Raspberry Pi Pico - Keypad 3x4
This guide shows you how to use a Raspberry Pi Pico with a 3x4 keypad. We will cover:
- Connecting a 3x4 keypad to a Raspberry Pi Pico.
- Programming a Raspberry Pi Pico to detect which key is pressed on a 3x4 keypad.
- Verifying the password entered using a keypad.
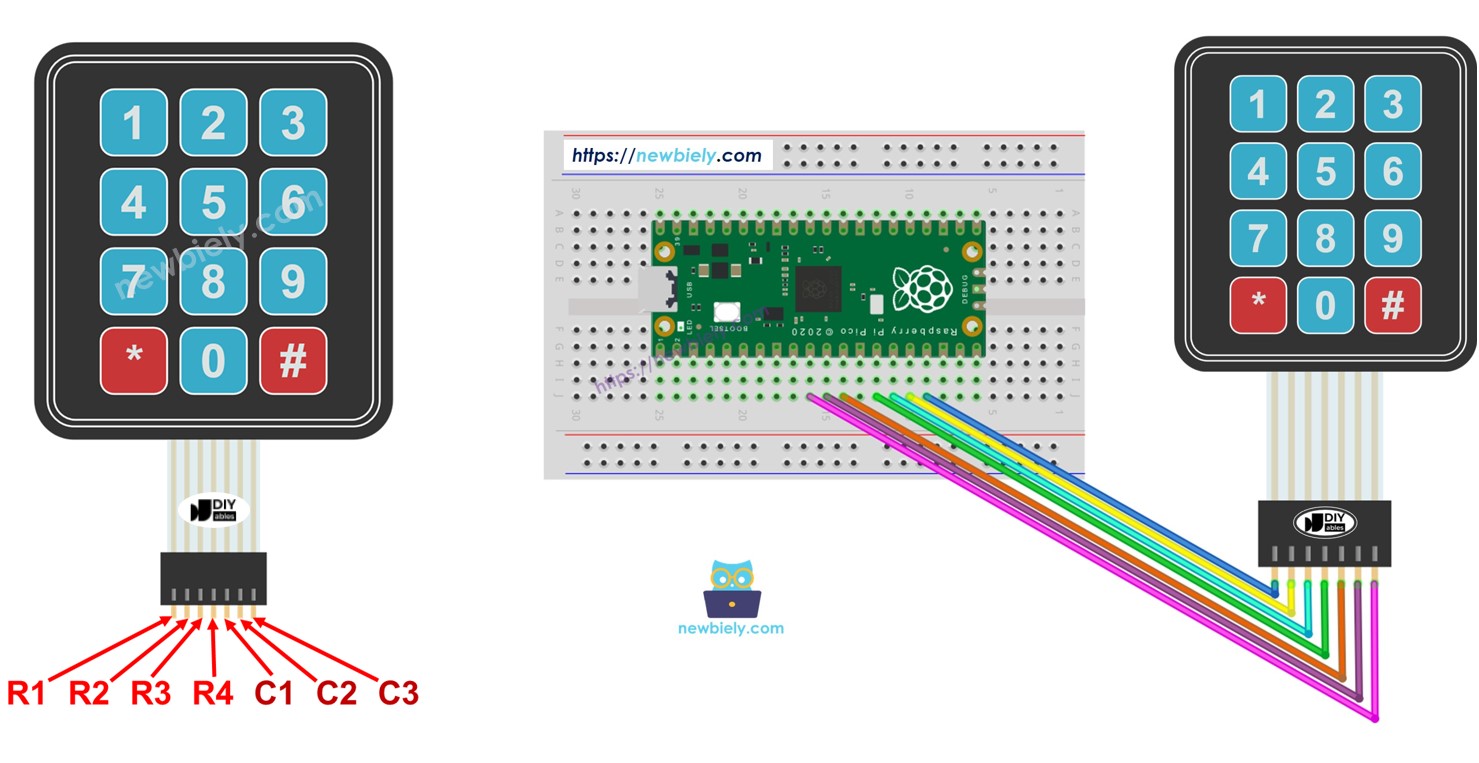
Hardware Preparation
1 | × | Raspberry Pi Pico W | |
1 | × | Raspberry Pi Pico (Alternatively) | |
1 | × | Micro USB Cable | |
1 | × | Keypad 3x4 | |
1 | × | Jumper Wires | |
1 | × | (Optional) Screw Terminal Expansion Board for Raspberry Pi Pico |
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables.
Overview of 3x4 Keypad
The keypad contains 12 soft buttons set up in rows and columns, making a grid. Each button is referred to as a key.
Pinout
A 3x4 keypad has 7 pins. These pins are split into two types: rows and columns.
- 4 pins connect to the rows (R1, R2, R3, R4).
- 3 pins connect to the columns (C1, C2, C3).
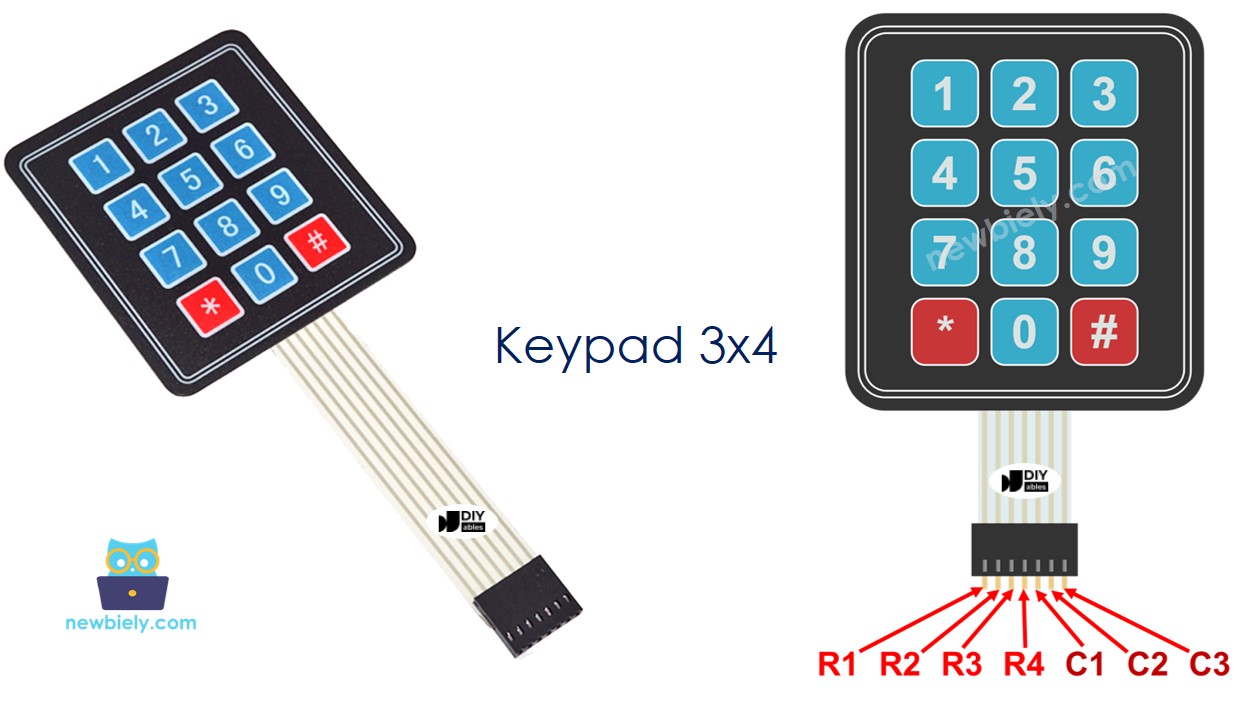
Wiring Diagram
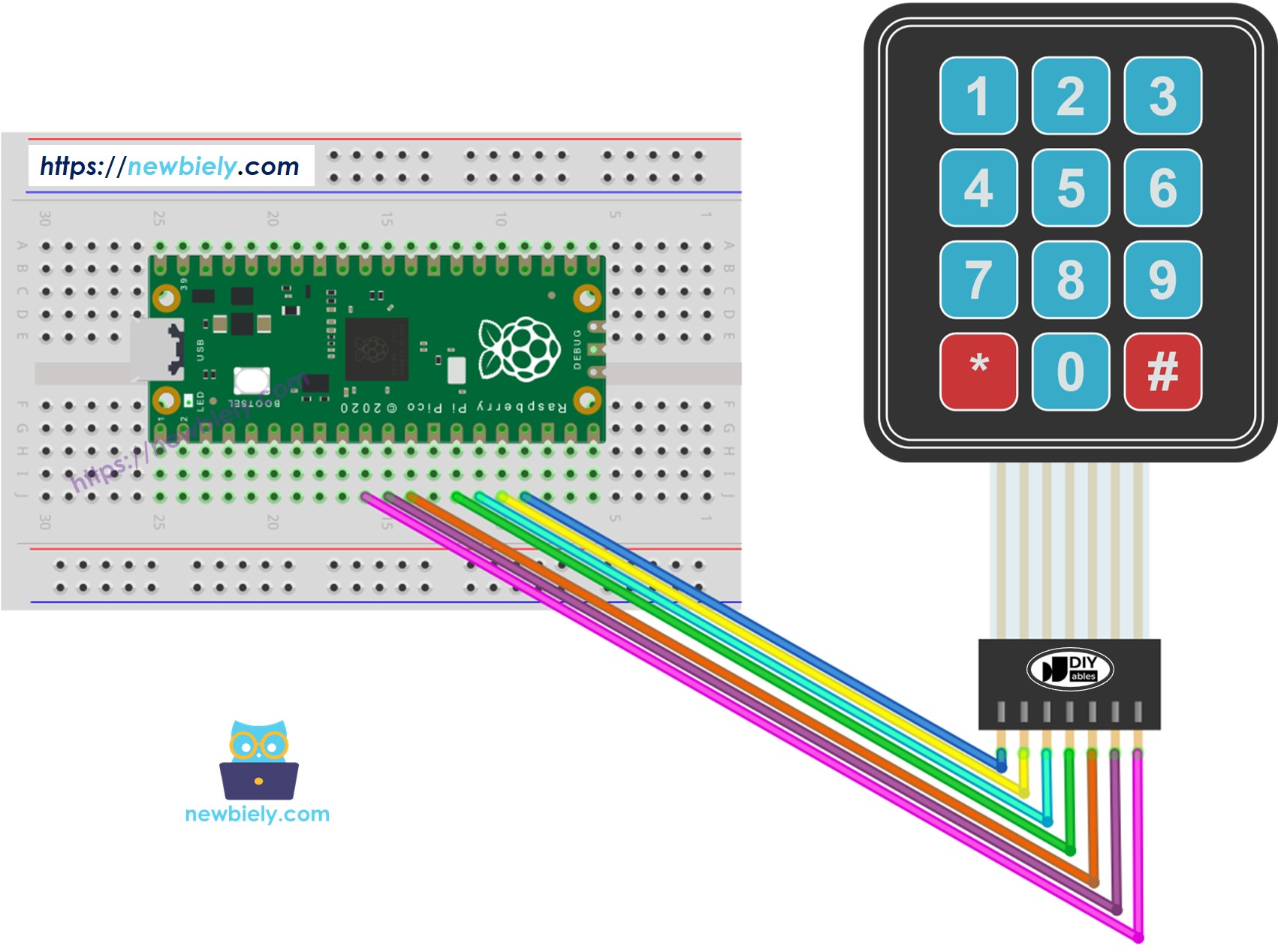
This image is created using Fritzing. Click to enlarge image
Raspberry Pi Pico Code
Detailed Instructions
Please follow these instructions step by step:
- Ensure that Thonny IDE is installed on your computer.
- Ensure that MicroPython firmware is installed on your Raspberry Pi Pico.
- If this is your first time using a Raspberry Pico, refer to the Raspberry Pi Pico - Getting Started tutorial for detailed instructions.
- Connect your Raspberry Pi Pico to the 3x4 keypad as shown in the diagram.
- Connect the Raspberry Pi Pico to your computer using a USB cable.
- Launch the Thonny IDE on your computer.
- On Thonny IDE, select MicroPython (Raspberry Pi Pico) Interpreter by navigating to Tools Options.
- In the Interpreter tab, select MicroPython (Raspberry Pi Pico) from the drop-down menu.
- Ensure the correct port is selected. Thonny IDE should automatically detect the port, but you may need to select it manually (e.g., COM3 on Windows or /dev/ttyACM0 on Linux).
- Navigate to the Tools Manage packages on the Thonny IDE.
- Search “DIYables-MicroPython-Keypad”, then find the Keypad library created by DIYables.
- Click on DIYables-MicroPython-Keypad, then click Install button to install Keypad library.
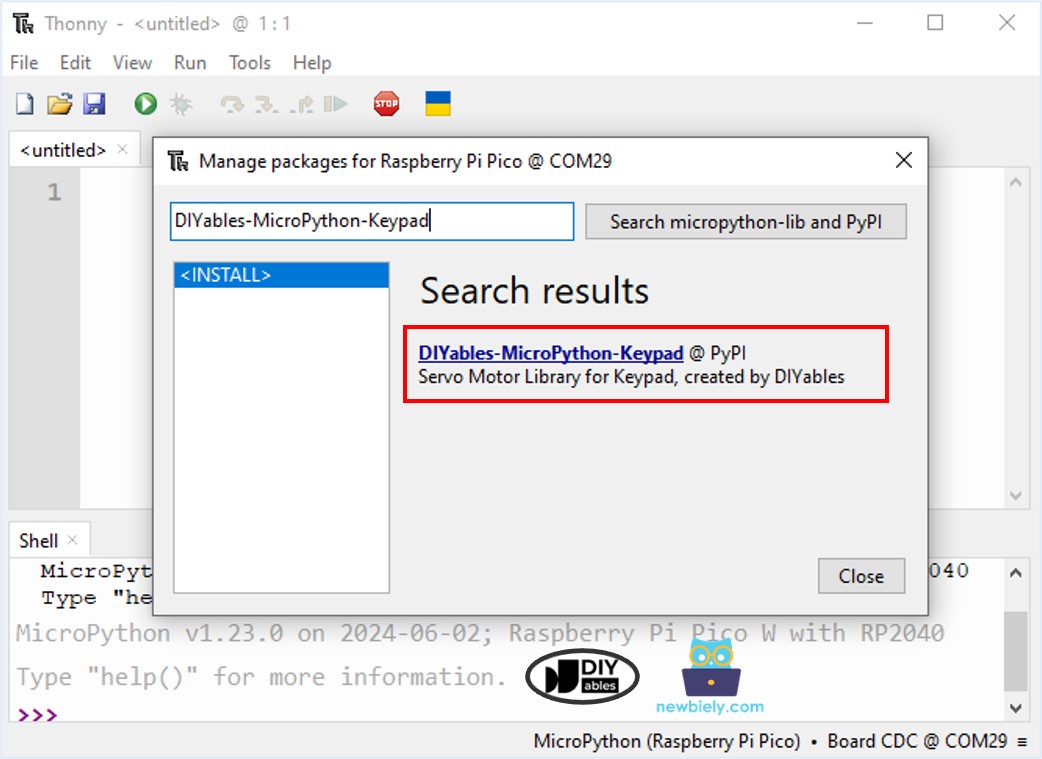
- Copy the above code and paste it to the Thonny IDE's editor.
- Save the script to your Raspberry Pi Pico by:
- Click the Save button, or use Ctrl+S keys.
- In the save dialog, you will see two sections: This computer and Raspberry Pi Pico. Select Raspberry Pi Pico
- Save the file as main.py
- Click the green Run button (or press F5) to run the script. The script will execute.
- Press a few keys on your keypad.
- Check out the message in the Shell at the bottom of Thonny.
If it does not work, please check your wiring carefully. It is very easy to confise when connecting Raspberry Pico to the keypad 3x4.
If you name your script main.py and save it to the root directory of the Raspberry Pi Pico, it will automatically run each time the Pico is powered on or reset. This is useful for standalone applications that need to start running immediately upon power-up. If you name your script another name other than main.py, you will need to manually run it from Thonnys's Shell.
Keypad and Password
A keypad is often used to type in a password. Here, we focus on two important keys:
- A key to start or restart typing the password. For example, use the key "*."
- A key to finish typing the customer. For instance, use the key "#."
The password will consist of the other keys, except for two special keys that are not included.
When you press a key.
- If the key is neither "*" nor "#", include it in the password being typed.
- If the key is "#", verify if the typed password is correct, then clear it.
- If the key is "*", clear the password.
Keypad - Password Code
- Copy the above code and paste it to the Thonny IDE's editor.
- Save the script to your Raspberry Pi Pico
- Type in "123", follow with pressing “#.”
- Type in "1234", then press "#."
- Check out the message in the Shell at the bottom of Thonny.