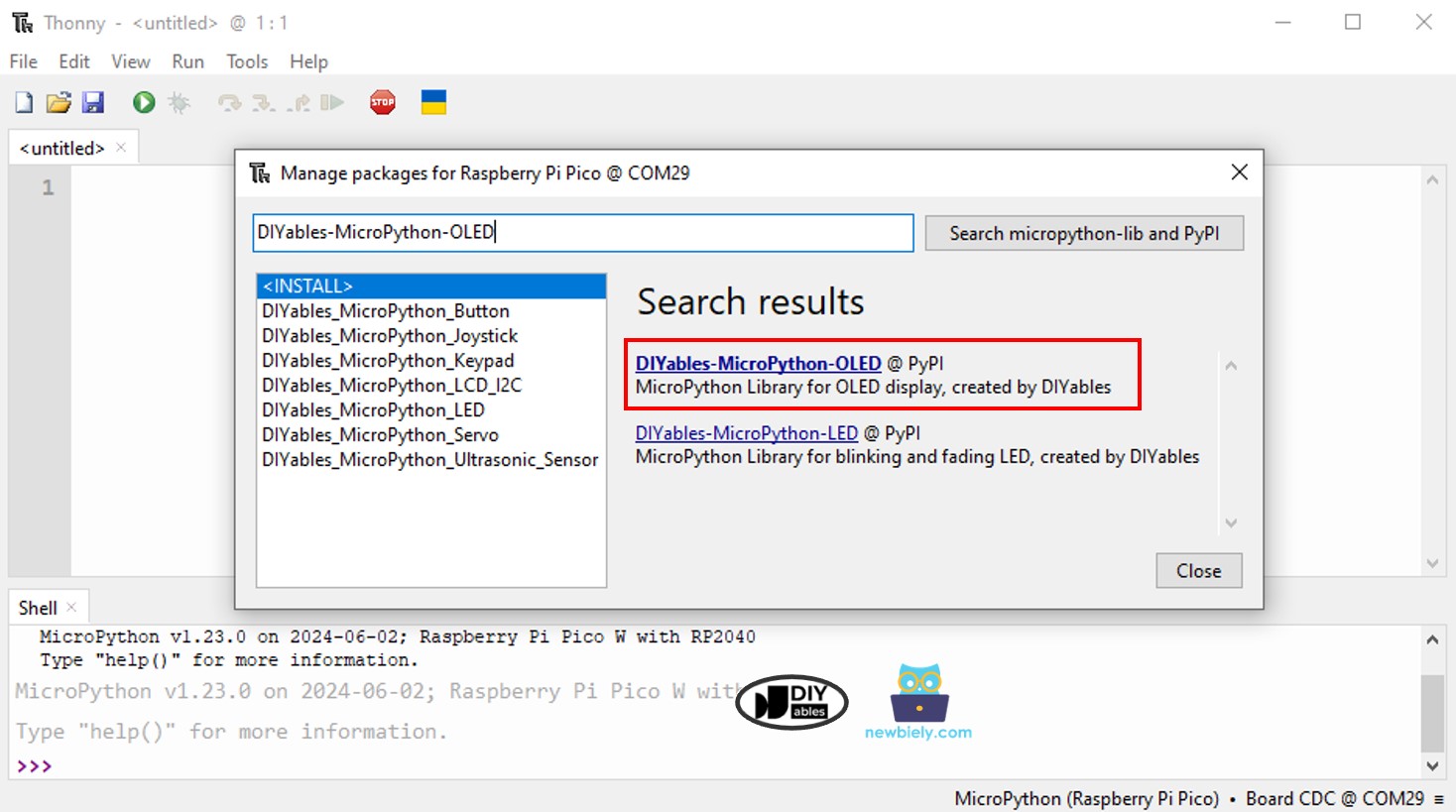
- Copy the above code and paste it to the Thonny IDE's editor.
- Save the script to your Raspberry Pi Pico by:
- Click the Save button, or use Ctrl+S keys.
- In the save dialog, you will see two sections: This computer and Raspberry Pi Pico. Select Raspberry Pi Pico
- Save the file as main.py
- Click the green Run button (or press F5) to run the script. The script will execute.
- Take a look at the output on the OLED display. It looks like this:
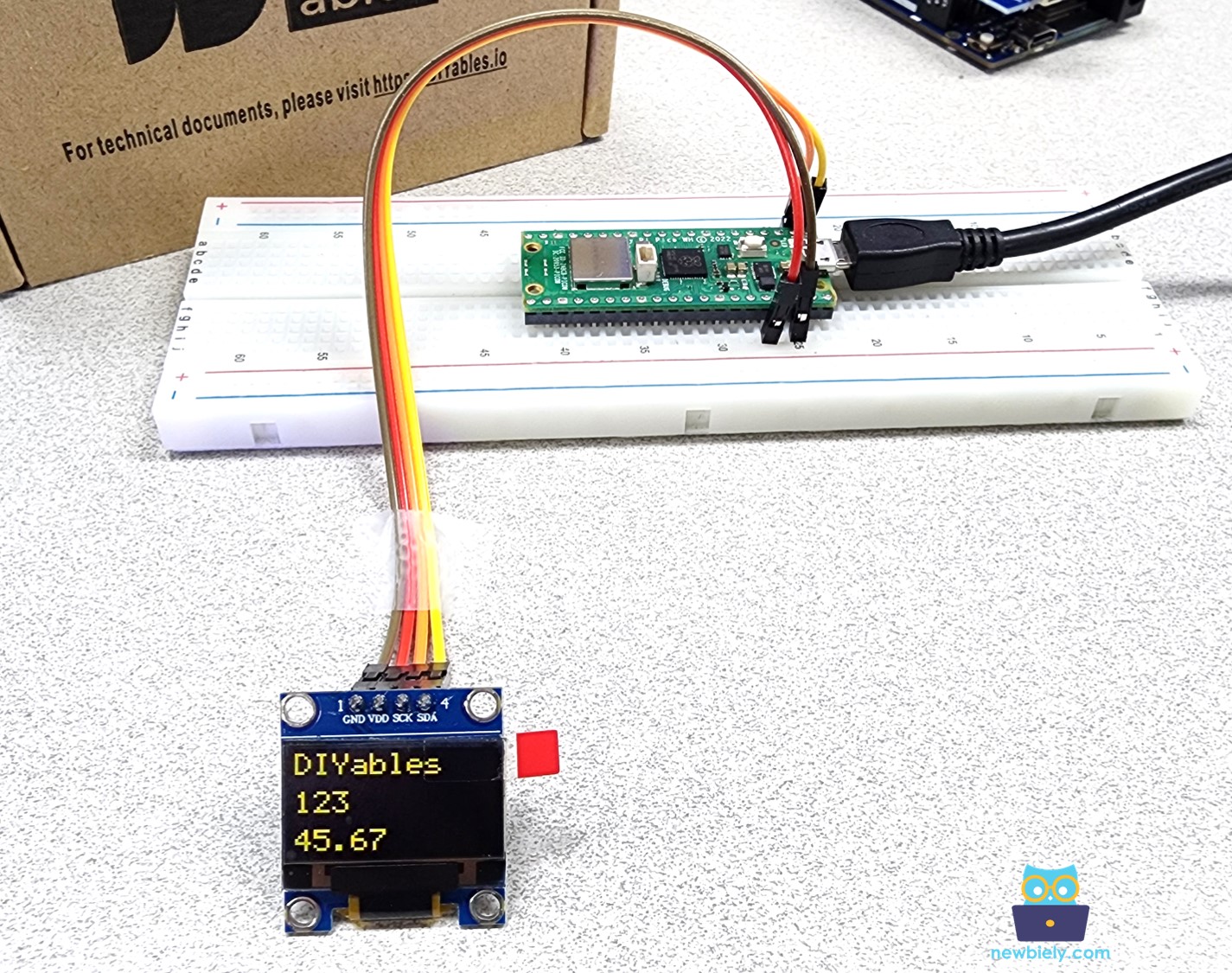
How to automatically vertical and horizontal center align text/number on OLED
The MicroPython code below automatically centers the text both vertically and horizontally on the OLED display.
After running the code, the text will be centered both vertically and horizontally on the OLED screen.
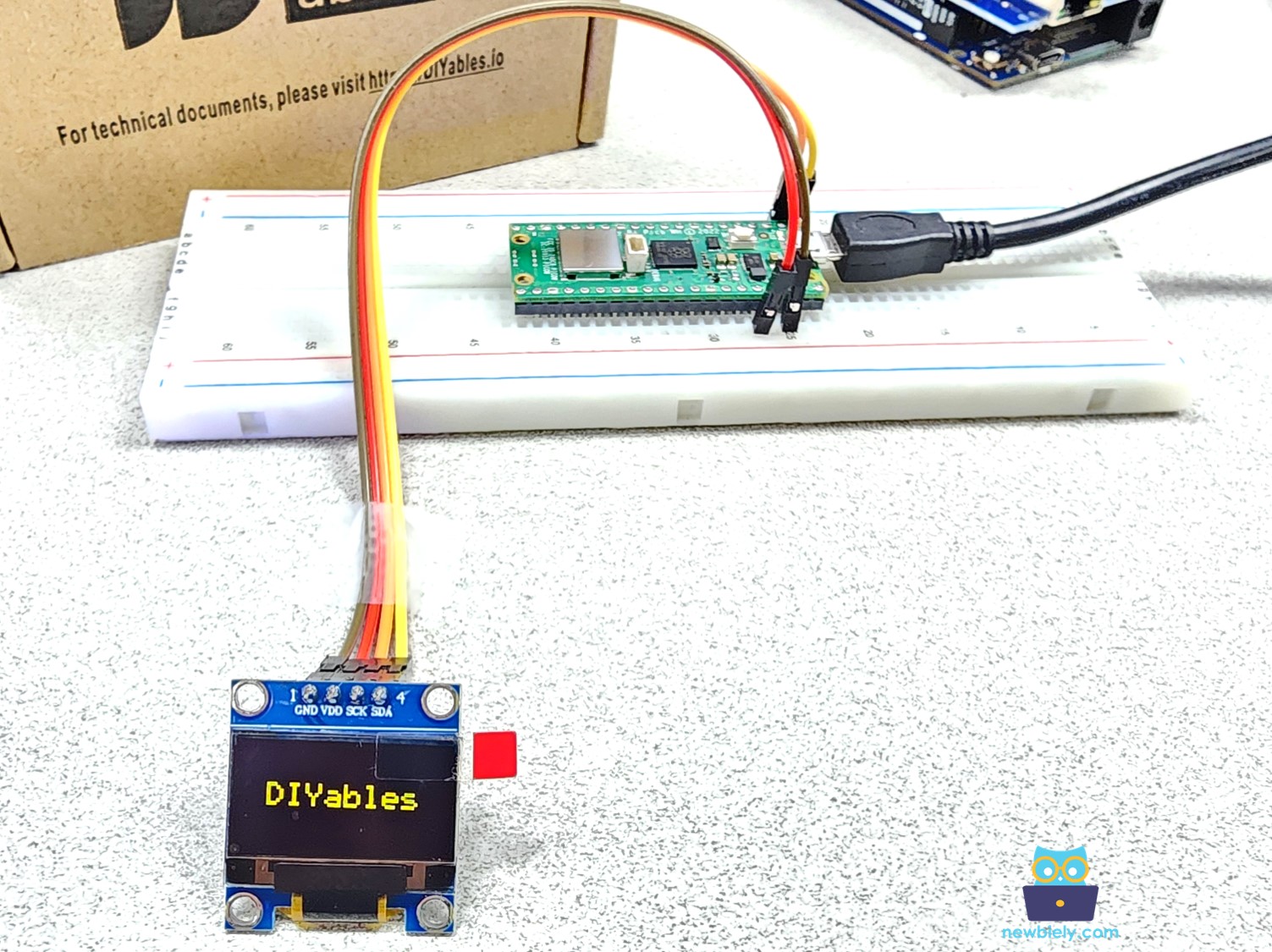
Raspberry Pi Pico Code - Drawing on OLED
By running the above code, you'll see a rectangle, circle, and triangle displayed on the OLED as shown below.
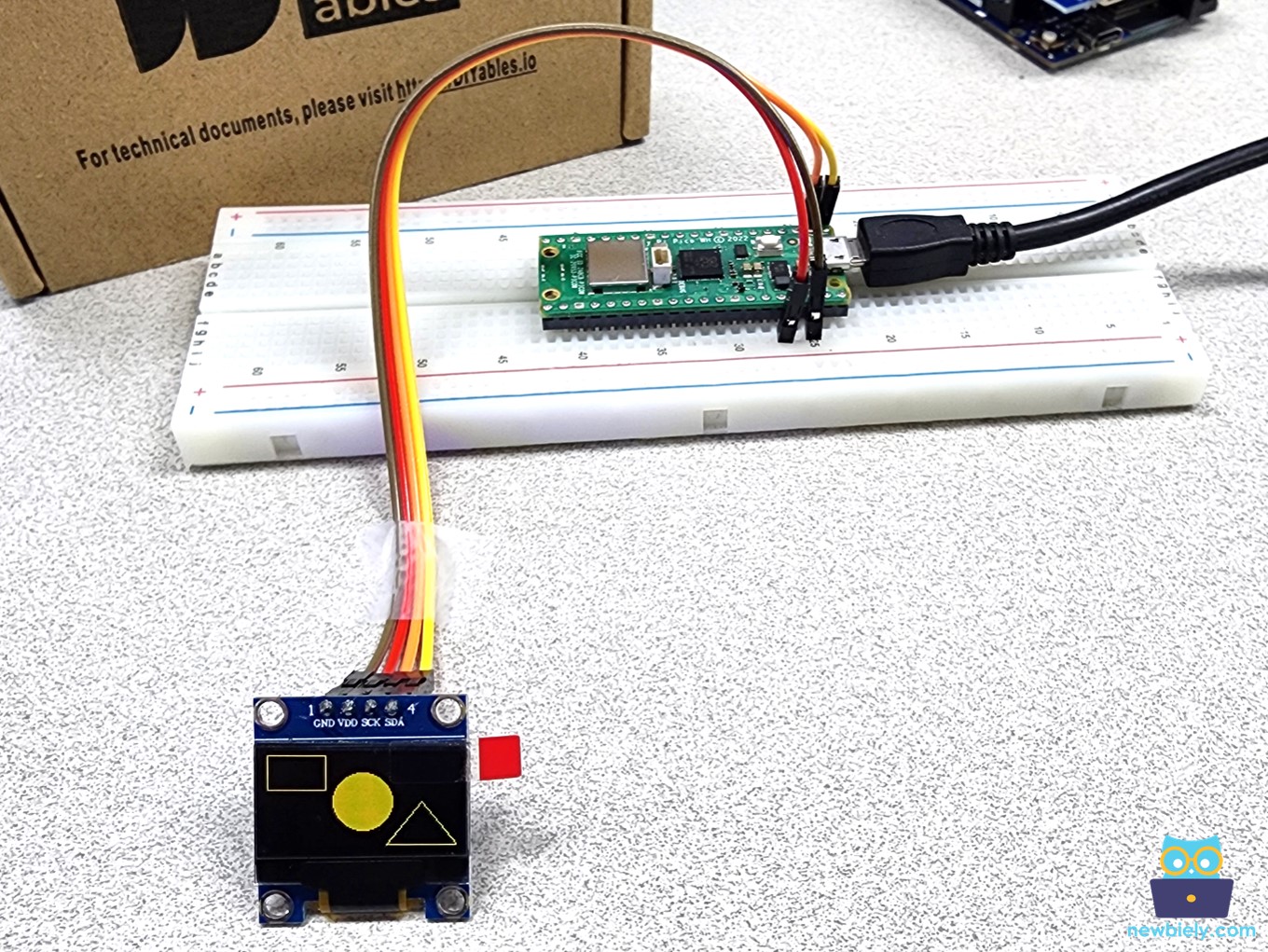
Raspberry Pi Pico Code – Display Image on OLED
The below code draws an image to LCD display. The image is DIYables icon.
By running the above code, you will see the image displayed on the OLED as below
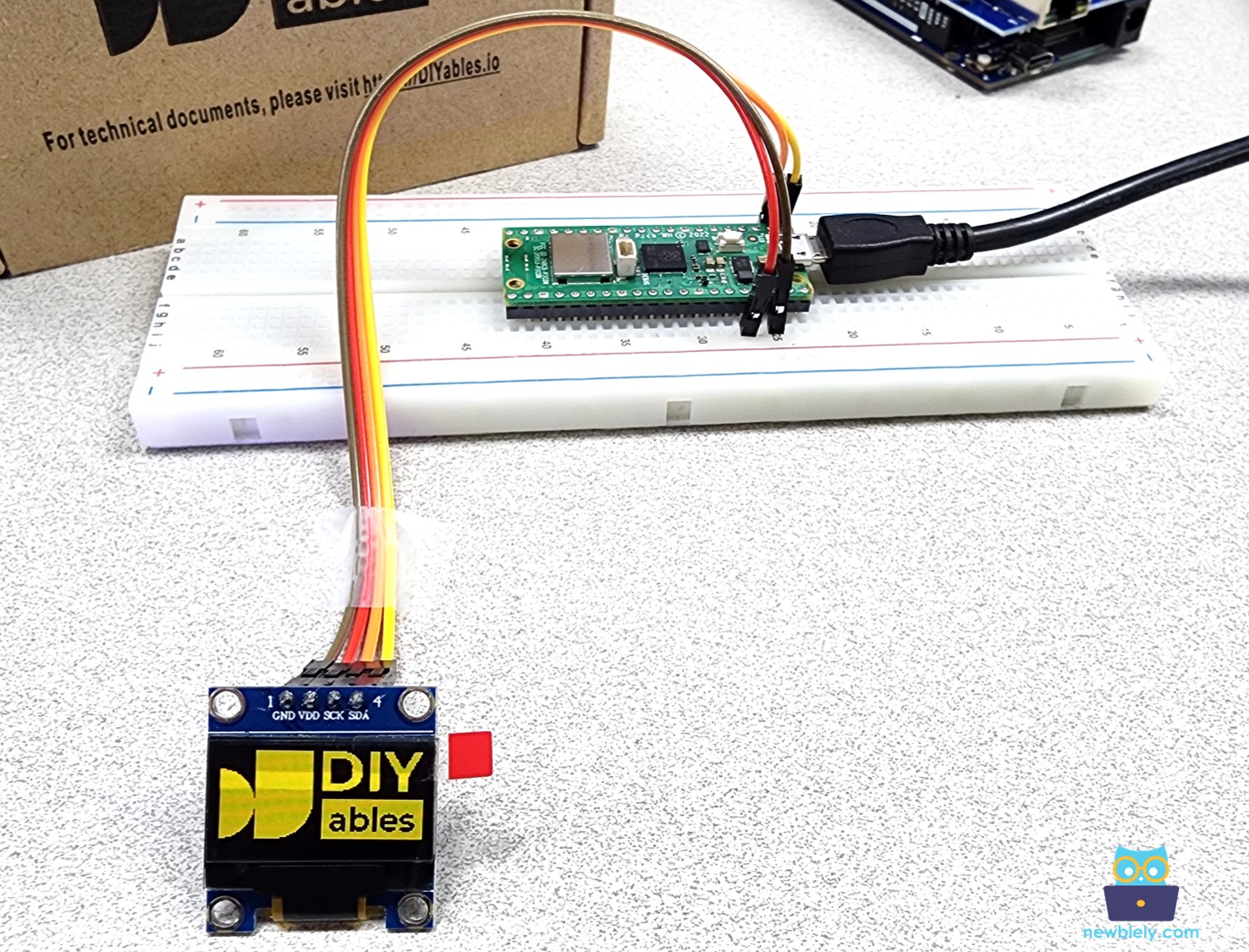
To show any other image on an OLED screen, you can follow the below steps:
- Change the image (in any format) into a bitmap array. You can use this online tool for conversion. See the picture below to learn how to convert an image into a bitmap array. I converted the Raspberry Pi Pico icon into a bitmap array.
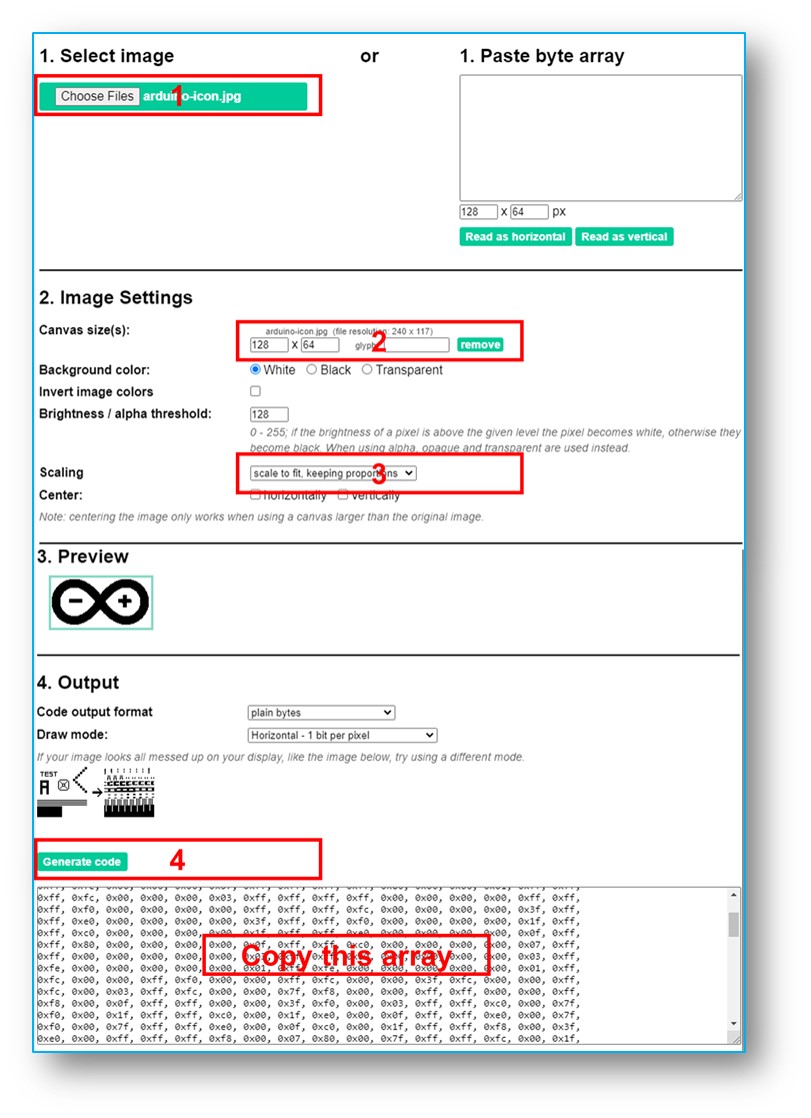
- Update the converted bitmap array into the Raspberry Pi Pico code with the new array code.
- Change the image width and height in the Raspberry Pi Pico code to match the dimension of image.
Please note that the image size must be equal to or smaller than the screen size.
OLED Troubleshooting
If nothing appears on the OLED screen, please follow these steps:
- Check that the wiring is correct.
- Make sure your I2c OLED has an SSD1306 driver.
- Find out the I2C address of your OLED by using this I2C Address Scanner code on Raspberry Pi Pico.
Output in the Shell at the bottom of Thonny: