ESP32 MicroPython MP3 Player
This guide teaches you how to build an MP3 player using an ESP32 microcontroller with MicroPython, an MP3 player module, a Micro SD Card, and a speaker. The MP3 player plays songs or audio files stored on the Micro SD Card. The ESP32 controls the MP3 player module to choose and play tracks from the SD card, turn them into sound, and send the audio to the speaker. In detail, we will learn:
- How to connect a MP3 player and speaker to ESP32
- How to write the MicroPython code for ESP32 to play music from a micro SD Card
- How to include buttons for play, pause, next, and previous functions
Then, you can modify the code to add a potentiometer or rotary encoder to control the volume.
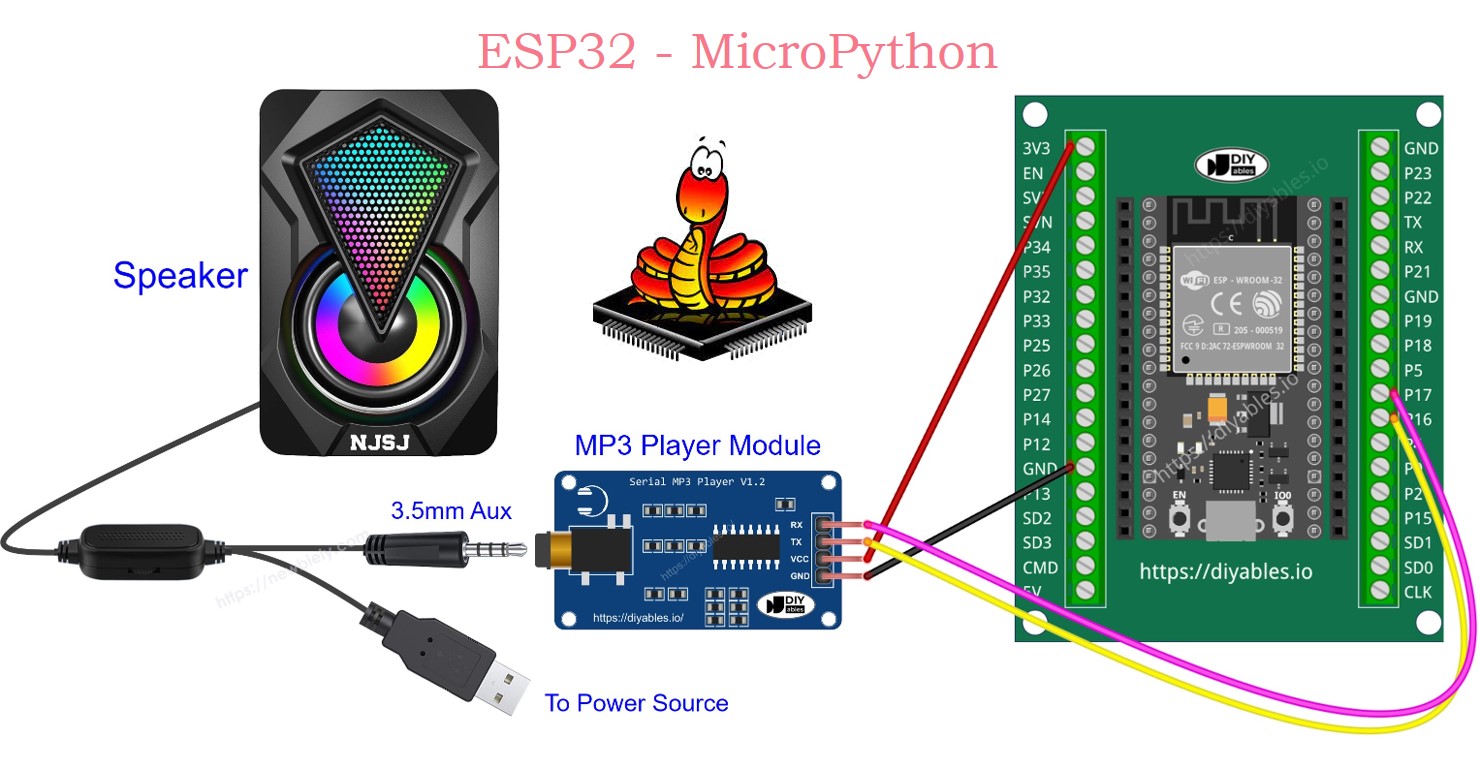
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables ESP32 Starter Kit (ESP32 included) | |
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Serial MP3 Player Module and Speaker
Serial MP3 Player Module Pinout
A serial MP3 player module has three main interfaces:
- ESP32 Interface:
- RX pin: Used to receive data. Connect this to the TX pin on your ESP32 using a Serial connection.
- TX pin: Used to send data. Connect this to the RX pin on your ESP32 using a Serial connection.
- VCC pin: Supplies power. Connect this to the 3.3V pin on your ESP32.
- GND pin: Ground pin. Connect this to a ground (0V) source.
- Speaker Interface:
- Comes with a 3.5mm Aux output female jack.
- Micro SD Card Interface:
- The Micro SD Card slot is located on the back of the MP3 module.
- Audio connection: It has a 3.5mm Aux male connector for connecting to an MP3 player.
- Power connection: It can connect to a USB, a 5V power adapter, or other power sources.
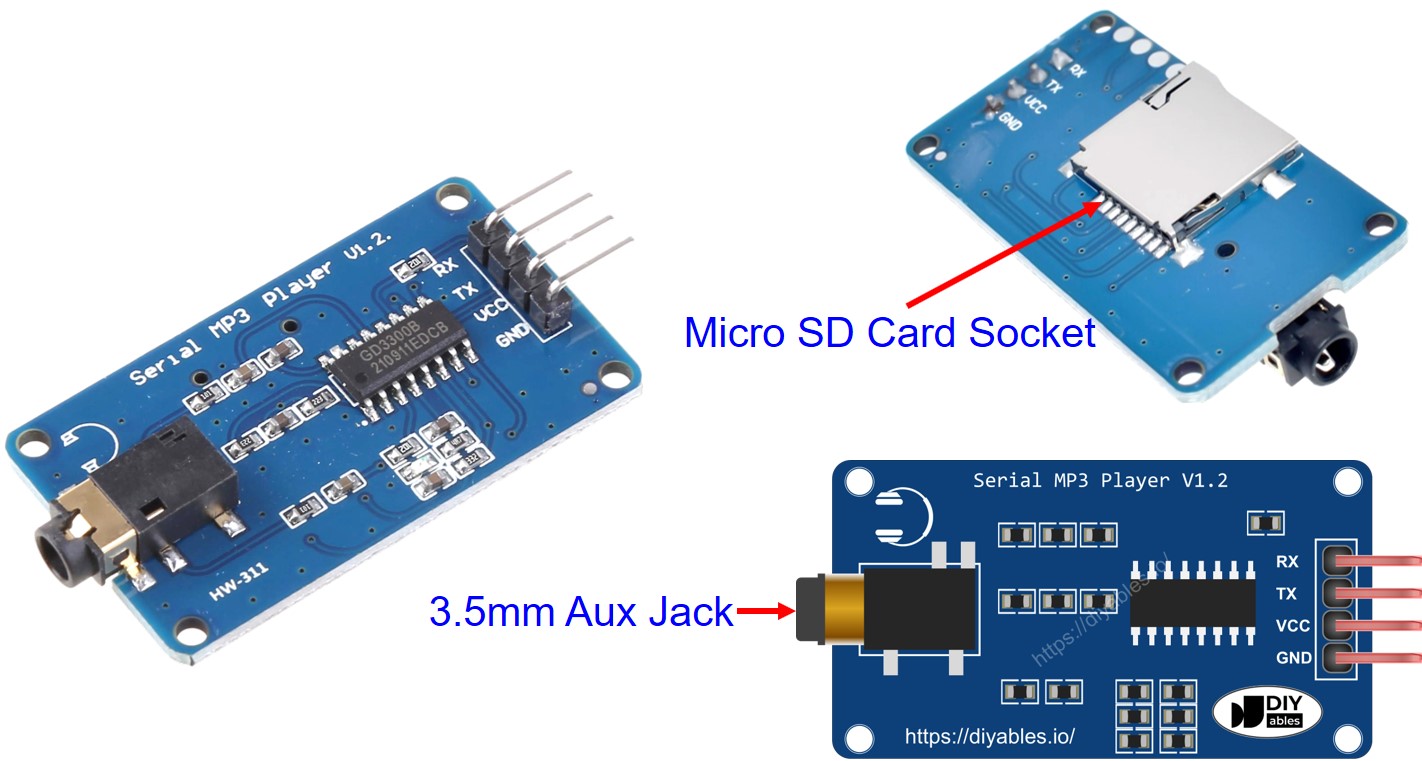
Speaker Pinout
A speaker usually has two connection points:
How It Works
Here's what you need to do:
- Load a list of songs or recordings onto a Micro SD card.
- Insert the Micro SD card into the MP3 player module.
- Connect the MP3 player module to the ESP32.
- Hook up the MP3 player module to a speaker.
- Plug the speaker into a power source. If you uses headphone or earphone, you do not need to do this step.
Each MP3 file on the Micro SD card is numbered, starting from 0, which sets the order of the songs. We will write the MicroPython script for the ESP32 to send different commands to the MP3 player module. It supports commands are:
- Start Playing
- Stop
- Play Next Track
- Play Previous Track
- Adjust Volume
Once receiving the command, The MP3 player module plays the files stored on the Micro SD card, turns them into audio signals, and sends these signals to the speaker through the 3.5mm Aux jack.
Wiring Diagram
- How to connect ESP32 and mp3 player using breadboard
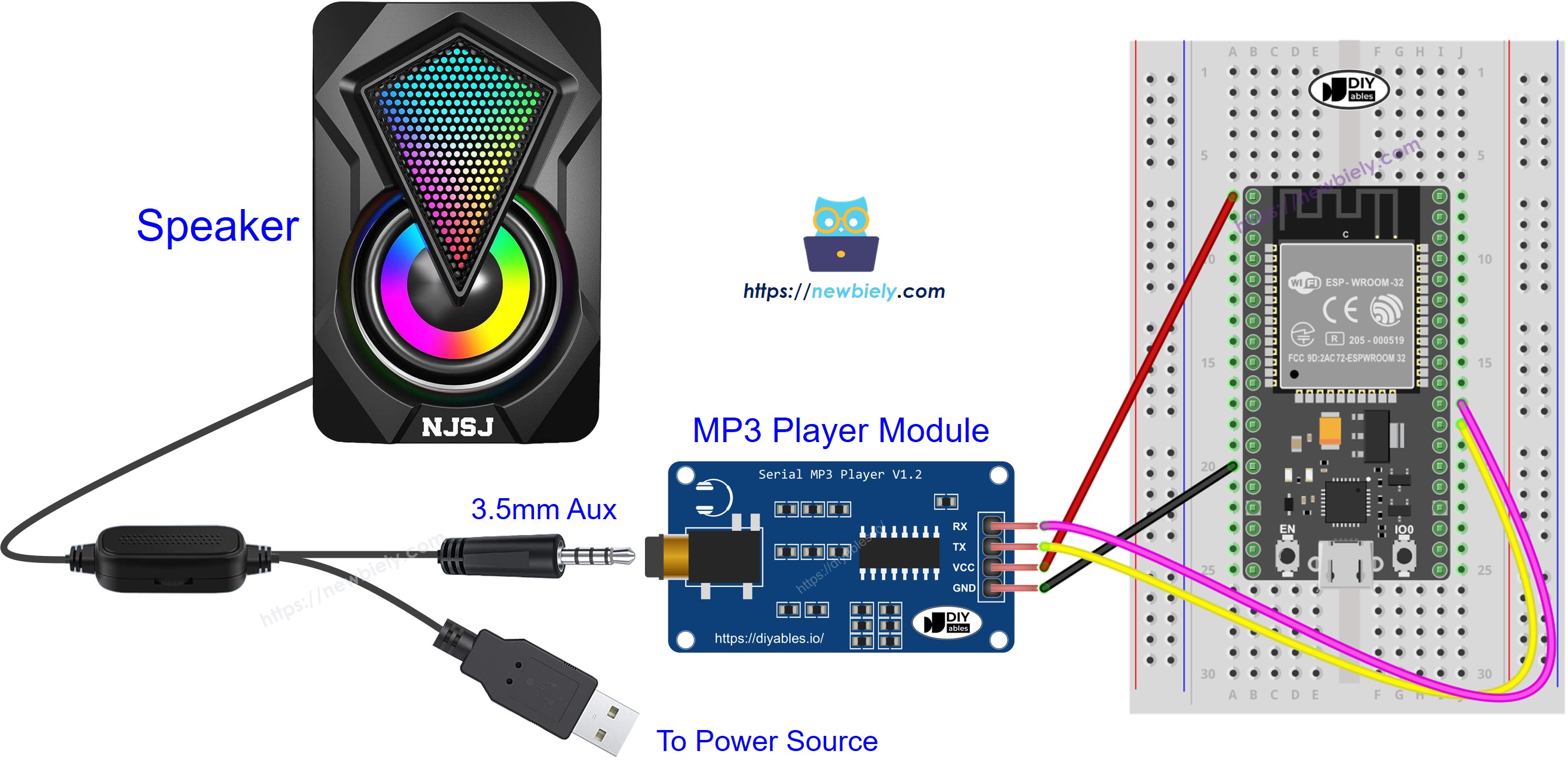
This image is created using Fritzing. Click to enlarge image
- How to connect ESP32 and mp3 player using screw terminal block breakout board
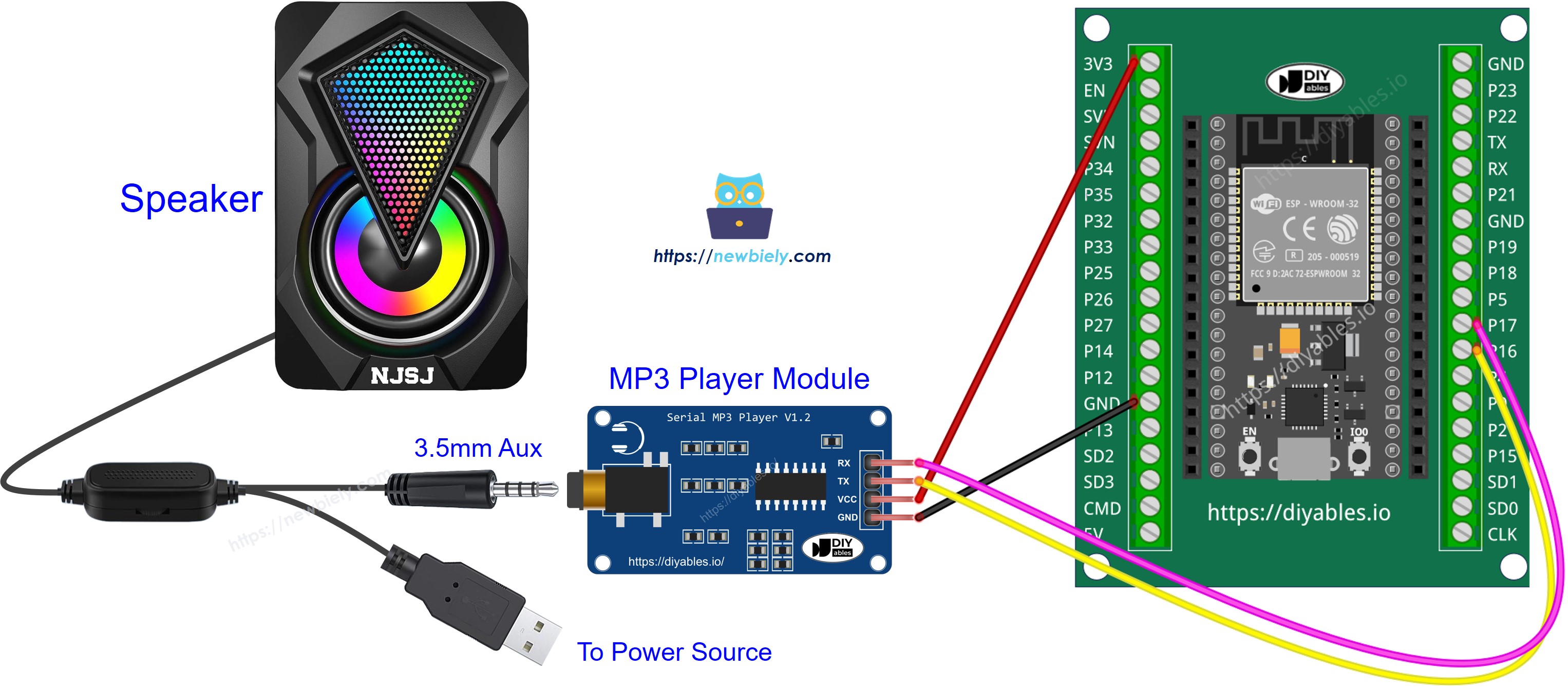
ESP32 MicroPython Code - Play Music
The code below begins to play the first song stored on the Micro SD Card.
Detailed Instructions
Here’s instructions on how to set up and run your MicroPython code on the ESP32 using Thonny IDE:
- Make sure Thonny IDE is installed on your computer.
- Confirm that MicroPython firmware is loaded on your ESP32 board.
- If this is your first time using an ESP32 with MicroPython, check out the ESP32 MicroPython Getting Started guide for step-by-step instructions.
- Connect the ESP32 board to the MP3 player module according to the provided diagram.
- Connect the ESP32 board to your computer with a USB cable.
- Open Thonny IDE on your computer.
- In Thonny IDE, go to Tools Options.
- Under the Interpreter tab, choose MicroPython (ESP32) from the dropdown menu.
- Make sure the correct port is selected. Thonny IDE usually detects it automatically, but you might need to select it manually (like COM12 on Windows or /dev/ttyACM0 on Linux).
- Copy the provided MicroPython code and paste it into Thonny's editor.
- Save the code to your ESP32 by:
- Clicking the Save button or pressing Ctrl+S.
- In the save dialog, choose MicroPython device.
- Name the file main.py.
- Click the green Run button (or press F5) to execute the script.
- Enjoy the music