Raspberry Pi Pico - Button
The button is a basic but essential part used in many Raspberry Pi Pico projects. It might look complex due to its mechanical and physical features, which might be hard for beginners. This guide is meant to make it easy for beginners to understand. Let's start!
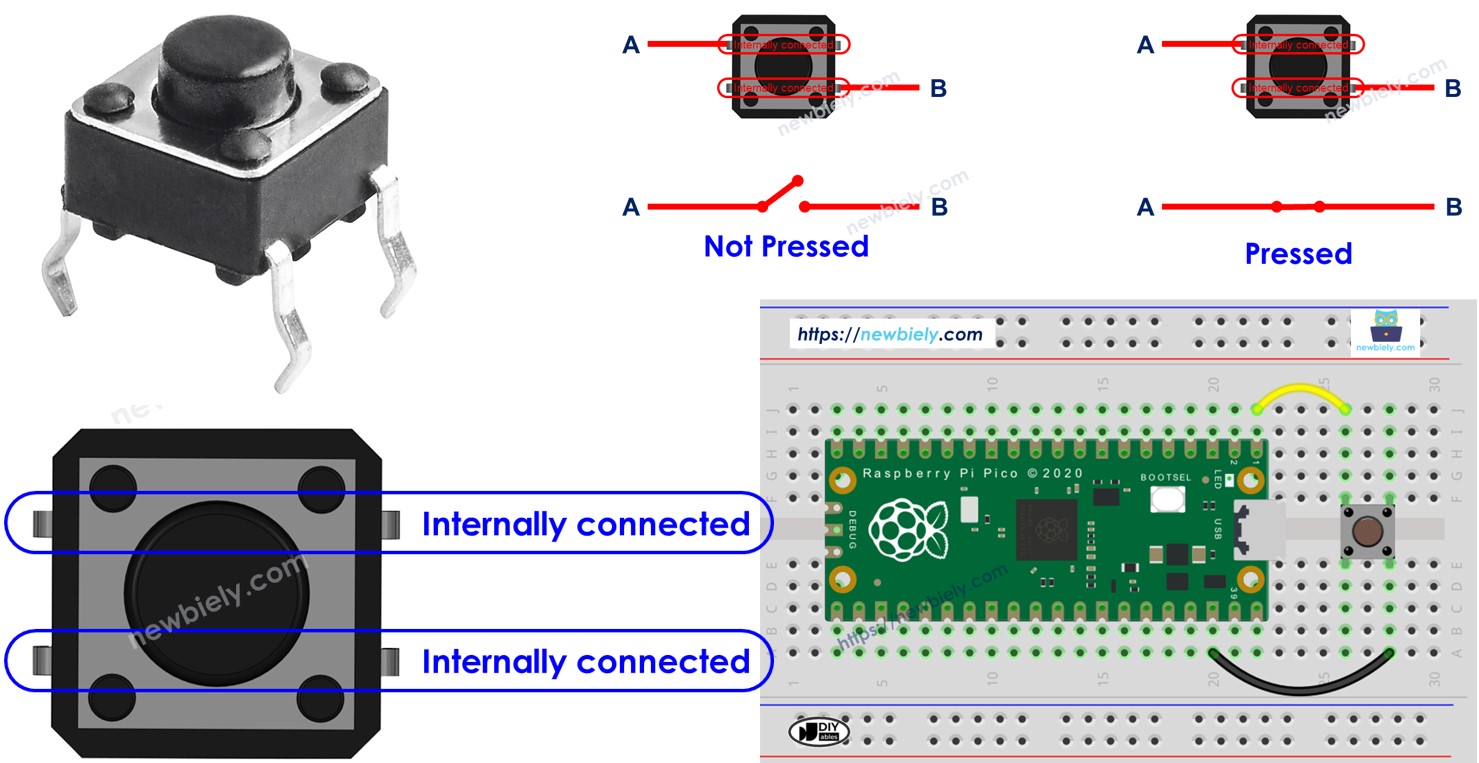
※ NOTE THAT:
Before we start using a button with Raspberry Pi Pico, please be aware of two typical issues that beginners often face:
The floating input problem:
Symptom: The state of the input pin connected to a button on the Raspberry Pi Pico is unstable and does not accurately reflect the button's state.
Cause: The button's pin lacks a connection to either a pull-down or a pull-up resistor.
Solution: Attach a pull-down or pull-up resistor to the input pin. We will explain this more later in the guide.
The chattering phenomenon:
Symptom: The code in Raspberry Pi Pico reads the button's state to notice presses by detecting state changes (from HIGH to LOW, or vice versa). But it might register multiple presses with just one actual button press.
Cause: The mechanical attributes of the button may cause the input pin’s state to quickly switch back and forth multiple times with a single press.
Solution: Use a method called debounce. Details will be provided in a later tutorial on debouncing.
Note, chattering mainly affects situations where you need to count button presses accurately. It might not be a significant issue in other scenarios.
Or you can buy the following kits:
Disclosure: Some of the links provided in this section are Amazon affiliate links. We may receive a commission for any purchases made through these links at no additional cost to you.
Additionally, some of these links are for products from our own brand,
DIYables .
The push button, sometimes called a tactile button or a momentary switch, is a type of switch that connects when you press and keep it down, and disconnects when you let it go. There are various kinds of push buttons, mainly categorized into two groups:
These pins are paired internally, so we just need to use two of the four pins that are not joined inside.
There are four methods to connect the button, as shown in the image, but effectively only two since they are similar.
A button has four pins, but we usually use only two. This helps it stay stable on the board and handle pressure well.
image source: diyables.io
GND: Connect this pin to ground.
VCC: Connect this pin to a 3.3V power supply.
OUT: Connect this pin to a digital input on your Raspberry Pi Pico Push.
With this configuration, the module outputs LOW when the button is not pressed and outputs HIGH when the button is pressed.
When the button is not pressed, pin A and pin B are disconnected.
When the button is pressed, pin A and pin B are connected.
One button pin connects to VCC or GND, while the other pin connects to a pin on the Raspberry Pi Pico.
We can find out if the button is pressed by checking the input pin state on the Raspberry Pi Pico.
The connection between the button's status and when it is pressed is determined by how we link the button to the Raspberry Pi Pico and the configuration of the Raspberry Pi Pico's pin.
You can use a button with Raspberry Pi Pico in two ways:
Connect one pin of the button to VCC and the other pin to a Raspberry Pi Pico pin. Include a pull-down resistor in this connection.
When the button is pressed, the Raspberry Pi Pico pin becomes HIGH. When not pressed, it is LOW.
You must use an extra resistor that you add yourself.
Connect one pin of the button to GNRD and the other pin to a Raspberry Pi Pico pin. Include a pull-up resistor in this connection.
When the button is pressed, the Raspberry Pi Pico pin becomes LOW. When not pressed, it is HIGH.
You may use a built-in resistor (internal) or add one yourself (external). To use the built-in resistor, set it up using Raspberry Pi Pico's code.
※ NOTE THAT:
Without a pull-down or pull-up resistor, the input pin is "floating" when the button is not pressed, causing the pin's state to unpredictably switch to HIGH or LOW, leading to inaccurate readings.
Poor practice: Configure the Raspberry Pi Pico pin as an input with button = Pin(BUTTON_PIN, Pin.IN) and no pull-down or pull-up resistor.
Good practice: Configure the Raspberry Pi Pico pin with an internal pull-up resistor using button = Pin(BUTTON_PIN, Pin.IN, Pin.PULL_UP). This eliminates the need for an external resistor.
This guide shows you the easiest way to start: we use a simple method where you set up a Raspberry Pi Pico pin with an internal pull-up input. This way, you don't need an external resistor. Beginners should just use the Raspberry Pi Pico code we provide.
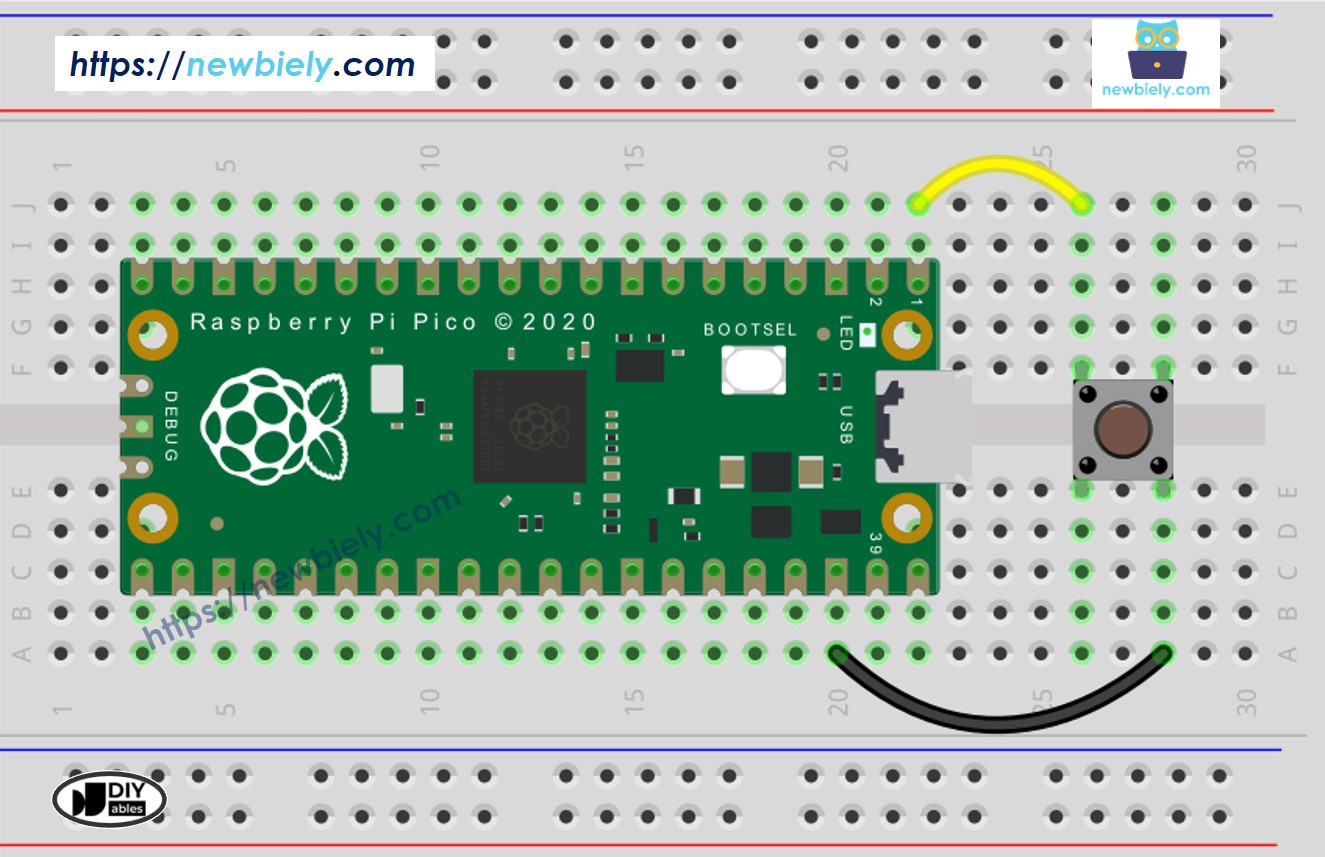
This image is created using Fritzing. Click to enlarge image
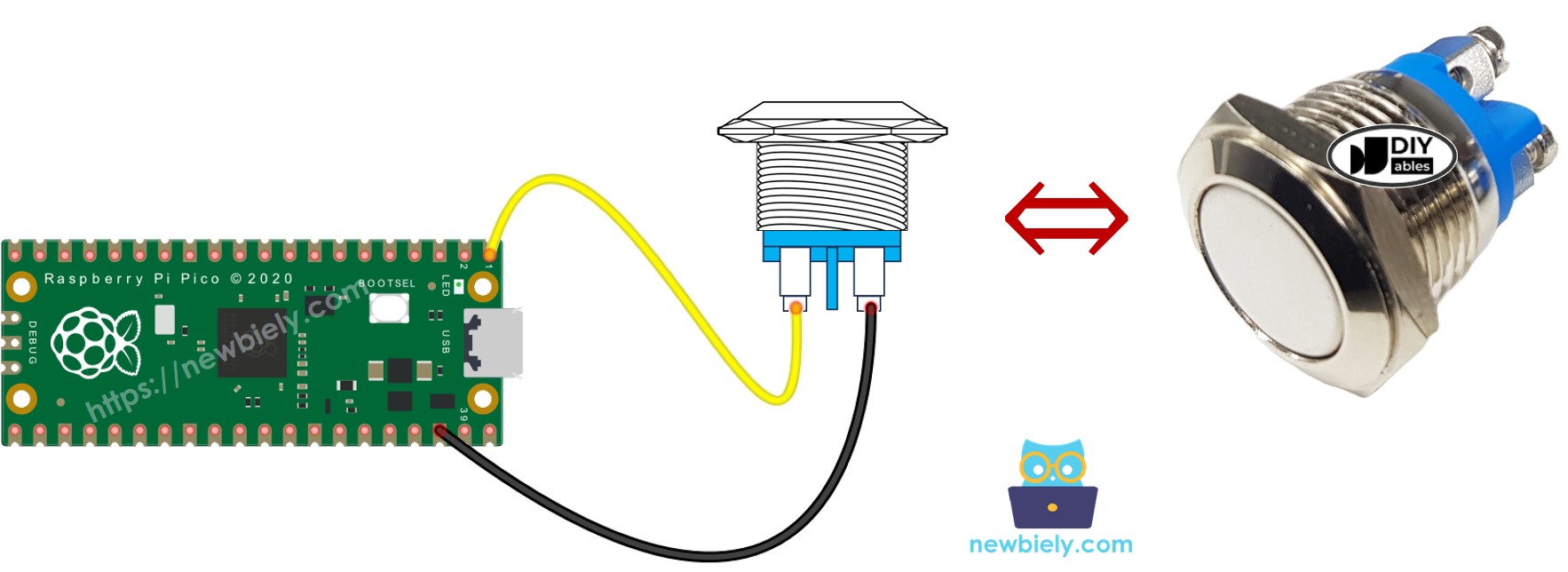
This image is created using Fritzing. Click to enlarge image
Configures pin of the Raspberry Pi Pico for use as an input with an internal pull-up resistor, employing the Pin(BUTTON_PIN, Pin.IN, Pin.PULL_UP) function.
button = Pin(BUTTON_PIN, Pin.IN, Pin.PULL_UP)
button_state = button.value()
※ NOTE THAT:
There are two common situations:
The first: When the input is HIGH, do something. When the input is LOW, do the opposite.
The second: When the input changes from LOW to HIGH (or from HIGH to LOW), do something.
Depending on the goal, we pick one of these options. For example, when using a button to control a light (LED):
If we need the light to turn ON when pressing the button and turn OFF when not pressing it, we should use the first situation.
If we want the light to toggle ON and OFF each time the button is pressed, we should use the second situation.
from machine import Pin
import time
BUTTON_PIN = 0
button = Pin(BUTTON_PIN, Pin.IN, Pin.PULL_UP)
while True:
button_state = button.value()
print(button_state)
time.sleep(0.5)
Please follow these instructions step by step:
Ensure that Thonny IDE is installed on your computer.
Ensure that MicroPython firmware is installed on your Raspberry Pi Pico.
Connect the button to the Raspberry Pi Pico according to the provided diagram.
Connect the Raspberry Pi Pico to your computer using a USB cable.
Launch the Thonny IDE on your computer.
On Thonny IDE, select MicroPython (Raspberry Pi Pico) Interpreter by navigating to Tools Options.
In the Interpreter tab, select MicroPython (Raspberry Pi Pico) from the drop-down menu.
Ensure the correct port is selected. Thonny IDE should automatically detect the port, but you may need to select it manually (e.g., COM3 on Windows or /dev/ttyACM0 on Linux).
Copy the above code and paste it to the Thonny IDE's editor.
Save the script to your Raspberry Pi Pico by:
Click the Save button, or use Ctrl+S keys.
In the save dialog, you will see two sections: This computer and Raspberry Pi Pico. Select Raspberry Pi Pico
Save the file as main.py
Click the green Run button (or press F5) to run the script. The script will execute.
Check out the message in the Shell at the bottom of Thonny.
>>> %Run -c $EDITOR_CONTENT
MPY: soft reboot
1
1
1
0
0
0
0
0
0
1
1
1
MicroPython (Raspberry Pi Pico) • Board CDC @ COM29 ≡
1 means not pressed, 0 means pressed.
If you name your script main.py and save it to the root directory of the Raspberry Pi Pico, it will automatically run each time the Pico is powered on or reset. This is useful for standalone applications that need to start running immediately upon power-up. If you name your script another name other than main.py, you will need to manually run it from Thonnys's Shell.
You can find the explanation in the comments section of the Raspberry Pi Pico code provided earlier.
Let's modify the code so it can detect when buttons are pressed and released.
from machine import Pin
import time
BUTTON_PIN = 0
button = Pin(BUTTON_PIN, Pin.IN, Pin.PULL_UP)
prev_button_state = 1
while True:
button_state = button.value()
if prev_button_state == 0 and button_state == 1:
print("The button is released")
if prev_button_state == 1 and button_state == 0:
print("The button is pressed")
prev_button_state = button_state
Copy the code above and paste it into Thonny IDE.
Click the green Run button (or press F5) to run the script. The script will execute.
Press the button and then let it go.
Check out the message in the Shell at the bottom of Thonny.
>>> %Run -c $EDITOR_CONTENT
MPY: soft reboot
The button is pressed
The button is released
MicroPython (Raspberry Pi Pico) • Board CDC @ COM29 ≡
※ NOTE THAT:
Sometimes, when you press and release a button once, the Thonny's Shell may show that it happened many times. This common issue is called "chattering phenomenon." More information and solution is available in the
Raspberry Pi Pico - Button Debounce tutorial.
If you’re using the
button module, set the pin as an input with
button = Pin(BUTTON_PIN, Pin.IN). In this configuration, the module outputs
LOW when the button is not pressed and
HIGH when it is pressed.