Arduino UNO R4 - Micro SD Card
In this guide, we will learn how to use a Micro SD Card with the Arduino UNO R4. We will cover the following details:
- Arduino UNO R4 - Opening or Creating a File on Micro SD Card
- Arduino UNO R4 - Writing Data to a File on Micro SD Card
- Arduino UNO R4 - Reading from a File on Micro SD Card Character by Character
- Arduino UNO R4 - Reading from a File on Micro SD Card Line by Line
- Arduino UNO R4 - Adding Content to an Existing File on Micro SD Card
- Arduino UNO R4 - Overwriting a File on Micro SD Card
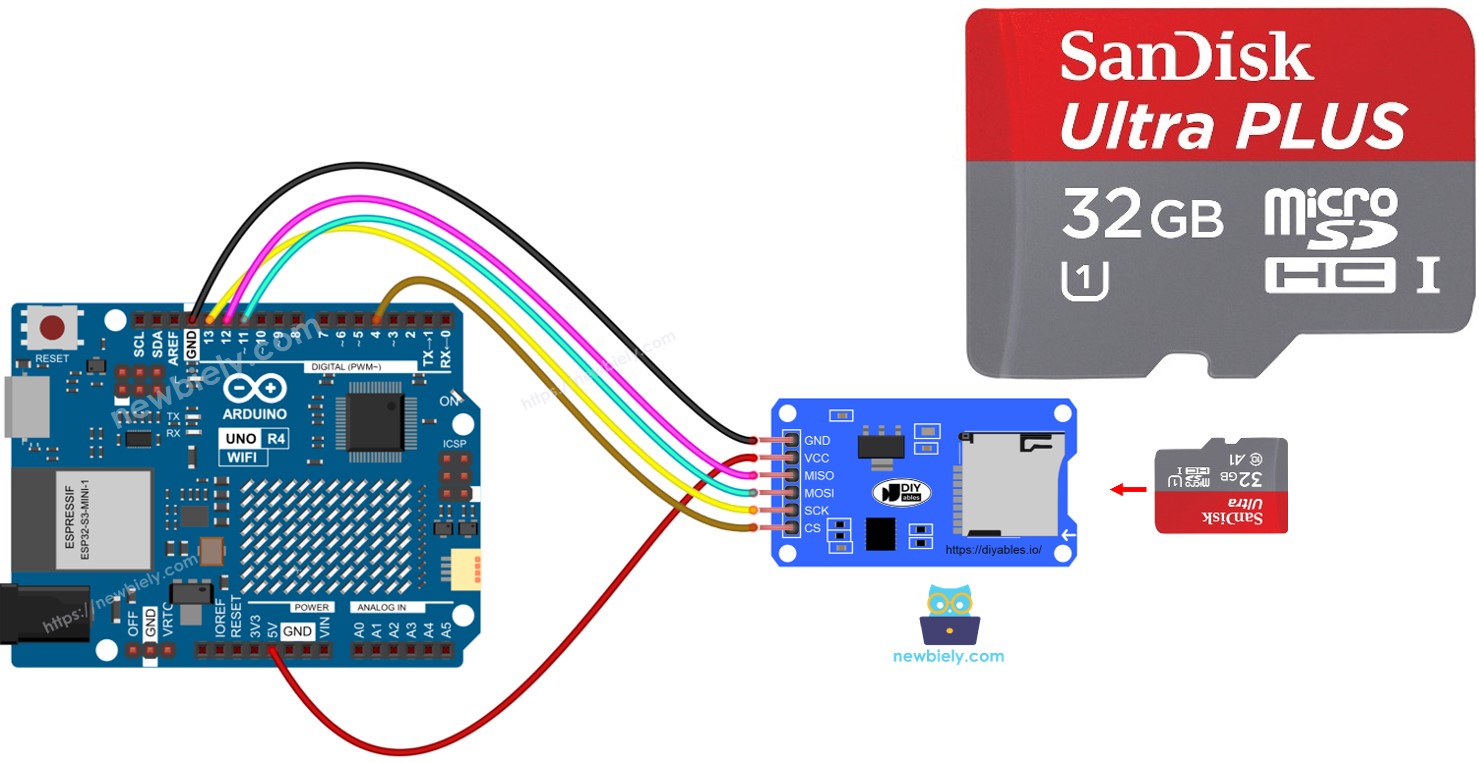
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Micro SD Card Module
The Micro SD Card Module connects with the Arduino UNO R4 and holds a Micro SD Card. This means the Micro SD Card Module acts as a link between the Arduino UNO R4 and the Micro SD Card.
Pinout
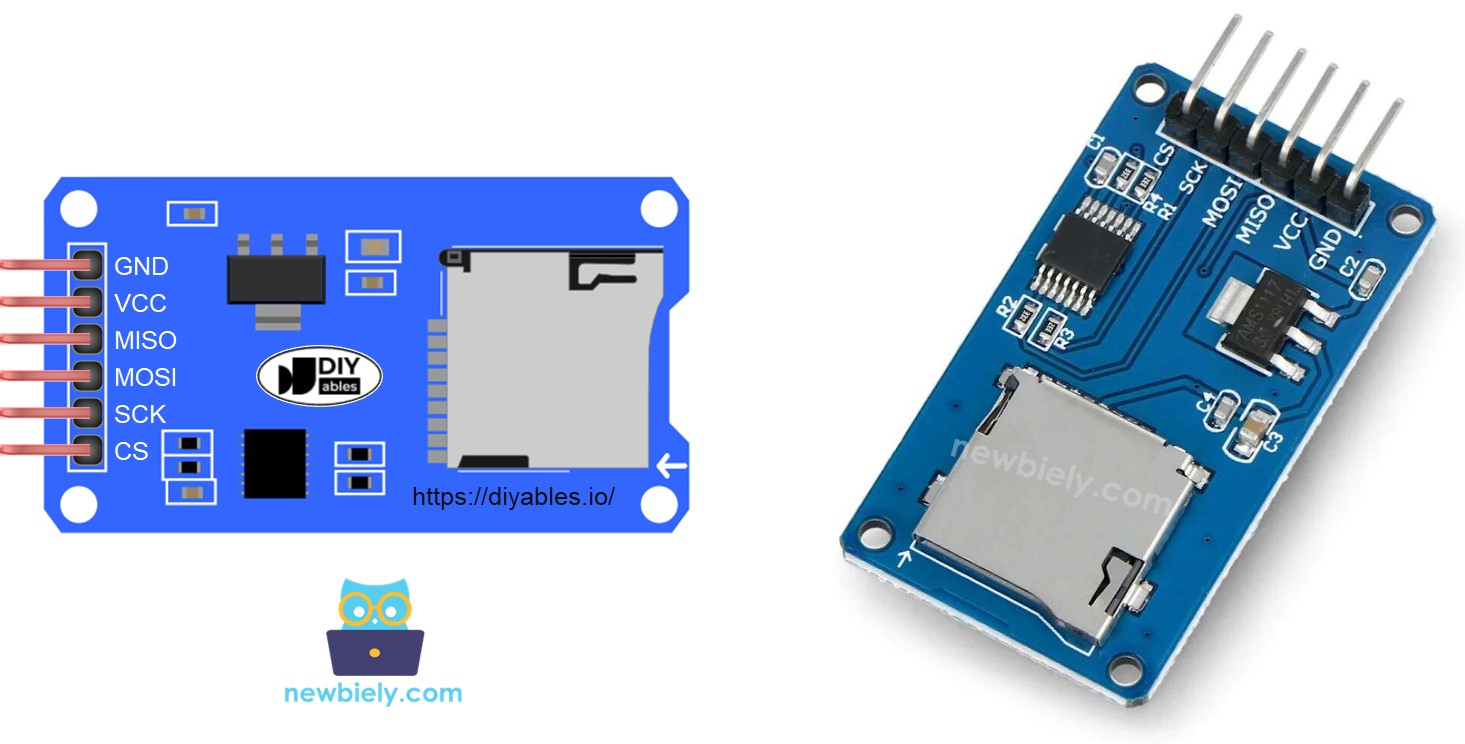
The Micro SD Card Module has 6 pins:
- Connect the VCC pin to the 5V pin on the Arduino UN to R4.
- Connect the GND pin to the GND on the Arduino UNO R4.
- Connect the MISO pin to the MISO pin on the Arduino UNO R4.
- Connect the MOSI pin to the MOSI pin on the Arduino UNO R4.
- Connect the SCK pin to the SCK pin on the Arduino UNO R4.
- Connect the SS pin to the pin identified as the SS pin in the Arduino UNO R4 code.
Preparation
- Plug the Micro SD Card into your computer using a USB 3.0 SD Card Reader.
- Check that the Micro SD Card is formatted as FAT16 or FAT32. You can search online to find out how to do this.
Wiring Diagram
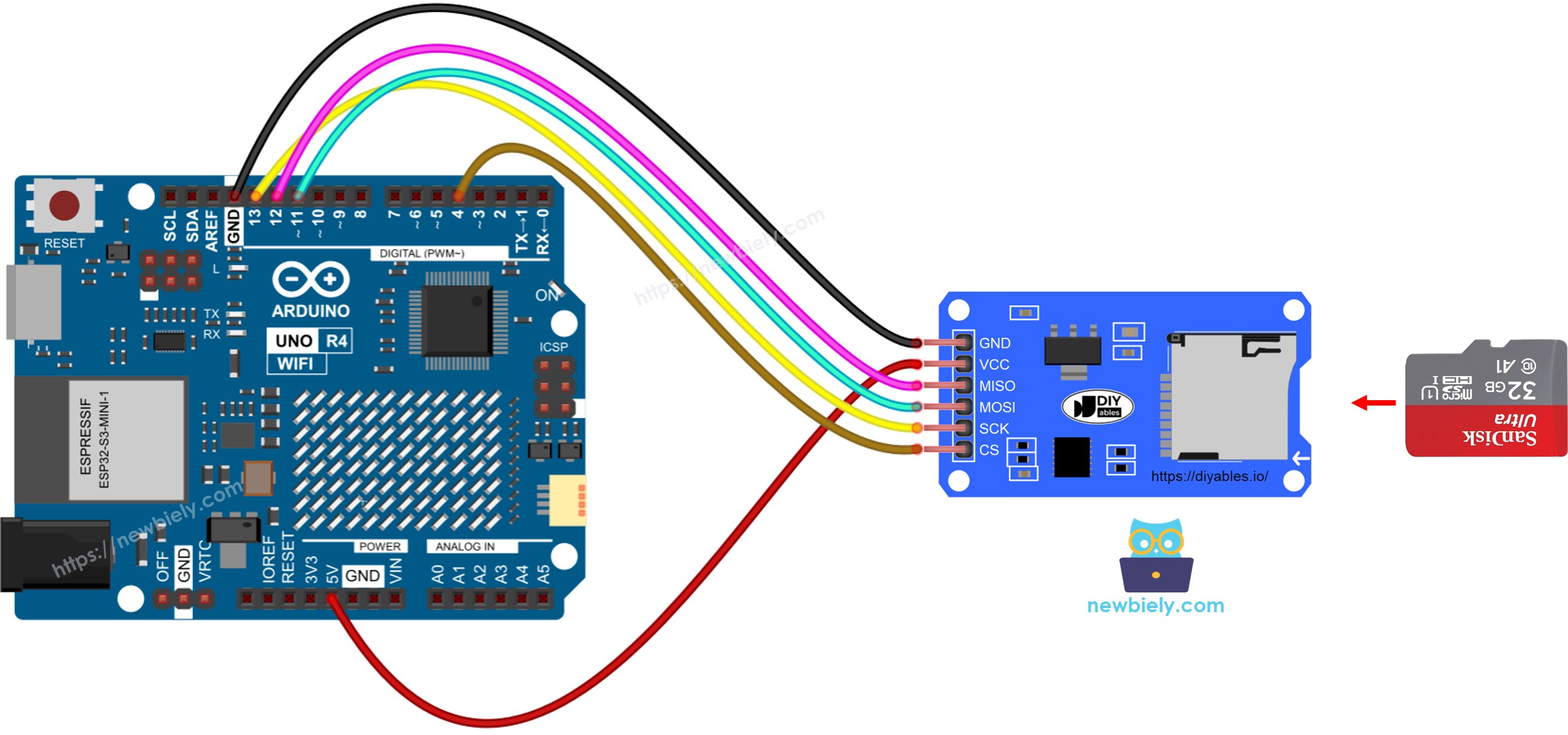
This image is created using Fritzing. Click to enlarge image
See The best way to supply power to the Arduino Uno R4 and other components.
※ NOTE THAT:
If you have an Ethernet shield or any shield with a Micro SD Card Holder, you don't need to use the Micro SD Card Module. Just put the Micro SD Card into the Micro SD Card Holder on the shield.
Arduino UNO R4 - How to open a file on Micro SD Card and create if not existed
Arduino UNO R4 Code
Detailed Instructions
Follow these instructions step by step:
- If this is your first time using the Arduino Uno R4 WiFi/Minima, refer to the tutorial on setting up the environment for Arduino Uno R4 WiFi/Minima in the Arduino IDE.
- Insert the Micro SD Card into the Micro SD Card module
- Follow the wiring diagram to connect the Micro SD Card module to the Arduino UNO R4
- Connect the Arduino Uno R4 board to your computer using a USB cable.
- Launch the Arduino IDE on your computer.
- Select the appropriate Arduino Uno R4 board (e.g., Arduino Uno R4 WiFi) and COM port.
- Open the Serial Monitor in the Arduino IDE
- Copy the provided code and paste it into the Arduino IDE
- Press the Upload button in the Arduino IDE to transfer the code to the Arduino UNO R4.
- Check the Serial Monitor for the outcome after the first upload.
- The outcome displayed on the Serial Monitor for subsequent attempts
※ NOTE THAT:
You might not see any output on the Serial Monitor if you open it after uploading for the first time.
- Remove the Micro SD Card from the module
- Place the Micro SD Card into a USB SD Card reader
- Attach the USB SD Card reader to your computer
- Verify whether the file is there or not
Arduino UNO R4 - How to write/read data to/from a file on Micro SD Card
This code performs the following actions:
- Save information in a file
- Load and display each character of a file one by one on the Serial Monitor
- The Serial Monitor displayed the file's contents.
※ NOTE THAT:
By default, the data is added to the end of the file. If you restart the Arduino UNO R4 with the given code, the text will be added again to the file. This means you will see additional lines like these on the Serial Monitor:
You can also remove the Micro SD Card from the module and use a USB SD Card reader to view the files on your computer.
Arduino UNO R4 - How to read a file on Micro SD Card line-by-line
- The outcome displayed on the Serial Monitor
※ NOTE THAT:
If you don’t delete the file content before, you might see additional lines on the Serial Monitor.
Arduino UNO R4 - How to overwrite a file on Micro SD Card
Normally, the content adds to the end of the file. To replace a file, first delete the old file and then make a new one with the same name.
- The output on the Serial Monitor
- Restart Arduino UNO R4
- Verify if the file's content in Serial Monitor has been added to.
You can also remove the Micro SD Card from the unit and use a USB SD Card reader to view its contents on your computer.