Arduino UNO R4 - Email
This tutorial instructs you how to program the Arduino UNO R4 to send an email from your Gmail account.
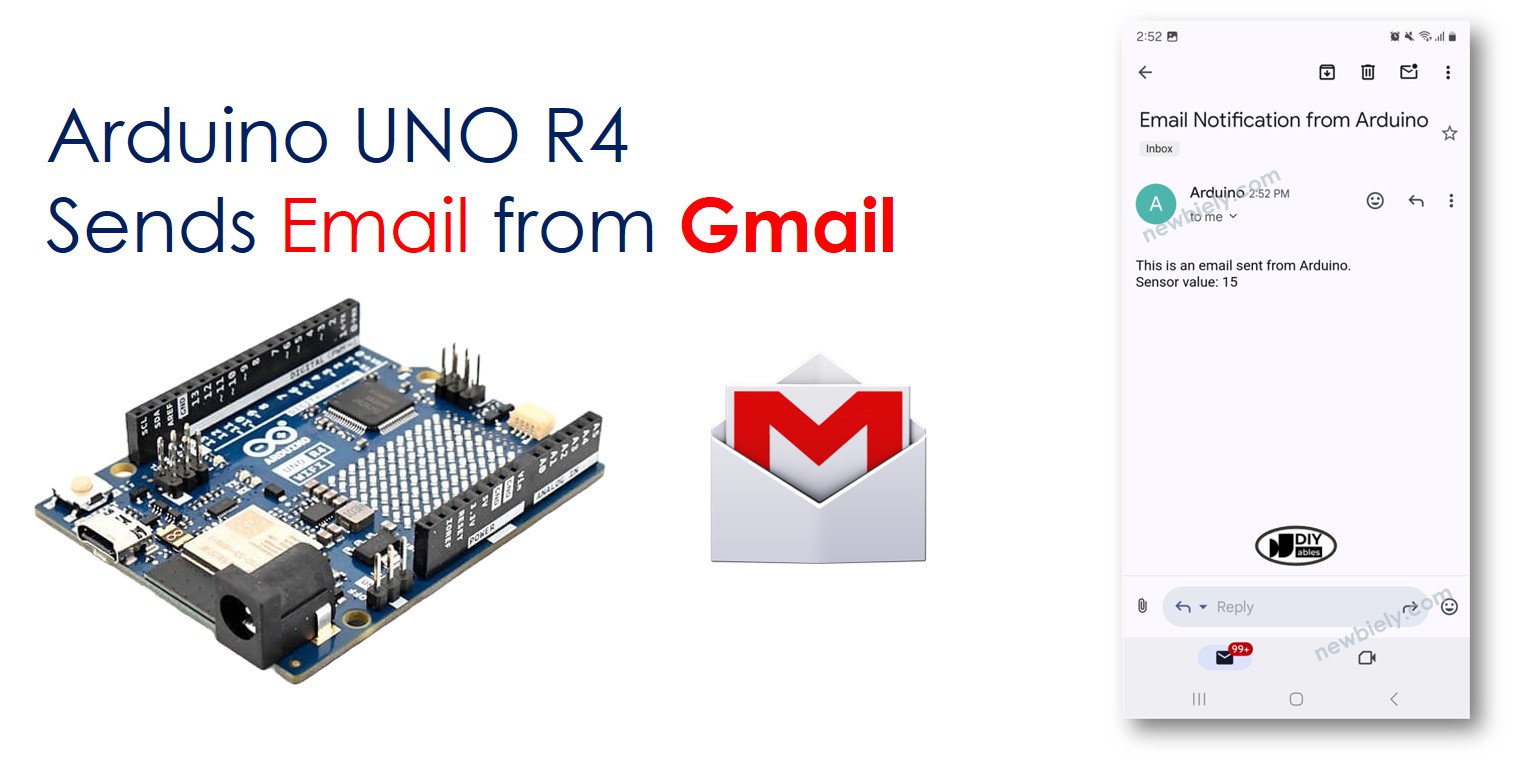
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Disclosure: Some of the links provided in this section are Amazon affiliate links. We may receive a commission for any purchases made through these links at no additional cost to you.
Additionally, some of these links are for products from our own brand, DIYables .
Additionally, some of these links are for products from our own brand, DIYables .
Pre-Preparation
To use the code, you need a Gmail account and a special password. Here are the key details to remember:
- Create a new Gmail account specifically for testing purposes. Do not use your regular account to protect your account from security issues.
- The password in the Arduino UNO R4 code is not the same as your Gmail login password. You need to generate an "App Password" from your Google Account.
You can generate an "App Password" from your Google Account by following below steps:
- Create a new Gmail account.
- Log into your newly created account.
- Access your Google Account.
- Navigate to the "Security" area.
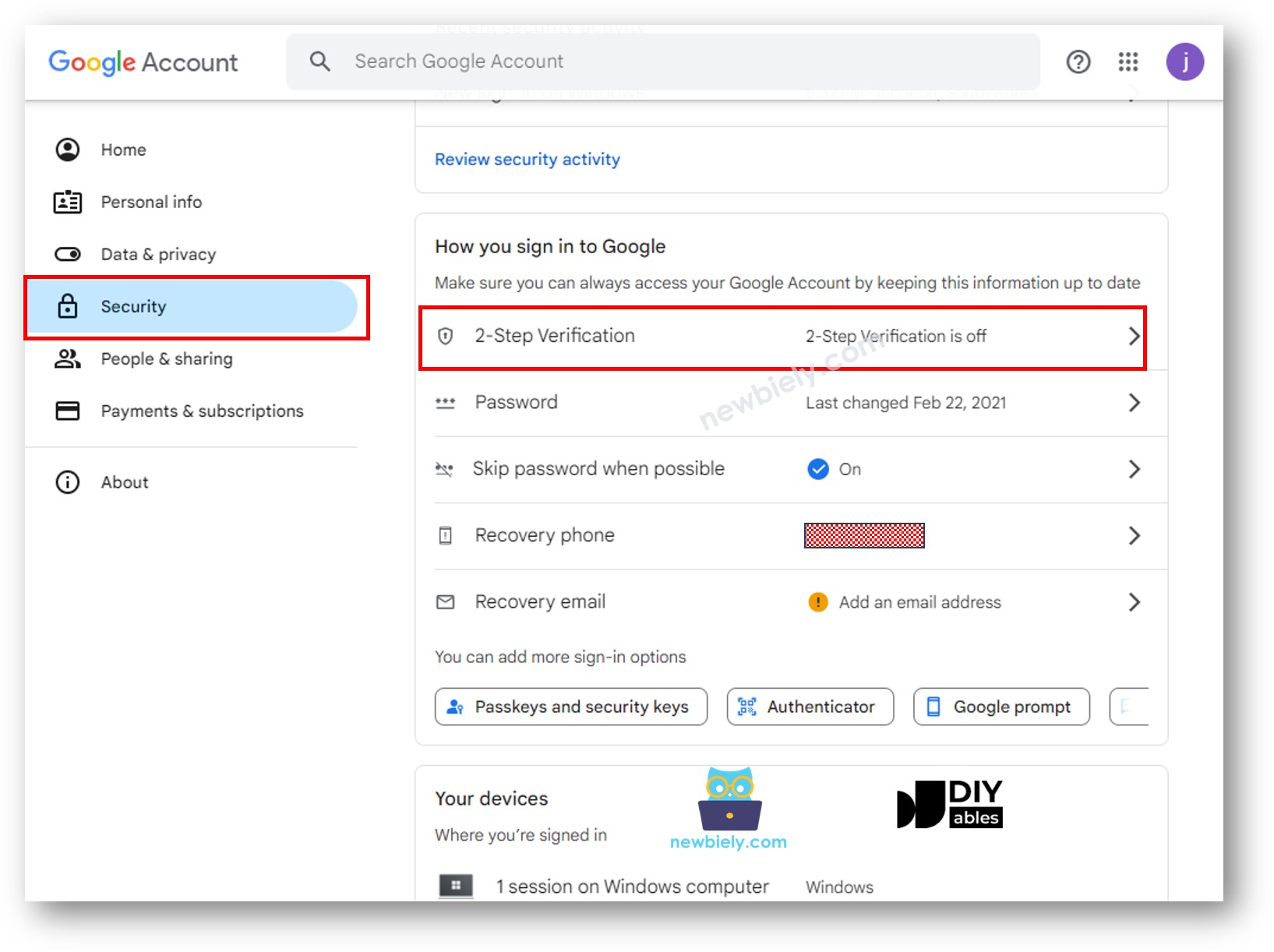
- Enable 2-Step Verification (It's necessary to enable 2-Step Verification to create app passwords).
- Visit the Google App Passwords webpage and create an app password. You can name it anything you like.
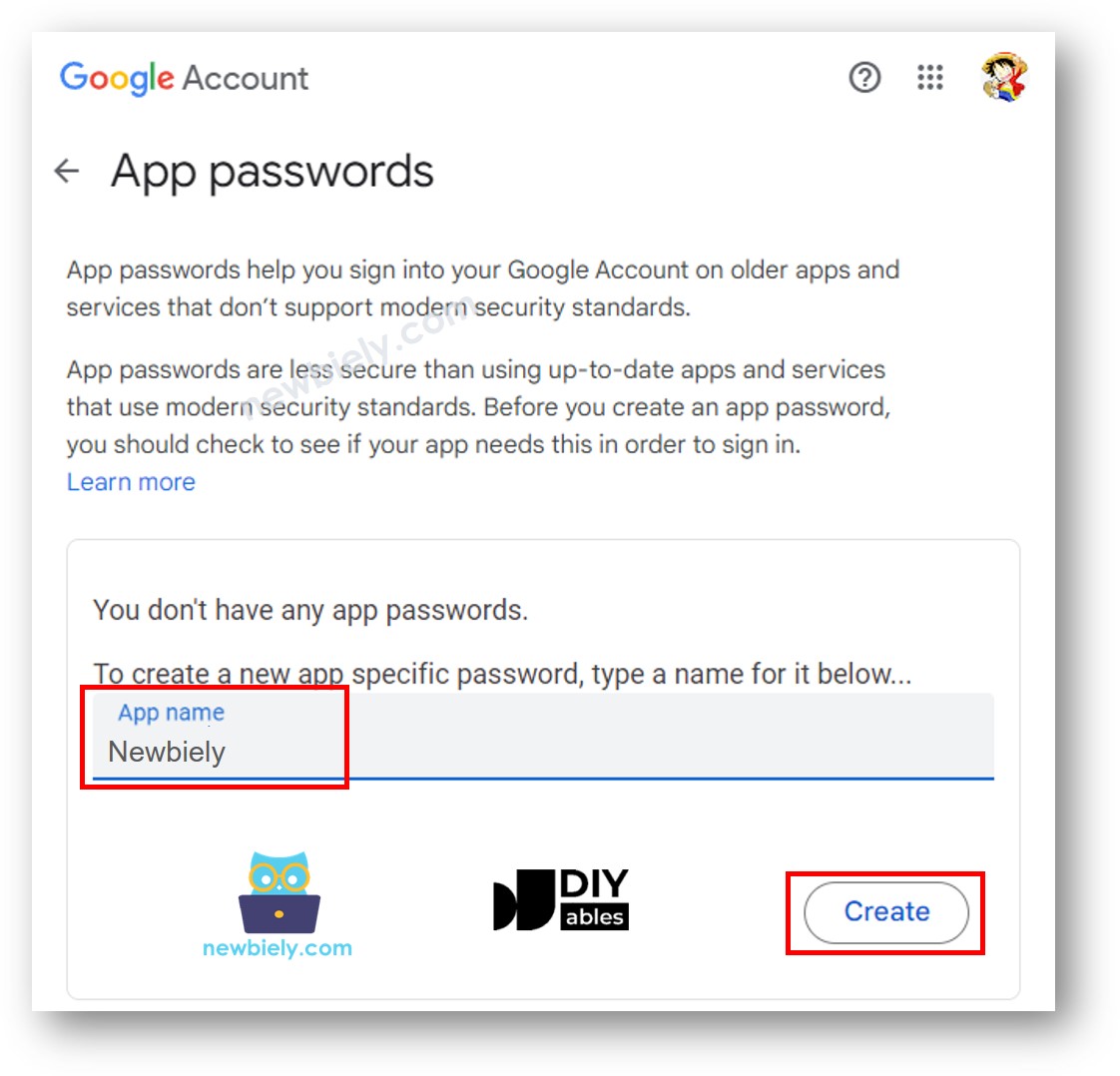
- Click the Create button to receive a 16-digit password displayed as follows:
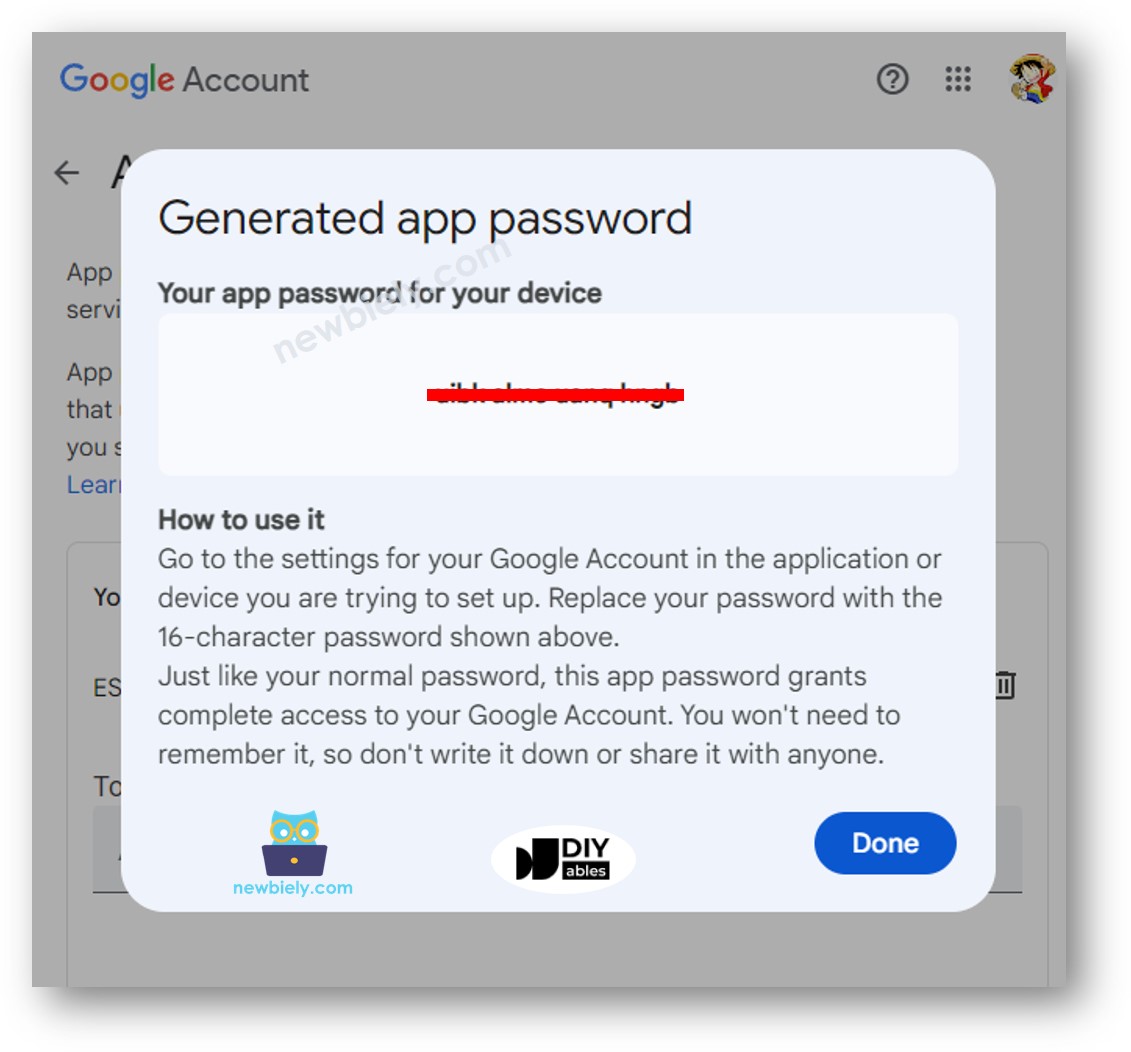
- Please keep and save this 16-digit number. You will need it for the Arduino UNO R4 code in the next step.
※ NOTE THAT:
Google's UI may be updated. If you cannot locate "App Passwords" as described, search online for "How to get Google App Passwords" to find the latest instructions.
Arduino UNO R4 Code
/*
* This Arduino UNO R4 code was developed by newbiely.com
*
* This Arduino UNO R4 code is made available for public use without any restriction
*
* For comprehensive instructions and wiring diagrams, please visit:
* https://newbiely.com/tutorials/arduino-uno-r4/arduino-uno-r4-email
*/
#include <WiFiS3.h>
#include <ESP_Mail_Client.h>
#define WIFI_SSID "YOUR_WIFI_SSID" // CHANGE IT
#define WIFI_PASSWORD "YOUR_WIFI_PASSWORD" // CHANGE IT
// the sender email credentials
#define SENDER_EMAIL "xxxxxx@gmail.com" // CHANGE IT
#define SENDER_PASSWORD "xxxx xxxx xxxx xxxx" // CHANGE IT to your Google App password
#define RECIPIENT_EMAIL "xxxxxx@gmail.com" // CHANGE IT
#define SMTP_HOST "smtp.gmail.com"
#define SMTP_PORT 587
SMTPSession smtp;
void setup() {
Serial.begin(9600);
WiFi.begin(WIFI_SSID, WIFI_PASSWORD);
Serial.print("Connecting to Wi-Fi");
while (WiFi.status() != WL_CONNECTED) {
Serial.print(".");
delay(300);
}
Serial.println();
Serial.print("Connected with IP: ");
Serial.println(WiFi.localIP());
Serial.println();
String subject = "Email Notification from Arduino";
String textMsg = "This is an email sent from Arduino.\n";
textMsg += "Sensor value: ";
textMsg += "15"; // OR replace this value read from a sensor
gmail_send(subject, textMsg);
}
void loop() {
// YOUR OTHER CODE HERE
}
void gmail_send(String subject, String textMsg) {
// set the network reconnection option
MailClient.networkReconnect(true);
smtp.debug(1);
smtp.callback(smtpCallback);
Session_Config config;
// set the session config
config.server.host_name = SMTP_HOST;
config.server.port = SMTP_PORT;
config.login.email = SENDER_EMAIL;
config.login.password = SENDER_PASSWORD;
config.login.user_domain = F("127.0.0.1");
config.time.ntp_server = F("pool.ntp.org,time.nist.gov");
config.time.gmt_offset = 3;
config.time.day_light_offset = 0;
// declare the message class
SMTP_Message message;
// set the message headers
message.sender.name = F("Arduino");
message.sender.email = SENDER_EMAIL;
message.subject = subject;
message.addRecipient(F("To Whom It May Concern"), RECIPIENT_EMAIL);
message.text.content = textMsg;
message.text.transfer_encoding = "base64";
message.text.charSet = F("utf-8");
message.priority = esp_mail_smtp_priority::esp_mail_smtp_priority_low;
// set the custom message header
message.addHeader(F("Message-ID: <abcde.fghij@gmail.com>"));
// connect to the server
if (!smtp.connect(&config)) {
Serial.println("Connection error: ");
Serial.print("- Status Code: ");
Serial.println(smtp.statusCode());
Serial.print("- Error Code: ");
Serial.println(smtp.errorCode());
Serial.print("- Reason: ");
Serial.println(smtp.errorReason().c_str());
return;
}
if (!smtp.isLoggedIn()) {
Serial.println("Not yet logged in.");
} else {
if (smtp.isAuthenticated())
Serial.println("Successfully logged in.");
else
Serial.println("Connected with no Auth.");
}
// start sending Email and close the session
if (!MailClient.sendMail(&smtp, &message)) {
Serial.println("Connection error: ");
Serial.print("- Status Code: ");
Serial.println(smtp.statusCode());
Serial.print("- Error Code: ");
Serial.println(smtp.errorCode());
Serial.print("- Reason: ");
Serial.println(smtp.errorReason().c_str());
}
}
// callback function to get the Email sending status
void smtpCallback(SMTP_Status status) {
// print the current status
Serial.println(status.info());
// print the sending result
if (status.success()) {
for (size_t i = 0; i < smtp.sendingResult.size(); i++) {
// get the result item
SMTP_Result result = smtp.sendingResult.getItem(i);
Serial.print("Status: ");
if (result.completed)
Serial.println("success");
else
Serial.println("failed");
Serial.print("Recipient: ");
Serial.println(result.recipients.c_str());
Serial.print("Subject: ");
Serial.println(result.subject.c_str());
}
Serial.println("----------------\n");
// free the memory
smtp.sendingResult.clear();
}
}
Detailed Instructions
Follow these instructions step by step:
- If this is your first time using the Arduino Uno R4 WiFi/Minima, refer to the tutorial on setting up the environment for Arduino Uno R4 WiFi/Minima in the Arduino IDE.
- Connect the Arduino Uno R4 board to your computer using a USB cable.
- Launch the Arduino IDE on your computer.
- Choose the correct Arduino UNO R4 board option (Arduino UNO R4 R4 WiFi) and COM port.
- Open the Library Manager by clicking on the Library Manager icon on the left side of the Arduino IDE screen.
- Search for ESP Mail Client to find the ESP Mail Client by Mobizt.
- Click the Install button to add the ESP Mail Client library.
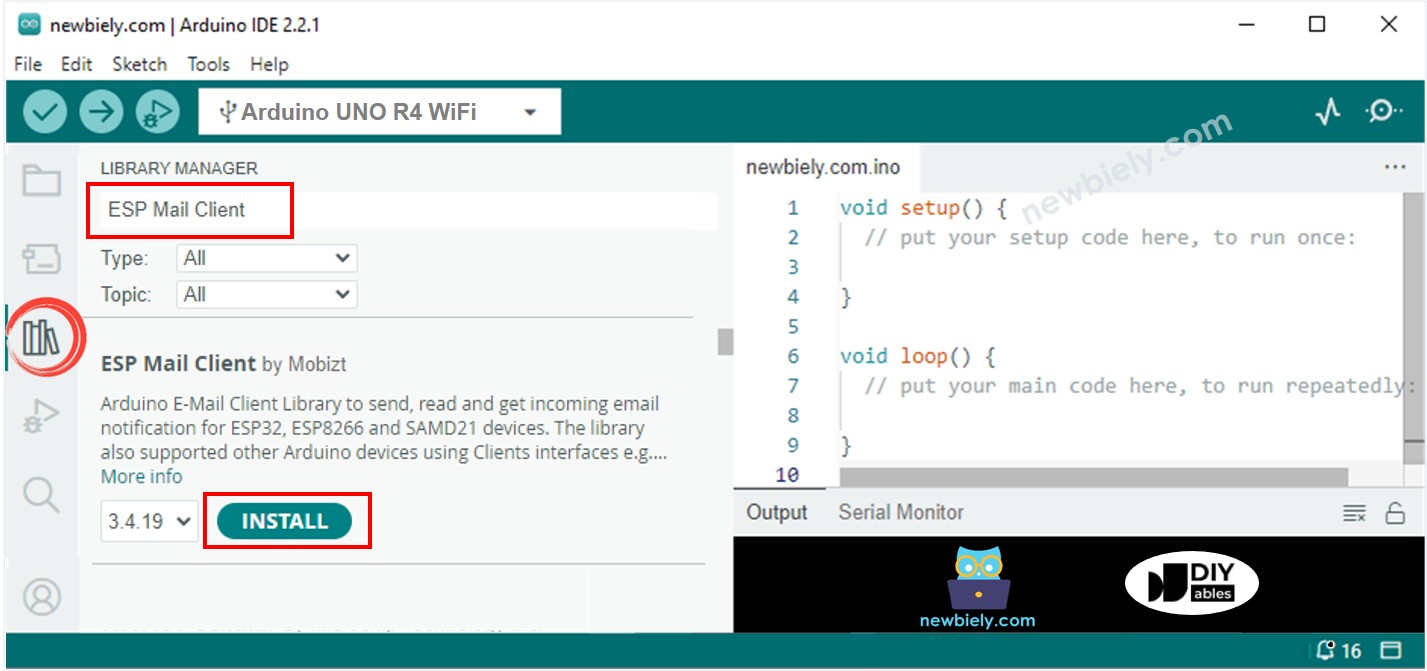
- Copy the code above and open it in the Arduino IDE.
- Update your WiFi network name and password where it says WIFI_SSID and WIFI_PASSWORD.
- Update your email and password in the constants: SENDER_EMAIL and SENDER_PASSWORD.
- Update RECIPIENT_EMAIL with the email address where you want to receive messages. This can be the same as the sender's email.
※ NOTE THAT:
- The sender's email should use Gmail.
- Use the App password given before as the sender's password.
- Any email type can be the recipient's email.
- Press the Upload button in Arduino IDE to upload the code to Arduino UNO R4.
- Open the Serial Monitor.
- View the output on Serial Monitor.
COM6
#### Message sent successfully
> C: message sent successfully
----------------
Message sent success: 1
Message sent failed: 0
----------------
Message No: 1
Status: success
Date/Time: May 27, 2024 04:42:50
Recipient: xxxxxx@gmail.com
Subject: Email Notification from Arduino
----------------
Autoscroll
Clear output
9600 baud
Newline
- Look in the email inbox of the receiver. You will find an email similar to this:
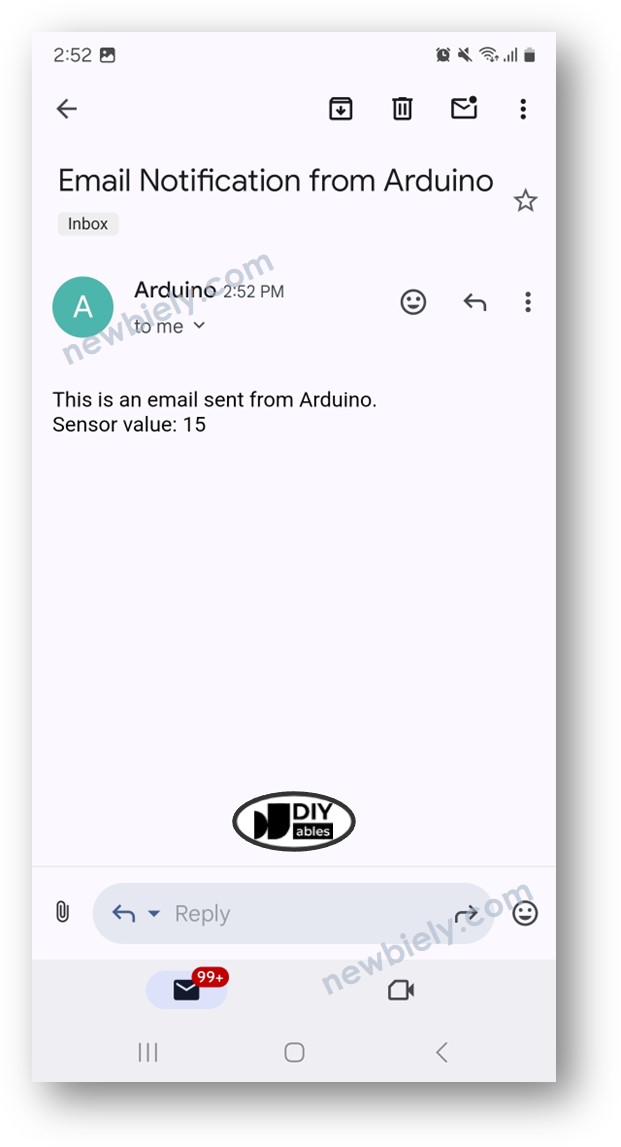