Raspberry Pi Pico - Joystick
In this guide, we will learn how to use a joystick with Raspberry Pi Pico. In detail, we will find out:
- How a joystick work
- How to connect a joystick to a Raspberry Pi Pico
- How to program Raspberry Pi Pico to read values from Joystick
- How to program Raspberry Pi Pico to translate joystick's data into useful data (like XY coordinates or motor directions)
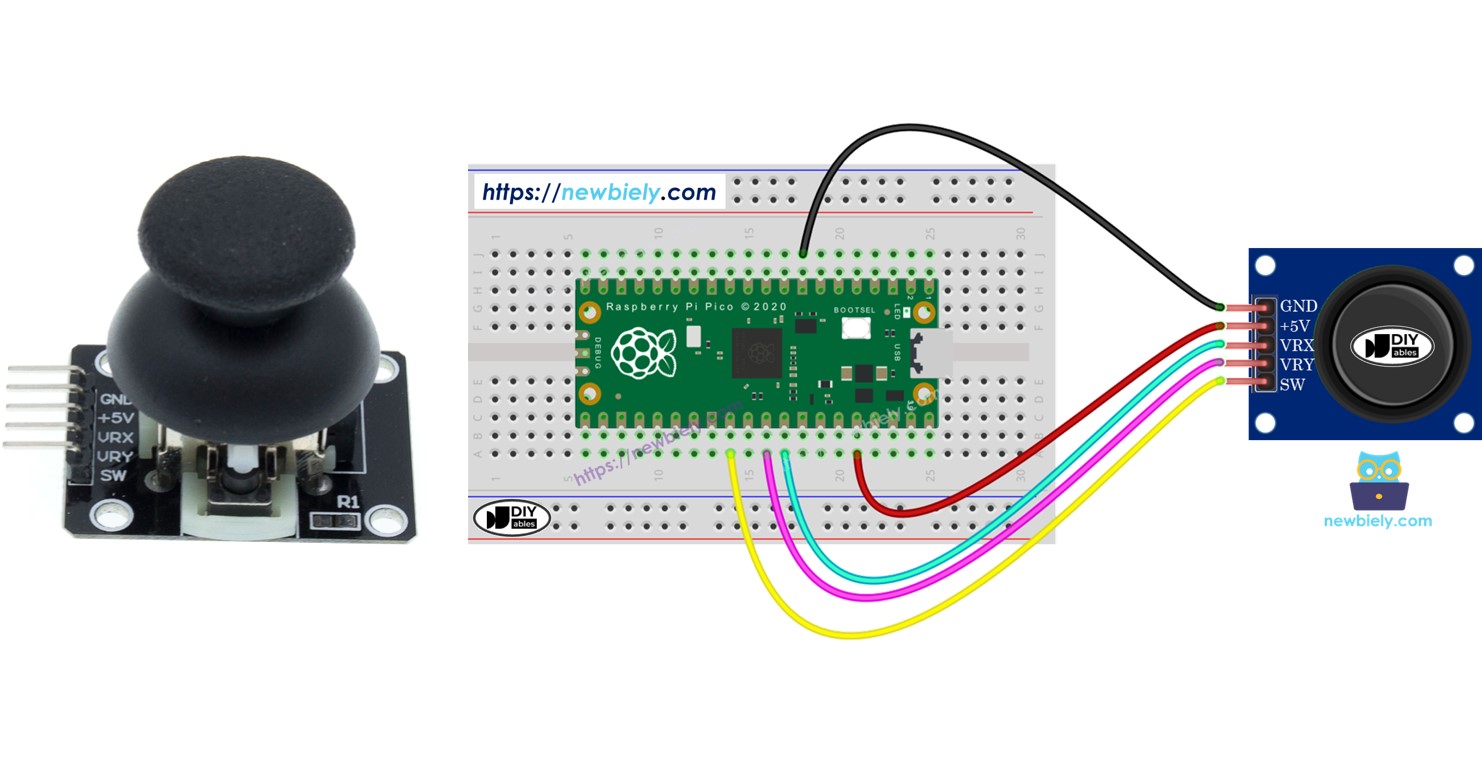
Hardware Preparation
1 | × | Raspberry Pi Pico W | |
1 | × | Raspberry Pi Pico Alternatively, | |
1 | × | Micro USB Cable | |
1 | × | Joystick | |
1 | × | Jumper Wires | |
1 | × | Breadboard | |
1 | × | Recommended: Screw Terminal Expansion Board for Raspberry Pi Pico |
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Joystick
You may have seen a joystick used for controlling games, toys, or even big machines like excavators.
The joystick has two potentiometers set in a square pattern and one button. It provides these outputs:
- A range from 0 to 1023 for the horizontal (left-right) position
- A range from 0 to 1023 for the vertical (up-down) position
- The button's status, either ON (HIGH) or OFF (LOW)
When you mix two analog values, they create 2-D coordinates. These coordinates are at the center when the joystick is still. You can discover the real direction of the coordinates by using a test code, which we will discuss in the next section.
Some applications may use all three outputs, while others may use only a few.
Pinout
A joystick has five pins.
- GND pin: connect to GND (0V)
- VCC pin: connect to VCC (3.3V) of Raspberry Pico
- VRX pin: provides an analog value for horizontal position (X-coordinate), connect this pin to an ADC pin of Raspberry Pico.
- VRY pin: provides an analog value for vertical position (Y-coordinate), connect this pin to an ADC pin of Raspberry Pico.
- SW pin: connected to the joystick's pushbutton. Generally open. Use a pull-up resistor to keep this pin HIGH when button is not pressed and LOW when pressed.
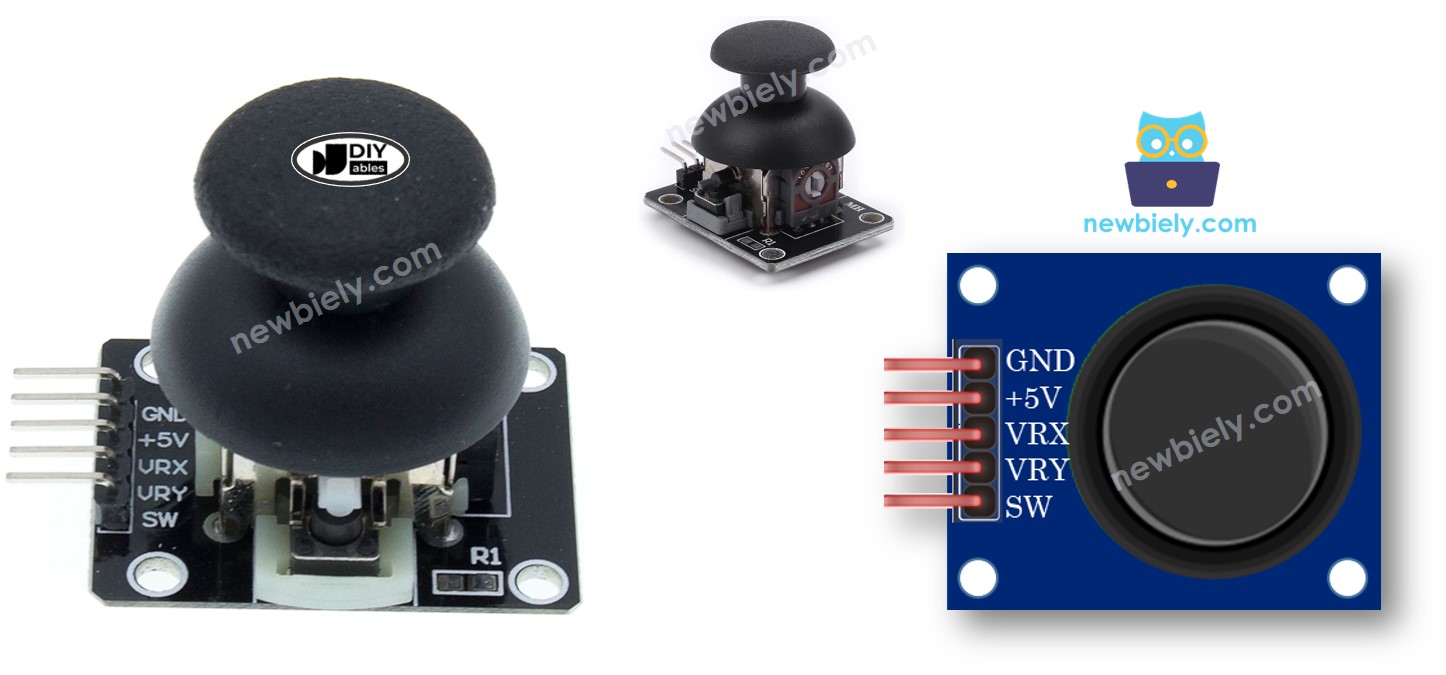
How It Works
- Left/Right Movement:
- Moving the joystick left or right changes the electrical signal (voltage) at the VRX pin.
- Moving it to the left reduces the voltage to 0 volts.
- Moving it to the right raises the voltage to 3.3 volts.
- The Raspberry Pi Pico measures this voltage and turns it into a number from 0 to 1023. Therefore, 0V is 0, and 5V is 1023.
- Up/Down Movement:
- Moving the joystick up or down alters the voltage at the VRY pin.
- Shifting it up decreases the voltage to 0 volts.
- Shifting it down increases the voltage to 3.3 volts.
- The Raspberry Pi Pico also reads this voltage, changing it into a number from 0 to 1023, similarly to VRX pin.
- Combined Movements:
- Moving the joystick in any direction changes the voltage at both VRX and VRY pins depending on its position along each axis.
- Pressing the Joystick:
- Pushing the joystick down activates an internal button.
- Connecting a pull-up resistor to the SW pin will cause the voltage at this pin to drop from 5V to 0V when pressed.
- The Raspberry Pi Pico detects this as a digital signal, showing HIGH (5V) when not pressed and LOW (0V) when pressed.
When you move the joystick, it adjusts the voltages at VRX and VRY. The Raspberry Pi Pico reads these changes as numbers from 0 to 1023. Pressing the joystick alters the voltage at the SW pin, and the Raspberry Pi Pico detects this as either HIGH or LOW.
Wiring Diagram
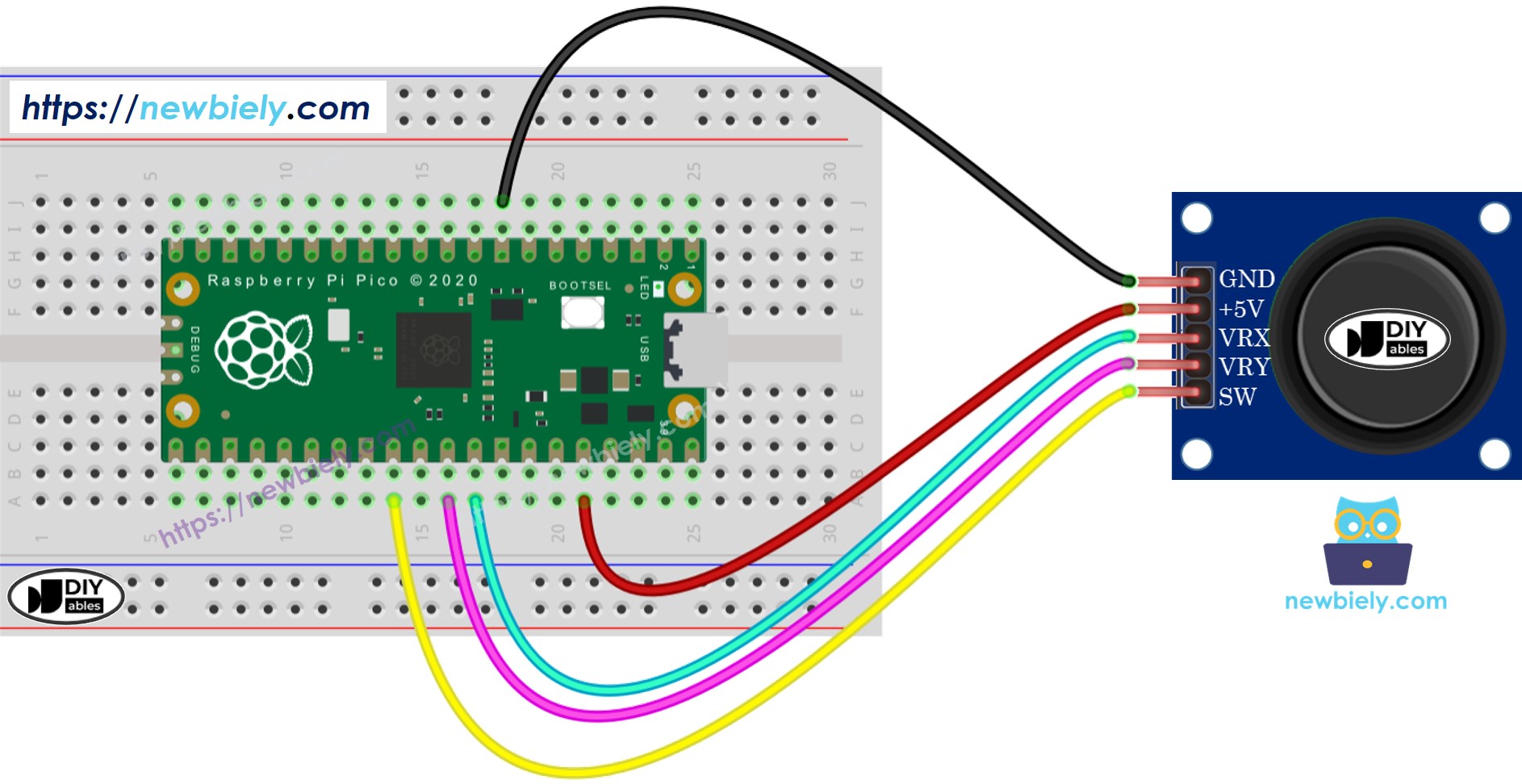
This image is created using Fritzing. Click to enlarge image
How To Program For Joystick
Fortunately, DIYables has developed a joystick library that greatly simplifies the process of using a joystick with the Raspberry Pi Pico.
Raspberry Pi Pico Code - Reads Joystick's state
The below MicroPython script does:
- Reads analog values from a joystick.
- Checks if the joystick's thump is presed or released
- Reads the joystick's thump press count
Detailed Instructions
Please follow these instructions step by step:
- Ensure that Thonny IDE is installed on your computer.
- Ensure that MicroPython firmware is installed on your Raspberry Pi Pico.
- If this is your first time using a Raspberry Pico, refer to the Raspberry Pi Pico - Getting Started tutorial for detailed instructions.
- Connect the joystick to the Raspberry Pi Pico according to the provided diagram.
- Connect the Raspberry Pi Pico to your computer using a USB cable.
- Launch the Thonny IDE on your computer.
- On Thonny IDE, select MicroPython (Raspberry Pi Pico) Interpreter by navigating to Tools Options.
- In the Interpreter tab, select MicroPython (Raspberry Pi Pico) from the drop-down menu.
- Ensure the correct port is selected. Thonny IDE should automatically detect the port, but you may need to select it manually (e.g., COM3 on Windows or /dev/ttyACM0 on Linux).
- Navigate to the Tools Manage packages on the Thonny IDE.
- Search “DIYables-MicroPython-Joystick”, then find the Joystick library created by DIYables.
- Click on DIYables-MicroPython-Joystick, then click Install button to install Joystick library.
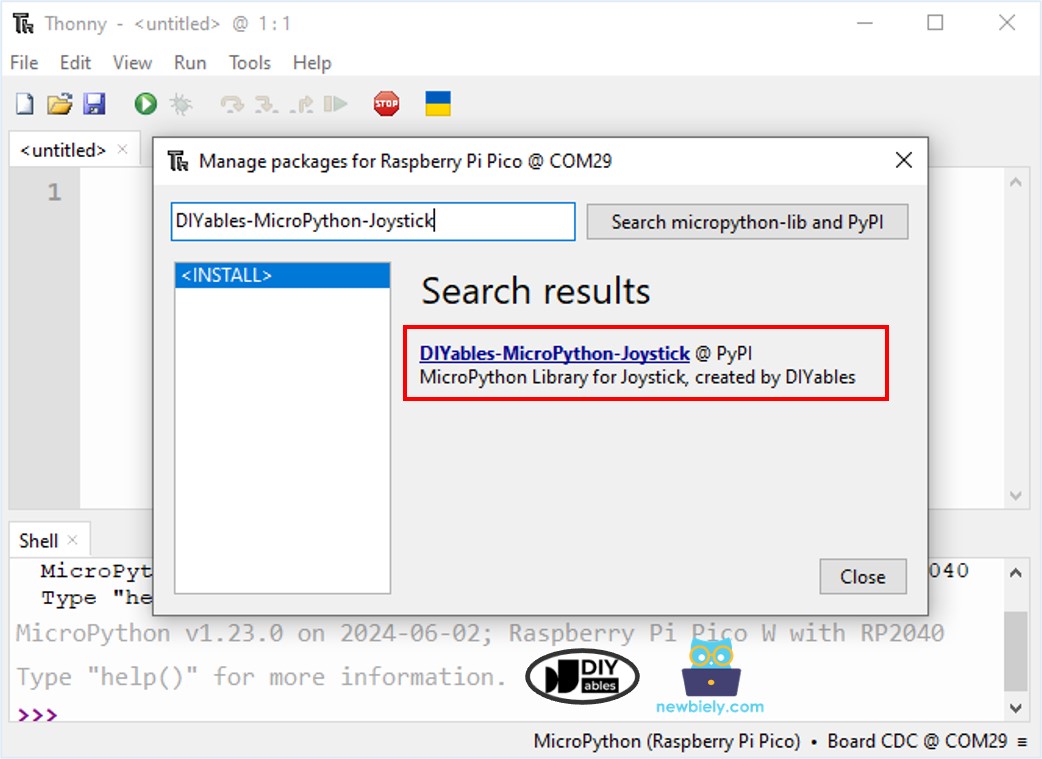
- Copy the above code and paste it to the Thonny IDE's editor.
- Save the script to your Raspberry Pi Pico by:
- Click the Save button, or use Ctrl+S keys.
- In the save dialog, you will see two sections: This computer and Raspberry Pi Pico. Select Raspberry Pi Pico
- Save the file as main.py
- Click the green Run button (or press F5) to run the script. The script will execute.
- Slide the joystick left, right, up, or down.
- Push down on the top of the joystick.
- Check out the message in the Shell at the bottom of Thonny.
- While using the joystick, check out the in the Shell at the bottom of Thonny. If the X value is 0, remember that this means left; otherwise, it means right. If the Y value is 0, this position is up; otherwise, it is down.
Note that while the theoretical range for the X/Y values is from 0 to 4095, in practice, the values typically do not reach these extremes due to the mechanical characteristics of the joystick. This variation is normal and acceptable for typical applications.
If you name your script main.py and save it to the root directory of the Raspberry Pi Pico, it will automatically run each time the Pico is powered on or reset. This is useful for standalone applications that need to start running immediately upon power-up. If you name your script another name other than main.py, you will need to manually run it from Thonnys's Shell.
Converts analog value to MOVE LEFT/RIGHT/UP/DOWN commands
The below MicroPython script converts analog values into commands: MOVE_LEFT, MOVE_RIGHT, MOVE_UP, MOVE_DOWN.
Detailed Instructions
- Copy the above code and open it in Thonny IDE.
- Save the script to your Raspberry Pi Pico.
- Move the joystick left, right, up, down, or in any direction.
- Check out the message in the Shell at the bottom of Thonny.
※ NOTE THAT:
There might be no command, one command, or even two commands at the same time, like UP and LEFT together.
Converts analog values to angles to control two servo motors
Learn detail in this Raspberry Pi Pico - Joystick Controls Servo Motor tutorial.