ESP32 MicroPython LCD I2C
This tutorial instructs you how to use ESP32 with a 16x2 LCD I2C, utilizing MicroPython. You will learn:
- How to connect an LCD I2C to an ESP32
- How to write MicroPython code for the ESP32 to display text on the LCD I2C
- How to write MicroPython code for the ESP32 to display numbers on the LCD I2C
- How to write MicroPython code for the ESP32 to display custom characters on the LCD I2C
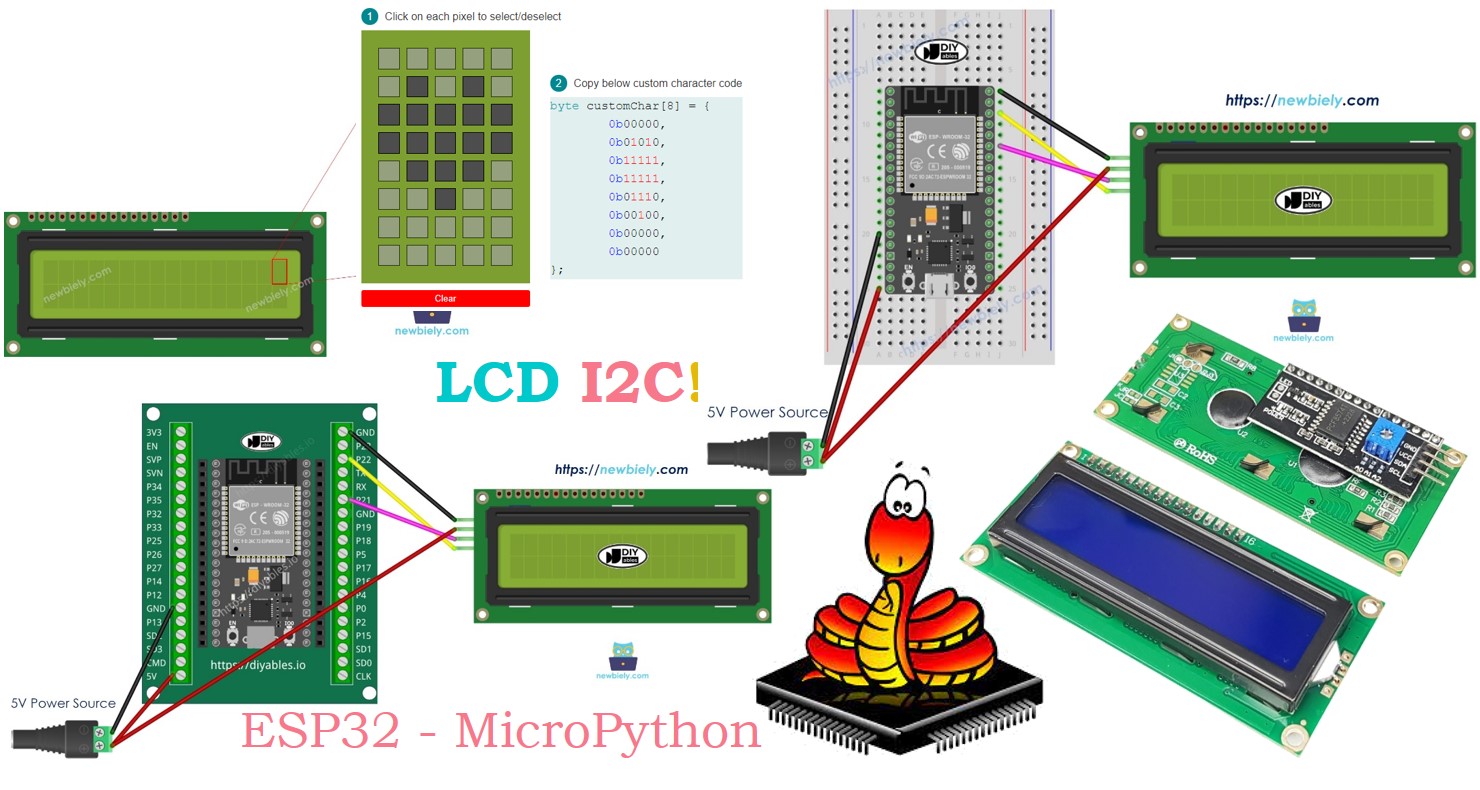
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables ESP32 Starter Kit (ESP32 included) | |
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of LCD I2C 16x2
The 16x2 LCD I2C is a screen with 16 columns and 2 rows. It has an I2C interface and comes with a potentiometer to adjust the screen's contrast.
Pinout
The LCD I2C uses an I2C interface and includes 4 pins.
- GND pin: connect to GND (0 volts).
- VCC pin: connect to VCC for power (5 volts).
- SDA pin: transfers I2C data signal.
- SCL pin: transfers I2C clock signal.
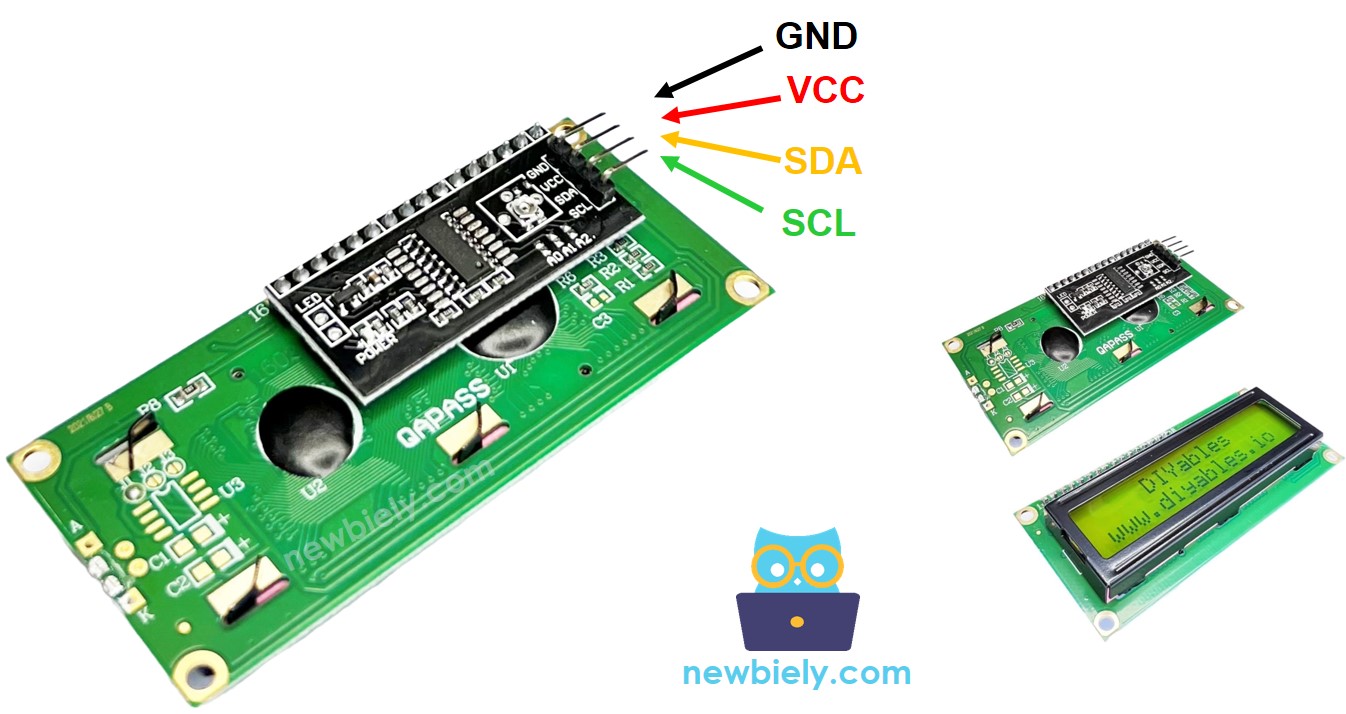
LCD Coordinate
The LCD I2C 16x2 includes 16 columns and 2 rows. The numbering of both columns and rows begins from 0.
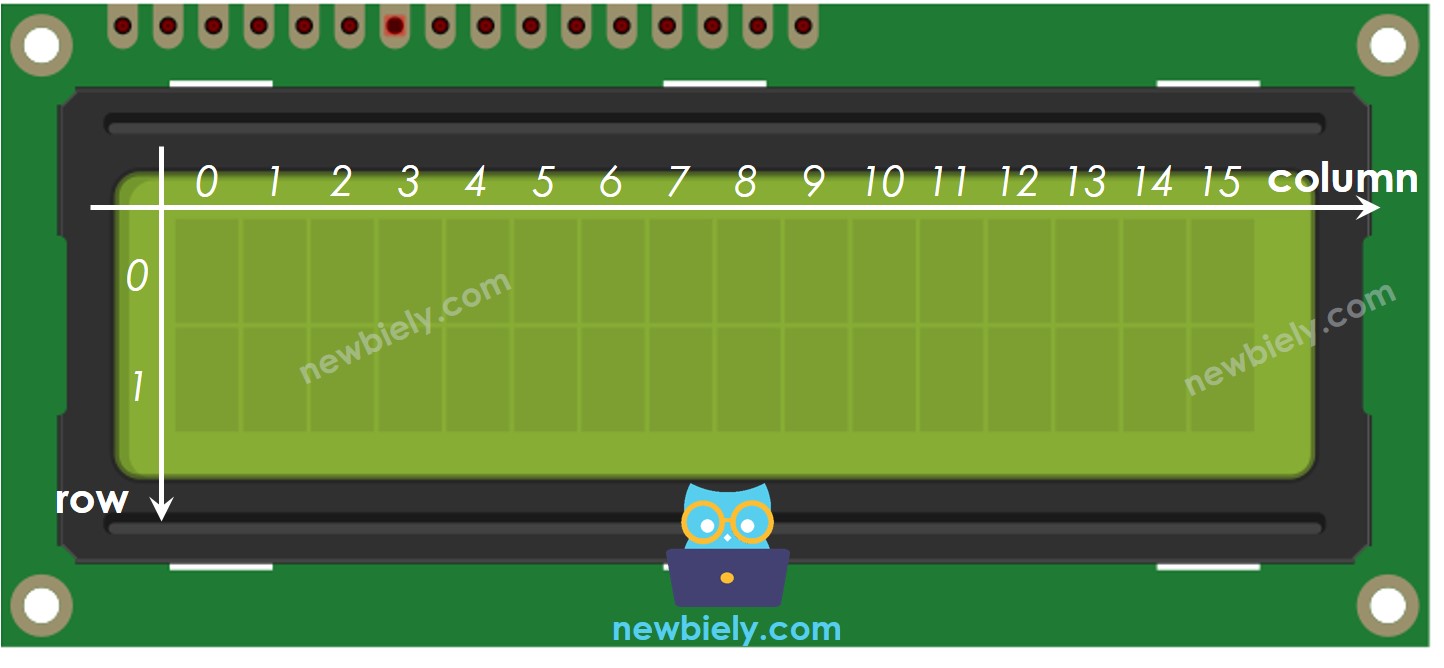
Wiring Diagram
- How to connect ESP32 and LCD i2c using breadboard (powered via USB cable)
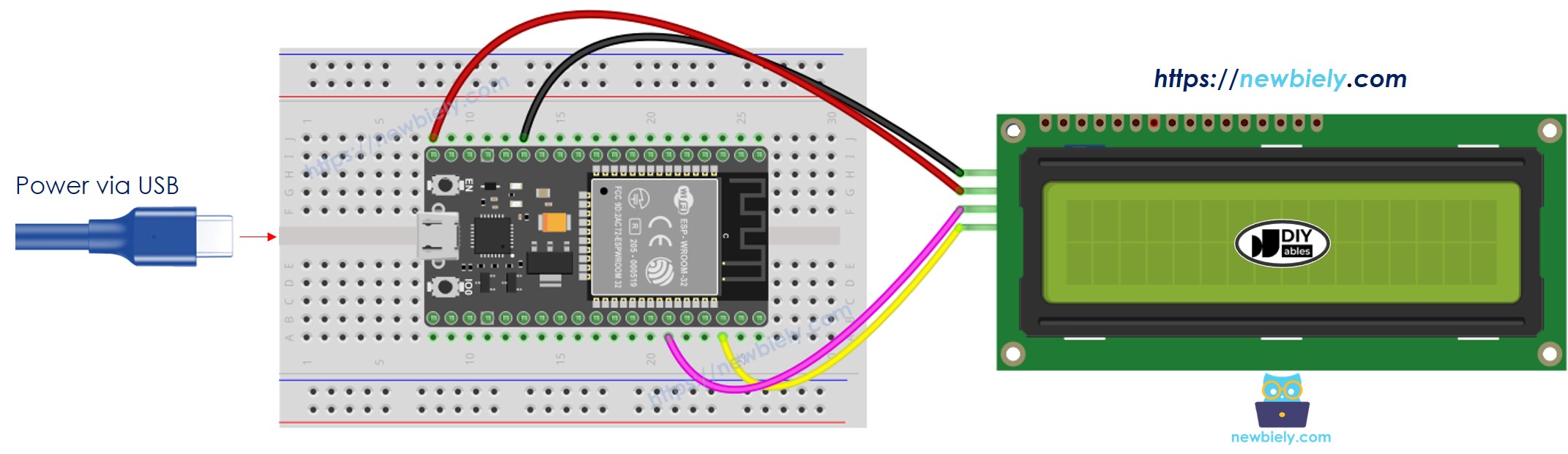
This image is created using Fritzing. Click to enlarge image
- How to connect ESP32 and LCD i2c using breadboard (powered via Vin pin)
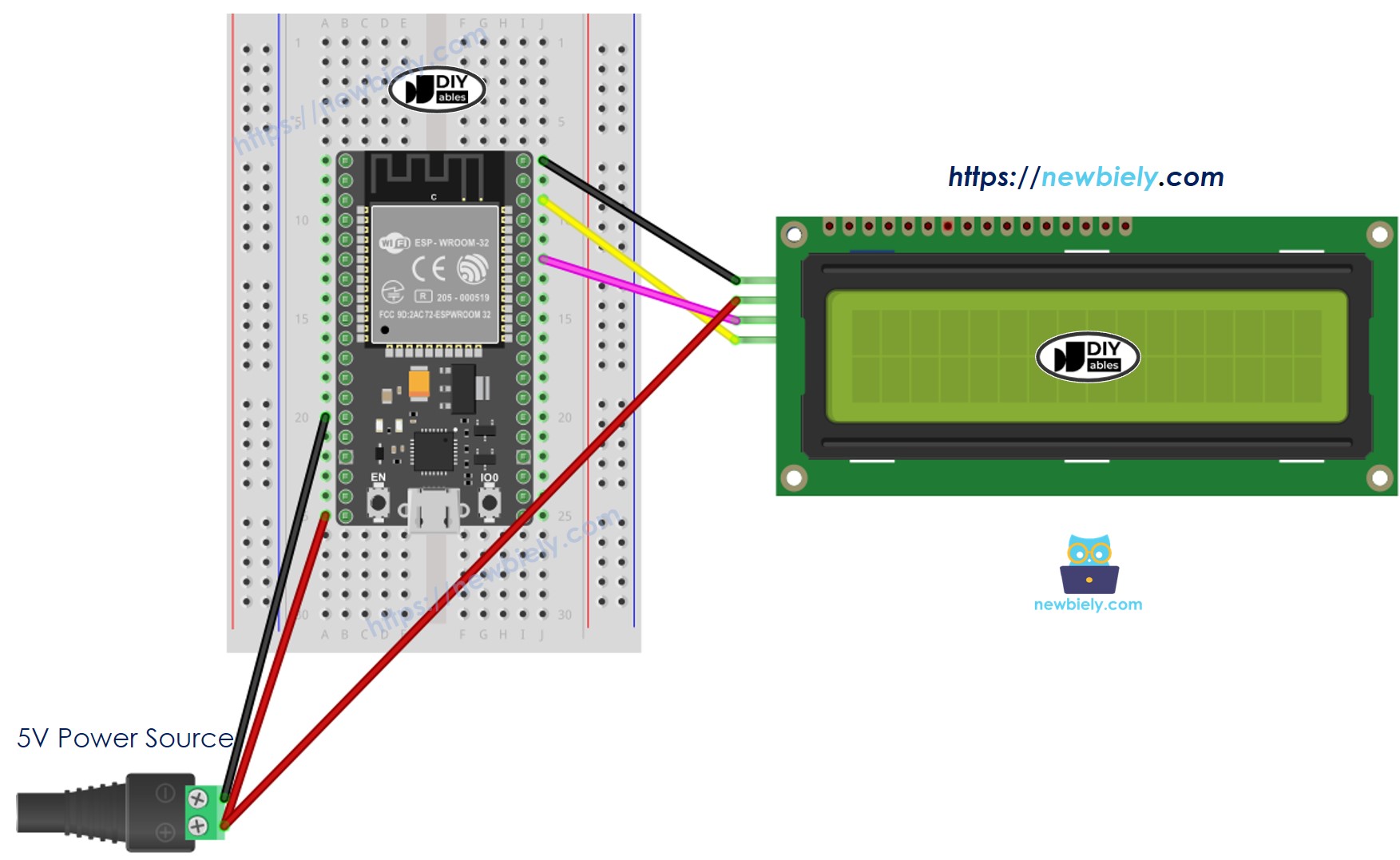
This image is created using Fritzing. Click to enlarge image
- How to connect ESP32 and LCD i2c using screw terminal block breakout board (powered via USB cable)
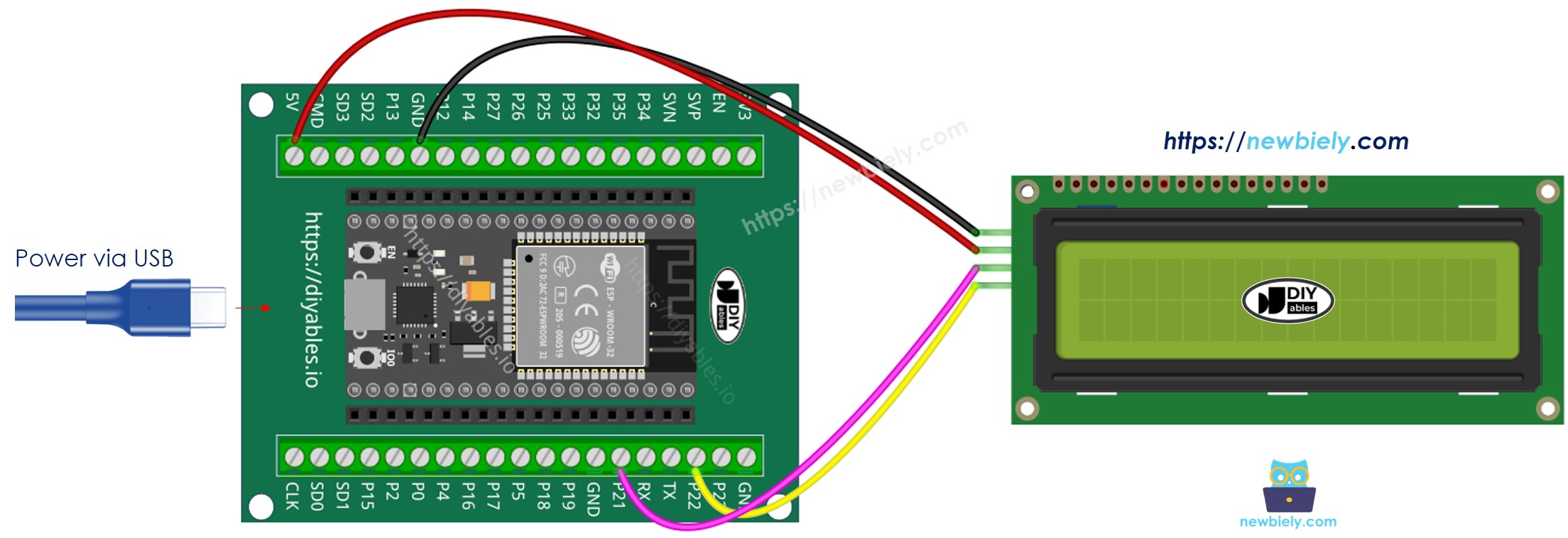
- How to connect ESP32 and LCD i2c using screw terminal block breakout board (powered via Vin pin)
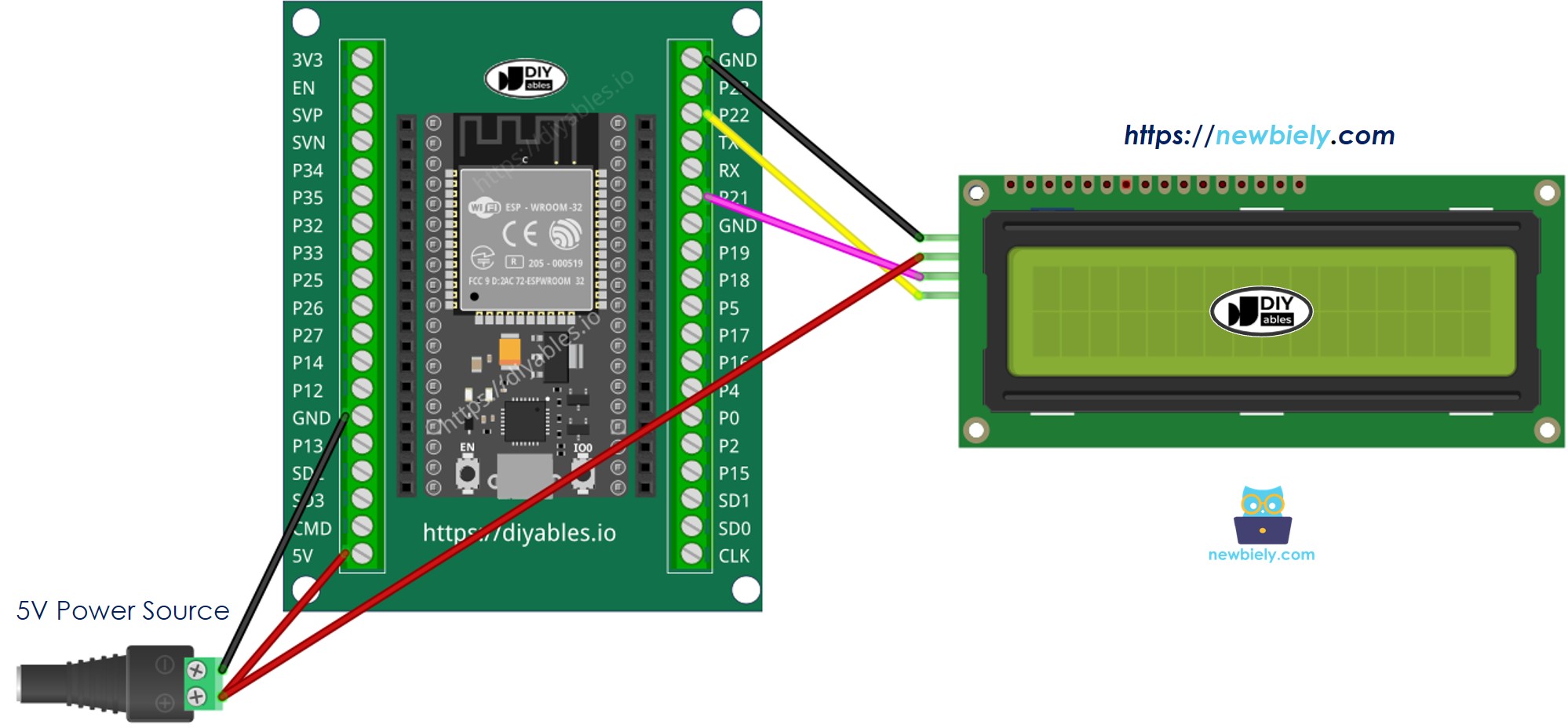
LCD I2C | ESP32 |
---|---|
VCC | Vin 5V |
GND | GND |
SDA | GPIO21 (SDA) |
SCL | GPIO22 (SCL) |
ESP32 MicroPython Code
Detailed Instructions
Here’s instructions on how to set up and run your MicroPython code on the ESP32 using Thonny IDE:
- Make sure Thonny IDE is installed on your computer.
- Confirm that MicroPython firmware is loaded on your ESP32 board.
- If this is your first time using an ESP32 with MicroPython, check out the ESP32 MicroPython Getting Started guide for step-by-step instructions.
- Connect the LCD I2C display to the ESP32 according to the provided diagram.
- Connect the ESP32 board to your computer with a USB cable.
- Open Thonny IDE on your computer.
- In Thonny IDE, go to Tools Options.
- Under the Interpreter tab, choose MicroPython (ESP32) from the dropdown menu.
- Make sure the correct port is selected. Thonny IDE usually detects it automatically, but you might need to select it manually (like COM12 on Windows or /dev/ttyACM0 on Linux).
- Navigate to the Tools Manage packages on the Thonny IDE.
- Search “DIYables-MicroPython-LCD-I2C”, then find the LCD I2C library created by DIYables.
- Click on DIYables-MicroPython-LCD-I2C, then click Install button to install LCD I2C library.
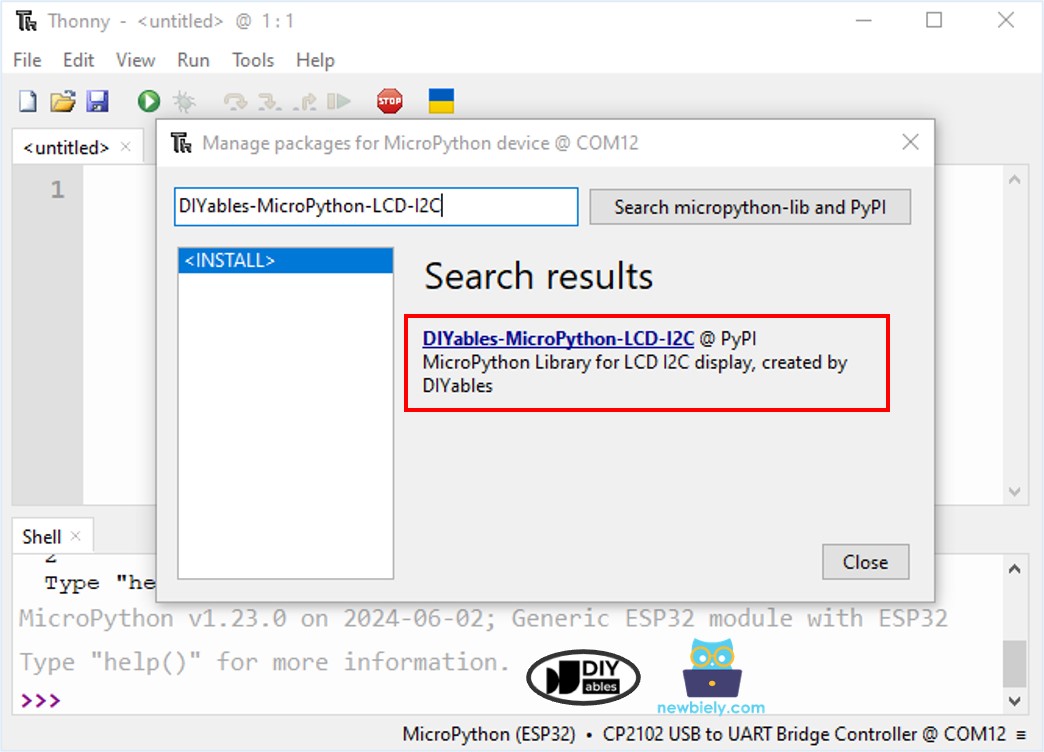
- Copy the provided MicroPython code and paste it into Thonny's editor.
- Save the code to your ESP32 by:
- Clicking the Save button or pressing Ctrl+S.
- In the save dialog, choose MicroPython device.
- Name the file main.py.
- Click the green Run button (or press F5) to execute the script.
- Check out the result on the LCD display.
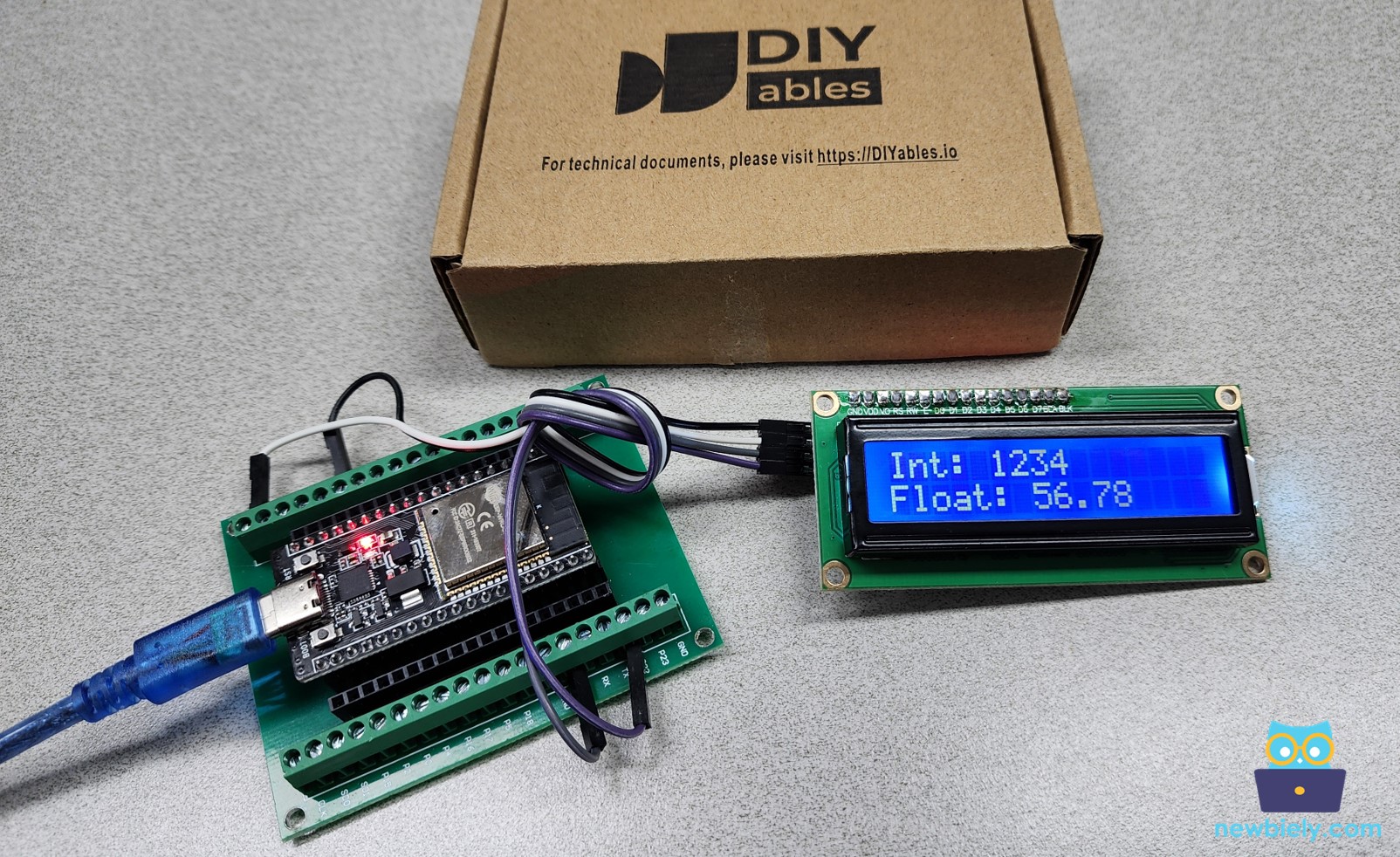
Do More with LCD
Displaying Custom Character on LCD
To display special characters or symbols (like a heart or an angry bird) on an LCD, you'll need to use a character generator. This is because the lcd.print() function can only display standard ASCII characters.
The LCD 16x2 can display 32 characters, arranged in 16 positions per line over 2 lines. Each character is composed of 40 pixels, organized in 8 rows and 5 columns.
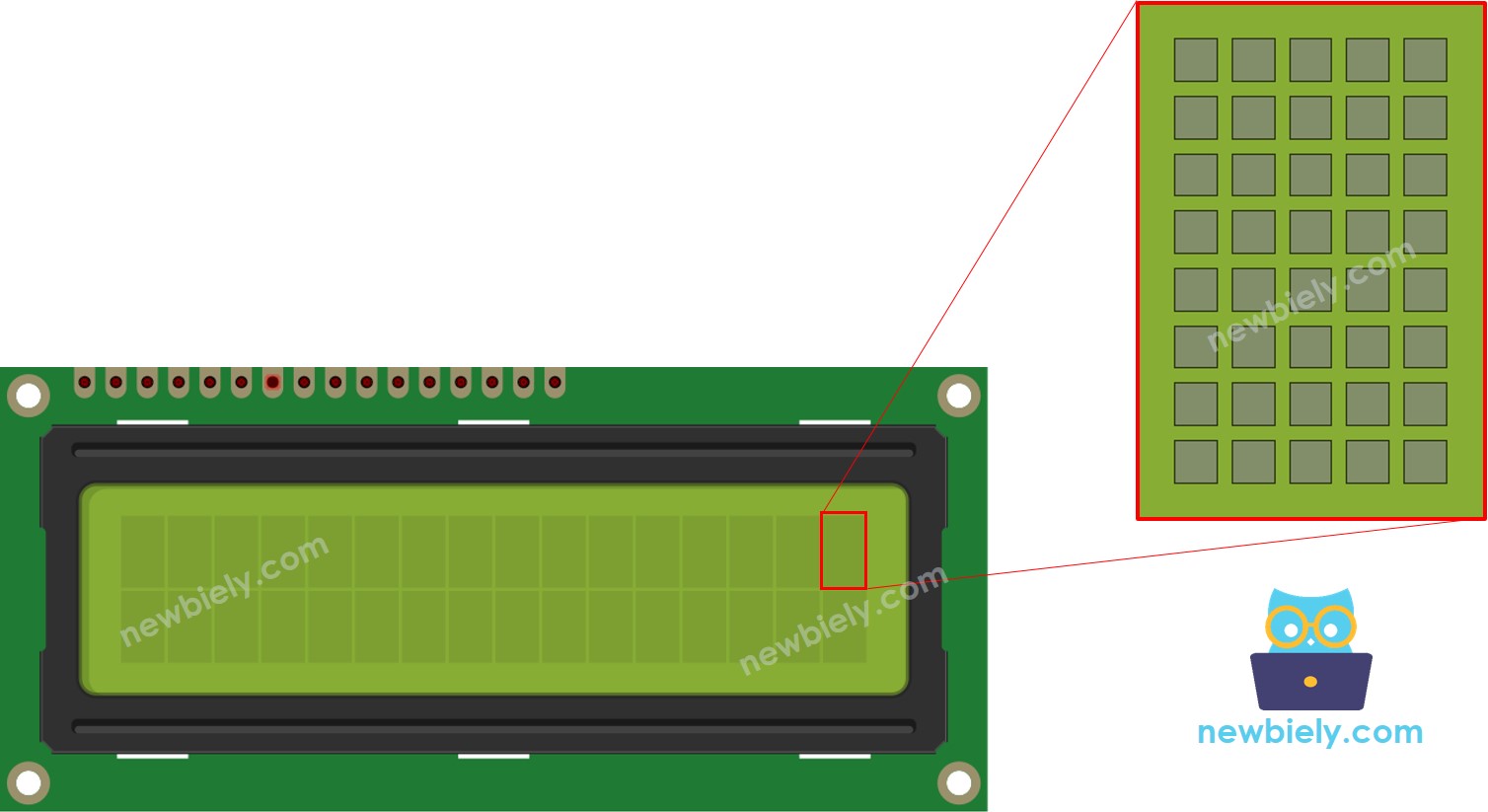
The character generator makes a character (40 pixels). You just need to follow these steps:
Result shown on the screen:
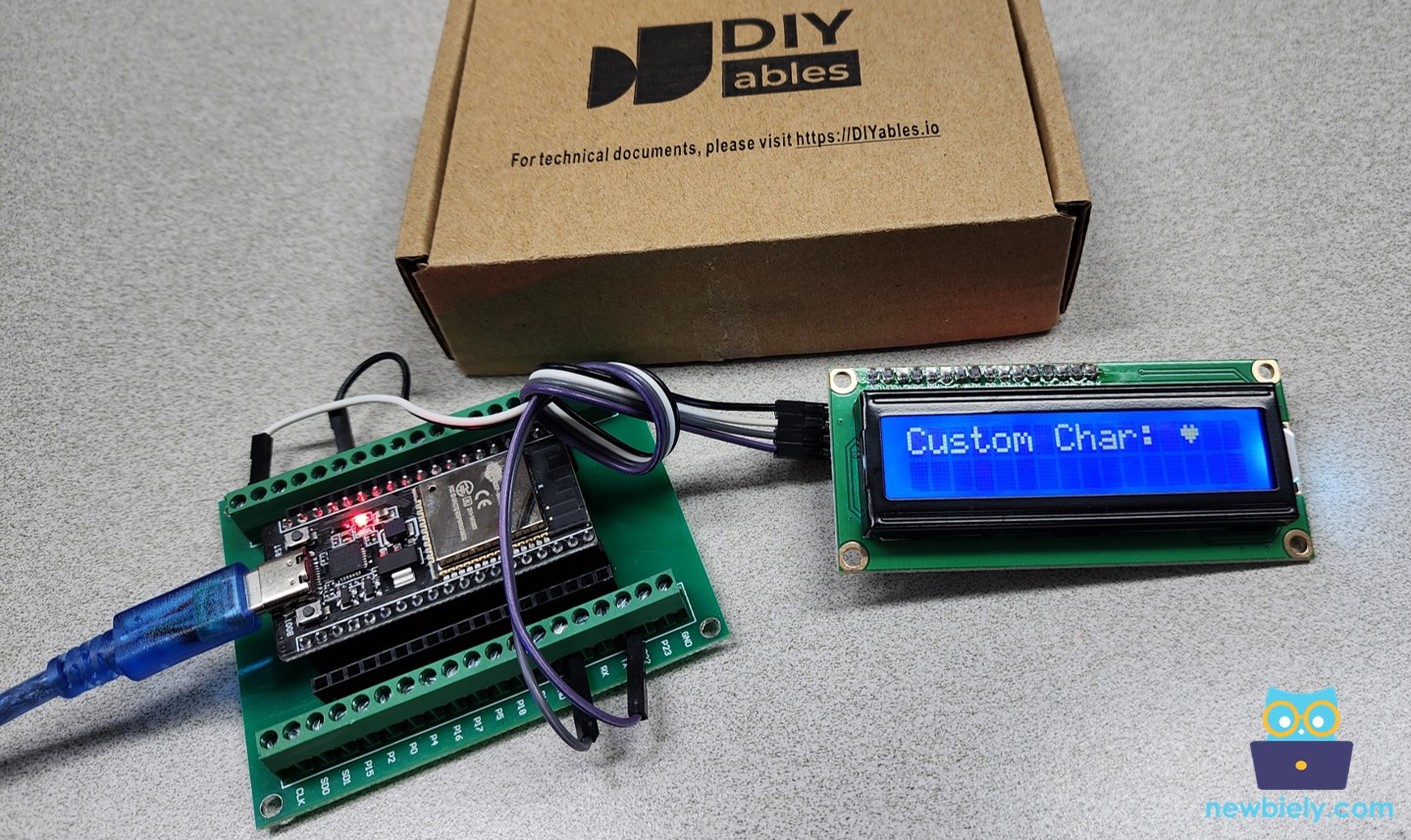
Displaying Multiple custom characters on LCD
We can create up to 8 special characters (from 0 to 7). Here is how to make and show three of these characters.
Result shown on LCD screen:
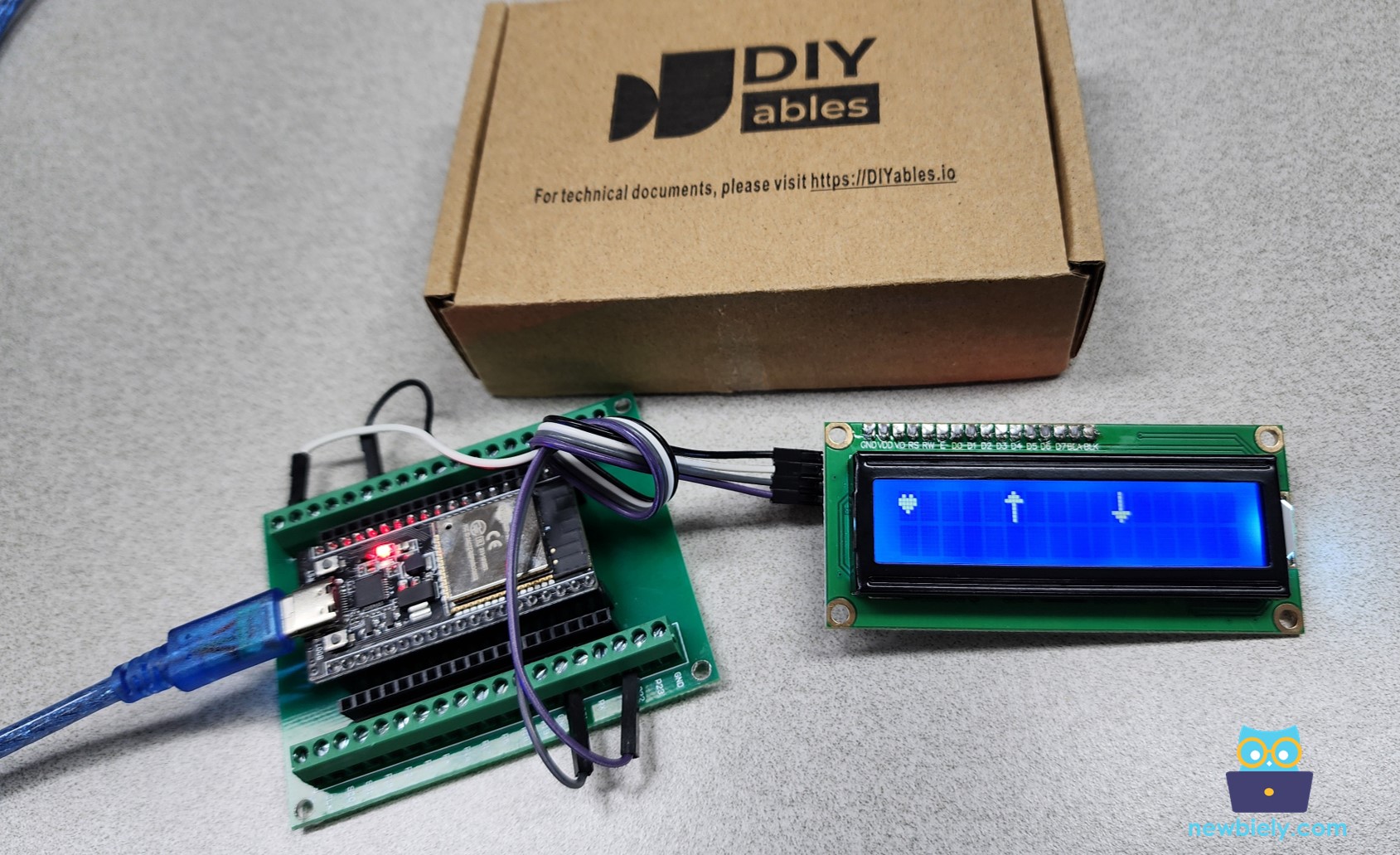
Summary: How to Use Custom Characters on an LCD
- Create binary code: Use the provided tool to generate the binary code for your custom character.
- Write down the code: Copy the binary code from the tool.
- Create a custom character and position it at a number from 0 to 7
- Show a custom symbol on the LCD display.
Troubleshooting on LCD I2C
If you're having trouble displaying text on your LCD I2C screen, try the following:
- Adjust the brightness: Rotate the potentiometer on the back of the LCD to increase or decrease the brightness.
- Check the I2C address: The I2C address for the LCD may vary between manufacturers. Common addresses are 0x27 and 0x3F. Experiment with these addresses to see if either works. If not, you can run the below I2C scanner code on ESP32 board to scan for the correct I2C address.
The result shown in the Shell at the bottom of Thonny: