Arduino UNO R4 - RFID
In this guide, we will learn to use RFID/NFC technology with an Arduino UNO R4. The system has two parts: a reader and a tag. We will discuss two types of readers: the RC522 and the PN532. This guide will focus on the RC551 reader, and we will cover the PN532 in another guide soon.
The RC522 RFID/NFC reader, which is also known as the RFID-RC522 Module, can:
- Check the UID of RFID/NFC tag
- Modify the UID of RFID/NFC tag (only possible if the tag allows UID changes)
- Add data to RFID/NFC tag
- Retrieve data from RFID/NFC tag
This tutorial shows you how to read the UID of an RFID/NFC tag using the Arduino UNO R4. This is a common use for this device. More tutorials on other features will come later.
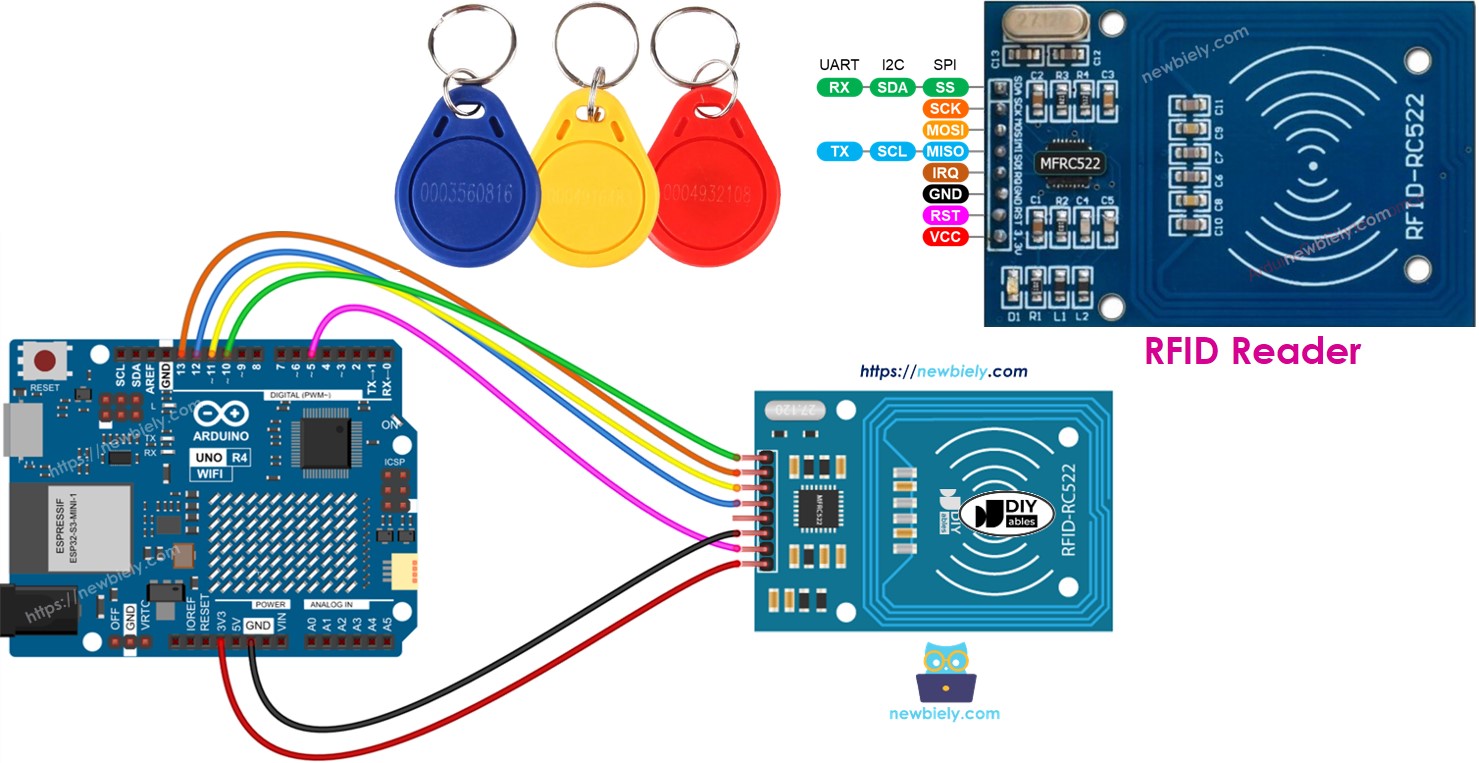
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables.
Overview of RFID-RC522 Module
RFID-RC522 Module Pinout
The RFID-RC522 includes 8 pins. Some pins are common, while others are used for three different communication methods: SPI, I2C, and UART. You can use only one communication method at a time. The pins are:
- GND pin: Connect this to GND (0 volts).
- VCC pin: Connect this to VCC (3.3 volts).
- RST pin: This is a reset and power-down pin. If this pin receives a low signal, it will activate a hard power-down. A rising signal resets the module.
- IRQ pin: This is an interrupt pin that notifies the microcontroller when an RFID tag is nearby.
- MISO/SCL/TX pin: Serves as MISO with SPI, SCL with I2C, and TX with UART.
- MOSI pin: Functions as MOSI with SPI enabled.
- SCK pin: Works as SCK with SPI enabled.
- SS/SDA/RX pin: Operates as SS with SPI, SDA with I2C, and RX with UART.
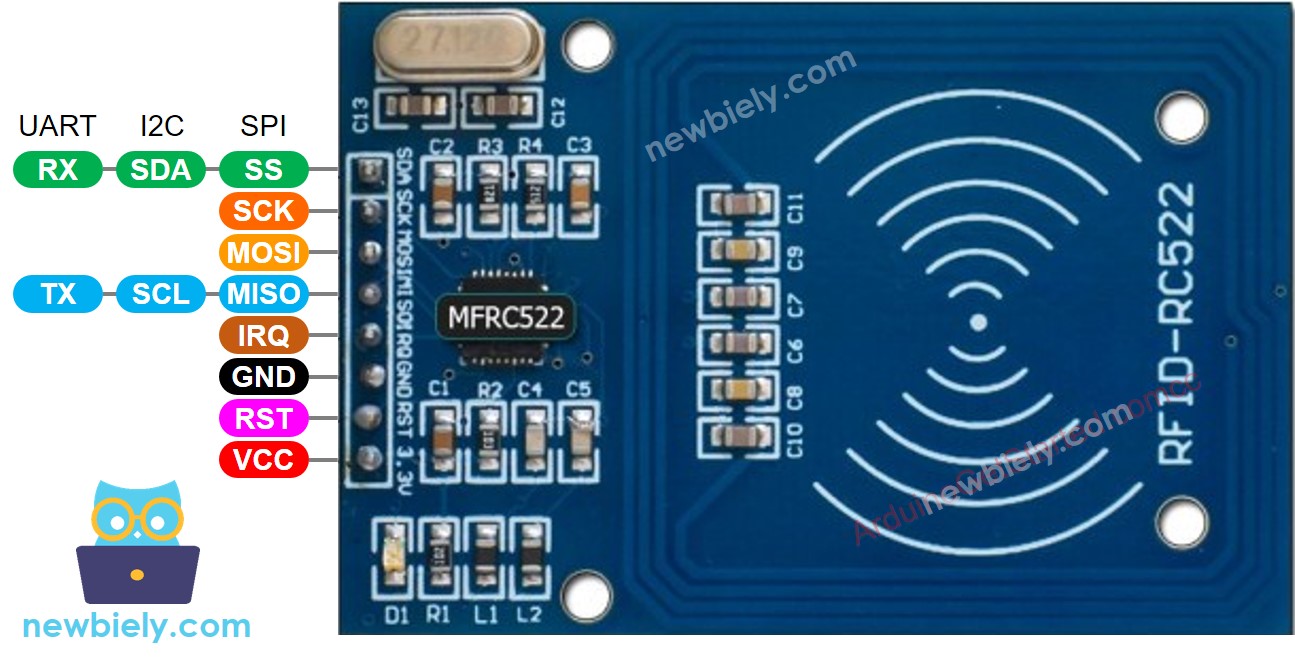
※ NOTE THAT:
- The arrangement of pins might be different for each manufacturer. Always follow the labels on the module itself. The image provided is specific to the DIYables manufacturer.
- Do not connect the VCC pin to the 5V pin, as it may damage your module.
- The MFRC522 library works with SPI mode only. Thus, this guide uses SPI communication exclusively.
How RFID/NFC Works
RFID/NFC has two parts: a reader and a tag.
- The reader includes a radio frequency module and an antenna that creates a high frequency electromagnetic field.
- The tag is a passive device that does not require its own power source. It has a microchip for storing and processing information, and an antenna to send and receive signals. The tag holds information like UID (Unique ID) and other data.
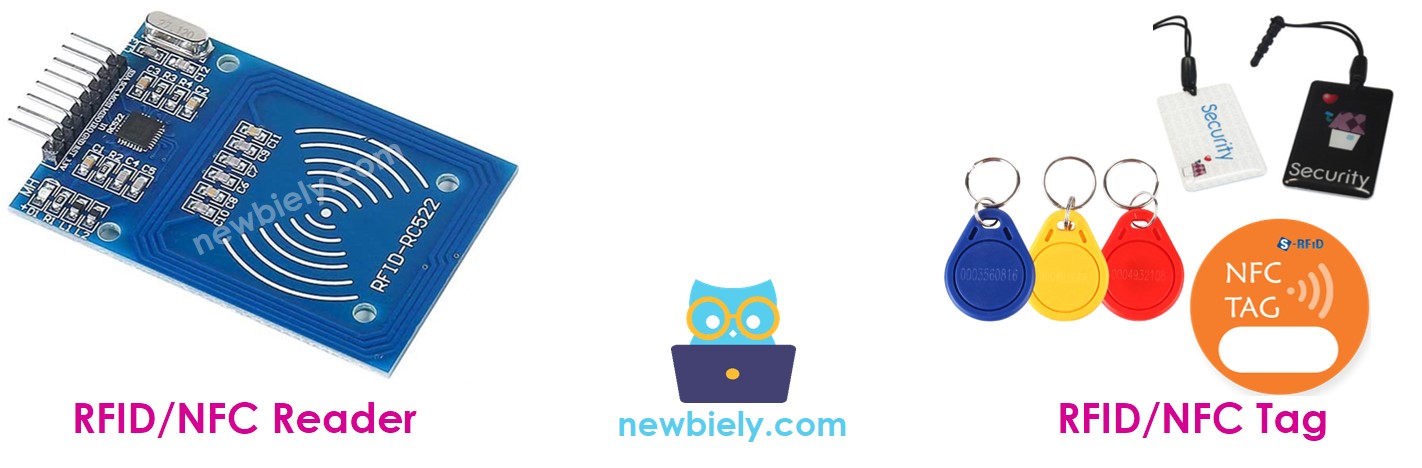
To read the information on a tag, the tag needs to be near the reader (it doesn't require being directly seen). The reading processes:
- The reader creates a magnetic field that makes electrons flow through the tag's antenna, which then activates the chip.
- Next, the chip in the tag sends back the needed information to the reader as a new radio signal.
- The reader picks up this signal and converts it into data.
- The Arduino UNO R4 processes the data received from the reader.
Wiring Diagram between RFID-RC522 Module and Arduino UNO R4
The RFID-RC522 Module works with 3.3V power, but the output pins of an Arduino UNO R4 give out 5V power.
- Ensure safety by reducing the voltage from 5V on the Arduino UNO R4 pins to 3.3V before linking to the RC522 Module. For simplicity and testing, you can connect the Arduino UNO R4 pins directly to the RC522 Module, but this may sometimes lead to the Arduino UNO R4 not functioning properly.
This guide offers two different wiring diagrams for each case:
- How to Connect RC522 and Arduino UNO R4 without a Voltage Regulator
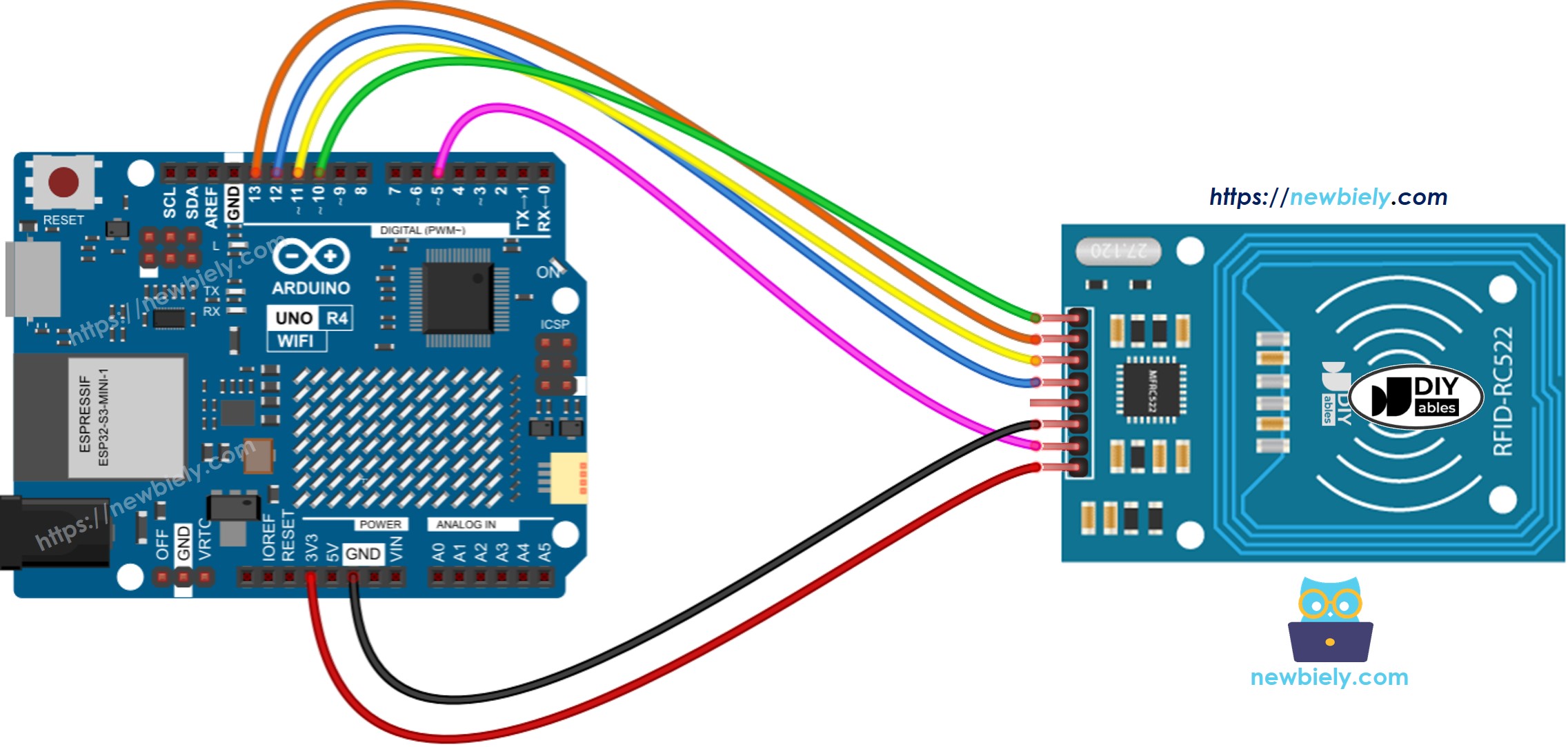
This image is created using Fritzing. Click to enlarge image
- Wiring Diagram for Connecting RC522 to Arduino UNO R4 with a Voltage Regulator
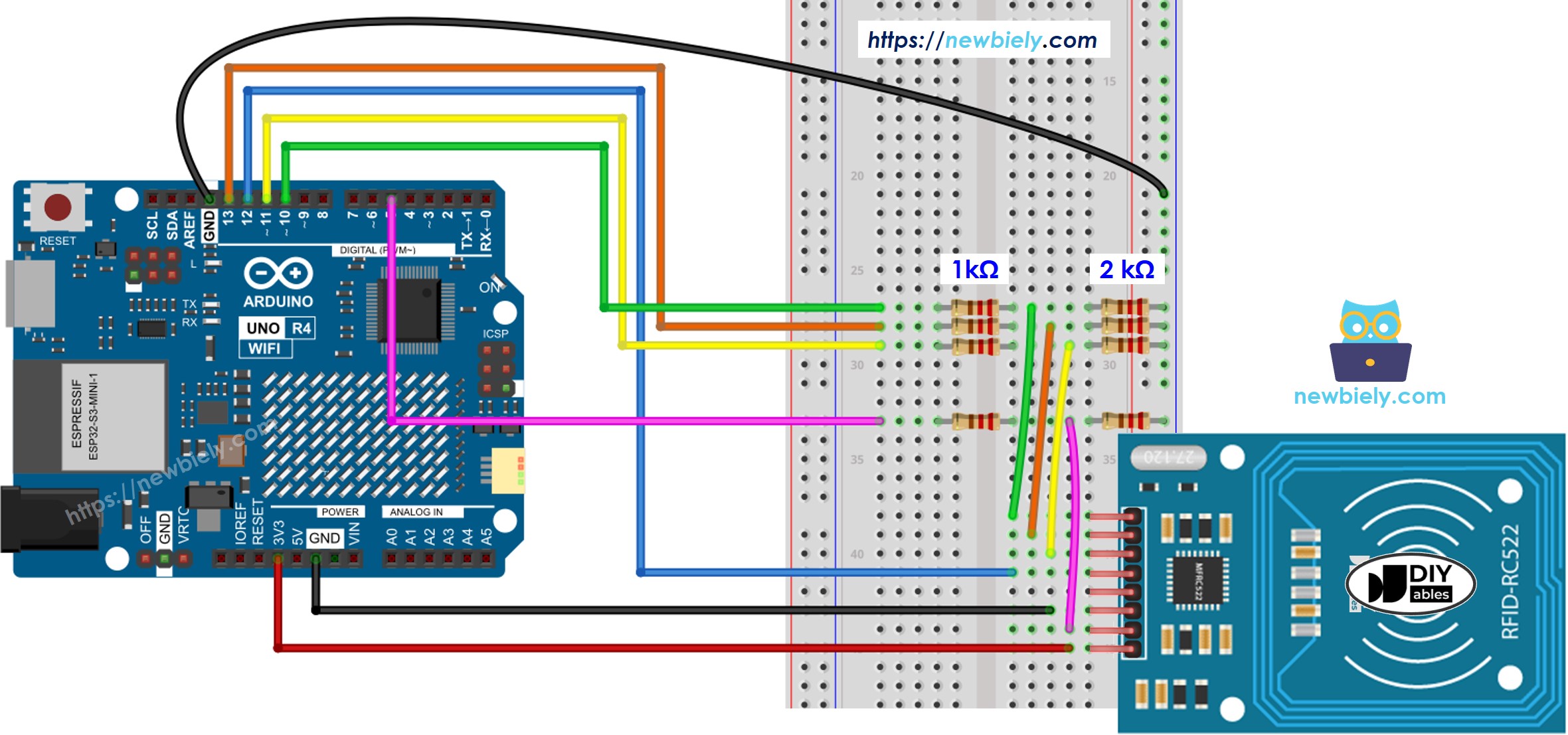
This image is created using Fritzing. Click to enlarge image
The wiring diagram shows that a pair of resistors, 1kOhm and 2kOhm, are used to regulate the voltage from 5V to 3.3V. There is no need to adjust the voltage between the Arduino UNO R4 pin and the MISO pin of the RC522 module. However, you need to regulate the voltage between the Arduino UNO R4 pins and the SS, SCK, MOSI, and RST pins of the RC522 module.
Wiring table of RFID/NFC RC522 Module and Arduino UNO R4
RFID/NFC RC522 | Arduino UNO R4 |
---|---|
SS | → 10 |
SCK | → 13 |
MOSI | → 11 |
MISO | → 12 |
IRQ(not connected) | |
GND | → GND |
RST | → 5 |
VCC | → 3.3V |
Arduino UNO R4 RFID/NFC Code
Detailed Instructions
Follow these instructions step by step:
- If this is your first time using the Arduino Uno R4 WiFi/Minima, refer to the tutorial on setting up the environment for Arduino Uno R4 WiFi/Minima in the Arduino IDE.
- Wire the components according to the provided diagram.
- Connect the Arduino Uno R4 board to your computer using a USB cable.
- Launch the Arduino IDE on your computer.
- Select the appropriate Arduino Uno R4 board (e.g., Arduino Uno R4 WiFi) and COM port.
- Go to the Libraries icon on the left side of the Arduino IDE.
- Search for “MFRC522” and locate the library by GithubCommunity.
- Click the Install button to add the MFRC522 library.
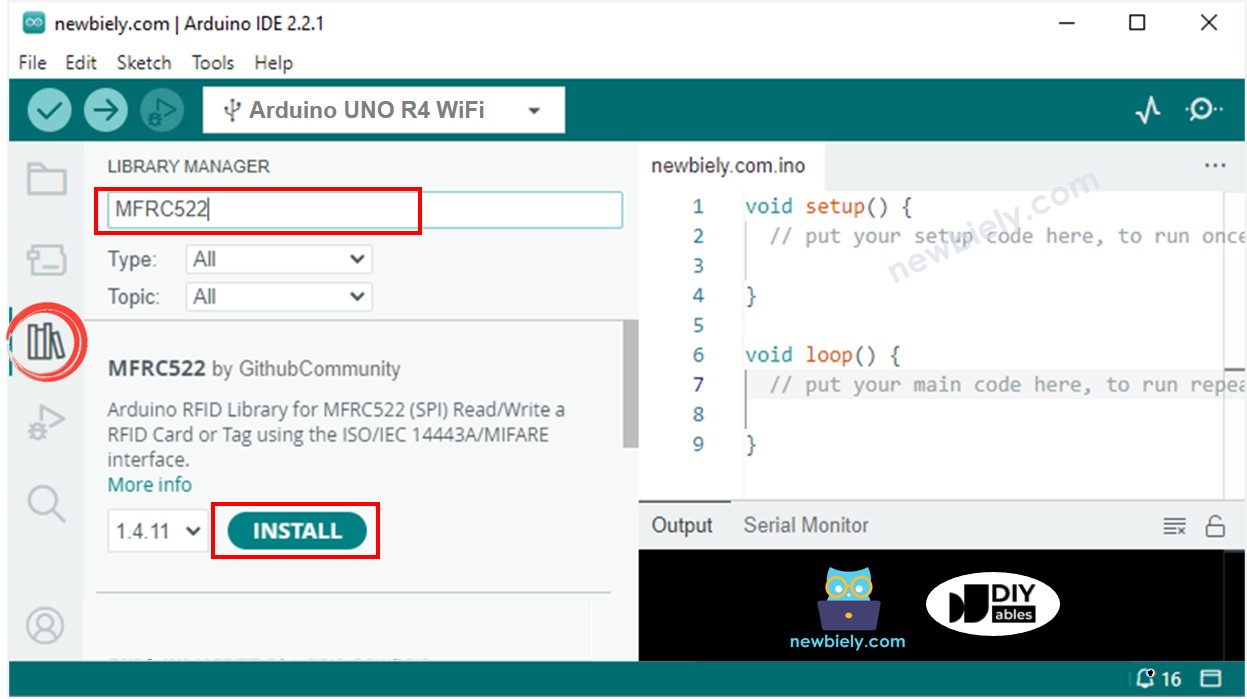
- Copy the code and open it in Arduino IDE
- Click the Upload button in Arduino IDE to upload the code to Arduino UNN R4
- Open the Serial Monitor
- Tap some RFID/NFC tags on the RFID-RC522 module
- Check the UID displayed on the Serial Monitor