Arduino UNO R4 - Button - Debounce
When programming the Arduino Uno R4 to detect a button press event, you may find that a single press is detected multiple times. This happens because, due to mechanical factors, the button or switch can quickly switch between LOW and HIGH several times. This is called "chattering." Chattering can cause one button press to be detected as multiple presses, which can cause errors in some applications. This tutorial explains how to fix this issue, a process known as debouncing the button.
Or you can buy the following sensor kits:
Disclosure: Some of the links provided in this section are Amazon affiliate links. We may receive a commission for any purchases made through these links at no additional cost to you.
Additionally, some of these links are for products from our own brand,
DIYables .
Learn about buttons (pinout, operation, programming) in the following tutorials if you're not familiar with them:
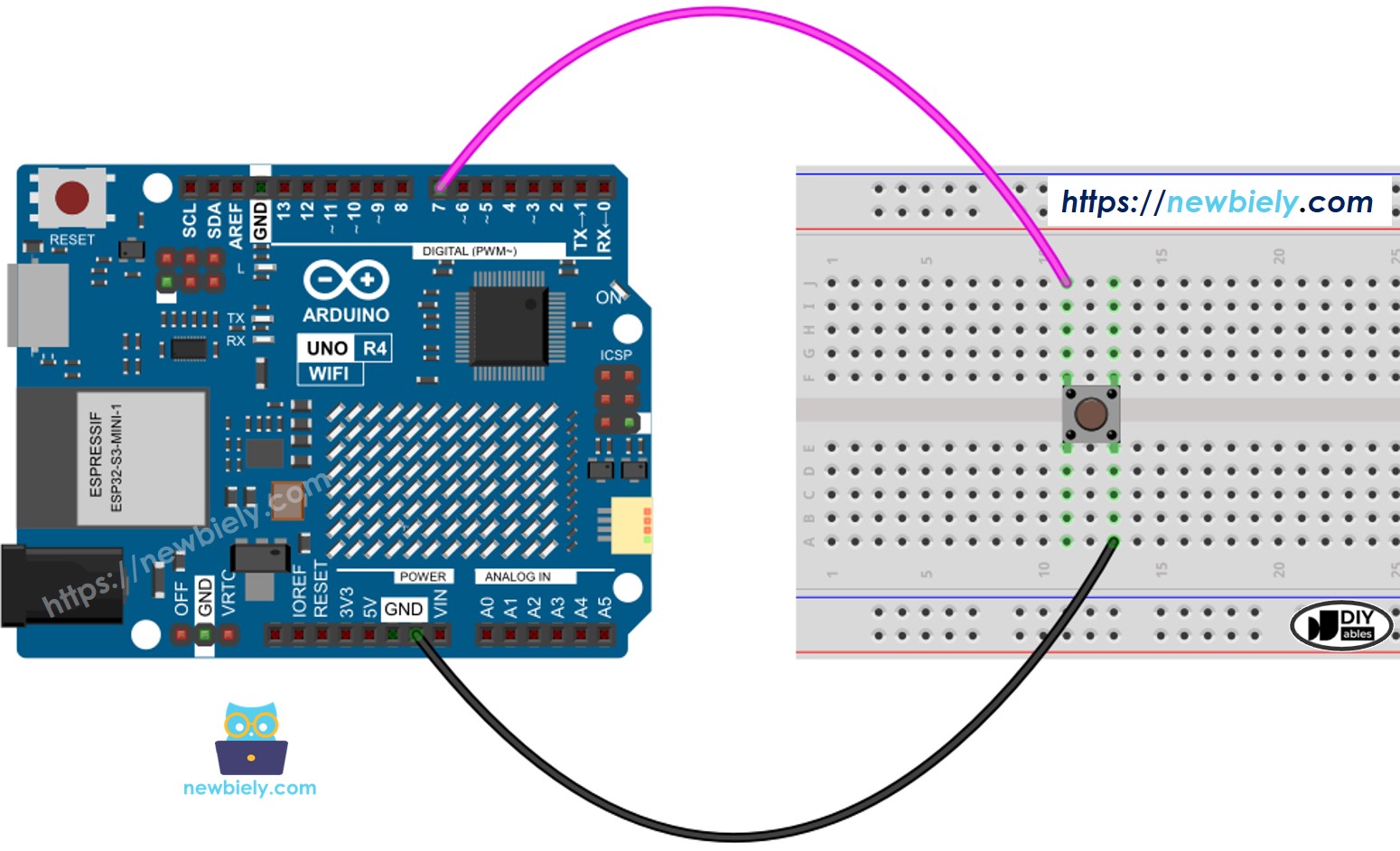
This image is created using Fritzing. Click to enlarge image
Let's examine and compare the Arduino UNO R4 code without and with debounce, and observe their behaviors.
Before we learn about debouncing, let's look at the code without it and see how it behaves.
#define BUTTON_PIN 7
int button_state;
int prev_button_state = LOW;
void setup() {
Serial.begin(9600);
pinMode(BUTTON_PIN, INPUT_PULLUP);
}
void loop() {
button_state = digitalRead(BUTTON_PIN);
if (prev_button_state == HIGH && button_state == LOW)
Serial.println("The button is pressed");
else if (prev_button_state == LOW && button_state == HIGH)
Serial.println("The button is released");
prev_button_state = button_state;
}
Follow these instructions step by step:
Wire the components according to the provided diagram.
Connect the Arduino Uno R4 board to your computer using a USB cable.
Launch the Arduino IDE on your computer.
Select the appropriate Arduino Uno R4 board (e.g., Arduino Uno R4 WiFi) and COM port.
Copy the above code and open it in the Arduino IDE.
Click the Upload button in Arduino IDE to send code to Arduino UNO R4.
Open the Serial Monitor.
Press and hold the button for a few seconds, then release it.
Check the Serial Monitor for the result.
The button is pressed
The button is pressed
The button is pressed
The button is released
The button is released
As you can see, you pressed and released the button only once. However, the Arduino recognizes it as multiple presses and releases.
※ NOTE THAT:
The value of DEBOUNCE_TIME varies with different applications. Each application might use a unique value.
#define BUTTON_PIN 7
#define DEBOUNCE_TIME 50
int last_steady_state = LOW;
int last_flickerable_state = LOW;
int current_state;
unsigned long last_debounce_time = 0;
void setup() {
Serial.begin(9600);
pinMode(BUTTON_PIN, INPUT_PULLUP);
}
void loop() {
current_state = digitalRead(BUTTON_PIN);
if (current_state != last_flickerable_state) {
last_debounce_time = millis();
last_flickerable_state = current_state;
}
if ((millis() - last_debounce_time) > DEBOUNCE_TIME) {
if (last_steady_state == HIGH && current_state == LOW)
Serial.println("The button is pressed");
else if (last_steady_state == LOW && current_state == HIGH)
Serial.println("The button is released");
last_steady_state = current_state;
}
}
Copy the above code and open it with Arduino IDE.
Press the Upload button in the Arduino IDE to send the code to the Arduino UNO R4.
Open the Serial Monitor.
Hold down the button for a few seconds before letting go.
Check out the Serial Monitor.
The button is pressed
The button is released
As you can see, you pressed and released the button once. The Arduino correctly detects it as a single press and release, eliminating any chattering.
We made a simpler way for beginners who use many buttons by creating a library named ezButton. You can find out more about the ezButton library here.
Let's see some example codes.
#include <ezButton.h>
ezButton button(7);
void setup() {
Serial.begin(9600);
button.setDebounceTime(50);
}
void loop() {
button.loop();
if(button.isPressed())
Serial.println("The button is pressed");
if(button.isReleased())
Serial.println("The button is released");
}
Let's debouce for 3 buttons. Here is the wiring diagram between Arduino UNO R4 and three buttons:
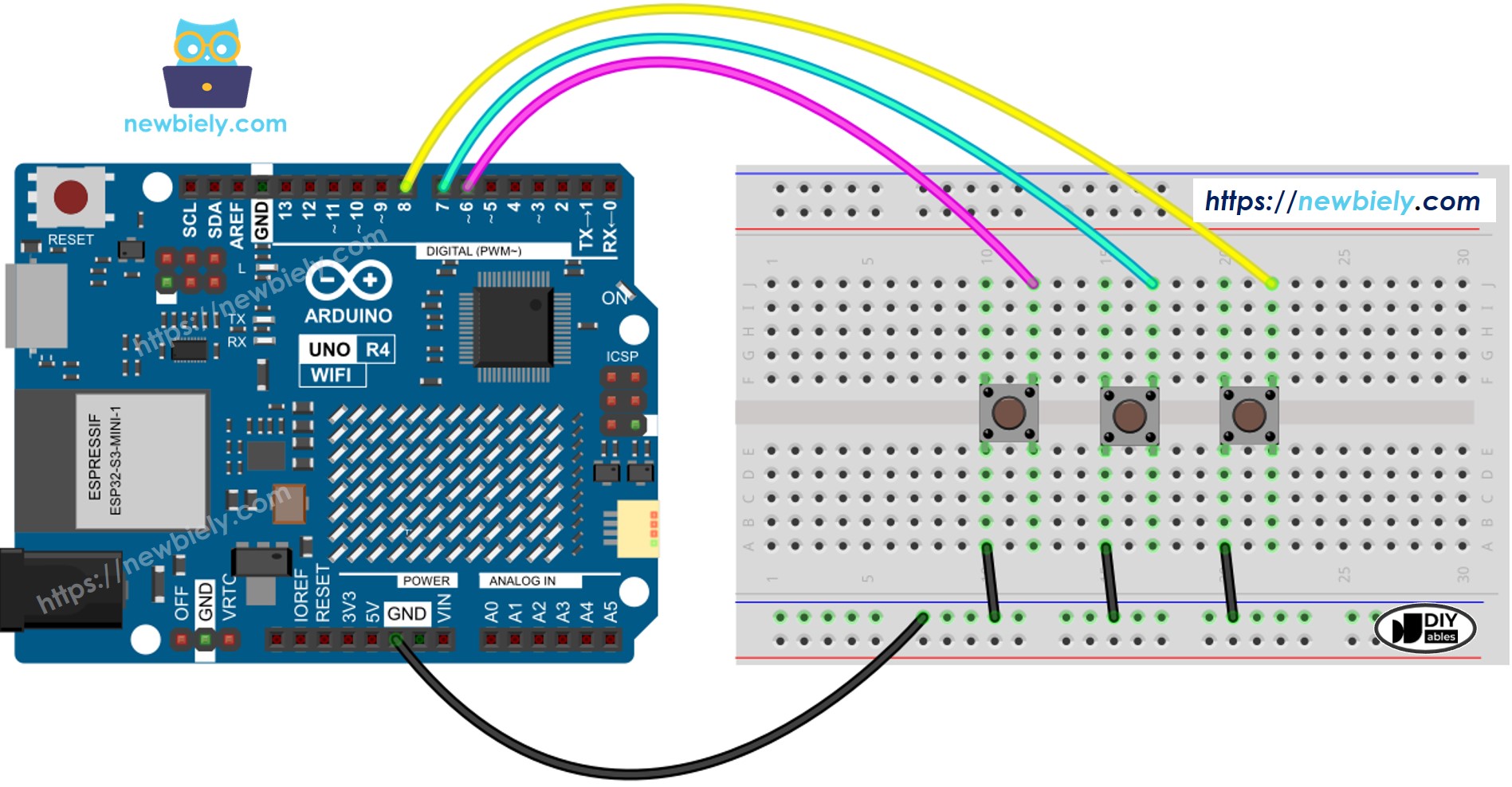
This image is created using Fritzing. Click to enlarge image
See The best way to supply power to the Arduino Uno R4 and other components.
#include <ezButton.h>
ezButton button_1(6);
ezButton button_2(7);
ezButton button_3(8);
void setup() {
Serial.begin(9600);
button_1.setDebounceTime(50);
button_2.setDebounceTime(50);
button_3.setDebounceTime(50);
}
void loop() {
button_1.loop();
button_2.loop();
button_3.loop();
if(button_1.isPressed())
Serial.println("The button 1 is pressed");
if(button_1.isReleased())
Serial.println("The button 1 is released");
if(button_2.isPressed())
Serial.println("The button 2 is pressed");
if(button_2.isReleased())
Serial.println("The button 2 is released");
if(button_3.isPressed())
Serial.println("The button 3 is pressed");
if(button_3.isReleased())
Serial.println("The button 3 is released");
}