Arduino MicroPython Force Sensor
This guide shows you how to use a force sensor with an Arduino and MicroPython to detect the force or weight applied. You will learn:
- How a force sensor works
- How to connect the force sensor to Arduino
- How to write MicroPython code for Arduino to read data from the force sensor
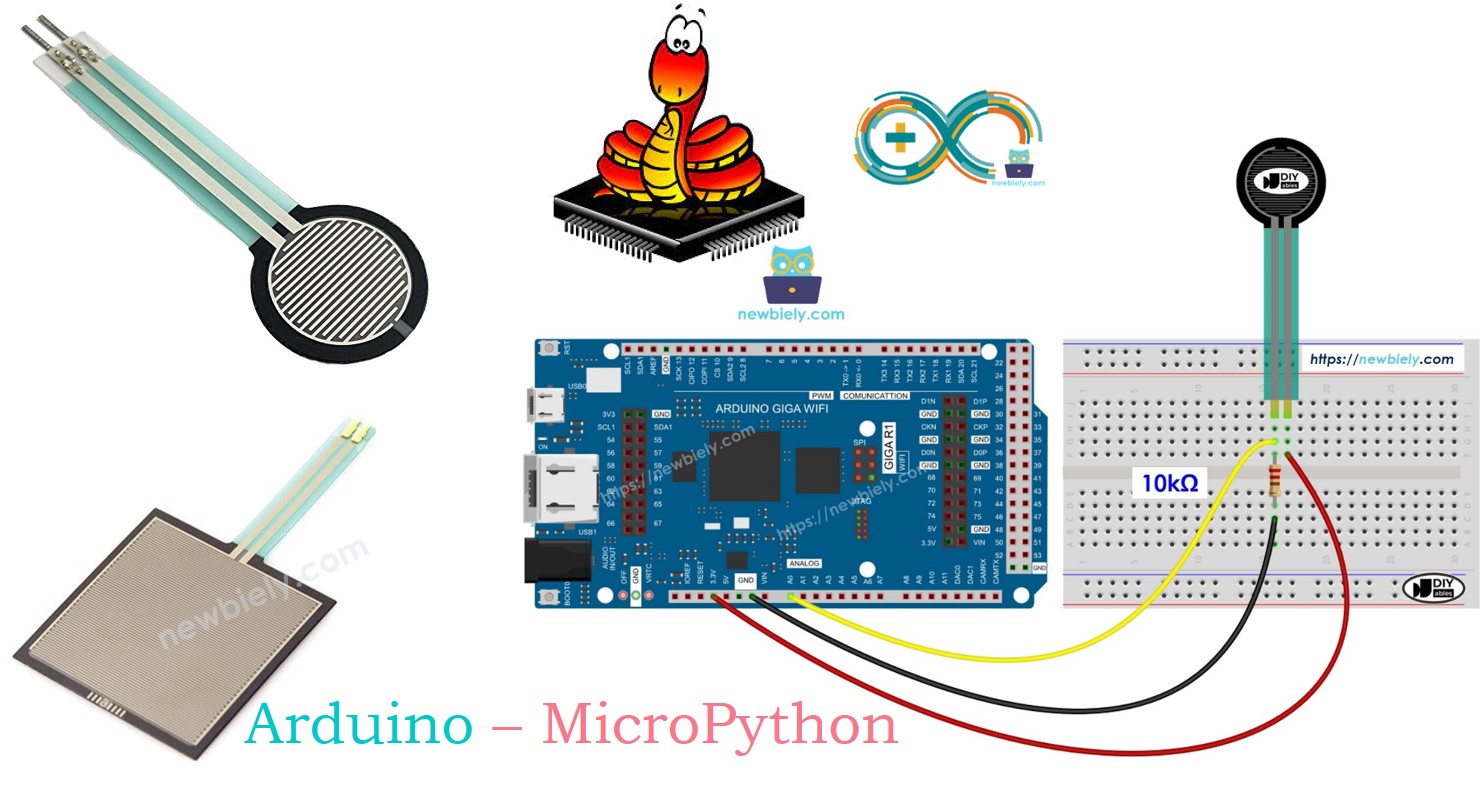
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Force Sensor
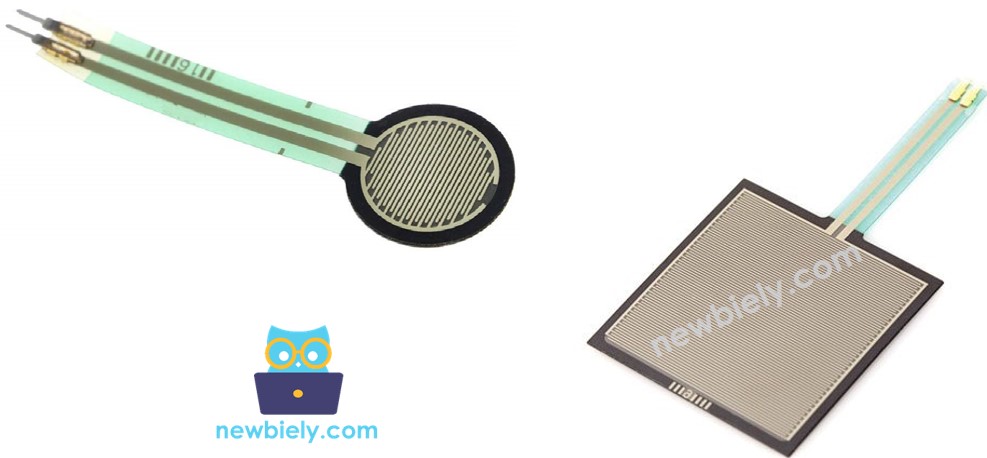
A force sensor, also known as a force-sensing resistor (FSR), changes its resistance when you apply pressure.
- Inexpensive and easy to use
- Good for detecting physical pressure or squeezing
- Not meant for measuring weight in pounds
You can find force sensors in electronic drums, mobile phones, handheld gaming devices, and many other small gadgets.
Pinout
A force sensor has only two wires. Because it works like a resistor, it doesn't matter which wire goes where. They're both the same.
How It Works
Think of the force sensor like a special button that changes its resistance based on how hard you press it. The harder you push, the closer the two ends get, resulting in lower resistance.
Wiring Diagram
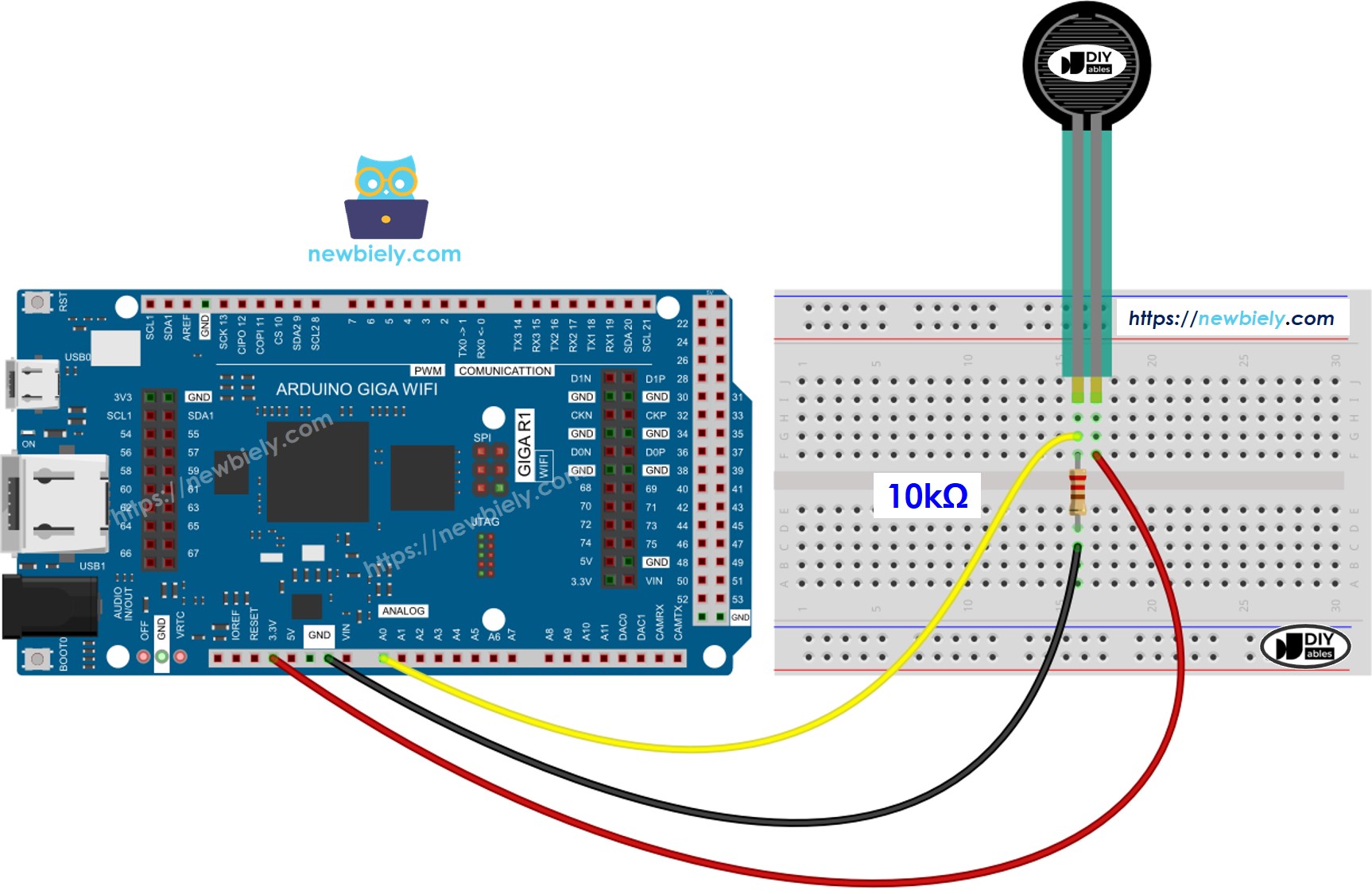
This image is created using Fritzing. Click to enlarge image
Arduino MicroPython Code for Force Sensor
Detailed Instructions
Here’s instructions on how to run the above MicroPython code on Arduino with Thonny IDE:
- Make sure Thonny IDE is installed on your computer.
- Make sure MicroPython firmware is installed on your Arduino board.
- If you are new to Arduino with MicroPython, see the Getting Started with Arduino and MicroPython.
- Connect the force sensor to the Arduino according to the provided diagram.
- Connect the Arduino board to your computer with a USB cable.
- Open Thonny IDE and go to Tools Options.
- Under the Interpreter tab, select MicroPython (generic) from the dropdown menu.
- Select the COM port corresponding to your Arduino board (e.g., COM33 on Windows or /dev/ttyACM0 on Linux).
- Copy the provided Arduino MicroPython code and paste it into Thonny's editor.
- Save the MicroPython code to your Arduino by:
- Clicking the Save button or pressing Ctrl+S.
- In the save dialog, choose MicroPython device and name the file main.py.
- Click the green Run button (or press F5) to execute the code.
- Press on the force sensor.
- Check out the message in the Shell at the bottom of Thonny.
Remember that the sensor's values can change slightly. It's a good idea to adjust the sensor's settings for each specific force level you want to measure.