ESP32 MicroPython Joystick
This tutorial instructs you how to use a joystick with ESP32 and MicroPython. In detail, we will learn:
- How a joystick work
- How to connect a joystick to a ESP32
- How to write MicroPython code for ESP32 to read values from Joystick
- How to write MicroPython code for ESP32 to translate joystick's data into useful data (like XY coordinates or motor directions)
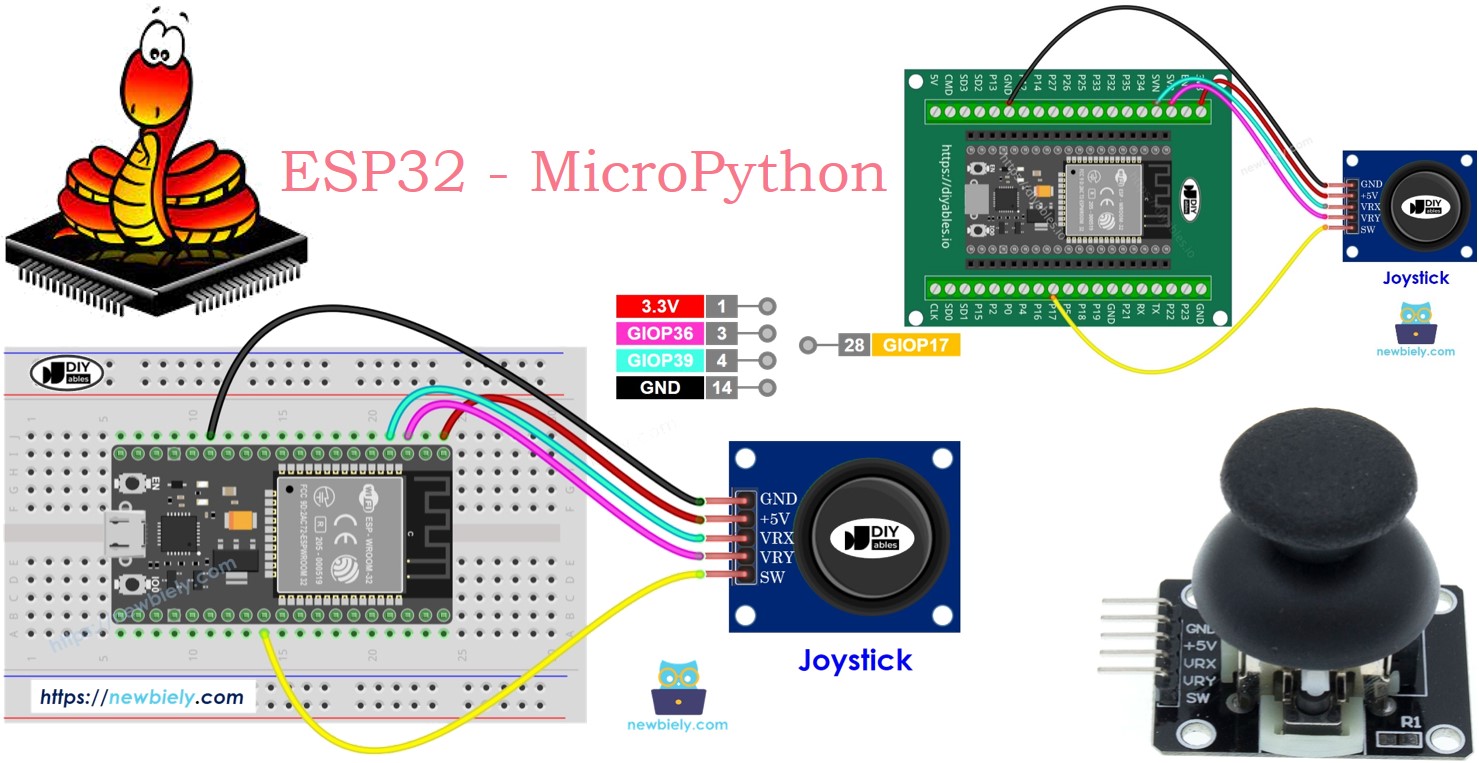
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Joystick
You might have seen a joystick used to control games, toys, or even large machines like excavators. The joystick consists of two potentiometers arranged in a square pattern and a button. It provides the following outputs:
- A range of 0 to 4095 for the horizontal (left-right) position.
- A range of 0 to 4095 for the vertical (up-down) position.
- The button's status, either ON (HIGH) or OFF (LOW).
By combining the two analog values, the joystick generates 2-D coordinates that are centered when the joystick is at rest. You can determine the actual direction of these coordinates using a test code, which we'll cover in the next section. Some applications may utilize all three outputs, while others may only use a few.
Pinout
A joystick has five pins:
- GND pin: Connects to ground (0V).
- VCC pin: Connects to the 3.3V supply of the ESP32.
- VRX pin: Provides an analog value for the horizontal position (X-coordinate); connect this pin to an ADC pin on the ESP32.
- VRY pin: Provides an analog value for the vertical position (Y-coordinate); connect this pin to an ADC pin on the ESP32.
- SW pin: Connected to the joystick's pushbutton, which is normally open. Use a pull-up resistor to keep this pin HIGH when the button is not pressed and LOW when it is pressed.
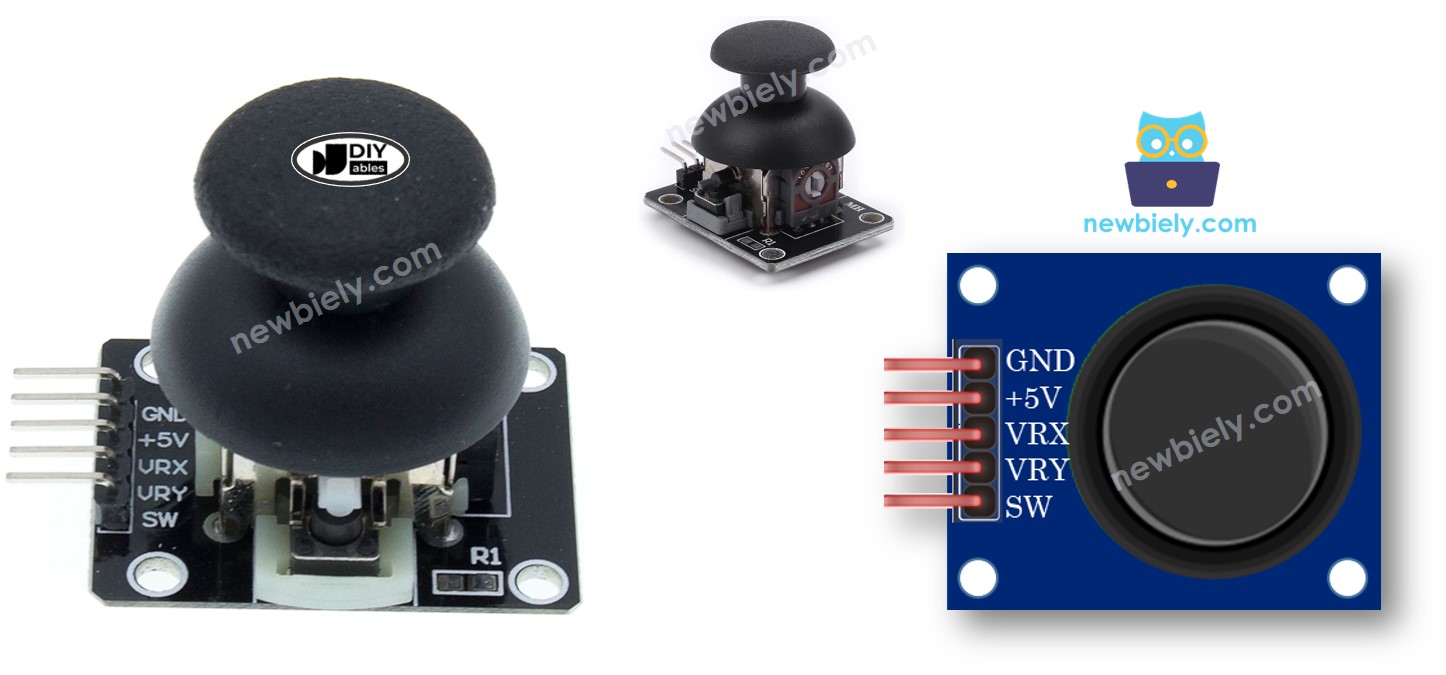
How It Works
- Left/Right Movement:
- Moving the joystick left or right changes the voltage at the VRX pin.
- Moving it left reduces the voltage to 0 volts, while moving it right increases the voltage to 3.3 volts.
- The ESP32 measures this voltage and converts it to a number between 0 and 4095, where 0V corresponds to 0, and 3.3V corresponds to 4095.
- Up/Down Movement:
- Moving the joystick up or down affects the voltage at the VRY pin.
- Moving it up decreases the voltage to 0 volts, and moving it down raises the voltage to 3.3 volts.
- The ESP32 reads this voltage and converts it to a number from 0 to 4095, similar to the VRX pin.
- Combined Movements:
- Moving the joystick in any direction changes the voltage at both the VRX and VRY pins, depending on its position along each axis.
- Pressing the Joystick:
- Pressing the joystick down activates an internal button.
- A pull-up resistor connected to the SW pin causes the voltage at this pin to drop from 3.3V to 0V when pressed.
- The ESP32 interprets this as a digital signal, reading HIGH (3.3V) when not pressed and LOW (0V) when pressed.
When the joystick is moved, it adjusts the voltages at the VRX and VRY pins, which the ESP32 reads as values from 0 to 4095. Pressing the joystick changes the voltage at the SW pin, and the ESP32 detects this change as either HIGH or LOW.
Wiring Diagram
- How to connect ESP32 and joystick using breadboard
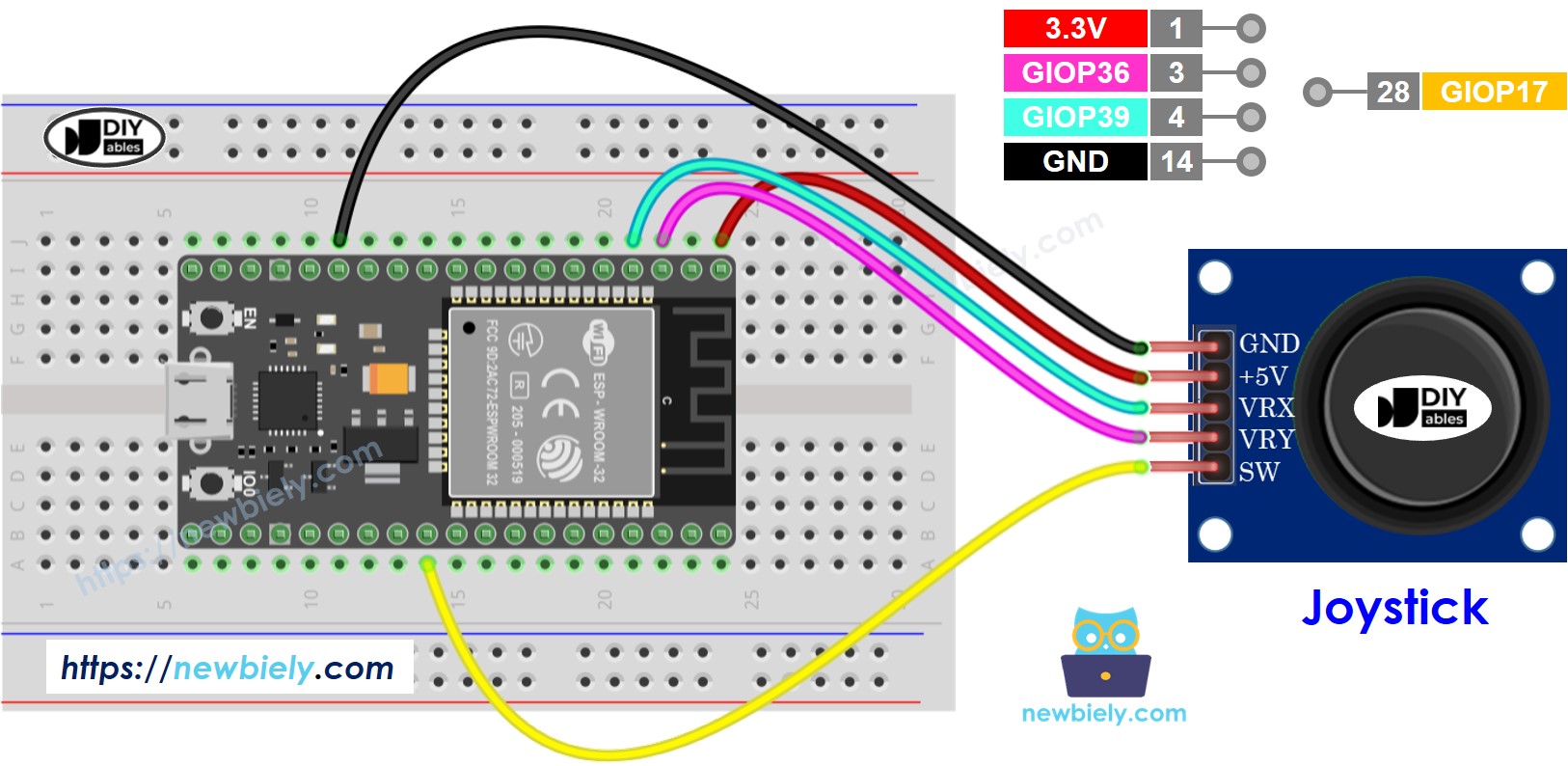
This image is created using Fritzing. Click to enlarge image
- How to connect ESP32 and joystick using screw terminal block breakout board
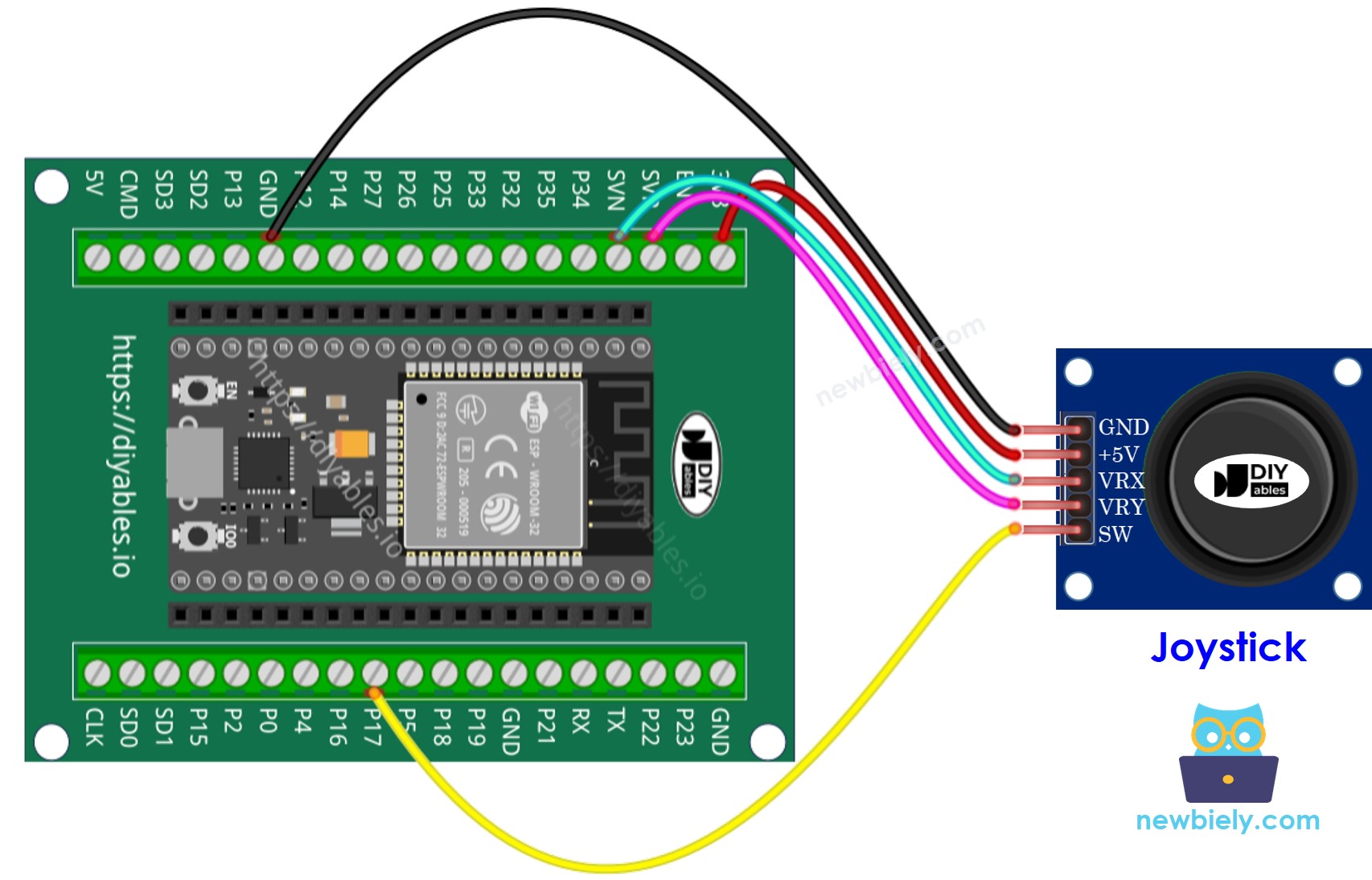
How To Program For Joystick
Fortunately, DIYables has developed a joystick library that greatly simplifies the process of using a joystick with the ESP32.
ESP32 MicroPython Code - Reads Joystick's state
The following MicroPython script:
- Reads analog values from a joystick.
- Checks if the joystick's thumb is pressed or released.
- Reads the joystick's thumb press count.
Detailed Instructions
Here’s instructions on how to set up and run your MicroPython code on the ESP32 using Thonny IDE:
- Make sure Thonny IDE is installed on your computer.
- Confirm that MicroPython firmware is loaded on your ESP32 board.
- If this is your first time using an ESP32 with MicroPython, check out the ESP32 MicroPython Getting Started guide for step-by-step instructions.
- Connect the joystick to the ESP32 according to the provided diagram.
- Connect the ESP32 board to your computer with a USB cable.
- Open Thonny IDE on your computer.
- In Thonny IDE, go to Tools Options.
- Under the Interpreter tab, choose MicroPython (ESP32) from the dropdown menu.
- Make sure the correct port is selected. Thonny IDE usually detects it automatically, but you might need to select it manually (like COM12 on Windows or /dev/ttyACM0 on Linux).
- Navigate to the Tools Manage packages on the Thonny IDE.
- Search “DIYables-MicroPython-Joystick”, then find the Joystick library created by DIYables.
- Click on DIYables-MicroPython-Joystick, then click Install button to install Joystick library.
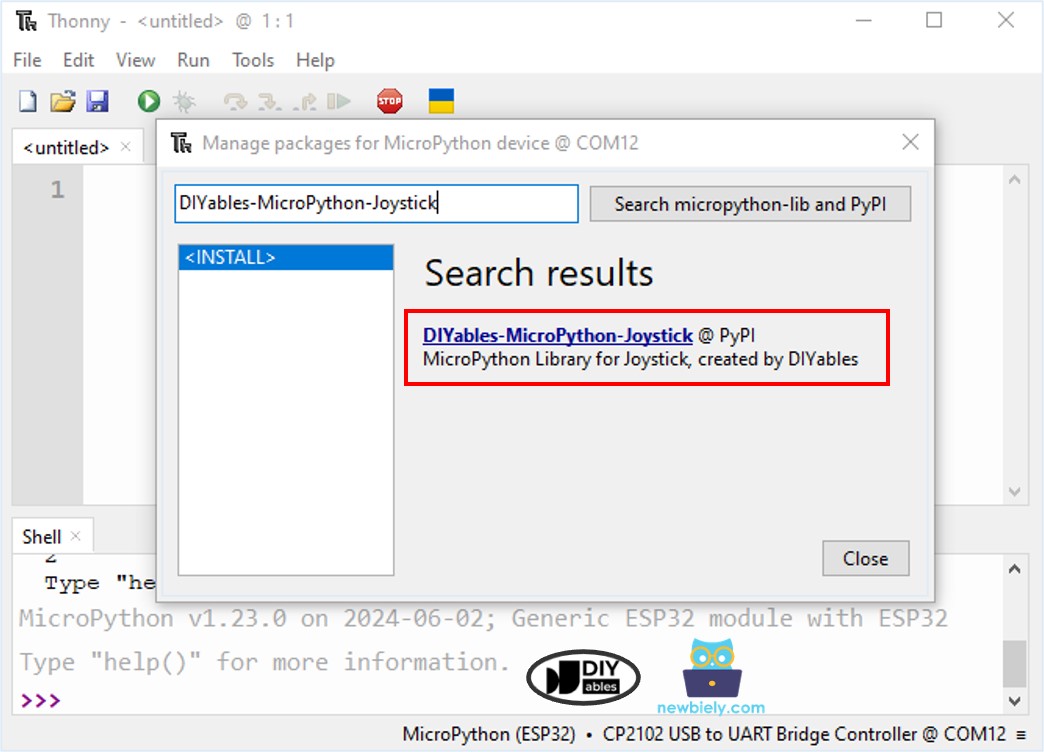
- Copy the provided MicroPython code and paste it into Thonny's editor.
- Save the code to your ESP32 by:
- Clicking the Save button or pressing Ctrl+S.
- In the save dialog, choose MicroPython device.
- Name the file main.py.
- Click the green Run button (or press F5) to execute the script.
- Slide the joystick left, right, up, or down.
- Push down on the top of the joystick.
- Check out the message in the Shell at the bottom of Thonny.
When using the joystick, observe the message in the Shell at the bottom of Thonny. If the X value is 0, it indicates a left position; otherwise, it indicates right. If the Y value is 0, it represents an upward position; otherwise, it indicates downward.
Keep in mind that although the theoretical range for X/Y values is from 0 to 4095, in practice, the values may not reach these extremes due to the joystick's mechanical characteristics. This variation is normal and acceptable for most applications.
※ NOTE THAT:
This tutorial demonstrates how to use the adc.read() function to read values from an ADC (Analog-to-Digital Converter) connected to a joystick. The ESP32's ADC is suitable for projects that do not require high precision. However, if your project needs accurate measurements, keep the following in mind:
- The ESP32 ADC is not perfectly accurate and may require calibration for precise results. Each ESP32 board may vary slightly, so calibration is necessary for each individual board.
- Calibration can be challenging, especially for beginners, and might not always yield the exact results you desire.
For projects requiring high precision, consider using an external ADC (e.g., ADS1115) with the ESP32 or opt for an Arduino, which has a more reliable ADC. If you still wish to calibrate the ESP32 ADC, refer to the ESP32 ADC Calibration Driver.
Converts analog value to MOVE LEFT/RIGHT/UP/DOWN commands
The below MicroPython code converts analog values into commands: MOVE_LEFT, MOVE_RIGHT, MOVE_UP, MOVE_DOWN.
Detailed Instructions
- Copy the above code and paste it into the editor of Thonny IDE.
- Save the script to your ESP32 board.
- Click the green Run button (or press F5) to execute the script.
- Move the joystick left, right, up, down, or in any direction.
- Check out the message in the Shell at the bottom of Thonny.
※ NOTE THAT:
There might be no command, one command, or even two commands at the same time, like UP and LEFT together.
Converts analog values to angles to control two servo motors
Learn detail in this ESP32 MicroPython Joystick Controls Servo Motor tutorial.