Arduino UNO R4 - TM1637 4-Digit 7-Segment Display
You need a standard 4-digit 7-segment display for projects like clocks, timers, and counters. Typically, this requires 12 connections. However, the TM1637 module simplifies this by needing only 4 connections: 2 for power and 2 to control the segments.
This guide won't go into detailed hardware explanations. We'll focus on how to connect a 4-digit 7-segment display to the Arduino UNO R4 and how to program it to show what we want.
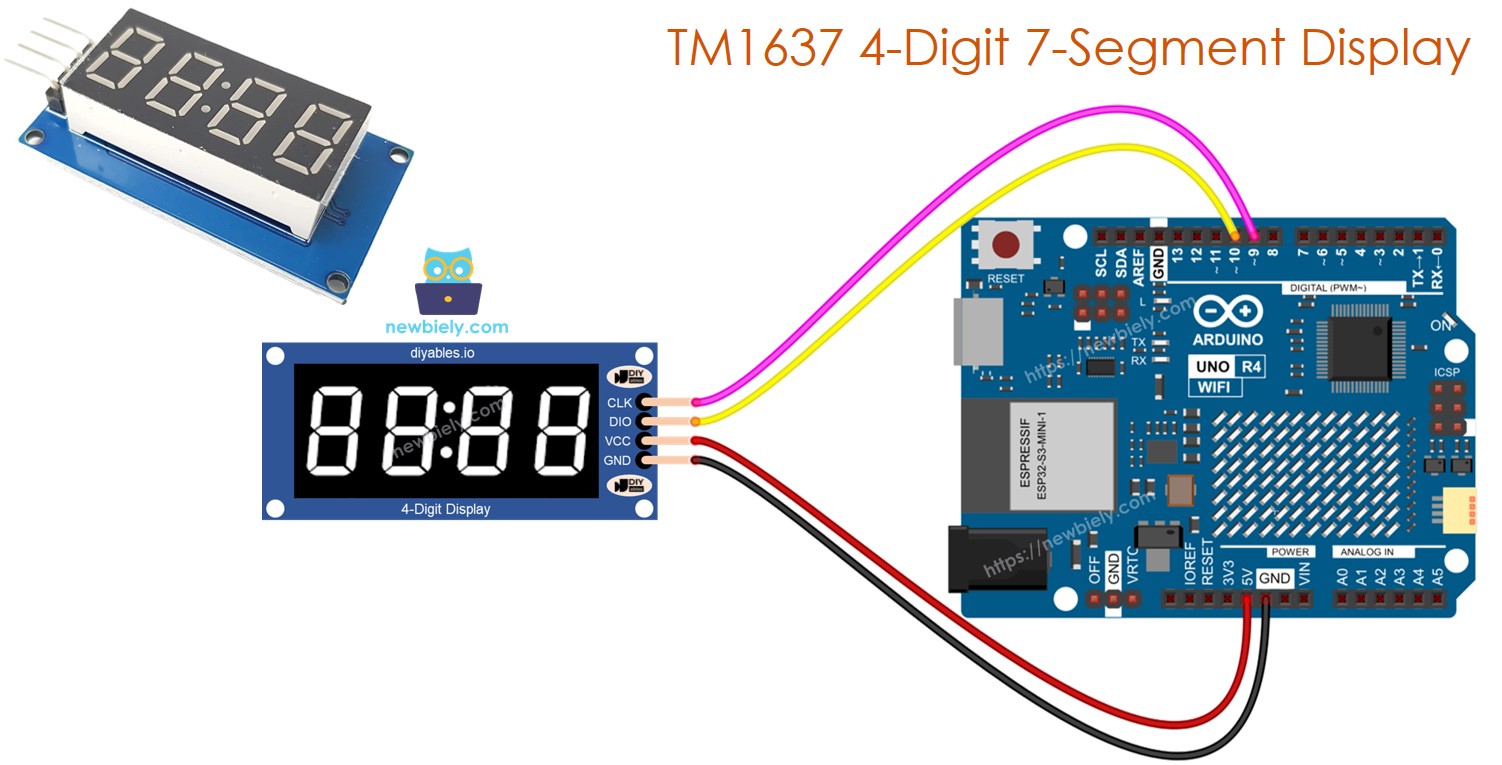
This guide will use a colon-separated 4-digit 7-segment display module. If you need to show decimal numbers, please use the 74HC30 4-digit 7-segment Display Module.
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of TM1637 4-digit 7-segment Display
A TM1637 module usually has four 7-segment LEDs and a colon-shaped LED between them. It is great for showing time in hours and minutes, minutes and seconds, or the scores of two teams.
Pinout
The TM1636 4-digit 7-segment display module has four pins:
- CLK pin: This is a clock input pin. You can connect it to any digital pin on the Arduino UNO R4.
- DIO pin: This is a Data Input/Output pin. Connect it to any digital pin on the Arduino UNO R4.
- VCC pin: This pin provides power to the module. Connect it between the 3.3V and 5V power supply.
- GND pin: This is a ground pin. Connect it to the ground.
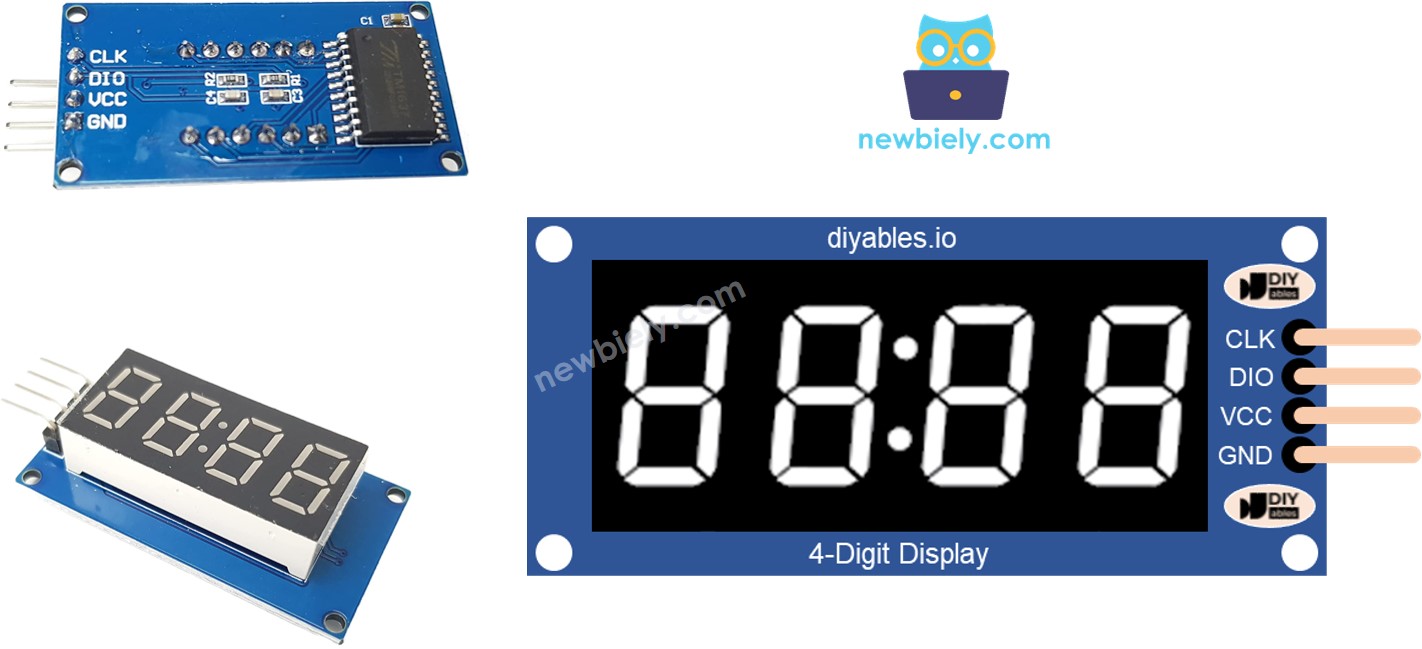
Wiring Diagram
To connect a TM1637 to an Arduino UNO R4, use four wires: two for power and two to control the display. Power the module using the 5-volt output from the Arduino UNO R4. Connect the CLK and DIO pins to any digital pins on the Arduino UNO R4, like pins 2 and 3. If you use different pins, remember to update the pin numbers in the code.
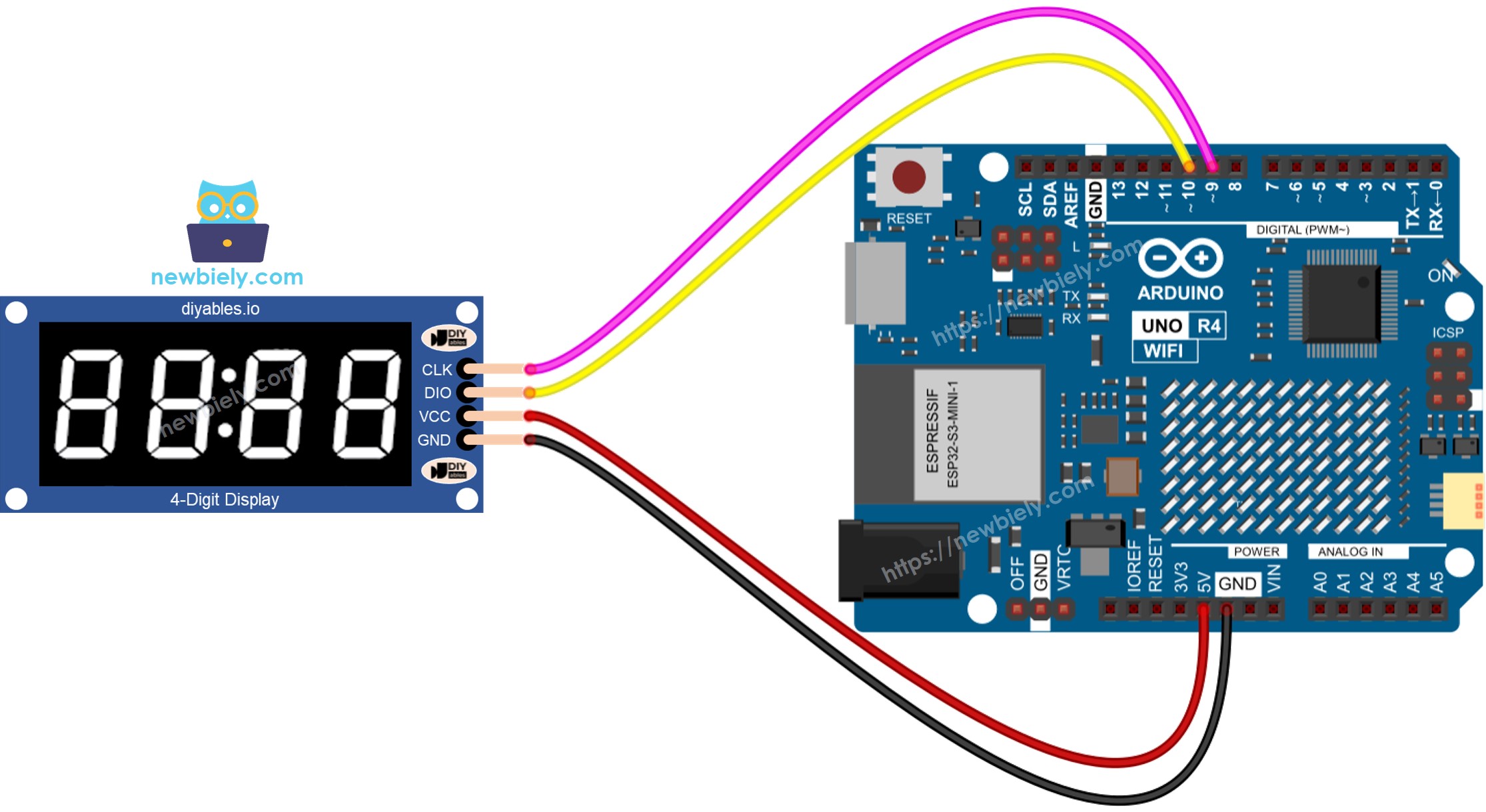
This image is created using Fritzing. Click to enlarge image
See The best way to supply power to the Arduino Uno R4 and other components.
Library Installation
To easily use the TM1637 4-digit 7-segment Display, you must install the TM1637Display library by Avishay Orpaz. Here are the steps to install the library:
- Go to the Libraries icon on the left side of the Arduino IDE.
- Type “TM1637” in the search box and look for the TM1637Display library by Avishay Orpaz.
- Press the Install button.
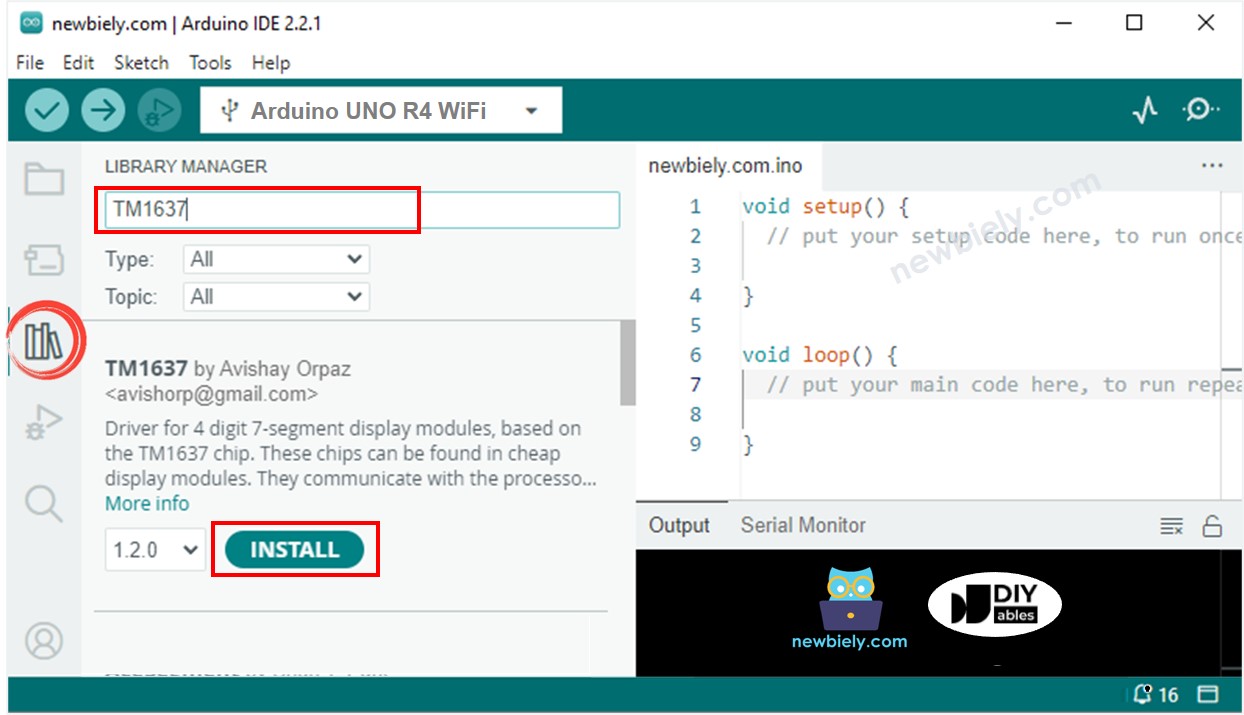
How To Program For TM1637 4-digit 7-segment using Arduino UNO R4
- Include the library
- Set the Arduino UNO R4 to connect to the display module through pins D9 for CLK and D10 for DIO.
- Make a display item called TM1637Display.
- You can show a number, a number with a decimal point, a negative number, or a letter. If using a letter, specify its shape. Here are examples for each case:
- To display a number, refer to the following examples. The symbol '_' stands for a digit that is not shown.
- Show the number with a colon or a dot.
Arduino UNO R4 Code
Detailed Instructions
Follow these instructions step by step:
- If this is your first time using the Arduino Uno R4 WiFi/Minima, refer to the tutorial on setting up the environment for Arduino Uno R4 WiFi/Minima in the Arduino IDE.
- Connect TM1637 4-digit 7-segment Display to Arduino UNO R4 according to the provided diagram.
- Connect the Arduino Uno R4 board to your computer using a USB cable.
- Launch the Arduino IDE on your computer.
- Select the appropriate Arduino Uno R4 board (e.g., Arduino Uno R4 WiFi) and COM port.
- Copy the code above and open it in Arduino IDE
- Click the Upload button in Arduino IDE to upload the code to Arduino UNO R4
- Observe the states of the 7-segment display