Arduino UNO R4 - RGB LED
This tutorial instructs you how to use Arduino to control the RGB LED. In detail, we will learn:
- How RGB LED works
- How to connect RGB LED to Arduino UNO R4
- How to connect RGB LED module to Arduino UNO R4
- How to program Arduino UNO R4 to control the color of RGB LED
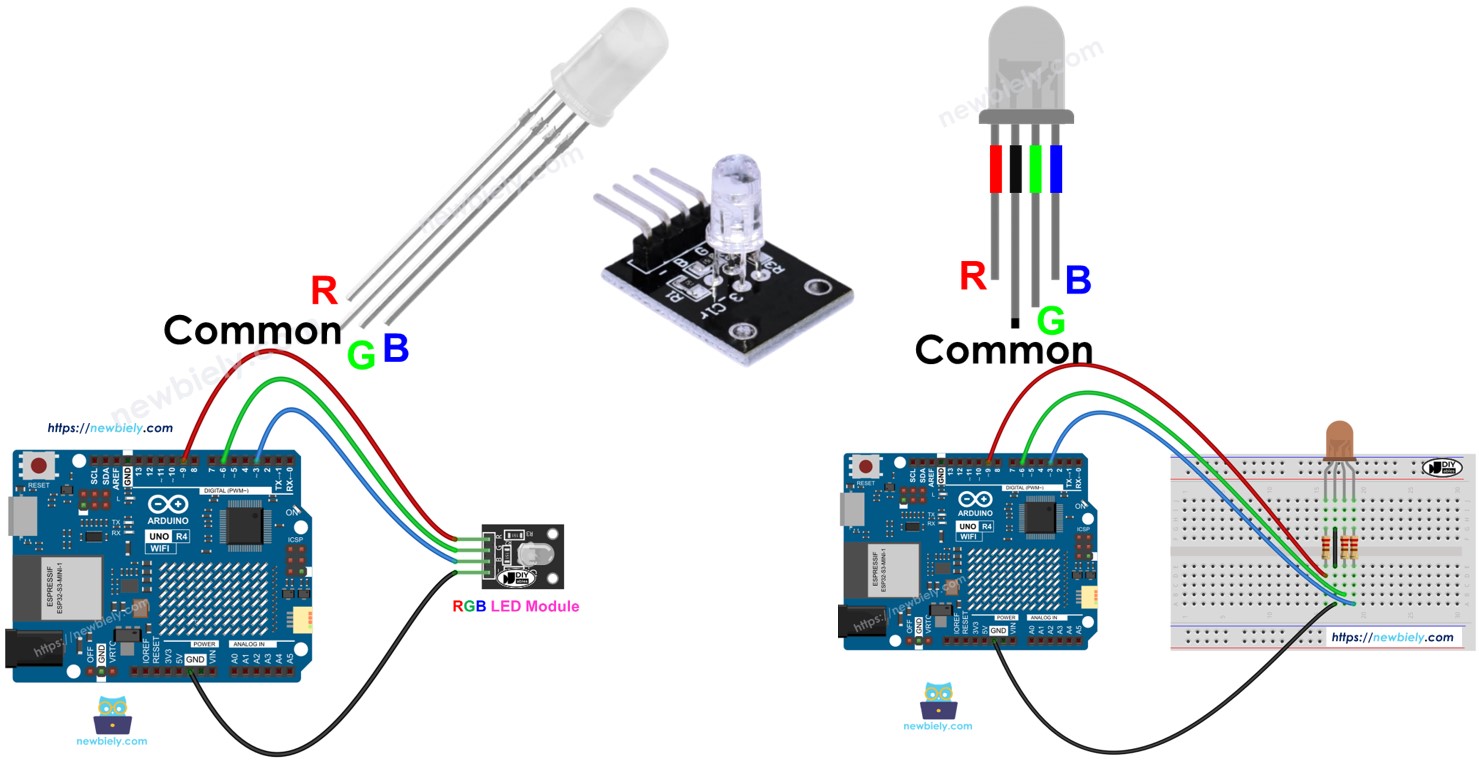
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of RGB LED
The RGB LED can create any color by mixing the three primary colors: red, green, and blue. It contains three separate LEDs: one red, one green, and one blue. All are housed together in one unit.
Pinout
An RGB LED has four pins.
- Connect the Common (Cathode-) pin to GND (0V).
- The R (red) pin controls the red color.
- The G (green) pin controls the green color.
- The B (blue) pin controls the blue color.
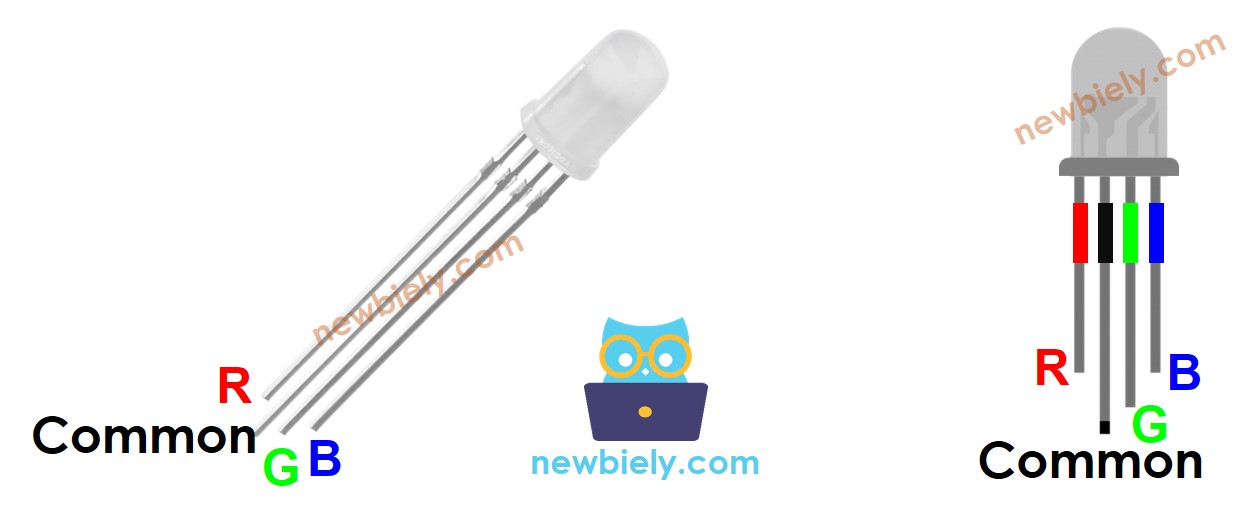
To connect an RGB LED to an Arduino UNO R4, we should use resistors that limit the current, which makes the setup a bit complicated. However, we can use the RGB LED module which has these resistors built-in already.
The RGB LED module has four pins too.
- Common (Cathode-) pin should be connected to GND (0V).
- R (red) pin controls the red color.
- G (green) pin controls the green color.
- B (blue) pin controls the blue color.
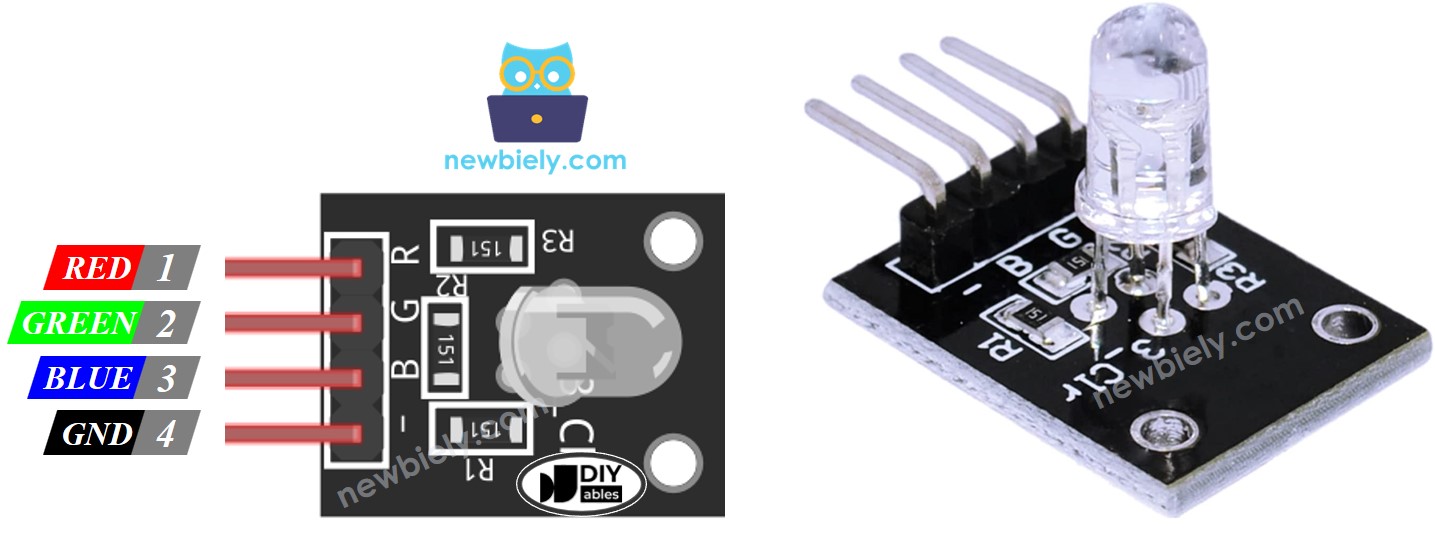
※ NOTE THAT:
This tutorial uses an RGB LED with a common cathode. This means the common pin is the cathode. Different RGB LEDs might have the common pin as the anode.
How it works
In physics, a color is made up of three values: Red (R), Green (G), and Blue (B). Each value can be from 0 to 255.
⇒ There are a total of 256 x 256 x 256 colors created by combining three different values.
⇒ If we send PWM signals (with a duty cycle between 0 and 255) to the R, G, and B pins, we can make the RGB LED display any color we choose. The duty cycle of PWM signals to the R, G, and B pins matches the color values of Red (R), Green (G), and Blue (B).
Wiring Diagram
- Wiring diagram between Arduino UNO R4 to an RGB LED.
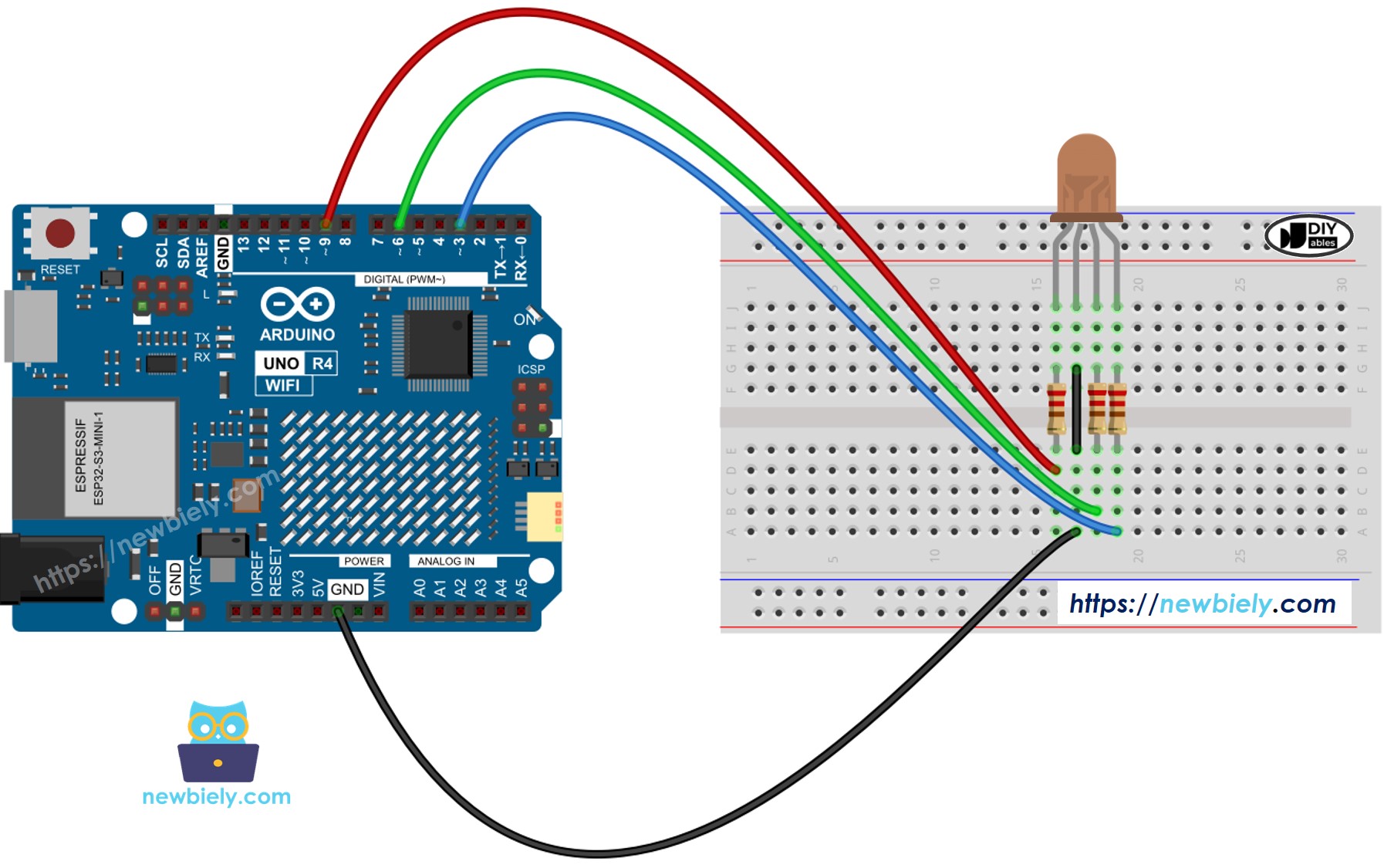
This image is created using Fritzing. Click to enlarge image
Do not use one resistor on the common pin of an RGB LED. Instead, use three separate resistors on the other pins as above diagram. Different LEDs in the RGB package do not share the exact same features, which means they do not equally share current. This can cause uneven brightness and potentially damage the LEDs if using a single resistor on the common pin.
- Wiring diagram between Arduino UNO R4 to an RGB LED module
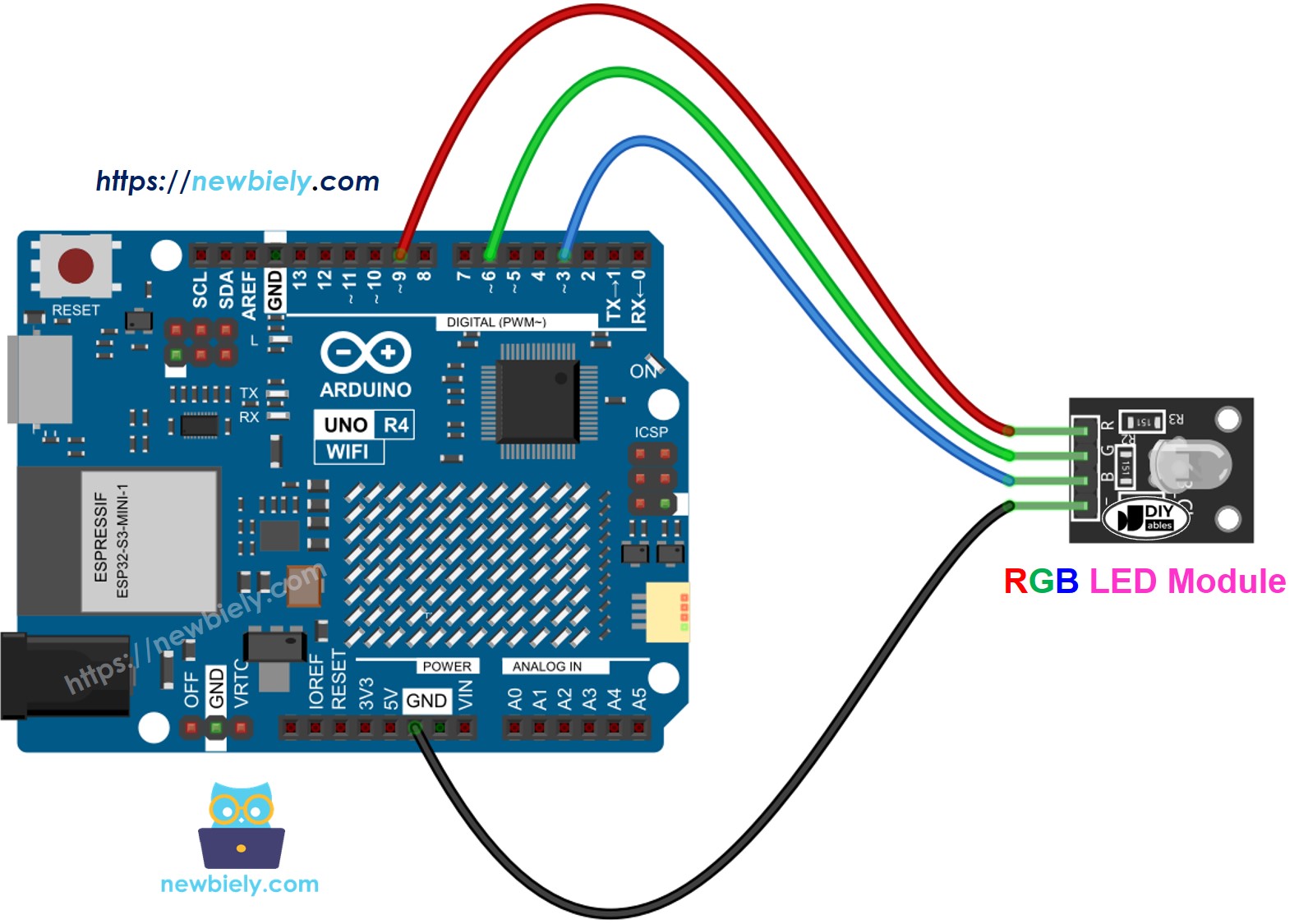
This image is created using Fritzing. Click to enlarge image
See The best way to supply power to the Arduino Uno R4 and other components.
How To Control RGB LED
Let's learn step-by-step how to change the RGB LED to any color, for example, the color #00979D.
- Choose the color you want to use and find its color code.
- You can select a color code from this color picker.
- If you need a color from a photo, use this Colors From Image tool.
- Convert the color code to RGB values using this tool. Remember these values: R = 0, G = 151, B = 157.
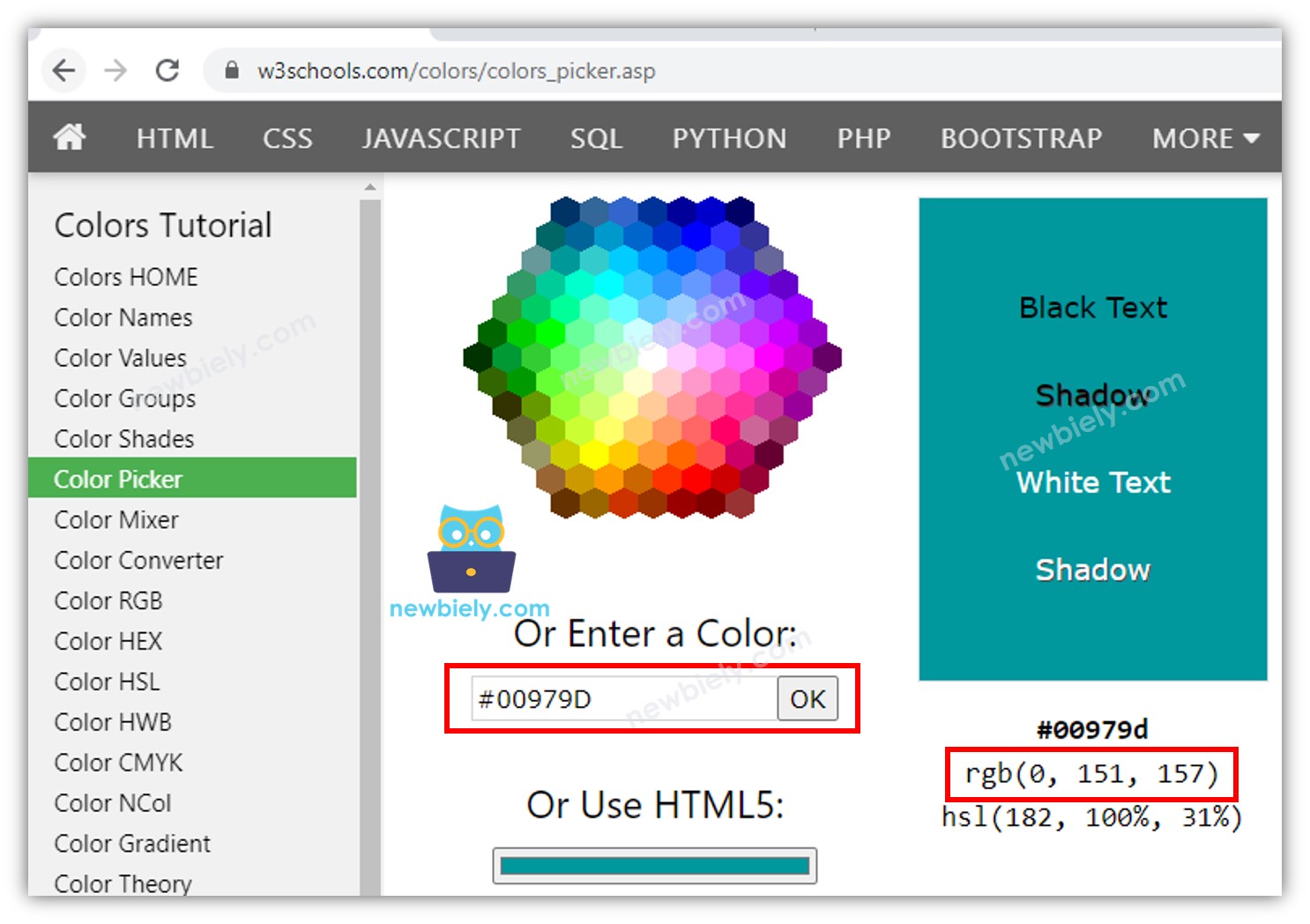
- Define the Arduino UNO R4 pins that connect to the R, G, and B pins. For instance:
- Configure these Arduino UNO R4 pins as outputs.
- Program Arduino pin to generate PWM signal to show the color (#00979D; R = 0, G = 151, B = 157).
Arduino UNO R4 - RGB LED Example Code
The code below changes the LED color in this order:
- #00C9CC (R = 0, G = 201, B = 204)
- #F7788A (R = 247, G = 120, B = 138)
- #34A853 (R = 52, G = 168, B = 83)
When using many colors, we could shorten the code by creating a function:
Addtional Knowledge
- To set up an RGB LED with a common Anode:
- Connect the common pin to the 3.3V pin on the Arduino UNO R4.
- In the analogWrite() function, adjust the R, G, and B values to 255 - R, 255 - G, and 255 - B, respectively.
- A series of RGB LEDs connected together forms an RGB LED Strip. There are two types of LED Strips: addressable and non-addressable. We will provide tutorials for each type.