Raspberry Pi Pico - Water Sensor
This guide will show you how to use a water sensor with Raspberry Pi Pico. A water sensor can detect water leaks, measure rainfall, see if a tank is too full, or monitor water levels. We will learn about these in detail.
- Steps to connect the water sensor to Raspberry Pi Pico
- Programming Raspberry Pi Pico to read values from the water sensor
- Detecting water leakage, rainfall, and tank overflow with Raspberry Pi Pico
- Measuring water levels with Raspberry Pi Pico
- Calibrating the water sensor with Raspberry Pi Pico
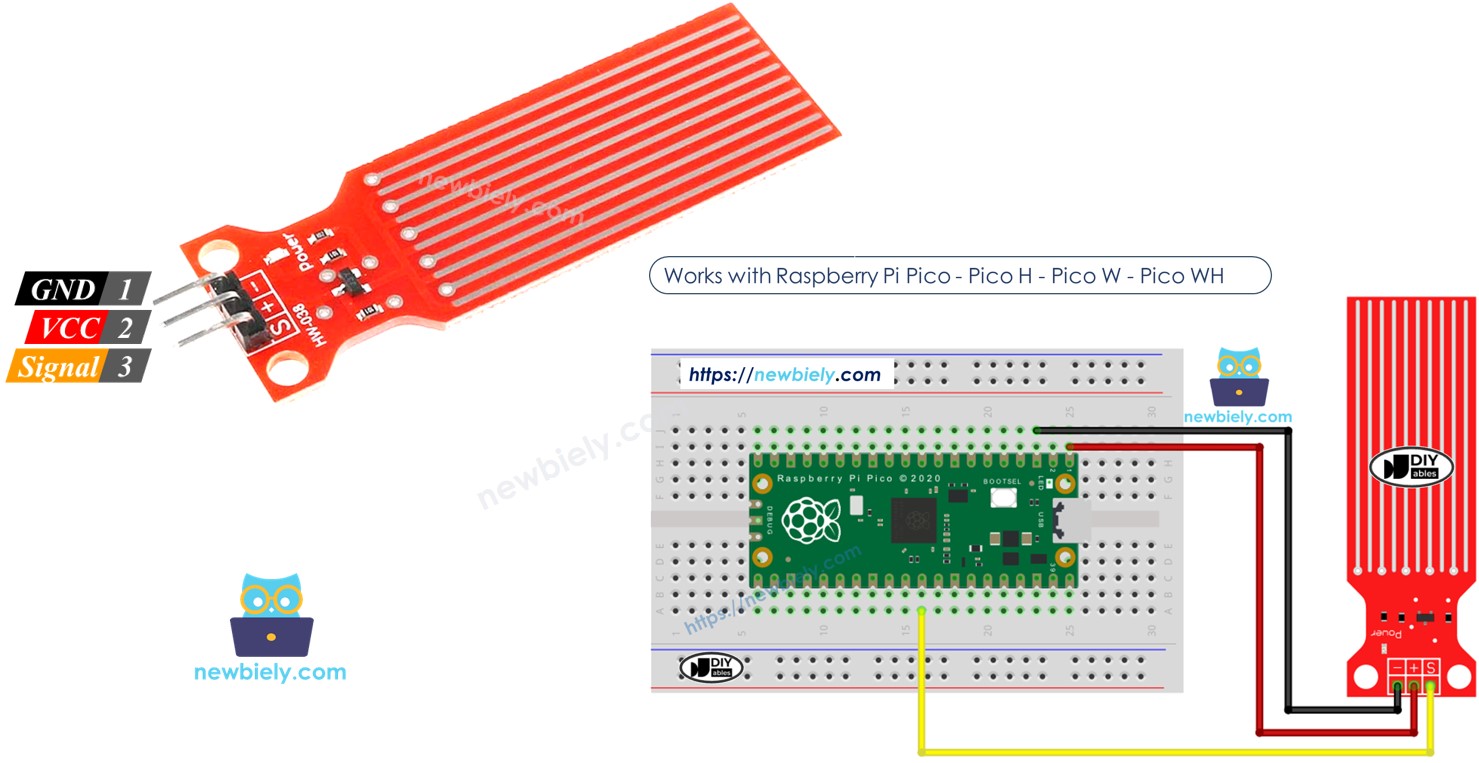
Hardware Preparation
1 | × | Raspberry Pi Pico W | |
1 | × | Raspberry Pi Pico Alternatively, | |
1 | × | Micro USB Cable | |
1 | × | Water level sensor | |
1 | × | Jumper Wires | |
1 | × | Breadboard | |
1 | × | Recommended: Screw Terminal Expansion Board for Raspberry Pi Pico |
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Water Level Sensor
Water Level Sensor Pinout
The water level sensor has three pins:
- S (Signal) pin: Analog output. Connect to Raspberry Pi Pico's analog input.
- + (VCC) pin: Provides power to the sensor. Use between 3.3V and 5V.
- - (GND) pin: Ground connection.
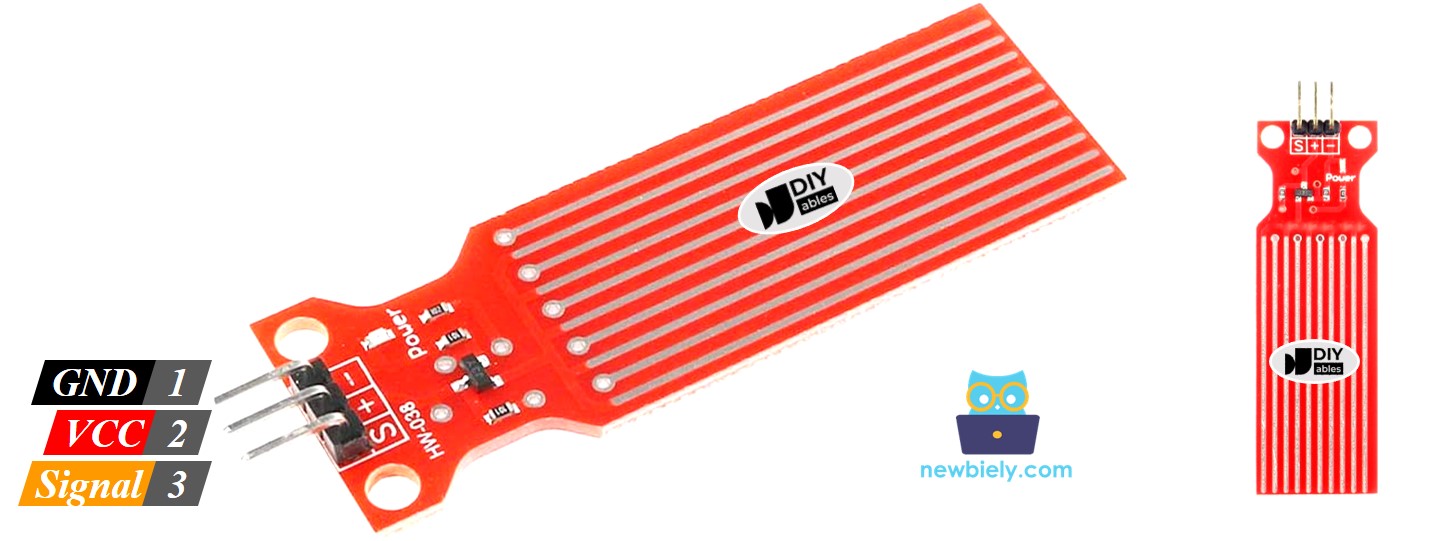
※ NOTE THAT:
The signal from the sensor changes when the voltage at its VCC pin is adjusted.
How Water Level Sensor Works
As the sensor encounters more water, the voltage on the signal pin goes up.
Let's examine this more carefully.
The sensor has ten copper lines visible. It contains five power lines and five sensor lines. These lines are placed side by side, with one sensor line between every two power lines. Normally, these lines are separate, but if the sensor is placed in water, the water links the lines.
The traces act like an adjustable resistor (like a potentiometer) that changes its resistance depending on the water level.
- The change in resistance indicates the distance from the top of the sensor to the surface of the water.
- The resistance gets lower as the water level rises.
- The more the sensor is submerged in water, the better it conducts electricity, which lowers the resistance.
- The less the sensor is submerged in water, the poorer it conducts electricity, which raises the resistance.
- The sensor produces an output voltage that depends on the resistance.
We can determine the water level by checking the voltage.
Wiring Diagram
Connect the sensor's VCC pin to the 5V pin on the Raspberry Pi Pico and the sensor's GND pin to the GND pin on the Raspberry Pi Pico to power the sensor.
It's not recommended to keep the sensor powered on in a wet environment because it can damage the sensor quickly and shorten its life. To prevent this, only turn on the sensor when you need to check its data. Connect the VCC pin of the sensor to a digital pin on the Raspberry Pi Pico. To read the sensor, set the Pico's pin to HIGH. After reading, set it to LOW.
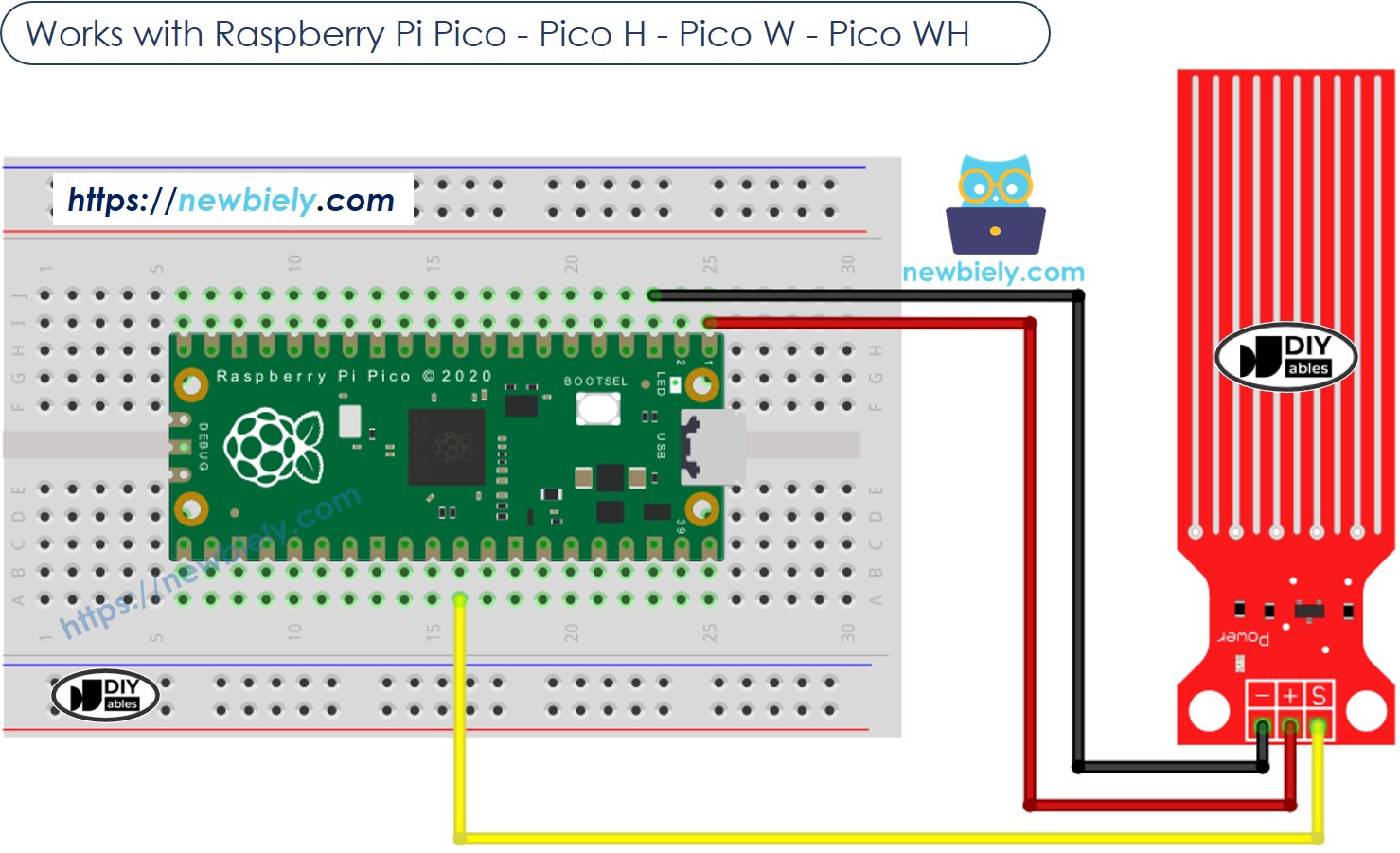
This image is created using Fritzing. Click to enlarge image
Raspberry Pi Pico Code - Reading Value from Water Sensor
Detailed Instructions
Please follow these instructions step by step:
- Ensure that Thonny IDE is installed on your computer.
- Ensure that MicroPython firmware is installed on your Raspberry Pi Pico.
- If this is your first time using a Raspberry Pico, refer to the Raspberry Pi Pico - Getting Started tutorial for detailed instructions.
- Connect the Raspberry Pi Pico to the water sensor according to the provided diagram.
- Connect the Raspberry Pi Pico to your computer using a USB cable.
- Launch the Thonny IDE on your computer.
- On Thonny IDE, select MicroPython (Raspberry Pi Pico) Interpreter by navigating to Tools Options.
- In the Interpreter tab, select MicroPython (Raspberry Pi Pico) from the drop-down menu.
- Ensure the correct port is selected. Thonny IDE should automatically detect the port, but you may need to select it manually (e.g., COM3 on Windows or /dev/ttyACM0 on Linux).
- Copy the above code and paste it to the Thonny IDE's editor.
- Save the script to your Raspberry Pi Pico by:
- Click the Save button, or use Ctrl+S keys.
- In the save dialog, you will see two sections: This computer and Raspberry Pi Pico. Select Raspberry Pi Pico
- Save the file as main.py
- Click the green Run button (or press F5) to run the script. The script will execute.
- Slowly put the sensor into a glass of water.
- Check out the message in the Shell at the bottom of Thonny.
. It will display a value of 0 if the sensor isn't touching anything.
※ NOTE THAT:
Do not put the sensor completely underwater; only the visible parts on the electronic board should touch the water. Install it with care.
If you name your script main.py and save it to the root directory of the Raspberry Pi Pico, it will automatically run each time the Pico is powered on or reset. This is useful for standalone applications that need to start running immediately upon power-up. If you name your script another name other than main.py, you will need to manually run it from Thonnys's Shell.
How To Detect Water Leakage
To check for water leaks, rain, or overflowing tanks, we compare the reading to a preset limit. We set this limit in the calibration part of this tutorial.
Raspberry Pi Pico Code - Finding Water Leaks
How To Measure The Water Level
To divide the highest water level into separate stages and find out which stage it is currently at, follow the method in the code below. Note, the highest water level is the same as the sensor's height. This code divides the top height into 4 stages.
※ NOTE THAT:
- SENSOR_MIN and SENSOR_MAX are set when calibrating.
- The mapping method described might not be very accurate, but it works well for many purposes. To increase accuracy, measure the threshold values for each level as described in the calibration section.
Water Level Sensor Calibration
The sensor's performance changes with the water level and its conductivity. Pure water cannot conduct electricity, but water with minerals and impurities can. If the water is more conductive, the sensor is more responsive. Also, the output value varies when different voltages are used at the sensor's VCC pin.
For precise measurements with the water sensor, we recommend setting it up for the specific water type you are checking.
Before setting a limit for an action, first test the real value from the sensor.
How to do the test:
- Look at the given drawing to check the sensor’s reading.
- Put the sensor in the water until the level you want.
- Write down the number you see in the Shell at the bottom of Thonny.
- Use this number as the limit to start an action.
You might need to try this test several times to succeed.
The test can also help to find out:
- Lowest sensor reading when not in water.
- Highest sensor reading when fully submerged in water.
- A set value to identify water leaks.
- Set values for different levels on your scale.