Arduino UNO R4 - LCD I2C
This tutorial instructs you how to use a 16x2 LCD I2C interface with an Arduino UNO R4. In detail, We will learn:
- How to connect LCD I2Cto Arduino UNO R4
- How to program Arduino UNO R4 to display text on LCD I2C
- How to program Arduino UNO R4 to display numbers on LCD I2C
- How to program Arduino UNO R4 to display custom characters on LCD I2C
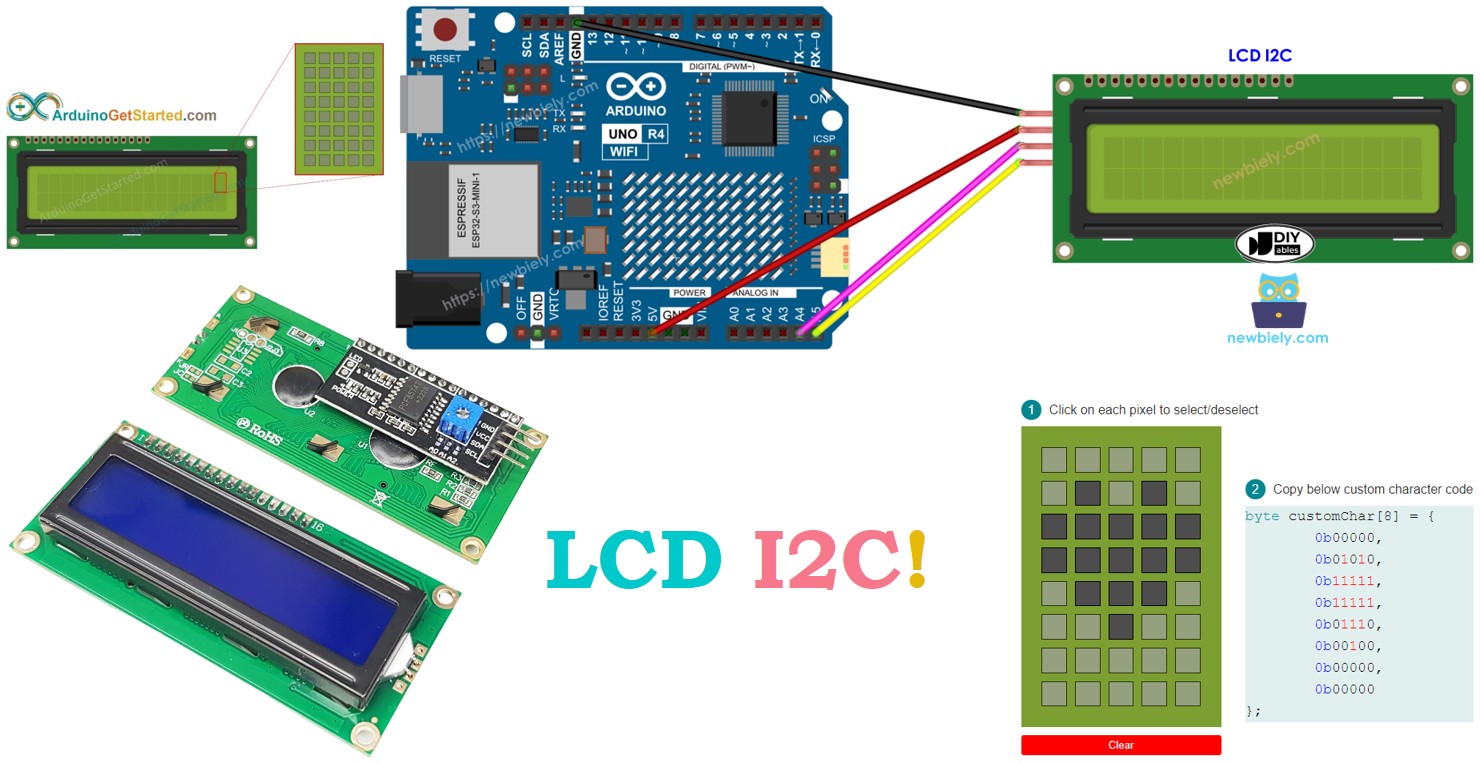
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of LCD I2C 16x2
The 16x2 LCD I2C is a display featuring 16 columns and 2 rows. It is equipped with an I2C interface and includes a potentiometer for contrast adjustment.
Pinout
The LCD I2C connects through an I2C interface and has 4 pins.
- GND pin: connect to GND (0 volts).
- VCC pin: connect to VCC for power (5 volts).
- SDA pin: carries I2C data signal.
- SCL pin: carries I2C clock signal.
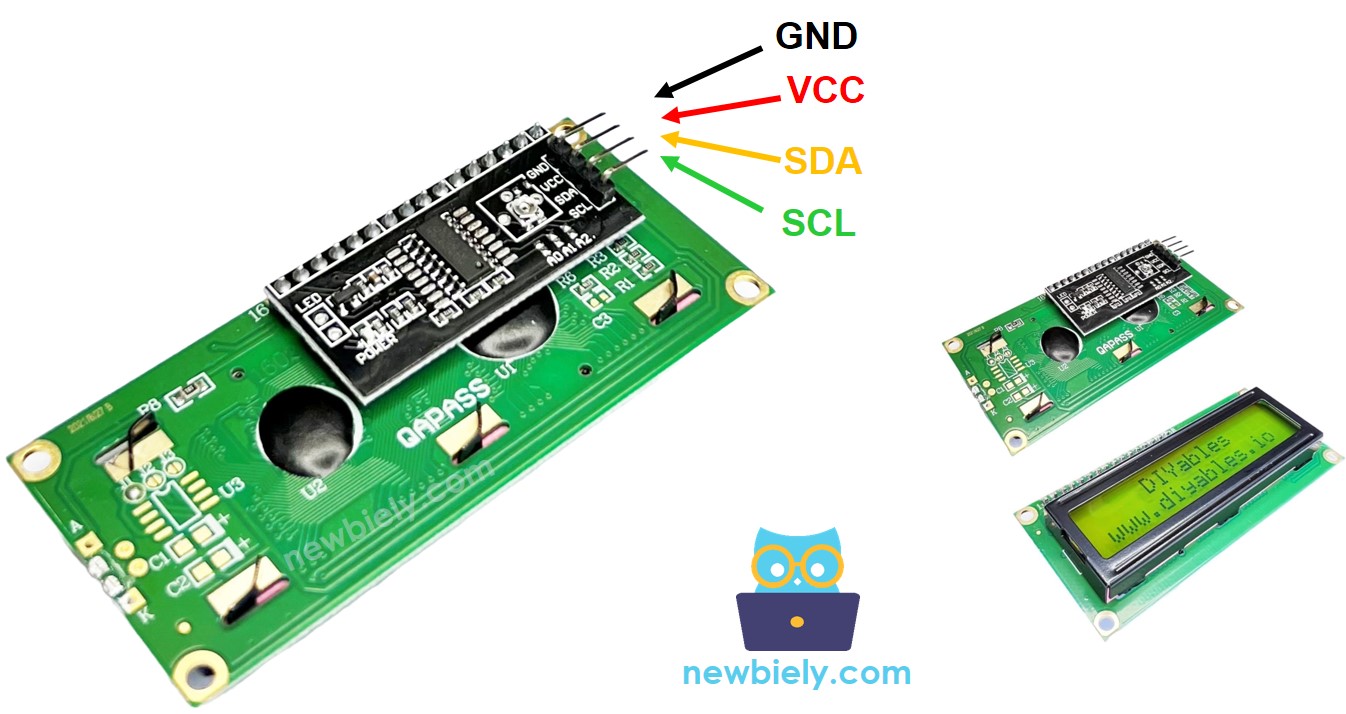
LCD Coordinate
The LCD I2C 16x2 has 16 columns and 2 rows. Both columns and rows start numbering from 0.
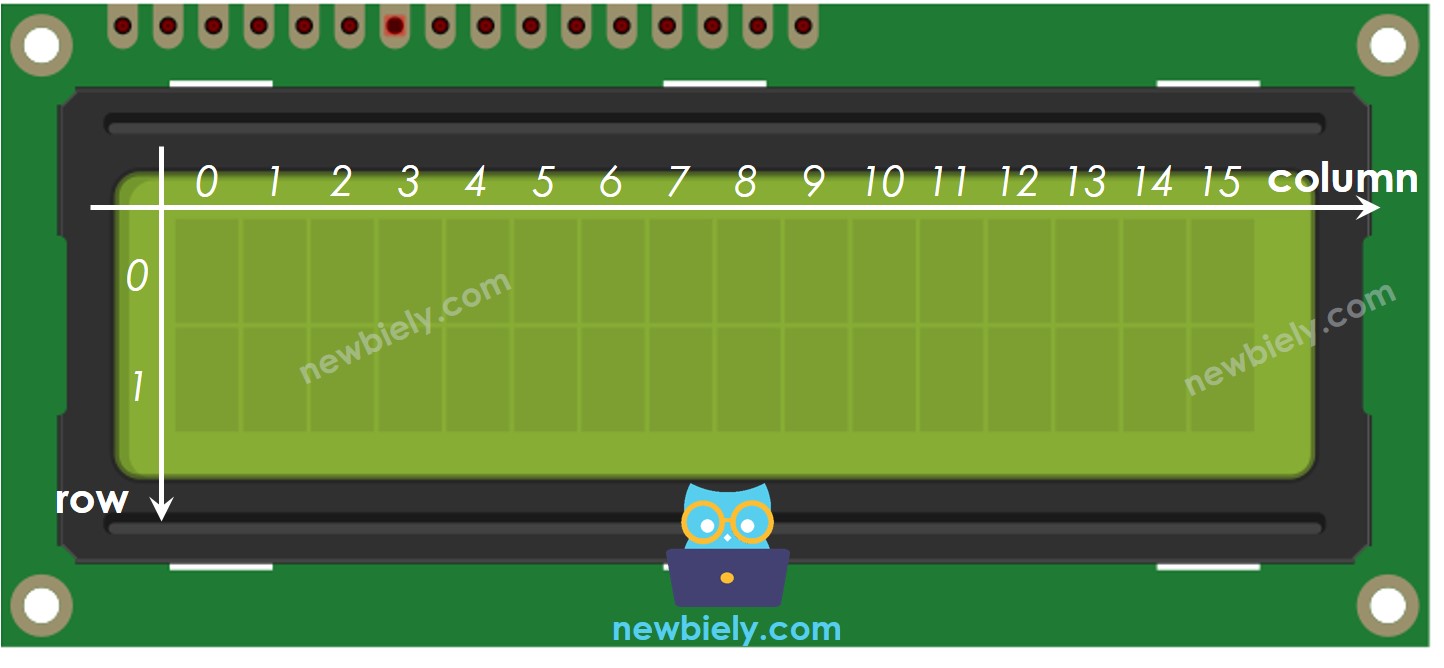
Wiring Diagram
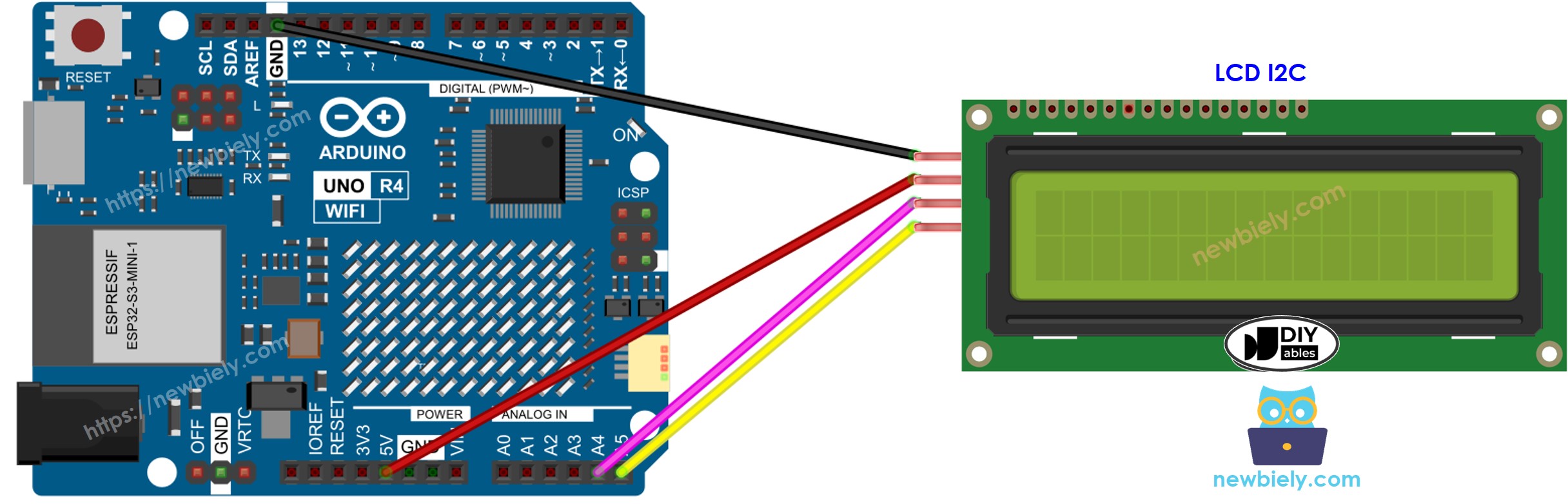
This image is created using Fritzing. Click to enlarge image
See The best way to supply power to the Arduino Uno R4 and other components.
LCD I2C | Arduino UNO R4, Nano | Arduino Mega |
---|---|---|
Vin | 5V | 5V |
GND | GND | GND |
SDA | A4 | 20 |
SCL | A5 | 21 |
How To Program For LCD I2C
Using the LiquidCrystal_I2C library makes handling the LCD very easy.
- Include the library:
- Create a LiquidCrystal_I2C object by specifying its I2C address, the number of columns, and the number of rows.
- Set up the LCD screen.
- Place the cursor at the chosen spot (column_index, row_index).
- Display a message on the LCD screen.
We can do many more things with an LCD (see the 'Do More with LCD' section).
※ NOTE THAT:
The I2C address for the LCD might be different depending on the manufacturer. In our code, we used the address 0x27 as specified by the manufacturer DIYables.
Arduino UNO R4 Code
Detailed Instructions
Follow these instructions step by step:
- If this is your first time using the Arduino Uno R4 WiFi/Minima, refer to the tutorial on setting up the environment for Arduino Uno R4 WiFi/Minima in the Arduino IDE.
- Connect LCD I2C display to Arduino UNO R4 according to the provided diagram.
- Connect the Arduino Uno R4 board to your computer using a USB cable.
- Launch the Arduino IDE on your computer.
- Select the appropriate Arduino Uno R4 board (e.g., Arduino Uno R4 WiFi) and COM port.
- Go to the Libraries icon on the left side of the Arduino IDE.
- Type "LiquidCrystal I2C" in the search box and look for the LiquidCrystal_I2C library by Frank de Brabander.
- Press the Install button to install the LiquidCrystal_I2C library.
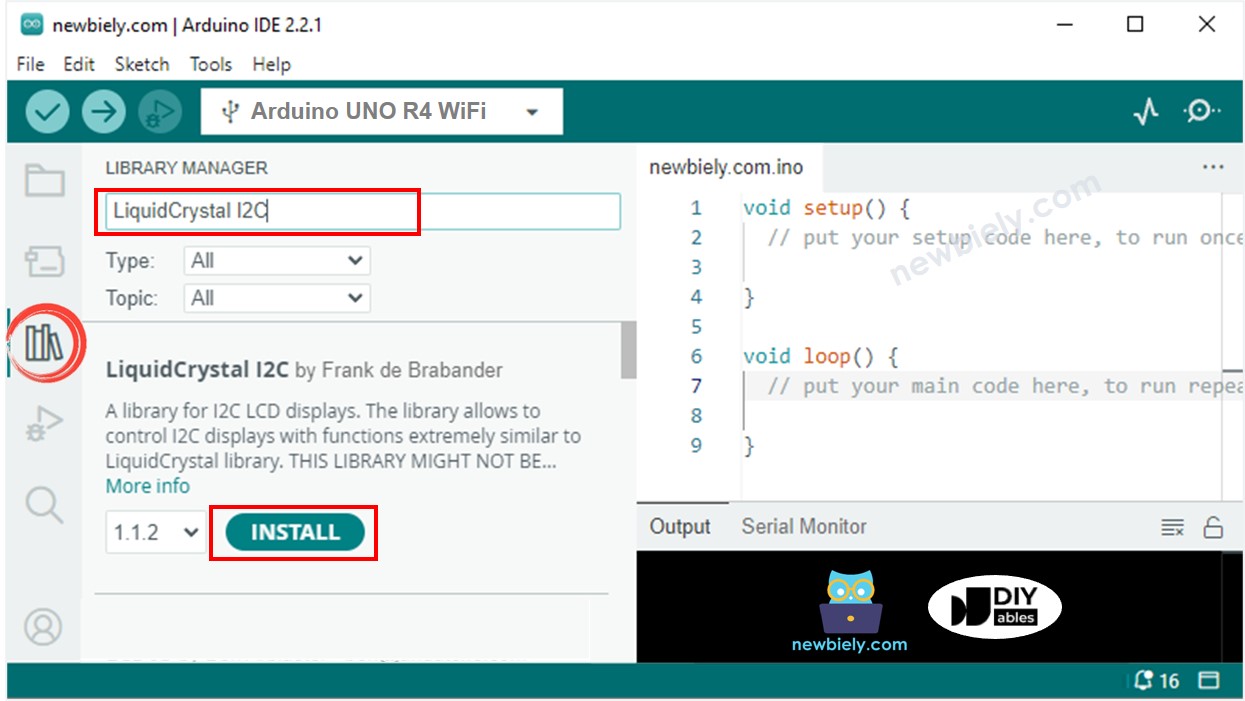
- Copy the code and open it in Arduino IDE
- Click the Upload button in Arduino IDE to upload the code to Arduino UNO R4
- Check out the result on the LCD screen
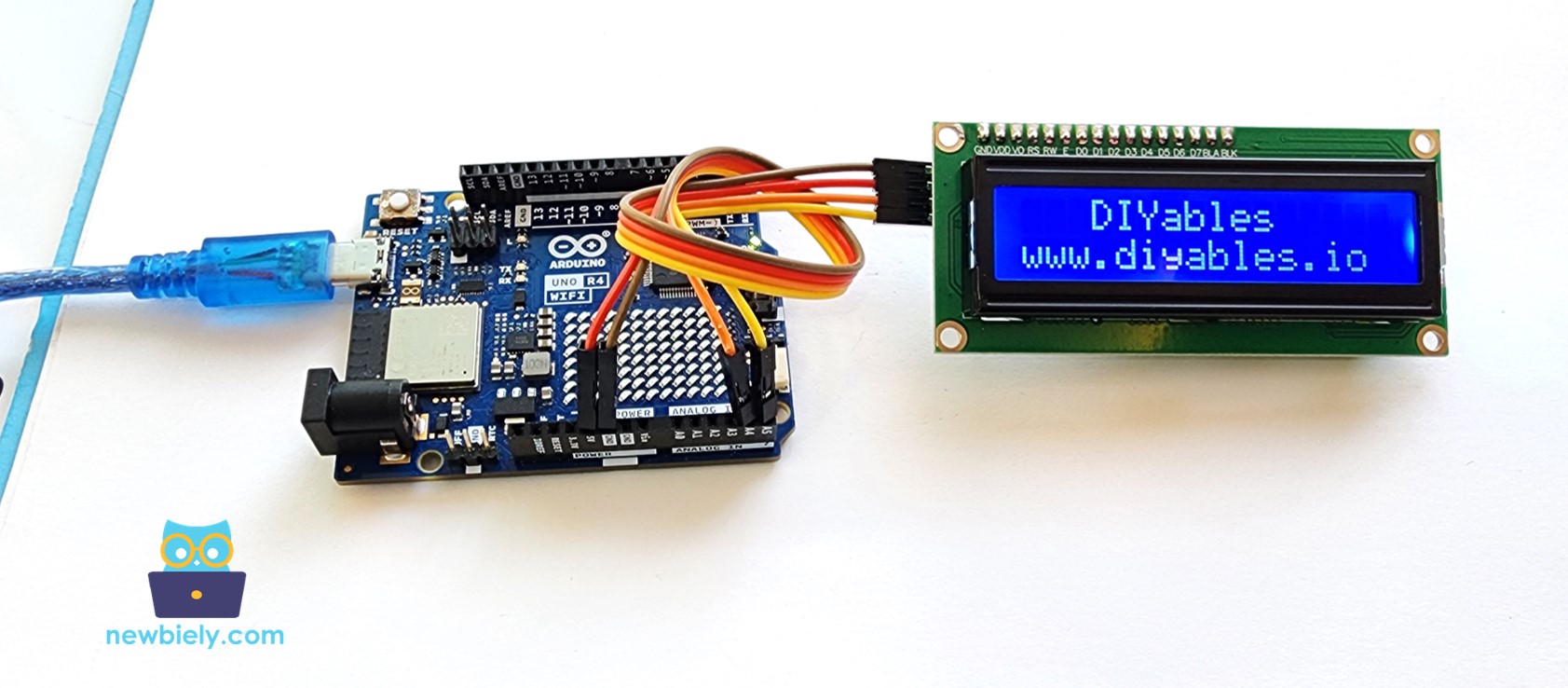
- Try changing the text and its location
Video Tutorial
Do More with LCD
Custom Character
To show special characters or symbols like a heart or an angry bird on an LCD, you must use the character generator because the lcd.print() function can only display ASCII characters.
The LCD 16x2 can show 32 characters across 2 lines, with 16 spots on each line. Every character uses 40 tiny squares, arranged in 8 lines and 5 columns.
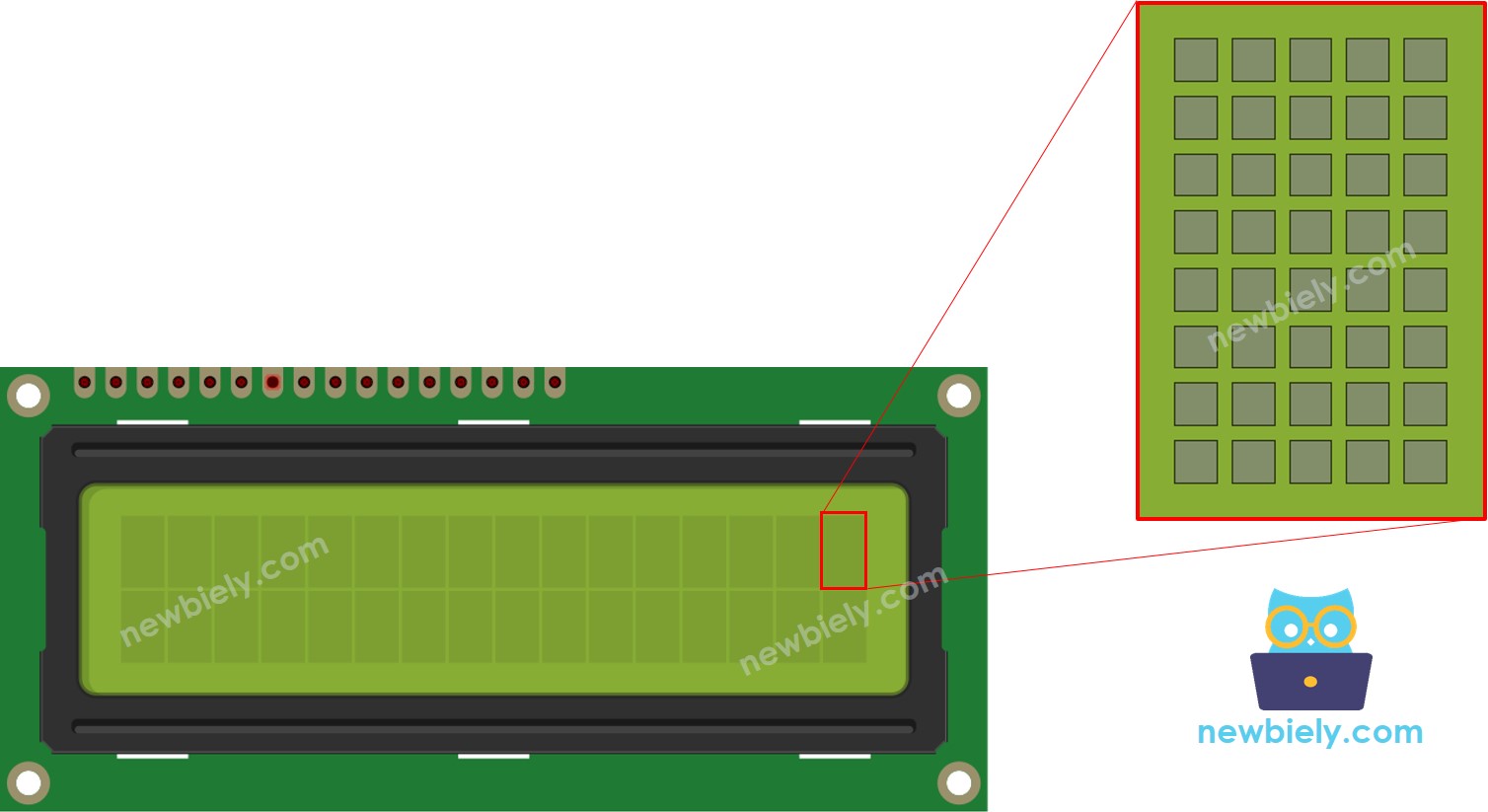
The character generator creates a character (40 pixels). You only need to follow these steps:
Result displayed on the screen:
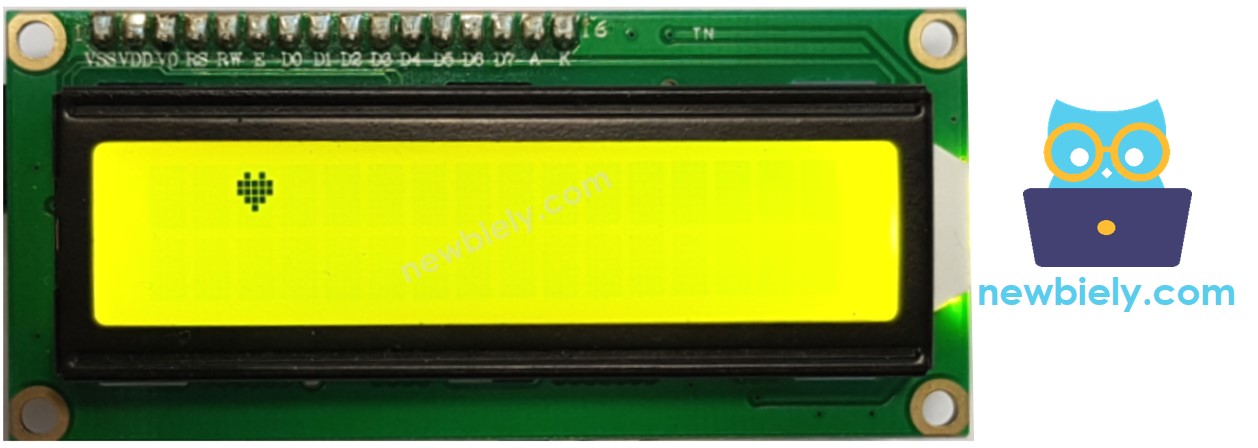
Multiple custom characters
We can make up to 8 special characters (numbered from 0 to 7). The example below shows how to create and display three characters.
Result displayed on liquid-crystal display (LCD):
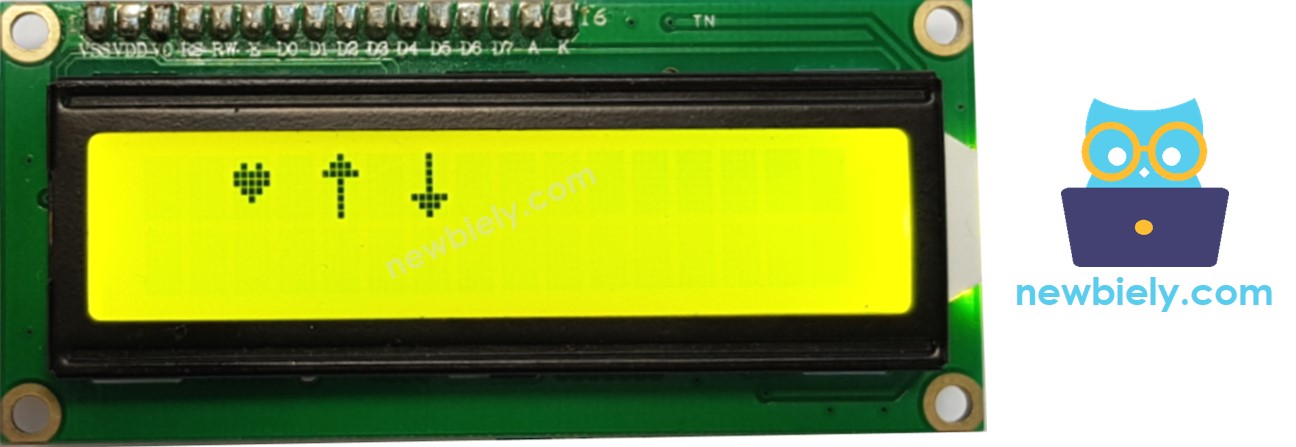
Summary: how to use custom character on LCD
- Use the character generator above to make binary code for your special character.
- Write down the binary code for your character (copy from the previous step).
- Make a personalized character and place it at a number between 0 and 7 in the setup() function.
- Display a special character on the LCD whenever you want, either in the setup() or loop() function.
Other functions
Insert the following functions into the loop() function one by one, adding a delay(5000) after each function.
- Easy-to-read LCD display
- Place the cursor in the top-left corner of the screen.
- Position the cursor at a specific place (column, row).
- Show the LCD screen cursor.
- Turns off the LCD screen cursor.
- Show the flashing LCD cursor.
- Stops the flashing LCD cursor.
- Find additional information at LiquidCrystal Library Reference.
Troubleshooting on LCD I2C
If the text does not appear on the LCD I2C, please check these issues:
- Change the LCD screen brightness by turning the potentiometer on its back.
- The I2_SELF CONT presence of the LCD might vary with different makers. It usually is 0x27 or 0x3F. Test these addresses individually. If neither works, use the following code to discover the correct I2C address.
The outcome displayed on the Serial Monitor: