Arduino MicroPython Button LED
This tutorial will guide you on how to control an LED with an Arduino and a button using MicroPython. We'll cover two different methods:
Method 1: Arduino controls the LED to match button status
Method 2: Arduino toggles the LED state on button press
Each time the button is pressed, the Arduino switches the LED between on and off.
The Arduino does not change the LED's state when the button is released.
For Method 2, we'll explain the importance of debouncing the button. Debouncing helps the Arduino prevent multiple unintended presses caused by contact bounce. We'll examine the LED's behavior in the MicroPython code, both with and without debouncing.
Or you can buy the following kits:
Disclosure: Some of the links provided in this section are Amazon affiliate links. We may receive a commission for any purchases made through these links at no additional cost to you.
Additionally, some of these links are for products from our own brand,
DIYables .
If you're just getting started with LED, Button, or MicroPython programming on the Arduino, we recommend exploring the following tutorials:
These tutorials will guide you through the essentials, from understanding LED and Button pinouts, how they work, to connecting these components to your Arduino and controlling them with MicroPython code.
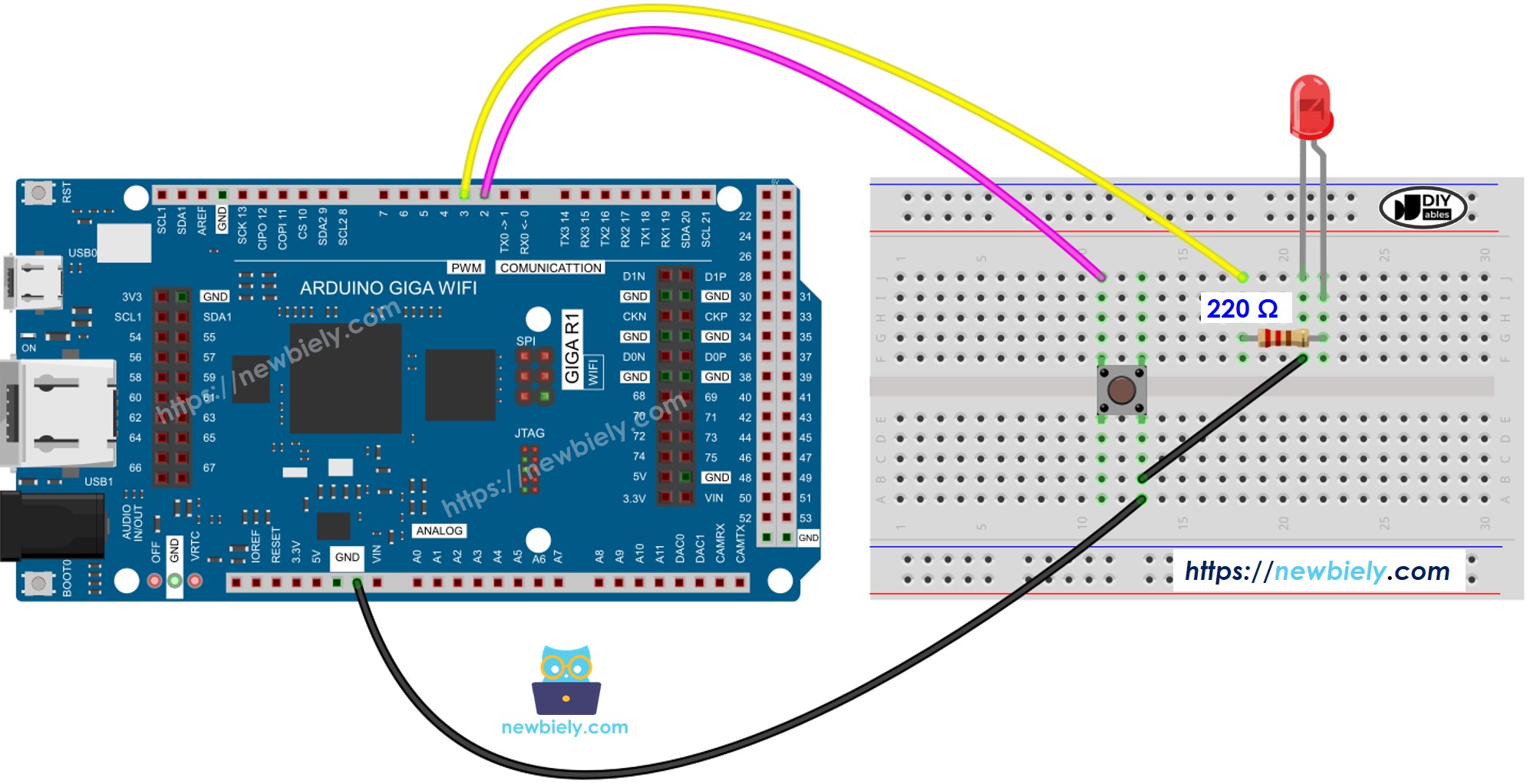
This image is created using Fritzing. Click to enlarge image
from machine import Pin
import time
BUTTON_PIN = 'D2'
LED_PIN = 'D3'
button = Pin(BUTTON_PIN, Pin.IN, Pin.PULL_UP)
led = Pin(LED_PIN, Pin.OUT)
while True:
button_state = button.value()
if button_state == 0:
print("The button is being pressed")
led.value(1)
else:
print("The button is unpressed")
led.value(0)
time.sleep(0.1)
Here’s instructions on how to run the above MicroPython code on Arduino with Thonny IDE:
Make sure Thonny IDE is installed on your computer.
Make sure MicroPython firmware is installed on your Arduino board.
Wire the components according to the provided diagram.
Connect the Arduino board to your computer with a USB cable.
Open Thonny IDE and go to Tools Options.
Under the Interpreter tab, select MicroPython (generic) from the dropdown menu.
Select the COM port corresponding to your Arduino board (e.g., COM33 on Windows or /dev/ttyACM0 on Linux).
Copy the provided Arduino MicroPython code and paste it into Thonny's editor.
Save the MicroPython code to your Arduino by:
Clicking the Save button or pressing Ctrl+S.
In the save dialog, choose MicroPython device and name the file main.py.
Click the green Run button (or press F5) to execute the code.
Press the button and hold it for a few seconds.
Check out the message in the Shell at the bottom of Thonny.
Observe the change in the LED's status.
The LED status matches the button status.
Read the detailed explanations in the source code's comments for each line!
from machine import Pin
import time
BUTTON_PIN = 'D2'
LED_PIN = 'D3'
button = Pin(BUTTON_PIN, Pin.IN, Pin.PULL_UP)
led = Pin(LED_PIN, Pin.OUT)
led_state = 0
button_state = button.value()
prev_button_state = button_state
while True:
prev_button_state = button_state
button_state = button.value()
if prev_button_state == 1 and button_state == 0:
print("The button is pressed")
led_state = not led_state
led.value(led_state)
time.sleep(0.1)
Copy the code and open it in Thonny IDE.
Save the code to the Arduino.
Click the green Run button (or press F5) to execute the code.
Press and release the button multiple times.
Observe how the LED's state changes.
Each time you press the button, the LED state changes once.
You can find the explanation in the comments of the above Arduino MicroPython code.
In the code, led_state = not led_state does the same thing as this code:
if led_state == 1:
led_state = 0
else:
led_state = 1