Arduino UNO R4 - DC Motor
In this guide, we will learn how to use the Arduino UNO R4 to control the DC motor. In detail, we will learn:
- How a DC motor works
- How to control the speed and direction of a DC motor.
- How to control a DC motor with an L298N driver
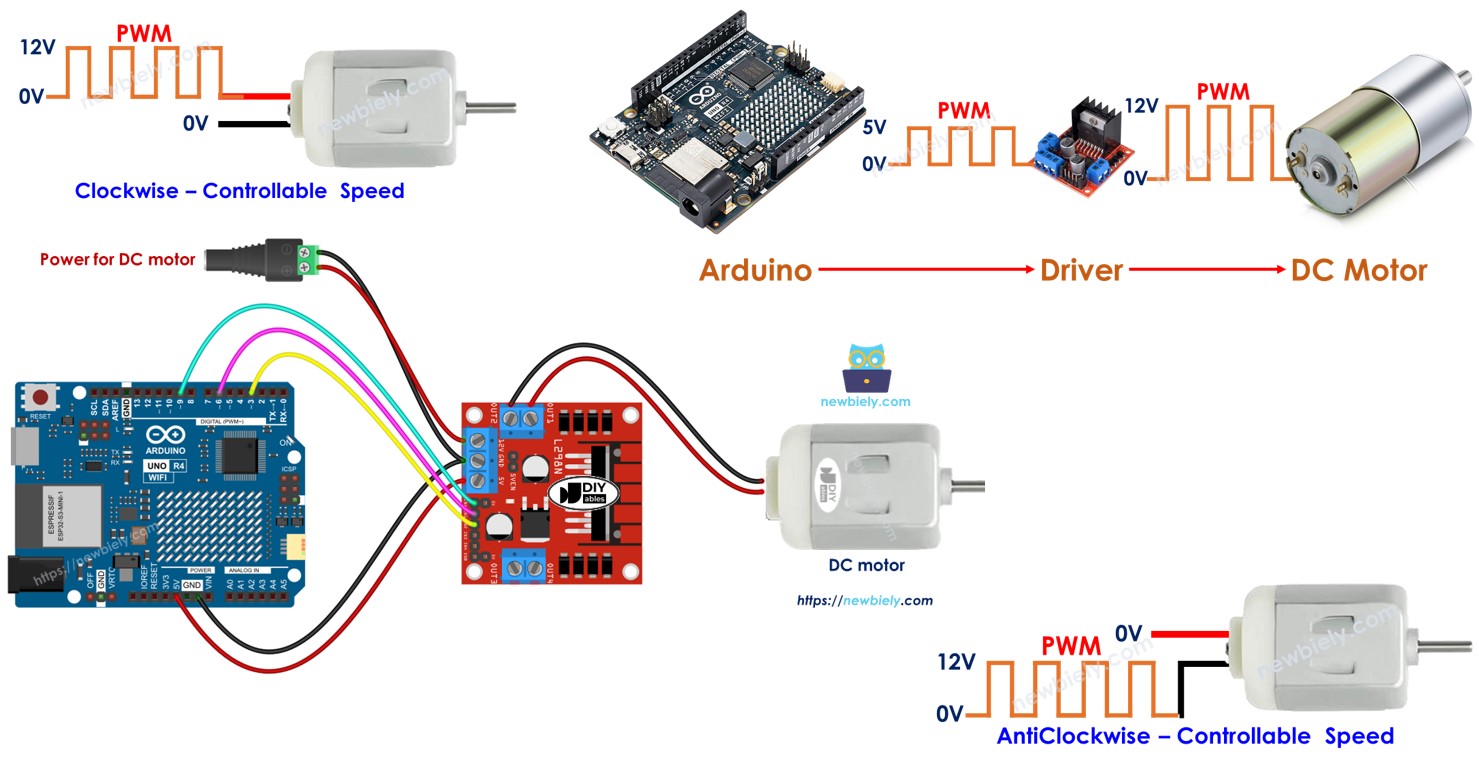
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables.
Overview of DC Motor
DC Motor Pinout
A DC motor has two wires.
- Positive wire: often red
- Negative wire: often black
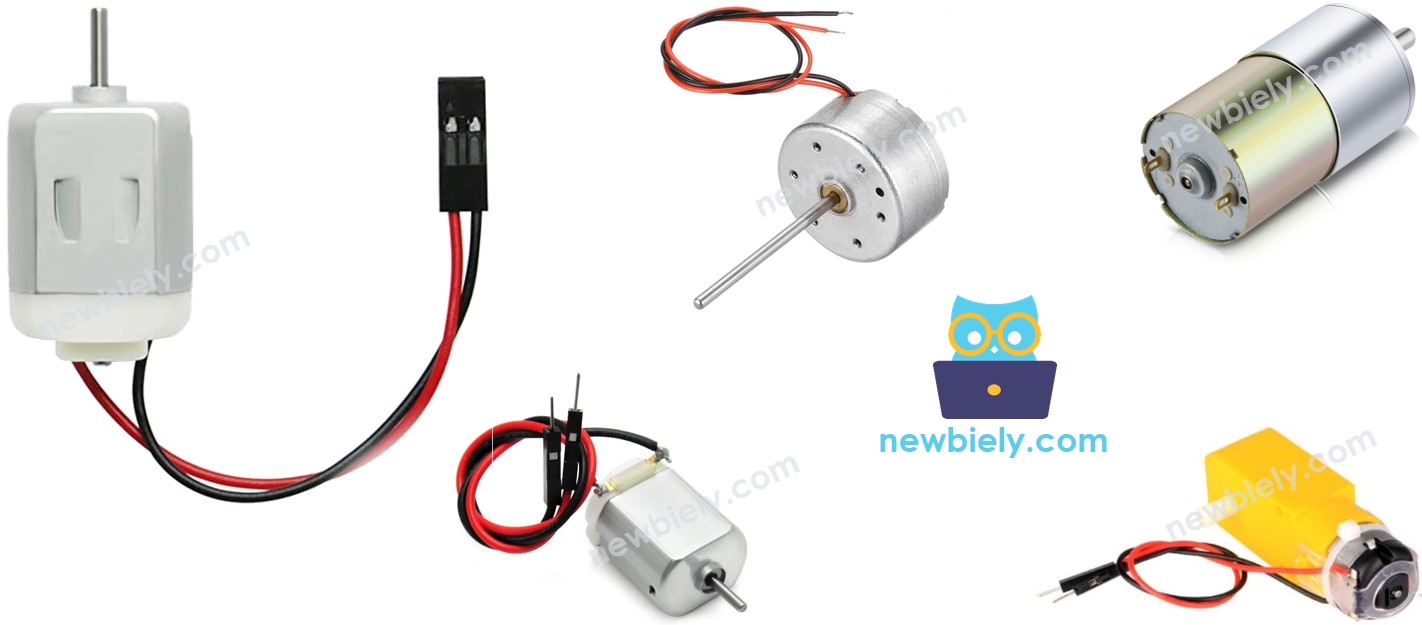
How It Works
When you purchase a DC motor, it's important to understand which voltage it operates on. For instance, consider a DC motor that works on 12 volts. When you connect the 12V DC motor to a 12Volt power source:
- Connect 12V to the positive wire and GND to the negative wire: the DC motor will turn at full speed clockwise.
- Connect 12V to the negative wire and GND to the positive wire: the DC motor will turn at full speed counterclockwise.
When you switch the connections on two wires of the DC motor as mentioned earlier, the direction it spins changes. This technique helps control the direction of DC motor. This isn't done by hand, but rather through programming.
If we give less than 12V to DC motors, the motor turns but not at full speed. This shows that changing the voltage changes the motor's speed. But, adjusting the voltage directly is hard in real situations. So, there is a simpler method to control the speed of DC motor. We keep the power supply voltage constant and use a PWM (Pulse Width Modulation) signal to control the motor speed. With a higher PWM duty cycle, the motor spins faster, and vice versa.
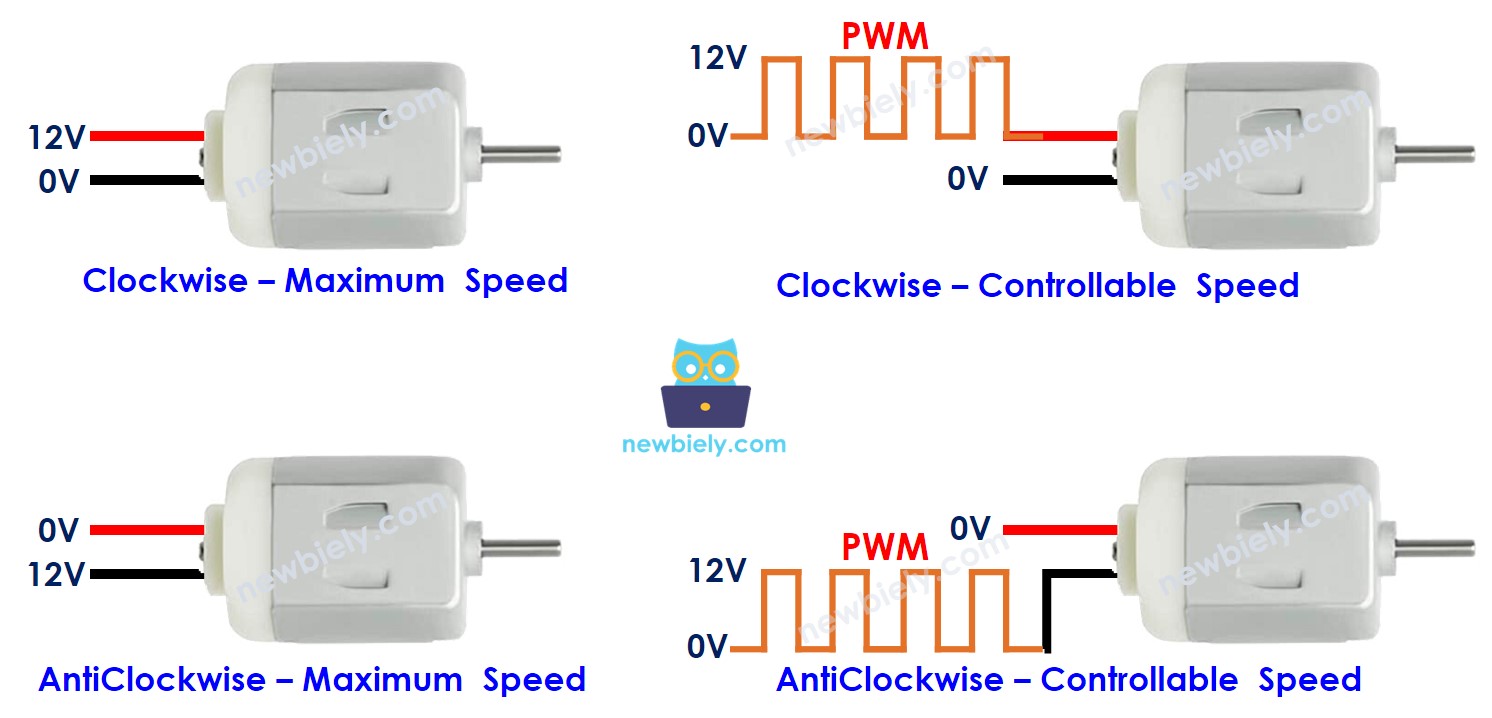
How to control DC motor using Arduino UNO R4
To control a DC motor, you need to control its speed and direction. The Arduino UNO R4 can create a PWM signal, but this signal is too weak in voltage and current to control the motor directly. Therefore, a hardware driver is needed to connect the Arduino UNO R4 to the DC motor. This driver performs two main tasks:
- Increase the PWM signal's strength from the Arduino UNO R4 (both current and voltage) for controlling speed.
- Receive the control signal from the Arduino UNO R4 to change the power supply's polarity for controlling direction.
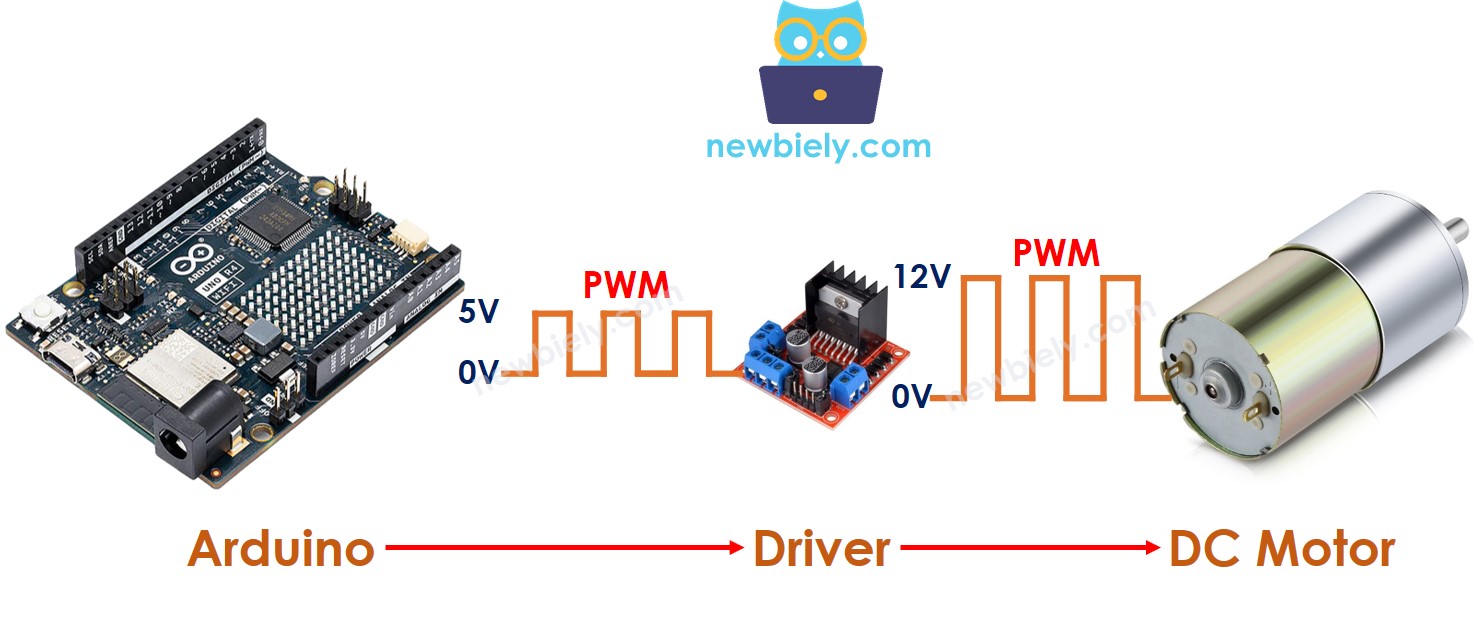
※ NOTE THAT:
- You can use this guide for any DC motor. We are using a 12V DC motor as an example.
- When you use a 5V DC motor, even though the Arduino UNO R4 pin outputs 5V (the same voltage as the DC motor), you still need a driver between the Arduino UNO R4 and the DC motor. This is because the Arduino UNO R4 pin cannot supply enough current for the DC motor.
This tutorial will use the L298N driver to control DC motors.
Overview of L298N Driver
In this guide, we will learn how to use the L298N Driver to control a DC motor.
L298N Driver Pinout
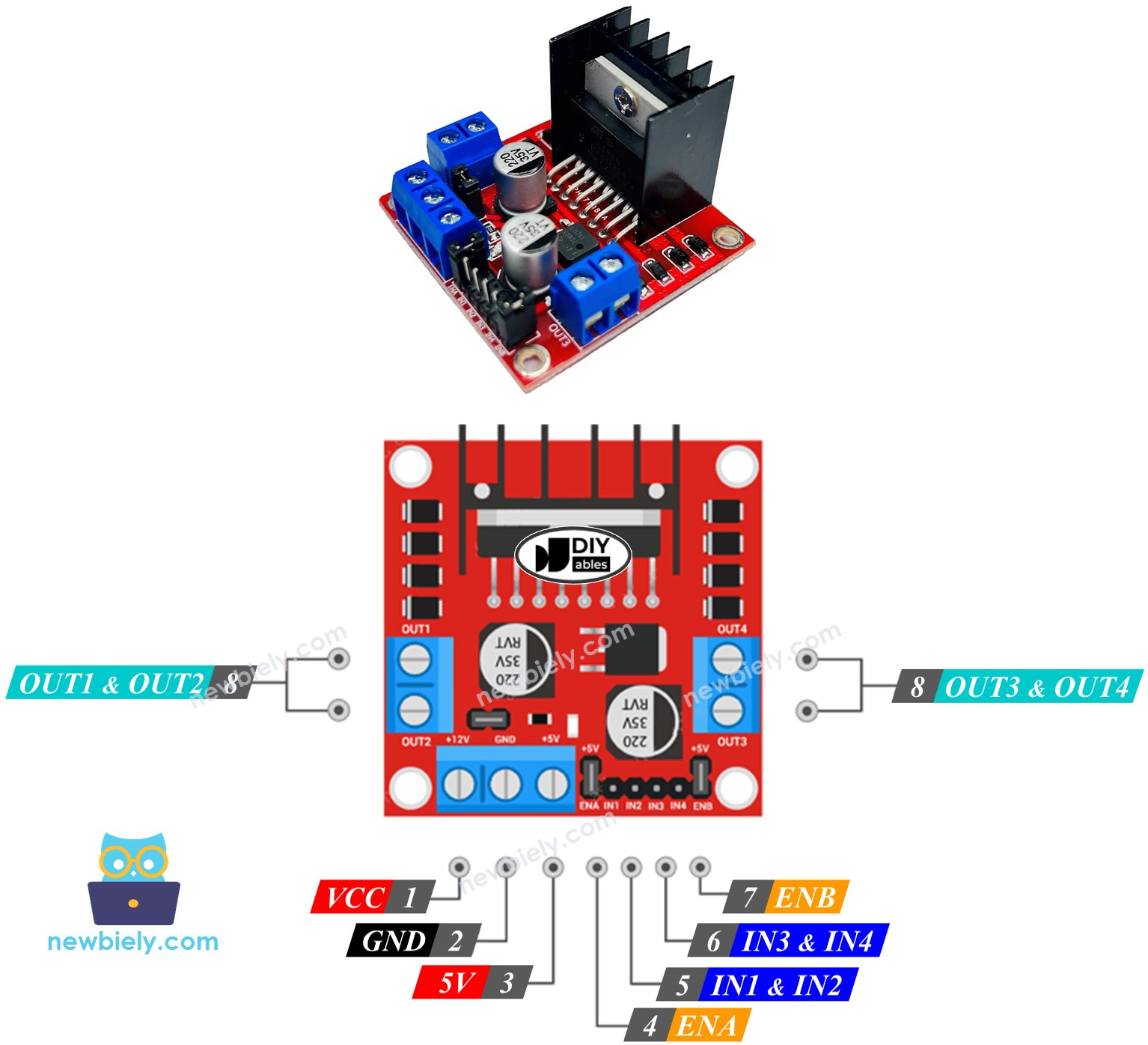
The L298N Driver can independently control two DC motors at the same time, named motor A and motor B. It has 13 pins.
The common pins for both motors:
- VCC pin: provides power to the motor. The voltage can range from 5 to 35V.
- GND pin: this is a common ground pin and should be connected to GND (0V).
- 5V pin: powers the L298N module. It can use 5V provided by an Arduino UNO R4.
Motor A pins (Channel A):
- ENA pins: These control the speed of Motor A. To adjust the speed, remove the jumper and connect the pin to a PWM input.
- IN1 & IN2 pins: These determine the direction Motor A spins. If one pin is HIGH and the other is LOW, Motor A will spin. If both pins are either HIGH or LOW, Motor A will stop.
- OUT1 & OUT2 pins: These are connected to Motor A.
Motor B pins (Channel B):
- ENB pins: These control Motor B's speed. If you remove the jumper and connect these pins to a PWM input, you can adjust how fast Motor B spins.
- IN3 & IN4 pins: These control the direction Motor B spins. If IN3 is HIGH and IN4 is LOW, or vice versa, Motor B will spin. If both are HIGH or both are LOW, Motor B will stop.
- OUT3 & OUT4 pins: These are connected to Motor B.
The L298N driver mentioned earlier has two types of input power:
- One for the DC motor (VCC and GND pins): from 5 to 35V.
- One for running the L298N module itself (5V and GND pins): from 5 to 7V.
The L298N driver has three jumpers for more complex uses. For simplicity, please take all jumpers off the L298N driver.
We can control two DC motors at the same time using an Arduino UNO R4 and an L298N Driver. To control each motor, we use three pins from the Arduino UNO R4.
※ NOTE THAT:
This tutorial will show you how to control a DC motor using channel A. Controlling another DC motor is done in a similar way.
Wiring Diagram
Take off all three jumpers from the L298N module before you start the wiring.
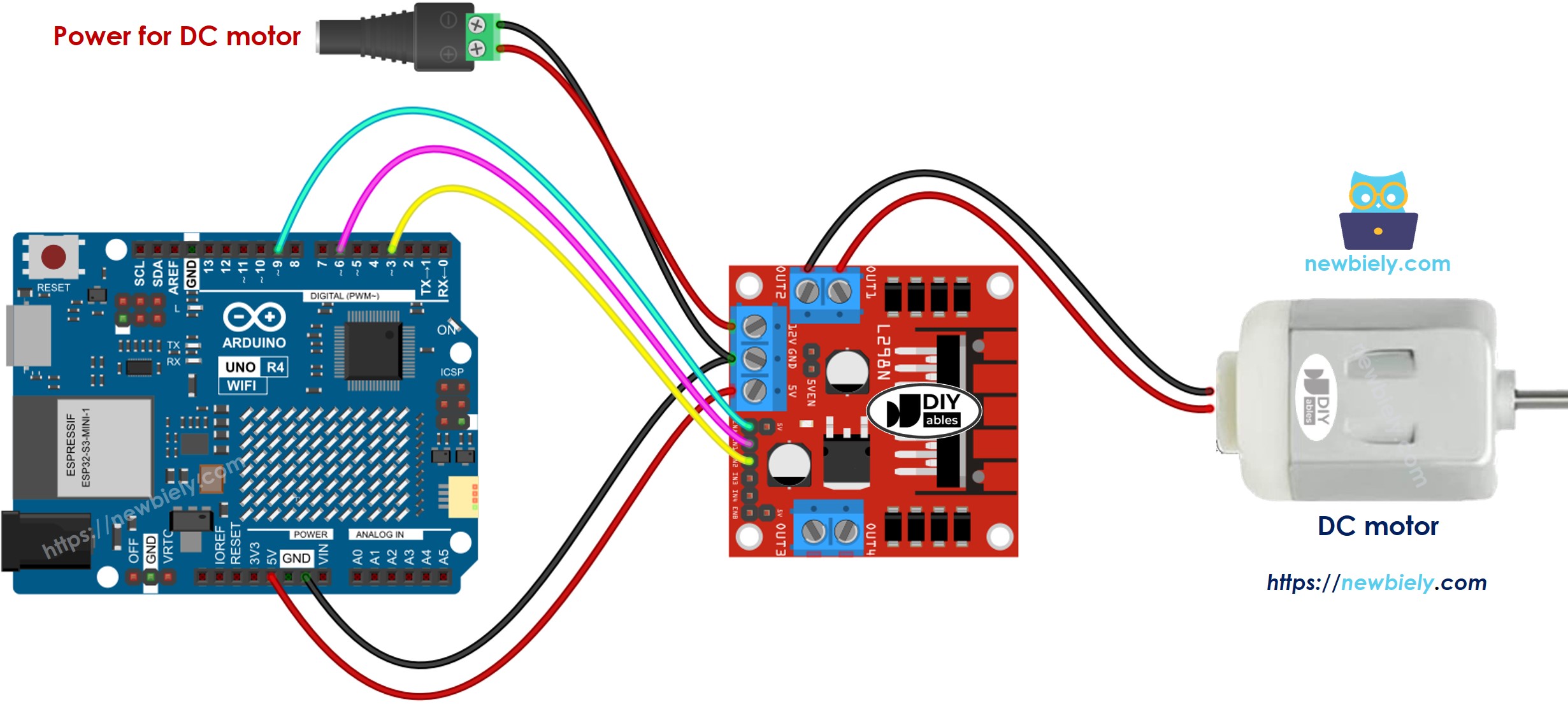
This image is created using Fritzing. Click to enlarge image
How To Control the Speed of DC Motor via L298N Driver
You can easily adjust the speed of the DC motor by sending a PWM signal to the ENA pin on the L298N. Here is how to do it:
- Connect an Arduino UNO R4 pin to the ENA pin on the L298N module.
- Use the analogWrite() function to send a PWM signal to the ENA pin. The L298N driver will increase the strength of this PWM signal to power the DC motor.
The speed can be any number from 0 to 255. At a speed of 0, the motor will stop. At a speed of 255, the motor will rotate at its highest speed.
How To Control the Direction of DC Motor via L298N Driver
The direction a motor rotates can be changed by setting IN1 and IN2 pins to HIGH or LOW. The table below shows how to control the direction for both channels.
IN1 pin | IN2 pin | Direction |
---|---|---|
LOW | LOW | Motor A stops |
HIGH | HIGH | Motor A stops |
HIGH | LOW | Motor A spins Clockwise |
LOW | HIGH | Motor A spins Anti-Clockwise |
- Motor A rotates in a clockwise direction.
- Motor A turns counter-clockwise
※ NOTE THAT:
If you connect the OUT1 and OUT2 pins to the DC motor pins in the opposite way, the motor will spin in the opposite direction. To fix this, simply switch the positions of the OUT1 and OUT2 pins, or change the control signals on the IN1 and IN2 pins in the program code.
How To Stop DC Motor Spinning
There are two methods to turn off a DC motor.
- Sets the speed to zero.
- Sets IN1 and IN2 pins to the same value (either LOW or HIGH).
- Or
How to control a DC motor using L298N driver.
Arduino UNO R4 Code
The code below does the following:
- Make the DC motor go faster
- Reverse the direction
- Make the DC motor go slower
- Stop the motor
Detailed Instructions
Follow these instructions step by step:
- If this is your first time using the Arduino Uno R4 WiFi/Minima, refer to the tutorial on setting up the environment for Arduino Uno R4 WiFi/Minima in the Arduino IDE.
- Wire the components according to the provided diagram.
- Connect the Arduino Uno R4 board to your computer using a USB cable.
- Launch the Arduino IDE on your computer.
- Select the appropriate Arduino Uno R4 board (e.g., Arduino Uno R4 WiFi) and COM port.
- Remove all three jumpers from the L298N module.
- Paste the code into the Arduino IDE.
- Press the Upload button in the Arduino IDE to upload the code to the Arduino UNO R4.
- Observations:
- The DC motor accelerates, then spins at maximum speed for 1 second.
- The direction of the DC motor changes.
- The DC motor spins at maximum speed for 1 second in the opposite direction.
- The DC motor decelerates.
- The DC motor stops for 1 second.
- This sequence repeats continuously.
※ NOTE THAT:
In this tutorial, we will learn how to adjust the relative speed of a DC motor in relation to its highest speed. To control the absolute speed in rotations per second, we must use a PID controller and an encoder. We will cover how to control the precise speed of the DC motor in a different tutorial.