Arduino UNO R4 - Button
The button is a simple but important component used in many Arduino UNO R4 projects. It might seem complicated because of its mechanical and physical properties, which can be challenging for beginners. This tutorial is designed to help beginners understand it easily. Let's begin!
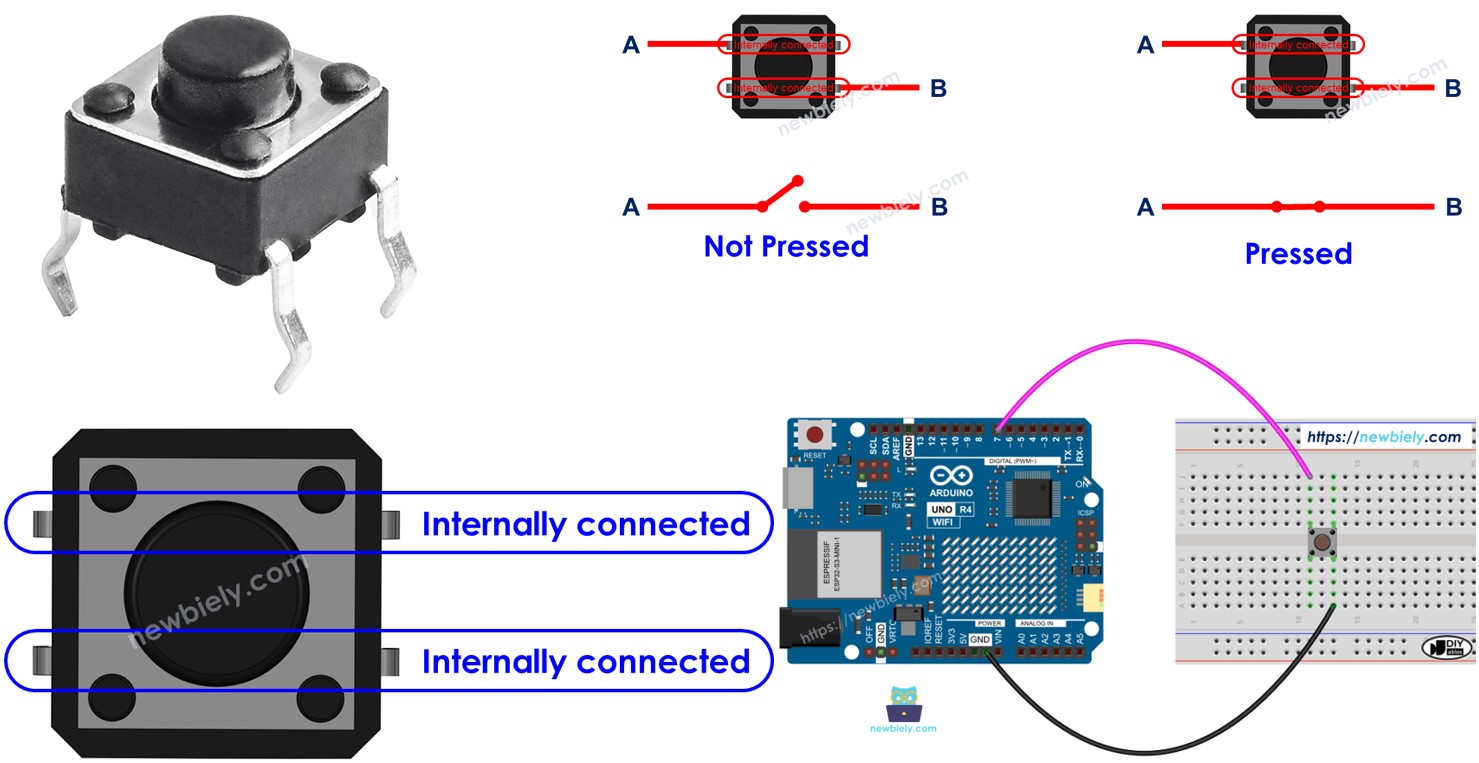
※ NOTE THAT:
Before we learn about how to use the button with Arduino Uno R4, we want to highlight two common errors often encountered by beginners:
The floating input problem:
Symptom: When a button is connected to an Arduino UNO R4 input pin, the input pin's state is unpredictable and does not reflect to the button state.
Cause: The button pin is not connected to a pull-down or pull-up resistor.
Solution: Connect a pull-down or a pull-up resistor to the input pin. More details will be provided later in this tutorial.
The chattering phenomenon:
Symptom: The Arduino UNO R4's code reads the button's state and tries to identify press events by detecting changes in state (from HIGH to LOW, or LOW to HIGH). However, when the button is pressed just once, the Arduino Uno R4 may detect multiple presses.
Cause: Mechanical properties cause the input pin's state to rapidly toggle between LOW and HIGH several times with only one press.
Chattering only affects applications that require a precise count of button presses. In other applications, it may not be a problem.
Or you can buy the following kits:
Disclosure: Some of the links provided in this section are Amazon affiliate links. We may receive a commission for any purchases made through these links at no additional cost to you.
Additionally, some of these links are for products from our own brand,
DIYables .
The push button, also known as a tactile button or momentary switch, is a switch that is closed when you press and hold it down, and opens when you release it. Push buttons come in different types, mainly divided into two groups:
These pins are connected together inside in pairs, so we only need to use two out of the four pins that are not connected together inside.
There are four ways, but really two because they are similar, to connect the button (refer to the image).
A button has four pins, but why do we only use two? ⇒ This is to keep it stable on the PCB (board) and withstand the pressure.
image source: diyables.io
GND: Connect this pin to ground.
VCC: Connect this pin to a 5V or 3.3V power supply.
OUT: Connect this pin to a digital input on your Arduino.
With this configuration, the module outputs LOW when the button is not pressed and outputs HIGH when the button is pressed.
When the button is not pressed, pin A and pin B are not connected.
When the button is pressed, pin A and pin B are connected.
One button pin connects to VCC or GND, and the other pin connects to a pin on the Arduino UNO R4.
We can determine if the button is pressed or not by checking the state of a pin on the Arduino UNO R4 set as an input pin.
The relationship between the button state and the pressing state depends on how we connect the button to the Arduino UNO R4 and the settings of the Arduino UNO R4's pin.
There are two ways to use a button with Arduino UNO R4:
Connect one button pin to VCC and the other to an Arduino UNO R4 pin with a pull-down resistor.
When the button is pressed, the Arduino UNO R4 pin state is HIGH. Otherwise, the Arduino UNO R4 pin state is LOW.
You MUST use an external resistor.
Connect one button pin to GND and the other to an Arduino UNO R4 pin with a pull-up resistor.
When the button is pressed, the Arduino UNO R4 pin state is LOW. Otherwise, the Arduino UNO R4 pin state is HIGH.
You can use either an internal or external resistor. The internal resistor is built into the Arduino UNO R4 and can be activated via Arduino code.
※ NOTE THAT:
If we do not use a pull-down or pull-up resistor, the input pin is "floating" when the button is not pressed. This means the state of the pin might change unpredictably to HIGH or LOW, causing incorrect readings.
The worst practice: Set up the Arduino UNO R4 pin as an input using pinMode(BUTTON_PIN, INPUT) without any pull-down or pull-up resistor.
The best practice: Set up the Arduino UNO R4 pin with an internal pull-up resistor using pinMode(BUTTON_PIN, INPUT_PULLUP). This does not require an external resistor.
To make it easy for beginners, this tutorial uses the simplest method: setting up the Arduino UNO R4 pin as an internal pull-up input without needing an external resistor. Beginners do not need to worry about connecting the pull-up or pull-down resistor. They just need to use the provided Arduino code.
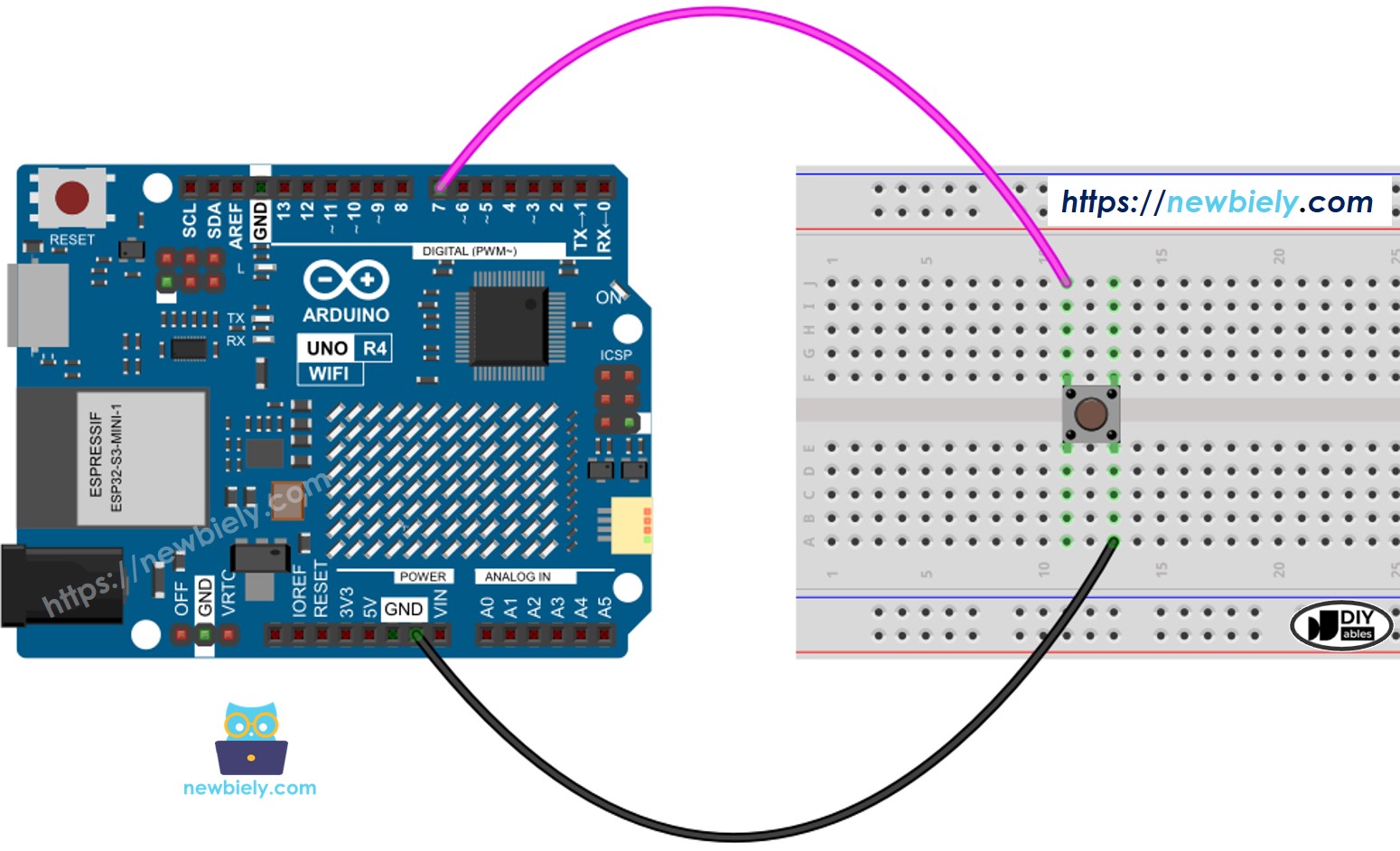
This image is created using Fritzing. Click to enlarge image
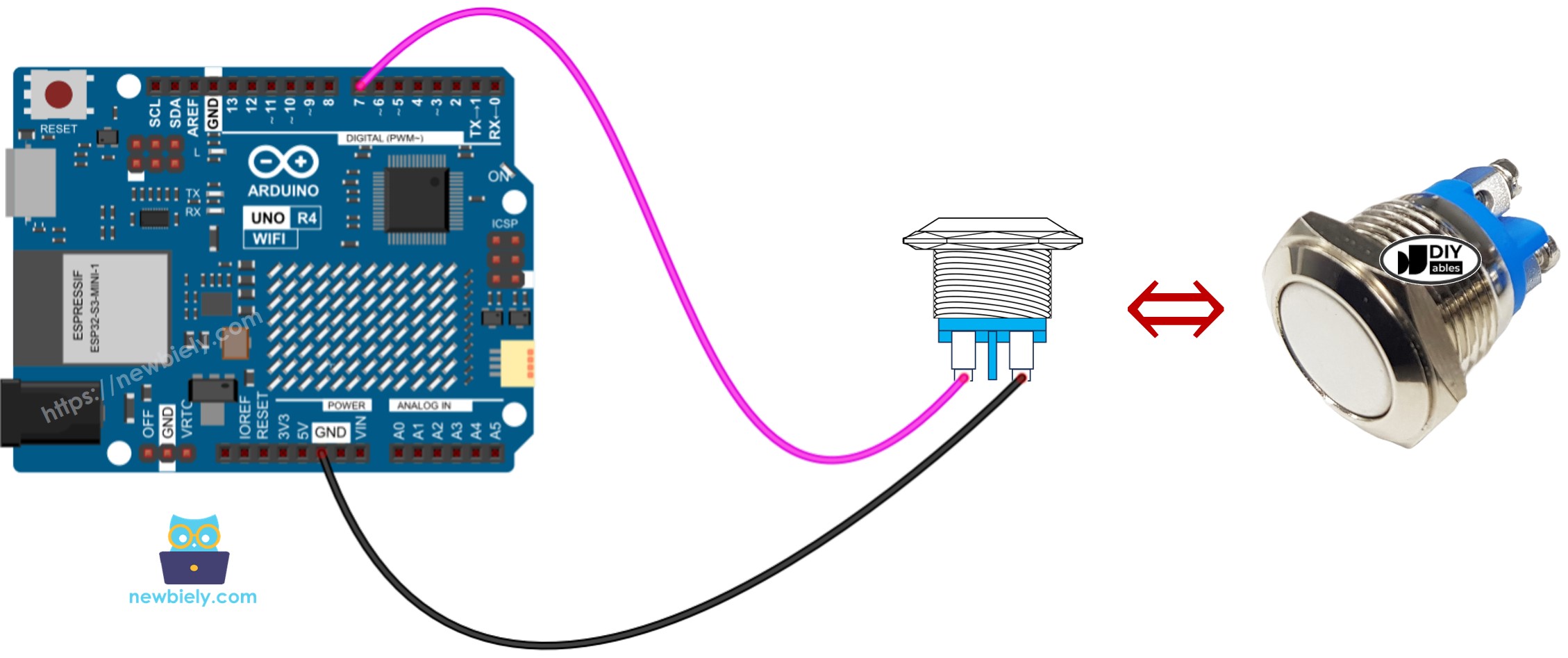
This image is created using Fritzing. Click to enlarge image
See The best way to supply power to the Arduino Uno R4 and other components.
pinMode(7, INPUT_PULLUP);
int button_state = digitalRead(BUTTON_PIN);
※ NOTE THAT:
There are two common use-cases:
The first: When the input is HIGH, perform an action. When the input is LOW, perform the opposite action.
The second: When the input changes from LOW to HIGH (or HIGH to LOW), perform an action.
Based on the purpose, we select one of these options. For instance, when using a button to control an LED:
If we need the LED to turn ON when the button is pressed and turn OFF when it is not pressed, we should choose the first scenario.
If we want the LED to switch between ON and OFF each time the button is pressed, we should opt for the second scenario.
#define BUTTON_PIN 7
void setup() {
Serial.begin(9600);
pinMode(BUTTON_PIN, INPUT_PULLUP);
}
void loop() {
int button_state = digitalRead(BUTTON_PIN);
Serial.println(button_state);
delay(500);
}
Follow these instructions step by step:
Connect the button to Arduino UNO R4 according to the provided diagram.
Connect the Arduino Uno R4 board to your computer using a USB cable.
Launch the Arduino IDE on your computer.
Select the appropriate Arduino Uno R4 board (e.g., Arduino Uno R4 WiFi) and COM port.
Copy the above code and open it in the Arduino IDE
Click the Upload button in Arduino IDE to send the code to your Arduino UNO R4.
1 means ON, 0 means OFF.
The explanation is in the comments section of the Arduino code above.
Let's change the code to recognize when buttons are pressed and released.
#define BUTTON_PIN 7
int button_state;
int prev_button_state = LOW;
void setup() {
Serial.begin(9600);
pinMode(BUTTON_PIN, INPUT_PULLUP);
}
void loop() {
button_state = digitalRead(BUTTON_PIN);
if (prev_button_state == HIGH && button_state == LOW)
Serial.println("The button is pressed");
else if (prev_button_state == LOW && button_state == HIGH)
Serial.println("The button is released");
prev_button_state = button_state;
}
Copy the above code and paste it to Arduino IDE
Click the Upload button in Arduino IDE to send code to Arduino UNO R4.
Open the Serial Monitor.
Press and release the button.
Check out the result on the Serial Monitor.
The button is pressed
The button is released
※ NOTE THAT:
Even if you press and release the button just once, the Serial Monitor might display multiple press and release events. This usual behavior is known as "chattering phenomenon". You can find more details in the
Arduino UNO R4 - Button Debounce tutorial.
If you are using the
button module, set the pin to input mode using
pinMode(BUTTON_PIN, INPUT). In this configuration, the module outputs
LOW when the button is not pressed and
HIGH when the button is pressed.