Arduino UNO R4 - WebSocket
This guide explains what WebSocket is, why it is helpful for using Arduino UNO R4, and how to use WebSocket with Arduino UNO R4. We will show you how to create a chat application that lets a web browser communicate with Arduino UNO R4, allowing you to:
- Send messages from your web browser's chat window to control the Arduino UNO R4.
- Get instant messages from the Arduino UNO R4. You can change this setup to watch the Arduino UNO R4 live.
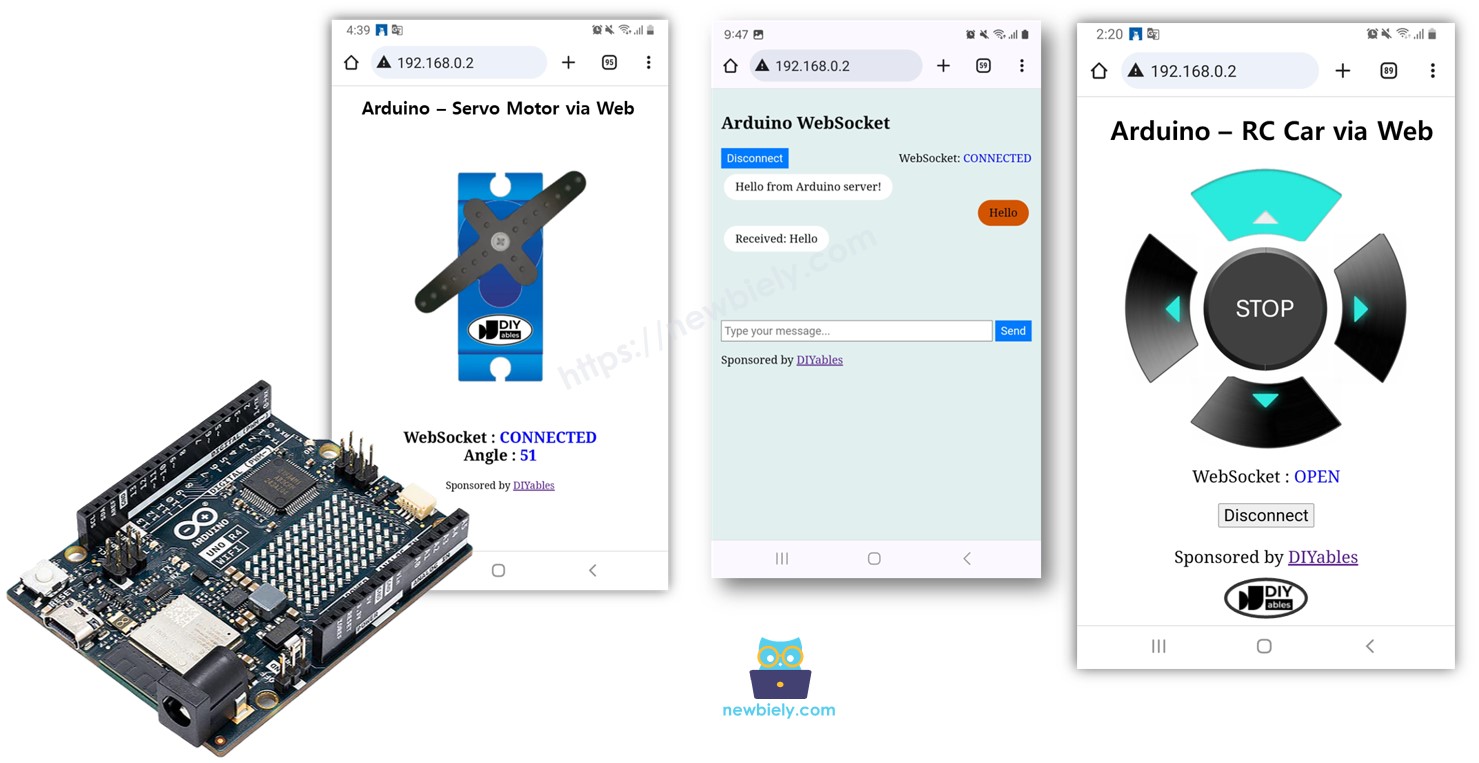
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables.
What is Arduino UNO R4 Websocket?
You may ask, "What is WebSocket?" It's simple: WebSocket is a technology that allows a web browser to talk directly with a web server instantly.
- Without WebSocket, you must reload the webpage to view new updates. This is not very convenient.
- With WebSocket, the webpage stays constantly connected to the server. This means they can share information immediately without reloading the page.
You often use WebSocket technology in daily web applications like online games, instant messaging, and stock market updates.
Why we need WebSocket to smoothly control Arduino UNO R4?
Suppose you want to control a remote-controlled car using your smart phone or computer's web browser. If you don't use WebSocket, you would have to refresh the web page every time you wish to change the car's direction or speed. It is similar to pressing a "reload" button every time you give a command to the car.
WebSocket allows a constant and direct connection between your web browser and the car. You can control the car's direction and speed without needing to refresh the page. It's like the car instantly responds to your commands in real-time, with no delays from reloading the page.
WebSocket makes it easier to:
- Sending data from the web browser to Arduino UNO R4 without having to reload the webpage.
- Sending data back to the web browser from Arduino UNO R4 without needing to refresh the page.
This allows for easy back-and-forth conversation in real time.
Benefits of WebSocket with Arduino UNO R4:
- Real-Time Control: WebSocket allows immediate interaction with the Arduino UNO R4, enabling fast responses to your commands for a smooth experience.
- Persistent Connection: Keep a constant connection without needing to refresh the control page, ensuring a communication line that's always ready for direct instructions.
- Efficiency: Enjoy quick replies and a better experience without having to reload the page repeatedly, making it more efficient and enjoyable.
Web Chat with Arduino UNO R4 via WebSocket
The webpage's materials like HTML, CSS, and JavaScript are kept in a separate file named index.h. So in the Arduino IDE, we will use two code files.
- A .ino file is Arduino UNO R4 code. It sets up a web server and a WebSocket server.
- A .h file contains the content for the webpage.
Detailed Instructions
Follow these instructions step by step:
- If this is your first time using the Arduino Uno R4 WiFi/Minima, refer to the tutorial on setting up the environment for Arduino Uno R4 WiFi/Minima in the Arduino IDE.
- Connect the Arduino Uno R4 board to your computer using a USB cable.
- Launch the Arduino IDE on your computer.
- Select the appropriate Arduino Uno R4 board (e.g., Arduino Uno R4 WiFi) and COM port.
- Open the Library Manager by clicking on the Library Manager icon on the left side of the Arduino IDE.
- Search for “mWebSockets” and locate the mWebSockets by Dawid Kurek.
- Click on the Install button to add the mWebSockets library.
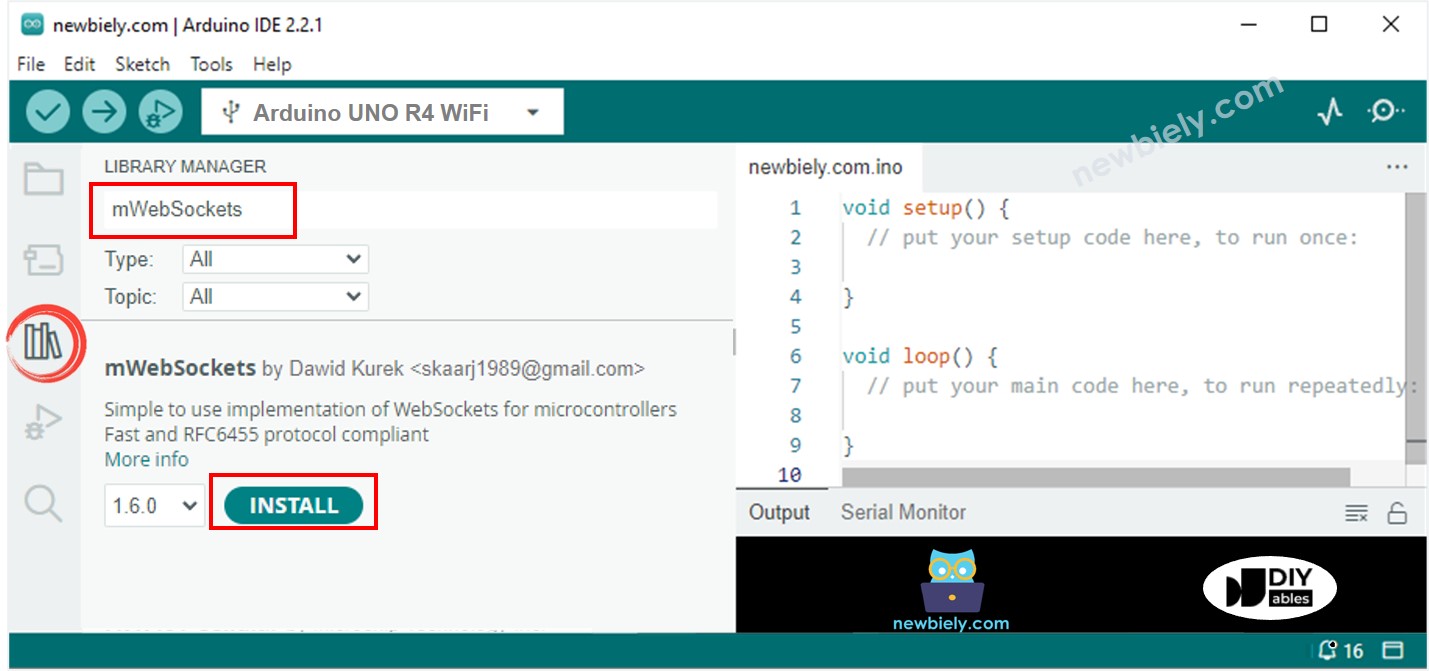
- In the Arduino IDE, create a new sketch and name it, for example, newbiely.com.ino
- Copy the following code and open it in Arduino IDE
- Change the WiFi details (SSID and password) in the code to yours.
- To make the index.h file in Arduino IDE:
- Click the button below the serial monitor icon and select New Tab, or press Ctrl+Shift+N keys.
- Name the file as index.h and press the OK button.
- Copy the following code and paste it into the index.h file.
- You now have the code in two files: newbiely.com.ino and index.h.
- Click the Upload button in Arduino IDE to upload code to your Arduino UNO R4.
- Navigate to the directory at C:\Users\YOUR_ACCOUNT\Documents\Arduino\libraries\mWebSockets\src/.
- Locate the file named config.h and open it using a text editor.
- Go to line 26 and you will see the following content:
- Change the line as shown below and then save it:
- Click the Upload button in Arduino IDE to upload the code to Arduino UNO R4.
- Open the Serial Monitor.
- View the results on the Serial Monitor.
- Write down the IP address shown and type it into the web browser's address bar on your smartphone or computer.
- The webpage will appear as described below:
- Press the CONNECT button to link the webpage with Arduino UNO R4 using WebSocket.
- Enter some text and send it to Arduino UNO R4.
- Observe the reply from Arduino UNO R4.
- If you only change the HTML in the file named index.h and do not modify the file named newbiely.com.ino, the Arduino IDE will not update the HTML when you compile and upload the code to the Arduino UNO R4.
- To update the HTML content through the Arduino IDE, make a small change in the newbiely.com.ino file, like adding an empty line or a comment.
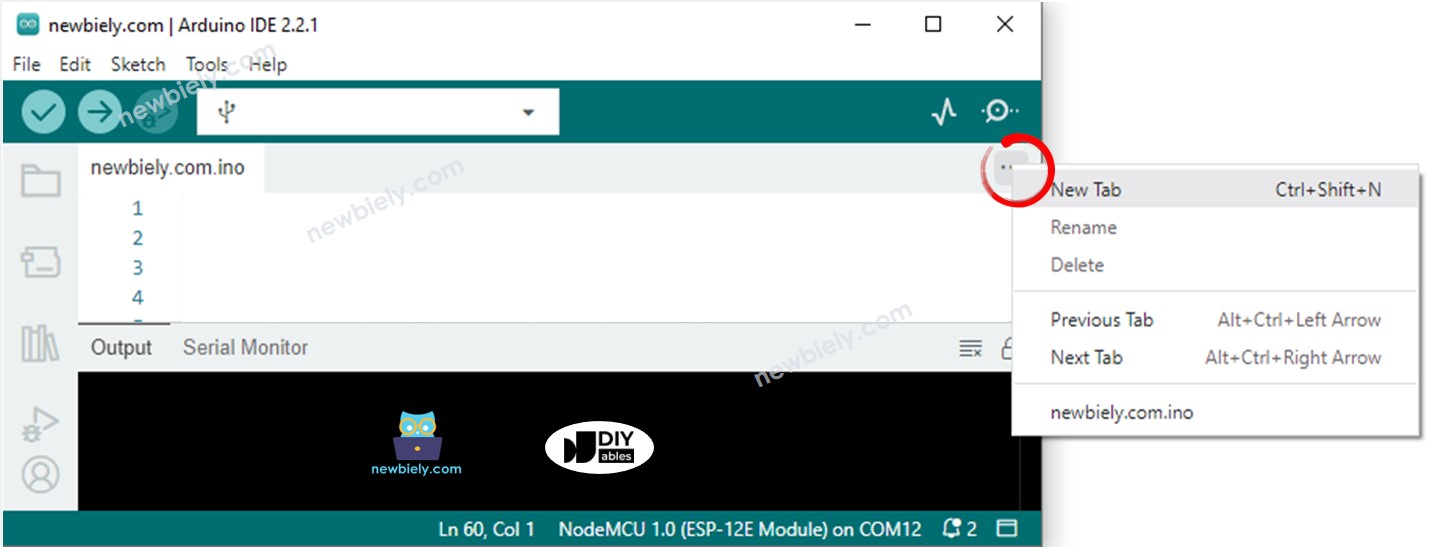
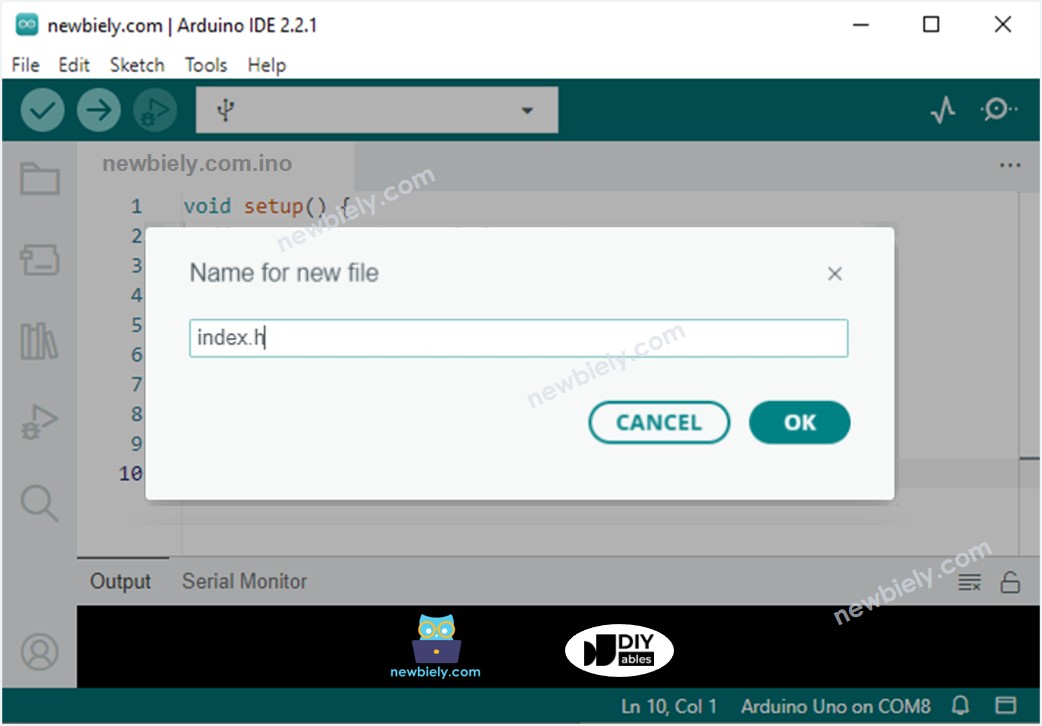
You will see an error message like this:
To solve this problem:
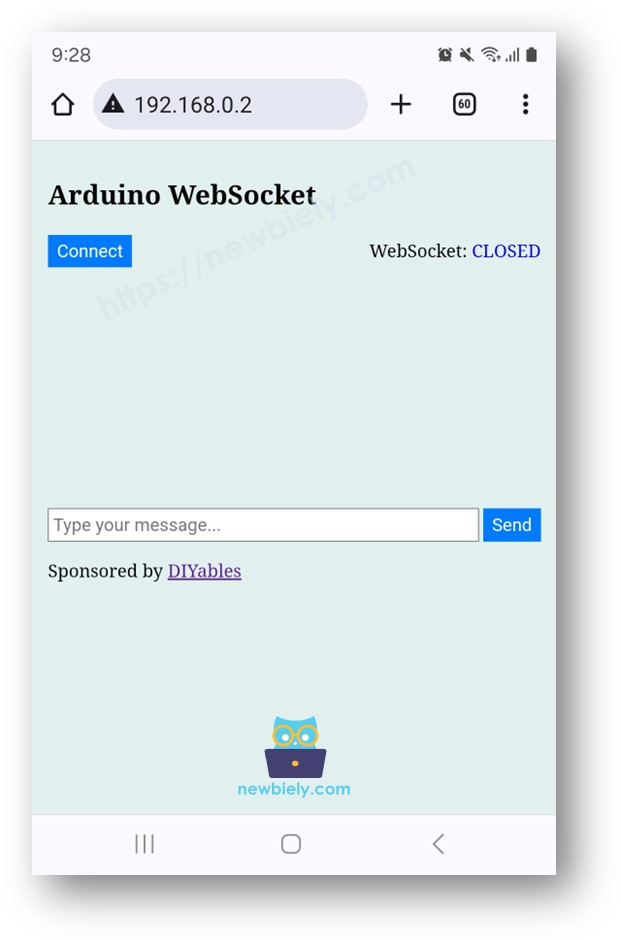
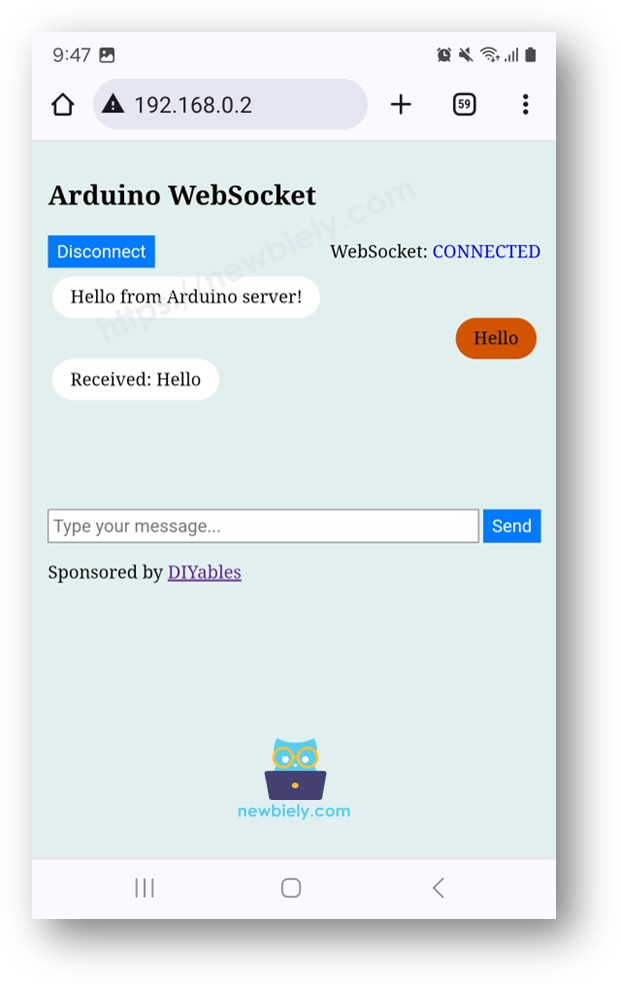
※ NOTE THAT:
Line-by-line Code Explanation
Please read the comments in the code for a line-by-line explanation of the above Arduino UNo R4 code.
How the System Works
The Arduino UNO R4 code sets up a web server and a WebSocket server. Here is how it functions:
- Type the IP address of the Arduino UNO R4 into a web browser.
- The Arduino UNO R4's web server sends the webpage (made of HTML, CSS, JavaScript) to your browser.
- Your browser shows the webpage.
- Click the CONNECT button on the webpage. This action starts a WebSocket connection with the server on the Arduino UNO R4.
- If you type text and click the SEND button, the JavaScript sends your text to the Arduino UNO R4 through the WebSocket.
- The WebSocket server on the Arduino UNO R4 receives your text and sends back a response to your webpage.
Here are other examples of Arduino UNO R4 WebSocket that you can learn:
Troubleshooting For Arduino Uno R4
If the above code does work, please update the latest version for the WiFi module of Arduino UNO R4
- Connect your Arduino Uno R4 WiFi to your PC
- Open Arduino IDE 2
- Go to Tools Firmware Updater
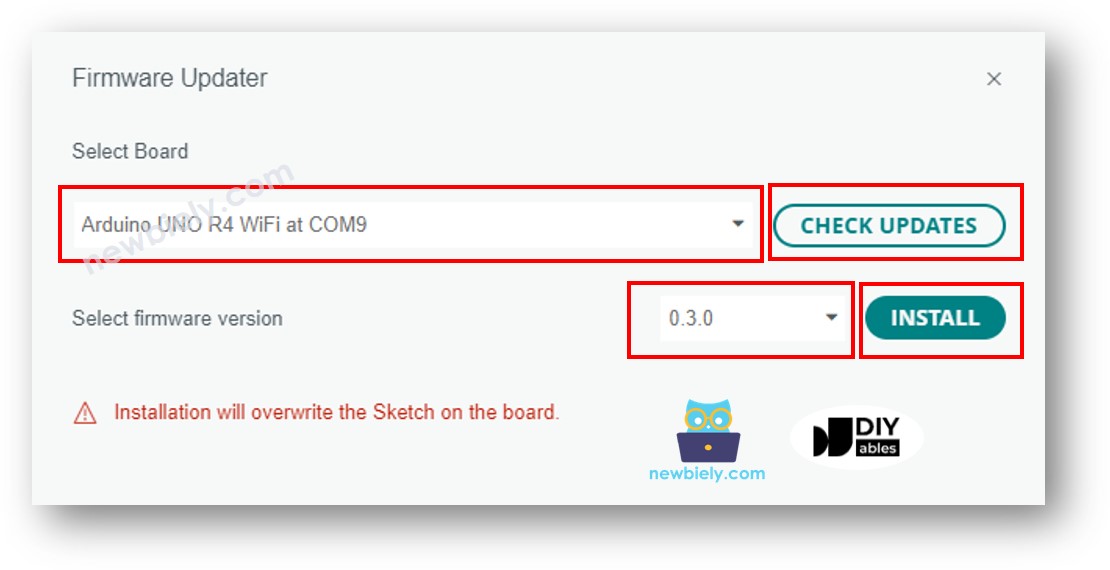
- Select the Arduino Uno R4 WiFi board and port
- Click CHECK UPDATES button
- A list of available firmware versions will appear
- Select the latest version of firmware
- Click INSTALL button
- Wait until it done
- Reboot your Arduino Uno R4 WiFi
- Re-compile and upload your code to Arduino Uno R4 WiFi
- Check the result