ESP32 MicroPython Flame Sensor
This tutorial instructs you how to use a flame sensor with ESP32 and MicroPython to detect and measure flames and fire. In detail, we will learn:
- How a flame sensor works.
- How to connect a flame sensor to a ESP32.
- How to write MicroPython code for the ESP32 to detect fire using the digital signal from the flame sensor.
- How to write MicroPython code for the ESP32 to measure flame intensity with the analog signal from the flame sensor.
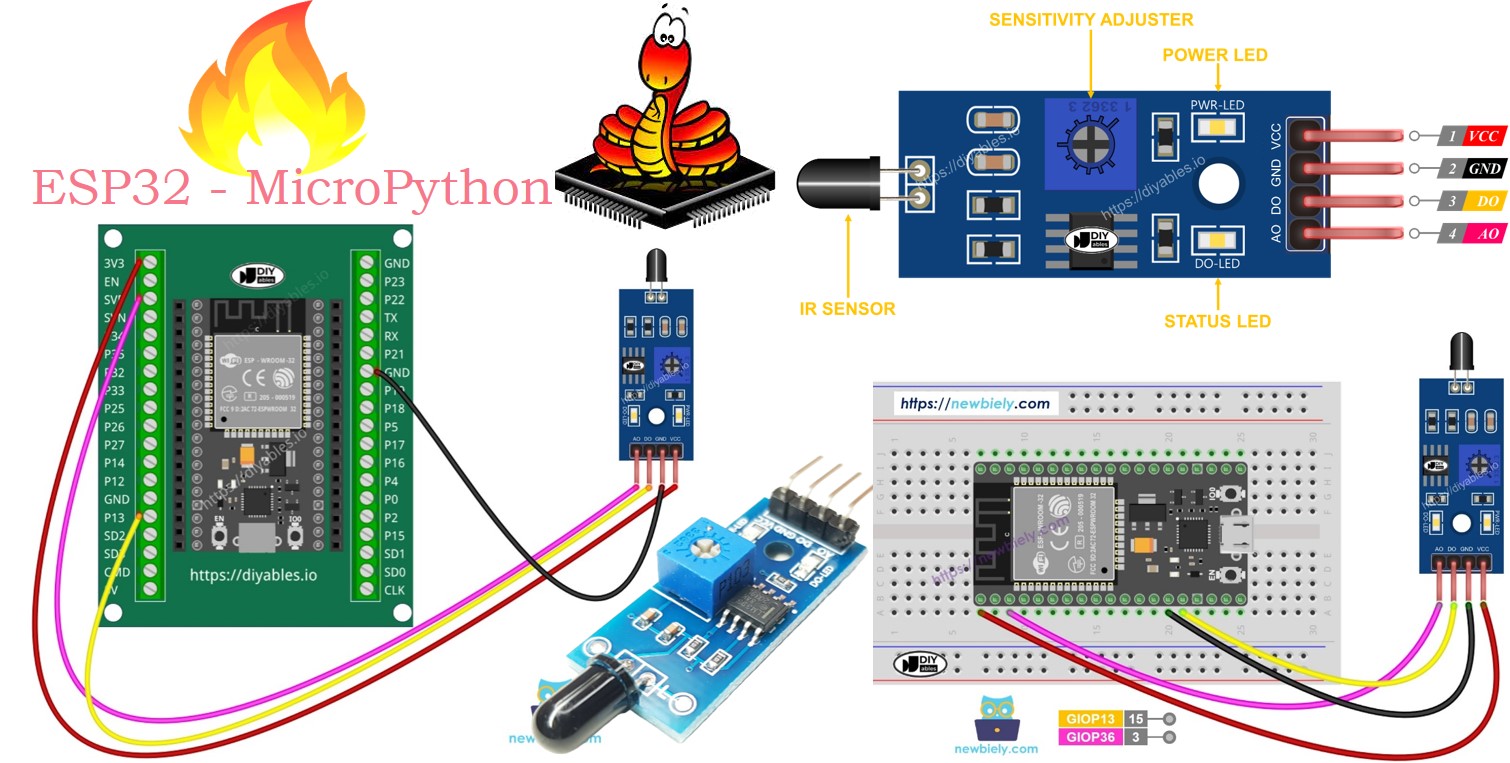
Then, you can adjust the code to activate a warning horn (using a relay) when it detects fire.
Hardware Preparation
1 | × | ESP-WROOM-32 Dev Module | |
1 | × | USB Cable Type-C | |
1 | × | Flame Sensor | |
1 | × | Jumper Wires | |
1 | × | (Recommended) Screw Terminal Expansion Board for ESP32 |
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables.
Overview of Flame Sensor
The flame sensor can detect and measure infrared light emitted by a flame, making it useful for fire detection. Also known as an infrared flame or fire sensor, it provides two types of signals: a digital output (LOW or HIGH) and an analog output.
Infrared flame sensors are specifically designed to detect certain wavelengths of infrared radiation produced by flames, reducing false alarms from other infrared sources like human body heat or lights. However, these sensors may still occasionally trigger false alarms or fail to detect actual flames.
Pinout
The flame sensor has four pins:
- VCC pin: Connect to the power supply (3.3V to 5V).
- GND pin: Connect to ground (0V).
- DO pin: Provides a digital output, which is HIGH when no flame is detected and LOW when a flame is present. The sensitivity to flames can be adjusted using the potentiometer on the board.
- AO pin: Outputs an analog signal, where the value decreases with lower infrared levels and increases with higher infrared levels.
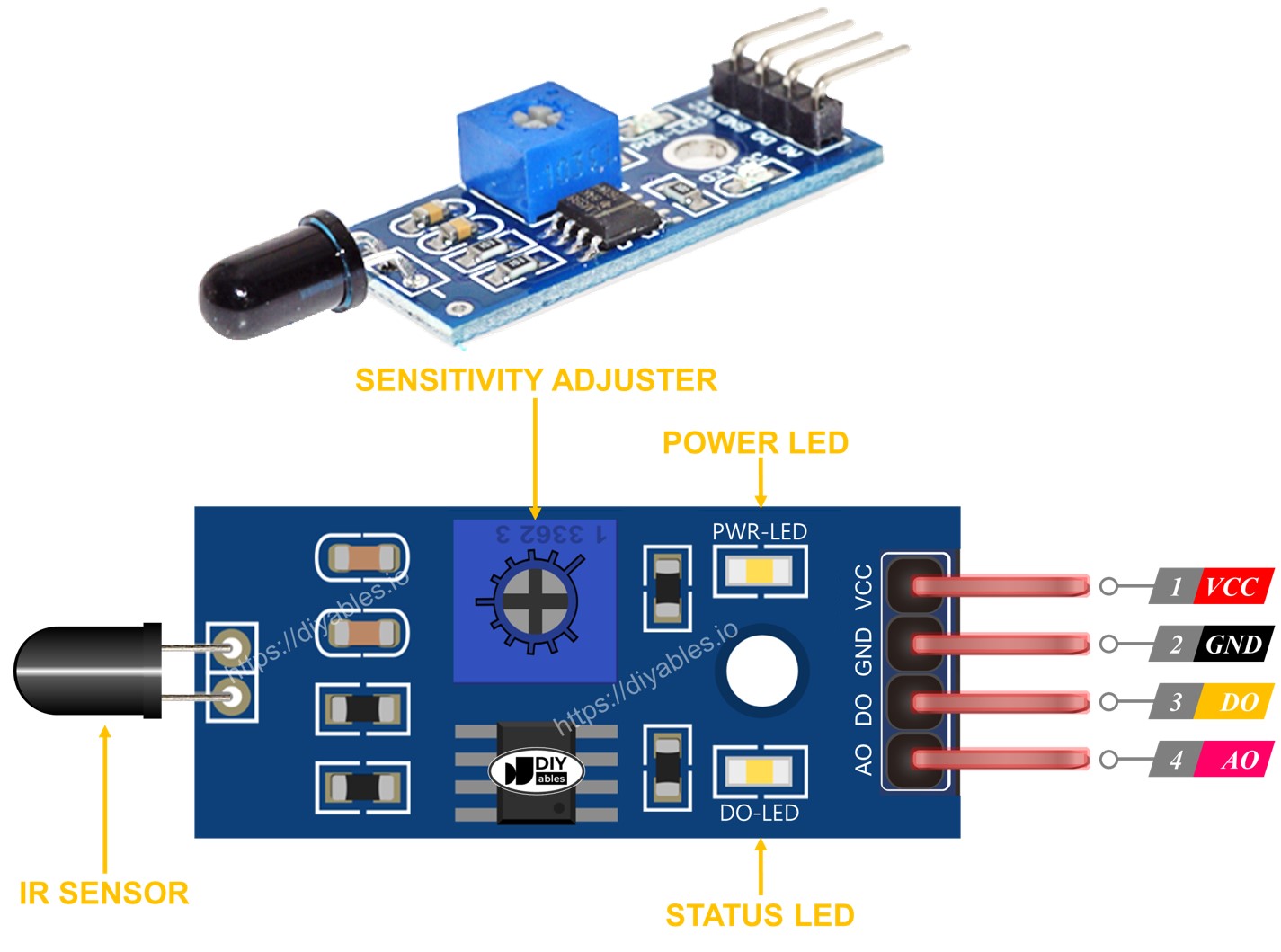
It also features two LED indicators:
- The PWR-LED lights up when the sensor is powered on.
- The DO-LED lights up when a flame is detected.
How It Works
For the DO pin:
- The module includes a potentiometer to adjust the infrared sensitivity threshold.
- When the infrared level exceeds this threshold, indicating a flame, the sensor's output pin goes LOW, and the DO-LED lights up.
- When the infrared level is below the threshold, indicating no flame, the sensor's output pin remains HIGH, and the DO-LED stays off.
For the AO pin:
- A high level of infrared light results in a high reading at the AO pin.
- A low level of infrared light results in a low reading at the AO pin.
- The potentiometer does not affect the value at the AO pin.
Wiring Diagram
- How to connect ESP32 and flame sensor using breadboard
The flame sensor module provides two output options. You can use one or both based on what you need.
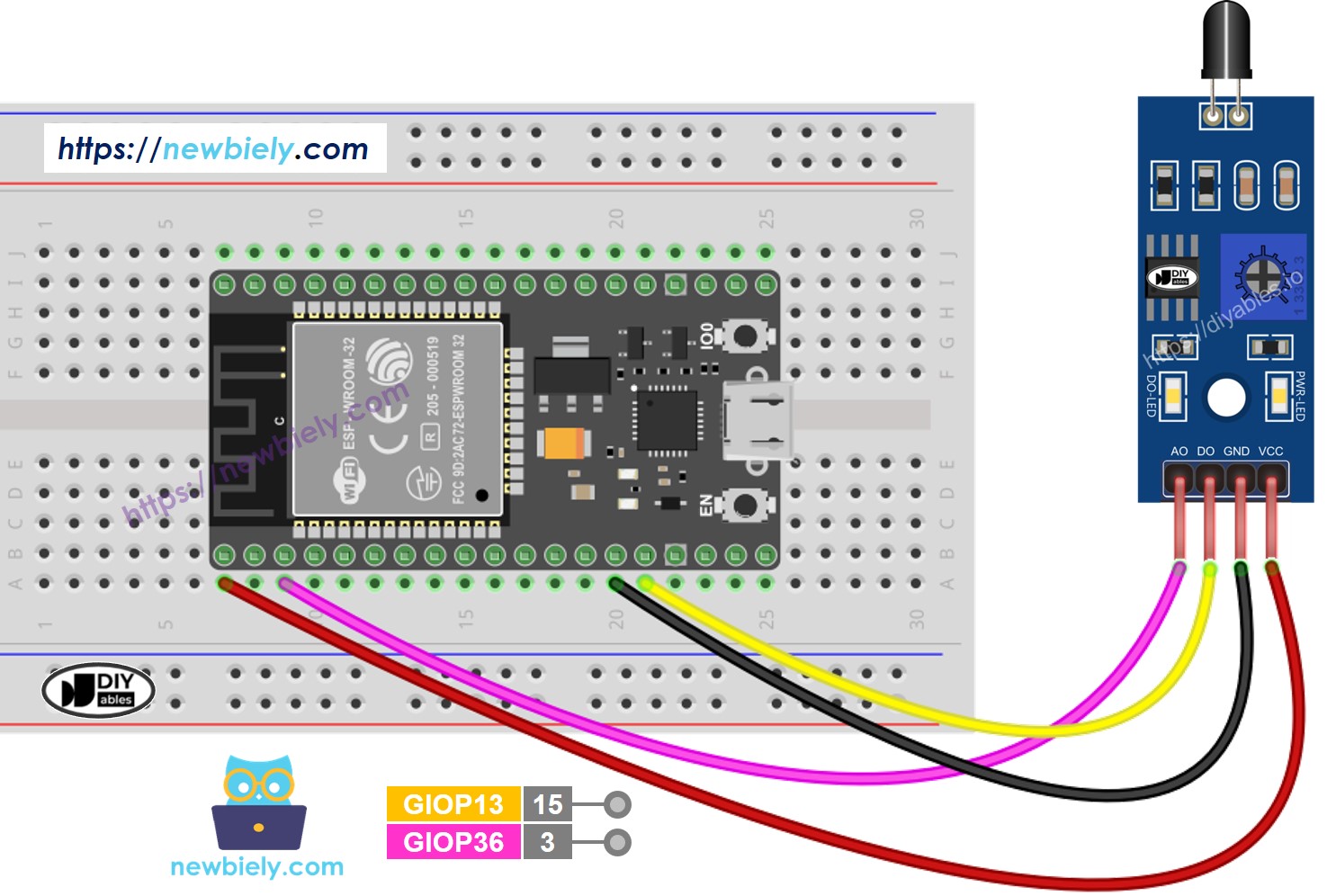
This image is created using Fritzing. Click to enlarge image
- How to connect ESP32 and flame sensor using screw terminal block breakout board
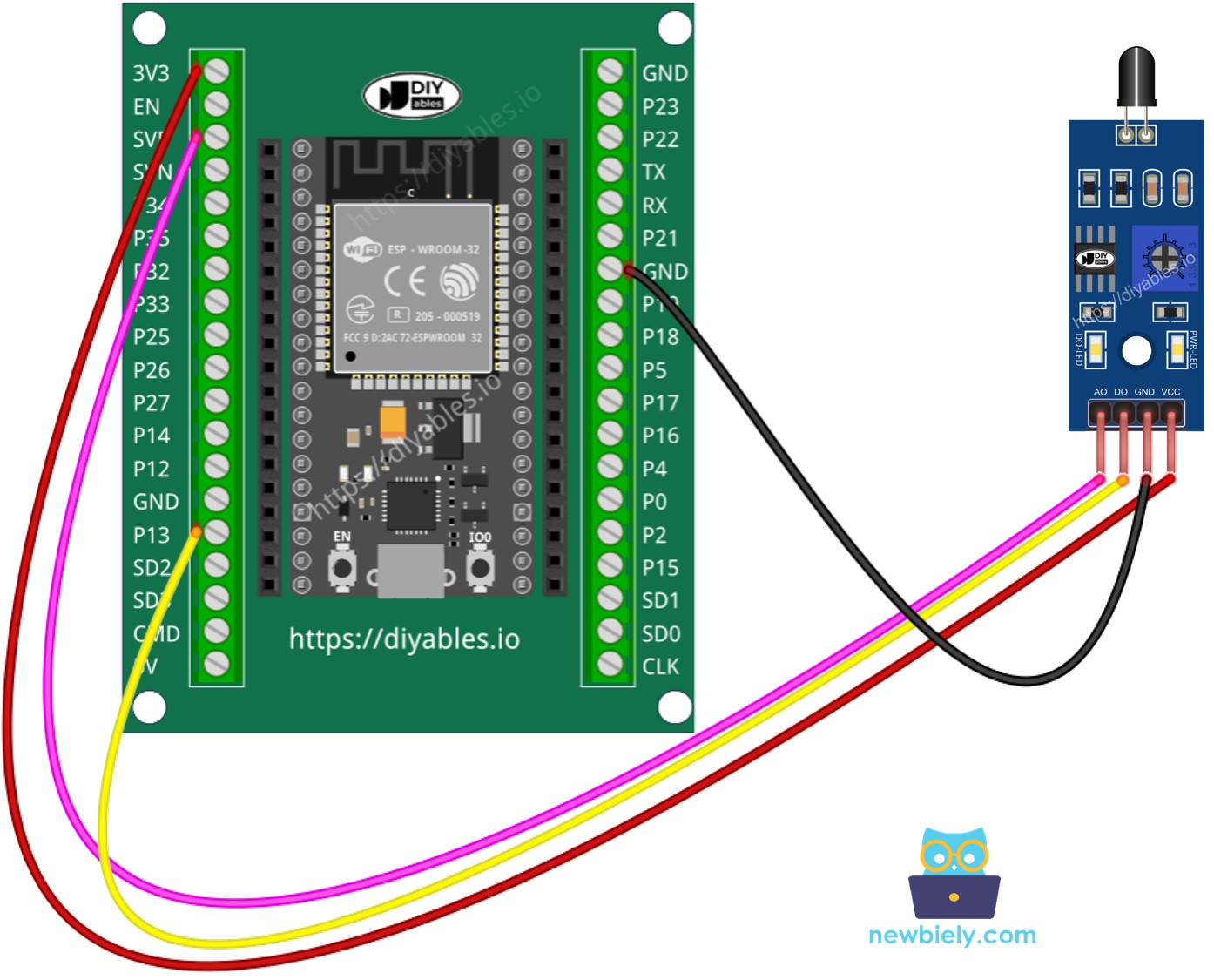
ESP32 MicroPython Code - Read value from DO pin
Detailed Instructions
Here’s instructions on how to set up and run your MicroPython code on the ESP32 using Thonny IDE:
- Make sure Thonny IDE is installed on your computer.
- Confirm that MicroPython firmware is loaded on your ESP32 board.
- If this is your first time using an ESP32 with MicroPython, check out the ESP32 MicroPython Getting Started guide for step-by-step instructions.
- Connect the ESP32 board to the flame sensor according to the provided diagram.
- Connect the ESP32 board to your computer with a USB cable.
- Open Thonny IDE on your computer.
- In Thonny IDE, go to Tools Options.
- Under the Interpreter tab, choose MicroPython (ESP32) from the dropdown menu.
- Make sure the correct port is selected. Thonny IDE usually detects it automatically, but you might need to select it manually (like COM12 on Windows or /dev/ttyACM0 on Linux).
- Copy the provided MicroPython code and paste it into Thonny's editor.
- Save the code to your ESP32 by:
- Clicking the Save button or pressing Ctrl+S.
- In the save dialog, choose MicroPython device.
- Name the file main.py.
- Click the green Run button (or press F5) to execute the script.
- Aim the flame sensor at a flame.
- Check out the message in the Shell at the bottom of Thonny.
If the LED light is always on or off, even when the sensor is facing a flame, you can turn the potentiometer to change the sensor's sensitivity.
ESP32 MicroPython Code - Read value from AO pin
Detailed Instructions
- Copy the provided MicroPython code and paste it into Thonny's editor.
- Save the code to your ESP32.
- Click the green Run button (or press F5) to execute the script.
- Aim the flame sensor at a flame.
- Check out the message in the Shell at the bottom of Thonny.
※ NOTE THAT:
This tutorial demonstrates how to use the adc.read() function to read values from an ADC (Analog-to-Digital Converter) connected to a flame sensor. The ESP32's ADC is suitable for projects that do not require high precision. However, if your project needs accurate measurements, keep the following in mind:
- The ESP32 ADC is not perfectly accurate and may require calibration for precise results. Each ESP32 board may vary slightly, so calibration is necessary for each individual board.
- Calibration can be challenging, especially for beginners, and might not always yield the exact results you desire.
For projects requiring high precision, consider using an external ADC (e.g., ADS1115) with the ESP32 or opt for an Arduino, which has a more reliable ADC. If you still wish to calibrate the ESP32 ADC, refer to the ESP32 ADC Calibration Driver.