Arduino UNO R4 - Temperature Sensor - LCD
This tutorial instructs you how to use Arduino UNO R4 to read temperature value from DS18B20 one-wire temperature sensor and display it on LCD I2C.
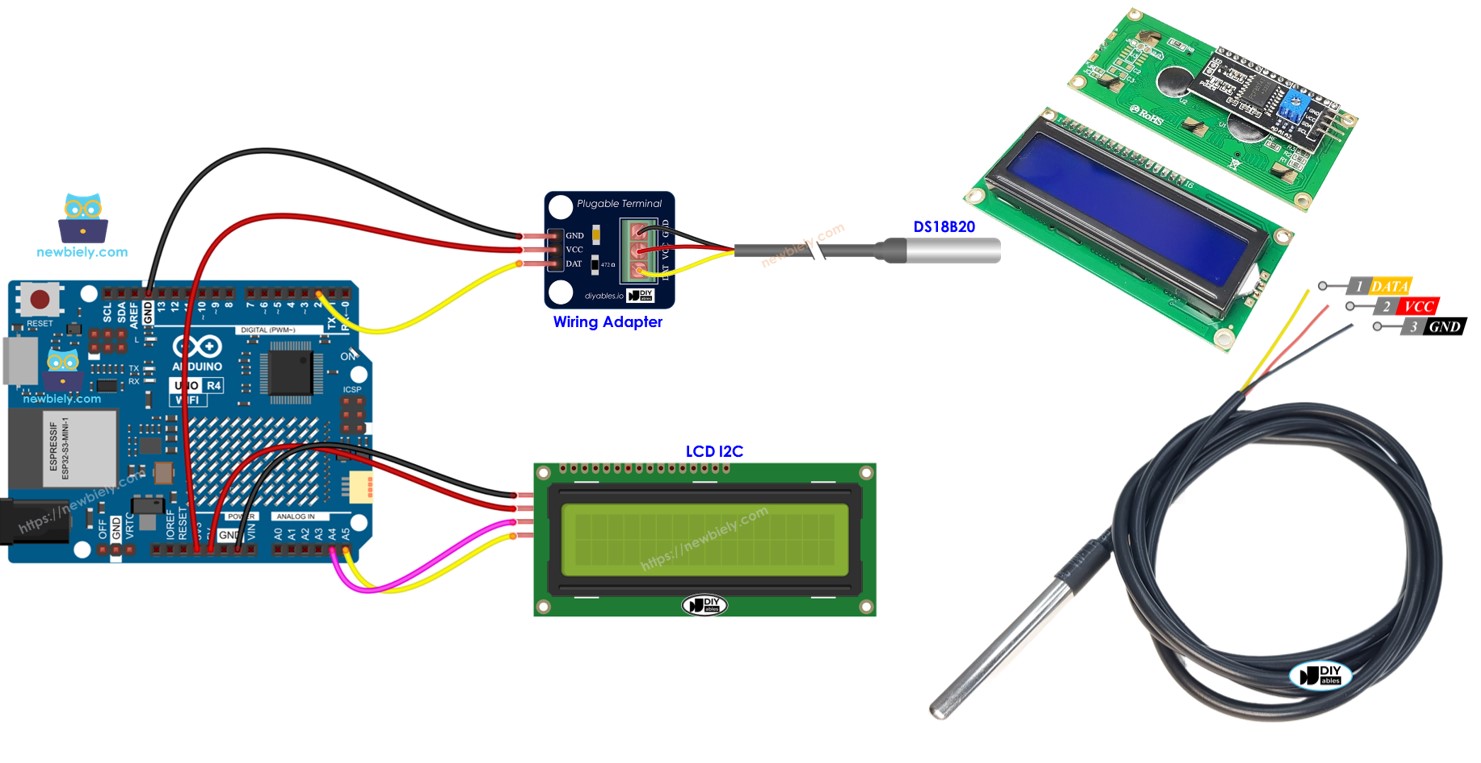
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Buy Note: Many DS18B20 sensors available in the market are unreliable. We strongly recommend buying the sensor from the DIYables brand using the link provided above. We tested it, and it worked reliably.
Overview of Temperature Sensor and LCD
Learn about temperature sensors and LCDs, including their pinout, functions, and programming, in the tutorials linked below:
Wiring Diagram
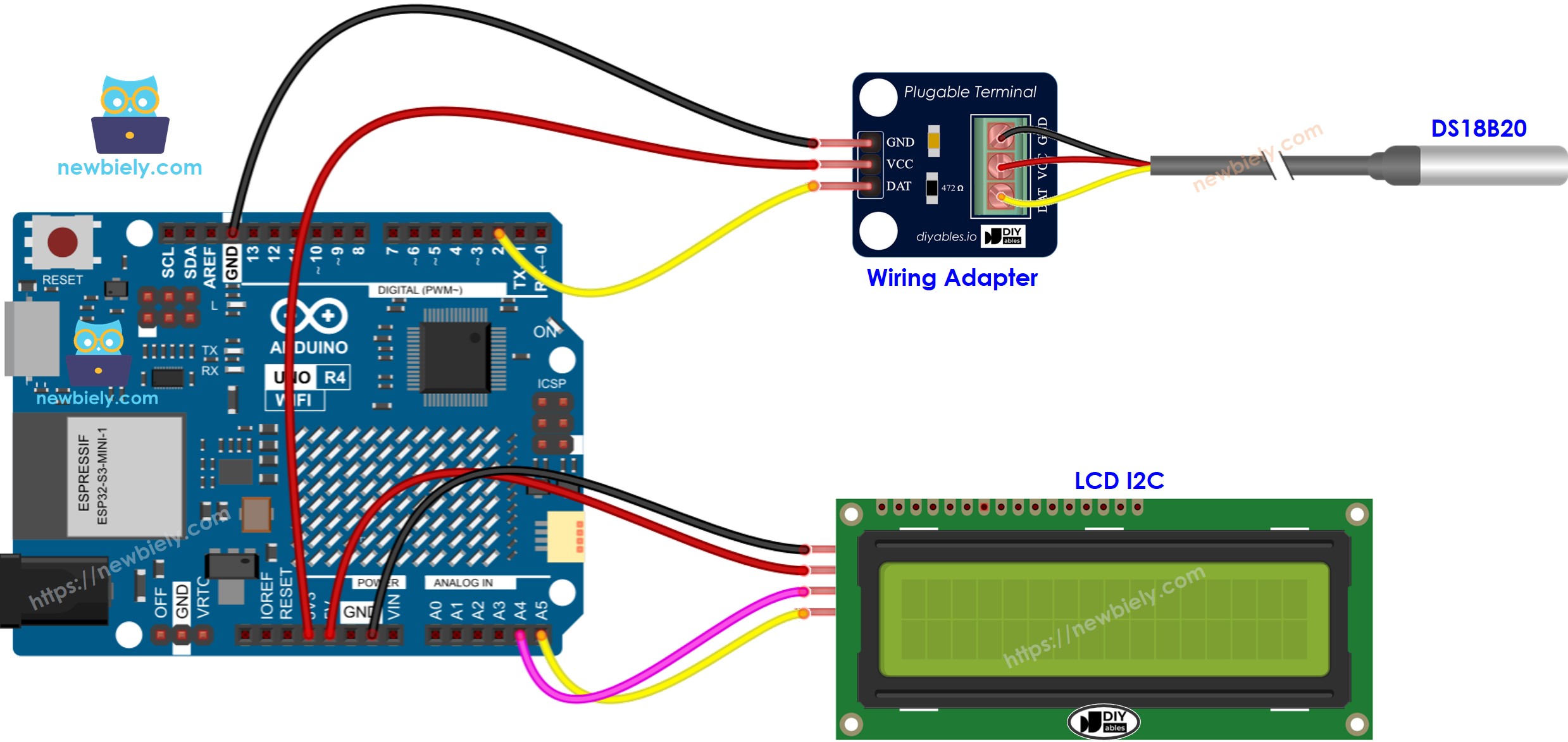
This image is created using Fritzing. Click to enlarge image
See The best way to supply power to the Arduino Uno R4 and other components.
Arduino UNO R4 Code
※ NOTE THAT:
The I2C address for the LCD might change depending on the maker. In our code, we used 0x27, which is the address given by the manufacturer DIYables.
Detailed Instructions
Follow these instructions step by step:
- If this is your first time using the Arduino Uno R4 WiFi/Minima, refer to the tutorial on setting up the environment for Arduino Uno R4 WiFi/Minima in the Arduino IDE.
- Connect the Arduino Uno R4 to the DS18B20 temperature sensor and LCD I2C according to the provided diagram.
- Connect the Arduino Uno R4 board to your computer using a USB cable.
- Launch the Arduino IDE on your computer.
- Select the appropriate Arduino Uno R4 board (e.g., Arduino Uno R4 WiFi) and COM port.
- Click on the Libraries icon on the left side of the Arduino IDE.
- Search for DallasTemperature and find the library by Miles Burton.
- Click the Install button to add the DallasTemperature library.
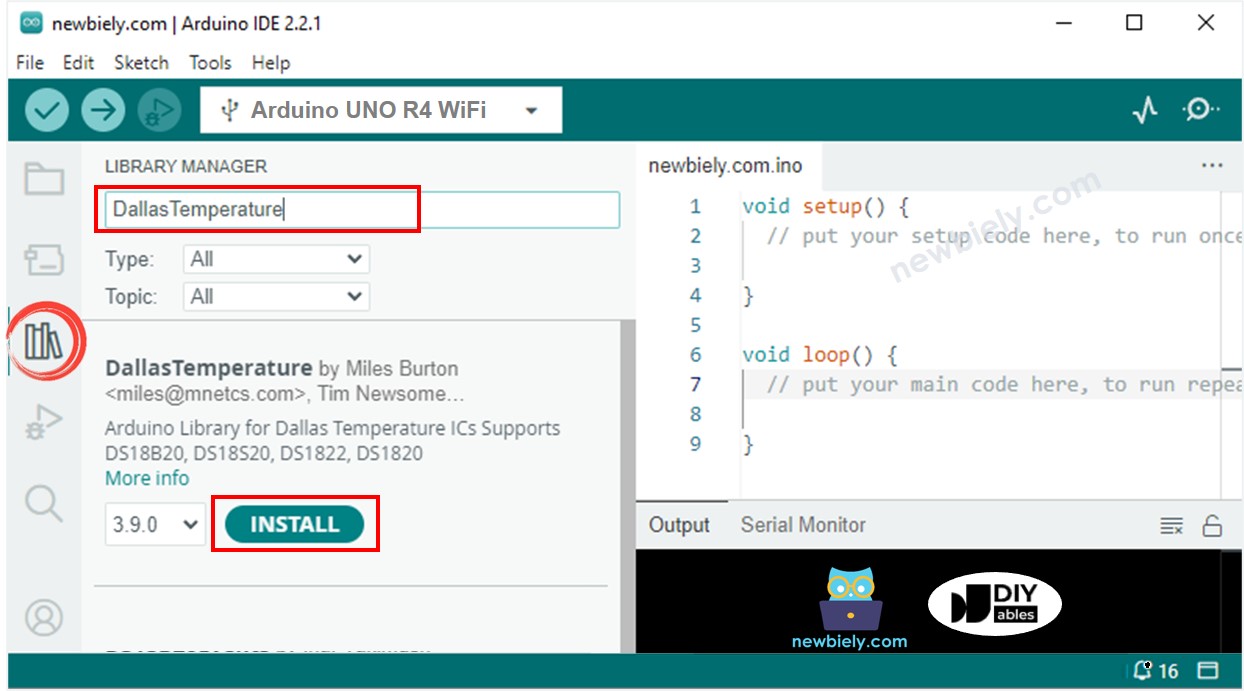
- You need to install a library dependency
- Click the Install All button to install the OneWire library.
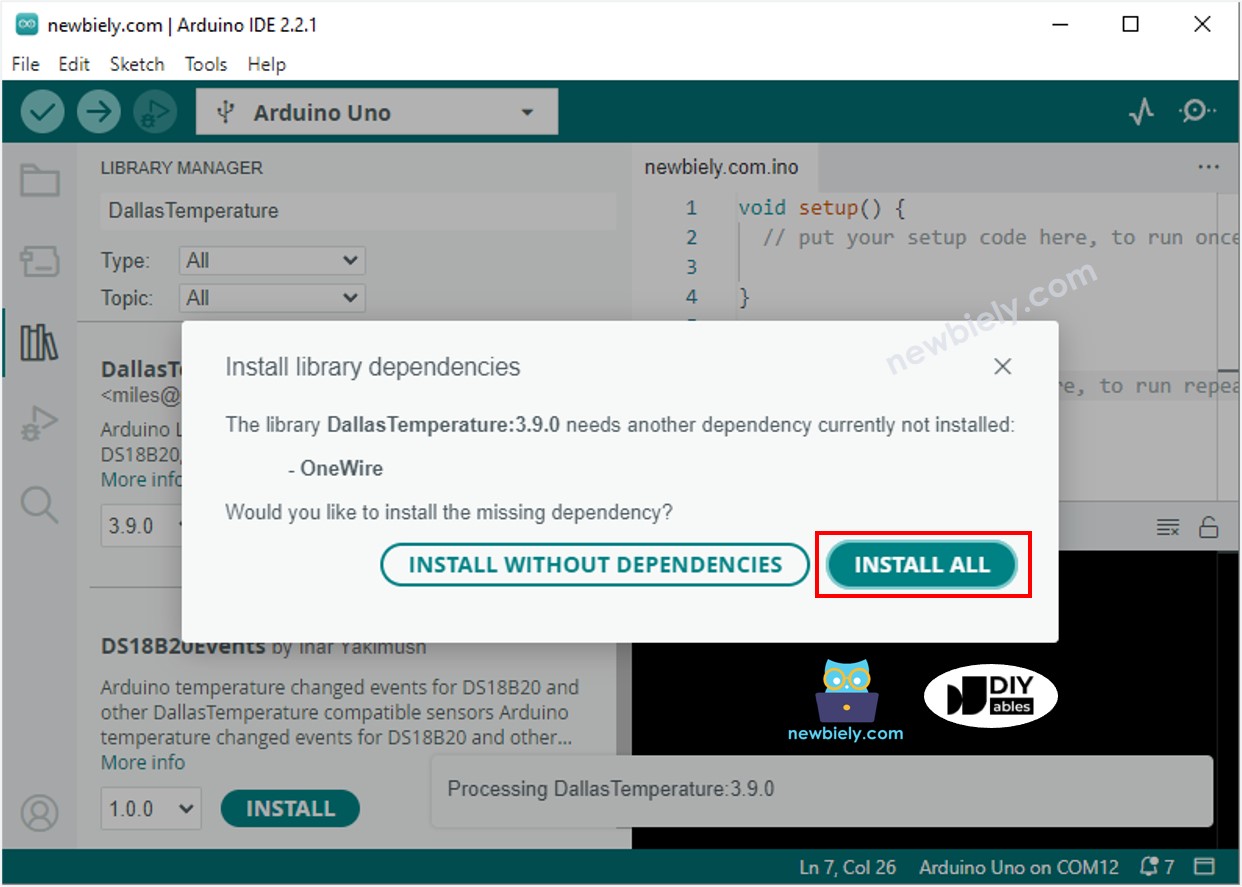
- Search for LiquidCrystal I2C and find the LiquidCrystal_I2C library by Frank de Brabander.
- Click the Install button to install the LiquidCrystal_I2C library.
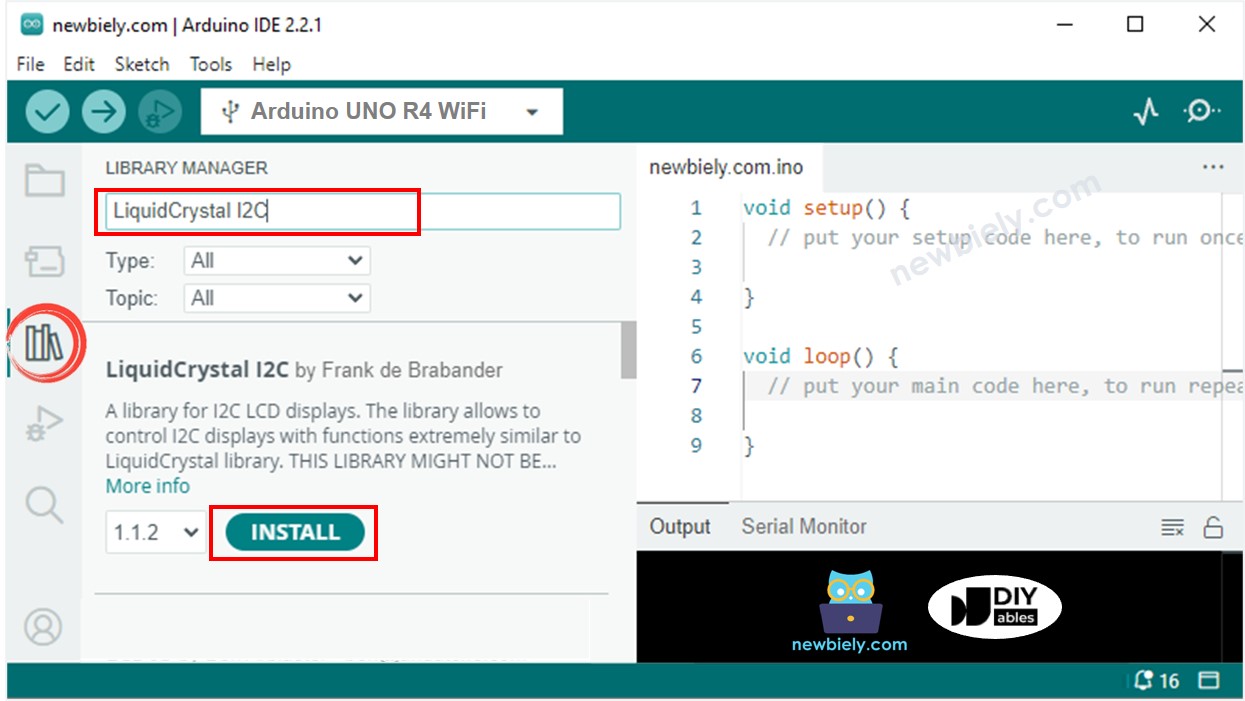
- Copy the code and open it using Arduino IDE
- Click the Upload button in Arduino IDE to send the code to Arduino UNO R4
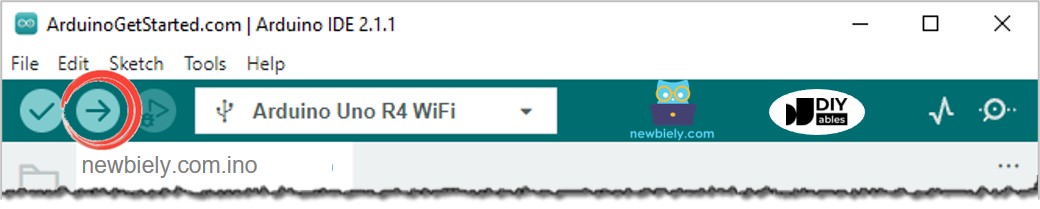
- Place the sensor on hot or cold water, or hold it in your hand.
- Check the display on the LCD screen.
If the LCD shows nothing, check Troubleshooting for LCD I2C.
Code Explanation
Check the explanation in the source code comments for details on each line!