ESP32 MicroPython Blink multiple LED
This guide shows you how to set up and write MicroPython code for an ESP32 to blink multiple LEDs at different speeds without using the time.sleep() function. It offers the instructions in two different ways:
- ESP32 makes multiple LEDs blink.
- ESP32 uses a array to blink multiple LEDs.
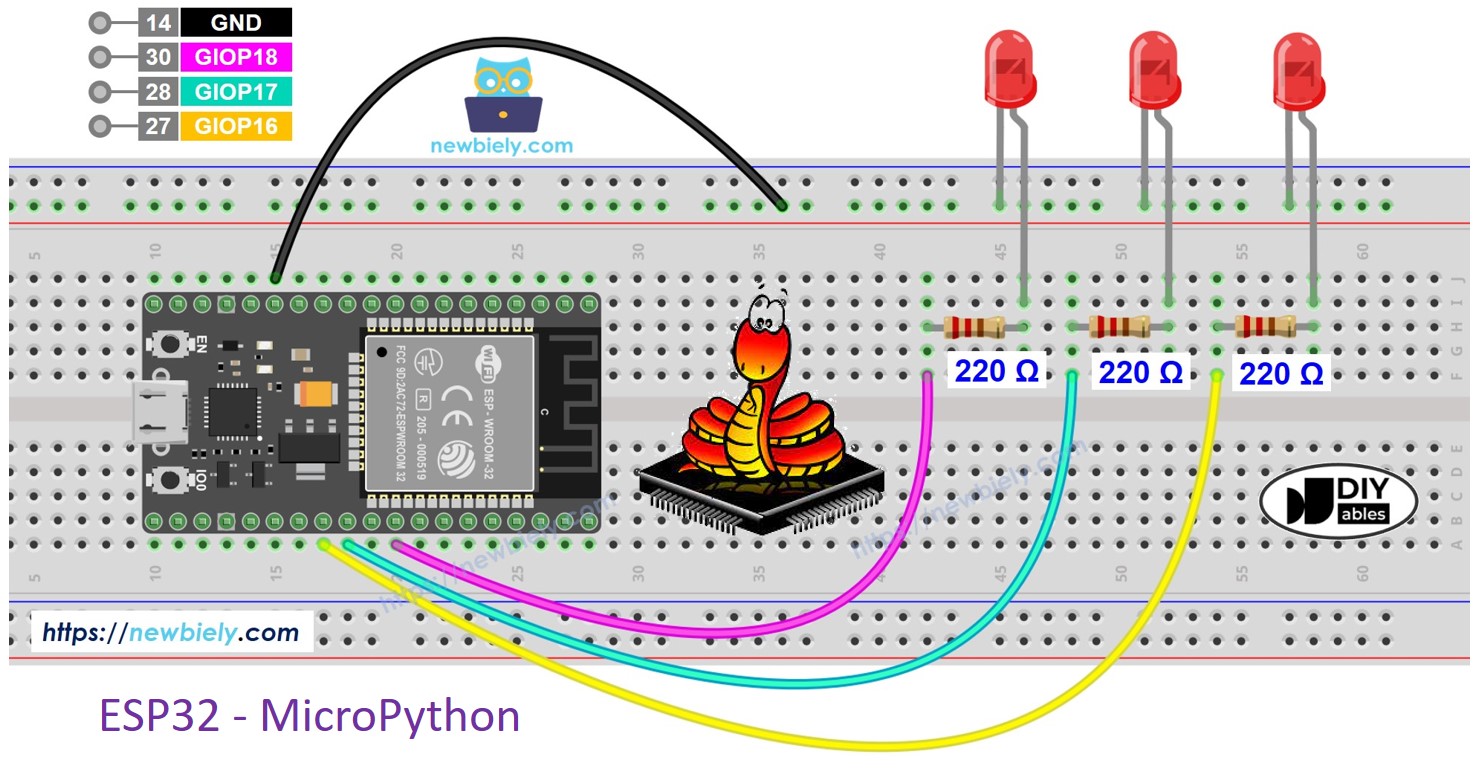
We'll explain how to do this with three LEDs. You can easily adjust it for two, four, or more LEDs.
Hardware Preparation
1 | × | ESP-WROOM-32 Dev Module | |
1 | × | USB Cable Type-C | |
1 | × | LED | |
1 | × | 220 ohm resistor | |
1 | × | Breadboard | |
1 | × | Jumper Wires | |
1 | × | (Recommended) Screw Terminal Expansion Board for ESP32 |
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables.
Overview of LED
If you're not yet familiar with LEDs (including their pinout, how it works, interfacing with the ESP32, and writing MicroPython code for the ESP32 to interact with them), you can learn more at:
Wiring Diagram
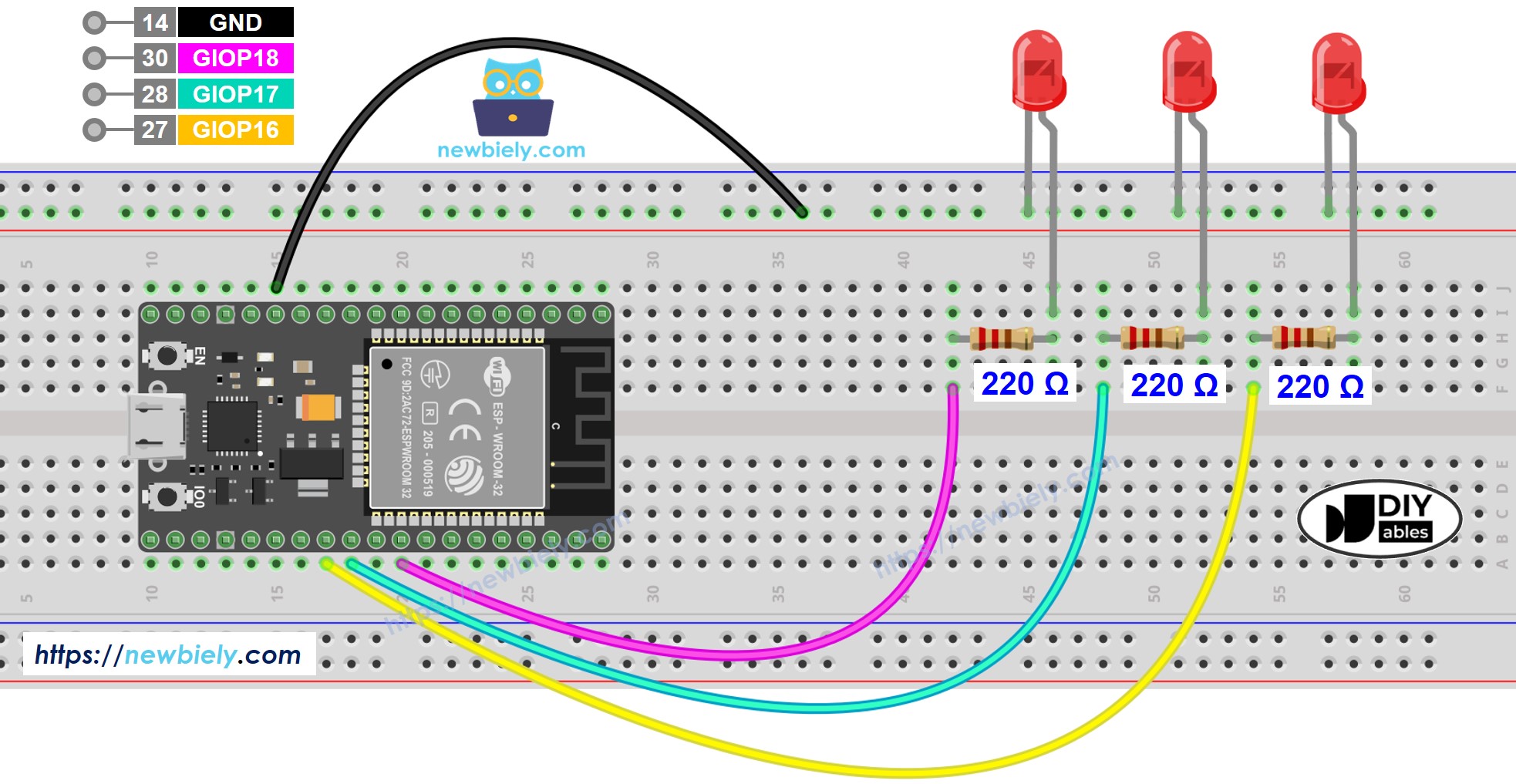
This image is created using Fritzing. Click to enlarge image
ESP32 MicroPython Code - Blink Multiple LEDs
To make several LEDs blink at the same time, don't use the time.sleep() function because it blocks other code from working. Use the timestamp to handle the timing instead.
If you are just starting with programming and struggle with controlling the timing for blinking several LEDs, the DIYables_MicroPython_LED library makes it easier by handling the timing for you. This means you won't have to worry about timing problems when using the library.
Detailed Instructions
Here’s instructions on how to set up and run your MicroPython code on the ESP32 using Thonny IDE:
- Make sure Thonny IDE is installed on your computer.
- Confirm that MicroPython firmware is loaded on your ESP32 board.
- If this is your first time using an ESP32 with MicroPython, check out the ESP32 MicroPython Getting Started guide for step-by-step instructions.
- Connect the ESP32 board to the LEDs as shown in the diagram.
- Connect the ESP32 board to your computer with a USB cable.
- Open the Thonny IDE on your computer.
- In Thonny IDE, go to Tools Options.
- Under the Interpreter tab, choose MicroPython (ESP32) from the dropdown menu.
- Make sure the correct port is selected. Thonny IDE usually detects it automatically, but you might need to select it manually (like COM12 on Windows or /dev/ttyACM0 on Linux).
- In Thonny IDE, Go to Tools Manage packages.
- Search for “DIYables-MicroPython-LED” and find the LED library by DIYables.
- Click on DIYables-MicroPython-LED and then click the Install button to install the LED library on the ESP32 board.
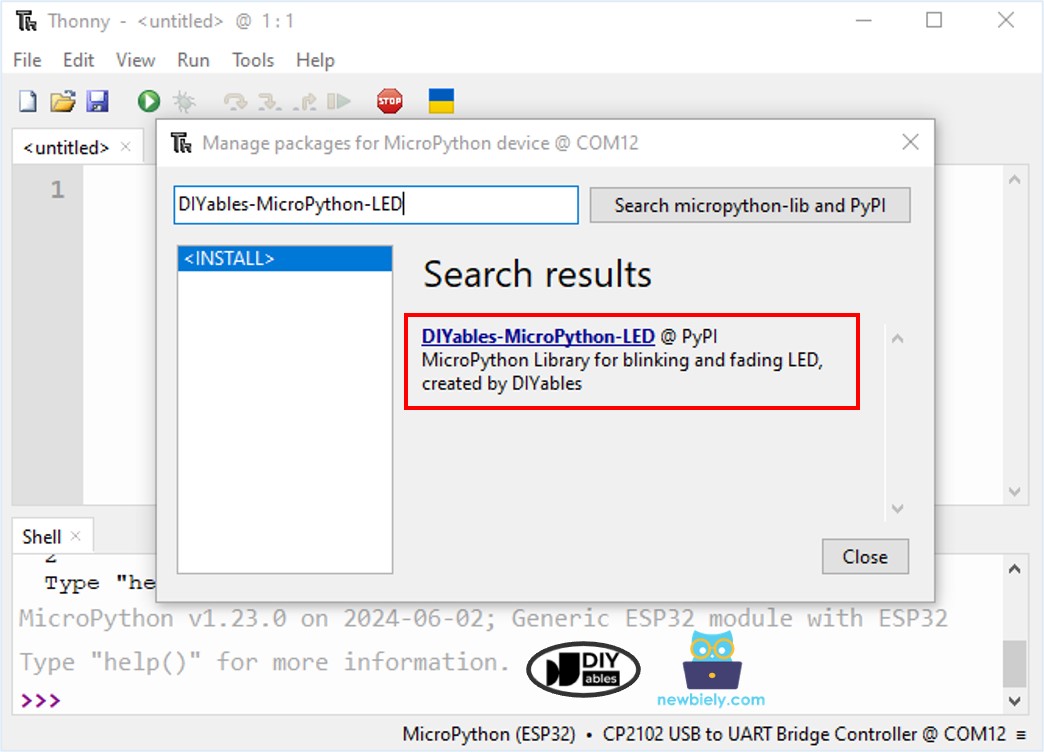
- Copy the provided MicroPython code and paste it into Thonny's editor.
- Save the code to your ESP32 by:
- Clicking the Save button or pressing Ctrl+S.
- In the save dialog, choose MicroPython device.
- Name the file main.py.
- Click the green Run button (or press F5) to execute the script.
- Check whether the LEDs are on or off.
ESP32 MicroPython Code - Blink Multiple LEDs by using array
We can make the earlier code better by using a array of LEDs. The code below uses this array to manage LED objects.
With the DIYables_MicroPython_LED library, you can quickly get several LEDs to blink in this way:
- Make several LEDs blink at different speeds.
- Make several LEDs start blinking at different times.
- Make each LED to blink for a certain amount of time.
- Make each LED to blink a certain number of times.