ESP32 MicroPython Motion Sensor
This tutorial instructs you how to detect the humman present using an HC-SR501 motion sensor and an ESP32 with MicroPython. In detail, we will learn:
- How the HC-SR501 motion sensor works
- How to write MicroPython code for ESP32 to read the state from the HC-SR501 motion sensor
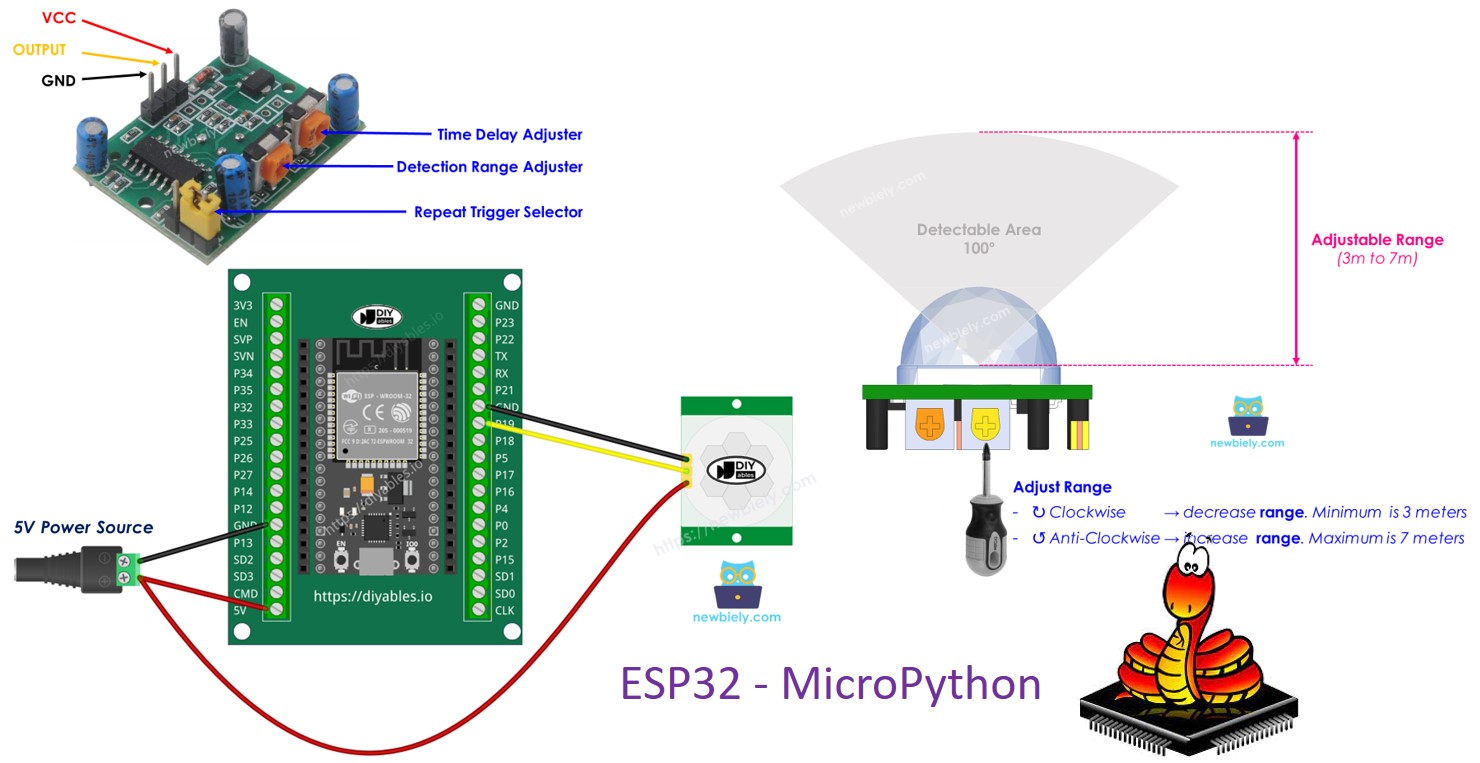
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables ESP32 Starter Kit (ESP32 included) | |
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of HC-SR501 Motion Sensor
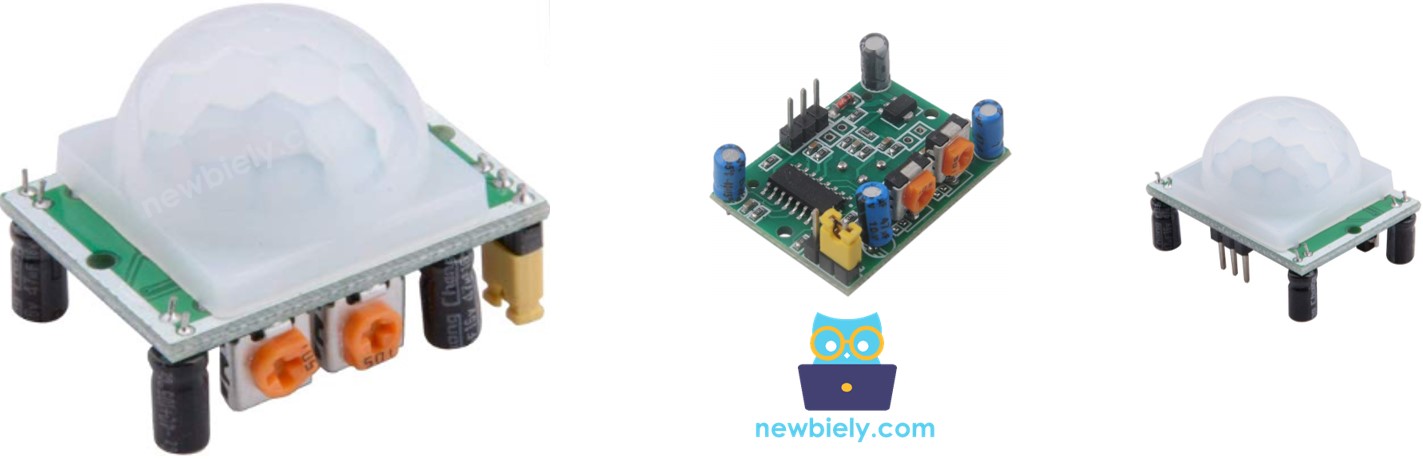
The HC-SR501 PIR sensor is a motion detector that can detect the movement of humans or animals. It's commonly used in various applications, such as:
- Automatic lighting: Switching lights on and off in response to motion.
- Door control: Automatically opening and closing doors.
- *Escalator control: Activating or deactivating escalators.
- Security systems: Detecting intruders.
Pinout
The HC-SR501 motion sensor includes three pins.
- GND pin: Connect to ground (0V).
- VCC pin: Connect to power (5V).
- OUTPUT Pin: Outputs a LOW signal when no movement is detected and a HIGH signal when movement is detected. Connect this pin to an input pin on the ESP32.
The HC-SR501 sensor features one jumper and two potentiometers that allow you to adjust its settings. For more details, refer to the Advanced Uses of Motion Sensor.
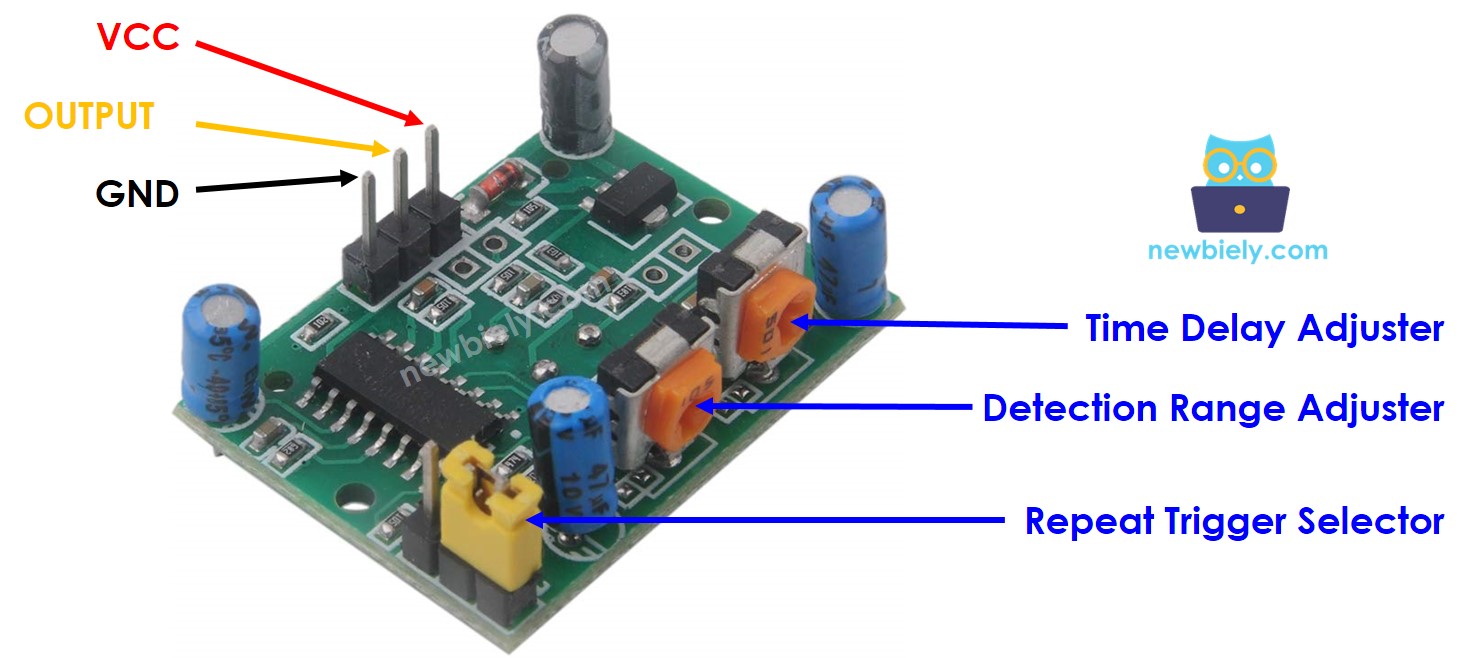
How It Works
The HC-SR501 sensor detects motion by sensing changes in infrared radiation. For the sensor to detect an object, two conditions must be met:
- The object must be moving.
- The object must emit infrared radiation.
Therefore:
- Objects that move but don't emit infrared rays (like some toys) won't be detected.
- Objects that emit infrared rays but don't move (like a still person) won't be detected.
Since humans and animals emit infrared radiation, the sensor can detect their movements.
Output Pin Behavior:
- No motion: When no person or animal is within the sensor's detection range, the OUTPUT pin stays LOW.
- Motion detected: When a person or animal enters the detection range, the OUTPUT pin switches from LOW to HIGH.
- Motion ended: When the person or animal exits the detection range, the OUTPUT pin returns from HIGH to LOW.
The video shows how the motion sensor generally works. In real situations, how it works might change a little based on its settings, as explained in the Advanced Uses section.
ESP32 - HC-SR501 Motion Sensor
When you set up a pin on the ESP32 as a digital input, it can detect whether the connected object is in a LOW or HIGH state.
Connect the ESP32's pin to the OUTPUT pin of the HC-SR501 sensor. Then, program the ESP32 to monitor the OUTPUT pin's value and detect movement.
Wiring Diagram
- How to connect ESP32 and motion sensor using breadboard (powered via USB cable)
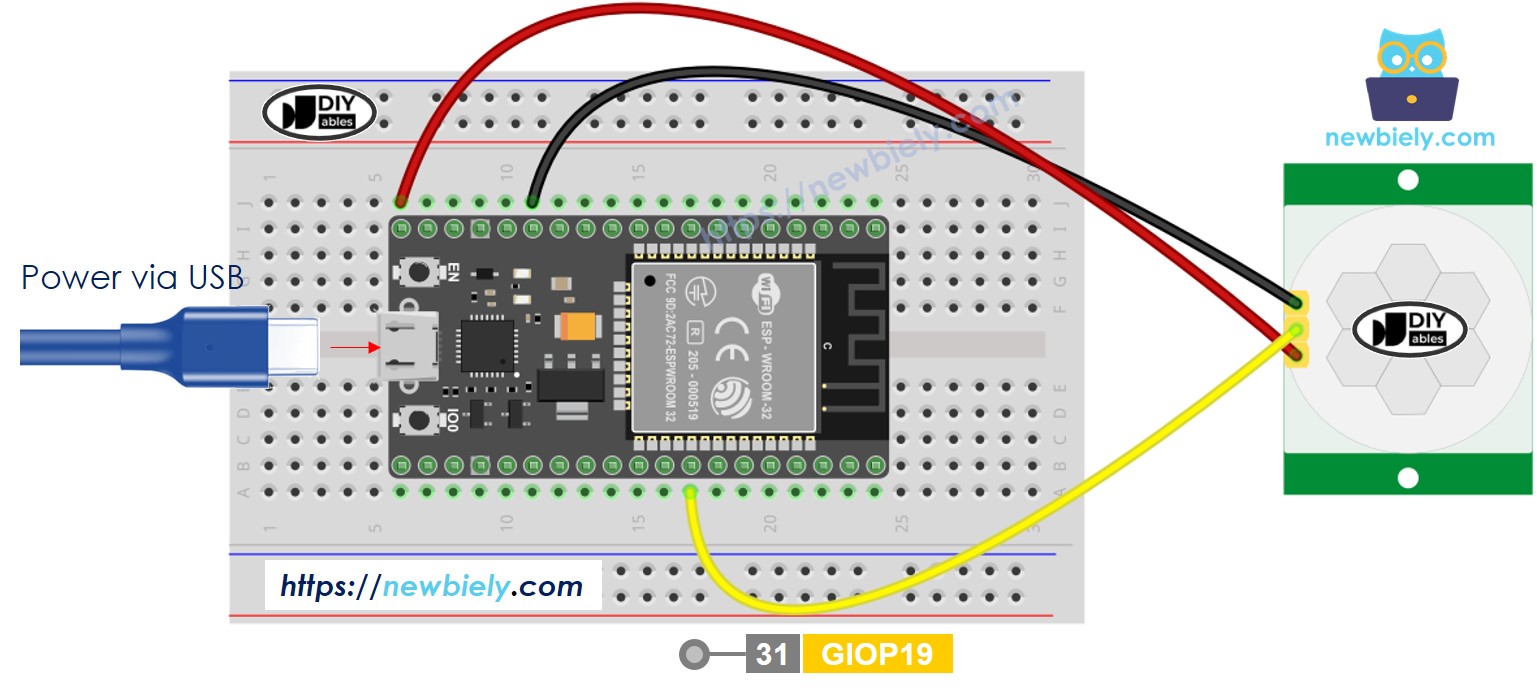
This image is created using Fritzing. Click to enlarge image
- How to connect ESP32 and motion sensor using breadboard (powered via Vin pin)
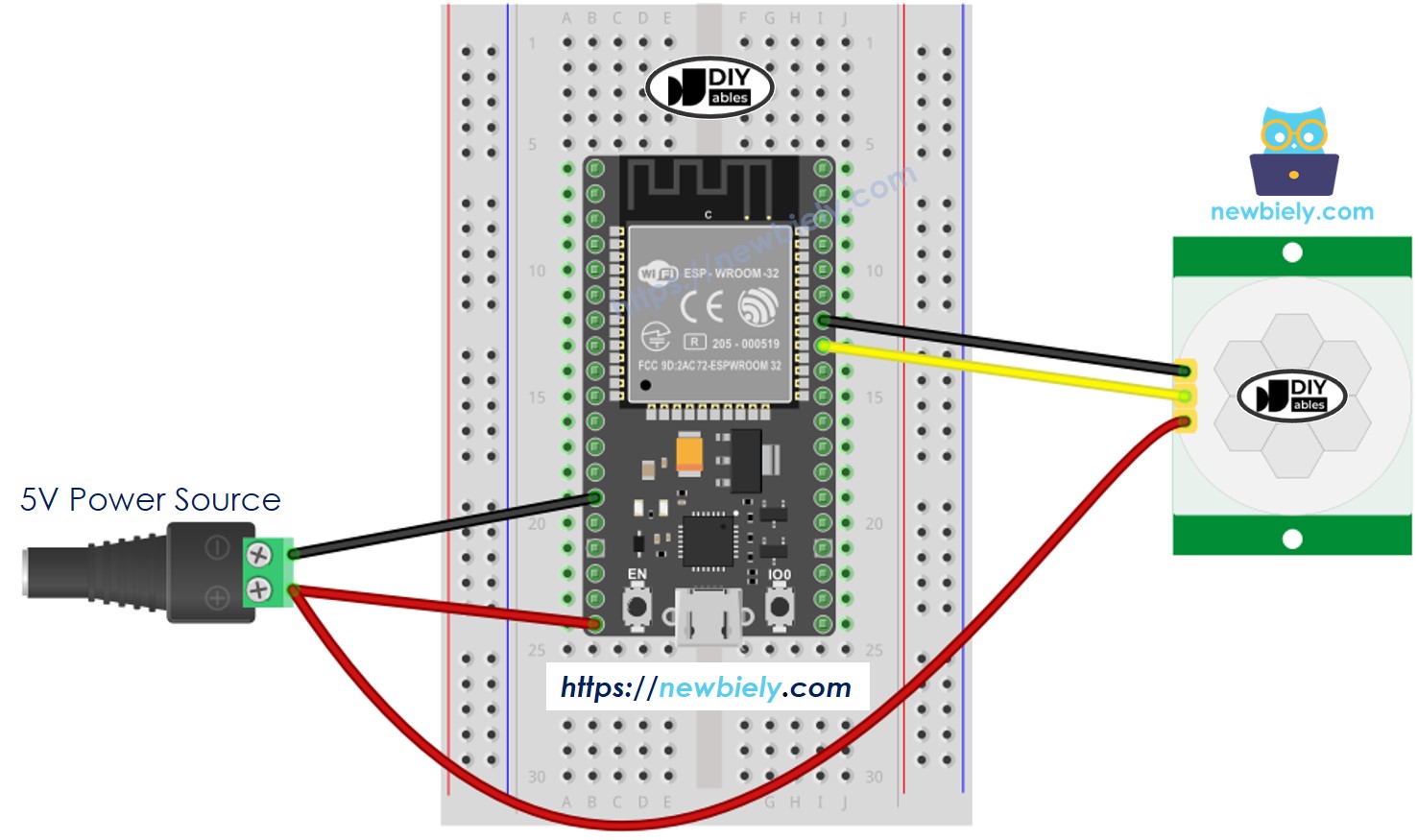
This image is created using Fritzing. Click to enlarge image
- How to connect ESP32 and motion sensor using screw terminal block breakout board (powered via USB cable)
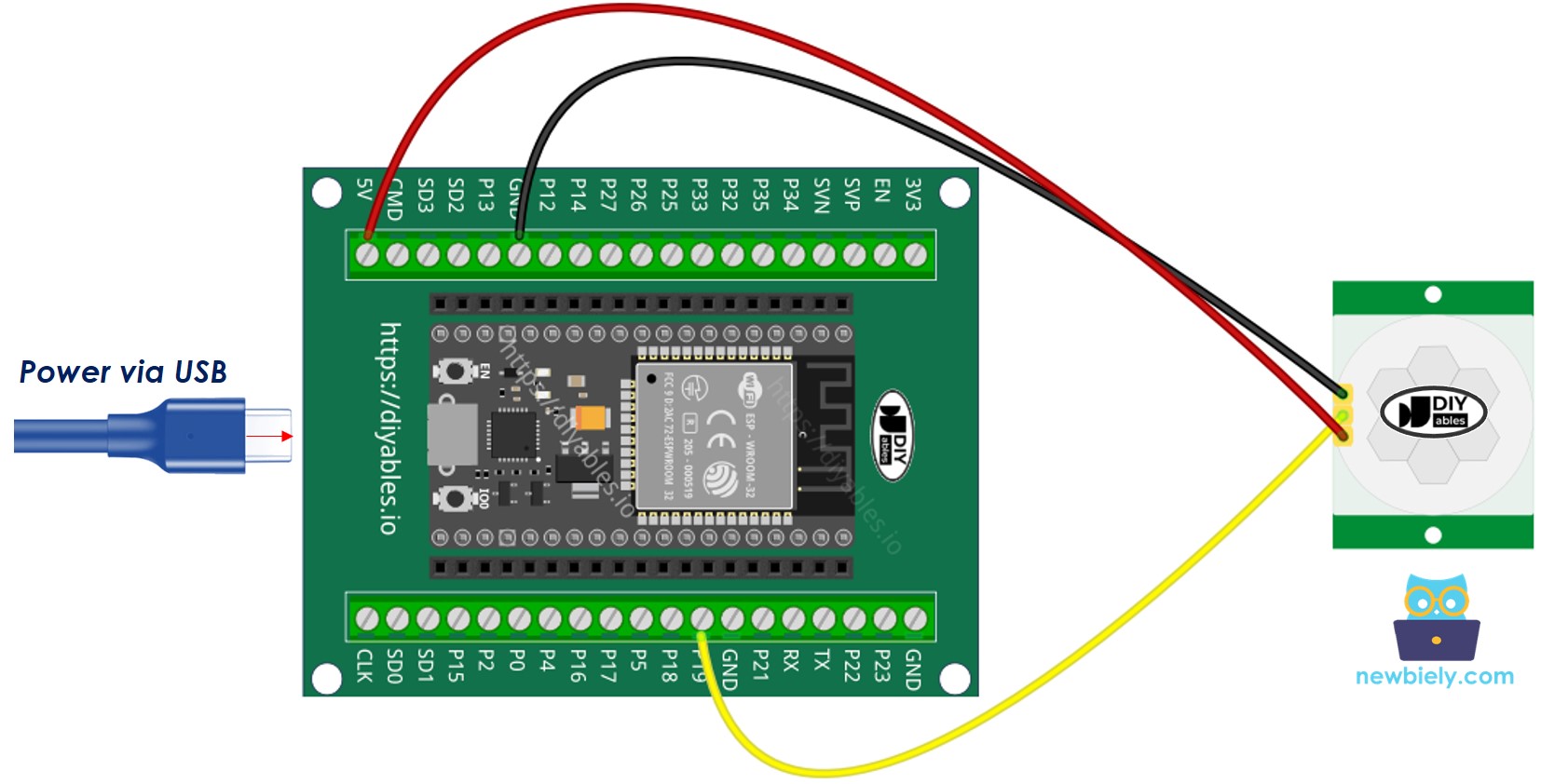
- How to connect ESP32 and motion sensor using screw terminal block breakout board (powered via Vin pin)
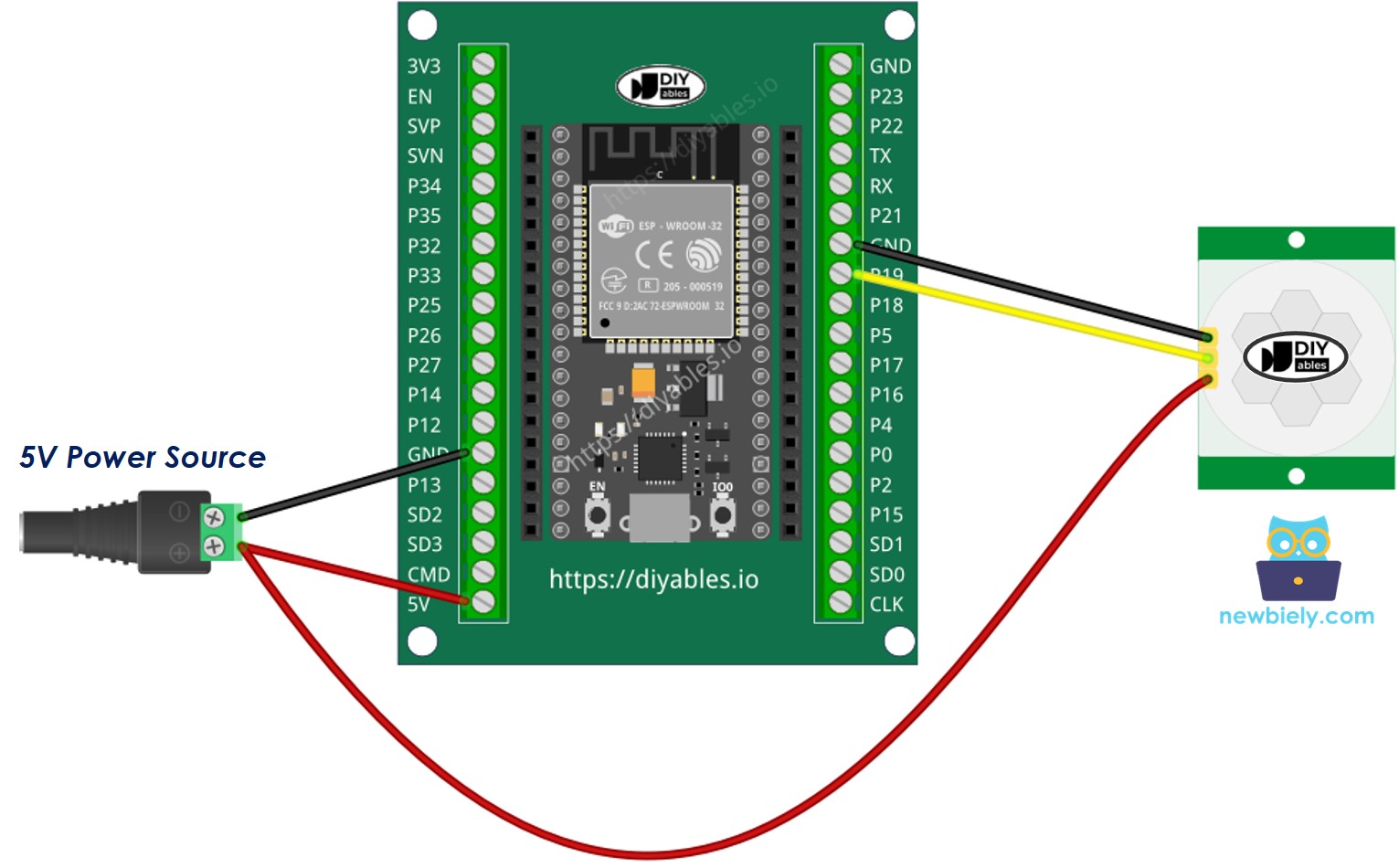
Initial Setting
Time Delay Adjuster | Screw it in anti-clockwise direction fully. |
Detection Range Adjuster | Screw it in clockwise direction fully. |
Repeat Trigger Selector | Put jumper as shown on the image. |
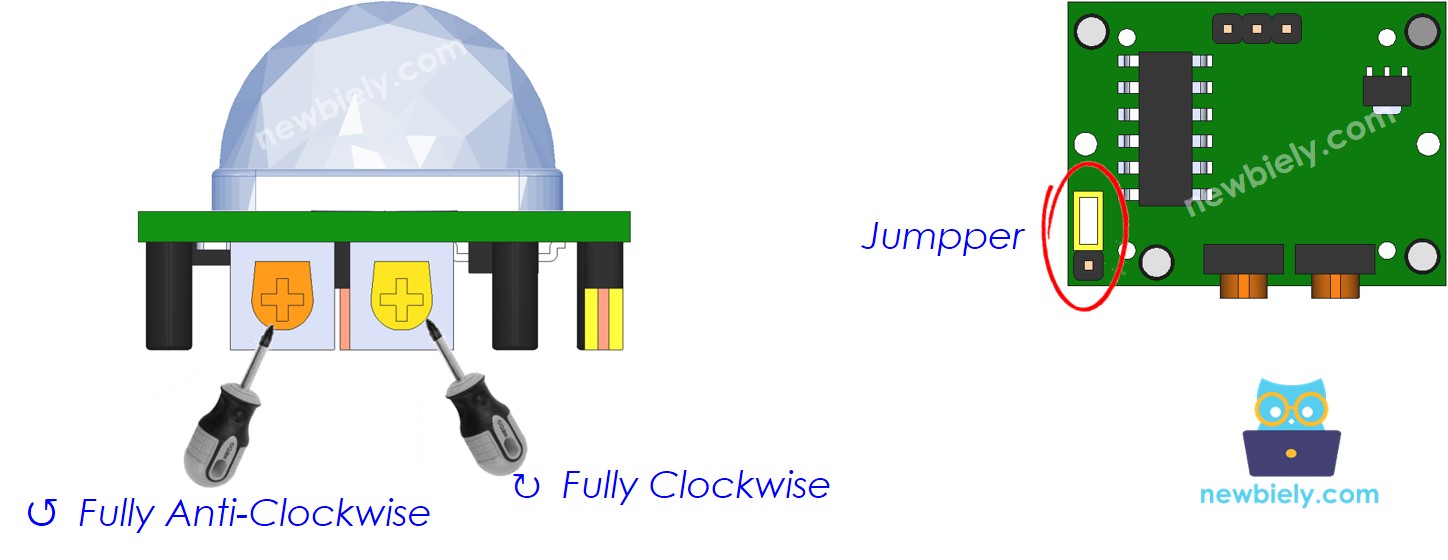
How To Program For Motion Sensor
- Set up an ESP32 pin as a digital input.
- Check the sensor's OUTPUT pin condition.
ESP32 MicroPython Code
Detailed Instructions
Here’s instructions on how to set up and run your MicroPython code on the ESP32 using Thonny IDE:
- Make sure Thonny IDE is installed on your computer.
- Confirm that MicroPython firmware is loaded on your ESP32 board.
- If this is your first time using an ESP32 with MicroPython, check out the ESP32 MicroPython Getting Started guide for step-by-step instructions.
- Connect your ESP32 board to the HC-SR501 motion sensor as shown in the diagram.
- Use a USB cable to connect your ESP32 board to your computer.
- Open Thonny IDE on your computer.
- In Thonny IDE, go to Tools Options.
- Under the Interpreter tab, choose MicroPython (ESP32) from the dropdown menu.
- Make sure the correct port is selected. Thonny IDE usually detects it automatically, but you might need to select it manually (like COM12 on Windows or /dev/ttyACM0 on Linux).
- Copy the provided MicroPython code and paste it into Thonny's editor.
- Save the code to your ESP32 by:
- Clicking the Save button or pressing Ctrl+S.
- In the save dialog, choose MicroPython device.
- Name the file main.py.
- Click the green Run button (or press F5) to execute the script.
- Wave your hand in front of the sensor.
- Check out the message in the Shell at the bottom of Thonny IDE to see the output.