ESP32 MicroPython Sound Sensor
This guide will teach us how to use a sound sensor and the ESP32 with MicroPython to detect sounds. In detail, we will learn:
- How to connect a sound sensor to a ESP32
- How to write MicroPython code for a ESP32 to detect sound using a sound sensor
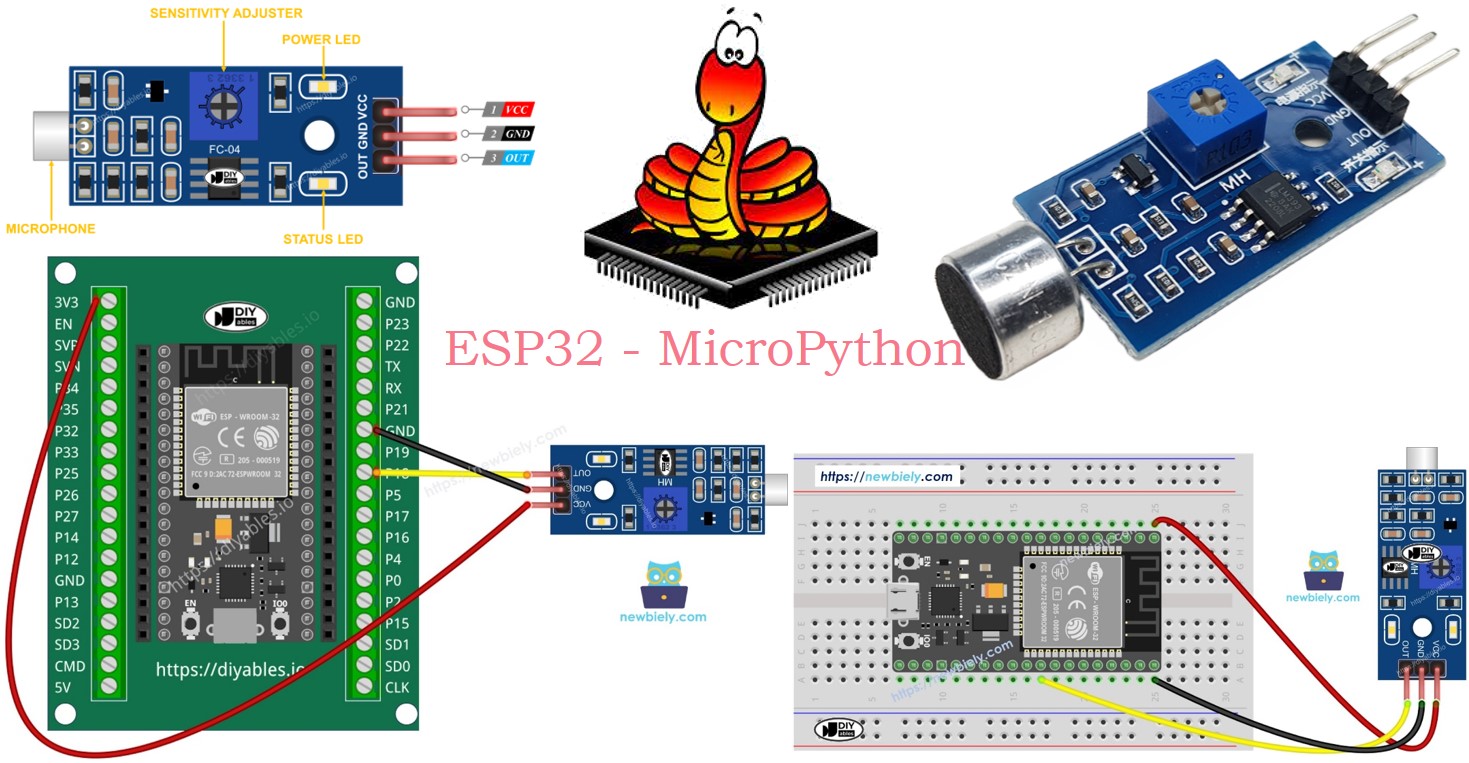
Later, you can change the code to make an LED or a light turn on (using a relay) when it detects sound, or to make a servo motor rotate.
Hardware Preparation
1 | × | ESP-WROOM-32 Dev Module | |
1 | × | USB Cable Type-C | |
1 | × | Digital Sound Sensor | |
1 | × | Analog Sound Sensor | |
1 | × | Jumper Wires | |
1 | × | (Recommended) Screw Terminal Expansion Board for ESP32 |
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables.
Overview of Sound Sensor
A sound sensor can be used to detect nearby sounds. There are two types of sound sensor modules:
- Digital sound sensor module: Provides a simple ON or OFF signal.
- Analog sound sensor module: Offers continuous range values along with ON/OFF signals.
The sensitivity of the digital output can be adjusted using a built-in potentiometer.
The Digital Sound Sensor Pinout
The digital sound sensor has three pins:
- VCC pin: Connect this to VCC, between 3.3V and 5V.
- GNC pin: Connect this to GND, which is 0V.
- OUT pin: This output pin stays HIGH when there is no sound and goes LOW when sound is detected. Connect it to the input pin on your ESP32.
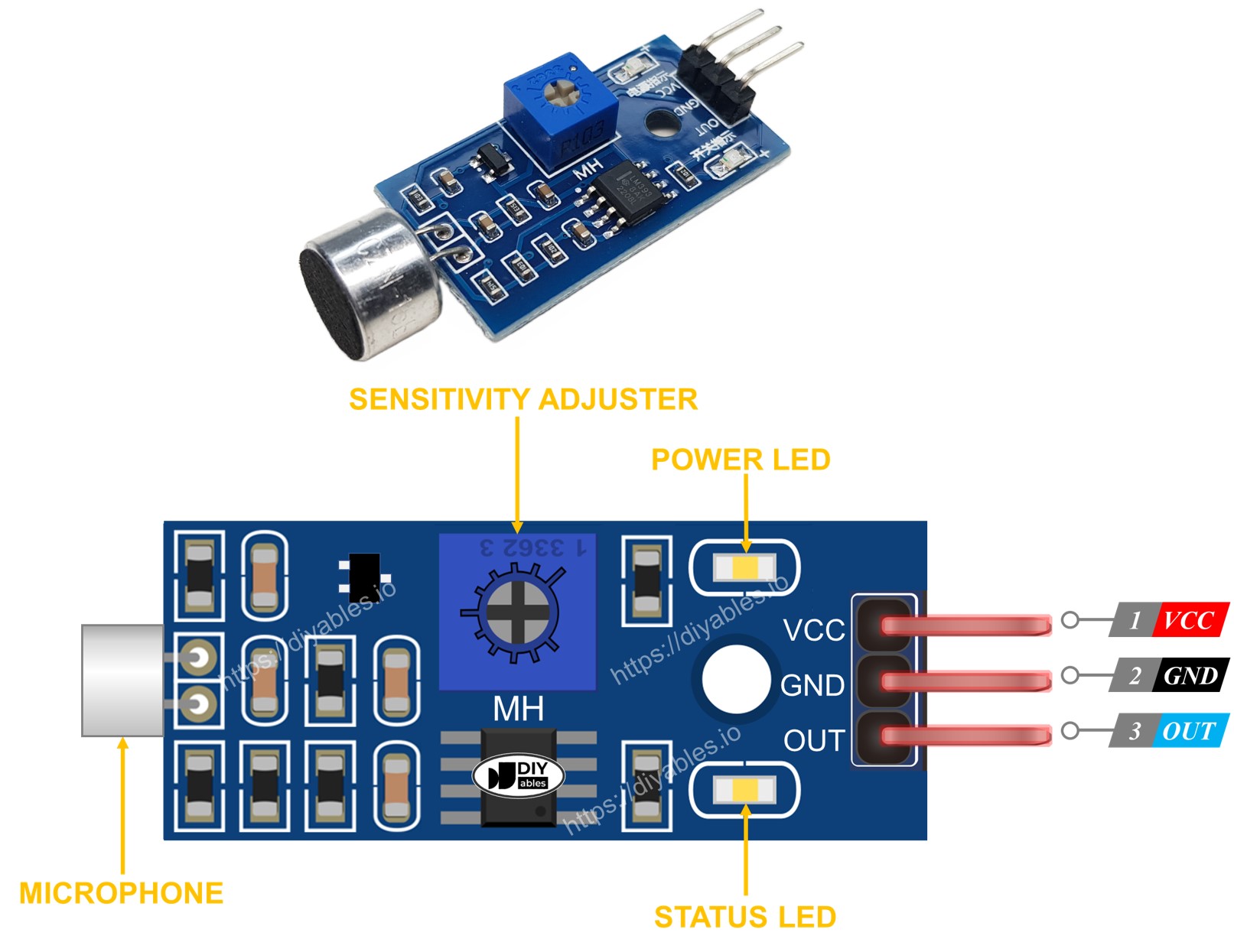
The sound sensor has a sensitivity control knob and two lights that show its status.
- One LED light shows power is on
- One LED light for sound: it lights up with sound, turns off when quiet
The Analog Sound Sensor Pinout
The analog sound sensor has four connectors.
- + pin: Connect to 5V or 3.3V.
- G pin: Connect to GND (0V).
- DO pin: This is a digital output pin that stays HIGH when no sound is present and goes LOW when sound is detected. Connect it to a digital input pin on the ESP32.
- AO pin: This analog output pin provides the sound level as an analog value. Connect it to an analog input pin on the ESP32.
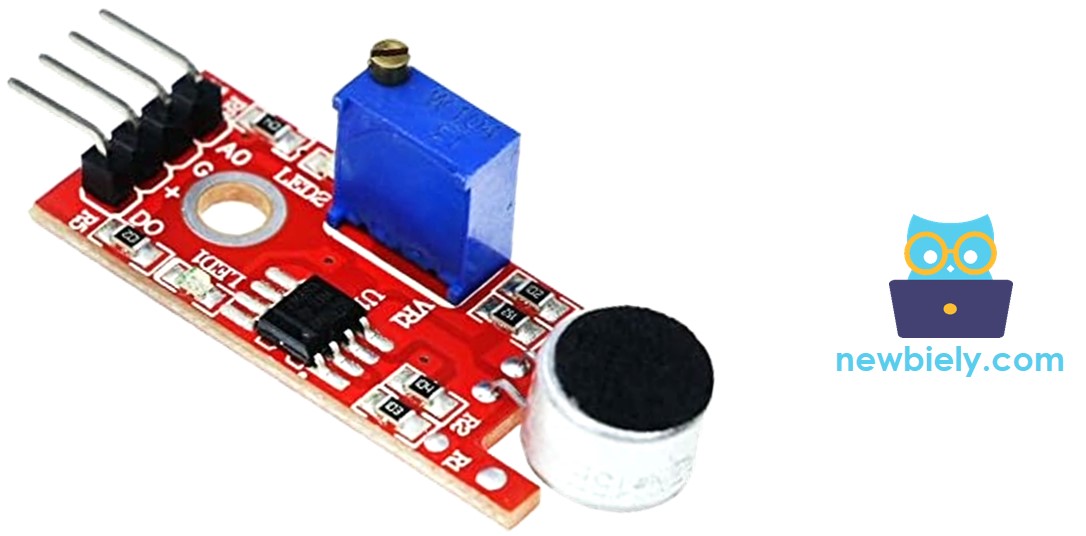
The analog sound sensor has a built-in potentiometric device for easy adjustment of sensitivity. It also features two LED indicators.
- One LED light indicates the power is on.
- Another LED light signals the presence of sound. It turns on when there is noise and turns off when it is silent.
How It Works
The module includes a potentiometer that allows you to adjust its sensitivity to sound. The output pin goes LOW when the sensor detects sound and remains HIGH when no sound is detected.
Wiring Diagram
- How to connect ESP32 and sound sensor using breadboard
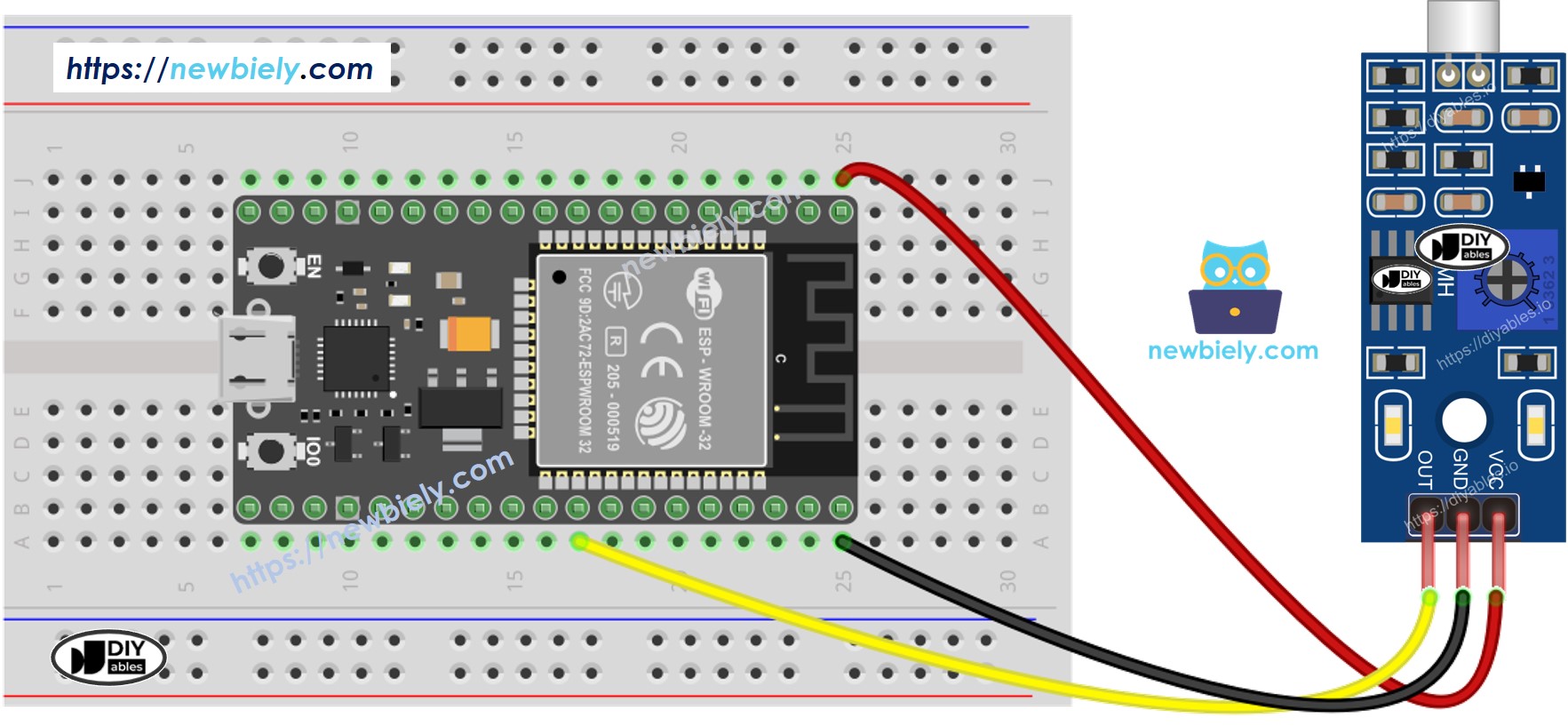
This image is created using Fritzing. Click to enlarge image
- How to connect ESP32 and sound sensor using screw terminal block breakout board
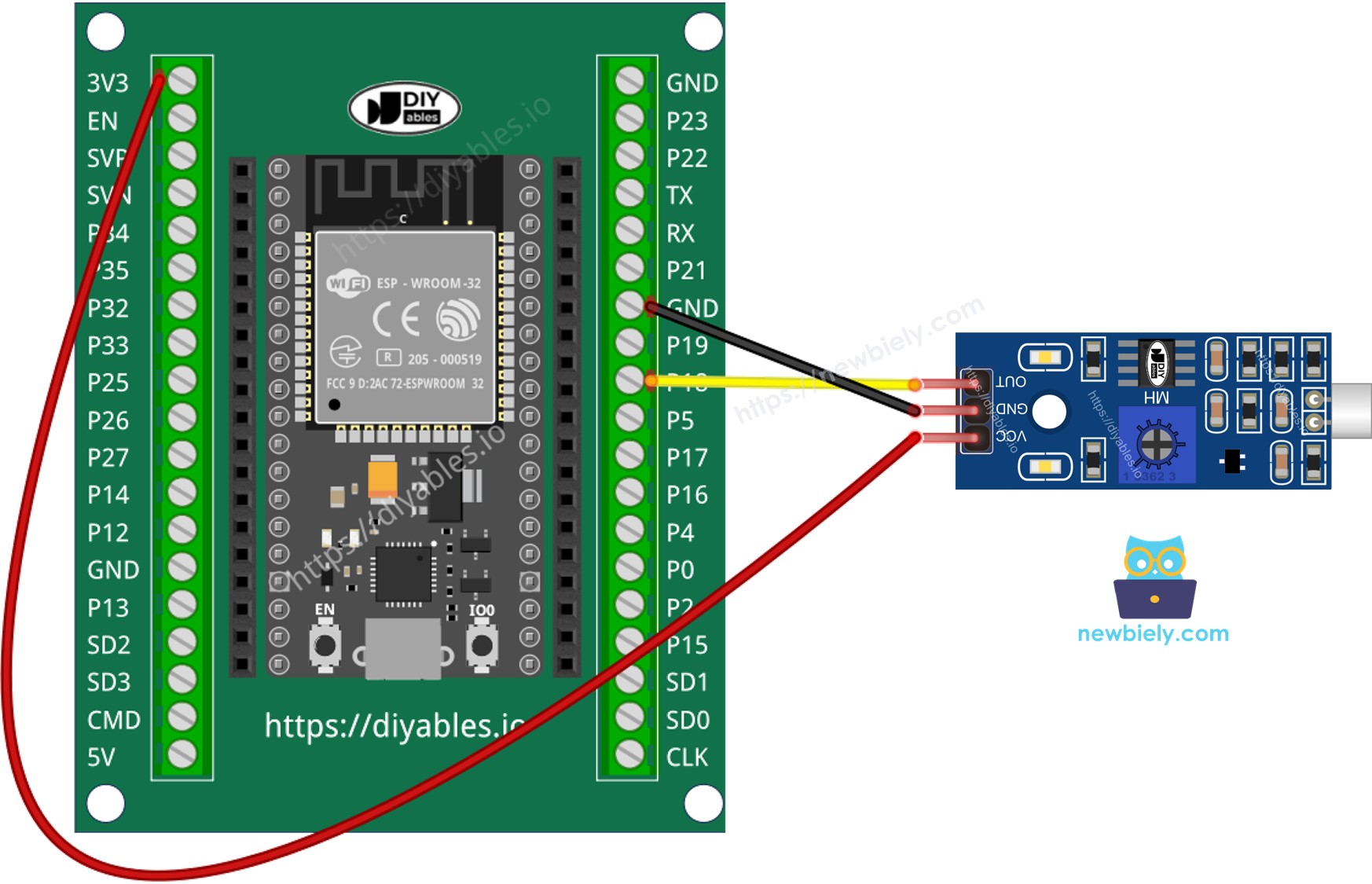
How To Program For Sound Sensor
- Sets the ESP32 pin 8 as a digital input.
- It checks the status of a pin on the ESP32.
ESP32 MicroPython Code - Detecting the sound
Detailed Instructions
Here’s instructions on how to set up and run your MicroPython code on the ESP32 using Thonny IDE:
- Make sure Thonny IDE is installed on your computer.
- Confirm that MicroPython firmware is loaded on your ESP32 board.
- If this is your first time using an ESP32 with MicroPython, check out the ESP32 MicroPython Getting Started guide for step-by-step instructions.
- Connect the sound sensor to the ESP32 according to the provided diagram.
- Connect the ESP32 board to your computer with a USB cable.
- Open Thonny IDE on your computer.
- In Thonny IDE, go to Tools Options.
- Under the Interpreter tab, choose MicroPython (ESP32) from the dropdown menu.
- Make sure the correct port is selected. Thonny IDE usually detects it automatically, but you might need to select it manually (like COM12 on Windows or /dev/ttyACM0 on Linux).
- Copy the provided MicroPython code and paste it into Thonny's editor.
- Save the code to your ESP32 by:
- Clicking the Save button or pressing Ctrl+S.
- In the save dialog, choose MicroPython device.
- Name the file main.py.
- Click the green Run button (or press F5) to execute the script.
- Clap near the sound sensor.
- Check out the message in the Shell at the bottom of Thonny.
If the LED light is always on or always off, even when there is sound, you can adjust the potometer settings to improve how the sensor responds to sound.
Troubleshooting
If the sound sensor isn't working properly, try the following steps:
- Adjust the sensitivity: Turn the small screw on the sensor to change its sensitivity level.
- Reduce vibrations: To prevent the sensor from picking up vibrations or wind noise, secure it firmly to a stable surface.
- Consider the sensing range: The sensor can only detect sounds within 10 inches. For accurate measurement, ensure sounds are made close to the sensor.
- Check the power supply: Make sure the power supply is stable, as fluctuations can affect the performance of this analog sensor.