Arduino UNO R4 - RTC Real-Time Clock
This tutorial instructs you how to use Arduino UNO R4 with RTC (Real-Time Clock). In detail, we will learn:
- How to connect Arduino UNO R4's RTC to backup battery
- How to program Arduino UNO R4 to get date and time (seconds, minutes, hours, days, months, years) from RTC
- How to program Arduino UNO R4 to update the date and time for RTC from Internet via NTP (Network Time Protocol)
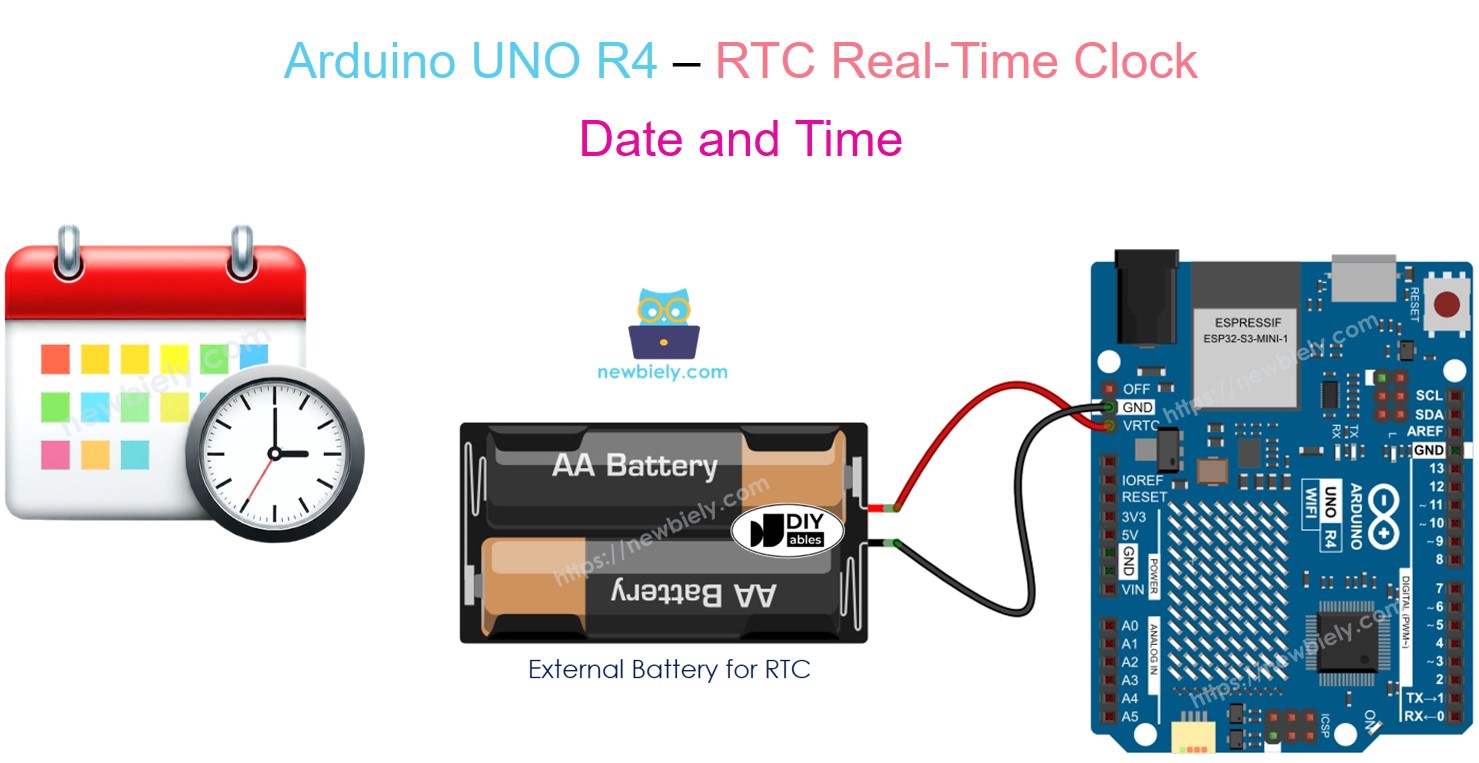
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables.
Overview of Real-Time Clock on Arduino UNO R4
Both the Arduino UNO R4 Minima and WiFi version have a built-in Real-Time Clock (RTC) embedded within its main microcontroller. This RTC allows your Arduino projects to keep track of date and time even when the board is turned off momentarily. You can access and control the RTC using the RTC library included in the Arduino IDE when you install the board package for your Arduino UNO R4. The library allows you to set the current date and time, read the current date and time, and set alarms for specific times.
However, there's a crucial point to consider regarding backup power:
- Arduino UNO R4 Minima: Arduino UNO R4 Minima doesn't have a dedicated backup battery for RTC. This means if the board completely loses power, the RTC resets, and you'll need to set the time again when you power it back on.
- Arduino UNO R4 WiFi: Arduino UNO R4 WiFi doesn't includes backup battery for RTC. However, it offers a solution to use an external backup battery for RTC. You can connect an external battery to power RTC on Arduino UNO R3 WiFi via the VRTC (Voltage for Real-Time Clock)** pin. By supplying an external voltage (between 1.6V and 3.6V) to this pin, you can provide backup power to the RTC. This ensures the clock keeps running even when the main power supply is off, allowing you to retain the time settings.
You can provide backup power for the RTC using two AA batteries with a battery holder, as shown in the following wiring diagram
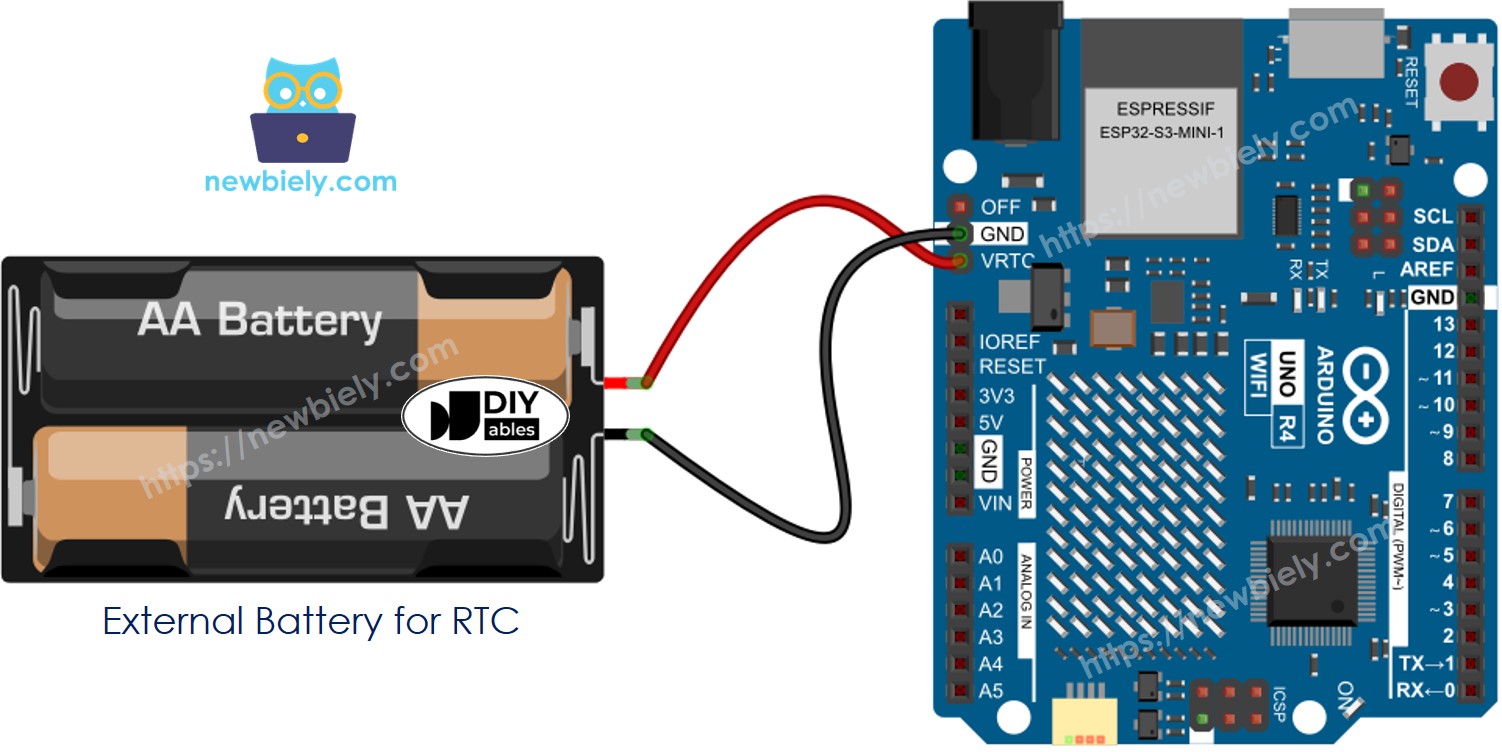
This image is created using Fritzing. Click to enlarge image
※ NOTE THAT:
That battery power source is for RTC only. You need to power Arduino UNO R4 via a separate power supply.
How To Program For RTC On Arduino UNO R4
- Add the library:
- Initialize the RTC:
- Check if the RTC is configured or not, if not, set the date and time.
- Displays date and time from the clock module
Arduino UNO R4 Code – How to get data and time
Detailed Instructions
Follow these instructions step by step:
- If this is your first time using the Arduino UNO R4 WiFi/Minima, refer to the tutorial on setting up the environment for Arduino UNO R4 WiFi/Minima in the Arduino IDE.
- If you use Arduino UNO R4 WiFi, Connect the Arduino UNO R4 WiFi to the backup battery according to the provided diagram.
- Connect the Arduino UNO R4 board to your computer using a USB cable.
- Launch the Arduino IDE on your computer.
- Select the appropriate Arduino UNO R4 board (e.g., Arduino UNO R4 WiFi) and COM port.
- Copy the code and paste it into Arduino IDE.
- Modify the time on the code to match your current time
- Press the Upload button in Arduino IDE to send the code to Arduino UNO R4.
- Open the Serial Monitor.
- Check the result on the Serial Monitor.
Everything looks fine, but wait! There could be a problem in the following scenarios:
- You use an Arduino UNO R4 WiFi without a backup battery or an Arduino UNO R4 Minima.
- The Arduino loses power temporarily and reboots. The Arduino then sets the current time to the time specified in the code, but real-time has passed. This leads to incorrect time information.
Solutions for this:
- Use a stable power source for the Arduino.
- Use a stable backup battery (only works for the UNO R4 WiFi).
- Update the RTC time from the Internet via Network Time Protocol (NTP) each time the Arduino reboots (requires an Internet connection).
Let's see how to use NTP to update the time on the Arduino UNO R4's RTC:
Arduino UNO R4 Code – Update RTC time via NTP
Detailed Instructions
- Open the Library Manager by clicking on the Library Manager icon on the left side of the Arduino IDE.
- Search for “NTPClient” and locate the NTPClient by Fabrice Weinberg.
- Click on the Install button to add the NTPClient library.
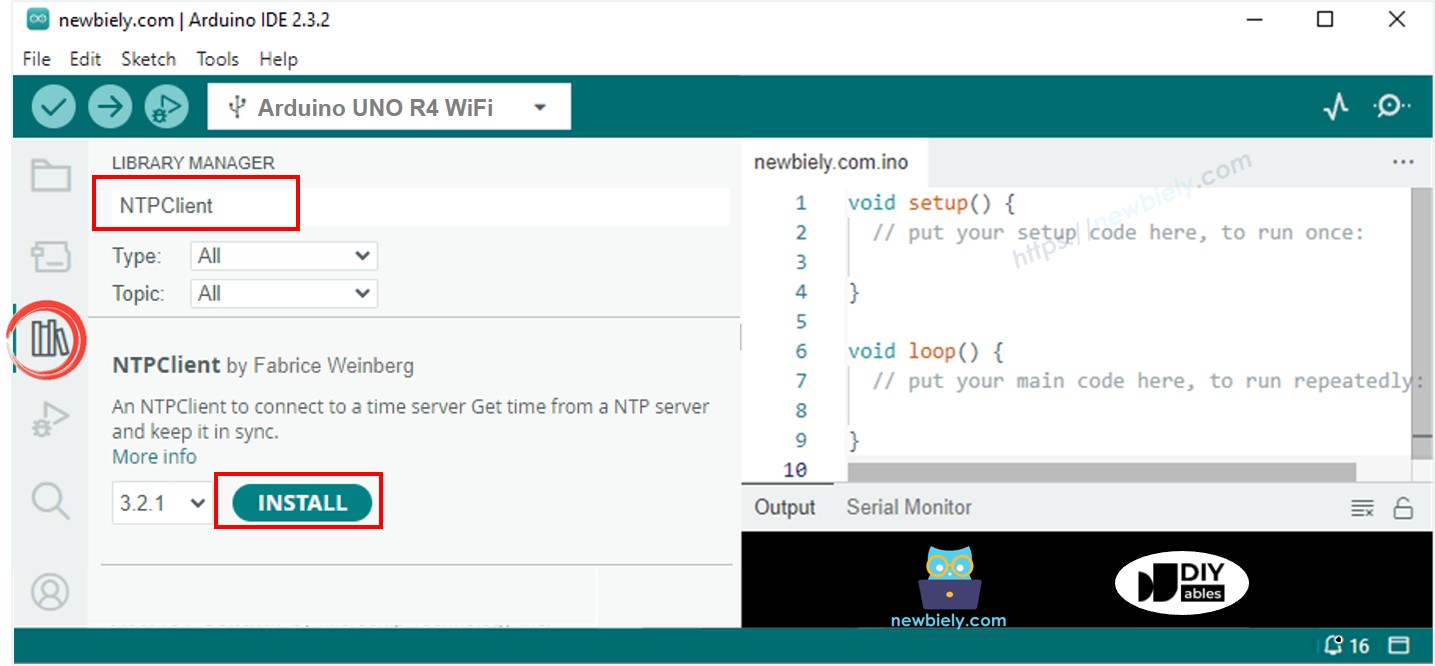
- Copy the code and paste it into Arduino IDE.
- Modify the WiFi SSID and password on the code to match your network credentials
- Press the Upload button in Arduino IDE to send the code to Arduino UNO R4.
- Open the Serial Monitor.
- Check the result on the Serial Monitor.