Raspberry Pi Pico - Actuator with Feedback
In a previous tutorial, we learned about how to use Raspberry Pi Pico with a linear actuator without feedback. In this tutorial, we will learn how to use Raspberry Pi Pico with the linear actuator with feedback. The feedback signal from the actuator tells provides its position during movement, which helps us control where it goes. We will examine the following details:
- How a feedback linear actuator works.
- How to program Raspberry Pi Pico to read the position of a feedback linear actuator.
- How to program Raspberry Pi Pico to control the position of a feedback linear actuator.
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Feedback Linear Actuator
A feedback linear actuator is a device that moves in a straight line and includes a system to check and adjust its position. It uses a potentiometer that sends out a voltage signal relating to where the actuator is positioned.
Feedback Linear Actuator Pinout
A feedback linear actuator comes with five wires.
- Positive Wire: This wire uses a high voltage (12V, 24V, 48V) to control the linear actuator.
- Negative Wire: This wire uses a high voltage (12V, 24V, 48V) to control the linear actuator.
- 5V Wire: Connect this wire to the feedback potentiometer at 5V or 3.3V.
- GND Wire: Connect this wire to the feedback potentiometer at the ground (GND).
- Potentiometer Wire: Also called the feedback or output wire, this wire changes its voltage value according to the stroke position.
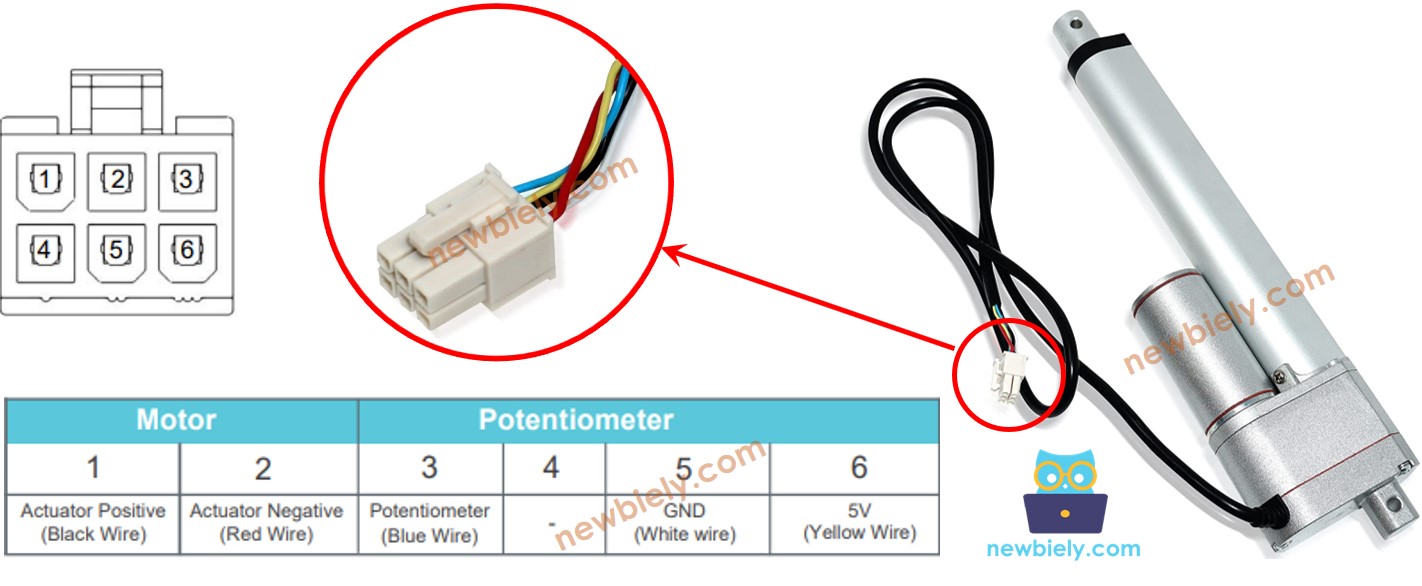
How It Works
When we apply a high voltage to the positive and negative wires, the actuator will either stretch out or pull back. To explain this, if we connect:
- Connect 12V (or 24V, 48V) to the positive wire and GND to the negative wire: the actuator will extend at its highest speed until it fully extends.
- Connect 12V (or 24V, 48V) to the negative wire and GND to the positive wire: the actuator will retract at its highest speed until it fully retracts.
- If the actuator's power is switched off (by connecting GND to both its wires) while it is extending or retracting, it will stop moving.
※ NOTE THAT:
- The voltage required to operate the actuator depends on its specific features. Look at the datasheet or manual to determine the correct voltage.
- The actuator can keep its position without electricity, even when supporting a weight.
The voltage in the potentiometer's wire changes when the actuator moves. By measuring this voltage, we can determine the position of the stroke.
Wiring Diagram
Remove all three jumpers from the L298N module before starting the wiring.
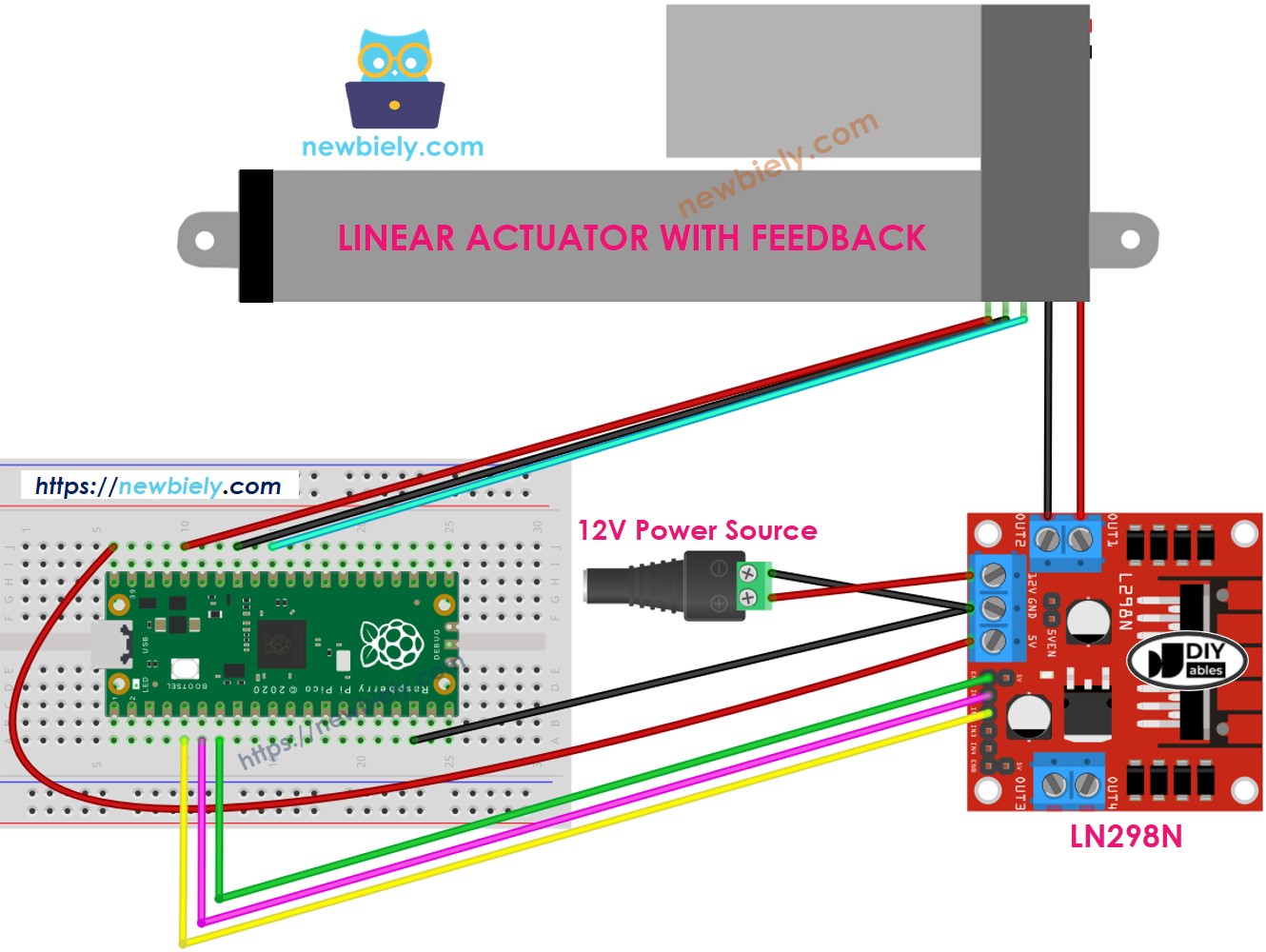
This image is created using Fritzing. Click to enlarge image
How to control extend/retract a linear actuator
Learn about the Raspberry Pi Pico Actuator by clicking here.
How to find the position of the linear actuator
Here's how to locate the stroke position on a linear actuator:
Calibration
- Use a ruler to find out how long the actuator moves or look at the datasheet for details.
- To see the values when the linear actuator is fully open or closed, run this code.
- You will see the record in the Shell at the bottom of Thonny like in the example below.
- Write these values.
- If the minimum value is more than the maximum value, exchange IN1_PIN with INI2_PIN.
- Change three values in the following code.
Raspberry Pi Pico code that calculate the position of the actuator
- Modify the three altered values in the code
- Upload the code to the Raspberry Pi Pico
- Check out the results in the Shell at the bottom of Thonny