Arduino MicroPython Temperature Sensor LCD
This guide shows you how to use an Arduino and MicroPython code to read the temperature from a DS18B20 sensor and display it on an LCD I2C display.
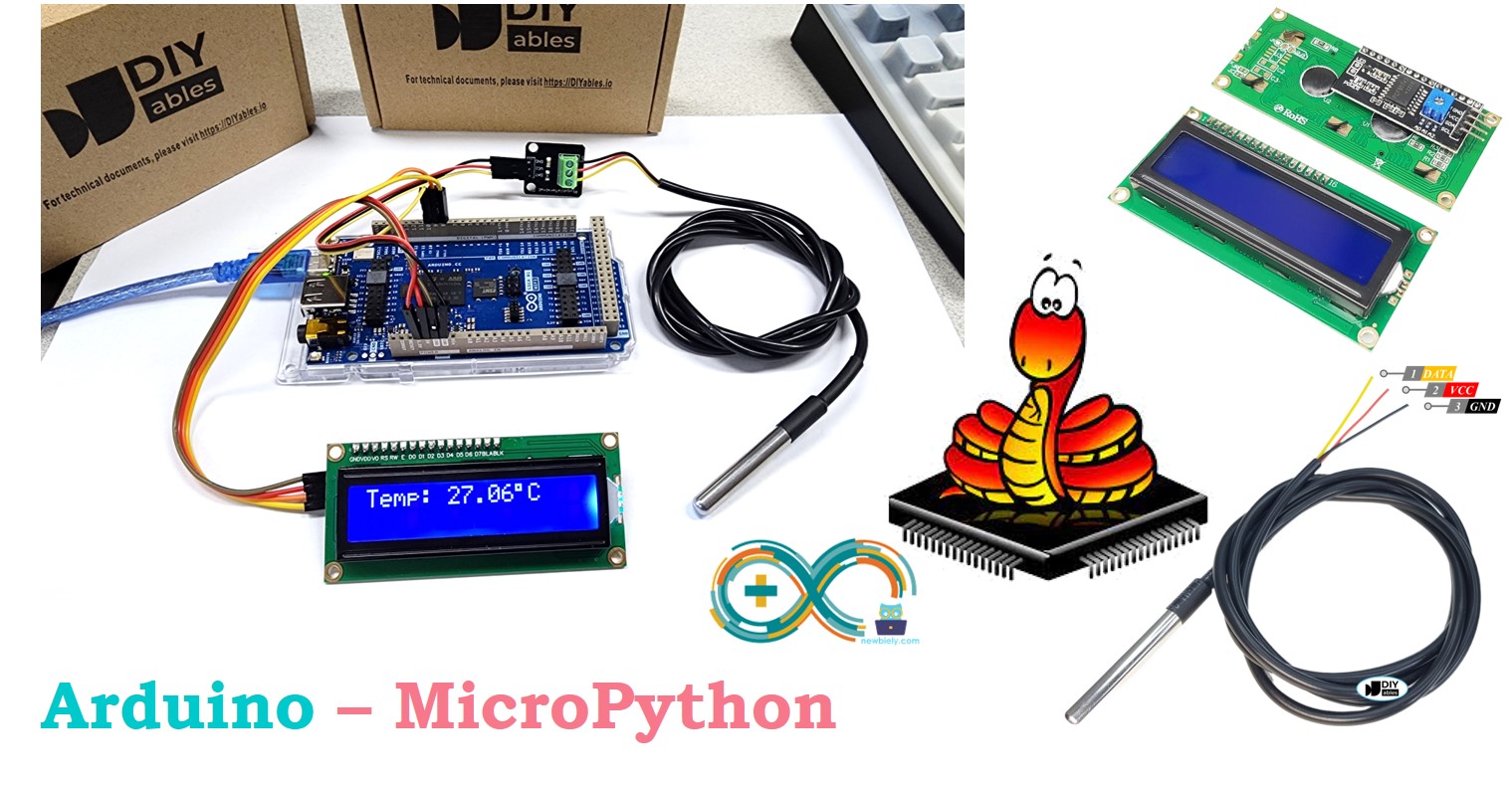
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Buy Note: Many DS18B20 sensors available in the market are unreliable. We strongly recommend buying the sensor from the DIYables brand using the link provided above. We tested it, and it worked reliably.
Overview of Temperature Sensor and LCD
If you're new to DS18B20 temperature sensor, LCD I2C, or MicroPython programming for the Arduino, I recommend checking out these tutorials:
- Arduino MicroPython Getting Started tutorial
These tutorials will provide you with a comprehensive understanding of DS18B20 temperature sensor and LCD, how to connect these components to the Arduino, and how to effectively control their behavior using MicroPython code.
Wiring Diagram
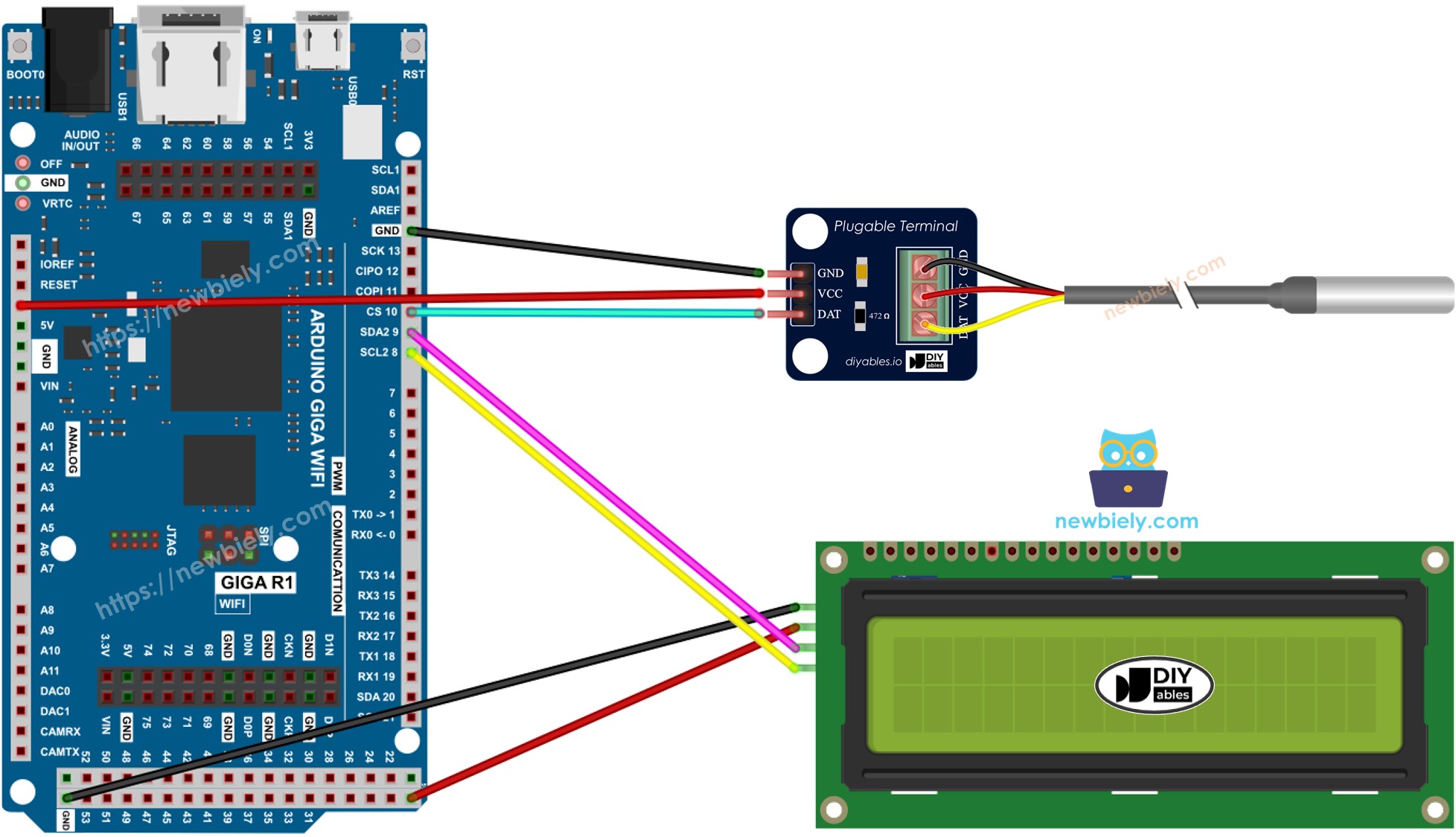
This image is created using Fritzing. Click to enlarge image
Arduino MicroPython Code
※ NOTE THAT:
Depending on the manufacturer, the I2C address for the LCD can vary. In our example, we used address 0x27 as specified by the manufacturer, DIYables.
Detailed Instructions
Here’s instructions on how to run the above MicroPython code on Arduino with Thonny IDE:
- Make sure Thonny IDE is installed on your computer.
- Make sure MicroPython firmware is installed on your Arduino board.
- If you are new to Arduino with MicroPython, see the Getting Started with Arduino and MicroPython.
- Connect the Arduino board to the DS18B20 temperature sensor and LCD I2C according to the provided diagram.
- Connect the Arduino board to your computer with a USB cable.
- Open Thonny IDE and go to Tools Options.
- Under the Interpreter tab, select MicroPython (generic) from the dropdown menu.
- Select the COM port corresponding to your Arduino board (e.g., COM33 on Windows or /dev/ttyACM0 on Linux).
- Navigate to the Tools Manage packages on the Thonny IDE.
- Search “DIYables-MicroPython-LCD-I2C”, then find the LCD I2C library created by DIYables.
- Click on DIYables-MicroPython-LCD-I2C, then click Install button to install LCD I2C library.
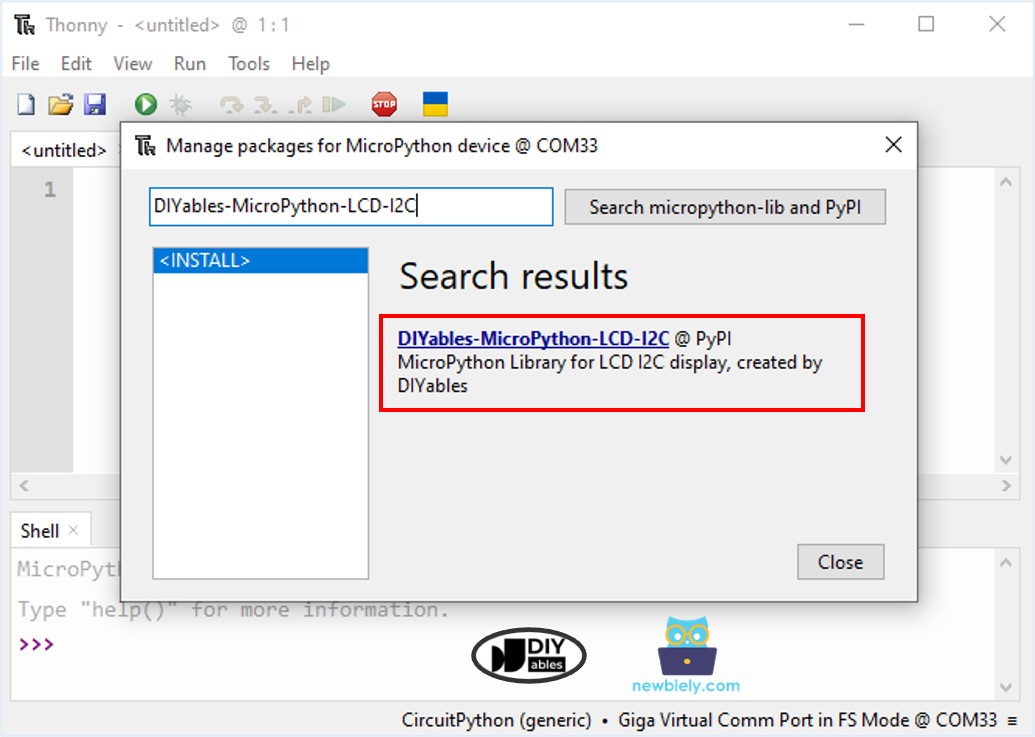
- Search “DIYables-MicroPython-DS18X20”, then find the DS18X20 library created by DIYables.
- Click on DIYables-MicroPython-DS18X20, then click Install button to install DS18X20 library.
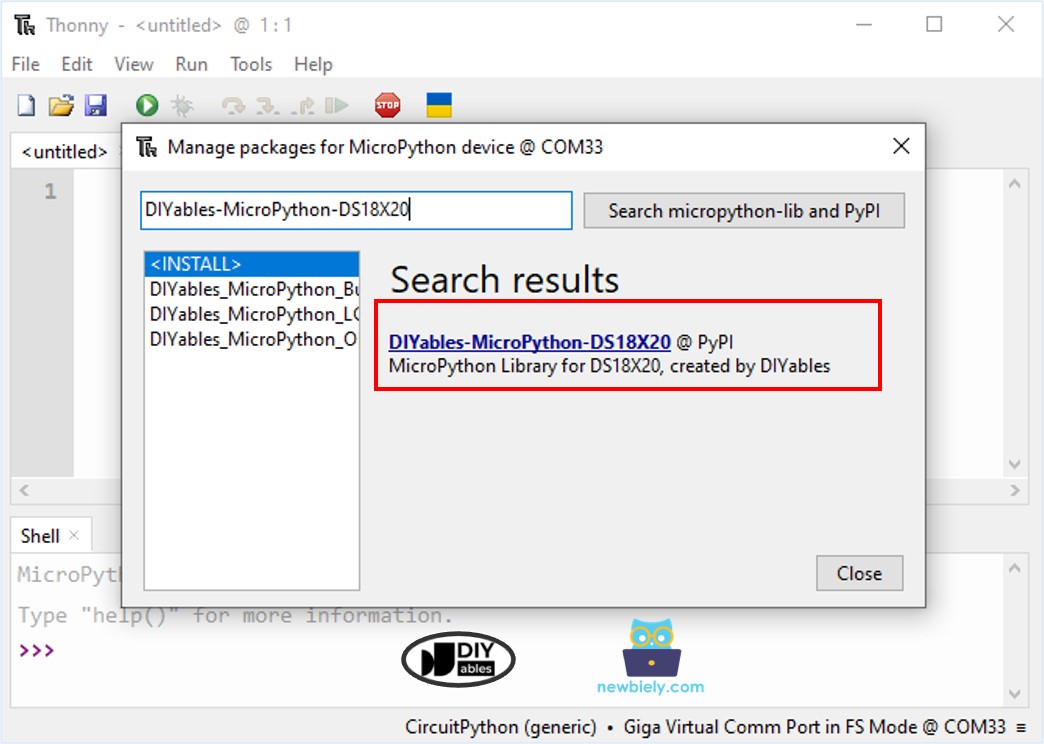
- Copy the provided Arduino MicroPython code and paste it into Thonny's editor.
- Save the MicroPython code to your Arduino by:
- Clicking the Save button or pressing Ctrl+S.
- In the save dialog, choose MicroPython device and name the file main.py.
- Click the green Run button (or press F5) to execute the code.
- Put the sensor on hot or cold water, or hold it in your hand.
- Look at the display on the LCD screen. It looks like the below image:
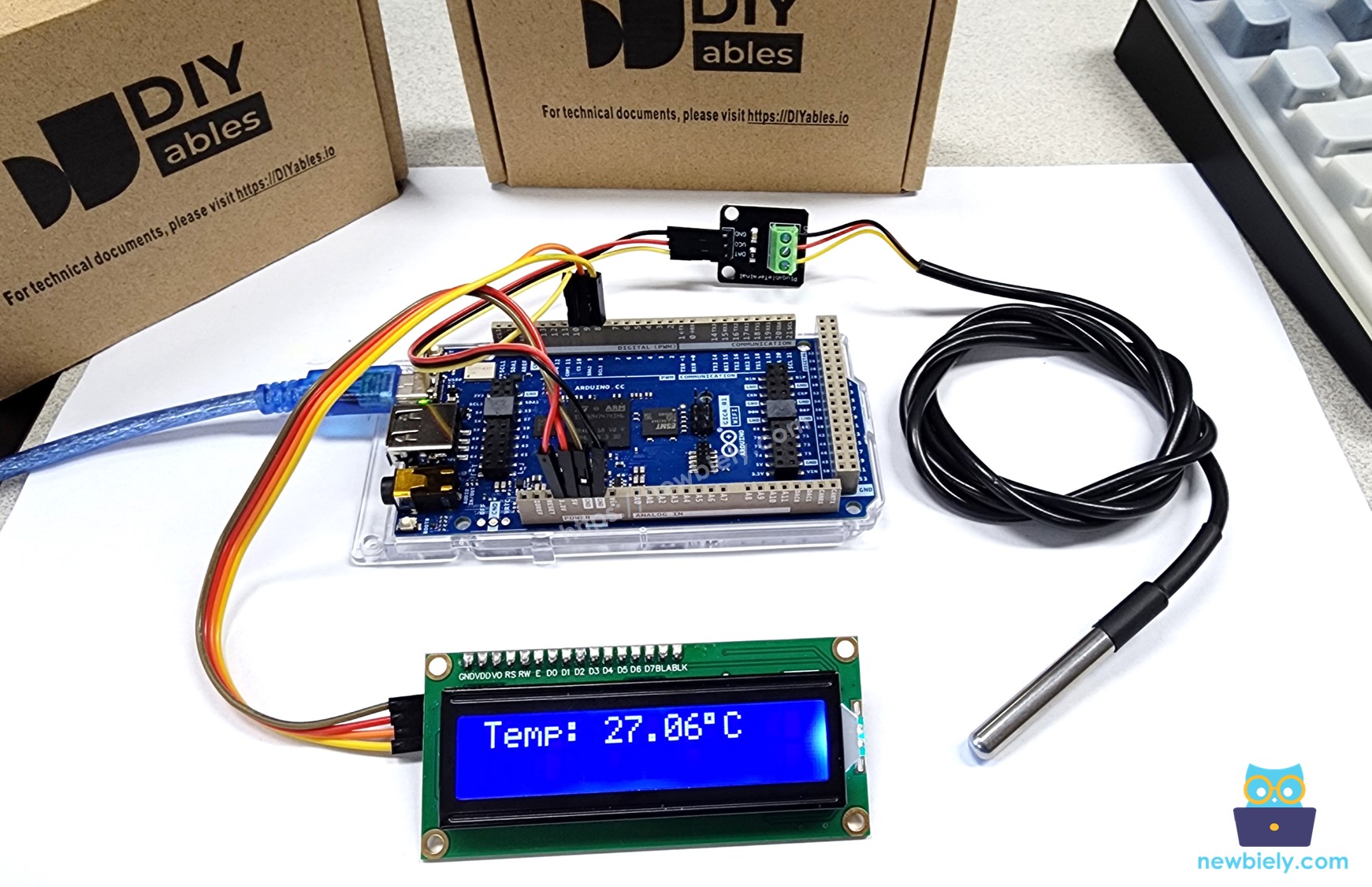
If the LCD screen is blank, please visit Troubleshooting for LCD I2C for help.
Code Explanation
Look at the comments in the source code for explanations of each line!