ESP32 MicroPython OLED 128x32
This tutorial instructs you how to use a ESP32 and MicroPython with an OLED 128x32 I2C display. You will learn:
- How to connect a 128x32 OLED display to the ESP32.
- How to write MicroPython code for ESP32 to display text and numbers on the 128x32 OLED screen using ESP32.
- How to write MicroPython code for ESP32 to draw on the 128x32 OLED screen with ESP32.
- How to write MicroPython code for ESP32 to show images on the 128x32 OLED screen using ESP32.
- How to write MicroPython code for ESP32 to align text and numbers in the middle of the 128x32 OLED screen.
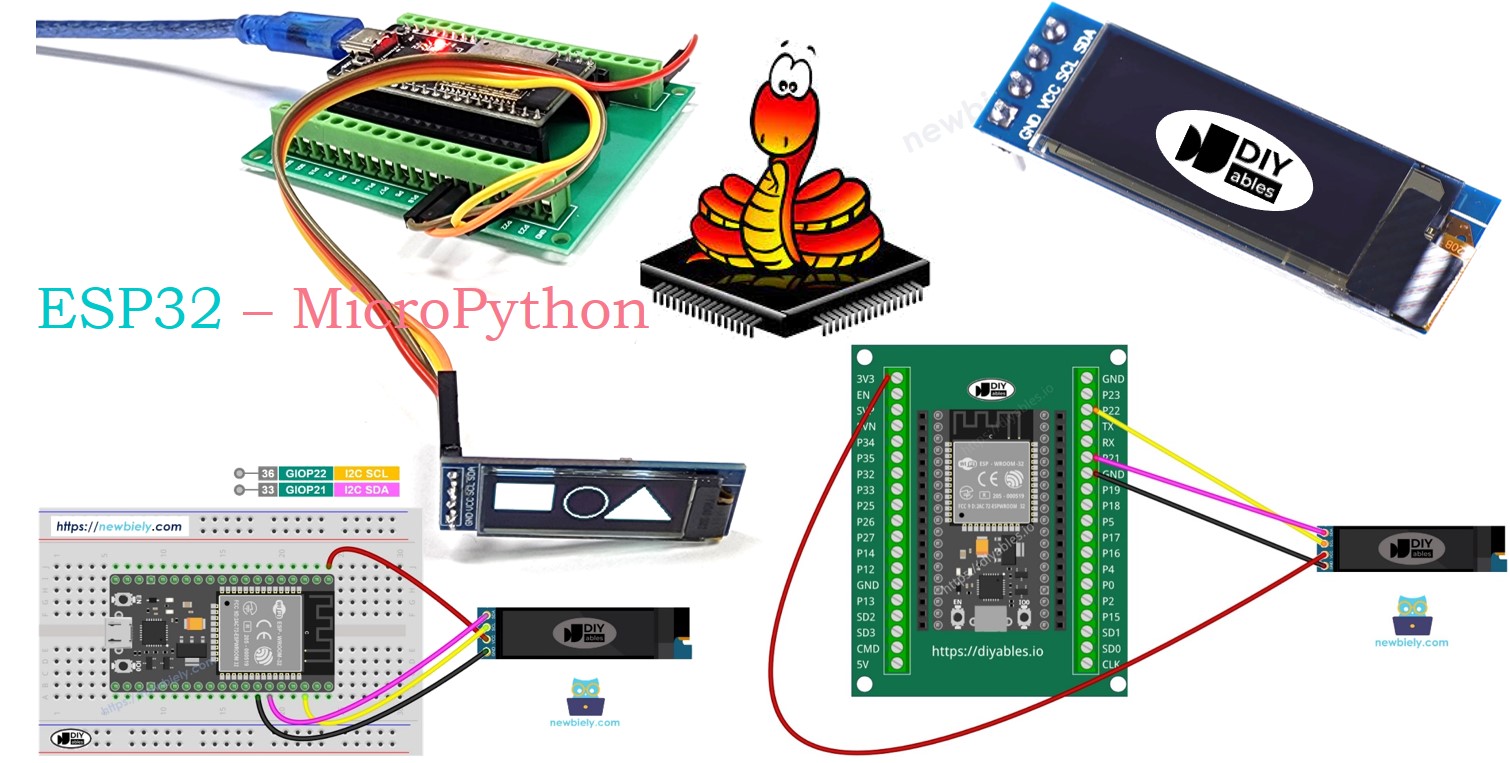
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables ESP32 Starter Kit (ESP32 included) | |
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of 128x32 I2C OLED Display
128x32 I2C OLED Display Pinout
- GND pin: Connect to ESP32's ground.
- VCC pin: Connect to the 5 volts pin on ESP32 for power.
- SDA pin: This is the data pin for I2C communication.
- SCL pin: This is the clock pin for I2C communication.
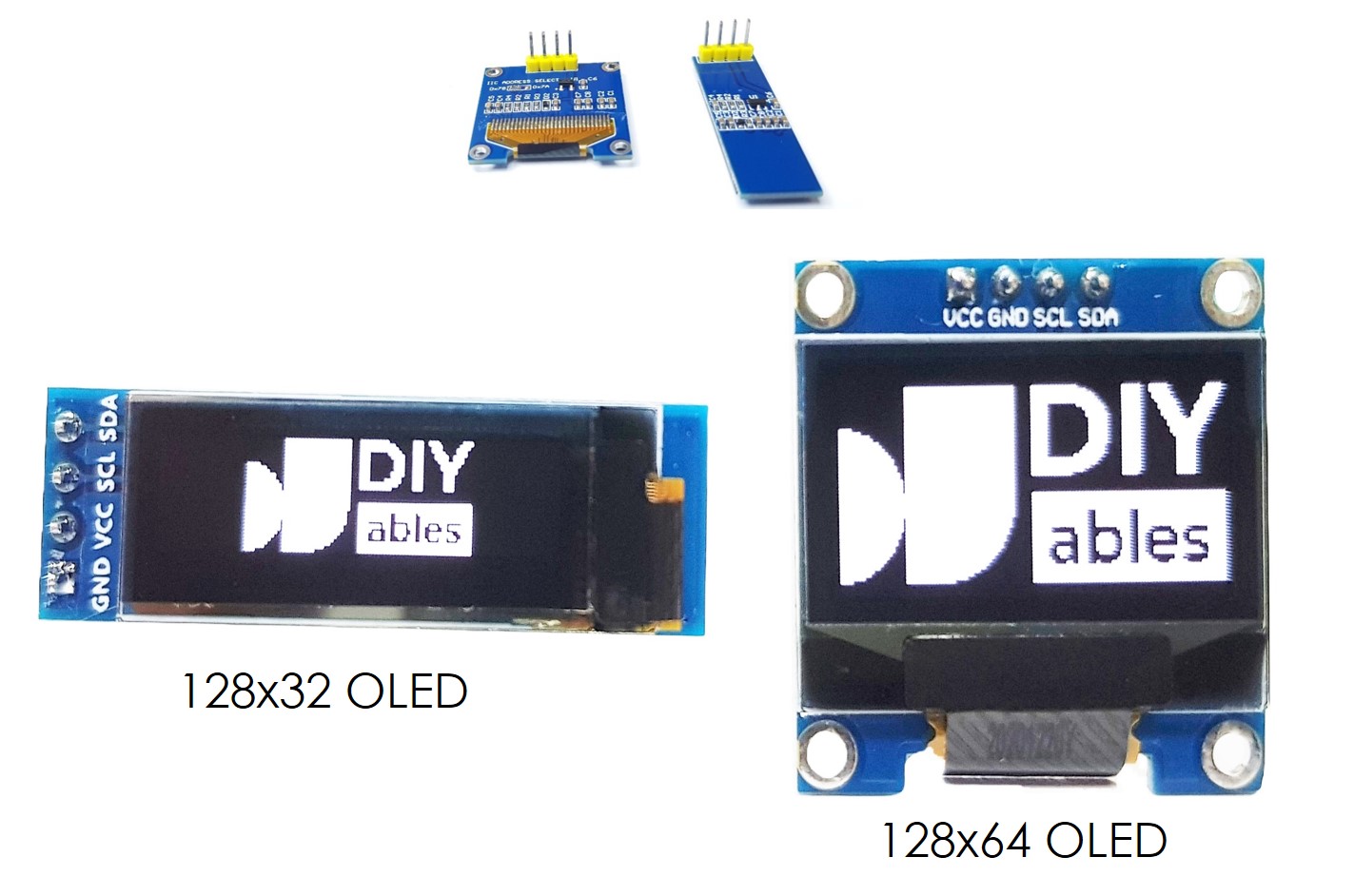
Wiring Diagram
- How to connect ESP32 and oled 128x32 using breadboard
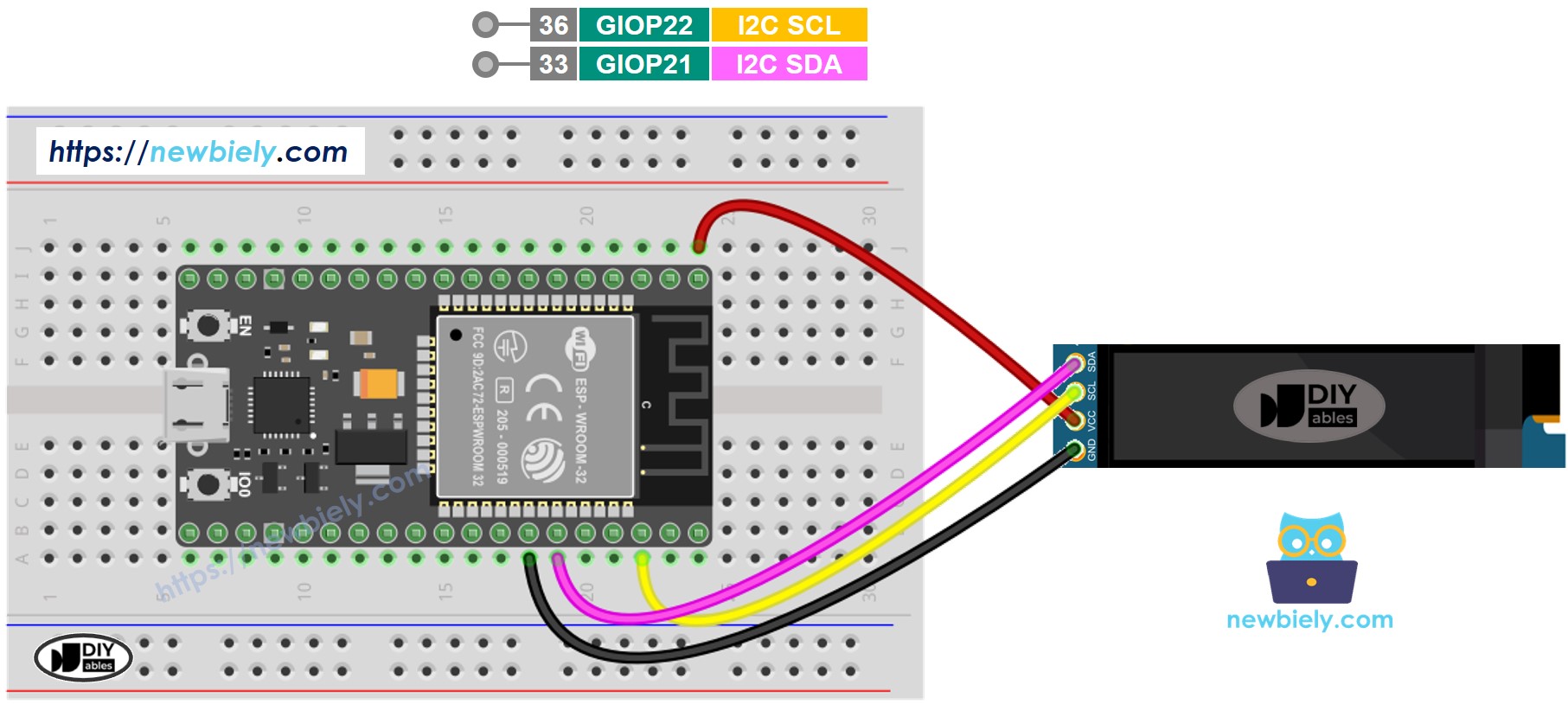
This image is created using Fritzing. Click to enlarge image
- How to connect ESP32 and oled 128x32 using screw terminal block breakout board
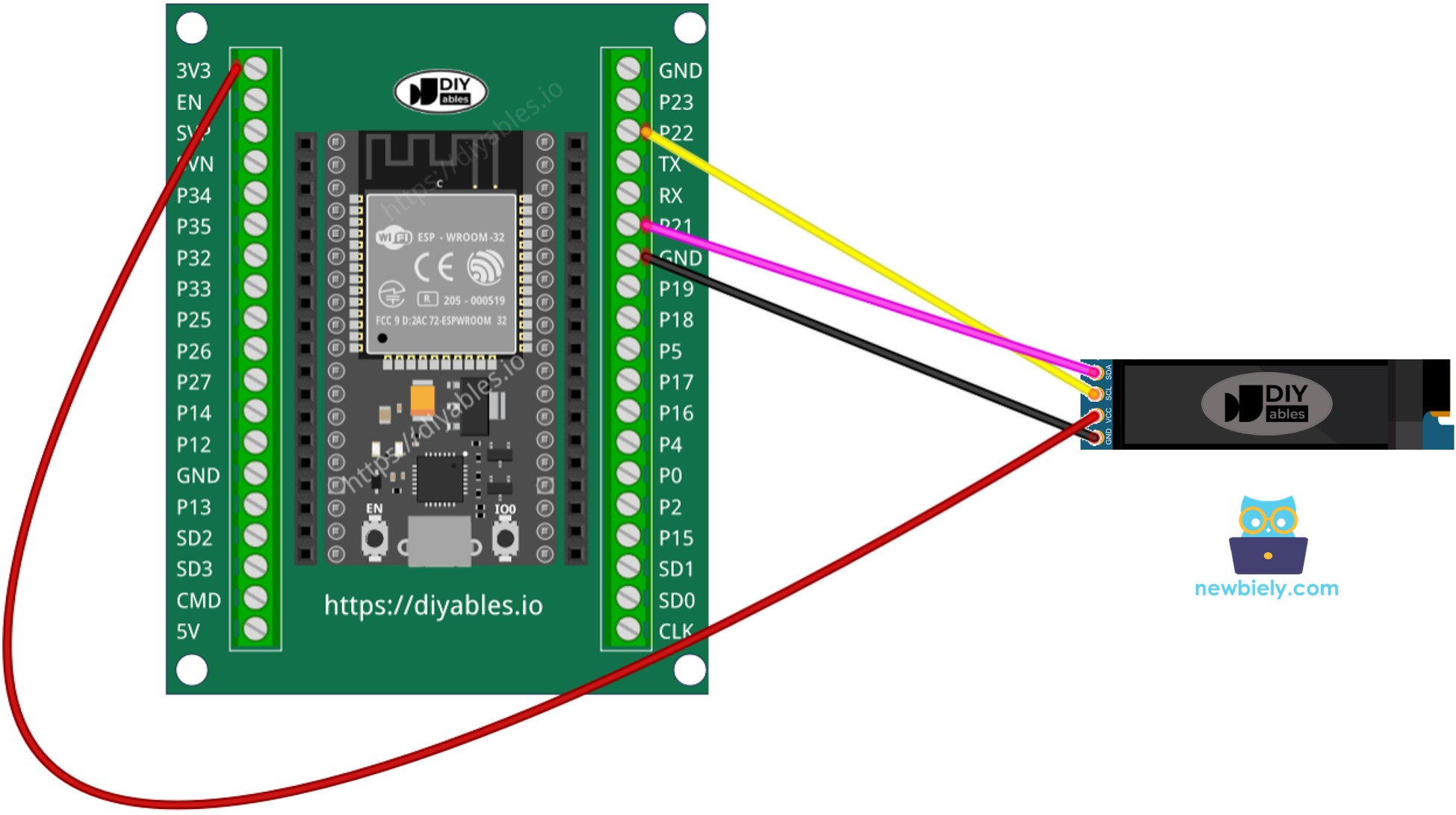
The below is the wiring table between 128x32 OLED Module and ESP32
128x32 OLED Module | ESP32 |
---|---|
VCC | 3.3V |
GND | GND |
SDA | GPIO21 |
SCL | GPIO22 |
ESP32 MicroPython Code - Display Text, Integer and Float Number on OLED
Detailed Instructions
Please follow these instructions step by step:
- Ensure that Thonny IDE is installed on your computer.
- Ensure that MicroPython firmware is installed on your ESP32 board by.
- If this is your first time using a ESP32 with MicroPython, refer to the ESP32 MicroPython Getting Started tutorial for detailed instructions.
- Connect the OLED display to the ESP32 according to the provided diagram.
- Connect the ESP32 board to your computer with a USB cable.
- Open Thonny IDE on your computer.
- In Thonny IDE, go to Tools Options.
- Under the Interpreter tab, choose MicroPython (ESP32) from the dropdown menu.
- Make sure the correct port is selected. Thonny IDE usually detects it automatically, but you might need to select it manually (like COM12 on Windows or /dev/ttyACM0 on Linux).
- Navigate to the Tools Manage packages on the Thonny IDE.
- Search “DIYables-MicroPython-OLED”, then find the OLED library created by DIYables.
- Click on DIYables-MicroPython-OLED, then click Install button to install OLED library.
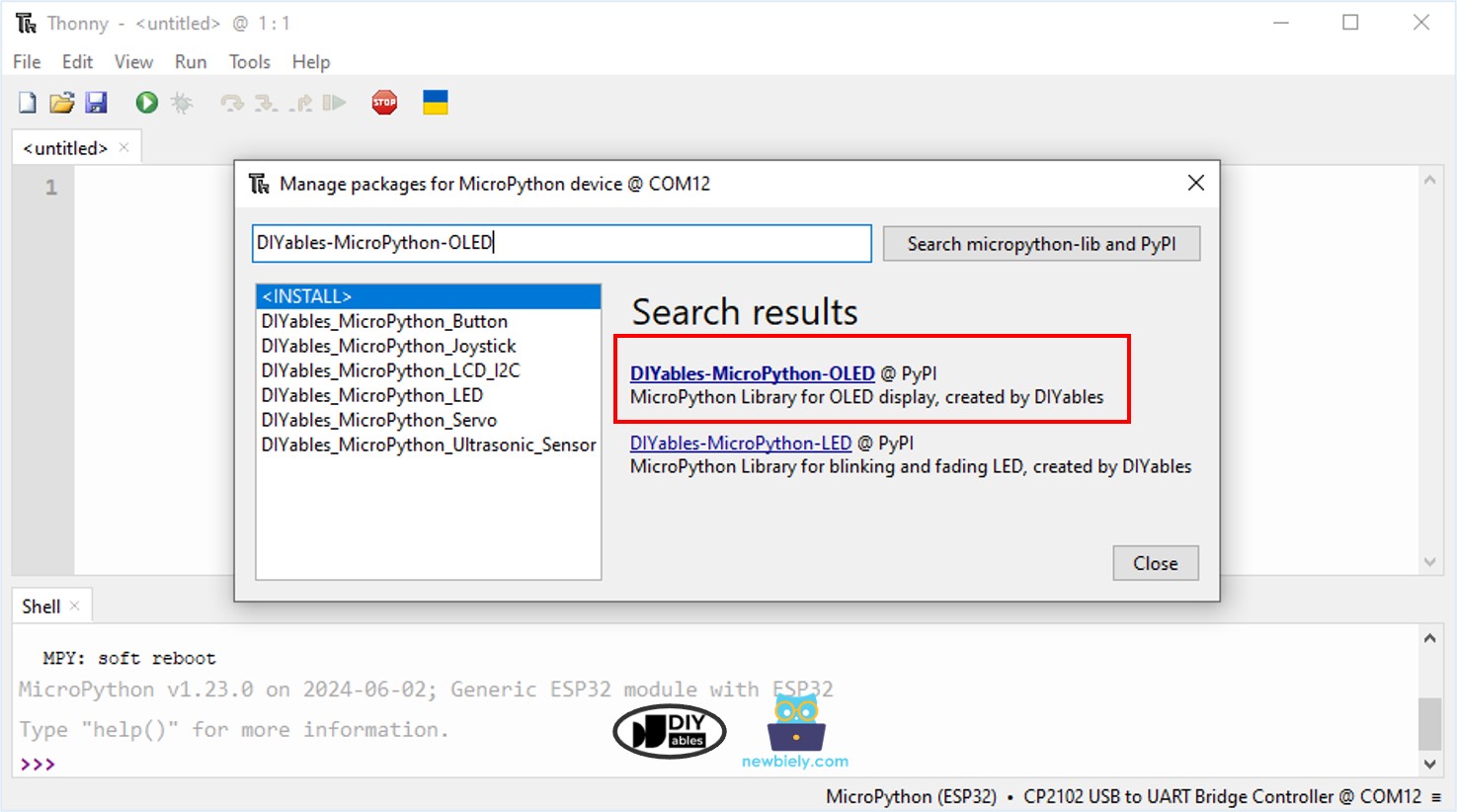
- Copy the above code and paste it to the Thonny IDE's editor.
- Save the script to your ESP32 board by:
- Click the Save button, or use Ctrl+S keys.
- In the save dialog, you will see two sections: This computer and MicroPython device. Select MicroPython device
- Save the file as main.py
- Click the green Run button (or press F5) to run the script. The script will execute.
- Check out on the OLED display. It looks like the below:
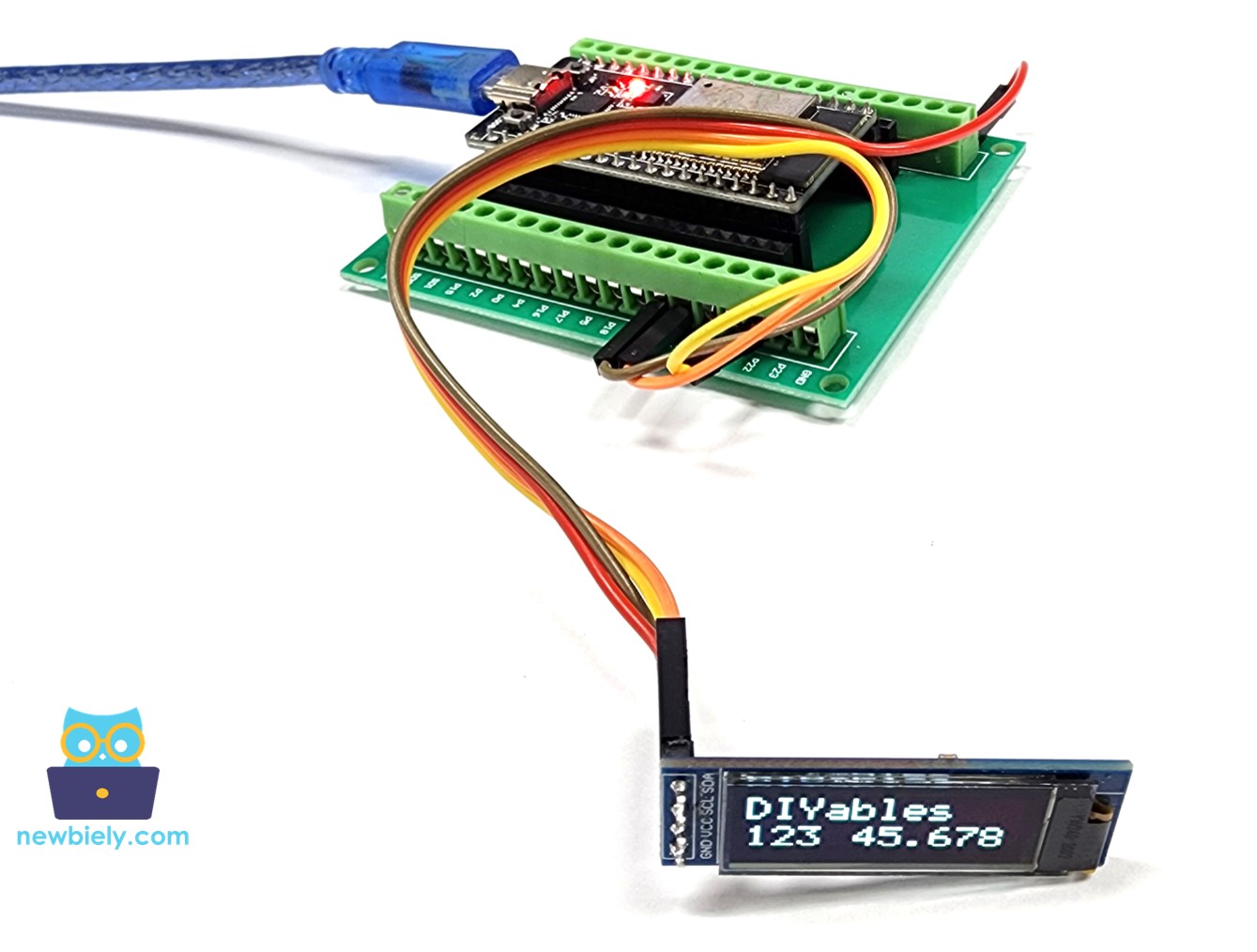
ESP32 MicroPython Code - Drawing on OLED
When you run the code above, a rectangle, circle, and triangle will appear on the OLED screen, as demonstrated below.
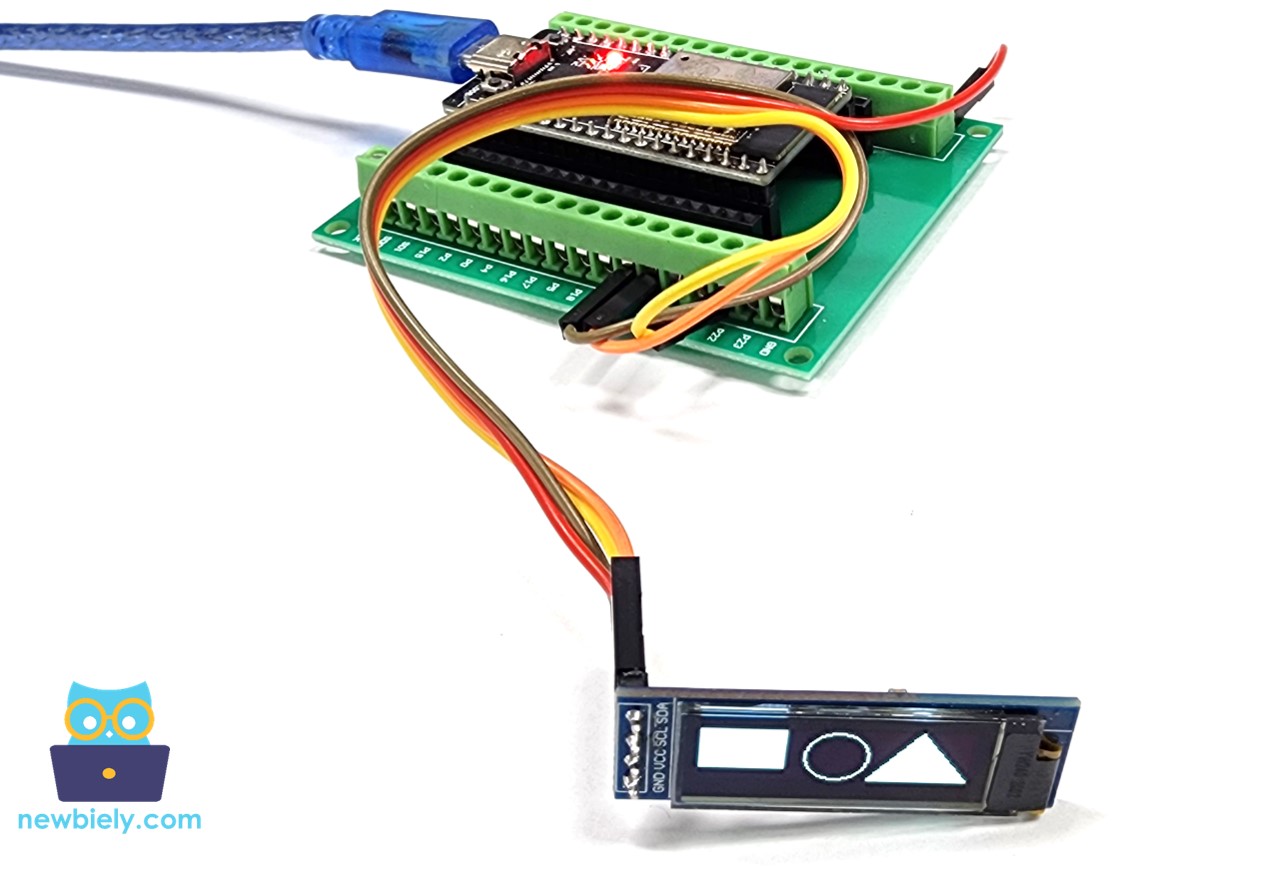
ESP32 MicroPython Code – Display Image on OLED
The below code draws an image to LCD display. The image is DIYables icon.
When you run the code above, the image will appear on the OLED screen, as shown below.
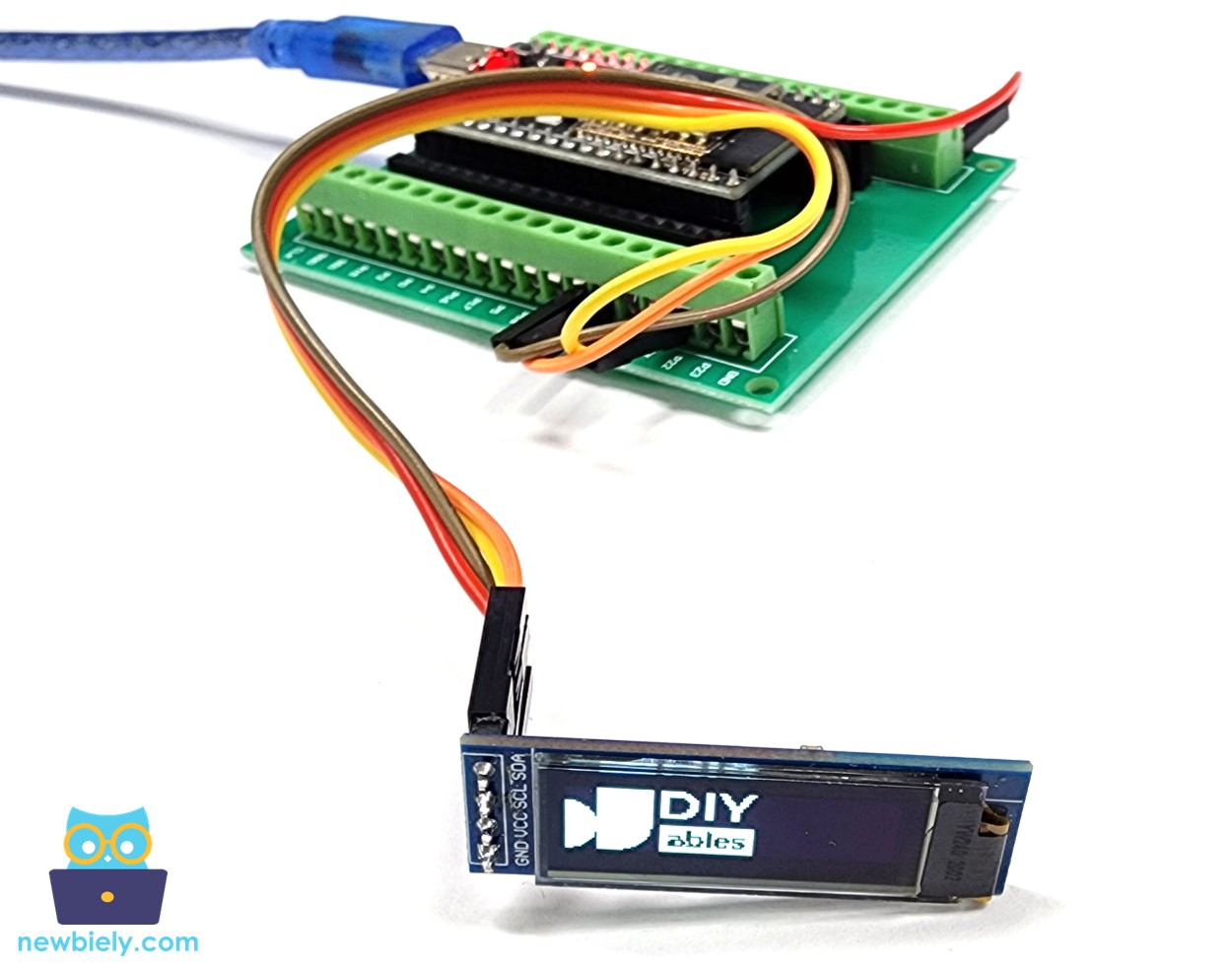
To display a different image on the OLED screen, follow these steps:
- Convert the image (in any format) to a bitmap array. You can use this online tool for conversion. Refer to the image below for guidance on how to convert an image to a bitmap array. In this example, I converted the ESP32 icon into a bitmap array.
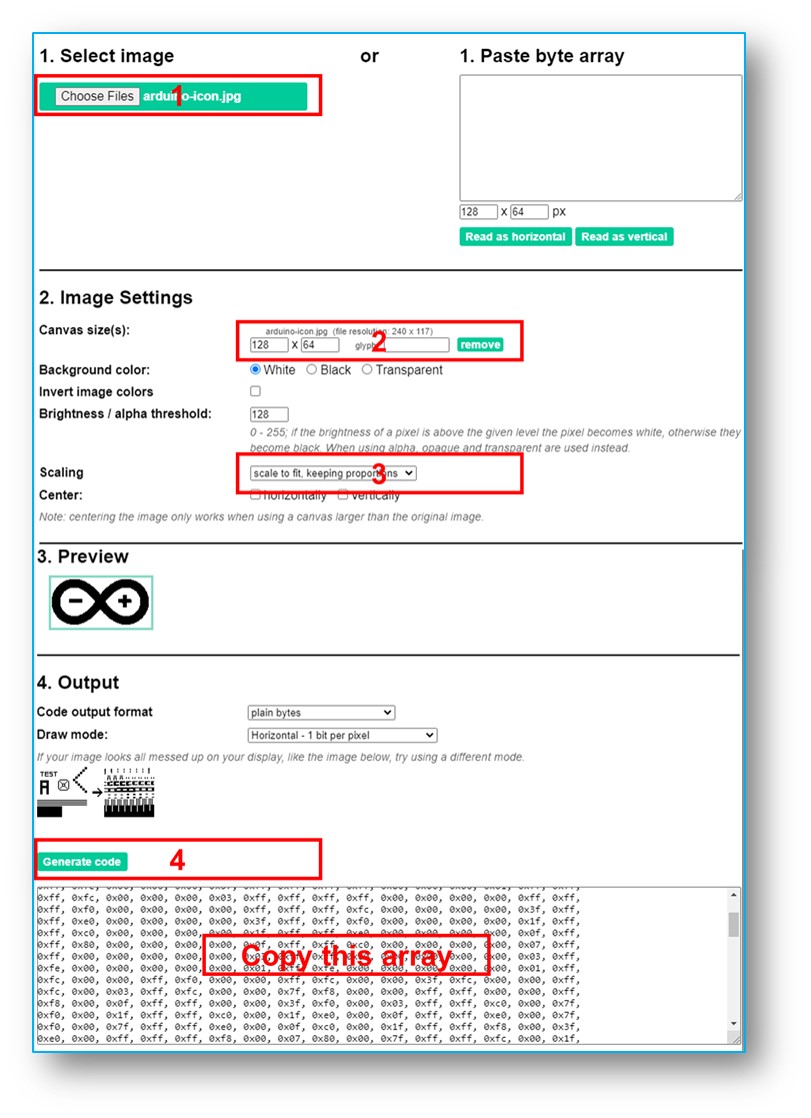
- Replace the existing bitmap array in your ESP32 MicroPython code with the newly converted array.
- Adjust the image width and height in your ESP32 MicroPython code to match the dimensions of the new image.
Note: Ensure the image size is equal to or smaller than the OLED screen size.
How to automatically vertical and horizontal center align text/number on OLED
The MicroPython code below automatically centers the text both vertically and horizontally on the OLED screen.
After running the code, the text will be centered both vertically and horizontally on the OLED screen.
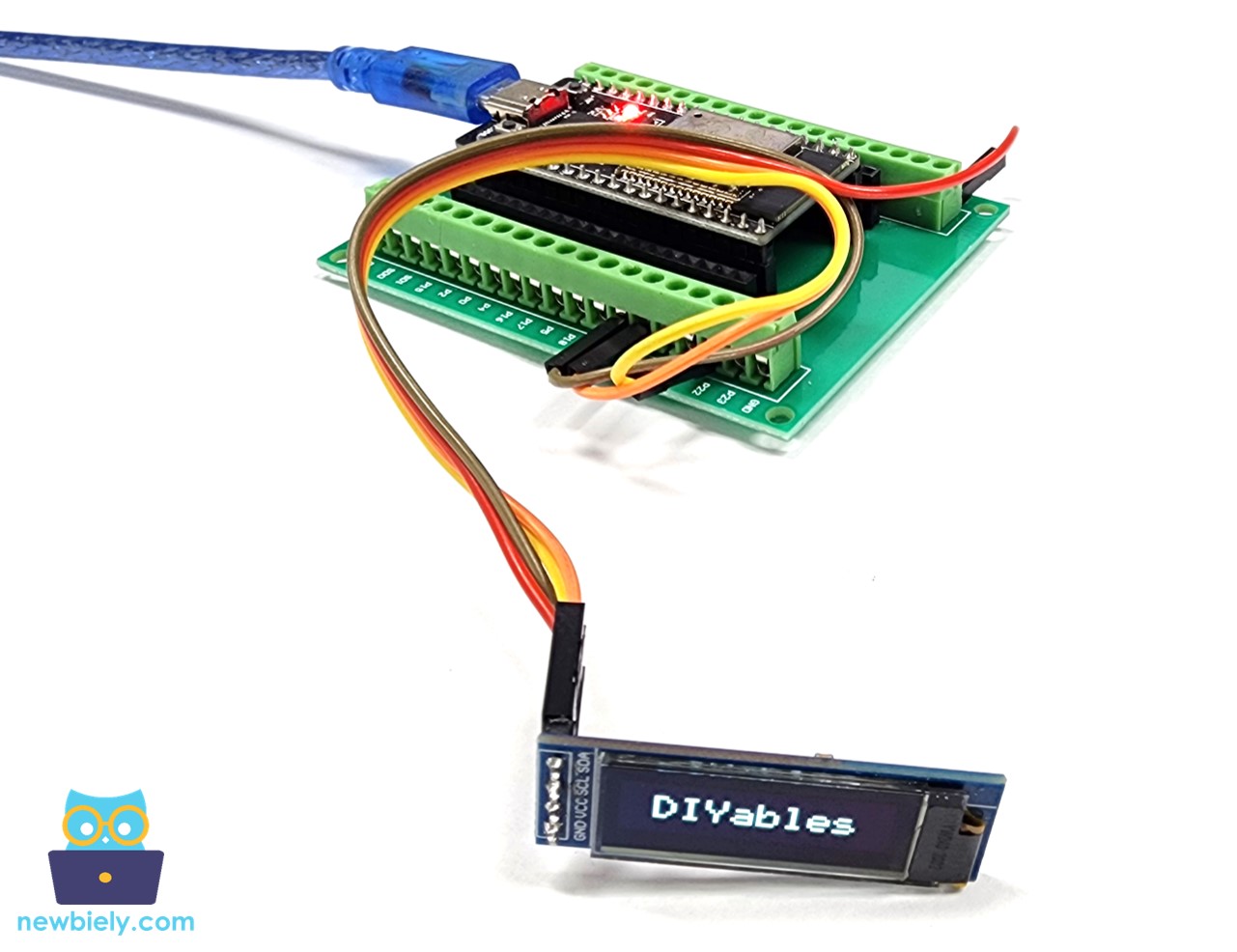