Raspberry Pi Pico - Button - Debounce
When you program the Raspberry Pi Pico to notice when a button is pressed, you might see that one press is read many times. This is because the button can flicker quickly between ON and OFF due to its design. This flickering is known as "chattering." Chattering can make it look like the button was pressed multiple times, which might cause mistakes in some programs. This guide shows how to solve this problem, a method called debouncing the button.
We will explore five different examples below and explain the differences between them:
Raspberry Pi Pico without debouncing a button.
Raspberry Pi Pico uses the utime.sleep_ms() function to debounce a button.
Raspberry Pi Pico uses the non-blocking method to debounce a button.
Raspberry Pi Pico uses the DIYables-MicroPython-Button library to debounce a button.
Raspberry Pi Pico uses the DIYables-MicroPython-Button library to debounce three buttons.
Or you can buy the following sensor kits:
Disclosure: Some of the links provided in this section are Amazon affiliate links. We may receive a commission for any purchases made through these links at no additional cost to you.
Additionally, some of these links are for products from our own brand,
DIYables .
Explore how buttons work, including their layout, usage, and how to program them, by checking out these guides.
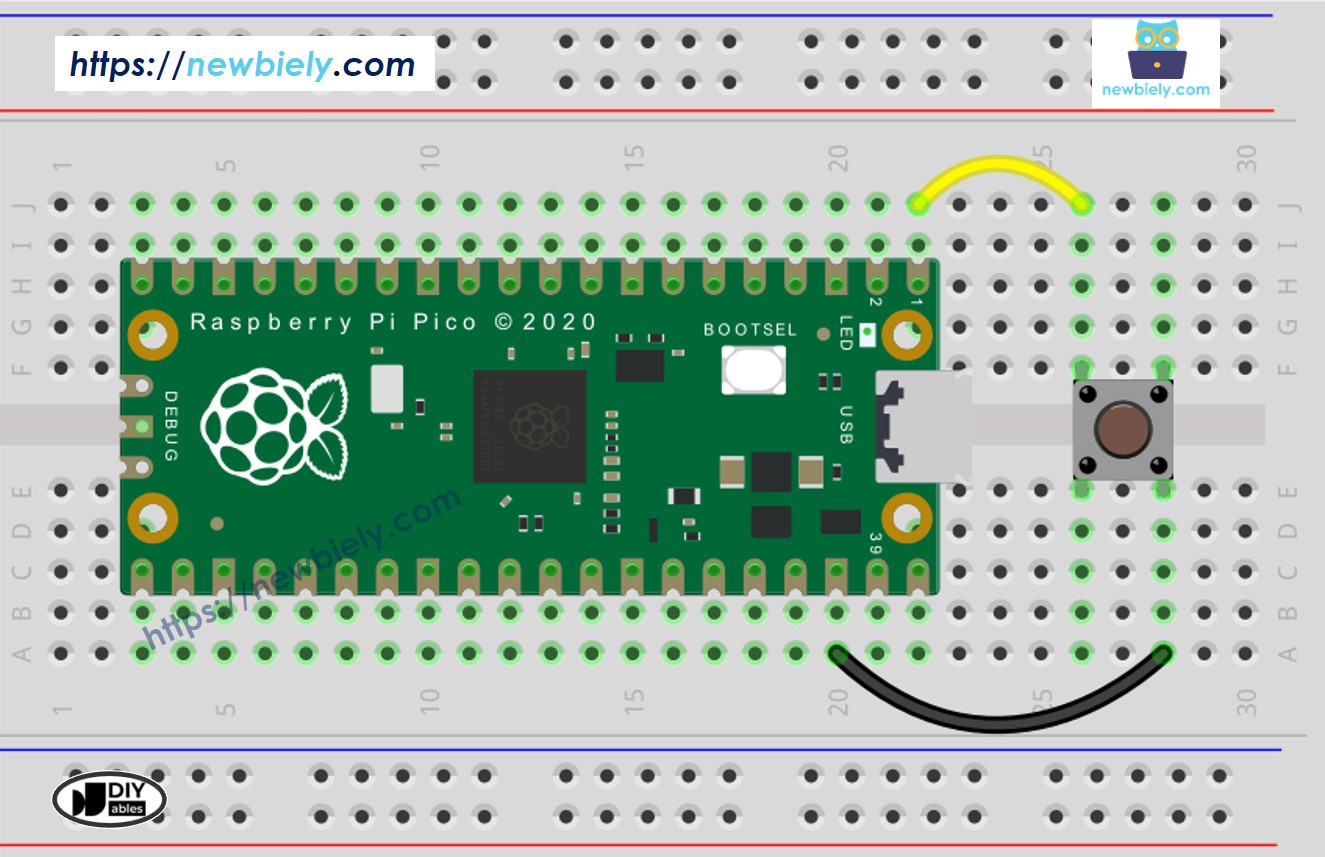
This image is created using Fritzing. Click to enlarge image
Let's look at and compare the Raspberry Pi Pico code without and with debounce, and see how they behave.
Let's first examine the code without debouncing to understand how it works.
from machine import Pin
import time
BUTTON_PIN = 0
button = Pin(BUTTON_PIN, Pin.IN, Pin.PULL_UP)
prev_button_state = 1
while True:
button_state = button.value()
if prev_button_state == 0 and button_state == 1:
print("The button is released")
if prev_button_state == 1 and button_state == 0:
print("The button is pressed")
prev_button_state = button_state
Please follow these instructions step by step:
Ensure that Thonny IDE is installed on your computer.
Ensure that MicroPython firmware is installed on your Raspberry Pi Pico.
Connect the button to the Raspberry Pi Pico according to the provided diagram.
Connect the Raspberry Pi Pico to your computer using a USB cable.
Launch the Thonny IDE on your computer.
On Thonny IDE, select MicroPython (Raspberry Pi Pico) Interpreter by navigating to Tools Options.
In the Interpreter tab, select MicroPython (Raspberry Pi Pico) from the drop-down menu.
Ensure the correct port is selected. Thonny IDE should automatically detect the port, but you may need to select it manually (e.g., COM3 on Windows or /dev/ttyACM0 on Linux).
Copy the above code and paste it to the Thonny IDE's editor.
Save the script to your Raspberry Pi Pico by:
Click the Save button, or use Ctrl+S keys.
In the save dialog, you will see two sections: This computer and Raspberry Pi Pico. Select Raspberry Pi Pico
Save the file as main.py
Click the green Run button (or press F5) to run the script. The script will execute.
Hold the button down for a few seconds and then let go.
Check out the message in the Shell at the bottom of Thonny.
>>> %Run -c $EDITOR_CONTENT
MPY: soft reboot
The button is pressed
The button is pressed
The button is pressed
The button is released
The button is released
MicroPython (Raspberry Pi Pico) • Board CDC @ COM29 ≡
You pressed and released the button just one time, but the Raspberry Pi Pico thinks it was done many times.
from machine import Pin
import utime
BUTTON_PIN = 0
DEBOUNCE_TIME = 100
button = Pin(BUTTON_PIN, Pin.IN, Pin.PULL_UP)
prev_button_state = 1
while True:
button_state = button.value()
if prev_button_state == 0 and button_state == 1:
print("The button is released")
utime.sleep_ms(DEBOUNCE_TIME)
if prev_button_state == 1 and button_state == 0:
print("The button is pressed")
utime.sleep_ms(DEBOUNCE_TIME)
prev_button_state = button_state
Copy the above MicroPython code and paste it into Thonny's editor.
Save the code to your Raspberry Pi Pico.
Click the green Run button (or press F5) to execute the script.
Press the button for a few seconds and then release it.
Check out the output in the Shell at the bottom of Thonny.
>>> %Run -c $EDITOR_CONTENT
MPY: soft reboot
The button is pressed
The button is released
MicroPython (Raspberry Pi Pico) • Board CDC @ COM29 ≡
You clicked the button one time, and the Raspberry Pi Pico recognized it accurately as one press and release, without any extra noise.
※ NOTE THAT:
Different applications use different DEBOUNCE_TIME values. Each application may have a specific value.
The above code uses utime.sleep_ms() to debounce the button. This function blocks Raspberry Pico from doing other tasks during the sleep time. Next, we'll look at another example that shows how to debounce without stopping other tasks.
from machine import Pin
import time
BUTTON_PIN = 0
DEBOUNCE_TIME = 100
button = Pin(BUTTON_PIN, Pin.IN, Pin.PULL_UP)
last_steady_state = button.value()
last_flickerable_state = button.value()
last_debounce_time = time.ticks_ms()
while True:
current_state = button.value()
current_time = time.ticks_ms()
if current_state != last_flickerable_state:
last_debounce_time = current_time
last_flickerable_state = current_state
if time.ticks_diff(current_time, last_debounce_time) > DEBOUNCE_TIME:
if current_state != last_steady_state:
if last_steady_state == 1 and current_state == 0:
print("The button is pressed")
elif last_steady_state == 0 and current_state == 1:
print("The button is released")
last_steady_state = current_state
time.sleep(0.01)
Debouncing code can seem complex, especially when using multiple buttons. Luckily, there's an easier method for beginners who need to manage several buttons. This method uses a library called DIYables_MicroPython_Button. You can learn more about the DIYables_MicroPython_Button library here.
Let's see some example codes.
from DIYables_MicroPython_Button import Button
import time
button = Button(0)
button.set_debounce_time(100)
while True:
button.loop()
if button.is_pressed():
print("The button is pressed")
if button.is_released():
print("The button is released")
On Thonny IDE, navigate to the Tools Manage packages on the Thonny IDE.
Search “DIYables-MicroPython-Button”, then find the Button library created by DIYables.
Click on DIYables-MicroPython-Button, then click Install button to install Button library.
Copy the above code and paste it to the Thonny IDE's editor.
Save the script to your Raspberry Pi Pico.
Click the green Run button (or press F5) to run the script. The script will execute.
Press the button.
Check out the message in the Shell at the bottom of Thonny.
Let's debounce for 3 buttons. Here is the wiring diagram between Raspberry Pi Pico and three buttons:
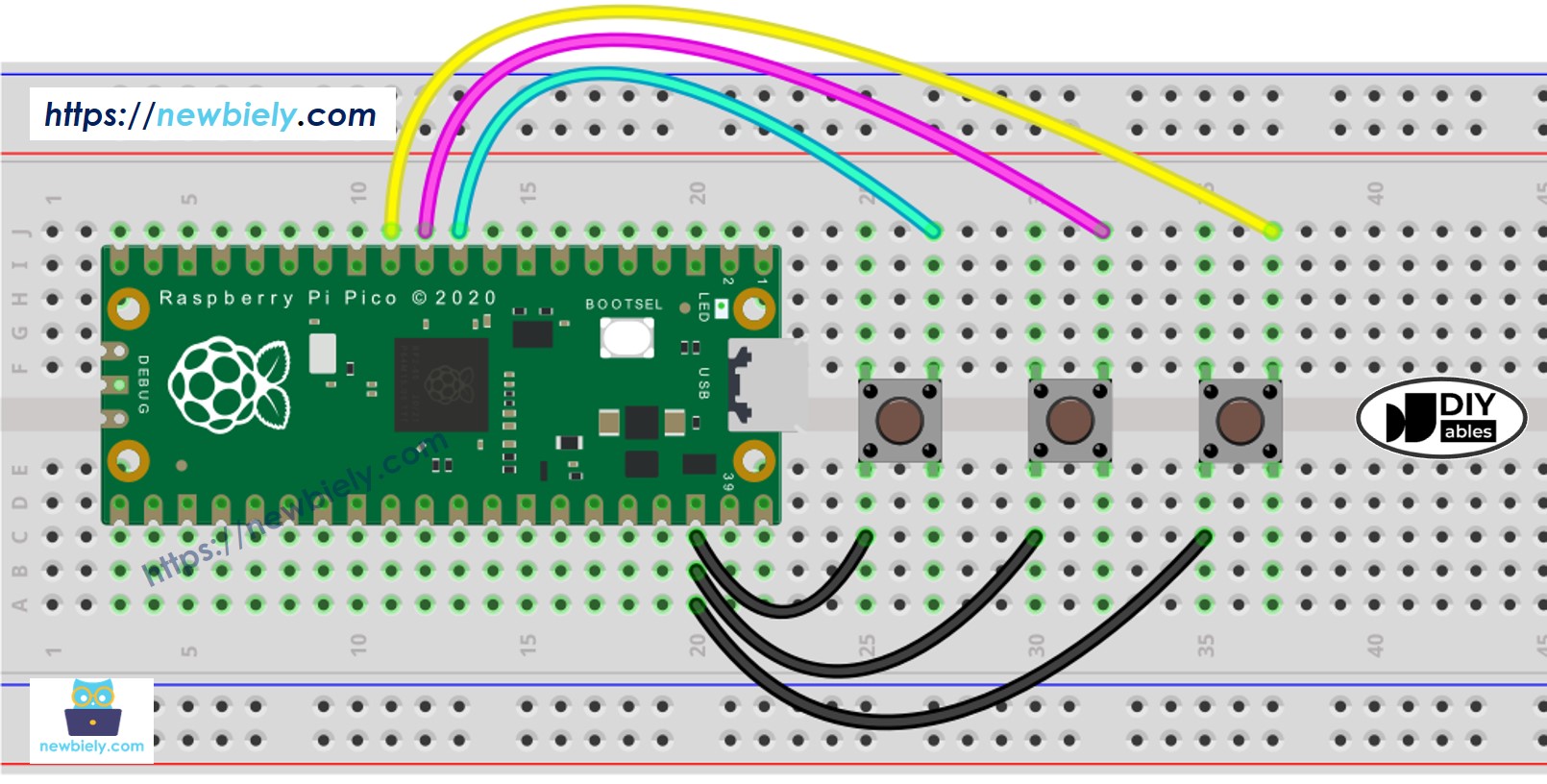
This image is created using Fritzing. Click to enlarge image
from DIYables_MicroPython_Button import Button
import time
button_1 = Button(7)
button_2 = Button(8)
button_3 = Button(9)
button_1.set_debounce_time(100)
button_2.set_debounce_time(100)
button_3.set_debounce_time(100)
while True:
button_1.loop()
button_2.loop()
button_3.loop()
if button_1.is_pressed():
print("The button 1 is pressed")
if button_1.is_released():
print("The button 1 is released")
if button_2.is_pressed():
print("The button 2 is pressed")
if button_2.is_released():
print("The button 2 is released")
if button_3.is_pressed():
print("The button 3 is pressed")
if button_3.is_released():
print("The button 3 is released")