ESP32 MicroPython Light Sensor
This guide will teach you how to use an LDR light sensor (also known as a photoresistor, light-dependent resistor, photocell) with an ESP32 microcontroller and MicroPython. We'll cover the following:
- How LDR light sensors work
- Connecting an LDR sensor to an ESP32
- Writing MicroPython code for ESP32 to read from the LDR sensor
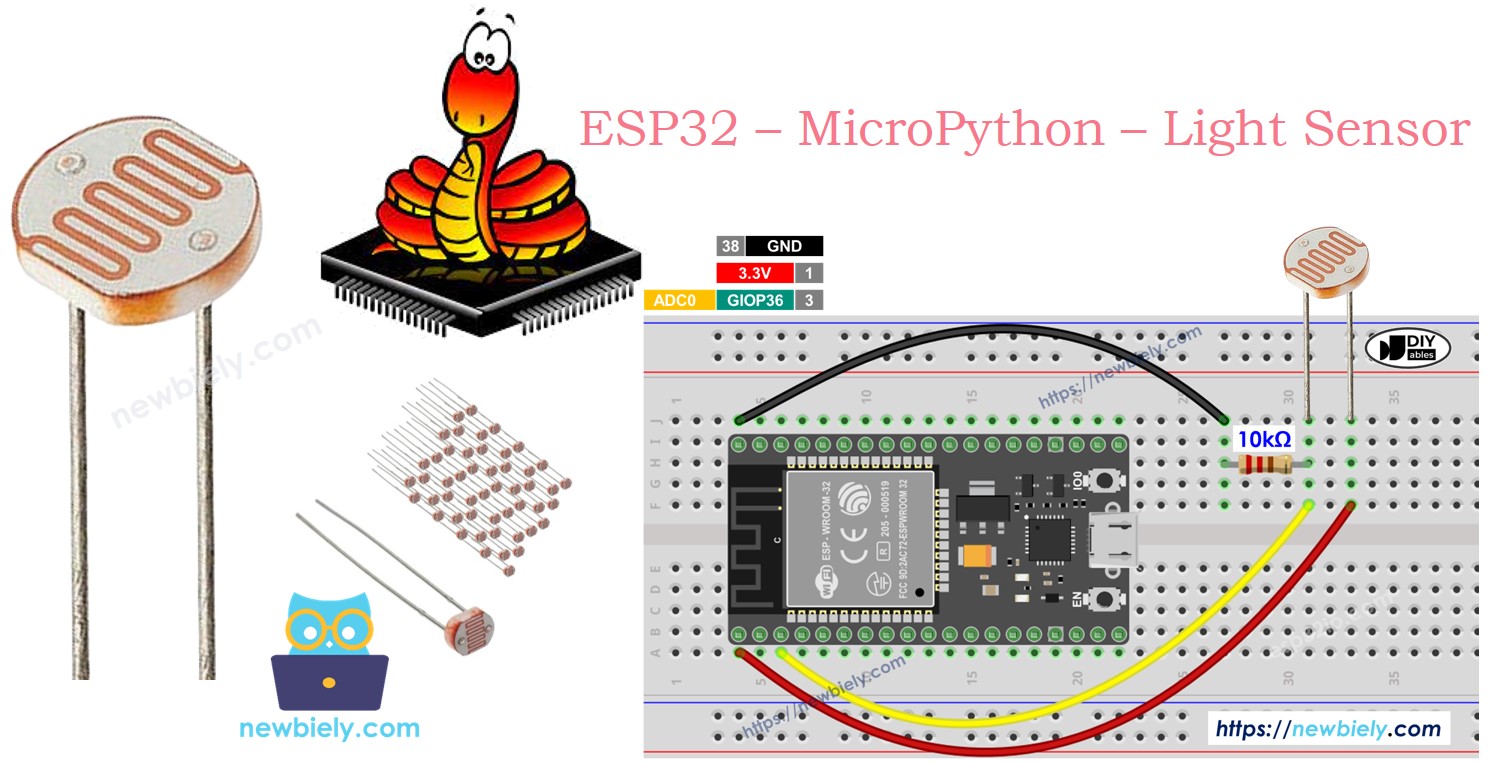
If you are looking for a module with a light sensor, check the tutorial ESP32 MicroPython LDR Light Sensor Module.
Hardware Preparation
1 | × | ESP-WROOM-32 Dev Module | |
1 | × | USB Cable Type-C | |
1 | × | Light Sensor | |
1 | × | 10 kΩ resistor | |
1 | × | Breadboard | |
1 | × | Jumper Wires | |
1 | × | (Recommended) Screw Terminal Expansion Board for ESP32 |
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables.
Overview of Light Sensor
This guide explains how to use a photoresistor, also known as a Light-Dependent Resistor (LDR), to measure the brightness of surrounding light.
Pinout
A photoresistor has two pins. Since it is a resistor, you do not need to distinguish these pins. They are identical.
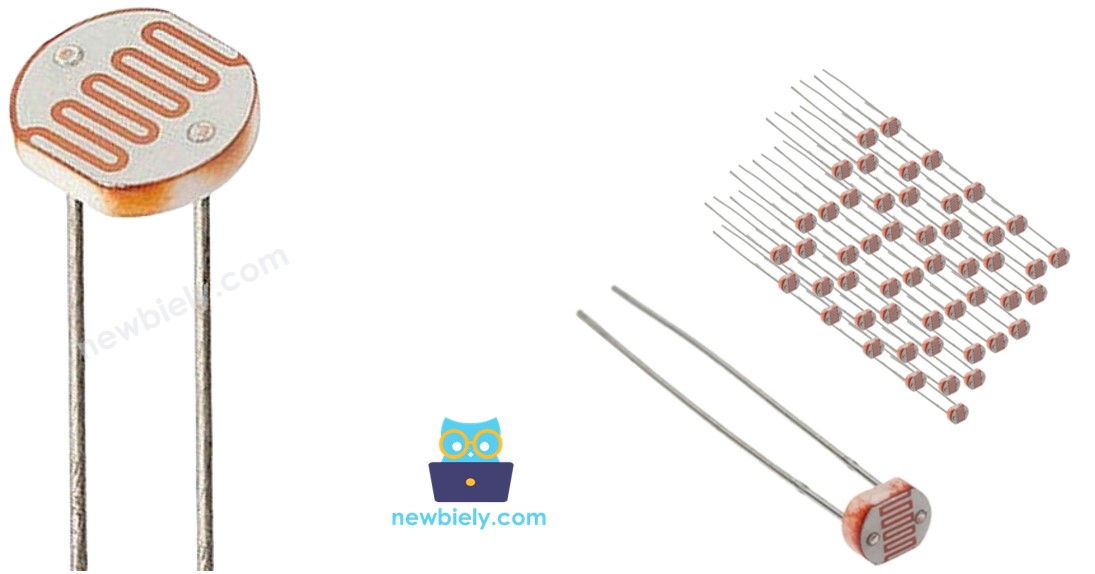
How It Works
A photoresistor is a unique resistor that changes its resistance in response to light. Its resistance decreases in bright light and increases in darkness or no light. By measuring the photoresistor's resistance, we can determine the brightness or darkness of the surrounding light, making it useful for measuring light levels in different environments.
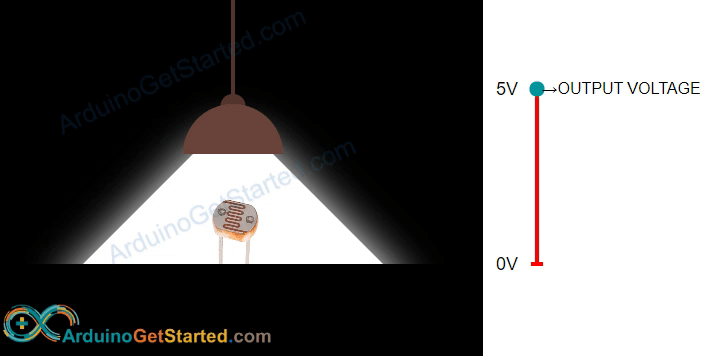
WARNING
The light sensor value provides a general indication of light brightness, but it's not a precise measurement. Use it when exact measurements are not required.
Wiring Diagram
How to connect ESP32 and light sensor
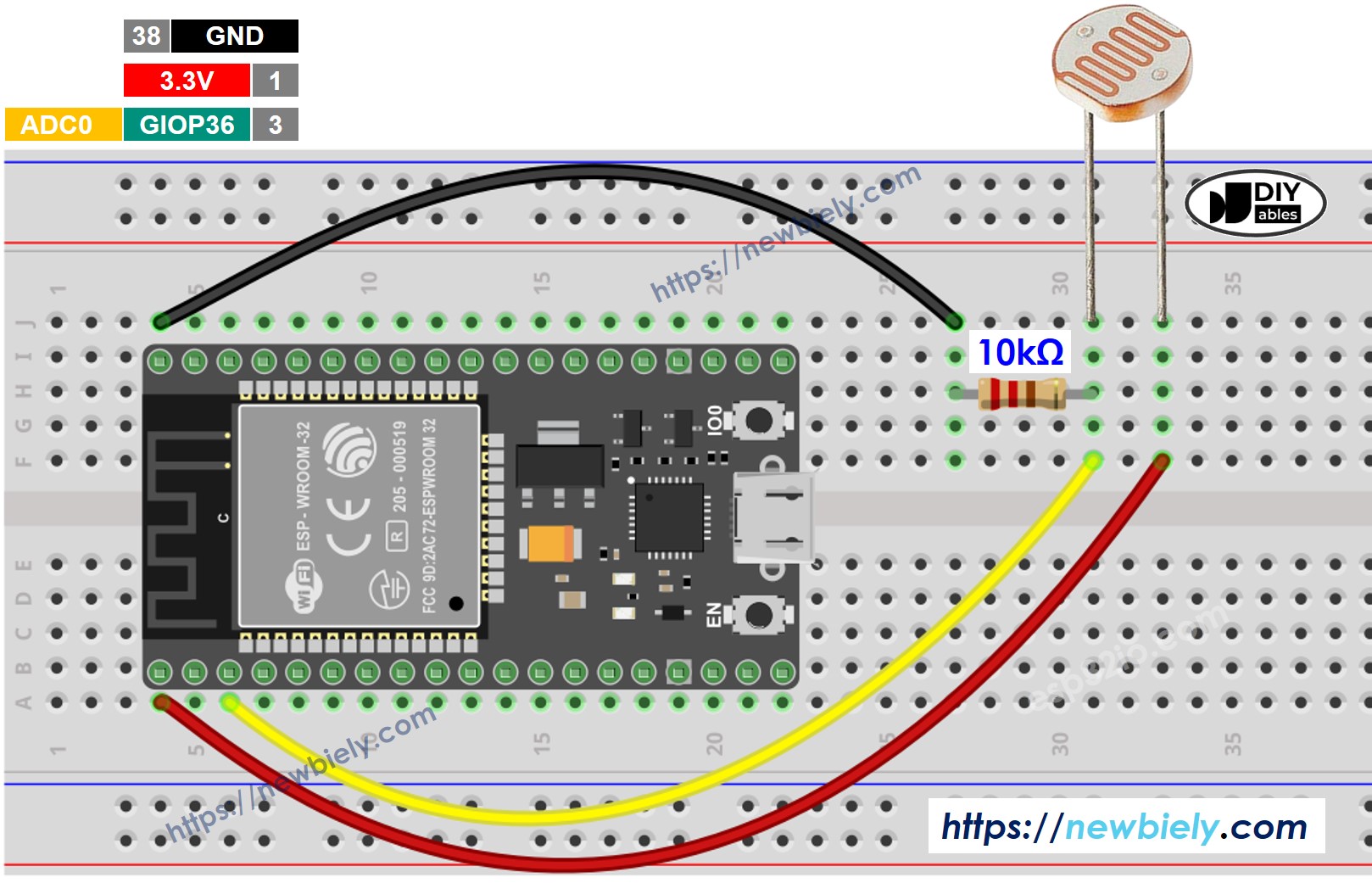
This image is created using Fritzing. Click to enlarge image
ESP32 MicroPython Code
This code checks the light amount using a photocell and describes how bright it is.
Detailed Instructions
Here’s instructions on how to set up and run your MicroPython code on the ESP32 using Thonny IDE:
- Make sure Thonny IDE is installed on your computer.
- Confirm that MicroPython firmware is loaded on your ESP32 board.
- If this is your first time using an ESP32 with MicroPython, check out the ESP32 MicroPython Getting Started guide for step-by-step instructions.
- Connect the LDR light sensor to the ESP32 according to the provided diagram.
- Connect the ESP32 board to your computer with a USB cable.
- Open Thonny IDE on your computer.
- In Thonny IDE, go to Tools Options.
- Under the Interpreter tab, choose MicroPython (ESP32) from the dropdown menu.
- Make sure the correct port is selected. Thonny IDE usually detects it automatically, but you might need to select it manually (like COM12 on Windows or /dev/ttyACM0 on Linux).
- Copy the provided MicroPython code and paste it into Thonny\'s editor.
- Save the code to your ESP32 by:
- Clicking the Save button or pressing Ctrl+S.
- In the save dialog, choose MicroPython device.
- Name the file main.py.
- Click the green Run button (or press F5) to execute the script.
- Direct light onto the sensor.
- Check out the message in the Shell at the bottom of Thonny.
※ NOTE THAT:
This tutorial demonstrates how to use the adc.read() function to read values from an ADC (Analog-to-Digital Converter) connected to a light sensor. The ESP32's ADC is suitable for projects that do not require high precision. However, if your project needs accurate measurements, keep the following in mind:
- The ESP32 ADC is not perfectly accurate and may require calibration for precise results. Each ESP32 board may vary slightly, so calibration is necessary for each individual board.
- Calibration can be challenging, especially for beginners, and might not always yield the exact results you desire.
For projects requiring high precision, consider using an external ADC (e.g., ADS1115) with the ESP32 or opt for an Arduino, which has a more reliable ADC. If you still wish to calibrate the ESP32 ADC, refer to the ESP32 ADC Calibration Driver.