Arduino MicroPython Limit Switch
This guide explains how to use a limit switch with an Arduino and MicroPython. You will learn:
- How to connect the limit switch to the Arduino.
- How to write MicroPython code for the Arduino to read the status of the limit switch.
- How to write MicroPython code for the Arduino to detect when the limit switch is activated (touched) or deactivated (untouched).
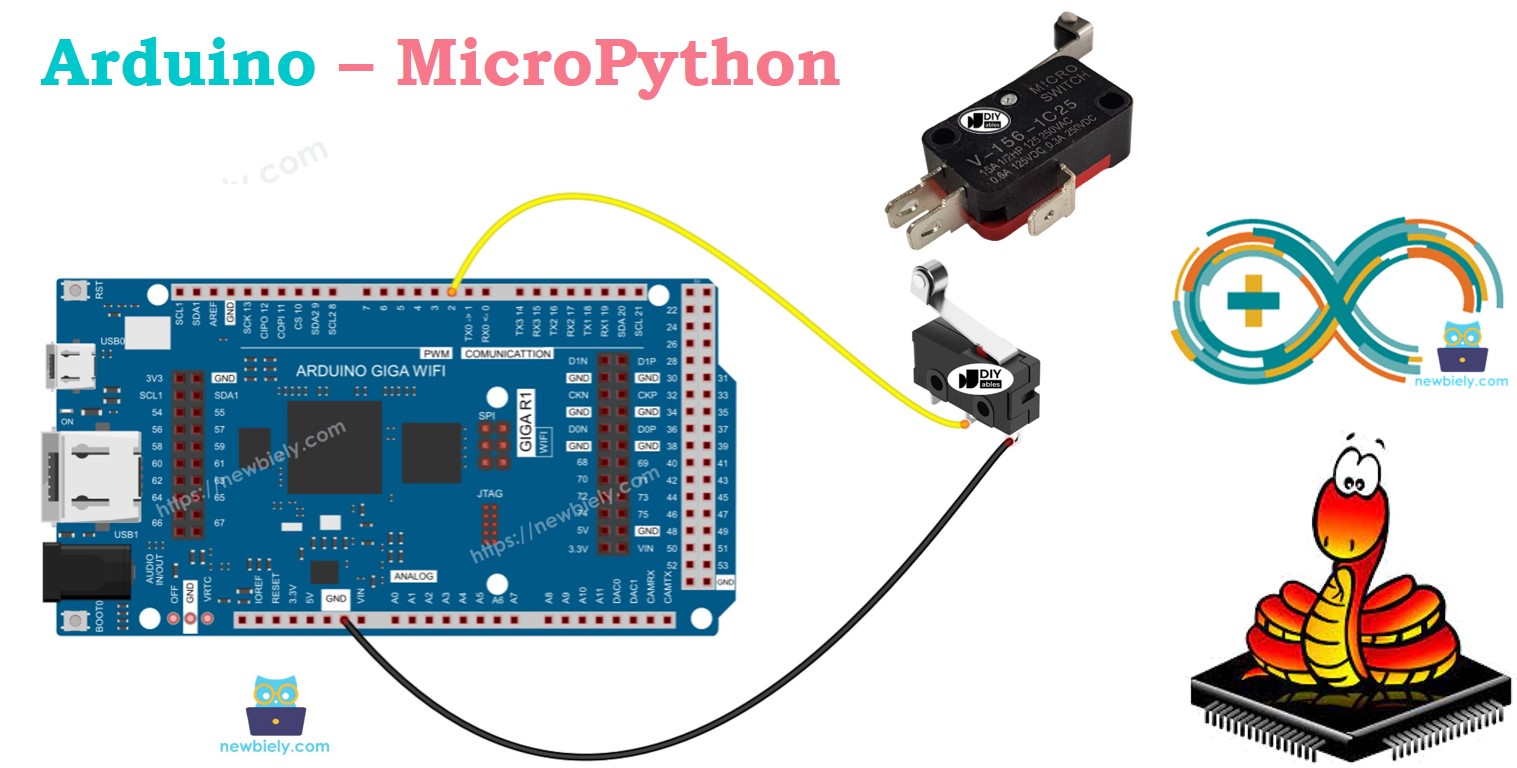
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Limit Switch
It is called a Limit Switch because its primary function is to detect when a moving object reaches its endpoint. It is also known as a Travel Switch.
Pinout
Various types of limit switches are commonly used, such as the KW12-3 and V-156-1C25. Each of these models has 3 pins:
- C pin: The common pin, used in both open and closed states.
- NO pin: The Normally Open pin, used when the circuit is open.
- NC pin: The Normally Closed pin, used when the circuit is closed.
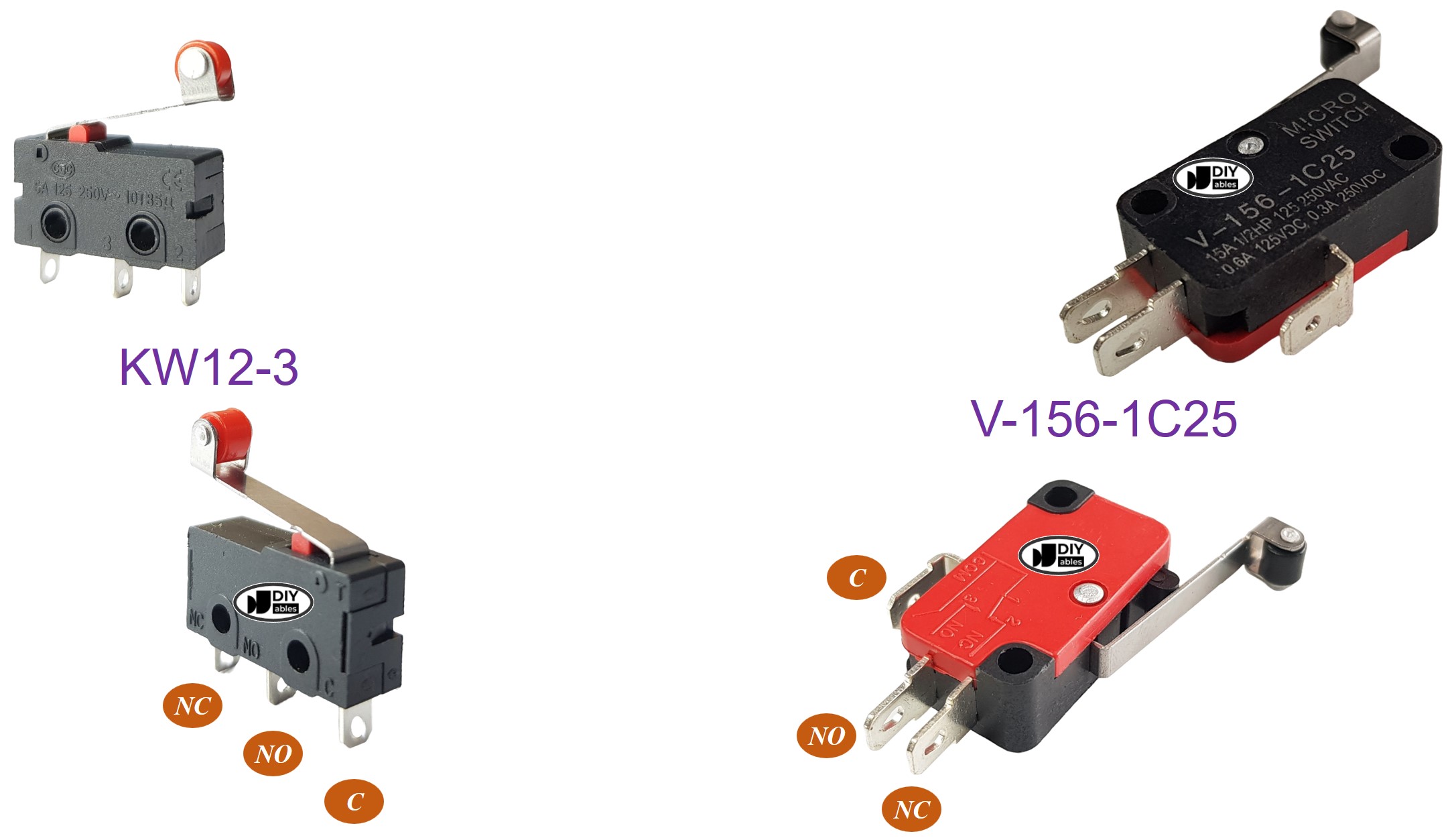
How It Works
The limit switch has three pins, but typically only two are used: the C pin and one of the other two pins. There are four possible ways to connect the limit switch. The table below shows the wiring configurations for the limit switch and the corresponding readings on the Arduino for each method:
C pin | NO pin | NC pin | Arduino Input Pin's State | |
---|---|---|---|---|
1 | GND | Arduino Input Pin (with pull-up) | NOT connected | HIGH when untouched, LOW when touched |
2 | GND | NOT connected | Arduino Input Pin (with pull-up) | LOW when untouched, HIGH when touched |
3 | VCC | Arduino Input Pin (with pull-down) | NOT connected | LOW when untouched, HIGH when touched |
4 | VCC | NOT connected | Arduino Input Pin (with pull-down) | HIGH when untouched, LOW when touched |
We can swap the positions of the GND pin and the input pin on the Arduino for each method, giving us a total of 8 different ways to connect the Arduino to a limit switch. However, we only need to choose one of the four methods mentioned earlier. For this tutorial, we will use the first method.
Wiring Diagram
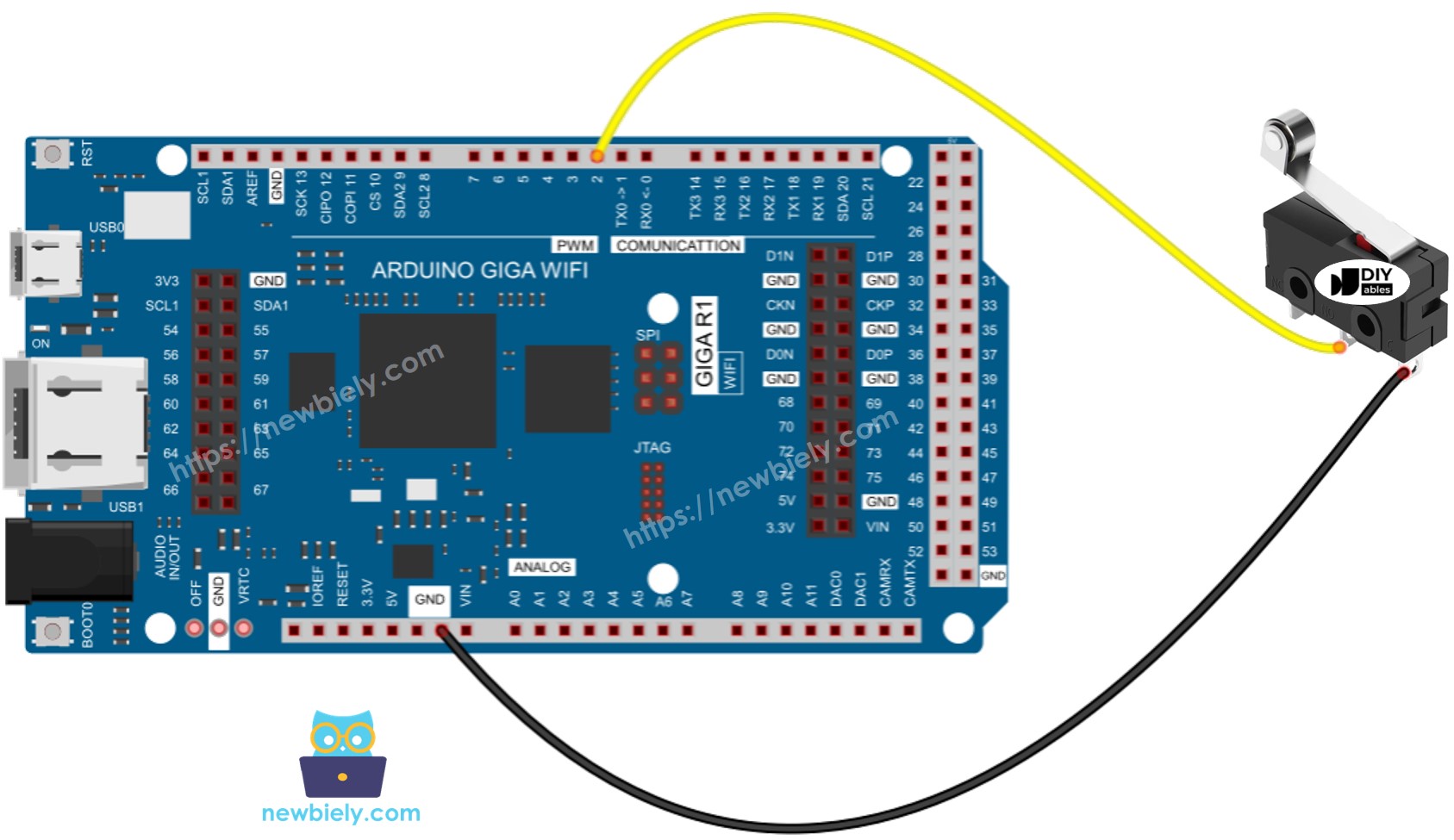
This image is created using Fritzing. Click to enlarge image
Arduino MicroPython Code - Limit Switch
A limit switch, similar to a button, requires debouncing to function properly. Debouncing can make the code more complex. Fortunately, the DIYables_MicroPython_Button library provides a debouncing feature and includes an internal pull-up resistor, which simplifies the programming process.
Here are two usual situations:
- The first: If the switch is TOUCHED, do something. If it is UNTOUCHED, do the opposite.
- The second: If the switch goes from UNTOUCHED to TOUCHED or from TOUCHED to UNTOUCHED, do something.
Let's see how to write the MicroPython script in both cases.
Arduino MicroPython Code - Read Limit Switch's State
Detailed Instructions
Here’s instructions on how to run the above MicroPython code on Arduino with Thonny IDE:
- Make sure Thonny IDE is installed on your computer.
- Make sure MicroPython firmware is installed on your Arduino board.
- If you are new to Arduino with MicroPython, see the Getting Started with Arduino and MicroPython.
- Connect the Arduino board to the limit switch according to the provided diagram.
- Connect the Arduino board to your computer with a USB cable.
- Open Thonny IDE and go to Tools Options.
- Under the Interpreter tab, select MicroPython (generic) from the dropdown menu.
- Select the COM port corresponding to your Arduino board (e.g., COM33 on Windows or /dev/ttyACM0 on Linux).
- On Thonny IDE, navigate to the Tools Manage packages on the Thonny IDE.
- Search “DIYables-MicroPython-Button”, then find the Button library created by DIYables.
- Click on DIYables-MicroPython-Button, then click Install button to install Button library.
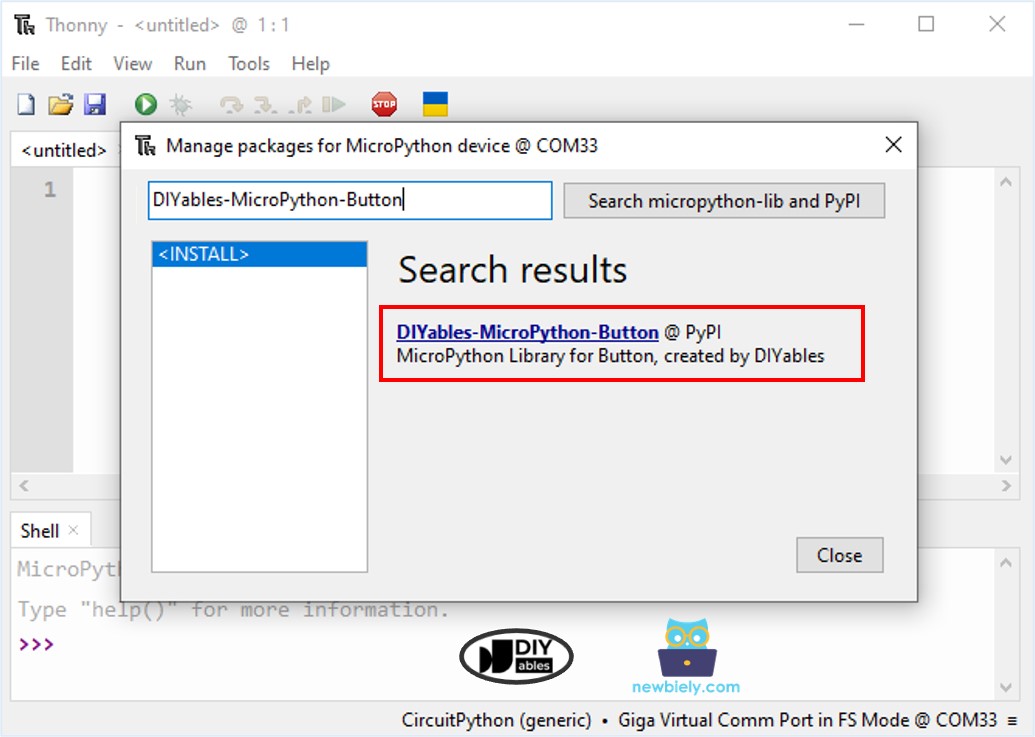
- Copy the provided Arduino MicroPython code and paste it into Thonny's editor.
- Save the MicroPython code to your Arduino by:
- Clicking the Save button or pressing Ctrl+S.
- In the save dialog, choose MicroPython device and name the file main.py.
- Click the green Run button (or press F5) to execute the code.
- Press, hold, then release the limit switch while checking out the message in the Shell at the bottom of Thonny.
Arduino MicroPython Code - Check Limit Switch's touched/untouched event
- Copy the above code and paste it to the Thonny IDE's editor.
- Save the script to your Arduino
- Click the green Run button (or press F5) to run the script. The script will execute.
- Press, hold, then release the limit switch while checking out the message in the Shell at the bottom of Thonny.