Arduino UNO R4 - OLED 128x32
This tutorial will show you how to use an Arduino UNO R4 with an OLED 128x32 I2C display. You will learn:
- How to connect an OLED 128x32 display to the Arduino UNO R4.
- How to program the Arduino UNO R4 to show text and numbers on the 128x32 OLED display.
- How to create drawings on the 128x32 OLED display with the Arduino UNO R4.
- How to display images on the 128x32 OLED display with the Arduino UNO R4.
- How to center text and numbers both vertically and horizontally on the 128x32 OLED display.
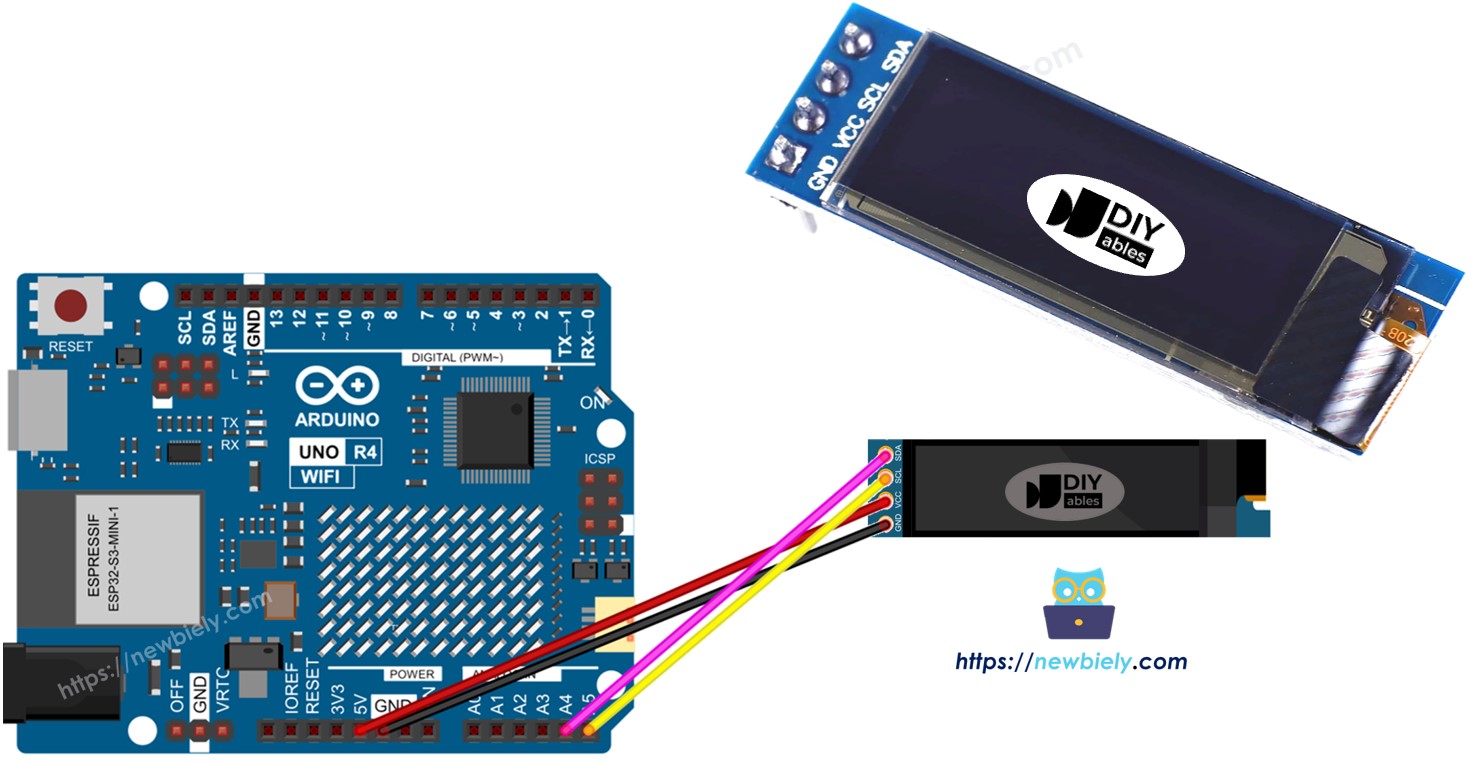
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of OLED Display
I2C OLED Display Pinout
- GND pin: should be connected to the ground of Arduino UNO R4
- VCC pin: is the power supply for the display which we connect the 5 volts pin on the Arduino UNO R4.
- SCL pin: is a serial clock pin for I2C interface.
- SDA pin: is a serial data pin for I2C interface.
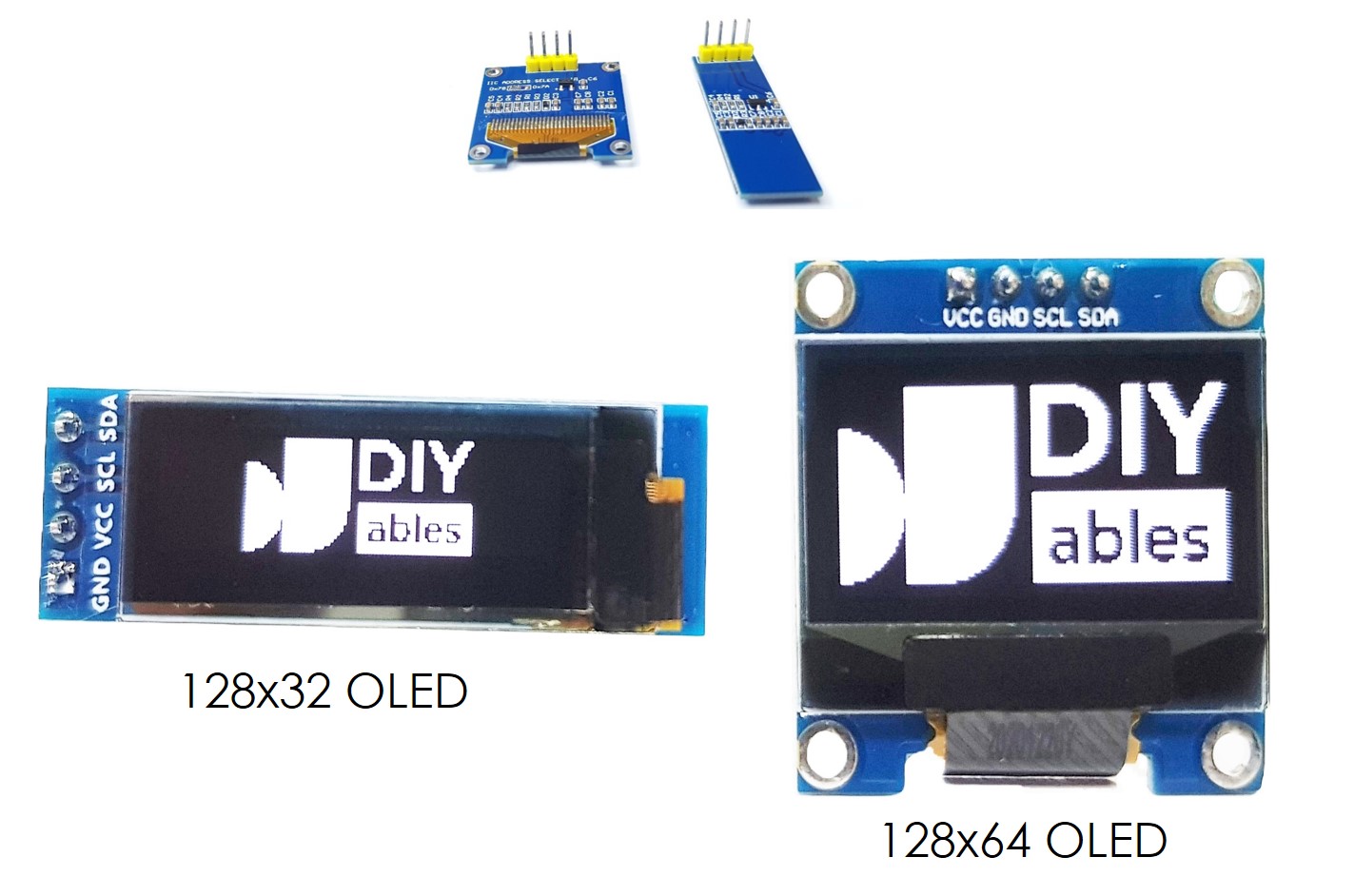
※ NOTE THAT:
The arrangement of pins on an OLED module can differ based on the manufacturer and the model of the module. Always check and follow the labels on the OLED module. Be attentive!
This guide is for an OLED display that uses the SSD1306 I2C driver. We tested it with an OLED display from DIYables. It functions perfectly without any problems.
Wiring Diagram
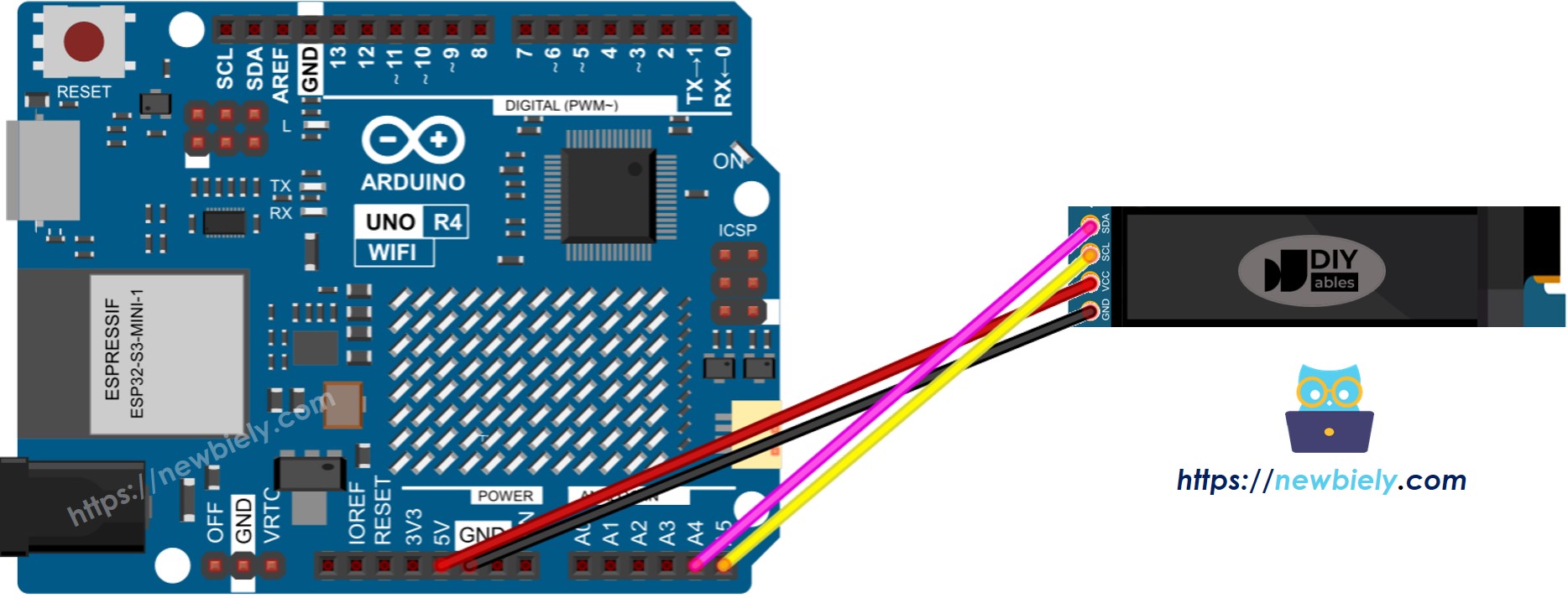
This image is created using Fritzing. Click to enlarge image
See The best way to supply power to the Arduino Uno R4 and other components.
If you use a different type of Arduino UNO R4, the pin layout will not be the same as the Uno. Look at the table below for information on other Arduino UNO R4 models.
128x32 OLED Module | Arduino UNO R4 |
---|---|
Vin | 5V |
GND | GND |
SDA | A4 |
SCL | A5 |
How To Use OLED with Arduino UNO R4
Install SSD1306 OLED library
- Go to the Libraries icon on the left side of the Arduino IDE.
- Type "SSD1306" in the search box and look for the SSD1306 library made by Adafruit.
- Press the Install button to add the library.
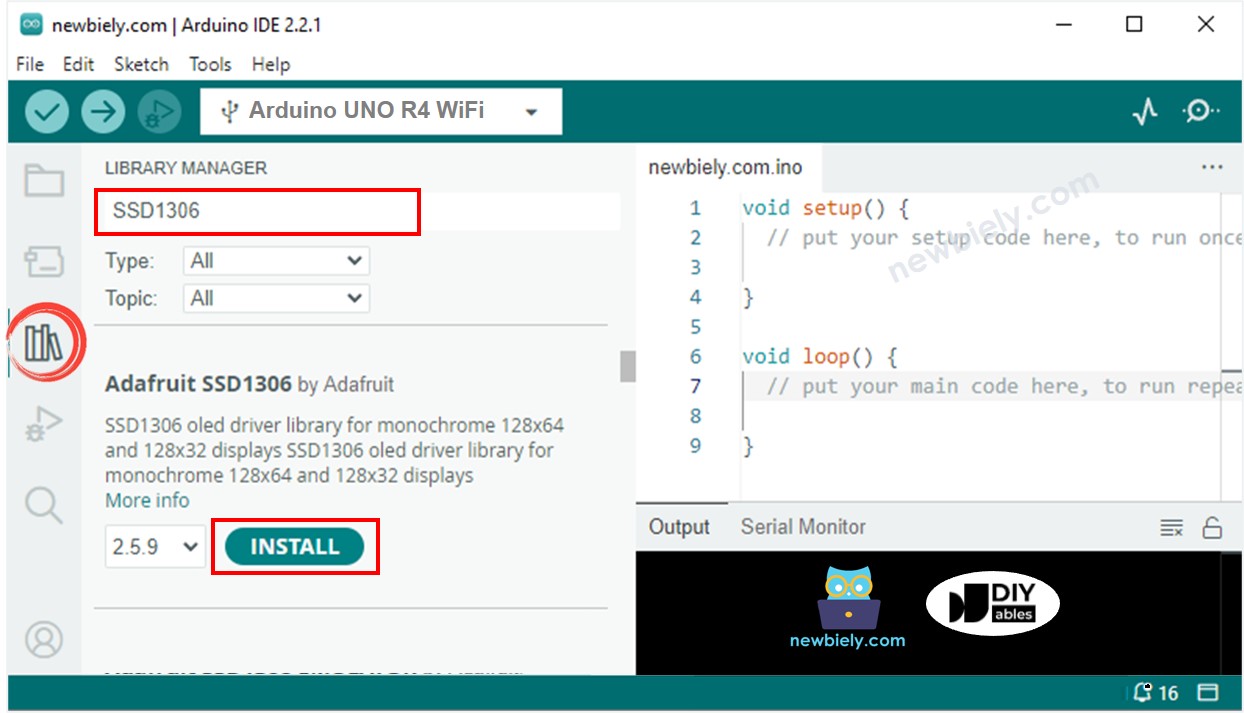
- You will need to install some additional libraries.
- Click the Install All button to install all the required libraries.
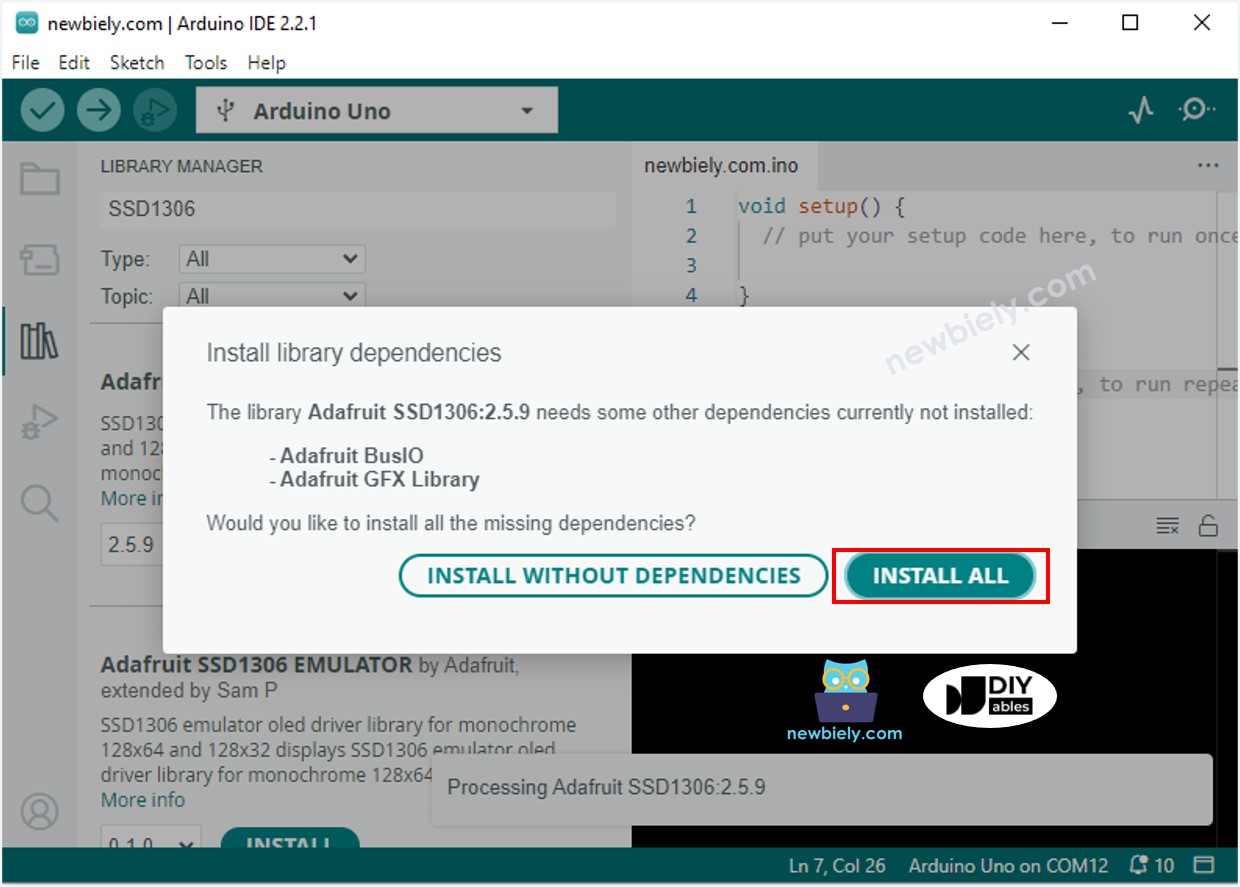
How to program for OLED
- Include a library.
- Set the screen size to OLED 123x32.
- Create an SSD1306 OLED item.
- In the setup() function, set up the OLED screen.
- Then you can show text, pictures, and draw lines.
Arduino UNO R4 Code - Display Text on OLED
Here are some functions you can use to show text on the OLED:
- oled.clearDisplay(): turns off all pixels.
- oled.drawPixel(x, y, color): draws a pixel at x, y coordinates.
- oled.setTextSize(n): changes text size, with choices from 1 to 8.
- oled.setCursor(x, y): sets the starting point for text.
- oled.setTextColor(WHITE): makes the text color white.
- oled.setTextColor(BLACK, WHITE): makes the text color black and background white.
- oled.println("message"): displays text.
- oled.println(number): displays a number.
- oled.println(number, HEX): shows a number in hexadecimal format.
- oled.display(): updates the display with changes.
- oled.startscrollright(start, stop): moves text from left to right.
- oled.startscrollleft(start, stop): moves text from right to left.
- oled.startscrolldiagright(start, stop): moves text diagonally from the bottom-left to top-right.
- oled.startscrolldiagleft(start, stop): moves text diagonally from the bottom-right to top-left.
- oled.stopscroll(): stops any scrolling text.
Arduino UNO R4 Code - Drawing on OLED
Arduino UNO R4 Code – Display Image
To display an image on an OLED screen, first turn the image (any format) into a bitmap array. You can use this online tool to convert it. Look at the image below to see how to change an image into a bitmap array. I changed the Arduino icon into a bitmap array.
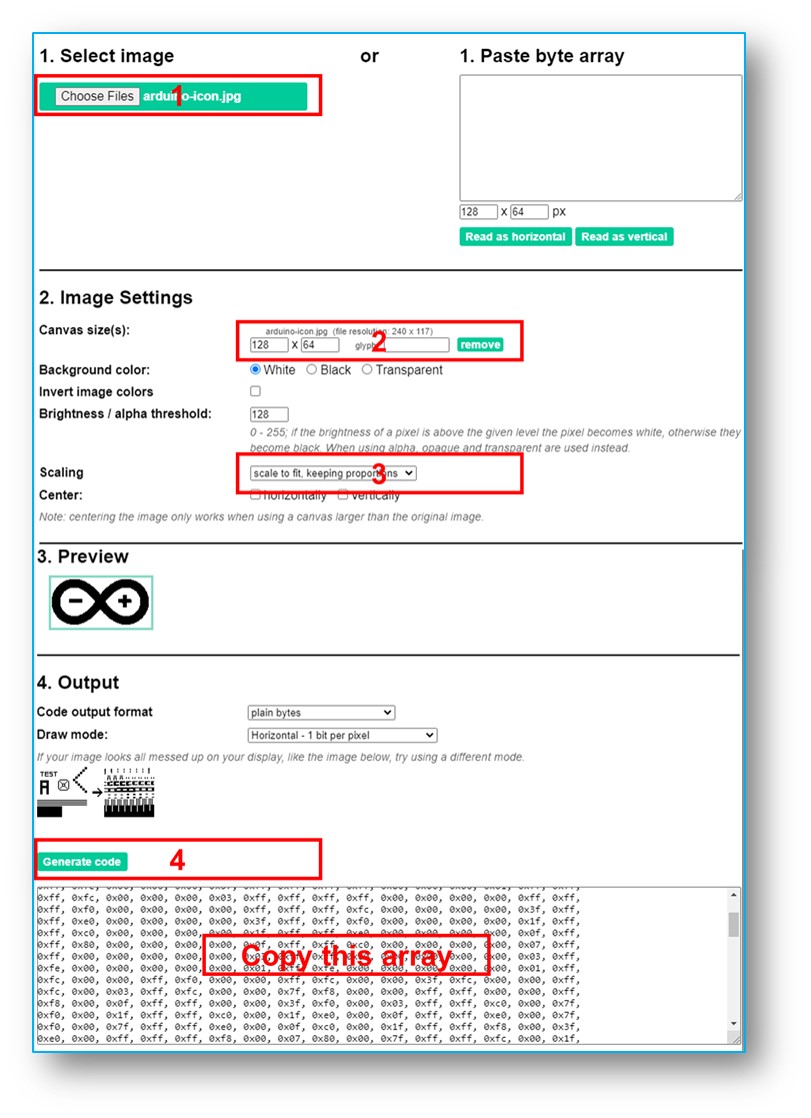
Copy the new array code and update it in the Arduino icon array in the code below.
The below video shown how to do it with OLED 128x64 display and Arduino Uno and Arduino icon
We can do similarly to make it work with Arduino Uno R4 and OLED 128x32. The below code display DIYables icon on OLED 128x32
※ NOTE THAT:
- The picture size must be as small as or smaller than the screen size.
- To use the given code for an OLED 128x32, you must resize the image and adjust the width and height in the oled.drawBitmap(); function.
How to vertical and horizontal center align text/number on OLED
OLED Troubleshooting
If the OLED screen shows nothing, please follow these steps:
- Ensure the wiring is done properly.
- Confirm that your I2c OLED is equipped with an SSD1306 driver.
- Verify the I2C address of your OLED using the following I2C Address Scanner code on Arduino UNO R4.
The output on the Serial Monitor: