Arduino UNO R4 - LED Matrix
This tutorial instructs you how to use Arduno UNO R4 with external LED matrix display modules. In detail, we will learn:
- How to connect Arduino UNO R4 to an 8x8 LED Matrix
- How to connect Arduino UNO R4 to a 32x8 LED Matrix
- How to program Arduino UNO R4 to display text, numbers, and animations on the LED Matrix
Then, you can simply modify the code for other LED matrices like the 16x8 or 64x8 LED matrix.
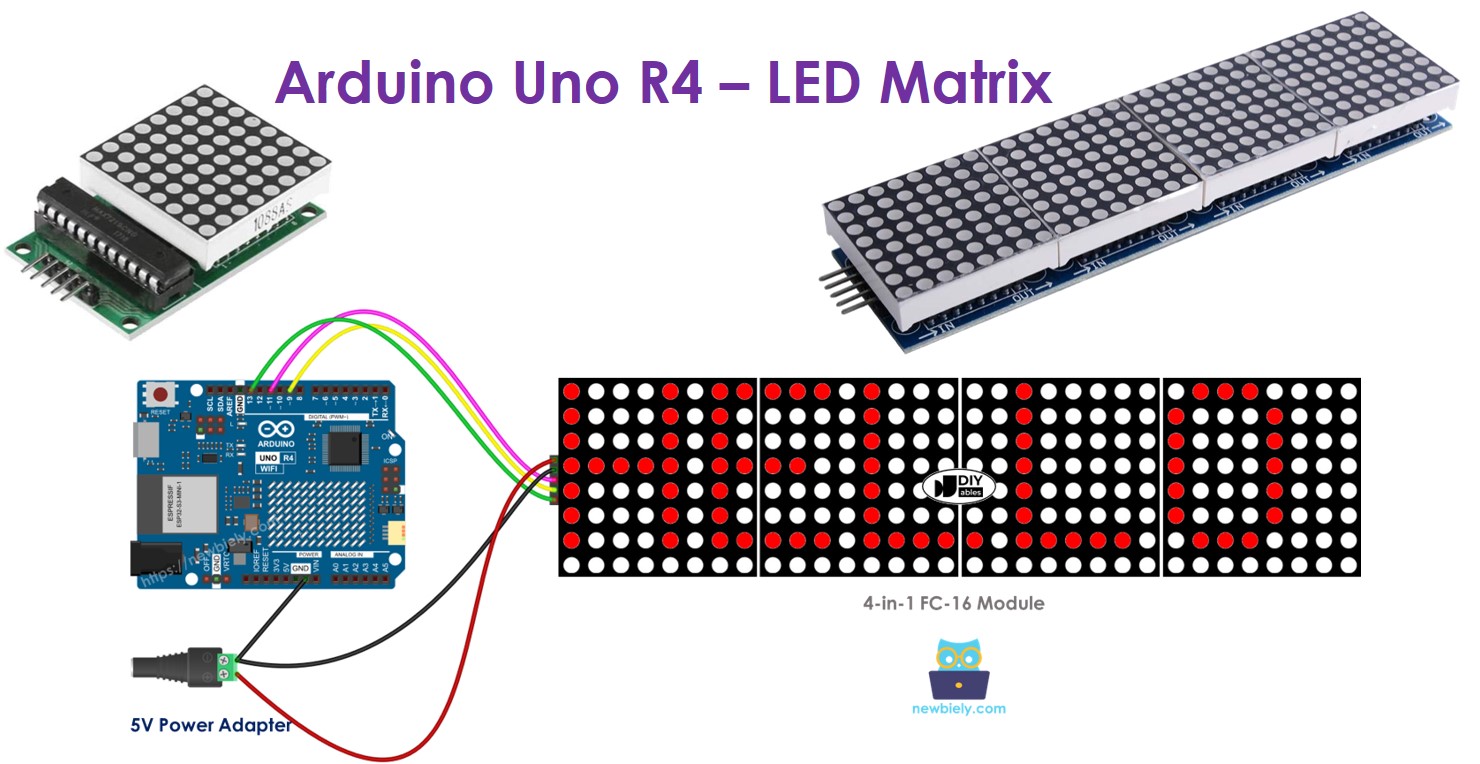
If you want to learn about how to use Arduino R4 with on-board LED Matrix, please refer to Arduino UNO R4 - Built-in LED Matrix
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of LED Matrix
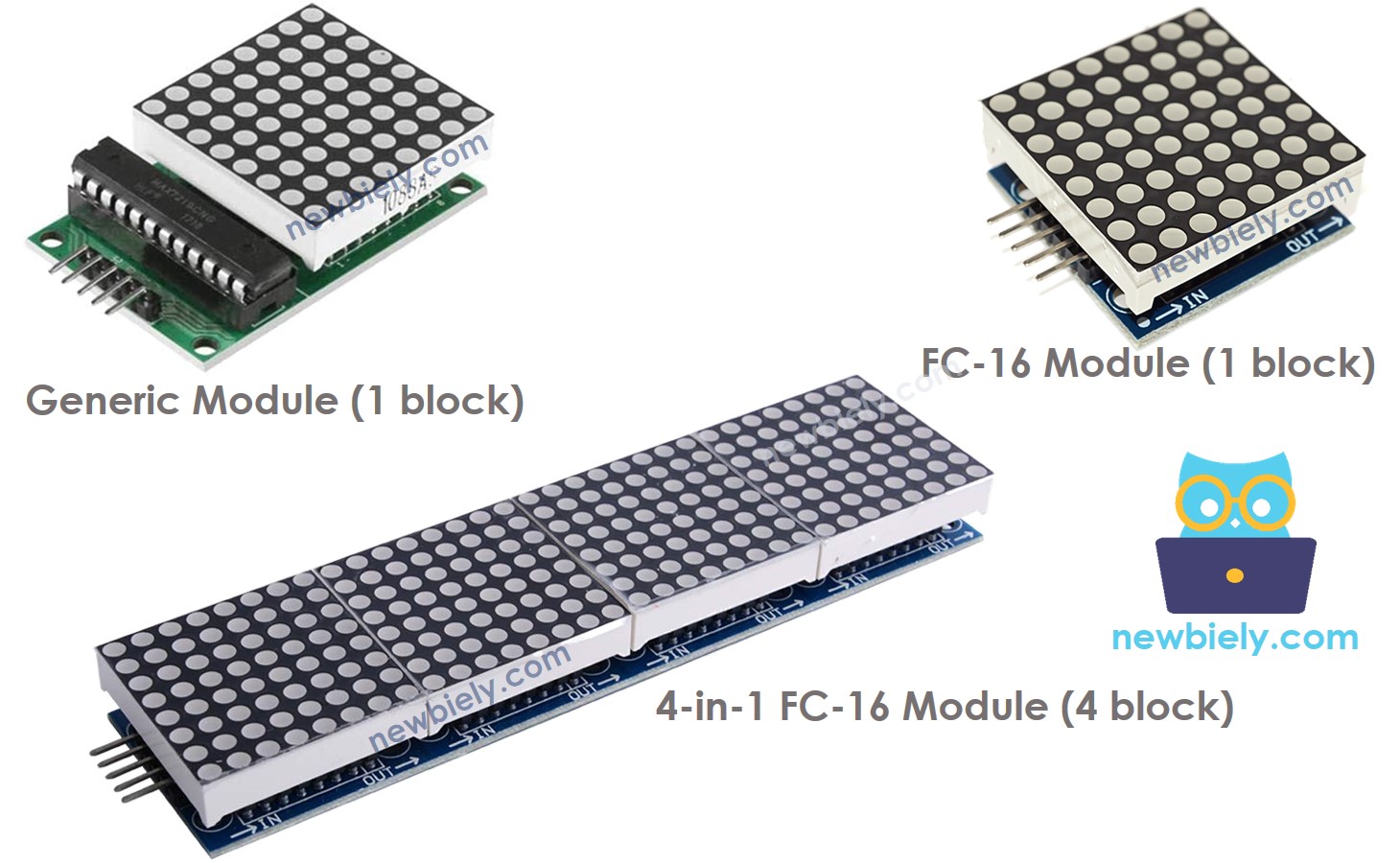
Various types of LED Matrix exist. The MAX7219-based LED matrix is commonly used with Arduino UNO R4. This LED matrix includes several features:
- The basic component of an LED matrix is called a block.
- Each block contains an 8x8 grid of LEDs, totaling 64 LEDs, and is controlled by a MAX7219 chip.
- There are two main types of blocks: the generic module and the FC-16 module.
- An LED matrix can have just one block or be expanded by connecting multiple blocks together like a chain.
- You can purchase LED matrices that already have multiple blocks connected, such as 4-block or 8-block configurations.
- Alternatively, you can buy individual blocks and connect them yourself to create an LED matrix of the size you need.
- In your Arduino UNO R4 program, you must specify the size of the LED matrix you're using.
Pinout
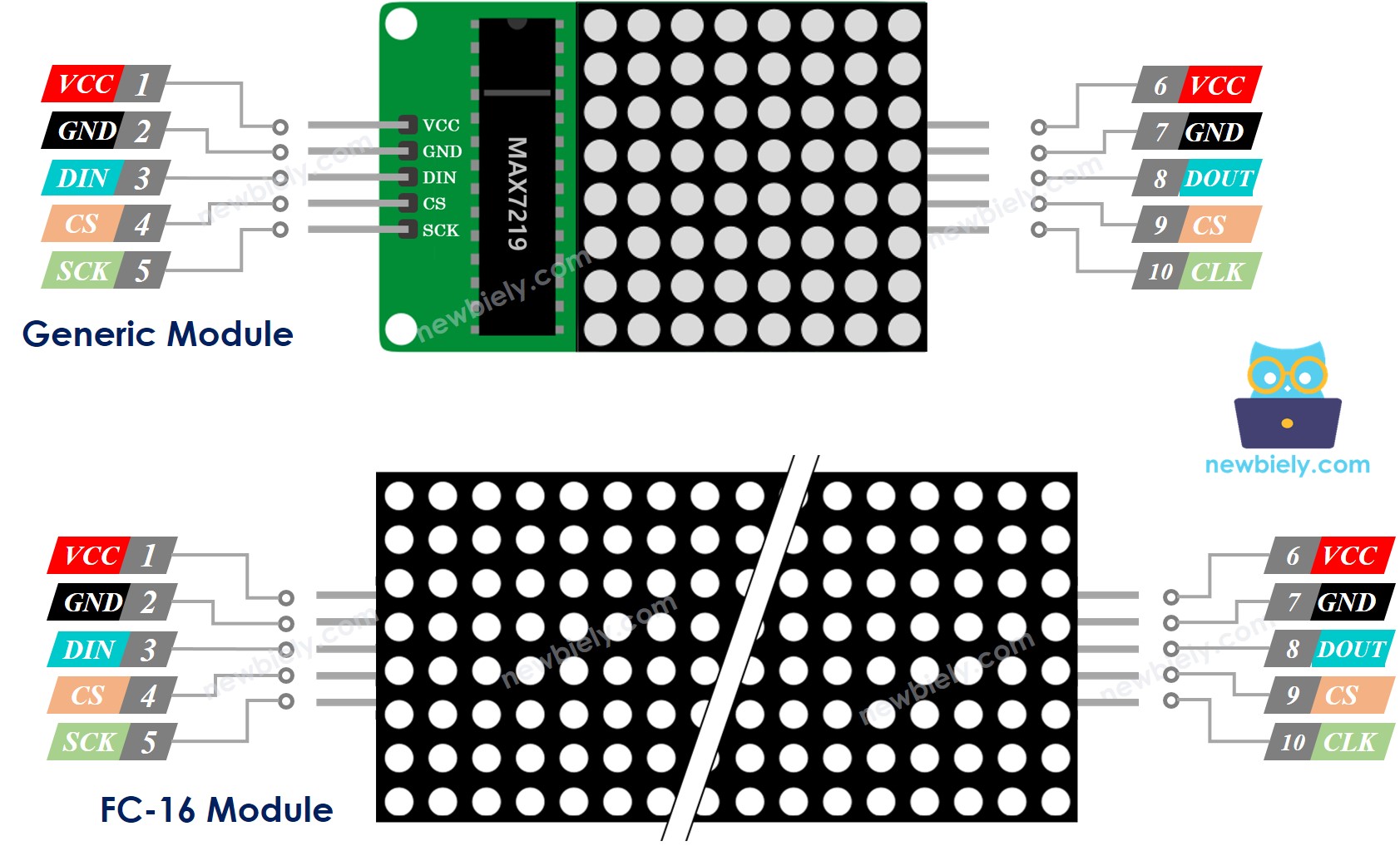
A LED Matrix is made up of one or more blocks. Each block has two sets of pins:
- Group of Input Pins:
- VCC: Link this to the 5V.
- GND: Link this to the GND.
- DIN: This is the Data pin. Connect it to the SPI MOSI pin on the Arduino UNO R4.
- CS: Chip Select. Connect this to any digital pin on the Arduino UNO R4.
- CLK: Clock pin. Connect this to the SPI CLK pin on the Arduino UNO R4.
- Group of Output Pins:
- VCC: Connect this to VCC on the subsequent module.
- GND: Connect this to GND on the subsequent module.
- DOUT: Data Out. This connects to the DIN pin on the next module.
- CS: Connect this to CS on the subsequent module.
- CLK: Connect this to CLK on the subsequent module.
Wiring Diagram
If the LED matrix consists of just one block:
- Connect the groups of input pins to the Arduino UNO R4.
- Leave the groups of output pins unconnected.
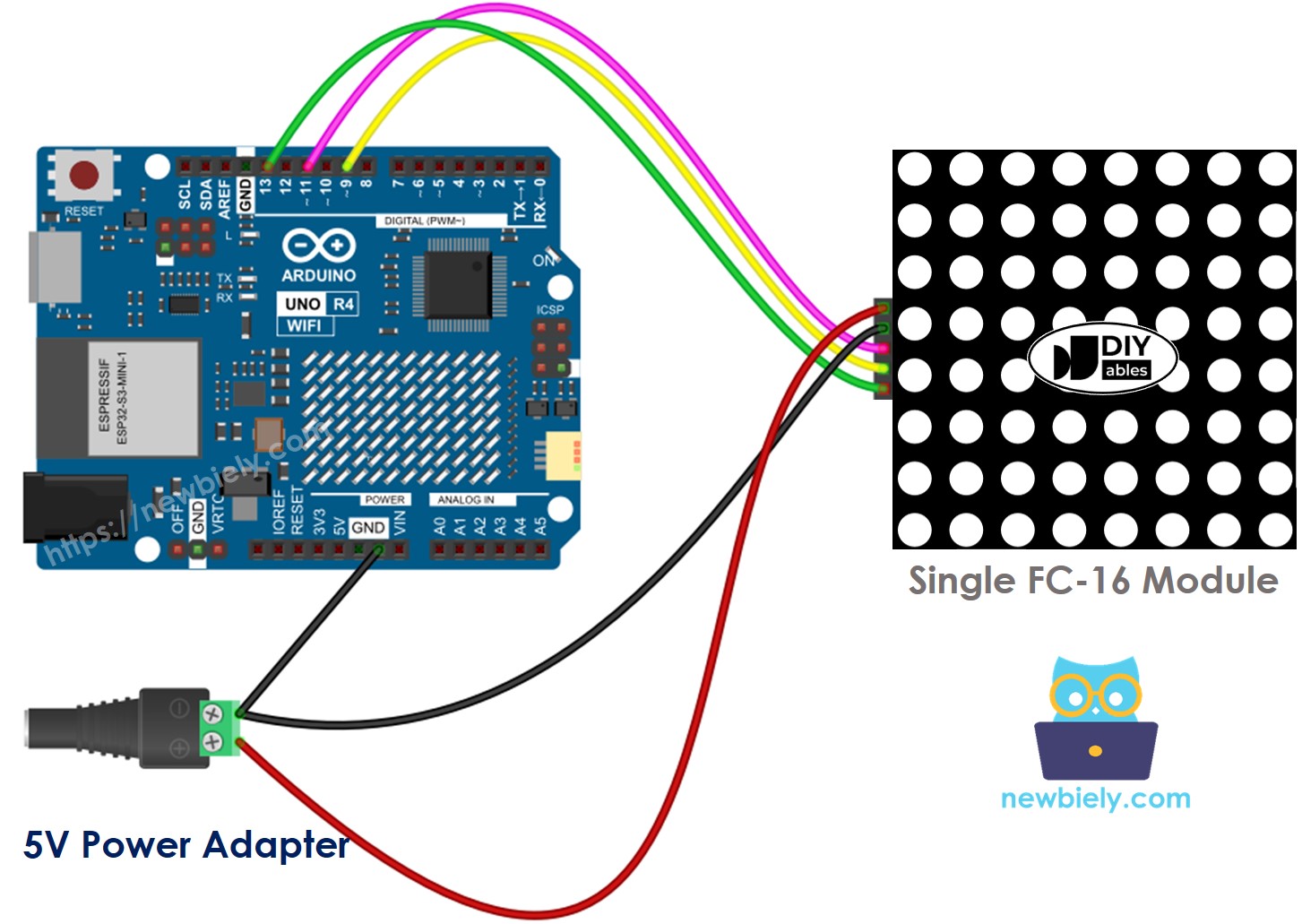
This image is created using Fritzing. Click to enlarge image
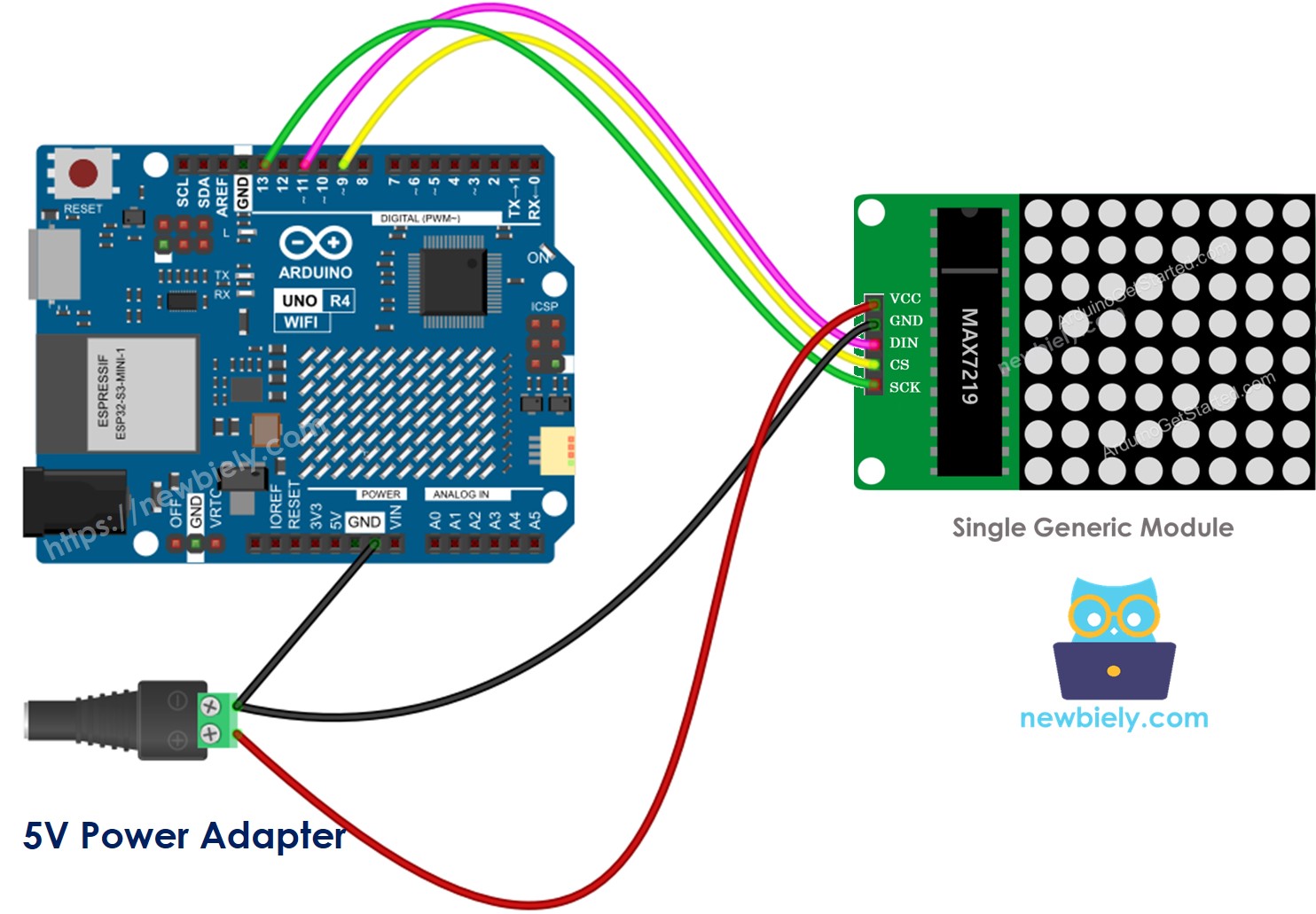
This image is created using Fritzing. Click to enlarge image
If the LED matrix comes already assembled into multiple blocks:
- Connect the group of input pins to the Arduino UNO R4.
- Leave the group of output pins unconnected.
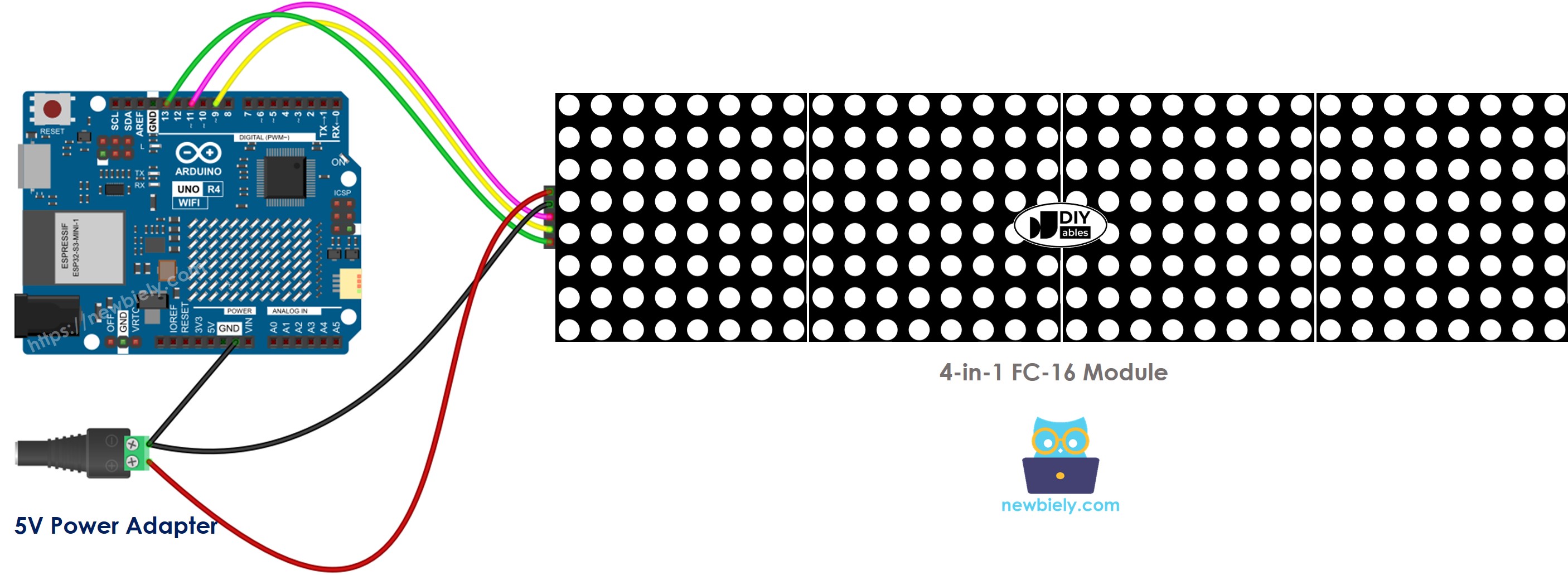
This image is created using Fritzing. Click to enlarge image
If you assemble the LED matrix from several parts:
- Connect the input pin group of the first block to the Arduino UNO R4.
- Connect the output pin group of each block to the input pin group of the following block.
- Leave the output pin group of the last block unconnected.
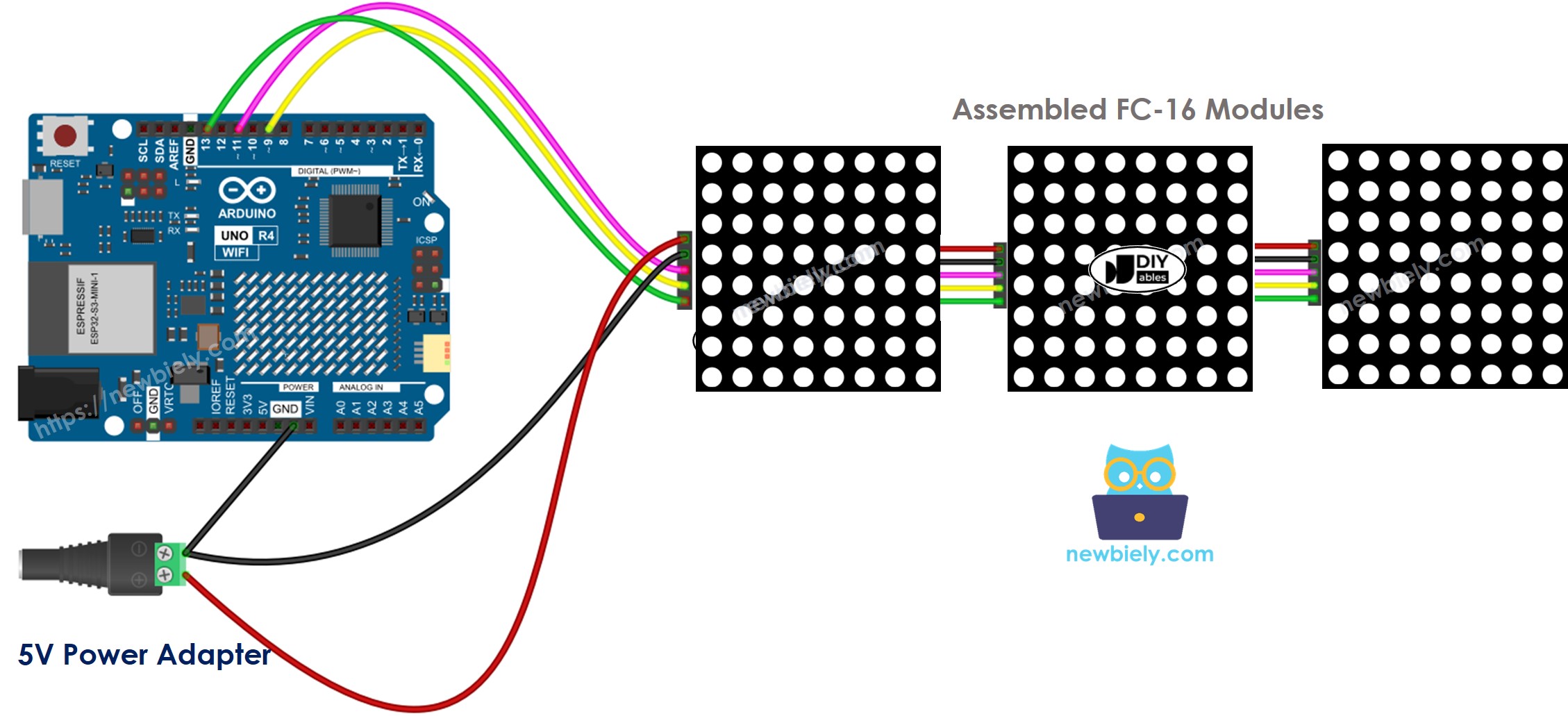
This image is created using Fritzing. Click to enlarge image
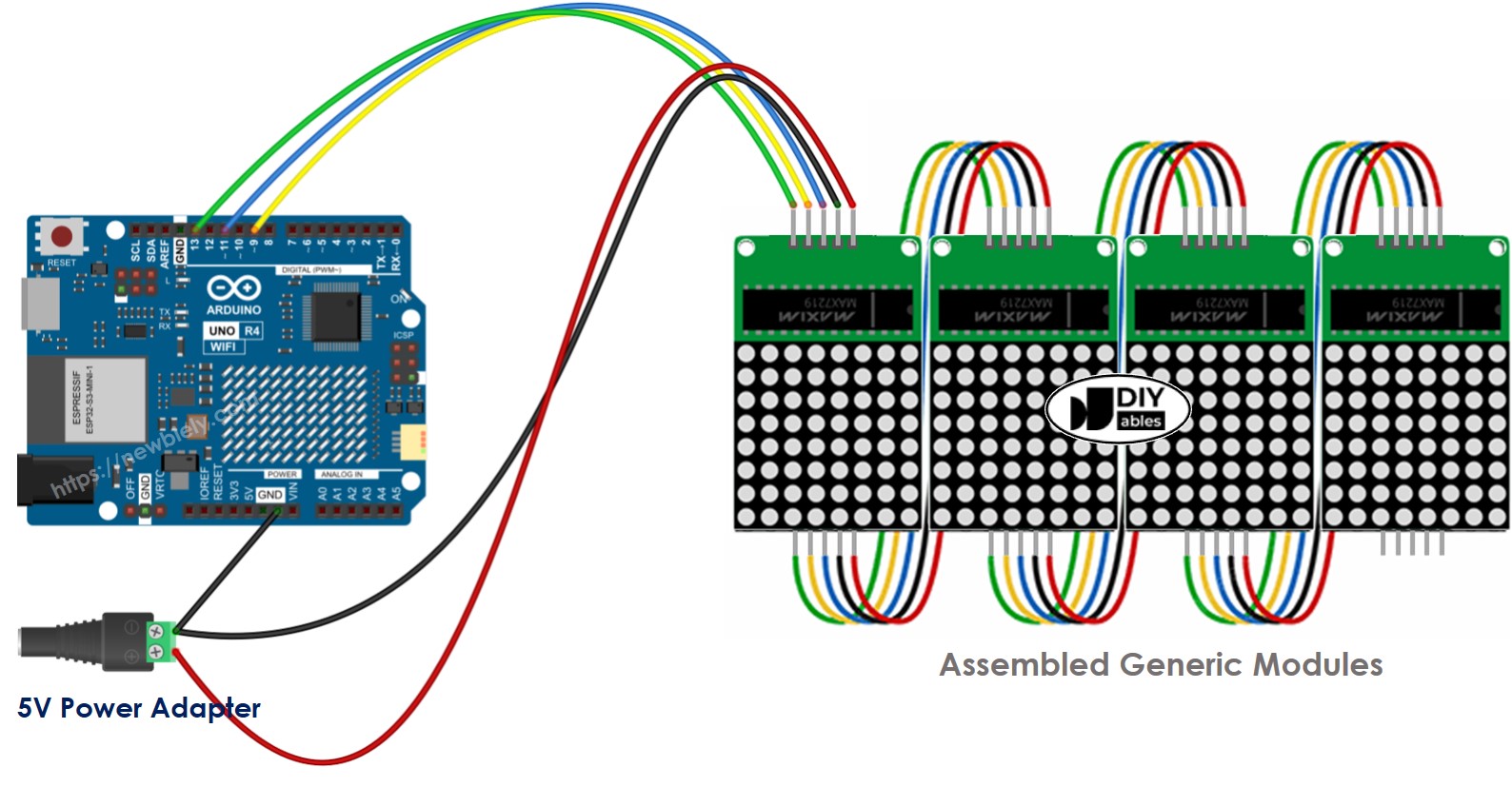
This image is created using Fritzing. Click to enlarge image
See The best way to supply power to the Arduino Uno R4 and other components.
The display uses a lot of power, up to 1 ampere at maximum brightness.
- Avoid using the 5V pin on the Arduino UNO R4 for power.
- Instead, connect an external 5V power supply. The Arduino UNO R4 and the LED matrix can both be powered by the same 5V adapter.
The Arduino UNO R4 connects to the LED matrix using SPI pins.
- Use pin 13 (SCK) and 11 (MOSI) on Arduino UNO R4. If using a different Arduino UNO R4 board, look at the official guide for the same SPI pins.
- You can use any other pin for Pin 3 (CS).
How To Program For LED Matrix
Controlling the LED matrix can be challenging. Luckily, there are libraries that simplify this task. Here are the steps to program an Arduino UNO R4 to control the LED matrix.
- Include libraries:
- Choose the type of hardware you are using: GENERIC_HW or FC16_HW.
- Determine how many LED blocks are used. For instance, a 4-in-1 LED matrix contains 4 blocks.
- Set the pin attached to the CS pin on the LED matrix. For example, use pin D9.
- Make a new version of the MD_Parola class for the LED matrix display.
- Set up the code in the setup() function.
- Show text, numbers, and animated effects: see the following part.
Arduino UNO R4 - LED Matrix Code
The code provided is for a 32x8 FC-16 LED matrix display with four blocks. However, it can be modified for other sizes like 8x8, 16x8, 64x8, and so on.
Detailed Instructions
Follow these instructions step by step:
- If this is your first time using the Arduino Uno R4 WiFi/Minima, refer to the tutorial on setting up the environment for Arduino Uno R4 WiFi/Minima in the Arduino IDE.
- Connect the Arduino Uno R4 board to the led matrix according to the provided diagram.
- Connect the Arduino Uno R4 board to your computer using a USB cable.
- Launch the Arduino IDE on your computer.
- Select the appropriate Arduino Uno R4 board (e.g., Arduino Uno R4 WiFi) and COM port.
- In the Arduino IDE, click on the Libraries icon on the left sidebar.
- Search for "MD_Parola" and locate the MD_Parola library.
- Click the Install button.
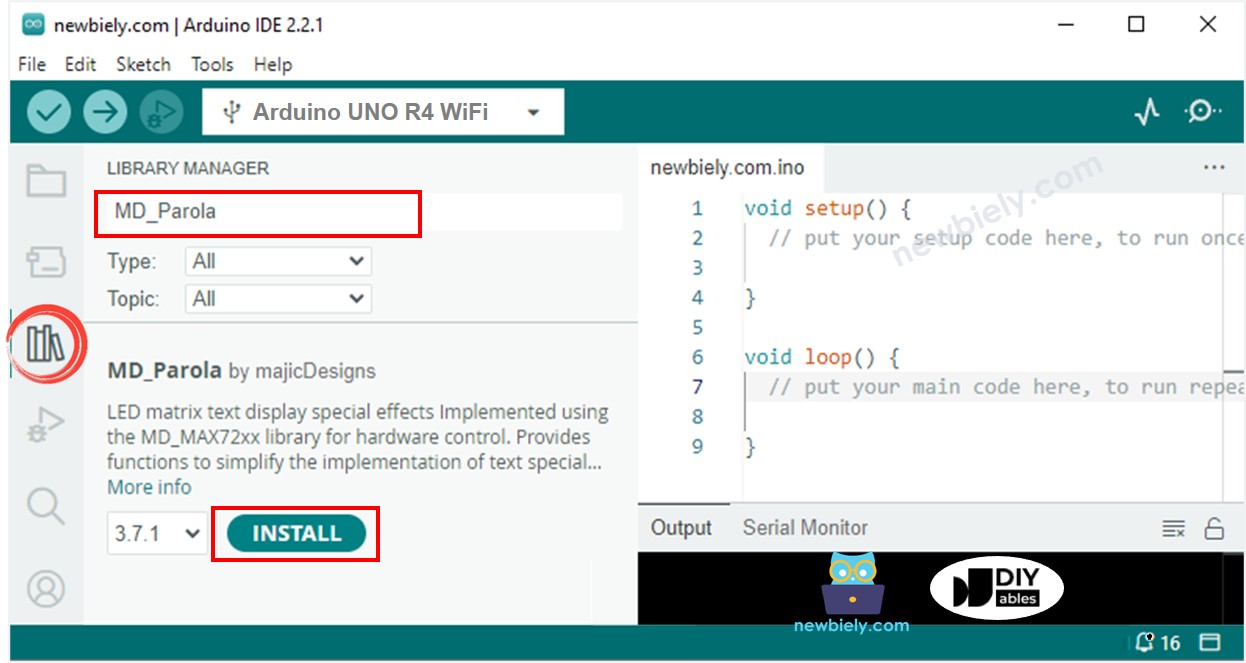
- You need to install the MD_MAX72XX library. Please click the Install All button.
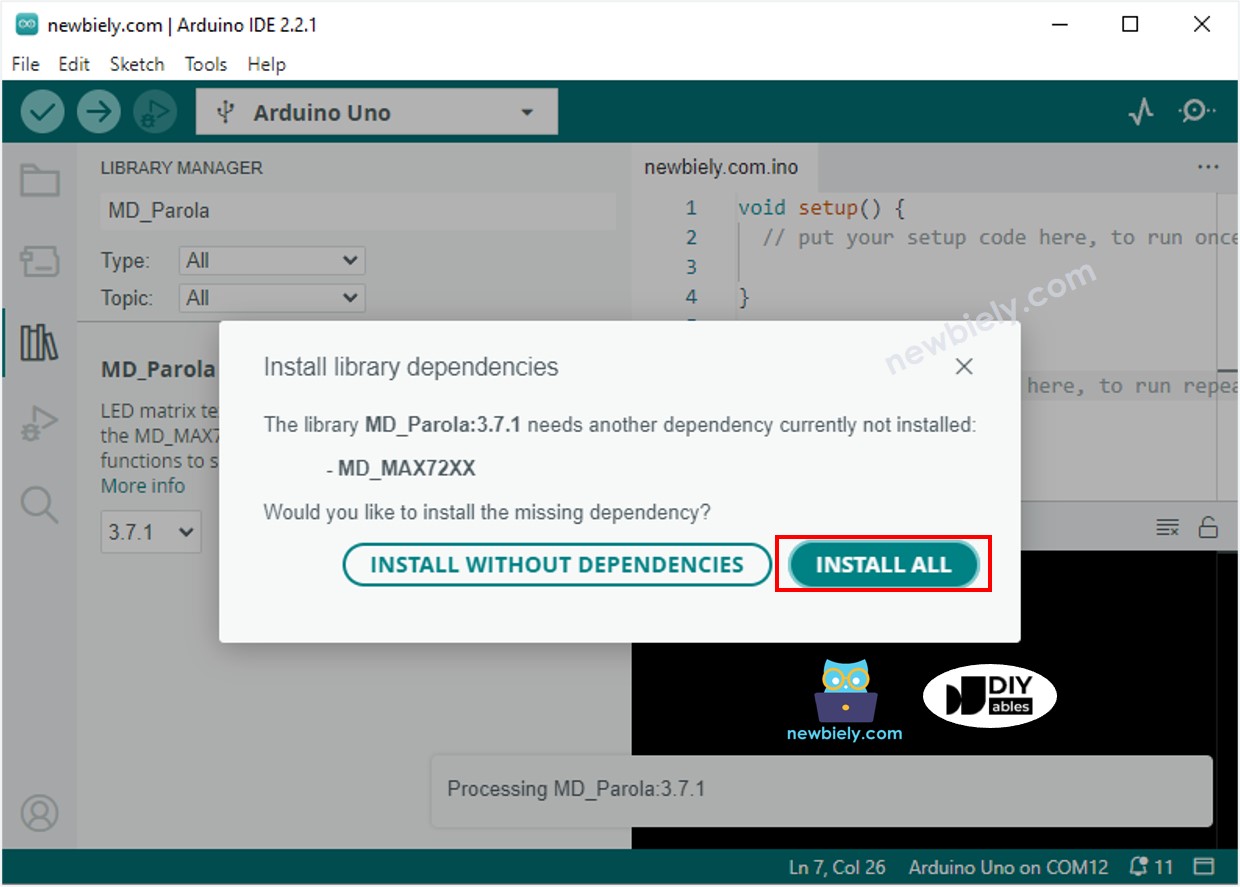
- Copy the code above and open it using Arduino IDE.
- Click the Upload button in Arduino IDE to upload the code to Arduino UNO R4.
- Watch the display on the LED matrix.
Arduino UNO R4 LED Matrix Code – Scrolling Text
When you need to show a long message on a LED matrix display and it is too long to fit, you can use the scrolling text effect.
This Arduino UNO R4 code demonstrates how to make a message scroll across the LED matrix display.
To see more text effects, go to MD_Parola Library Reference.