Arduino MicroPython Button Debounce
When you connect a button to an Arduino, it may sometimes appear as if the button has been pressed multiple times, even though you only pressed it once. This happens because the button can rapidly switch between pressed and unpressed states, a phenomenon known as bouncing or chattering. Bouncing can create issues in your Arduino program.
This guide will teach you how to resolve this problem on the Arduino by using a technique called debouncing with MicroPython. Debouncing ensures that the Arduino interprets each button press as a single, distinct action.
We will go through the following steps:
Writing Arduino MicroPython code without debouncing a button.
Writing Arduino MicroPython code with debouncing for a single button.
Writing Arduino MicroPython code with debouncing for multiple buttons.
Or you can buy the following sensor kits:
Disclosure: Some of the links provided in this section are Amazon affiliate links. We may receive a commission for any purchases made through these links at no additional cost to you.
Additionally, some of these links are for products from our own brand,
DIYables .
If you're not yet familiar with button (including their pinout, how it works, interfacing with the Arduino, and writing MicroPython code for the Arduino to interact with them), you can learn more at:
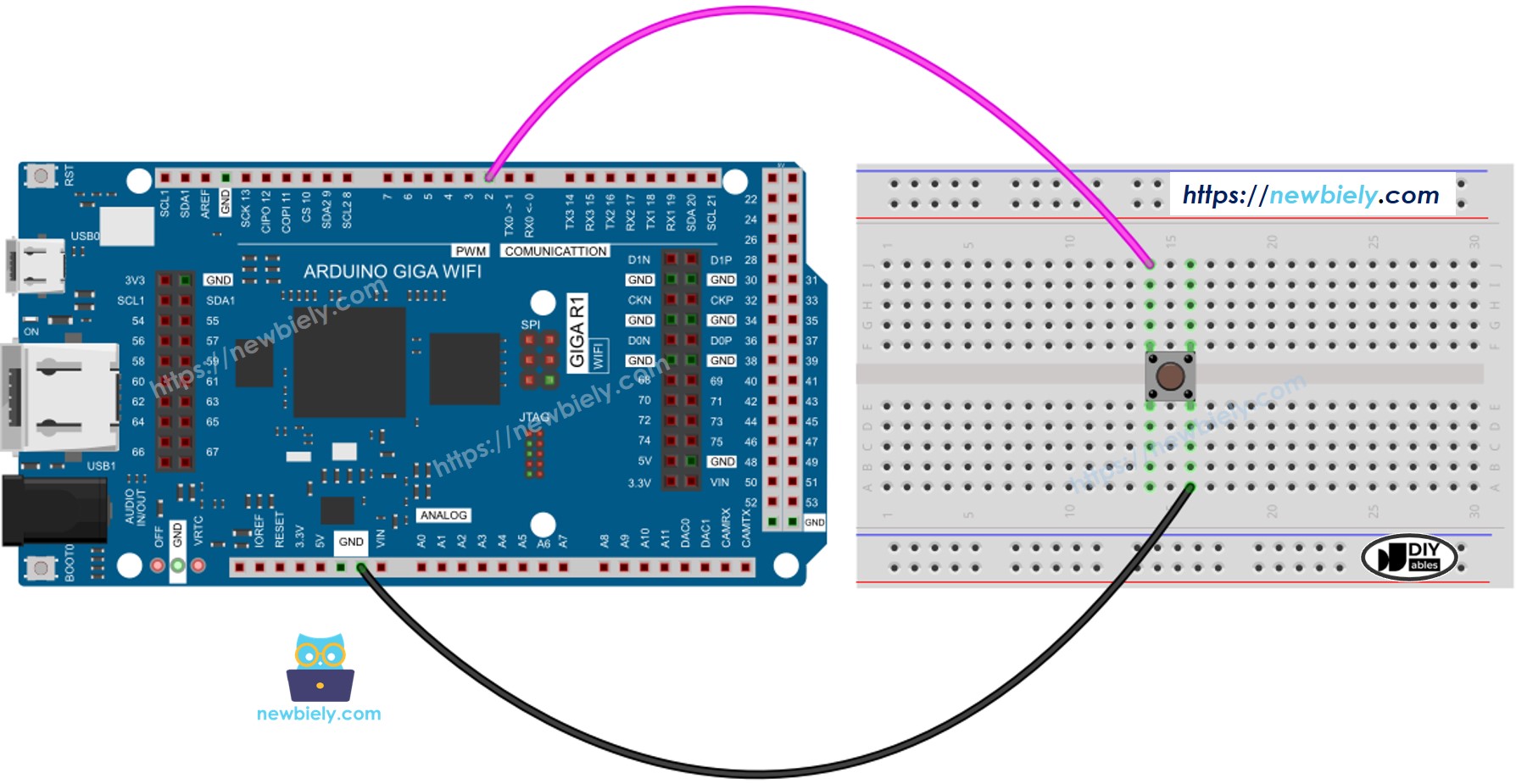
This image is created using Fritzing. Click to enlarge image
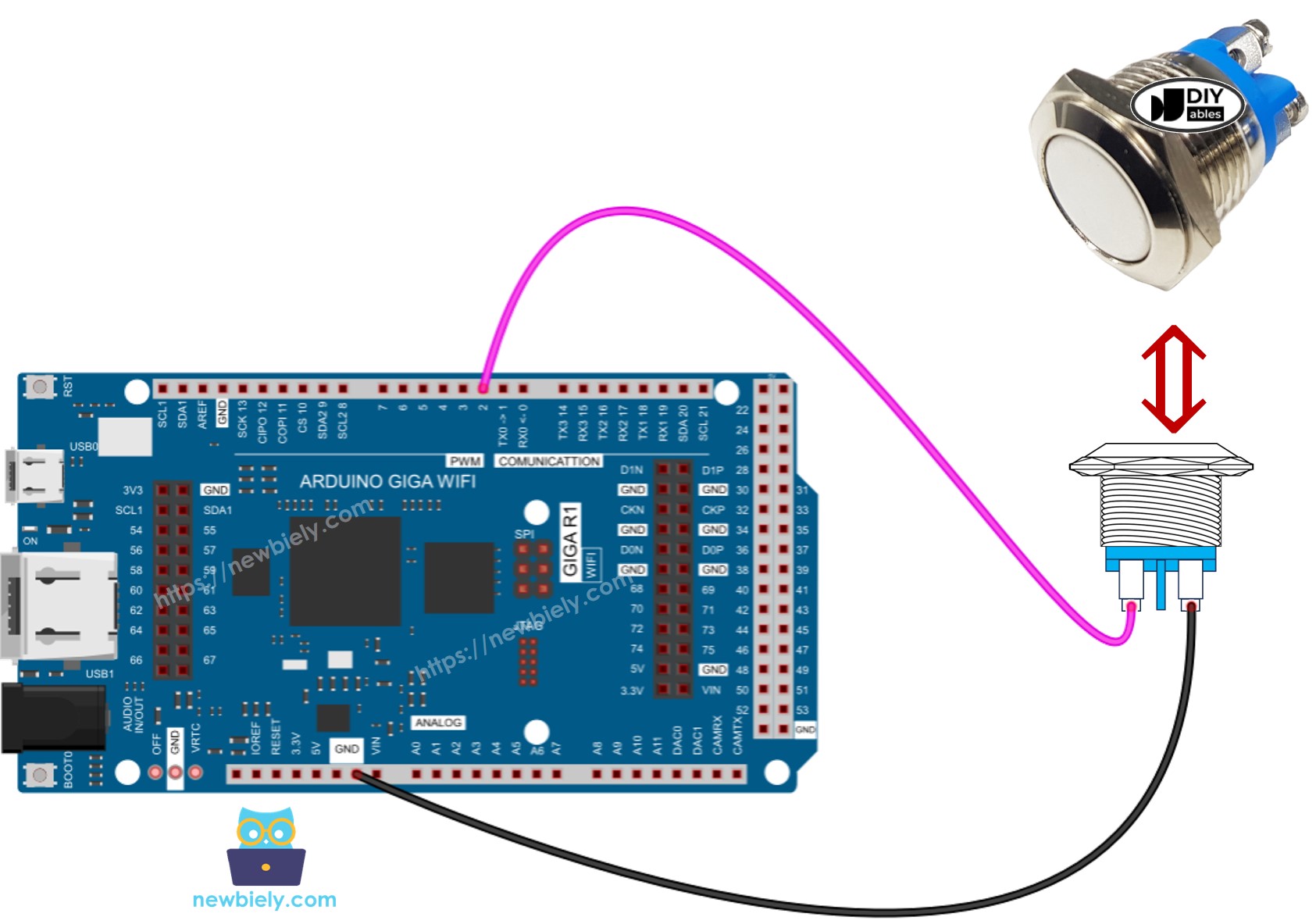
This image is created using Fritzing. Click to enlarge image
Let's compare the Arduino MicroPython code without and with debouncing to see how debouncing affects the behavior.
First, let's examine the MicroPython code for Arduino without debouncing to understand its behavior.
from machine import Pin
import time
BUTTON_PIN = 'D2'
button = Pin(BUTTON_PIN, Pin.IN, Pin.PULL_UP)
prev_button_state = 1
while True:
button_state = button.value()
if prev_button_state == 0 and button_state == 1:
print("The button is released")
if prev_button_state == 1 and button_state == 0:
print("The button is pressed")
prev_button_state = button_state
Here’s instructions on how to run the above MicroPython code on Arduino with Thonny IDE:
Make sure Thonny IDE is installed on your computer.
Make sure MicroPython firmware is installed on your Arduino board.
Connect the button to the Arduino as shown in the diagram.
Connect the Arduino board to your computer with a USB cable.
Open Thonny IDE and go to Tools Options.
Under the Interpreter tab, select MicroPython (generic) from the dropdown menu.
Select the COM port corresponding to your Arduino board (e.g., COM33 on Windows or /dev/ttyACM0 on Linux).
Copy the provided Arduino MicroPython code and paste it into Thonny's editor.
Save the MicroPython code to your Arduino by:
Clicking the Save button or pressing Ctrl+S.
In the save dialog, choose MicroPython device and name the file main.py.
Click the green Run button (or press F5) to execute the code.
Press and hold the button for a few seconds, then release.
Check out the message in the Shell at the bottom of Thonny IDE.
>>> %Run -c $EDITOR_CONTENT
MPY: soft reboot
The button is pressed
The button is pressed
The button is pressed
The button is released
The button is released
MicroPython (generic) • Giga Virtual Comm Port in FS Mode @ COM33 ≡
As you can see in the log, you pressed the button only once, but the Arduino detected multiple presses.
from DIYables_MicroPython_Button import Button
import time
button = Button('D2')
button.set_debounce_time(100)
while True:
button.loop()
if button.is_pressed():
print("The button is pressed")
if button.is_released():
print("The button is released")
In Thonny IDE, navigate to the Tools Manage packages on the Thonny IDE.
Search “DIYables-MicroPython-Button”, then find the Button library created by DIYables.
Click on DIYables-MicroPython-Button, then click Install button to install Button library.
Copy the given code and paste it into the Thonny IDE's editor.
Save the script on your Arduino board.
Click the green Run button (or press F5) to start the script. The script will start running.
Press the button.
Check out the message in the Shell at the bottom of Thonny IDE.
>>> %Run -c $EDITOR_CONTENT
MPY: soft reboot
The button is pressed
The button is released
MicroPython (generic) • Giga Virtual Comm Port in FS Mode @ COM33 ≡
You pressed the button once, and the Arduino correctly identified it as a single push and release, with no additional disturbances.
※ NOTE THAT:
Different apps use different DEBOUNCE_TIME settings. Each app might have its own specific value.
Let's add debouncing to five buttons using Arduino with MicroPython. Here's how to connect them to your Arduino:
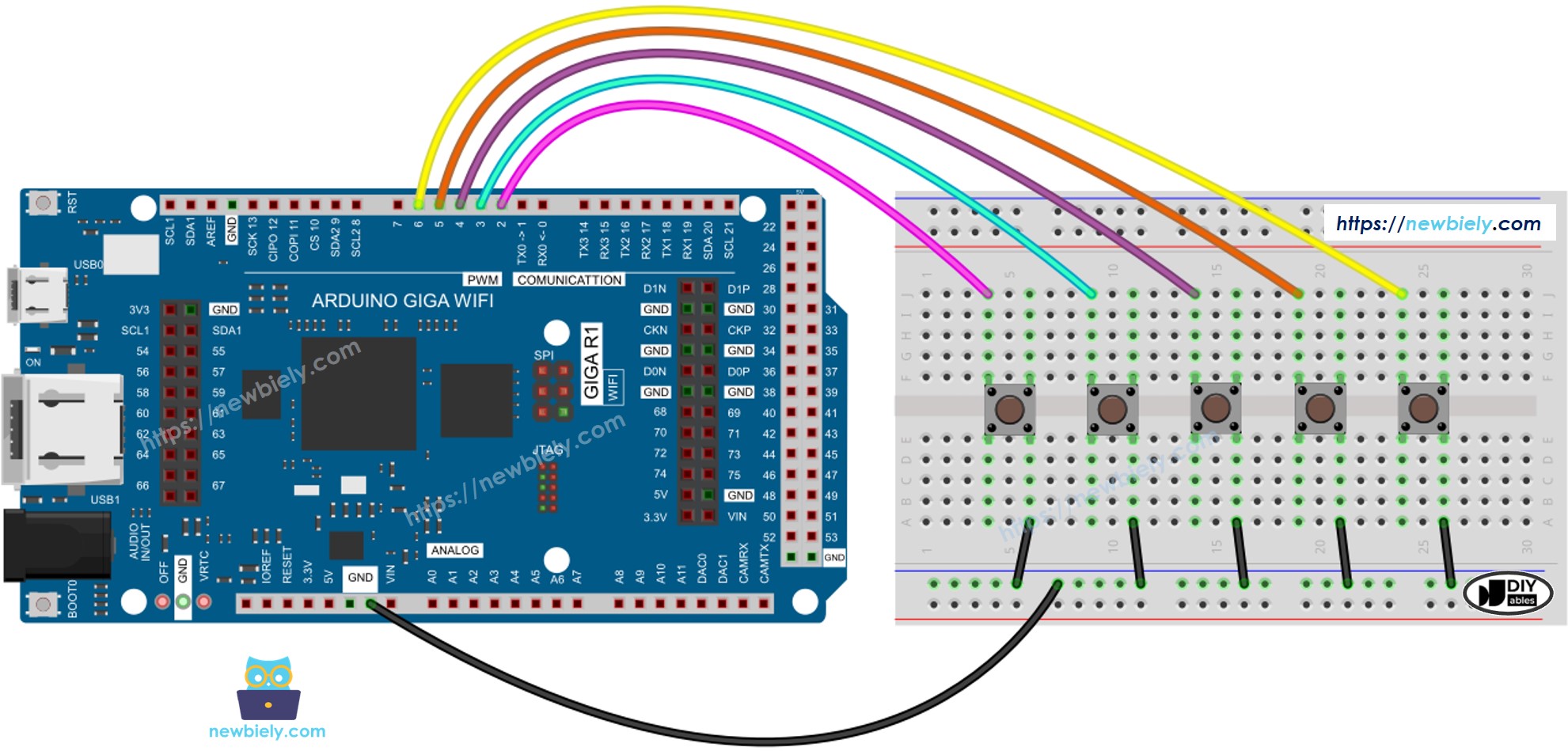
This image is created using Fritzing. Click to enlarge image
from DIYables_MicroPython_Button import Button
import time
button_1 = Button('D2')
button_2 = Button('D3')
button_3 = Button('D4')
button_4 = Button('D5')
button_5 = Button('D6')
button_1.set_debounce_time(100)
button_2.set_debounce_time(100)
button_3.set_debounce_time(100)
button_4.set_debounce_time(100)
button_5.set_debounce_time(100)
while True:
button_1.loop()
button_2.loop()
button_3.loop()
button_4.loop()
button_5.loop()
if button_1.is_pressed():
print("The button 1 is pressed")
if button_1.is_released():
print("The button 1 is released")
if button_2.is_pressed():
print("The button 2 is pressed")
if button_2.is_released():
print("The button 2 is released")
if button_3.is_pressed():
print("The button 3 is pressed")
if button_3.is_released():
print("The button 3 is released")
if button_4.is_pressed():
print("The button 4 is pressed")
if button_4.is_released():
print("The button 4 is released")
if button_5.is_pressed():
print("The button 5 is pressed")
if button_5.is_released():
print("The button 5 is released")