Arduino UNO R4 - 74HC595 4-Digit 7-Segment Display
A typical 4-digit 7-segment display is used in projects like clocks, timers, and counters, and usually needs 12 connections. The 74HC595 module simplifies this by using only 5 connections: 2 for power and 3 to control the segments.
This tutorial will not give you too much complex information about hardware. Instead, you will learn how to connect a 4-digit 7-segment display to an Arduino UNO R4 and how to program it to display information.
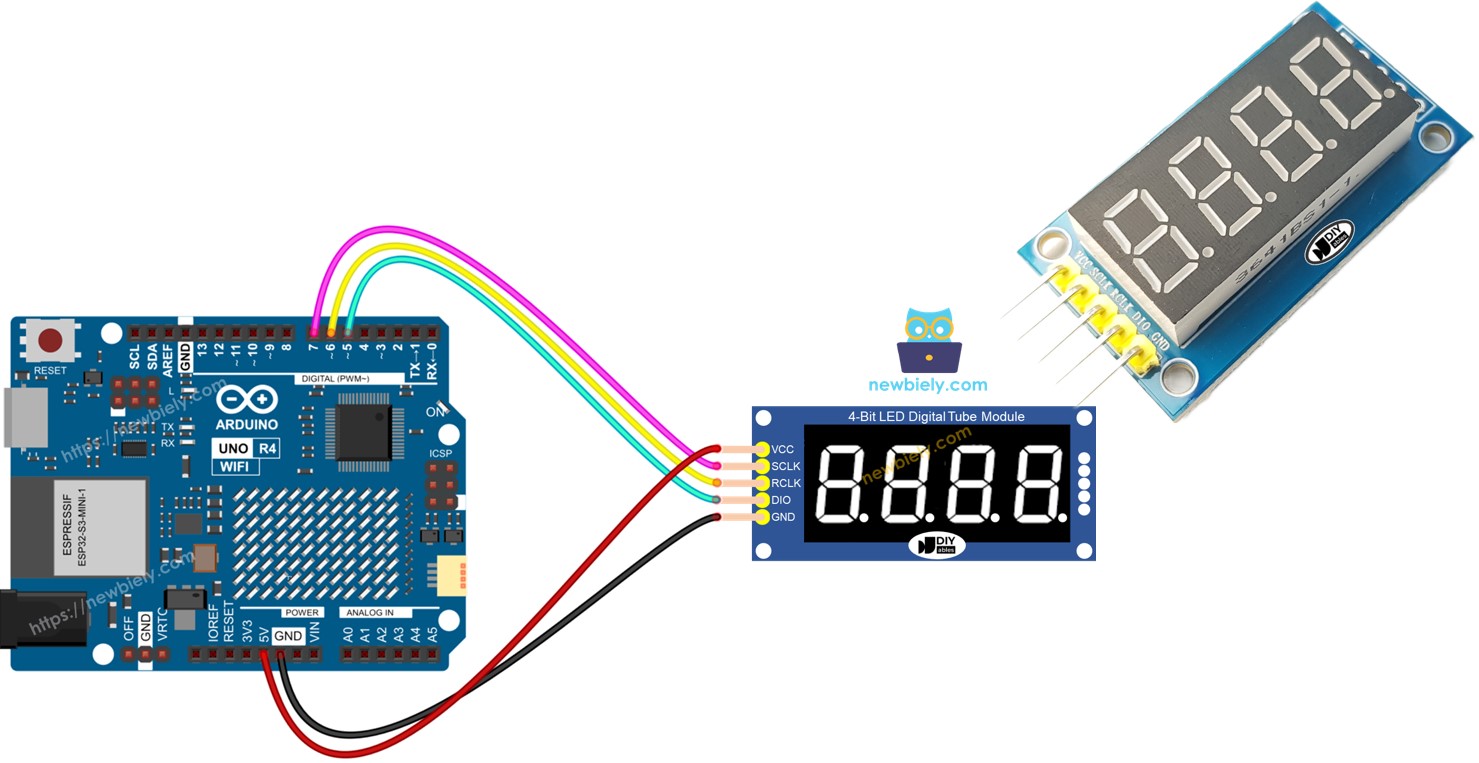
This guide will show how to use the 4-dot, 4-digit, 7-seegment display module that can show float values. If you need to display a colon separator, please use the TM1637 4-digit 7-segment Display Module.
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of 74HC595 4-digit 7-segment Display
A 74HC595 4-digit 7-segment display module usually includes 4 7-segment LEDs, 4 small dot LEDs, and a 74HC595 driver for each digit. It is perfect for showing temperatures or any numbers with decimals.
Pinout
The 74HC595 module for a 4-digit 7-segment display has 5 pins:
- SCLK pin: This is a clock input pin. Connect it to any digital pin on the Arduino UNO R4.
- RCLK pin: This is another clock input pin. Connect it to any digital pin on the Arduino UNO R4.
- DIO pin: This is a Data Input/Output pin. Connect it to any digital pin on the Arduino UNO R4.
- VCC pin: This pin provides power to the module. Connect it to a power supply between 3.3V and 5V.
- GND pin: This is a ground pin. Connect it to the ground.
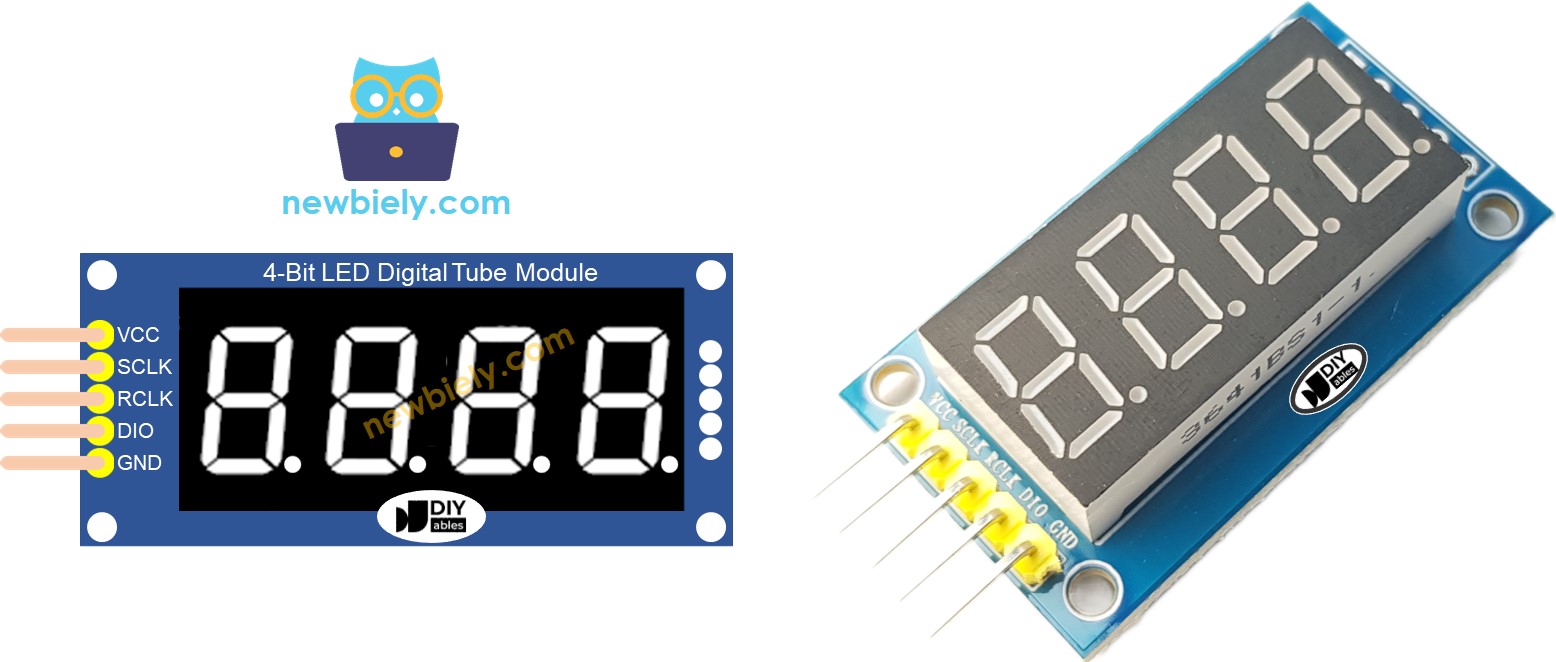
Wiring Diagram
This table shows how to connect the Arduino UNO R4 pins to a 74HC595 4-digit 7-segment display:
Arduino UNO R4 | 74HC595 7-segment display |
---|---|
5V | 5V |
7 | SCLK |
6 | RCLK |
5 | DIO |
If you use different pins, change the pin numbers in the code.
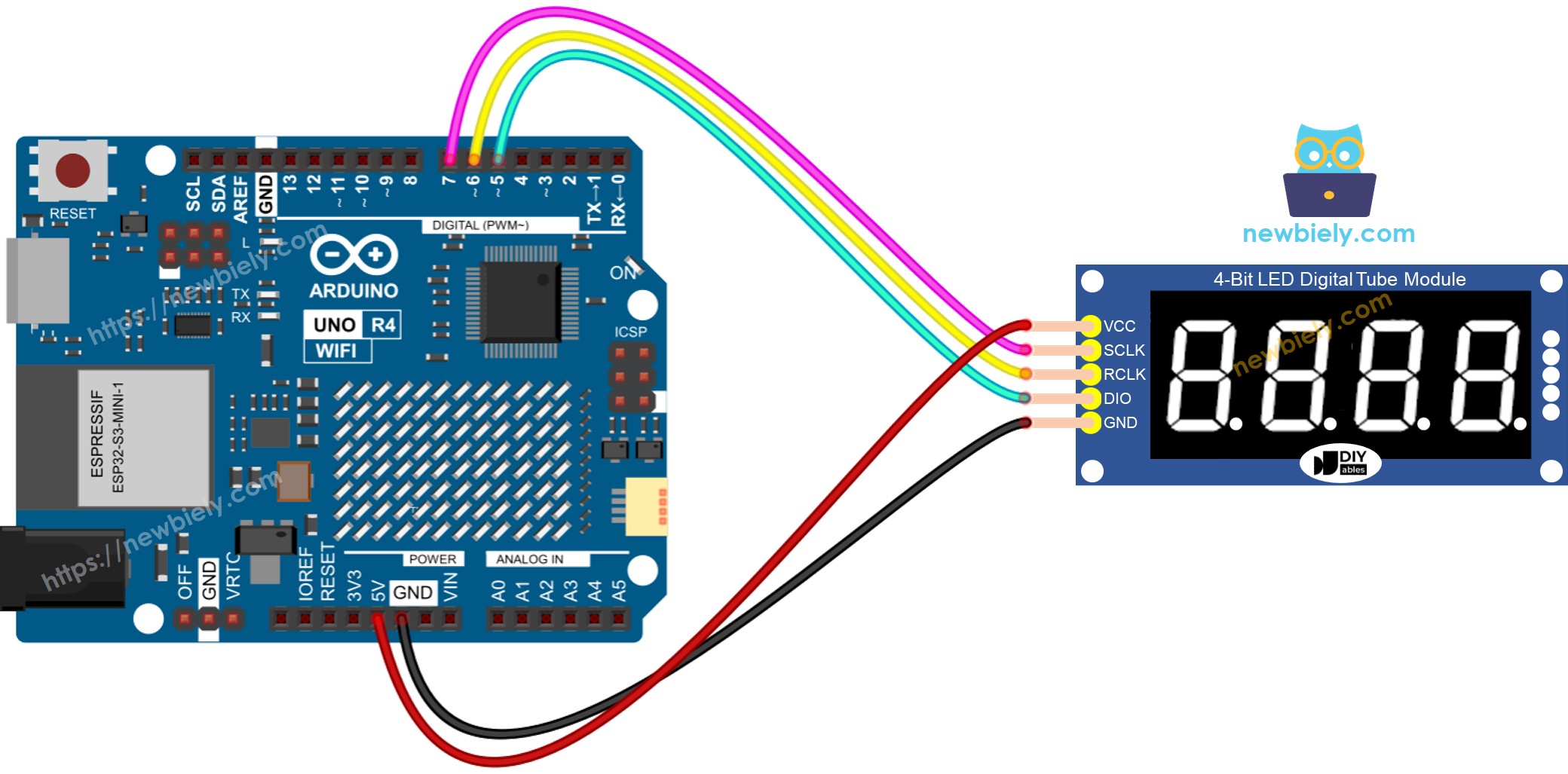
This image is created using Fritzing. Click to enlarge image
See The best way to supply power to the Arduino Uno R4 and other components.
Library Installation
To easily set up programming for a 74HC595 4-digit 7-segment Display, install the DIYables_4Digit7Segment_74HC595 library from DIYables.io. Here are the steps to install the library:
- Click on the Libraries icon on the left side of the Arduino IDE.
- Type “DIYables_4Digit7Segment_74HC595” in the search box, and look for the DIYables_4Digit7Segment_74HC595 library from DIYables.io
- Press the Install button.
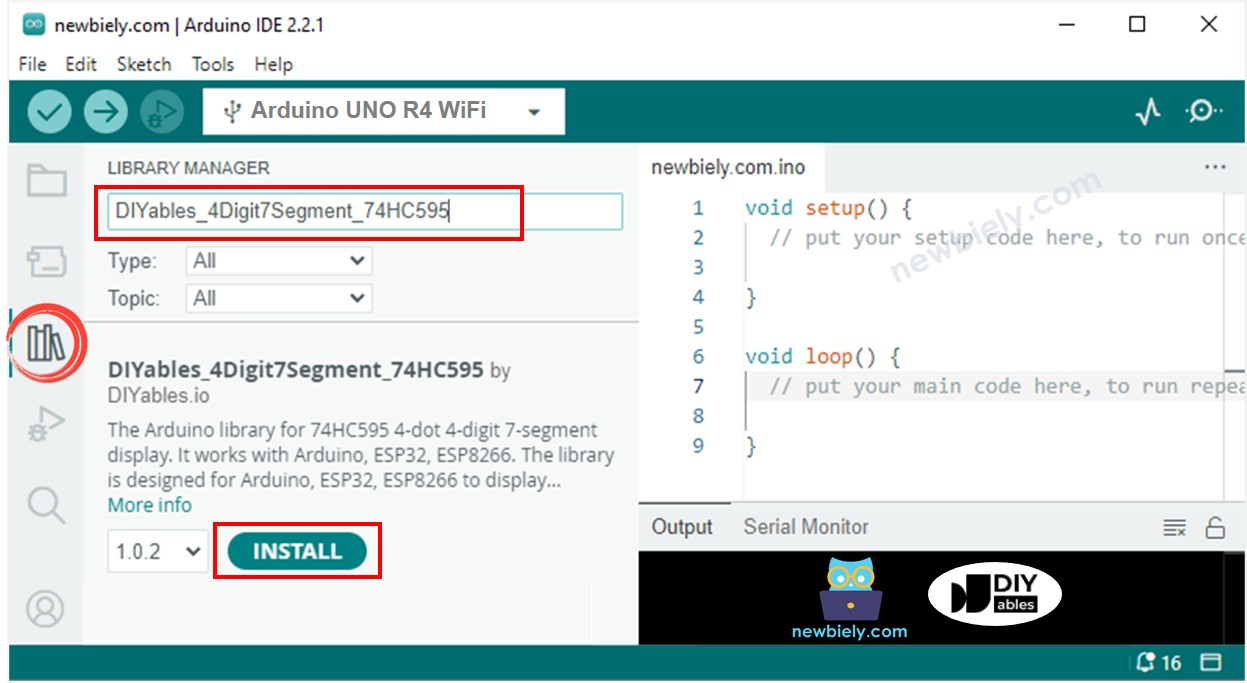
You can also view this library on Github
How To Program For 74HC595 4-digit 7-segment using Arduino UNO R4
- Include the library
- Connect the Arduino UNO R4 to the display module like this: D7 to SCLK, D6 to RCLK, and D5 to DIO.
- Make a display item called DIYables_4Digit7Segment_74HC595.
- You can show whole numbers with leading zeros, including negative numbers.
- You can show numbers with decimal points, add zeros in front, and include negative numbers.
- You can also show each number, decimal point, or character one by one using basic functions.
- The 74HC595 4-digit 7-segment module controls each segment and LED by using a method called multiplexing. Therefore, the Arduino UNO R4 code MUST:
- Use the display.show() function in the main loop.
- Avoid using the delay() function in the main loop.
For more details, visit the library reference.
Arduino UNO R4 Code - Display Integer
Detailed Instructions
Follow these instructions step by step:
- If this is your first time using the Arduino Uno R4 WiFi/Minima, refer to the tutorial on setting up the environment for Arduino Uno R4 WiFi/Minima in the Arduino IDE.
- Connect the 74HC595 4-digit 7-segment Display to the Arduino Uno R4 according to the provided diagram.
- Connect the Arduino Uno R4 board to your computer using a USB cable.
- Launch the Arduino IDE on your computer.
- Select the appropriate Arduino Uno R4 board (e.g., Arduino Uno R4 WiFi) and COM port.
- Copy the code and open it using Arduino IDE.
- Click the Upload button in Arduino IDE to send the code to Arduino UNO R4.
- Observe the changes on the 7-segment display.