Arduino UNO R4 - DHT11 Temperature Humidity Sensor - OLED
In this guide, we will learn to measure temperature and humidity using a DHT11 module and show it on an OLED screen.
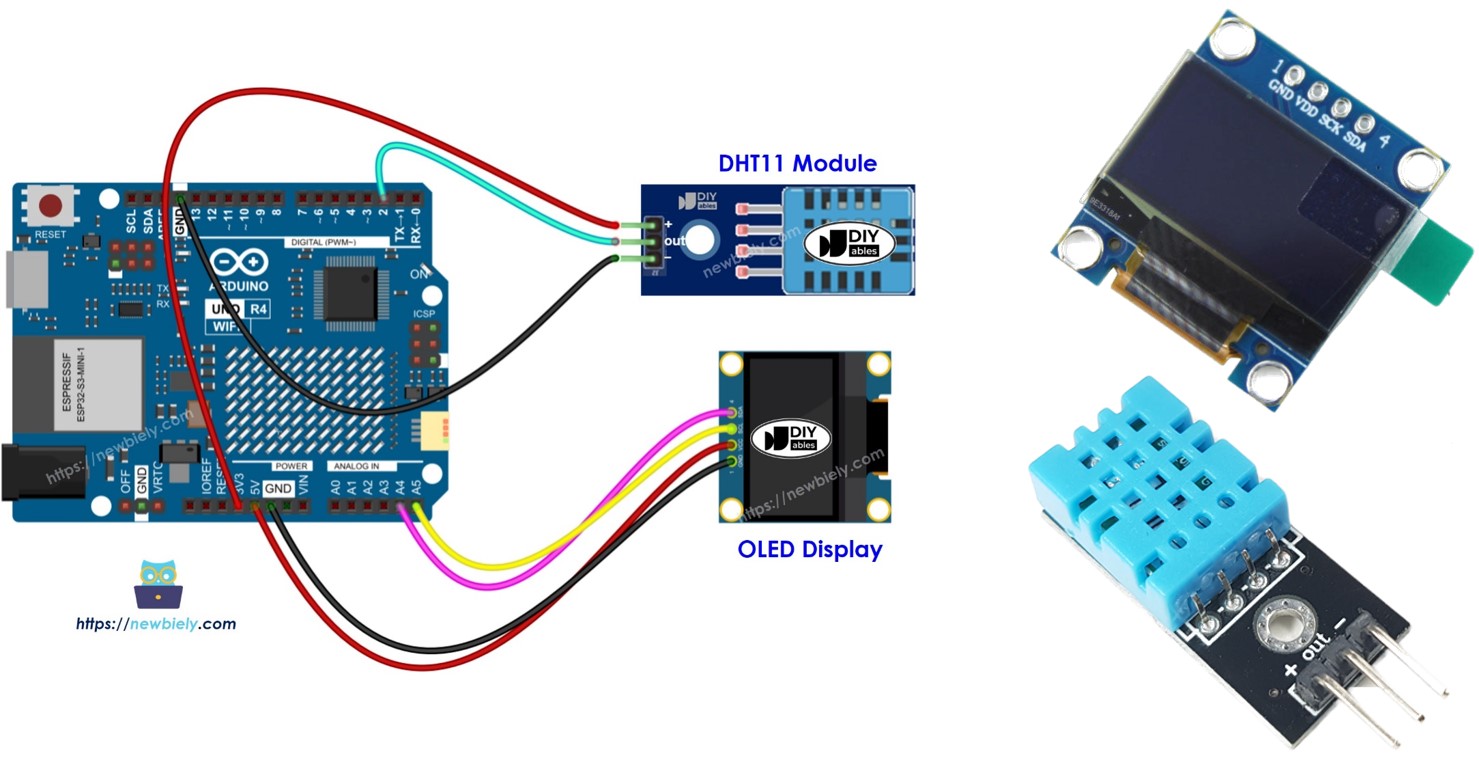
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Disclosure: Some of the links provided in this section are Amazon affiliate links. We may receive a commission for any purchases made through these links at no additional cost to you.
Additionally, some of these links are for products from our own brand, DIYables.
Additionally, some of these links are for products from our own brand, DIYables.
Overview of OLED display, DHT11 Temperature Humidity Sensor
Learn about OLED displays and DHT11 temperature humidity sensors (their setup, functions, and programming) in the tutorials below:
- Arduino UNO R4 - OLED tutorial
- Arduino UNO R4 - DHT11 tutorial
Wiring Diagram
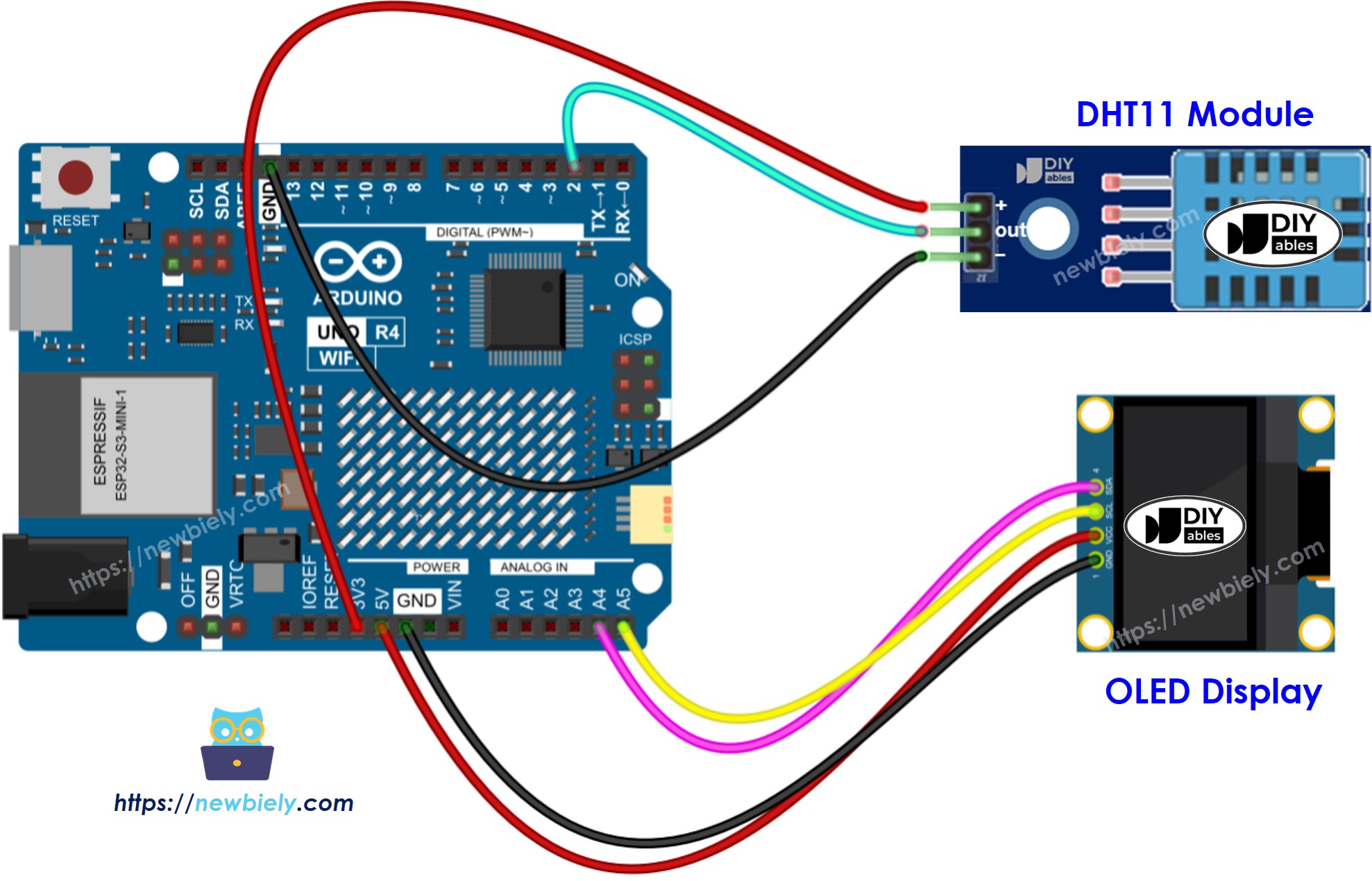
This image is created using Fritzing. Click to enlarge image
Arduino UNO R4 Code - DHT11 Sensor - OLED
/*
* This Arduino UNO R4 code was developed by newbiely.com
*
* This Arduino UNO R4 code is made available for public use without any restriction
*
* For comprehensive instructions and wiring diagrams, please visit:
* https://newbiely.com/tutorials/arduino-uno-r4/arduino-uno-r4-dht11-temperature-humidity-sensor-oled
*/
#include <Wire.h>
#include <Adafruit_GFX.h>
#include <Adafruit_SSD1306.h>
#include <DHT.h>
#define SCREEN_WIDTH 128 // OLED display width, in pixels
#define SCREEN_HEIGHT 64 // OLED display height, in pixels
#define DHT11_PIN 2 // The Arduino UNO R4 pin connected to DHT11 sensor
Adafruit_SSD1306 oled(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, -1); // create SSD1306 display object connected to I2C
DHT dht11(DHT11_PIN, DHT11);
String temperature;
String humidity;
void setup() {
Serial.begin(9600);
// initialize OLED display with address 0x3C for 128x64
if (!oled.begin(SSD1306_SWITCHCAPVCC, 0x3C)) {
Serial.println(F("SSD1306 allocation failed"));
while (true)
;
}
delay(2000); // wait for initializing
oled.clearDisplay(); // clear display
oled.setTextSize(3); // text size
oled.setTextColor(WHITE); // text color
oled.setCursor(0, 10); // position to display
dht11.begin(); // initialize DHT11 the temperature and humidity sensor
temperature.reserve(10); // to avoid fragmenting memory when using String
humidity.reserve(10); // to avoid fragmenting memory when using String
}
void loop() {
float humi = dht11.readHumidity(); // read humidity
float tempC = dht11.readTemperature(); // read temperature
// check if any reads failed
if (isnan(humi) || isnan(tempC)) {
temperature = "Failed";
humidity = "Failed";
} else {
temperature = String(tempC, 1); // one decimal places
temperature += char(247); // degree character
temperature += "C";
humidity = String(humi, 1); // one decimal places
humidity += "%";
}
Serial.print(tempC); // print to Serial Monitor
Serial.print("°C | " ); // print to Serial Monitor
Serial.print(humi); // print to Serial Monitor
Serial.println("%"); // print to Serial Monitor
oledDisplayCenter(temperature, humidity); // display temperature and humidity on OLED
}
void oledDisplayCenter(String temperature, String humidity) {
int16_t x1;
int16_t y1;
uint16_t width_T;
uint16_t height_T;
uint16_t width_H;
uint16_t height_H;
oled.getTextBounds(temperature, 0, 0, &x1, &y1, &width_T, &height_T);
oled.getTextBounds(temperature, 0, 0, &x1, &y1, &width_H, &height_H);
// display on horizontal and vertical center
oled.clearDisplay(); // clear display
oled.setCursor((SCREEN_WIDTH - width_T) / 2, SCREEN_HEIGHT / 2 - height_T - 5);
oled.println(temperature); // text to display
oled.setCursor((SCREEN_WIDTH - width_H) / 2, SCREEN_HEIGHT / 2 + 5);
oled.println(humidity); // text to display
oled.display();
}
Detailed Instructions
Follow these instructions step by step:
- If this is your first time using the Arduino Uno R4 WiFi/Minima, refer to the tutorial on setting up the environment for Arduino Uno R4 WiFi/Minima in the Arduino IDE.
- Connect the Arduino Uno R4 board to the DHT11 temperature and humidity sensor module and OLED display according to the provided diagram.
- Connect the Arduino Uno R4 board to your computer using a USB cable.
- Launch the Arduino IDE on your computer.
- Select the appropriate Arduino Uno R4 board (e.g., Arduino Uno R4 WiFi) and COM port.
- Go to the Libraries icon on the left side of the Arduino IDE.
- Type "SSD1306" in the search box, then look for the SSD1306 library by Adafruit.
- Press the Install button to add the library.
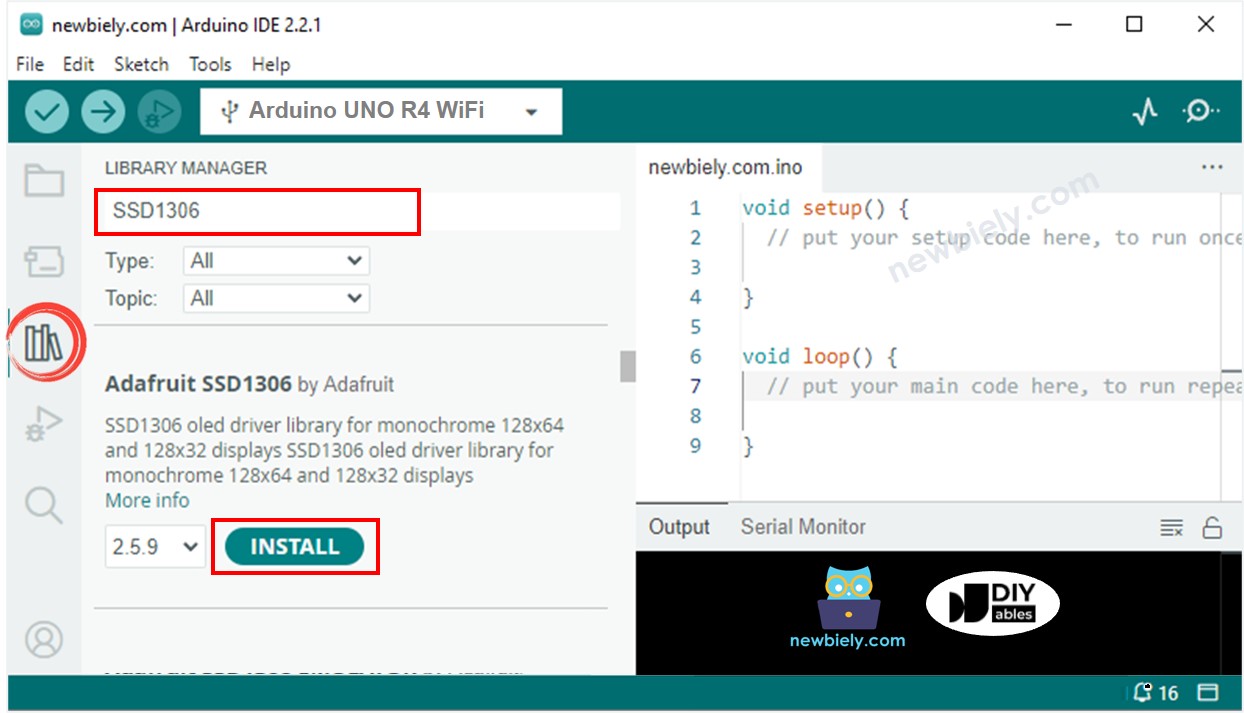
- You will need to install additional library dependencies.
- Click the Install All button to install all library dependencies.
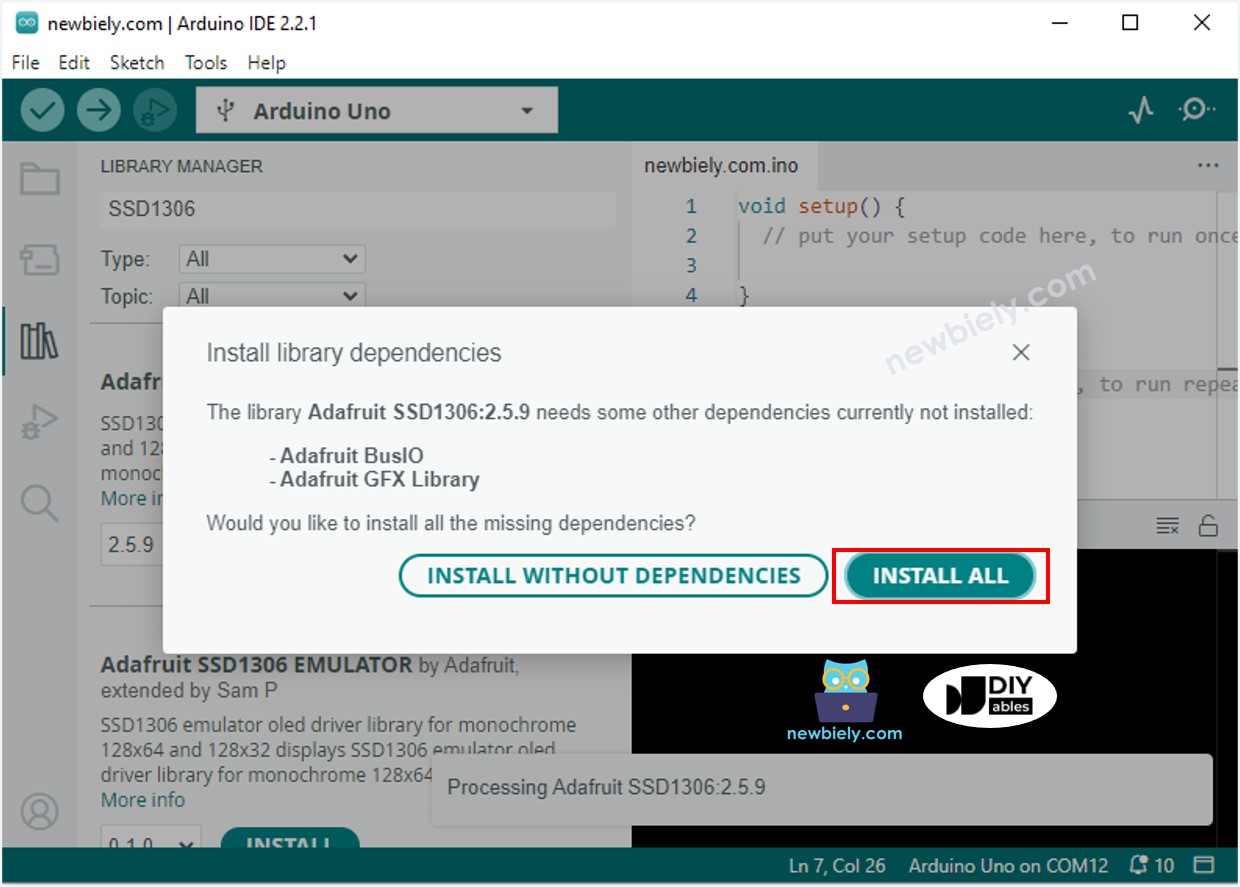
- Look up "DHT," then locate the DHT sensor library created by Adafruit.
- Press the Install button to add the library.
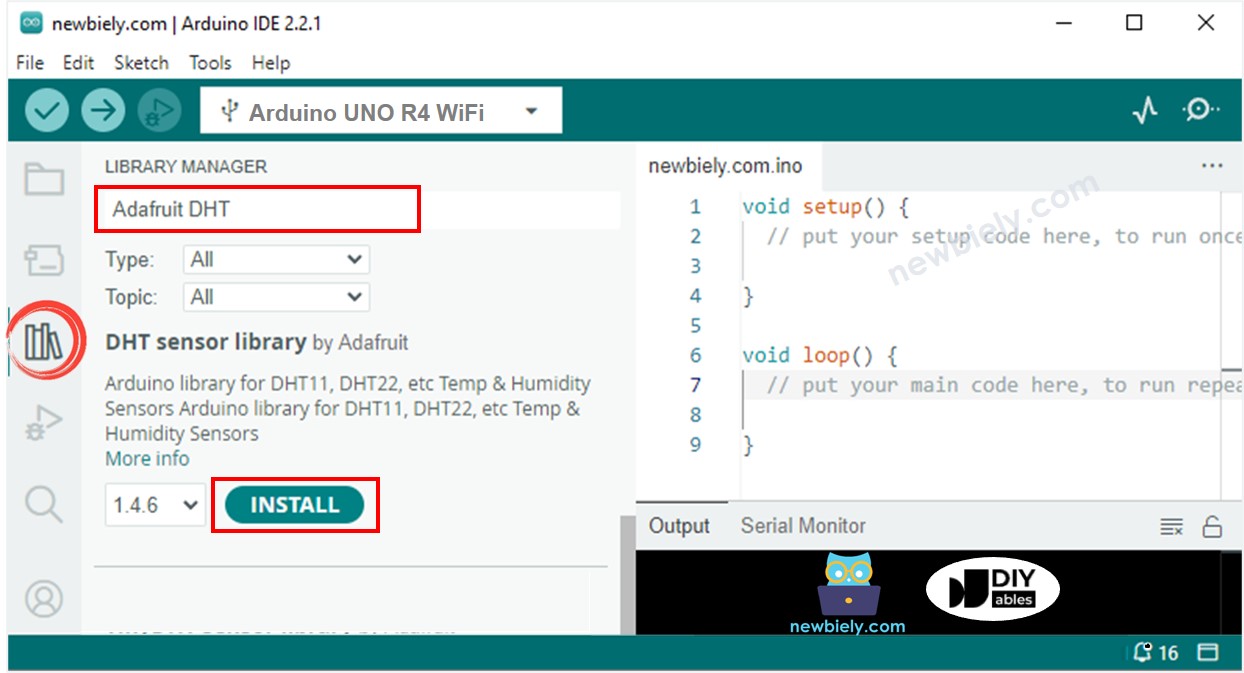
- You will need to install additional library dependencies.
- Click the Install All button to install all library dependencies.
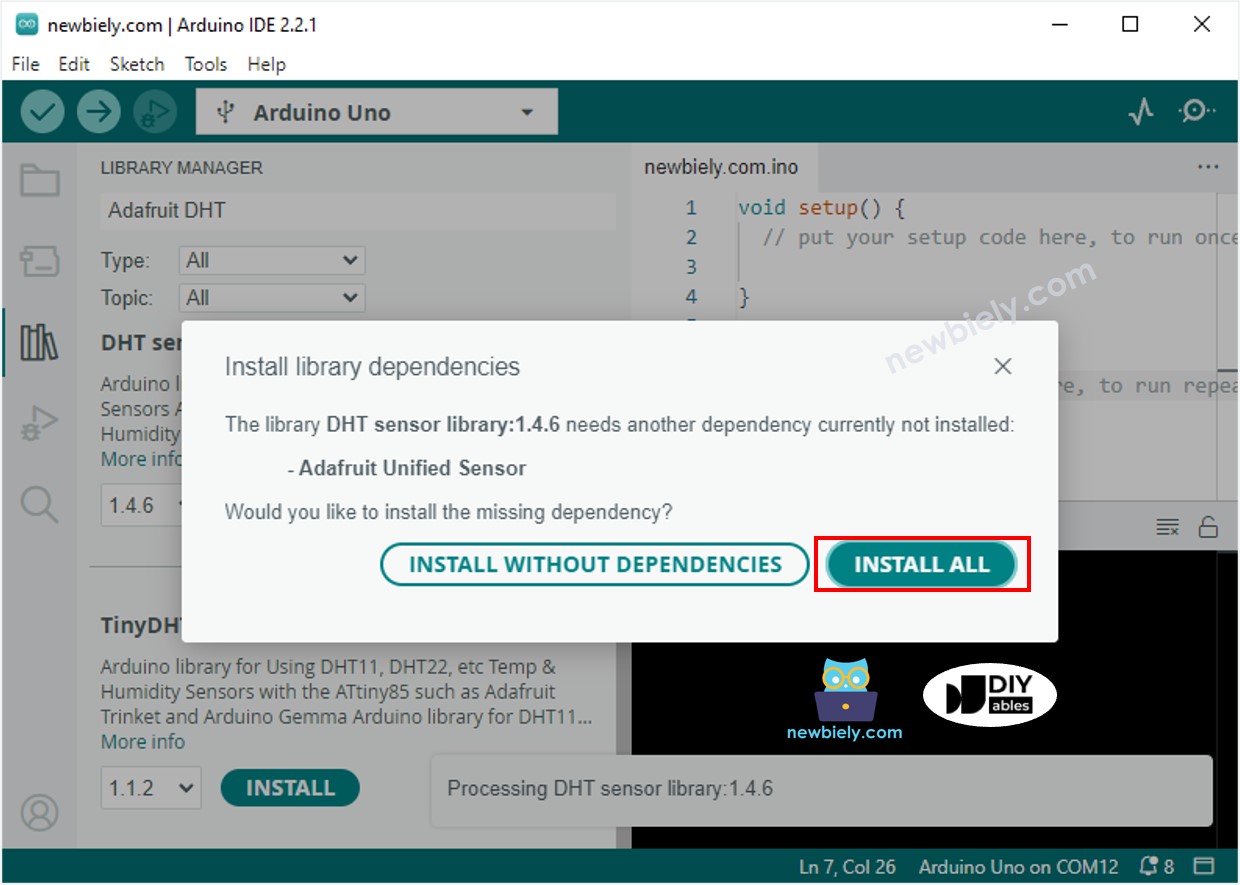
- Copy the above code and open it in Arduino IDE
- Click the Upload button in Arduino Tooltip top guide the code to your Arduino UNO R4
- Place the sensor in hot and cold water, or hold it in your hand
- Check the results on the OLED display and Serial Monitor
※ NOTE THAT:
The code automatically centers the text horizontally and vertically on the OLED display.