Arduino UNO R4 - Ultrasonic Sensor
This tutorial instructs you how to use the ultrasonic sensor and Arduino UNO R4 to measure distance to an object. In detail, we will learn:
- How to connect an ultrasonic sensor to Arduino UNO R4
- How to program Arduino to read the value from the ultrasonic sensor and convert it into distance value.
- How to eliminate noise in distance data from an ultrasonic sensor in Arduino UNO R4
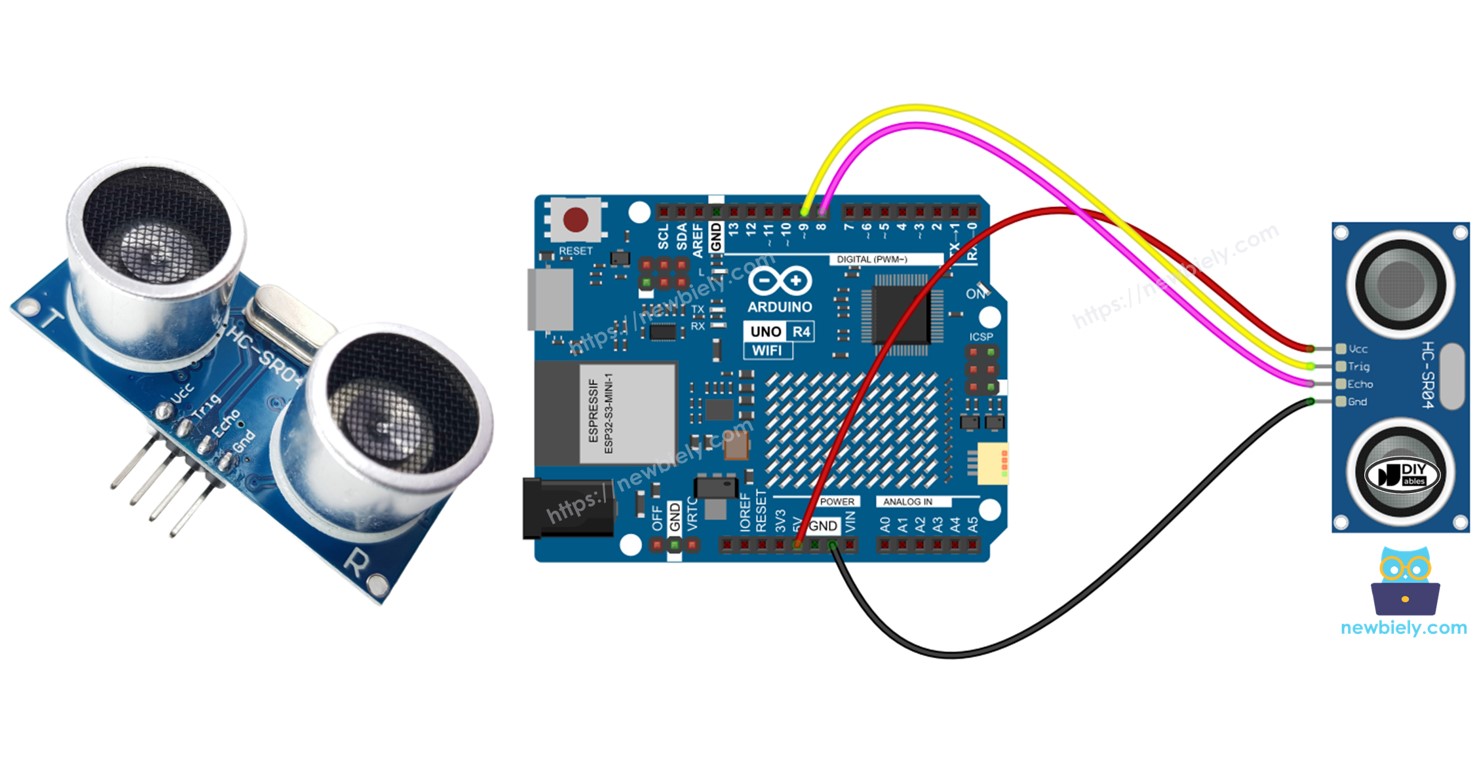
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Ultrasonic Sensor
The HC-SR04 ultrasonic sensor measures the distance to objects using sound waves. It sends out a sound wave that humans cannot hear, and then listens for the echo when the sound wave bounces back from an object. By measuring the time it takes for the sound wave to return, the sensor can calculate how far away the object is.
Pinout
The ultrasonic sensor HC-SR04 comes with four pins:
- VCC pin: Connect this pin to VCC (5V).
- GND pin: Connect this pin to GND (0V).
- TRIG pin: Connect this pin to Arduino UNO R4 to send control signals (pulses).
- ECHO pin: This pin sends signals (pulses) back to Arduino UNO R4. The Arduino UNO R4 then calculates the duration of these pulses to determine distance.
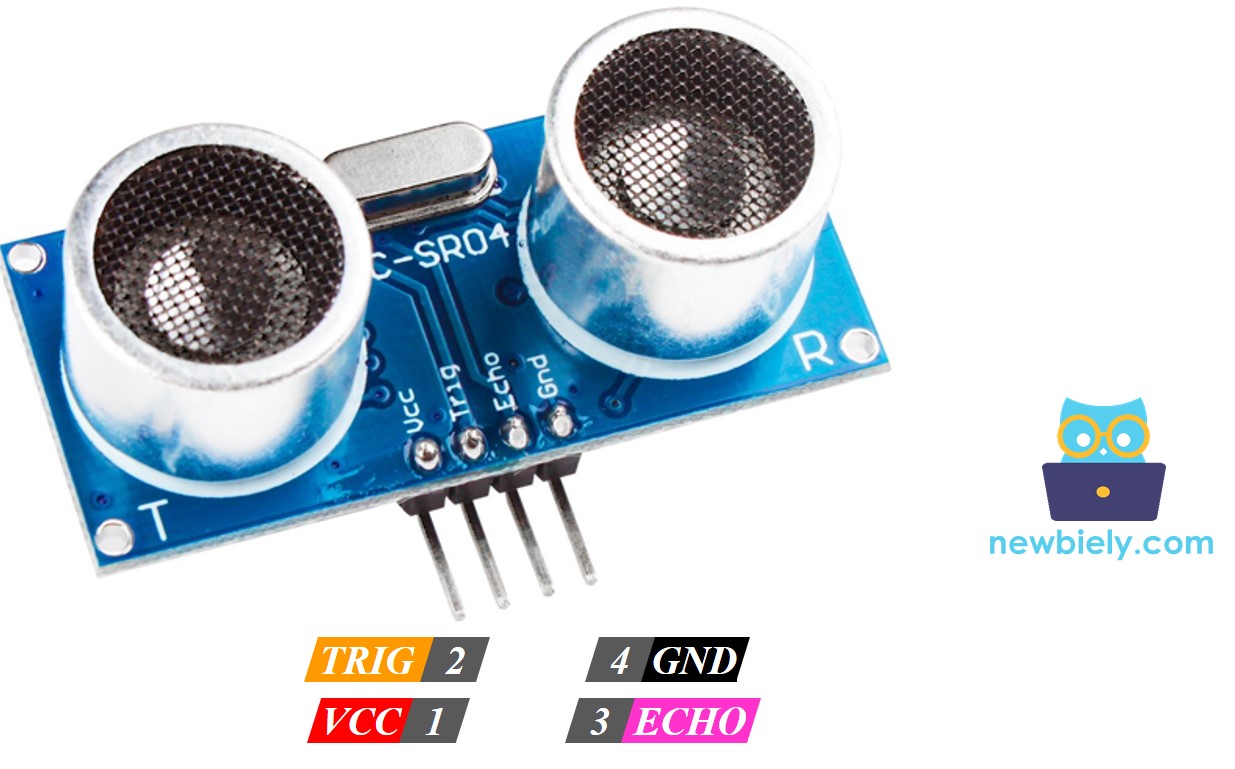
Wiring Diagram
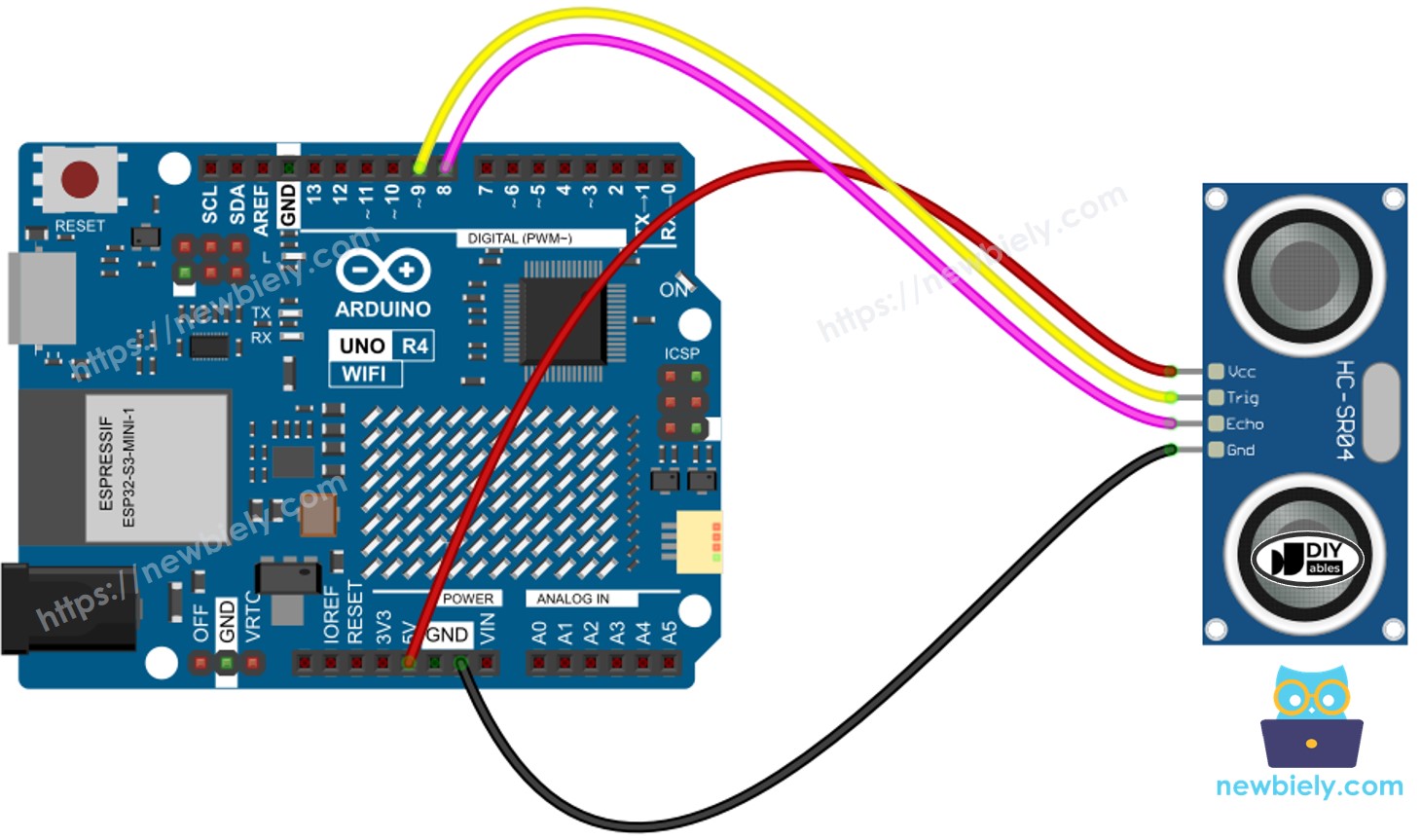
This image is created using Fritzing. Click to enlarge image
See The best way to supply power to the Arduino Uno R4 and other components.
Arduino UNO R4 Code
Detailed Instructions
Follow these instructions step by step:
- If this is your first time using the Arduino Uno R4 WiFi/Minima, refer to the tutorial on setting up the environment for Arduino Uno R4 WiFi/Minima in the Arduino IDE.
- Wire the components according to the provided diagram.
- Connect the Arduino Uno R4 board to your computer using a USB cable.
- Launch the Arduino IDE on your computer.
- Select the appropriate Arduino Uno R4 board (e.g., Arduino Uno R4 WiFi) and COM port.
- Copy the code above and open it with Arduino IDE
- Click the Upload button in Arduino IDE to send the code to Arduino UNO R4
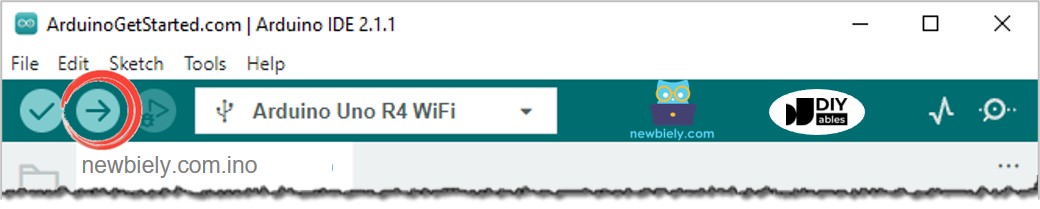
- Open the Serial Monitor.
- Wave your hand in front of the ultrasonic sensor.
- Check the distance between your hand and the sensor on the Serial Monitor.
Code Explanation
The explanation is in the comments of the Arduino code above.
How to Filter Noise from Distance Measurements of Ultrasonic Sensor
The reading from the ultrasonic sensor includes noise. In some cases, this noisy data can lead to incorrect functioning. We can eliminate the noise using this method:
- Take several measurements and save them in an array.
- Sort the array from smallest to largest.
- Remove noise from the data:
- Disregard the smallest values as they are noise.
- Disregard the largest values as they are noise.
- Calculate the average using the remaining middle values.
- Ignore the five smallest samples and the five largest samples as they are seen as noise. Calculate the average of the ten middle samples, from the 5th to the 14th.
The example code below takes 20 measurements.
Video Tutorial
Ultrasonic Sensor Applications
- Avoiding Collisions
- Detecting Fullness
- Measuring Level
- Detecting Closeness