Arduino UNO R4 - Ultrasonic Sensor - OLED
In this guide, we will learn how to measure distance using an ultrasonic sensor and display it on an OLED screen.
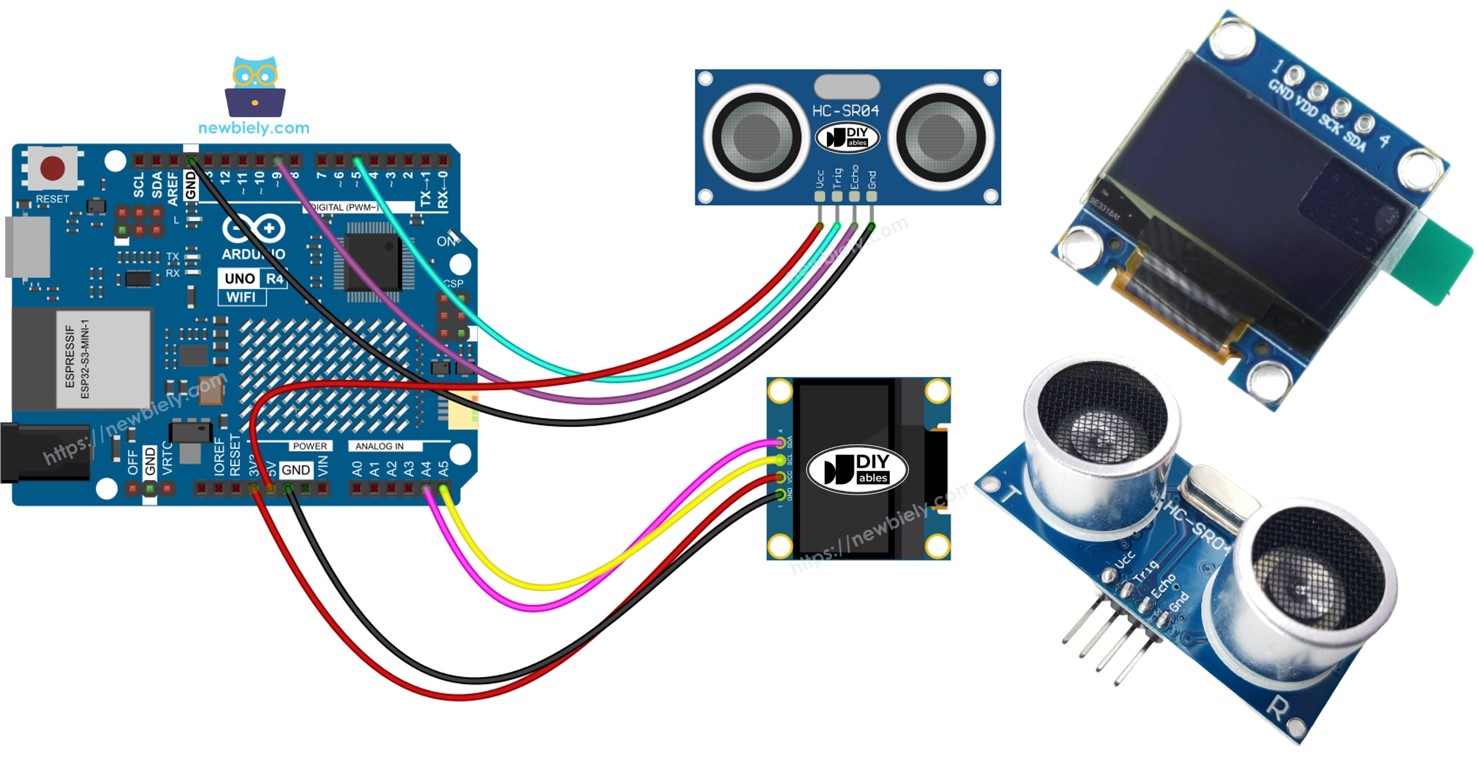
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Disclosure: Some of the links provided in this section are Amazon affiliate links. We may receive a commission for any purchases made through these links at no additional cost to you.
Additionally, some of these links are for products from our own brand, DIYables .
Additionally, some of these links are for products from our own brand, DIYables .
Overview of OLED and Ultrasonic Sensor
Learn about OLED and Ultrasonic Sensor (pinout, functions, programming) in these tutorials:
- Arduino UNO R4 - OLED tutorial
- Arduino UNO R4 - Ultrasonic Sensor tutorial
Wiring Diagram
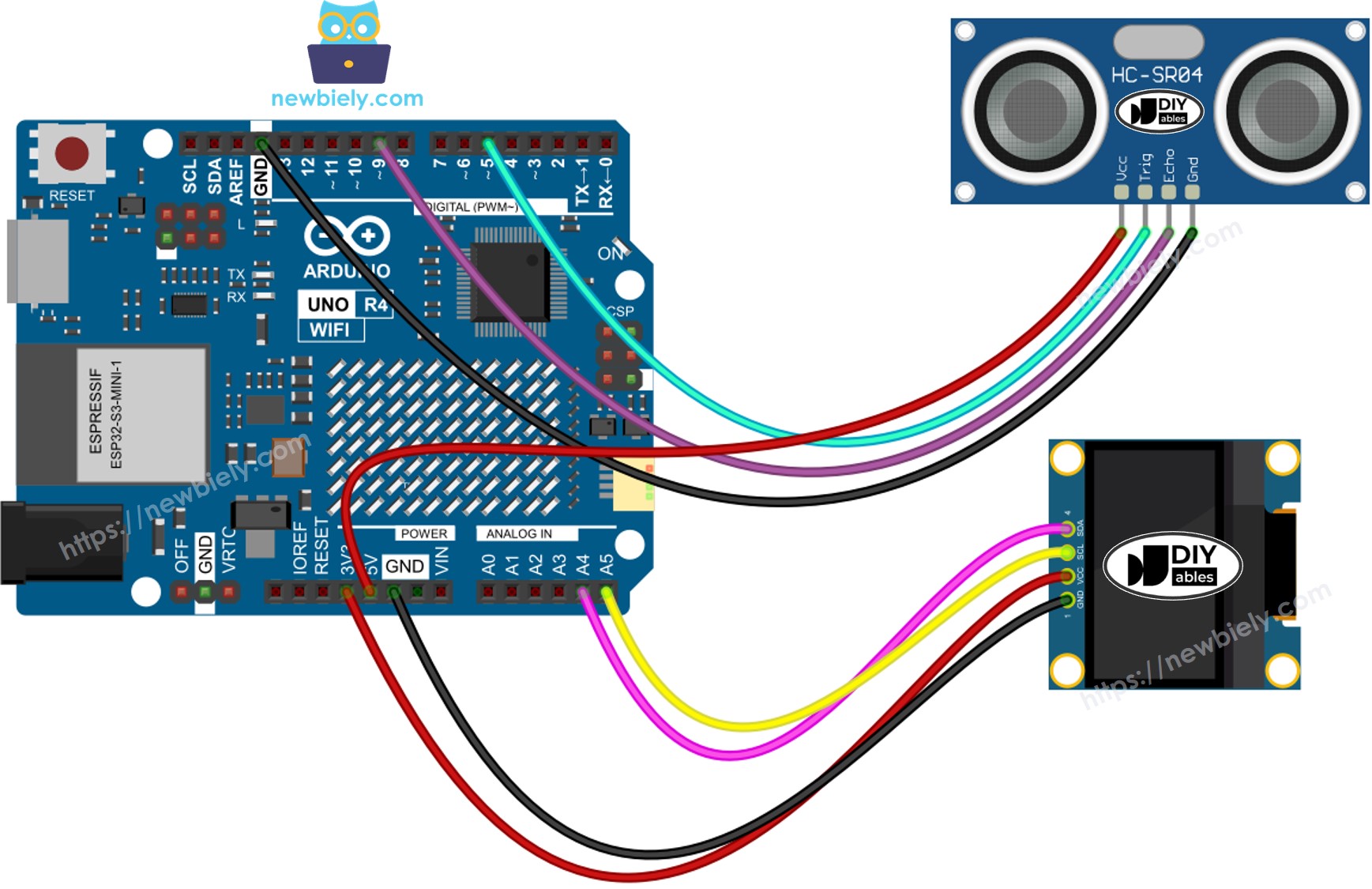
This image is created using Fritzing. Click to enlarge image
See The best way to supply power to the Arduino Uno R4 and other components.
Arduino UNO R4 Code - Ultrasonic Sensor - OLED
/*
* This Arduino UNO R4 code was developed by newbiely.com
*
* This Arduino UNO R4 code is made available for public use without any restriction
*
* For comprehensive instructions and wiring diagrams, please visit:
* https://newbiely.com/tutorials/arduino-uno-r4/arduino-uno-r4-ultrasonic-sensor-oled
*/
#include <Wire.h>
#include <Adafruit_GFX.h>
#include <Adafruit_SSD1306.h>
#define SCREEN_WIDTH 128 // OLED display width, in pixels
#define SCREEN_HEIGHT 64 // OLED display height, in pixels
#define TRIG_PIN 5 // The Arduino UNO R4 pin connected to Ultrasonic Sensor's TRIG pin
#define ECHO_PIN 9 // The Arduino UNO R4 pin connected to Ultrasonic Sensor's ECHO pin
Adafruit_SSD1306 oled(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, -1); // create SSD1306 display object connected to I2C
String tempString;
void setup() {
Serial.begin(9600);
// initialize OLED display with address 0x3C for 128x64
if (!oled.begin(SSD1306_SWITCHCAPVCC, 0x3C)) {
Serial.println(F("SSD1306 allocation failed"));
while (true);
}
delay(2000); // wait for initializing
oled.clearDisplay(); // clear display
oled.setTextSize(2); // text size
oled.setTextColor(WHITE); // text color
oled.setCursor(0, 10); // position to display
tempString.reserve(10); // to avoid fragmenting memory when using String
}
void loop() {
// generate 10-microsecond pulse to TRIG pin
digitalWrite(TRIG_PIN, HIGH);
delayMicroseconds(10);
digitalWrite(TRIG_PIN, LOW);
// measure duration of pulse from ECHO pin
long duration_us = pulseIn(ECHO_PIN, HIGH);
// calculate the distance
float distance_cm = 0.017 * duration_us;
// print the value to Serial Monitor
Serial.print("distance: ");
Serial.print(distance_cm);
Serial.println(" cm");
tempString = String(distance_cm, 2); // two decimal places
tempString += " cm";
Serial.println(tempString); // print the temperature in Celsius to Serial Monitor
oledDisplayCenter(tempString); // display temperature on OLED
}
void oledDisplayCenter(String text) {
int16_t x1;
int16_t y1;
uint16_t width;
uint16_t height;
oled.getTextBounds(text, 0, 0, &x1, &y1, &width, &height);
// display on horizontal and vertical center
oled.clearDisplay(); // clear display
oled.setCursor((SCREEN_WIDTH - width) / 2, (SCREEN_HEIGHT - height) / 2);
oled.println(text); // text to display
oled.display();
}
Detailed Instructions
Follow these instructions step by step:
- If this is your first time using the Arduino Uno R4 WiFi/Minima, refer to the tutorial on setting up the environment for Arduino Uno R4 WiFi/Minima in the Arduino IDE.
- Connect the Arduino Uno R4 board to the ultrasonic sensor and OLED display according to the provided diagram.
- Connect the Arduino Uno R4 board to your computer using a USB cable.
- Launch the Arduino IDE on your computer.
- Select the appropriate Arduino Uno R4 board (e.g., Arduino Uno R4 WiFi) and COM port.
- Click on the Libraries icon located on the left side of the Arduino IDE.
- Type “SSD1306” in the search box, and look for the SSD1306 library created by Adafruit.
- Press the Install button to add the library.
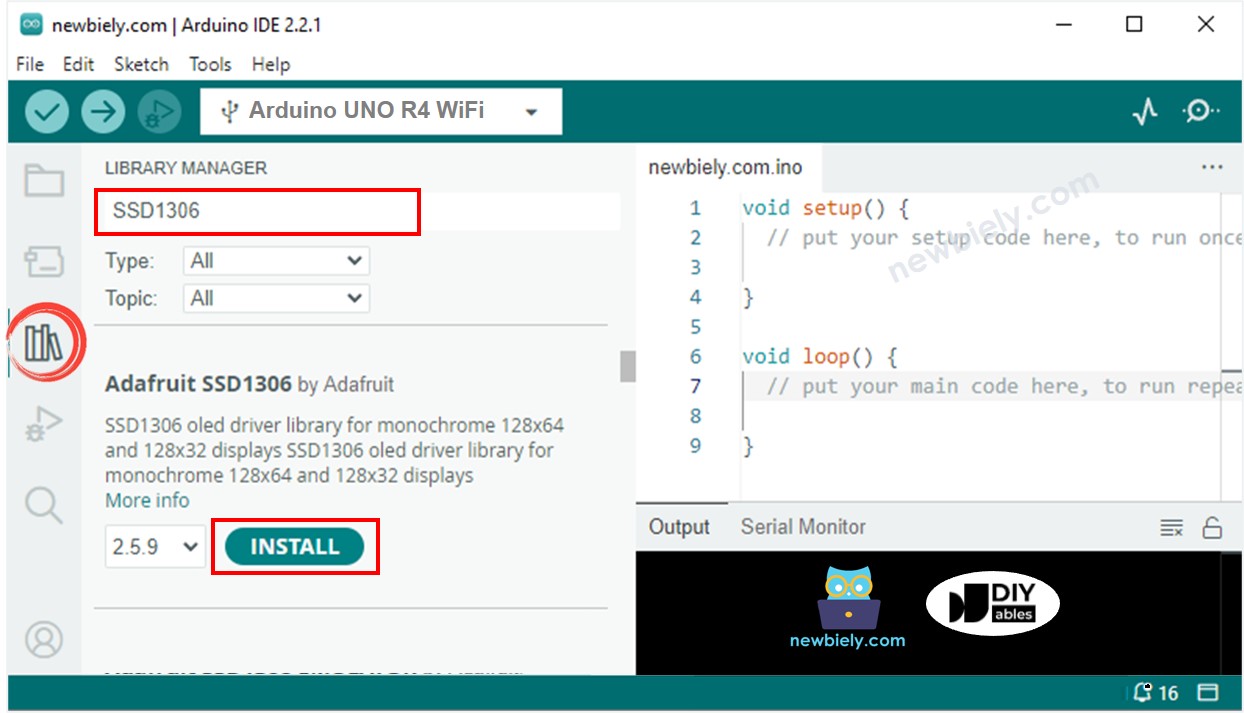
- You will need to install additional library dependencies.
- Click the Install All button to install all the required libraries.
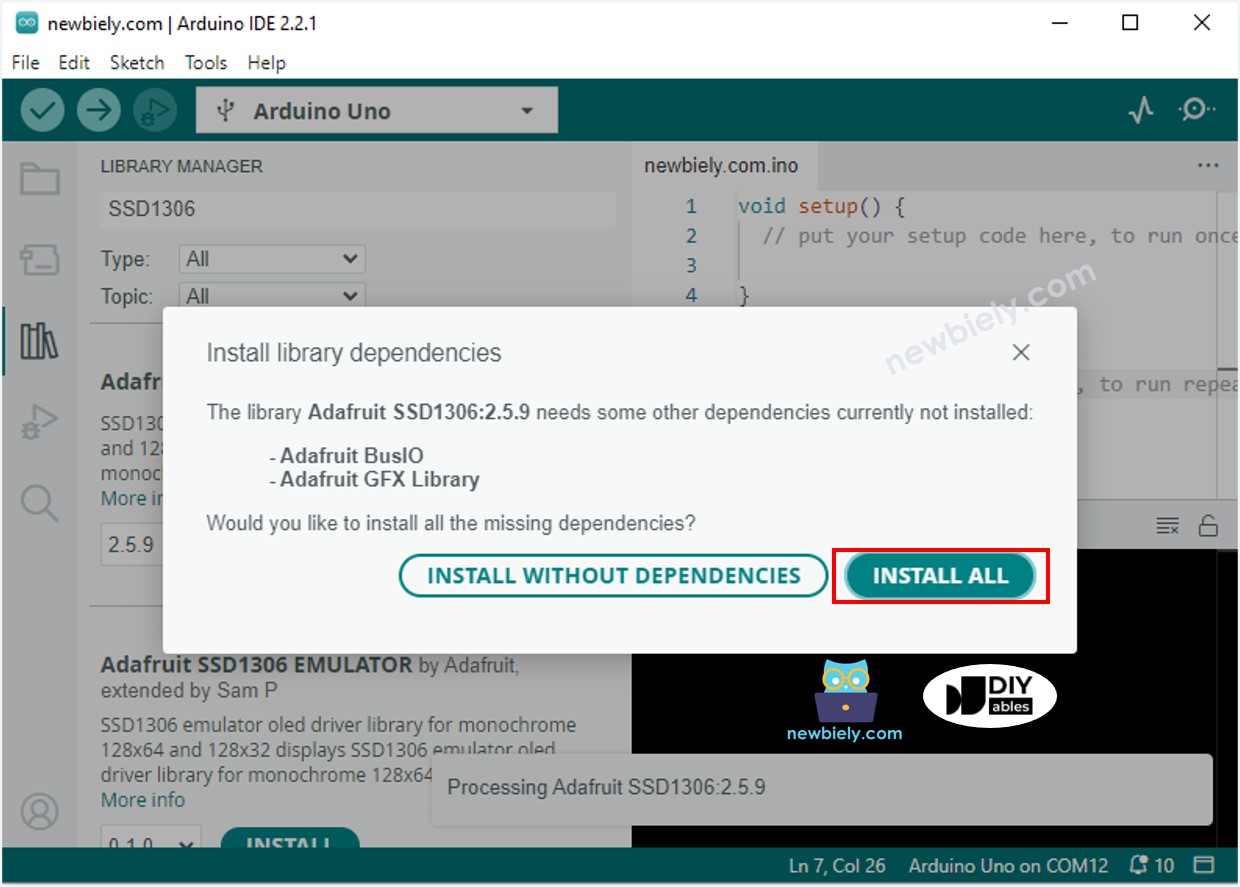
- Copy the code and open it in Arduino IDE
- Press the Upload button in Arduino IDE to send the code to Arduino UNO R4
- Wave your hand in front of the sensor
- Check the result on OLED and Serial Monitor
※ NOTE THAT:
The code centers the text both horizontally and vertically on the OLED display. For more details, see the guide on how to center text on OLED at How to vertical/horizontal center on OLED.