ESP32 MicroPython Gas Sensor
This tutorial instructs you how to monitor air quality using a ESP32 and MQ2 gas sensor with MicroPython programming. This allows to measure levels of various flammable gases such as LPG, smoke, alcohol, propane, hydrogen, methane, and carbon monoxide. In detail, we will learn:
- How to connect the MQ2 gas sensor with ESP32
- How to write MicroPython code for ESP32 to read data from the MQ2 gas sensor
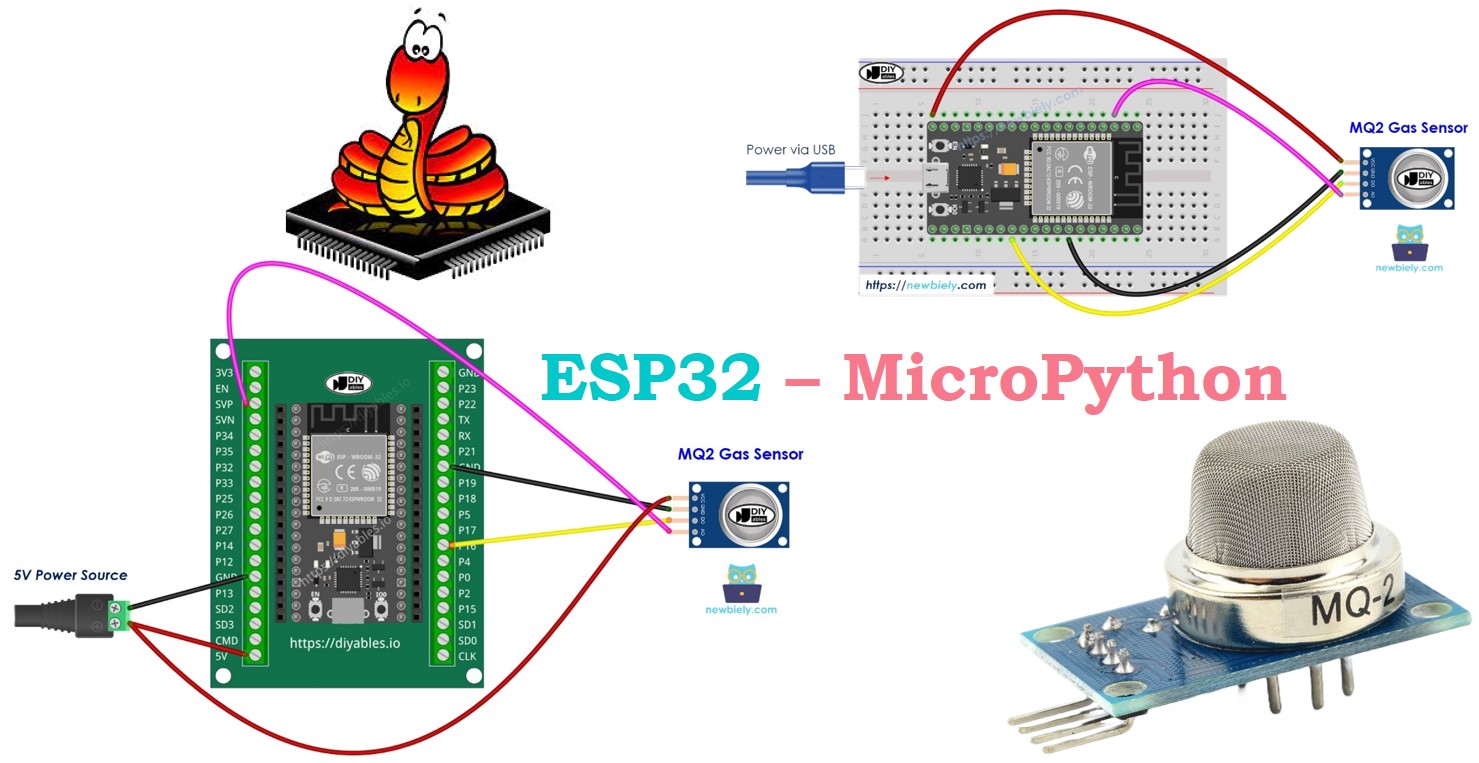
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables ESP32 Starter Kit (ESP32 included) | |
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of MQ2 Gas Sensor
The MQ2 gas sensor can detect various gases, including LPG, smoke, alcohol, propane, hydrogen, methane, and carbon in the environment. It features both a digital output pin and an analog output pin for sending signals.
The MQ2 sensor does not provide specific information about individual gases but indicates the presence of a mixture or multiple gases at once.
We can use the MQ2 sensor to detect gas leaks or monitor poor air quality, enabling appropriate actions like triggering an alarm or activating air systems.
Pinout
Here is the revised version:
The MQ2 gas sensor has four pins:
- VCC pin: Connect this pin to the VCC (5V).
- GND pin: Connect this pin to the GND (0V).
- DO pin: This is the digital output pin. It goes LOW when flammable gases are detected and HIGH when no gases are present. You can adjust the detection threshold using a small adjustable component.
- AO pin: This is the analog output pin, which outputs a voltage that varies based on the gas concentration. Higher gas levels increase the voltage, while lower gas levels decrease it.
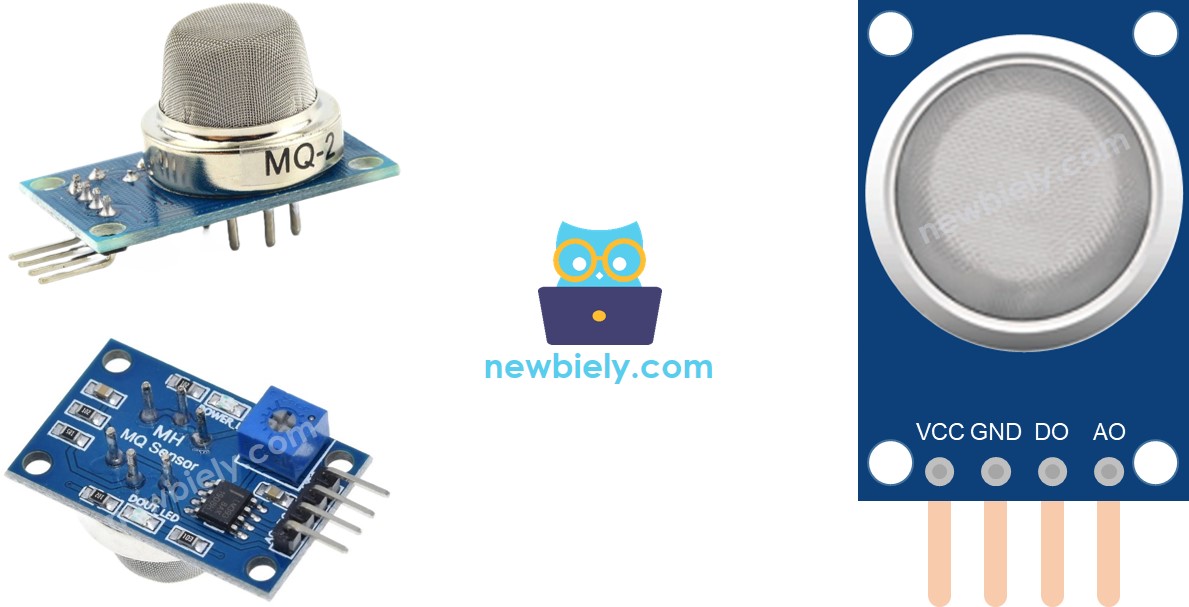
The sensor also has two LED indicators:
- PWR-LED: Indicates that the sensor is powered on.
- DO-LED: Lights up when gas is detected according to the DO pin value, and turns off when no gas is detected.
How It Works
Regarding the DO pin:
- The module has a knob to adjust its sensitivity to gas levels.
- When the gas level exceeds the set threshold, the sensor's output pin goes LOW, and the DO-LED light turns on.
- When the gas level is below the set threshold, the sensor's output pin goes HIGH, and the DO-LED light turns off.
For the AO pin:
- As the gas concentration increases, the voltage rises.
- As the gas concentration decreases, the voltage falls.
The potentiometer does not affect the value on the AO pin.
The MQ2 Sensor Warm-up
The MQ2 gas sensor requires a warm-up period before use. If the sensor has not been used for over a month, allow it to warm up for 24-48 hours to ensure accurate readings. If it has been used recently, a warm-up of 5-10 minutes is sufficient. Initially, the readings may be high, but they will stabilize over time.
To warm up the MQ2 sensor, connect its VCC and GND pins to a power source or to the VCC and GND pins on an ESP32, and let it remain connected for the required time.
Wiring Diagram
The MQ2 gas sensor module provides two outputs. You can use one or both, based on your requirements.
- How to connect ESP32 and gas sensor using breadboard (powered via USB cable)
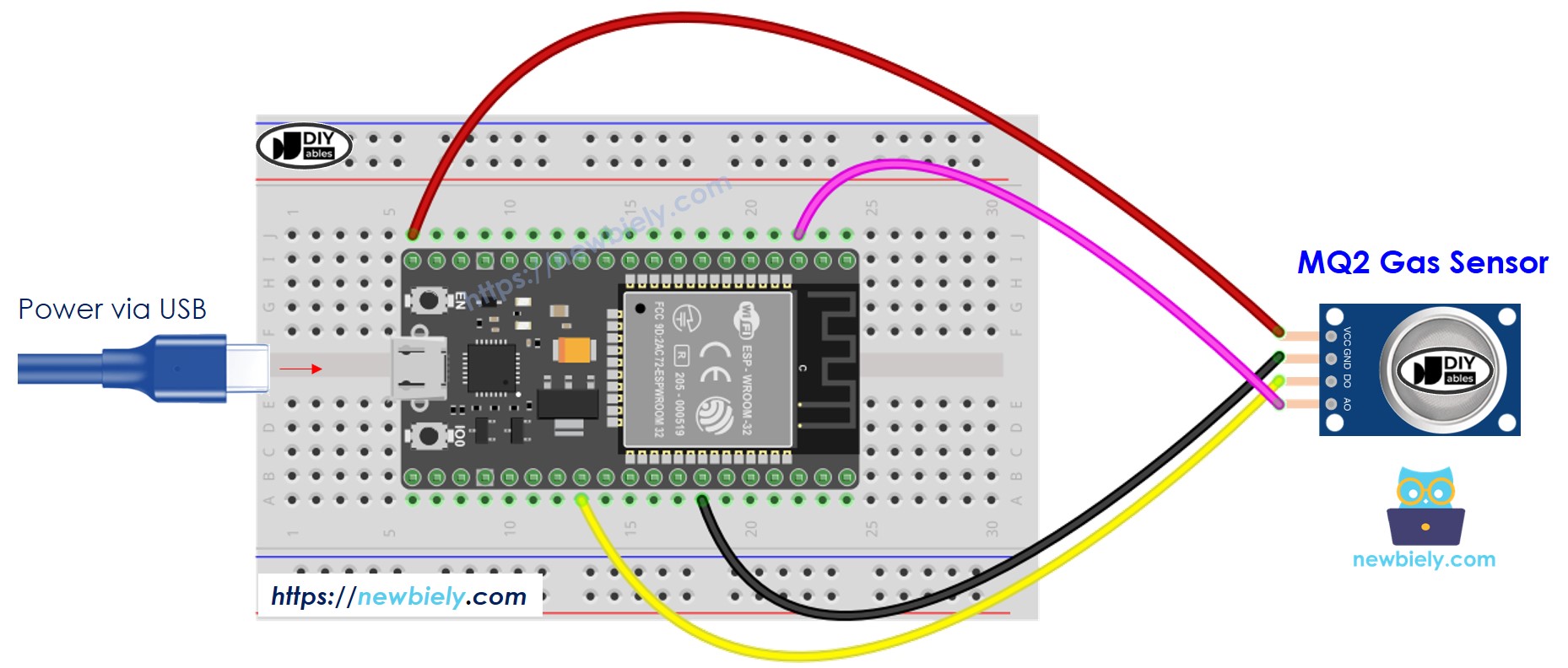
This image is created using Fritzing. Click to enlarge image
- How to connect ESP32 and gas sensor using breadboard (powered via Vin pin)
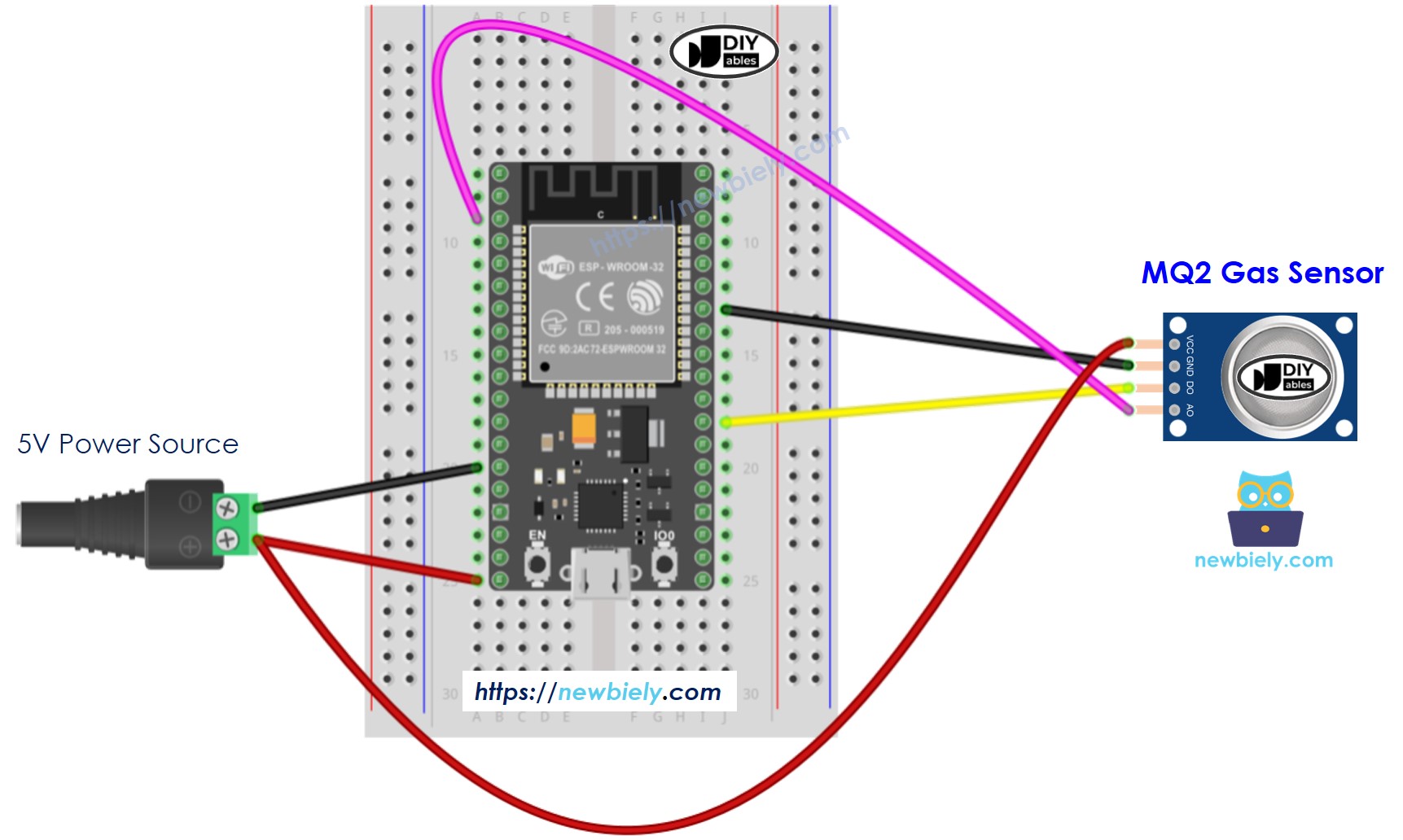
This image is created using Fritzing. Click to enlarge image
- How to connect ESP32 and gas sensor using screw terminal block breakout board (powered via USB cable)
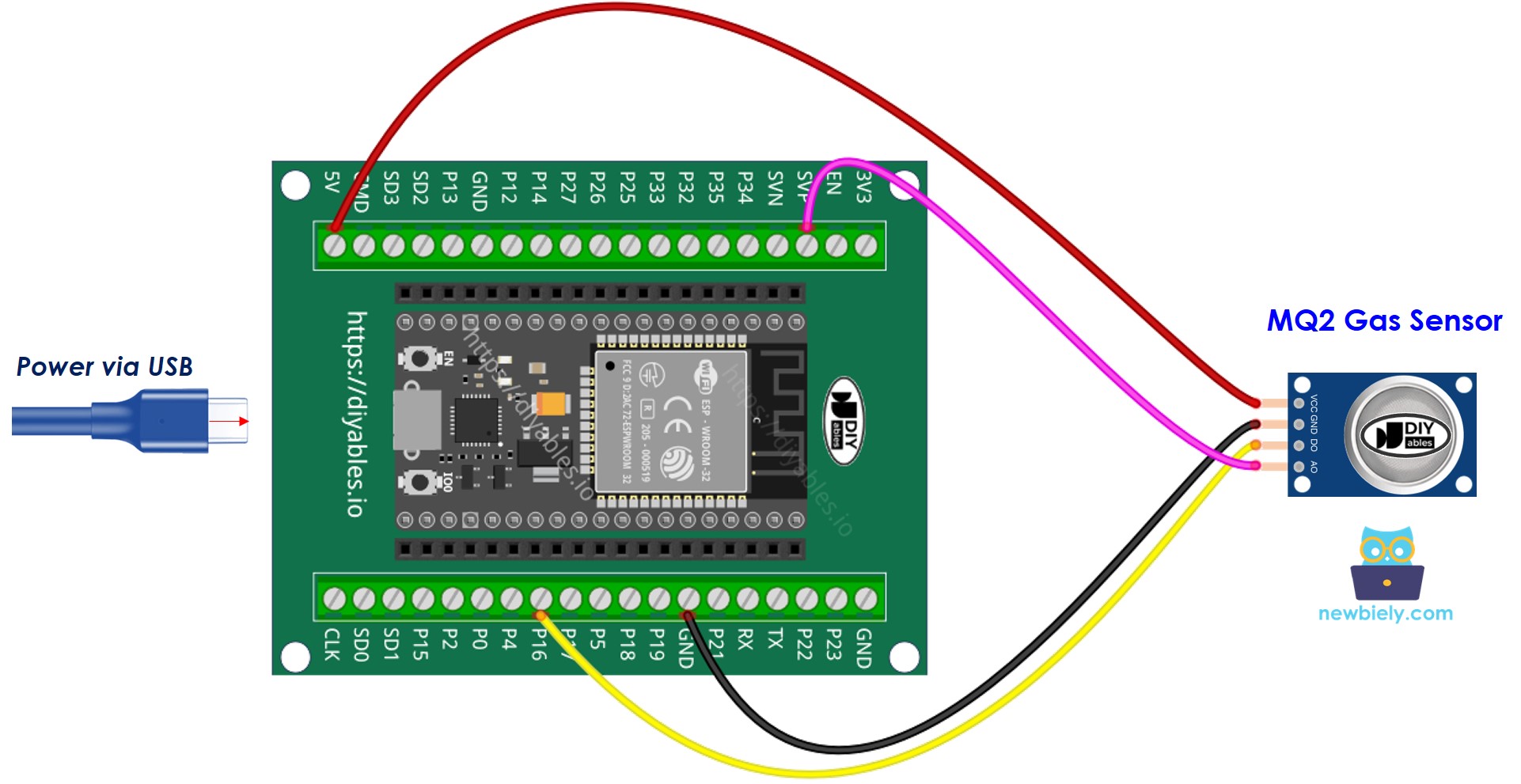
- How to connect ESP32 and gas sensor using screw terminal block breakout board (powered via Vin pin)
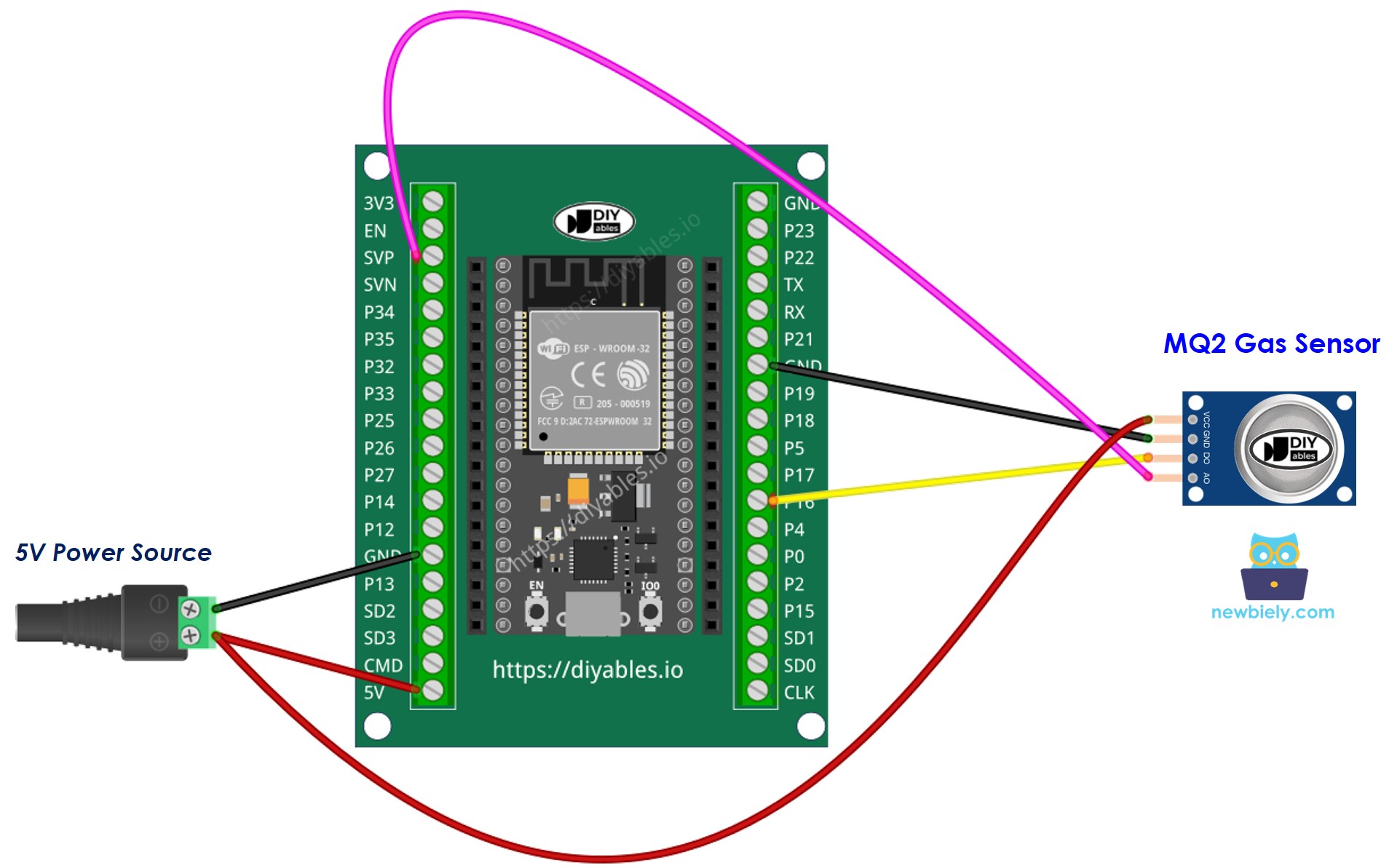
ESP32 MicroPython Code - Read value from DO pin
Detailed Instructions
Here’s instructions on how to set up and run your MicroPython code on the ESP32 using Thonny IDE:
- Make sure Thonny IDE is installed on your computer.
- Confirm that MicroPython firmware is loaded on your ESP32 board.
- If this is your first time using an ESP32 with MicroPython, check out the ESP32 MicroPython Getting Started guide for step-by-step instructions.
- Connect the ESP32 board to the MQ2 gas sensor according to the provided diagram.
- Connect the ESP32 board to your computer with a USB cable.
- Open Thonny IDE on your computer.
- In Thonny IDE, go to Tools Options.
- Under the Interpreter tab, choose MicroPython (ESP32) from the dropdown menu.
- Make sure the correct port is selected. Thonny IDE usually detects it automatically, but you might need to select it manually (like COM12 on Windows or /dev/ttyACM0 on Linux).
- Copy the provided MicroPython code and paste it into Thonny's editor.
- Save the code to your ESP32 by:
- Clicking the Save button or pressing Ctrl+S.
- In the save dialog, choose MicroPython device.
- Name the file main.py.
- Click the green Run button (or press F5) to execute the script.
- Put the MQ2 gas sensor near the smoke or gas that needs detection.
- Check out the message in the Shell at the bottom of Thonny.
If the LED light remains on always or doesn't turn on, you can adjust the sensor's sensitivity by turning the small knob.
ESP32 MicroPython Code - Read value from AO pin
Detailed Instructions
- Copy the provided MicroPython code and paste it into Thonny's editor.
- Save the code to your ESP32 board.
- Click the green Run button (or press F5) to execute the script.
- Position the MQ2 gas sensor near the smoke or gas you want to detect.
- Check out the message in the Shell at the bottom of Thonny.
You can use the values from DO or AO to check if the air quality meets your standards, to trigger an alarm, or to turn on ventilation systems.
※ NOTE THAT:
This tutorial demonstrates how to use the adc.read() function to read values from an ADC (Analog-to-Digital Converter) connected to a gas sensor. The ESP32's ADC is suitable for projects that do not require high precision. However, if your project needs accurate measurements, keep the following in mind:
- The ESP32 ADC is not perfectly accurate and may require calibration for precise results. Each ESP32 board may vary slightly, so calibration is necessary for each individual board.
- Calibration can be challenging, especially for beginners, and might not always yield the exact results you desire.
For projects requiring high precision, consider using an external ADC (e.g., ADS1115) with the ESP32 or opt for an Arduino, which has a more reliable ADC. If you still wish to calibrate the ESP32 ADC, refer to the ESP32 ADC Calibration Driver.