ESP32 MicroPython Switch
This tutorial instructs you how to use the ON/OFF switch with a ESP32 and MicroPython. In detail, you will learn:
- How to connect the ON/OFF switch to the ESP32.
- How to write MicroPython code for the ESP32 to read the ON/OFF switch's status.
- How to write MicroPython code for the ESP32 to detect events from the ON/OFF switch.
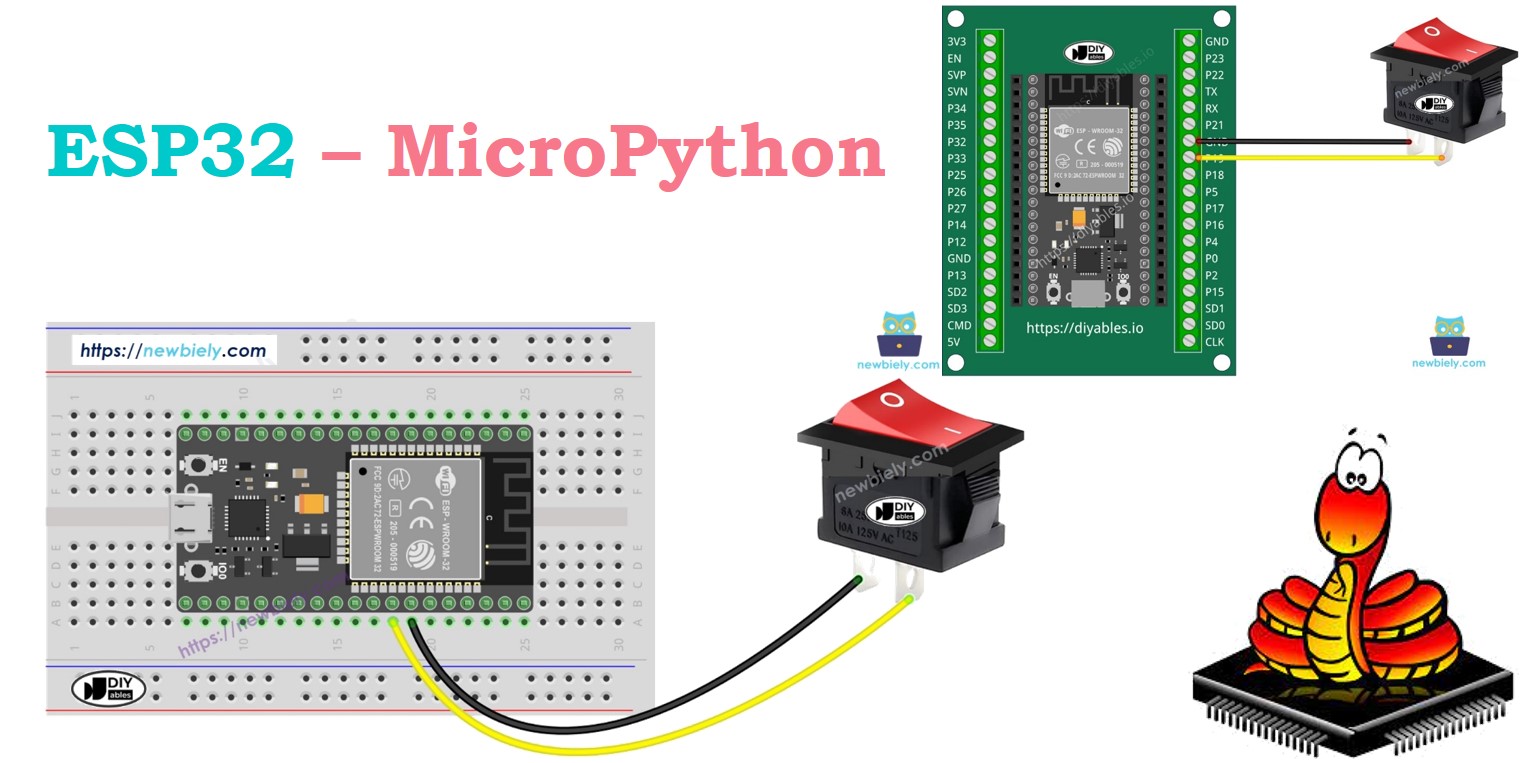
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables ESP32 Starter Kit (ESP32 included) | |
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of ON/OFF Switch
A switch marked ON/OFF moves from ON to OFF, or from OFF to ON, when pressed. It remains in the new position after you release it. Press it once more to switch it back.
Pinout
There are two primary kinds of ON/OFF switches: the two-pin switch and the three-pin switch.
In this guide, we use a switch with two pins. You do not need to worry about which pin is which.
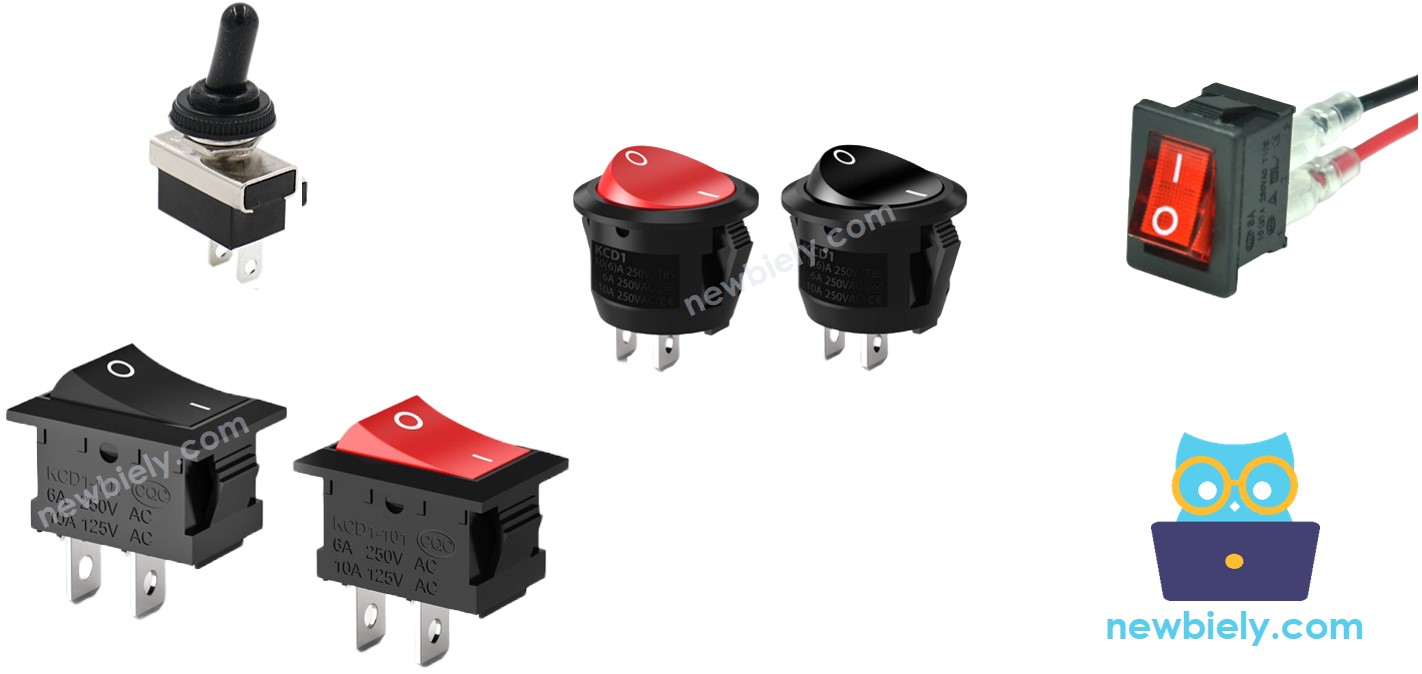
How It Works
Here are two ways to use an ON/OFF switch. Below, you will find the wiring instructions for the ON/OFF switch and the status readings on the ESP32 for each way:
pin 1 | pin 2 | ESP32 Input Pin's State | |
---|---|---|---|
1 | GND | ESP32 Input Pin (with pull-up) | HIGH ⇒ OFF, LOW ⇒ ON |
2 | VCC | ESP32 Input Pin (with pull-down) | HIGH ⇒ ON, LOW ⇒ OFF |
We just have to choose one of the two methods. The rest of the guide will use the first method.
Wiring Diagram
- How to connect ESP32 and switch using breadboard
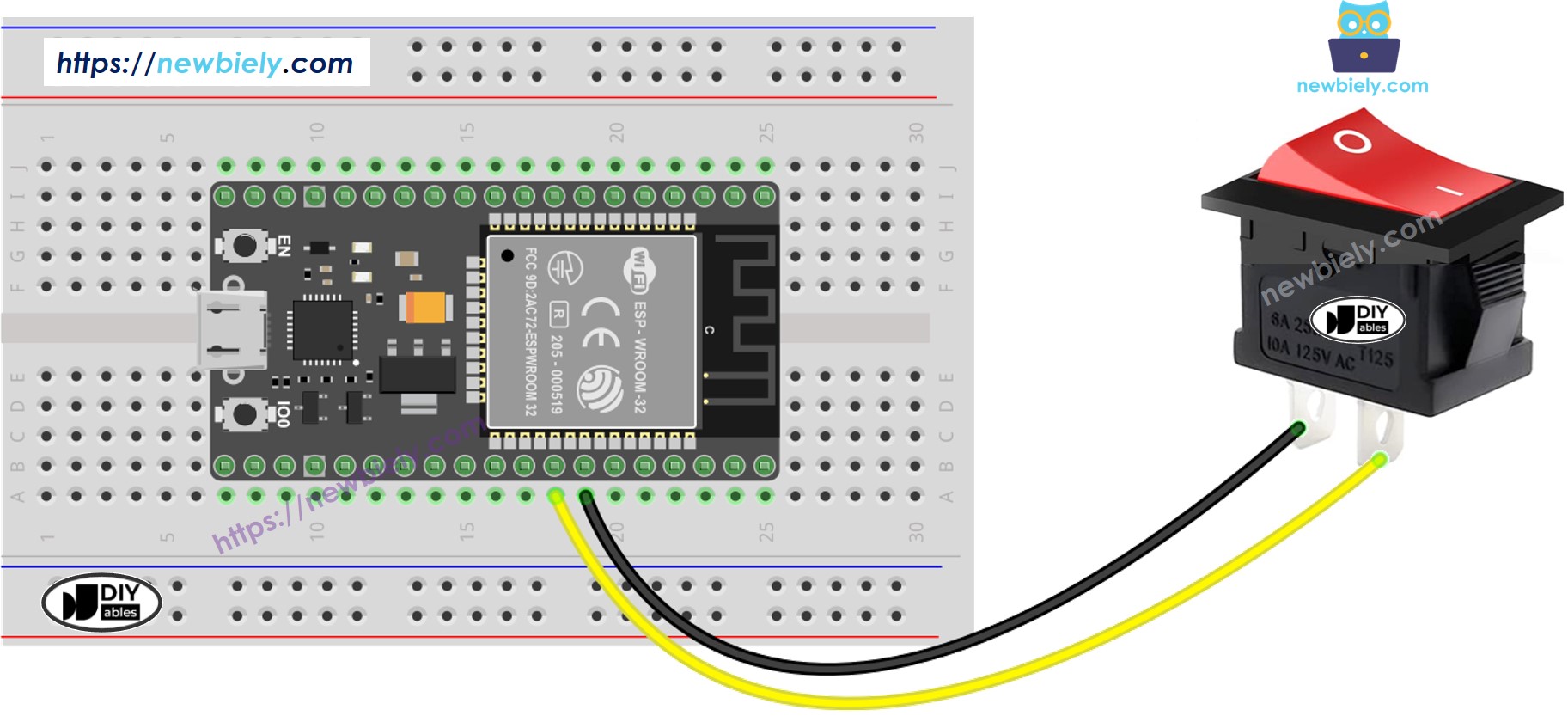
This image is created using Fritzing. Click to enlarge image
- How to connect ESP32 and switch using screw terminal block breakout board
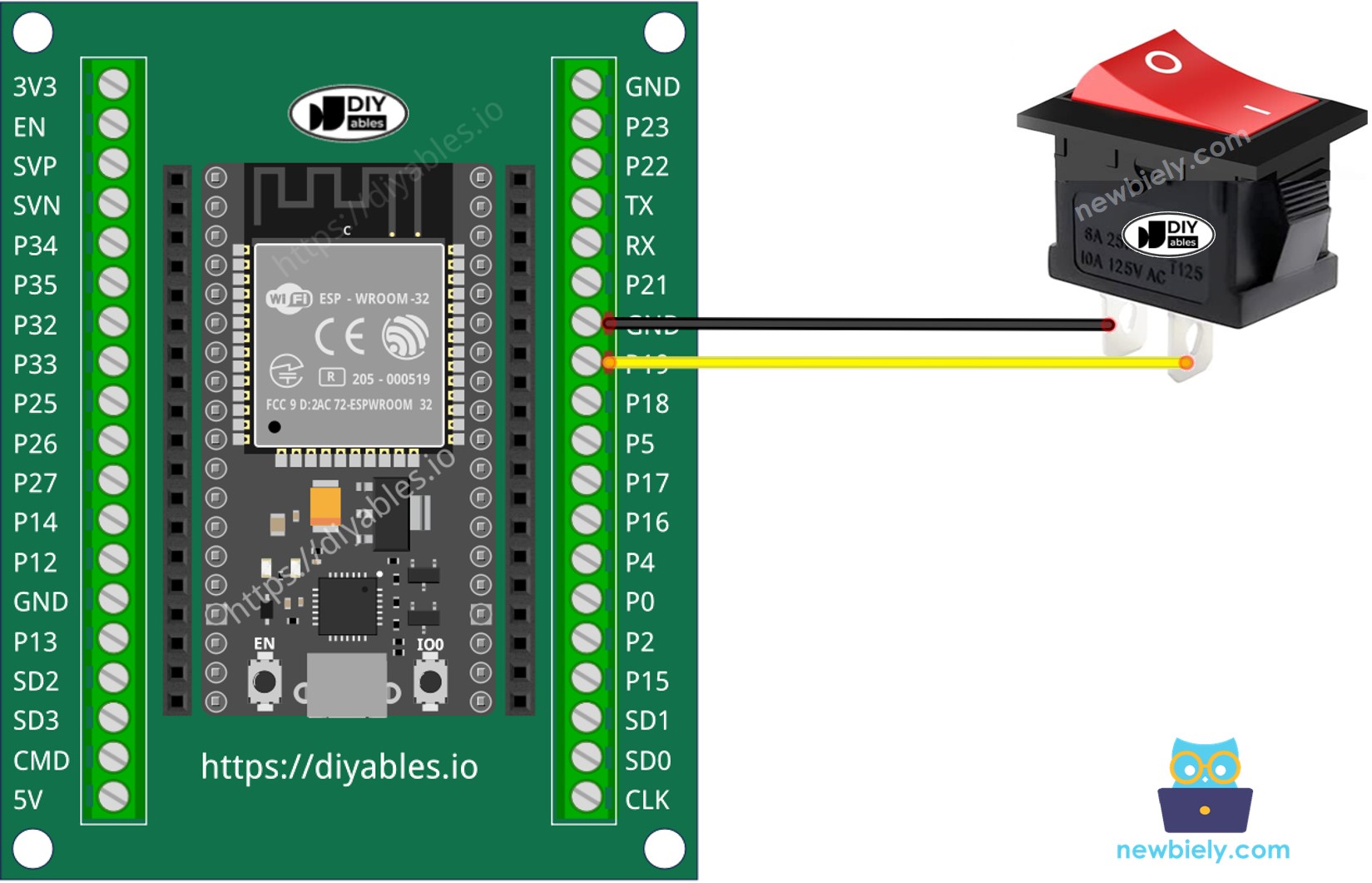
We recommend using a Soldering Iron to securely solder the wires and the ON/OFF switch pin. After that, cover them with Heat Shrink Tube for protection.
ESP32 MicroPython Code - ON/OFF Switch
An ON/OFF switch, similar to a button, requires debouncing. Debouncing can make the code more complex. Fortunately, the DIYables_MicroPython_Button library helps by providing a debouncing feature and includes an internal pull-up resistor, which makes programming easier.
※ NOTE THAT:
There are two use cases:
- First: If the switch is ON, do something. If the switch is OFF, do the opposite.
- Second: If the switch goes from ON to OFF or from OFF to ON, do something.
Let's learn how to program ESP32 one by one.
ESP32 - Read state of ON/OFF Switch
Detailed Instructions
Here’s instructions on how to set up and run your MicroPython code on the ESP32 using Thonny IDE:
- Make sure Thonny IDE is installed on your computer.
- Confirm that MicroPython firmware is loaded on your ESP32 board.
- If this is your first time using an ESP32 with MicroPython, check out the ESP32 MicroPython Getting Started guide for step-by-step instructions.
- Connect the ON/OFF switch to the ESP32 according to the provided diagram.
- Connect the ESP32 board to your computer with a USB cable.
- Open Thonny IDE on your computer.
- In Thonny IDE, go to Tools Options.
- Under the Interpreter tab, choose MicroPython (ESP32) from the dropdown menu.
- Make sure the correct port is selected. Thonny IDE usually detects it automatically, but you might need to select it manually (like COM12 on Windows or /dev/ttyACM0 on Linux).
- On Thonny IDE, navigate to the Tools Manage packages on the Thonny IDE.
- Search “DIYables-MicroPython-Button”, then find the Button library created by DIYables.
- Click on DIYables-MicroPython-Button, then click Install button to install Button library.
- Copy the provided MicroPython code and paste it into Thonny's editor.
- Save the code to your ESP32 by:
- Clicking the Save button or pressing Ctrl+S.
- In the save dialog, choose MicroPython device.
- Name the file main.py.
- Click the green Run button (or press F5) to execute the script.
- Move the switch to the ON position.
- Check out the message in the Shell at the bottom of Thonny.
- Switch to the OFF position.
- Check out the message in the Shell at the bottom of Thonny.
ESP32 - Detect Event from ON/OFF Switch
Detailed Instructions
- Copy the above code and paste it to the Thonny IDE's editor.
- Save the script to your ESP32
- Move switch between ON/OFF several time.
- Check out the message in the Shell at the bottom of Thonny.