Arduino UNO R4 - Gas Sensor
This guide will show you how to use Arduino UNO R4 and MQ2 gas sensor to monitor air quality by measuring levels of LPG, smoke, alcohol, propane, hydrogen, methane, and carbon monoxide, among other flammable gases.
- How to connect the gas sensor to Arduino UNO R4
- How to write a code for Arduino UNO R4 to get readings from the gas sensor
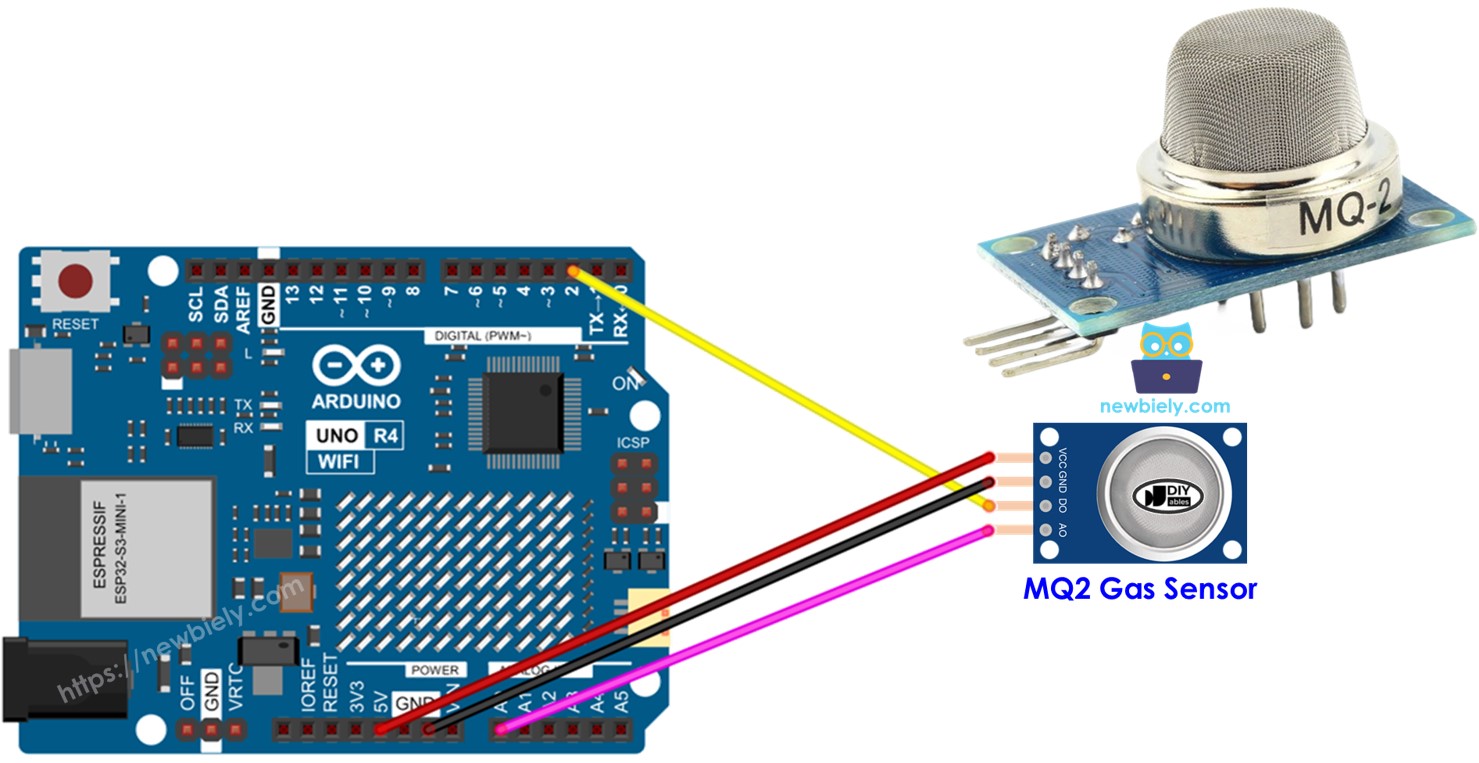
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of MQ2 Gas Sensor
The MQ2 gas sensor can detect LPG, smoke, alcohol, propane, hydrogen, methane, and carbon monoxide levels in the area. It offers a digital output pin and an analog output pin for communication.
The MQ2 gas sensor does not give details for each gas individually. Instead, it provides information about the mix of gases or if there are gases present together.
We can use the MQ2 sensor to find out if there is a gas leak or if the air quality is poor. This helps us to act safely, such as setting off an alarm or starting ventilation systems.
Pinout
The MQ2 gas sensor has four pins:
- VCC pin: Connect this pin to VCC (5V).
- GND pin: Connect this pin to GND (0V).
- DO pin: This is a digital output pin. It shows LOW when flammable gases are detected and HIGH if not. You can adjust the level at which gas is detected using a small adjustable component.
- AO pin: This is an analog output pin. It produces a voltage that changes depending on the amount of gas. More gas makes the voltage go up, less gas makes it go down.
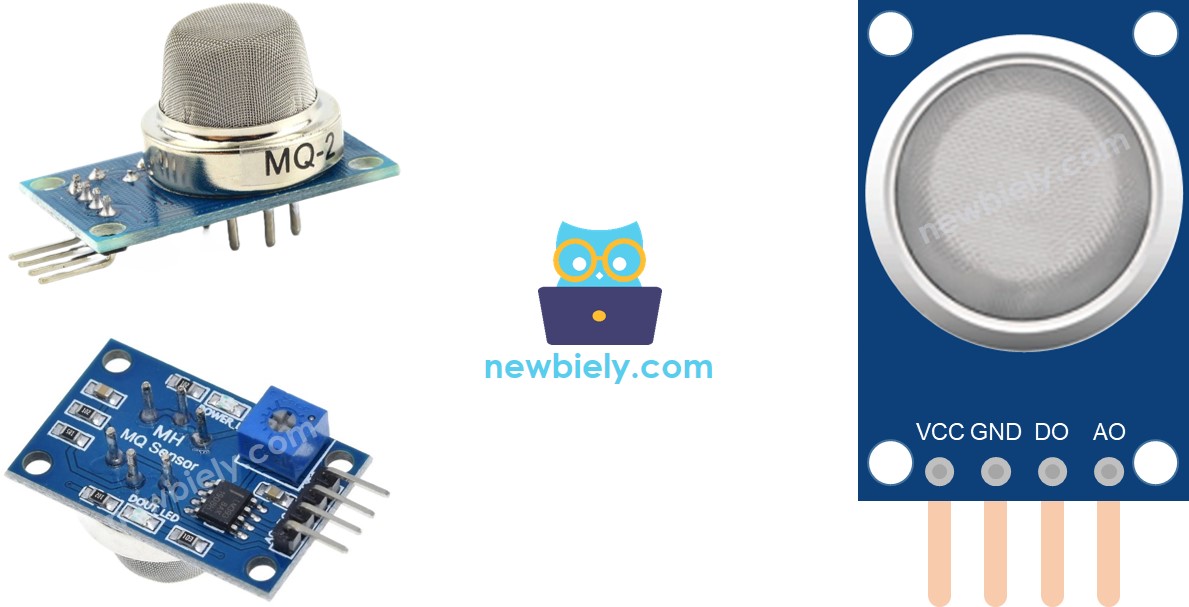
It also has two LED lights.
- One PWR-LED light shows power is on.
- One DO-LED light shows gas levels based on DO pin value: it lights up when there is gas and turns off when there is none.
How It Works
For the DO pin:
- The module includes a potentiometer to adjust the sensitivity for detecting gas concentration.
- If the gas concentration around is higher than the set level, the sensor’s output pin turns LOW and the DO-LED light turns on.
- If the gas concentration around is lower than the set level, the sensor’s output pin turns HIGH and the DO-LED light turns off.
For the AO pin:
- If there is more gas, the voltage goes up.
- If there is less gas, the voltage goes down.
The potentiometer does not change the value on the AO pin.
The MQ2 Sensor Warm-up
The MQ2 gas sensor must be heated before use.
- If the sensor hasn't been used for a long period (over a month), please warm it up for 24-48 hours before using it to get accurate results.
- If the sensor was used not long ago, it requires only 5-10 minutes to warm up. At first, the readings might be high, but they will lower and become steady after a short while.
To heat up the MQ2 sensor, connect its VCC and GND pins to a power source or to the VCC and GND on an Arduino UNO R4, and leave it connected for some time.
Wiring Diagram
The MQ2 gas sensor module has two outputs. You can use one or both, depending on your needs.
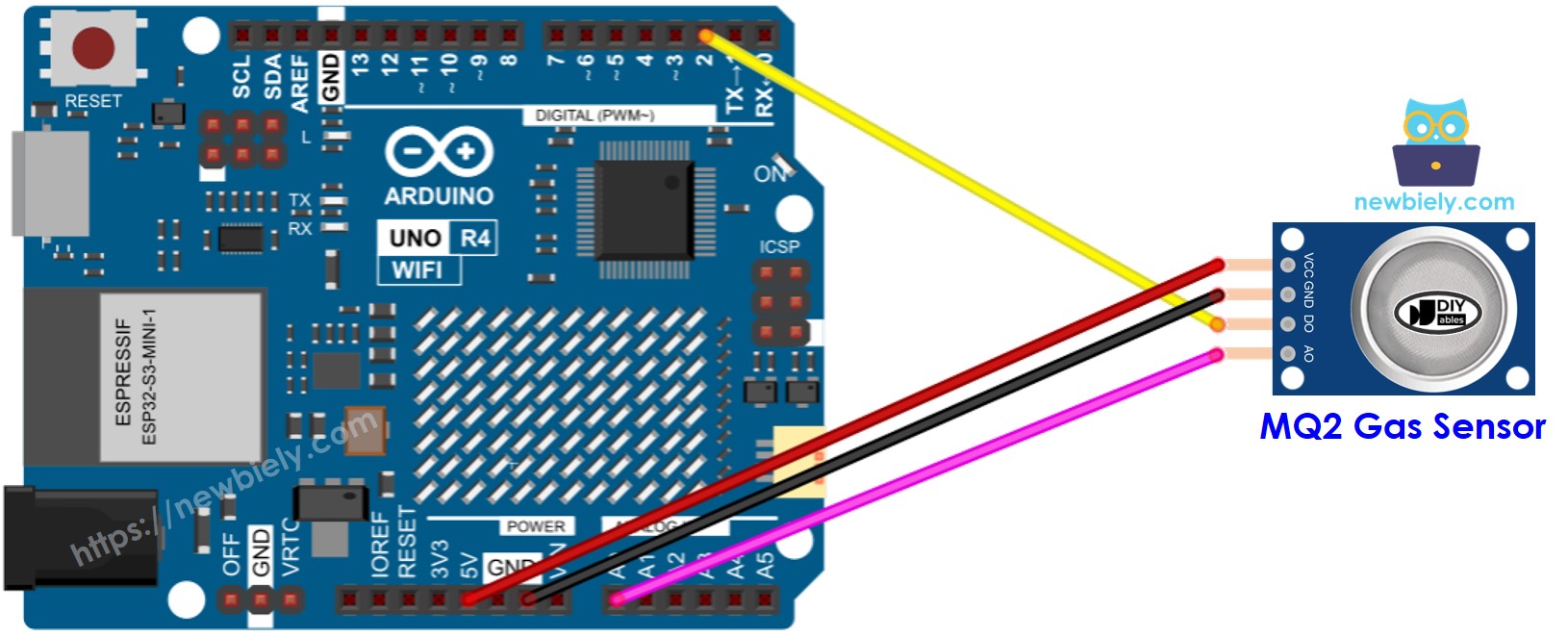
This image is created using Fritzing. Click to enlarge image
See The best way to supply power to the Arduino Uno R4 and other components.
Arduino UNO R4 Code - Read value from DO pin
Detailed Instructions
Follow these instructions step by step:
- If this is your first time using the Arduino Uno R4 WiFi/Minima, refer to the tutorial on setting up the environment for Arduino Uno R4 WiFi/Minima in the Arduino IDE.
- Connect the Arduino Uno R4 board to the gas sensor according to the provided diagram.
- Connect the Arduino Uno R4 board to your computer using a USB cable.
- Launch the Arduino IDE on your computer.
- Select the appropriate Arduino Uno R4 board (e.g., Arduino Uno R4 WiFi) and COM port.
- Copy the code above and open it in Arduino IDE.
- Click the Upload button in Arduino IDE to upload the code to Arduino UNO R4.
- Place the MQ2 gas sensor close to the smoke or gas you wish to detect.
- Check the result on the Serial Monitor.
Please remember that if the LED light stays on all the time or does not turn on at all, you can turn the small knob (potentiometer) to adjust the sensor's sensitivity.
Arduino UNO R4 Code - Read value from AO pin
Detailed Instructions
- Copy the code above and open it in Arduino IDE
- Click the Upload button in Arduino IDE to upload the code to Arduino UNO R4
- Put the MQ2 gas sensor close to the smoke or gas that needs to be detected
- Check the result on the Serial Monitor.
Based on the values from DO or AO, you can determine the air quality according to your standards, or activate an alarm or start the ventilation systems.