Arduino UNO R4 RS232
In this tutorial, we will learn how to use RS232 communication with Arduino UNO R4. We will cover:
- How to connect Arduino UNO R4 with the TTL-to-RS232 module
- How to program Arduino UNO R4 to receive data from the serial device via the TTL-to-RS232 module
- How to program Arduino UNO R4 to send data to the serial device via the TTL-to-RS232 module
The tutorial gives guidance on using both Hardware Serial and SoftwareSerial.
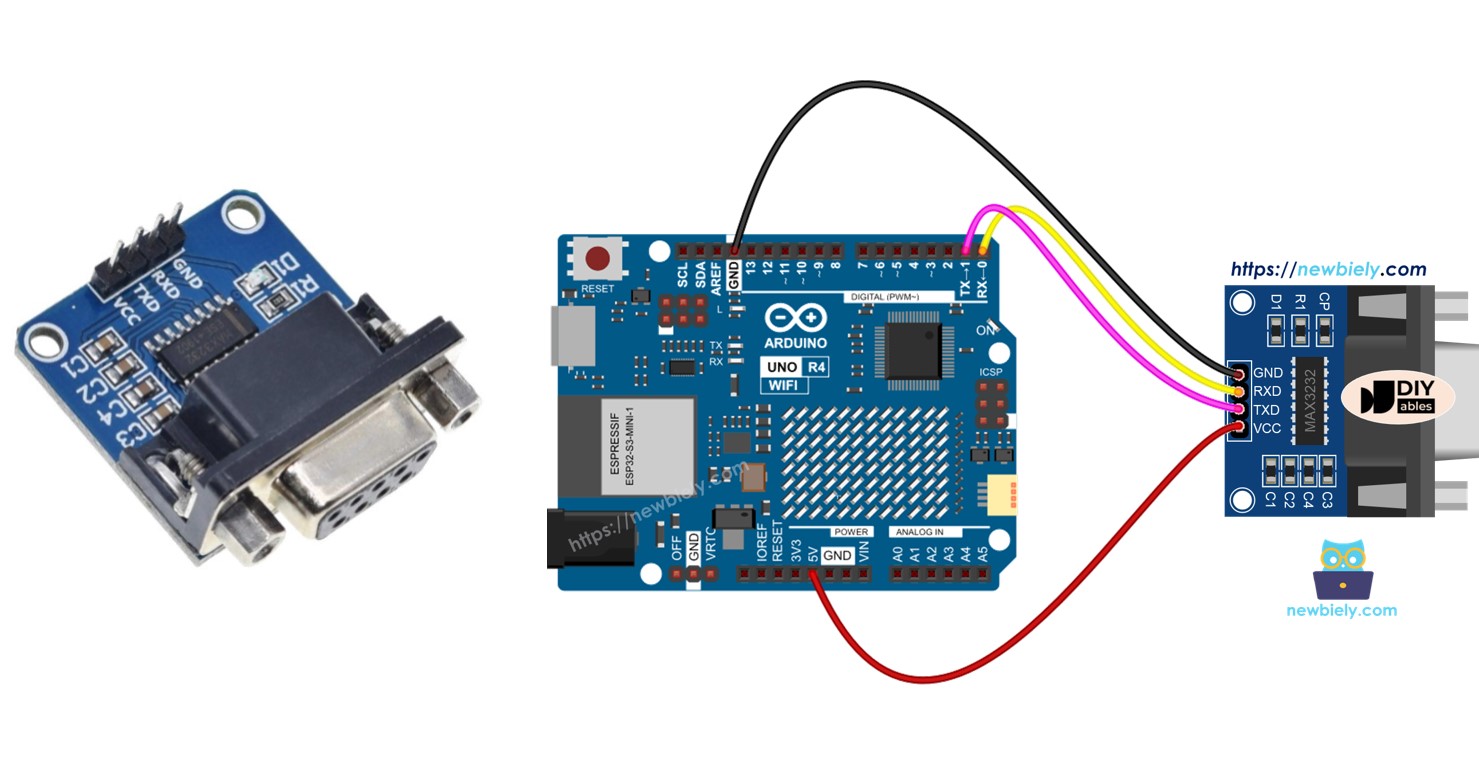
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of TTL to RS232 Module
When using the Serial.print(), Serial.read(), Serial.write() functions on Arduino UNR R4, it sends out data through the TX pin or receives data from the RX pin. The signals on these pins are TTL level, which cannot travel long distances. To communicate over longer distances, you need to convert the TTL signal to RS232, RS485, or RS422 signals.
The TTL-to-RS232 module changes TTL signals into RS232 signals, and also does the opposite.
Pinout
The RS232 to TTL module features two types of connections:
- The TTL interface, which connects to Arduino UNG R4, has 4 pins:
- VCC pin: This is the power pin. Connect it to the VCC (5V/3.3V).
- GND pin: This is the power pin. Connect it to the GND (0V).
- RXD pin: This is the data pin. Connect it to the RX pin on the Arduino UNO R4.
- TXD pin: This is the data pin. Connect it to the TX pin on the Arduino UNO R4.
- The RS232 interface has a DB9 female D-Sub connector. Connect this to your serial device.
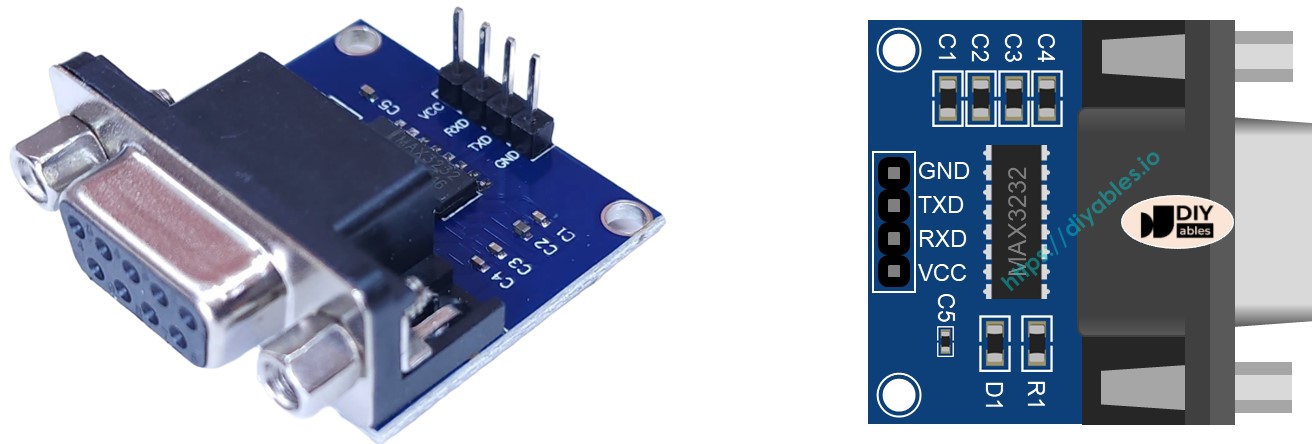
Wiring Diagram
- If you are using hardware serial, here is the wiring diagram.
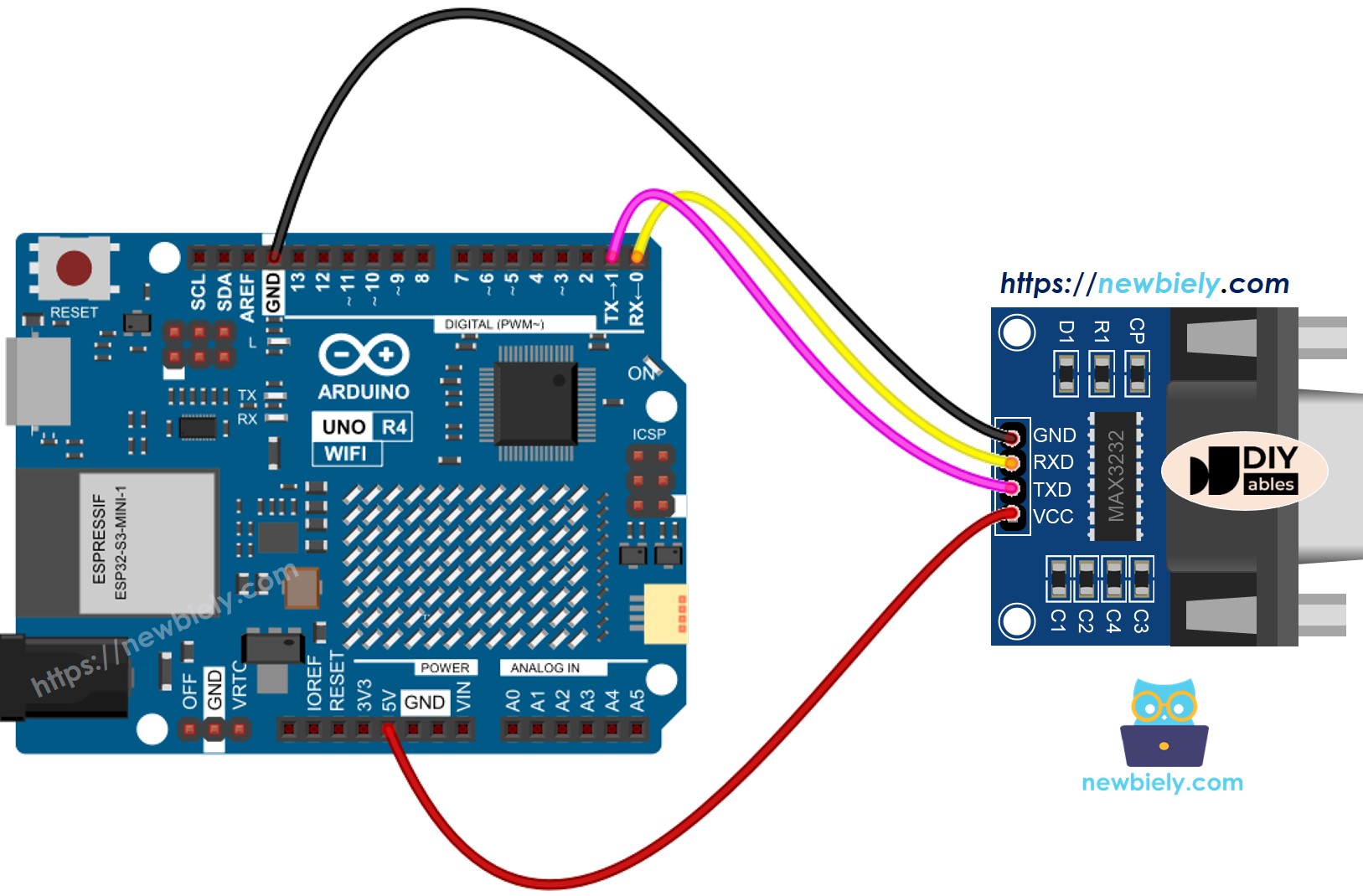
This image is created using Fritzing. Click to enlarge image
- Wiring diagram for using software serial
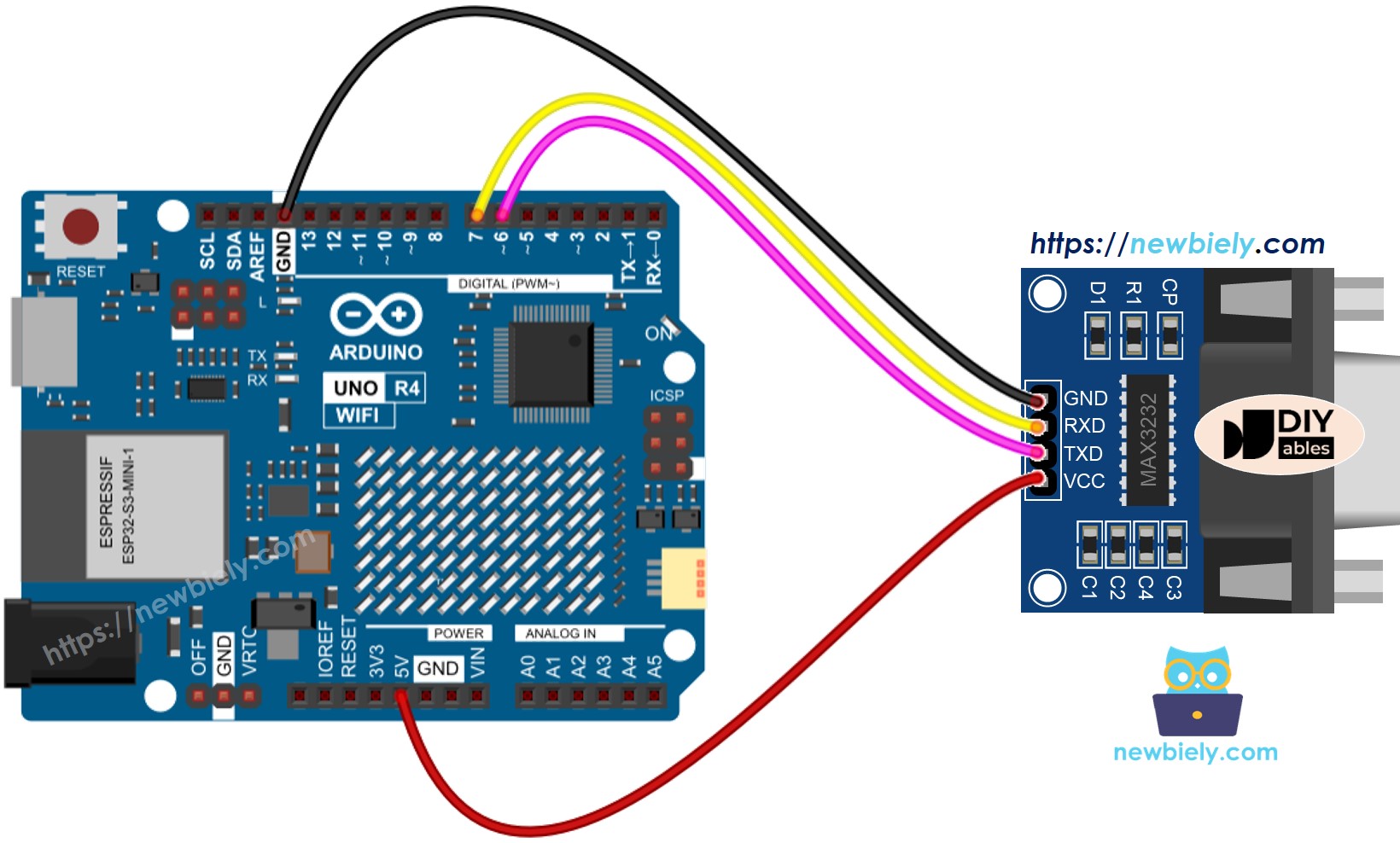
This image is created using Fritzing. Click to enlarge image
See The best way to supply power to the Arduino Uno R4 and other components.
How To Program Arduino UNO R4 to use the RS232 module
- Sets up the Serial connection.
- To use SoftwareSerial, first include the library and then create a SoftwareSerial object.
- To read data from RS232, use these functions:
- To send data to RS232, use these functions:
- Find more functions for RS232 at Serial reference.
Arduino UNO R4 Code for Hardware Serial
Arduino UNO R4 Code for Software Serial
Testing
Follow these instructions step by step:
- If this is your first time using the Arduino Uno R4 WiFi/Minima, refer to the tutorial on setting up the environment for Arduino Uno R4 WiFi/Minima in the Arduino IDE.
- Connect the Arduino Uno R4 to the TTL-to-RS232 module according to the provided diagram.
- Connect the Arduino Uno R4 to board to your computer using a USB cable.
- Launch the Arduino IDE on your computer.
- Select the appropriate Arduino Uno R4 board (e.g., Arduino Uno R4 WiFi) and COM port.
- Copy the provided code and paste it into the Arduino IDE.
- Click the Upload button in the Arduino IDE to transfer the code to the Arduino UNO R4.
To perform a test where you send data between your PC and Arduino UNO R4 using RS232, follow these steps:
- Connect the Arduino UNO R4 to your computer using the RS232-to-USB cable as shown:
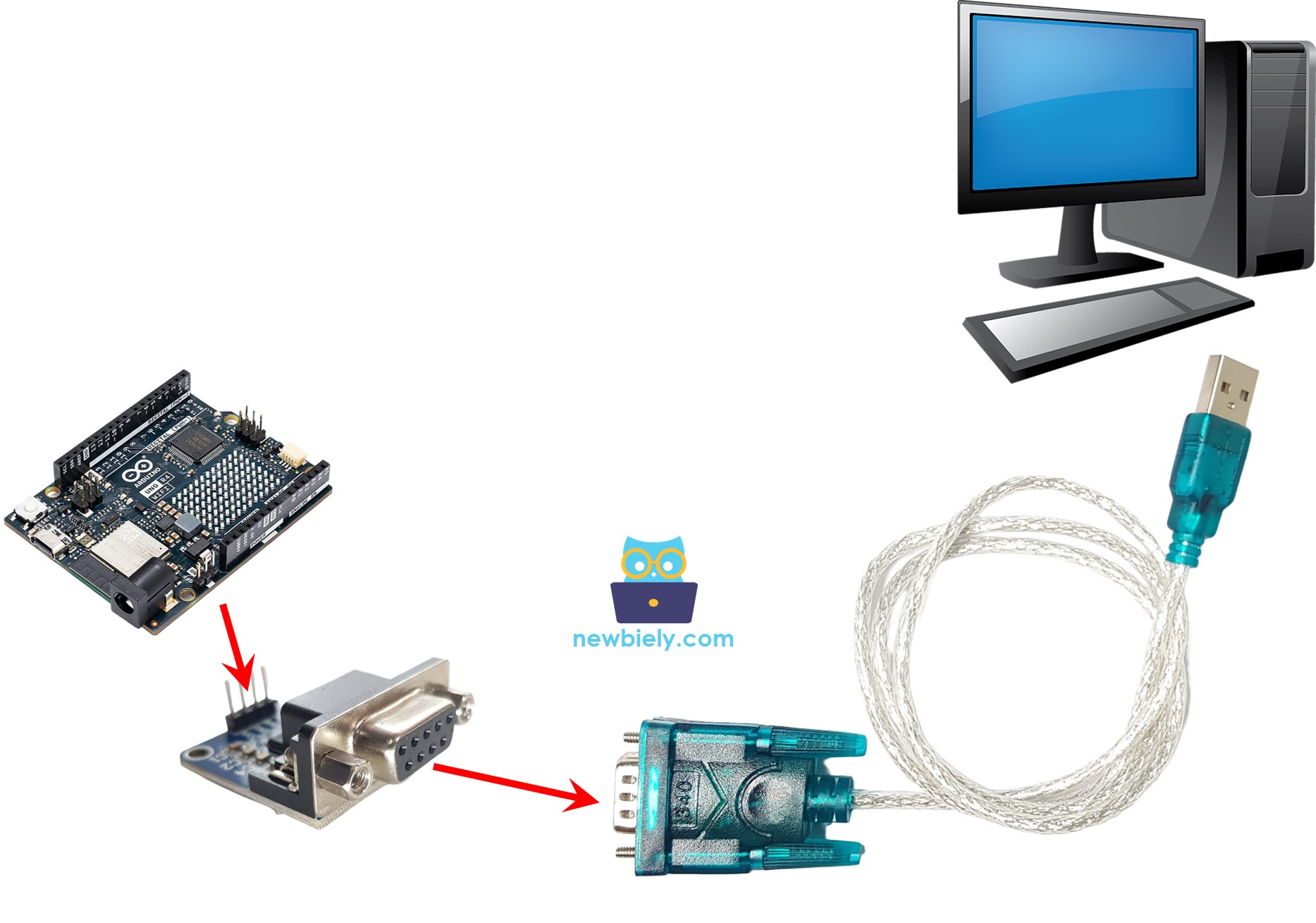
- Open the Serial Terminal Program and set up the Serial parameters (COM port, baud rate, etc.).
- Enter some data in the Serial Terminal to send to the Arduino UNO R4.
- If it works, you will see the echoed data appear on the Serial Terminal.