Arduino MicroPython Rain Sensor
This guide teaches you how to use a rain sensor with an Arduino and MicroPython to detect rain or snow. You will learn:
- How to connect a rain sensor to an Arduino.
- How to write MicroPython code for the Arduino to detect rain using the digital signal from the rain sensor.
- How to write MicroPython code for the Arduino to measure the intensity of rain using the analog signal from the rain sensor.
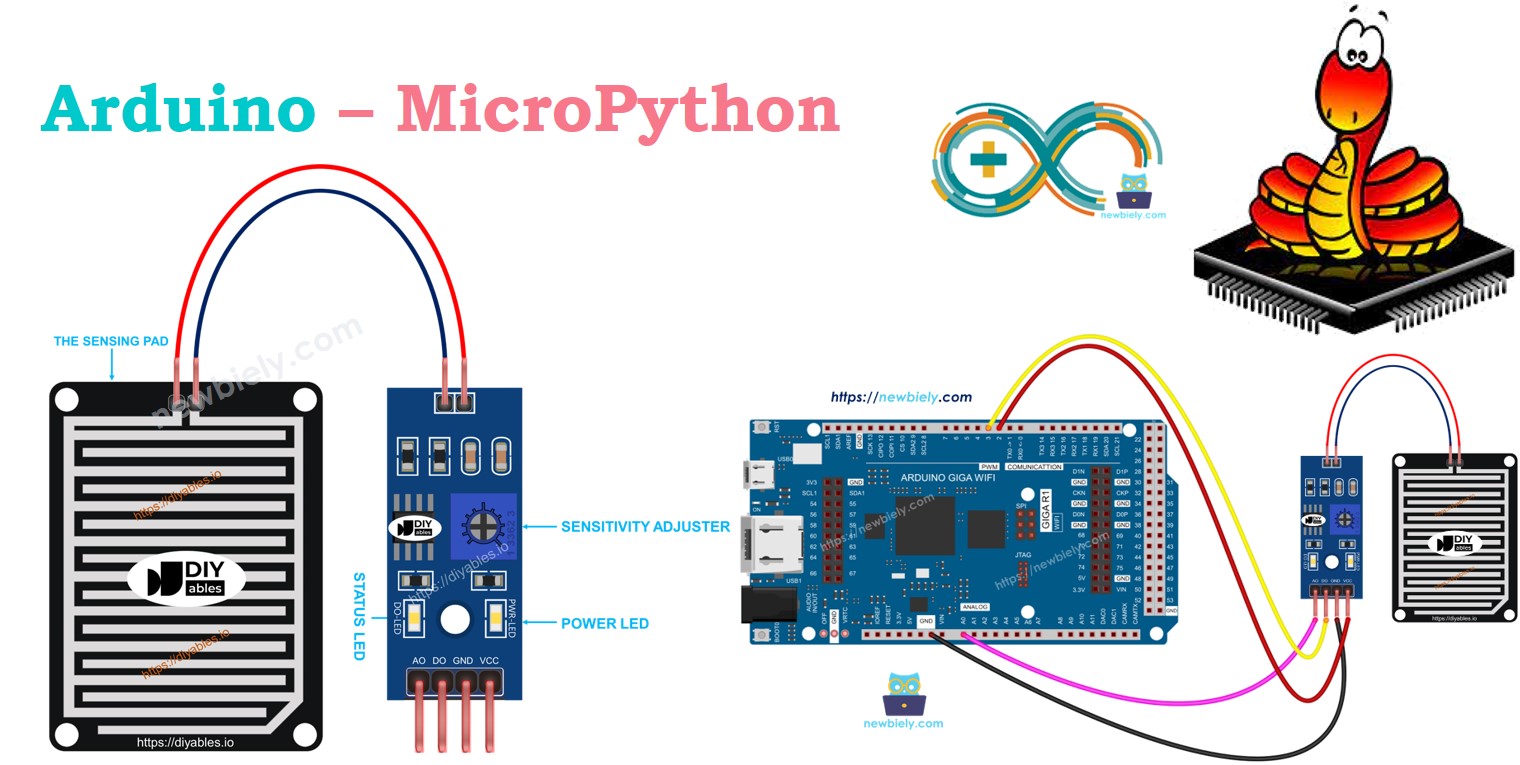
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables.
Overview of Rain Sensor
The rain sensor detects and measures the amount of rain or snow. It offers two types of output signals: a digital signal (LOW or HIGH) and an analog signal.
The rain sensor consists of two components:
- The sensor pad
- The electronic module
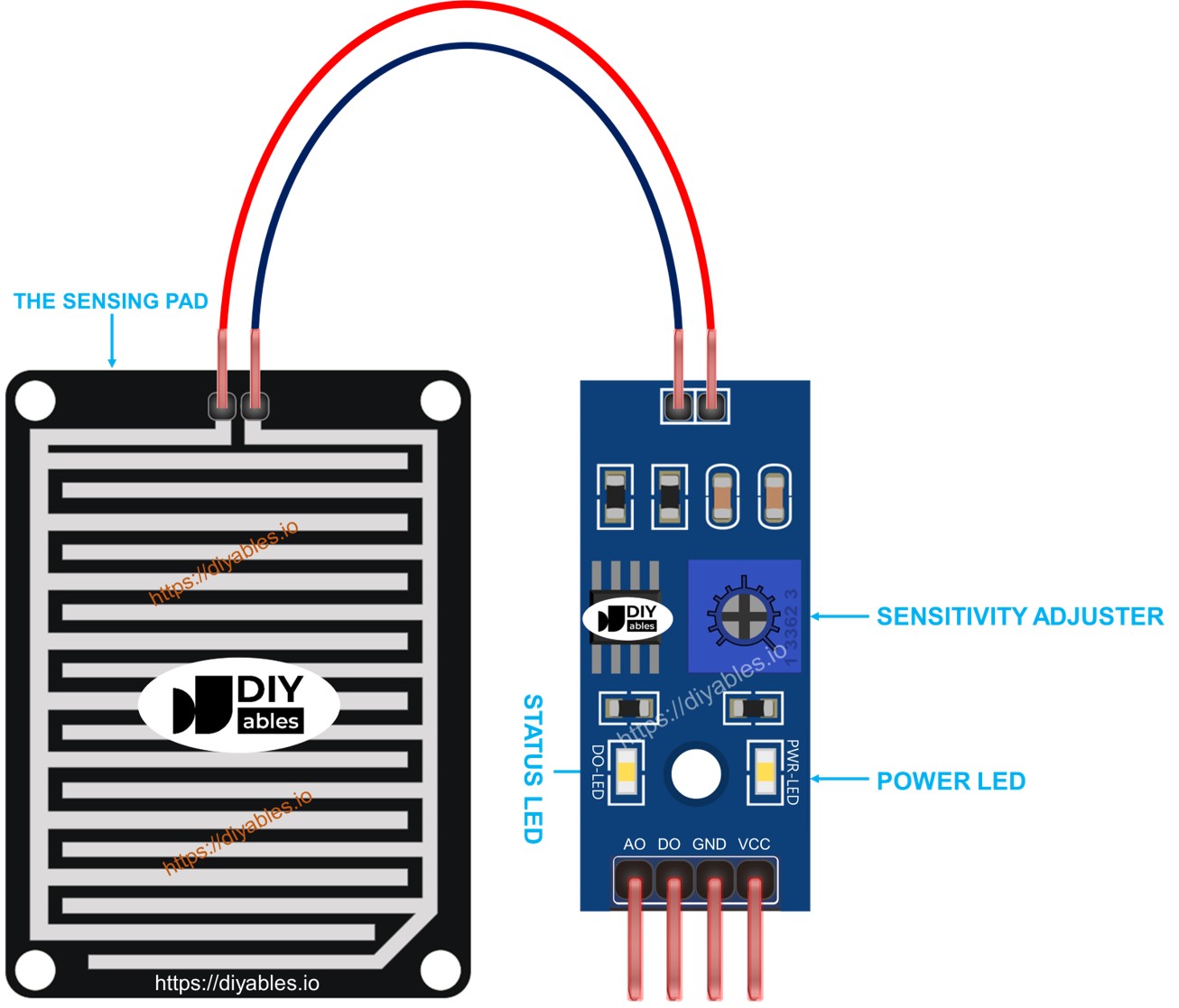
The Sensing Pad
The sensing pad is placed outdoors, where it can be exposed to rain or snow, such as on a roof. It is made up of multiple copper lines, which are categorized into two types: power lines and sensor lines. These lines stay separated and only connect when water or snow makes contact with them. Both types of lines work the same way, so you can assign any line as a power line and another as a sensor line.
The Electronic Module
The electronic module of the rain sensor converts the signal from the sensing pad into a format (analog or digital) that the Arduino can understand. It has four pins:
- VCC pin: Connects to VCC (3.3V to 5V).
- GND pin: Connects to GND (0V).
- DO pin: Digital output pin that goes HIGH when no rain is detected and LOW when rain is detected. The potentiometer can be adjusted to change the rain detection sensitivity.
- AO pin: Analog output pin where the value decreases as more water collects on the sensing pad and increases as the water dries up.
The module also features two LED indicators:
- Power indicator (PWR-LED): Lights up when the module is powered.
- Rain status indicator (DO-LED): Connected to the DO pin, it lights up when rain is detected.
How It Works
For the AO pin:
- As the sensing pad detects more water, the AO pin value decreases.
- As the sensing pad detects less water, the AO pin value increases.
- The potentiometer does not affect the AO pin value.
For the DO pin:
- The module has a potentiometer to adjust sensitivity.
- When the rain level is high, the sensor's output pin goes LOW, and the DO-LED light turns on.
- When the rain level is low, the sensor's output pin stays HIGH, and the DO-LED light remains off.
Wiring Diagram
Connect the sensor's VCC pin to a 3.3V or 5V pin on the Arduino. However, a direct connection may shorten the sensor's lifespan due to electrochemical corrosion. To prevent this, connect the VCC pin to a programmable output pin on the Arduino, supplying power to the sensor only when taking readings. This method helps minimize electrochemical corrosion.
The rain sensor module offers two outputs. You can use either one or both as required.
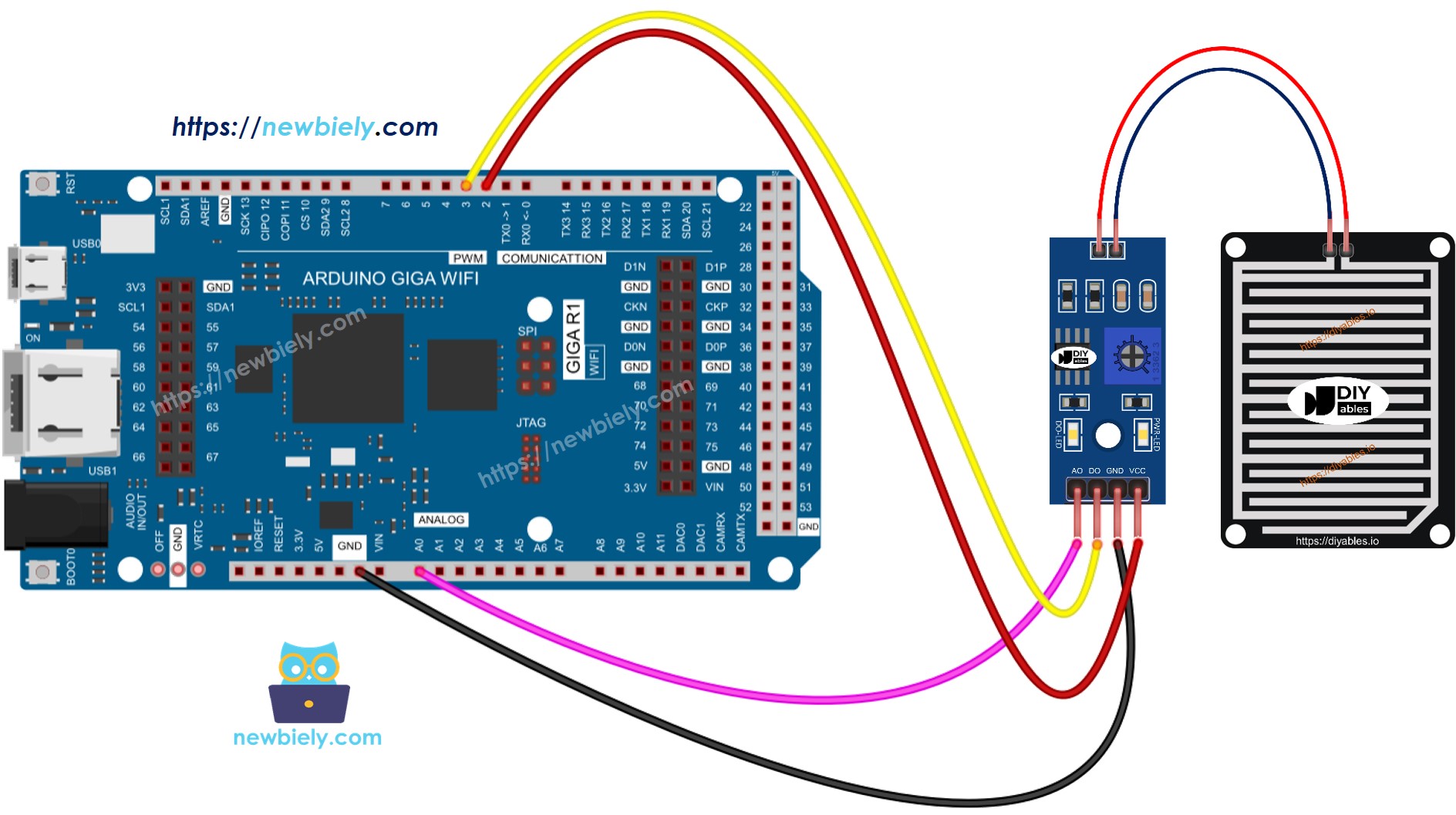
This image is created using Fritzing. Click to enlarge image
Arduino MicroPython Code - Read value from DO pin
Detailed Instructions
Here’s instructions on how to run the above MicroPython code on Arduino with Thonny IDE:
- Make sure Thonny IDE is installed on your computer.
- Make sure MicroPython firmware is installed on your Arduino board.
- If you are new to Arduino with MicroPython, see the Getting Started with Arduino and MicroPython.
- Connect the Arduino board to the rain sensor according to the provided diagram.
- Connect the Arduino board to your computer with a USB cable.
- Open Thonny IDE and go to Tools Options.
- Under the Interpreter tab, select MicroPython (generic) from the dropdown menu.
- Select the COM port corresponding to your Arduino board (e.g., COM33 on Windows or /dev/ttyACM0 on Linux).
- Copy the provided Arduino MicroPython code and paste it into Thonny's editor.
- Save the MicroPython code to your Arduino by:
- Clicking the Save button or pressing Ctrl+S.
- In the save dialog, choose MicroPython device and name the file main.py.
- Click the green Run button (or press F5) to execute the code.
- Place some water drops on the rain sensor. Do not use pure water.
- Check out the message in the Shell at the bottom of Thonny.
Please note, if the LED light remains constantly on or stays off even during rain, adjust the sensor's sensitivity by changing the potentiometer.
Arduino MicroPython Code - Read value from AO pin
Detailed Instructions
- Copy the code and open it in Thonny IDE.
- Click the green Run button (or press F5) to run the script. The script will execute.
- Put some water on the rain sensor.
- Check out the message in the Shell at the bottom of Thonny.