Arduino UNO R4 - DHT11 - LCD
We will learn to program the Arduino UNO R4 to read temperature and humidity from the DHT11 module and show them on an LCD I2C display.
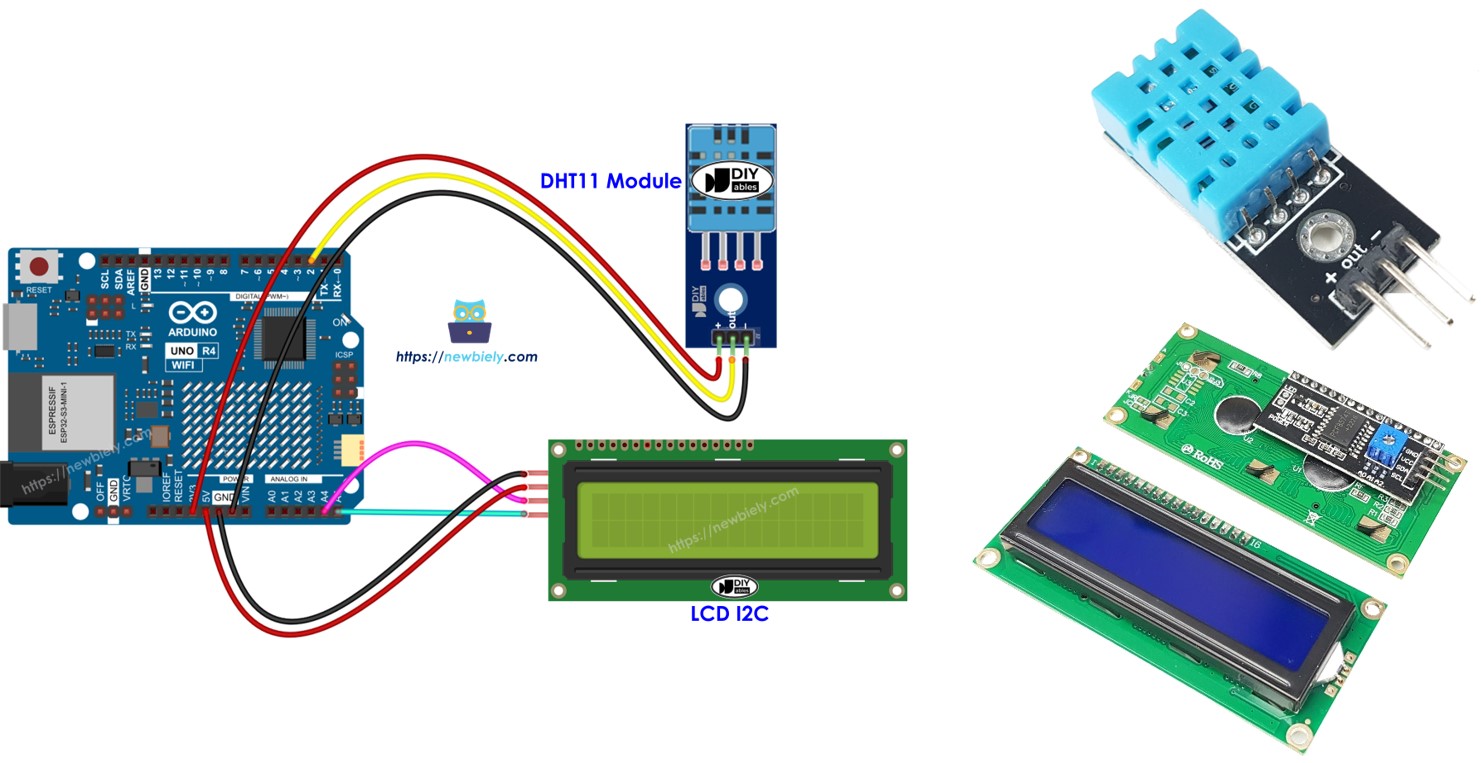
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Disclosure: Some of the links provided in this section are Amazon affiliate links. We may receive a commission for any purchases made through these links at no additional cost to you.
Additionally, some of these links are for products from our own brand, DIYables .
Additionally, some of these links are for products from our own brand, DIYables .
Overview of DHT11 and LCD
Learn about the DHT11 sensor and LCD, including their pinout, functions, and programming in these tutorials:
Wiring Diagram
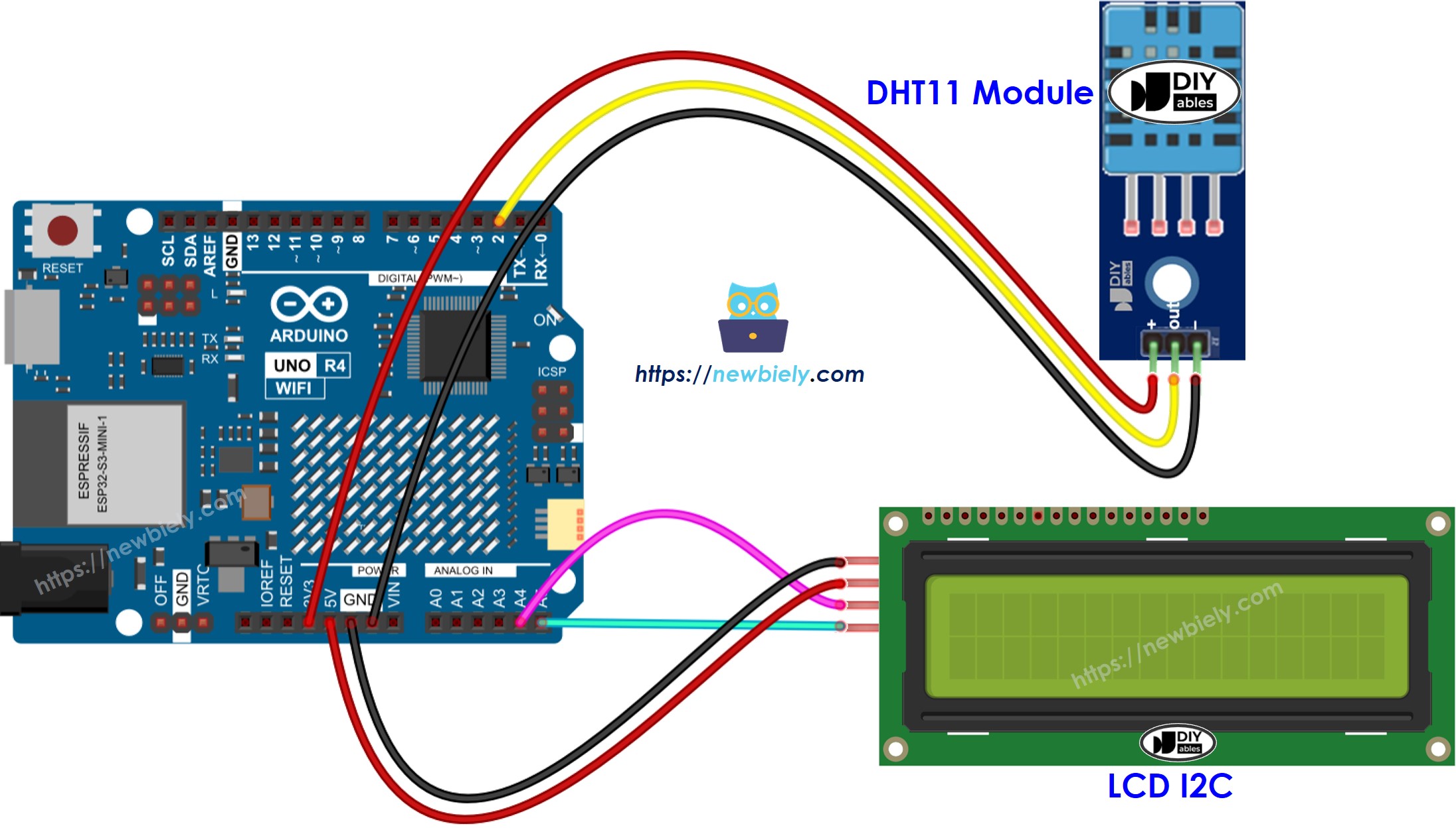
This image is created using Fritzing. Click to enlarge image
See The best way to supply power to the Arduino Uno R4 and other components.
Arduino UNO R4 Code - DHT11 Sensor - LCD I2C
/*
* This Arduino UNO R4 code was developed by newbiely.com
*
* This Arduino UNO R4 code is made available for public use without any restriction
*
* For comprehensive instructions and wiring diagrams, please visit:
* https://newbiely.com/tutorials/arduino-uno-r4/arduino-uno-r4-dht11-lcd
*/
#include <LiquidCrystal_I2C.h>
#include <DHT.h>
#define DHT11_PIN 2 // The Arduino Uno R4 pin connected to DHT11 module
LiquidCrystal_I2C lcd(0x27, 16, 2); // I2C address 0x27 (from DIYables LCD), 16 column and 2 rows
DHT dht11(DHT11_PIN, DHT11);
void setup()
{
dht11.begin(); // initialize the sensor
lcd.init(); // initialize the lcd
lcd.backlight(); // open the backlight
}
void loop()
{
delay(2000); // wait a few seconds between measurements
float humi = dht11.readHumidity(); // read humidity
float tempC = dht11.readTemperature(); // read temperature
lcd.clear();
// check if any reads failed
if (isnan(humi) || isnan(tempC)) {
lcd.setCursor(0, 0);
lcd.print("Failed");
} else {
lcd.setCursor(0, 0); // start to print at the first row
lcd.print("Temp: ");
lcd.print(tempC); // print the temperature
lcd.print((char)223); // print ° character
lcd.print("C");
lcd.setCursor(0, 1); // start to print at the second row
lcd.print("Humi: ");
lcd.print(humi); // print the humidity
lcd.print("%");
}
}
※ NOTE THAT:
The LCD I2C address can differ based on the manufacturer. In our code, we used the address 0x27 as specified by the manufacturer DIYables.
Detailed Instructions
Follow these instructions step by step:
- If this is your first time using the Arduino Uno R4 WiFi/Minima, refer to the tutorial on setting up the environment for Arduino Uno R4 WiFi/Minima in the Arduino IDE.
- Connect the Arduino Uno R4 to the DHT11 module and LCD I2C according to the provided diagram.
- Connect the Arduino Uno R4 board to your computer using a USB cable.
- Launch the Arduino IDE on your computer.
- Select the appropriate Arduino Uno R4 board (e.g., Arduino Uno R4 WiFi) and COM port.
- Click on the Libraries icon on the left sidebar of the Arduino IDE.
- Type "DHT" in the search box and locate the DHT sensor library from Adafruit.
- Press the Install button to add the library.
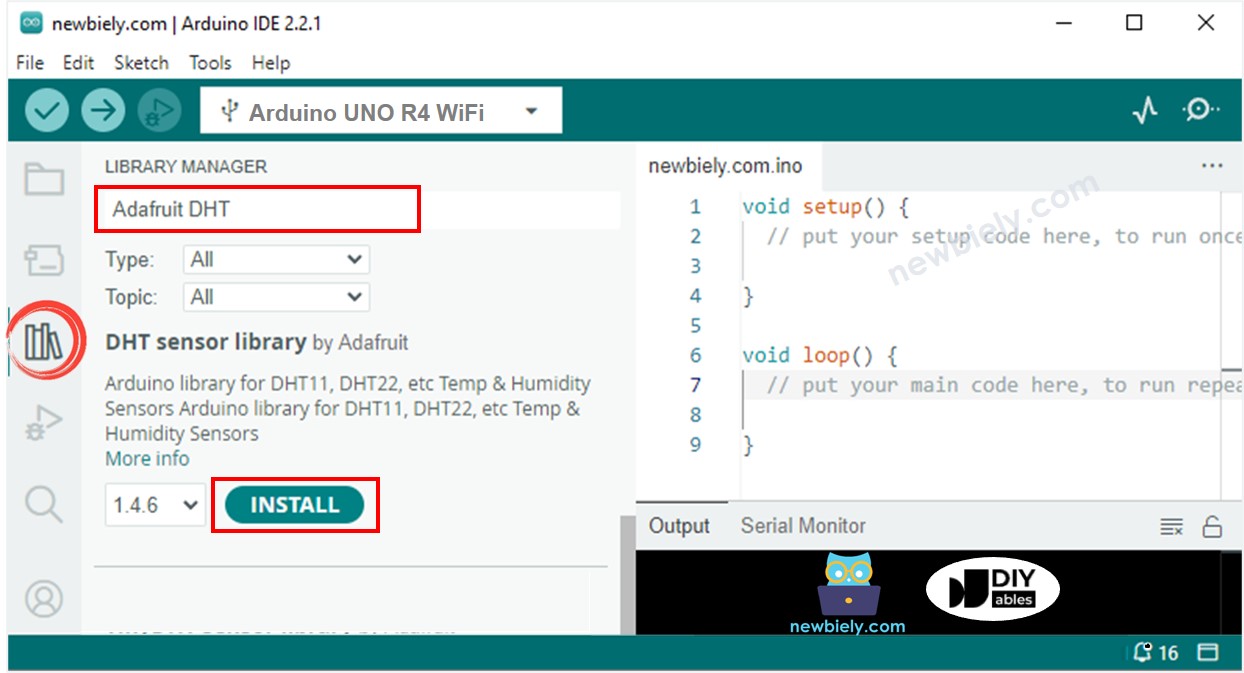
- You will need to install additional library dependencies.
- Click the Install All button to install all library dependencies.
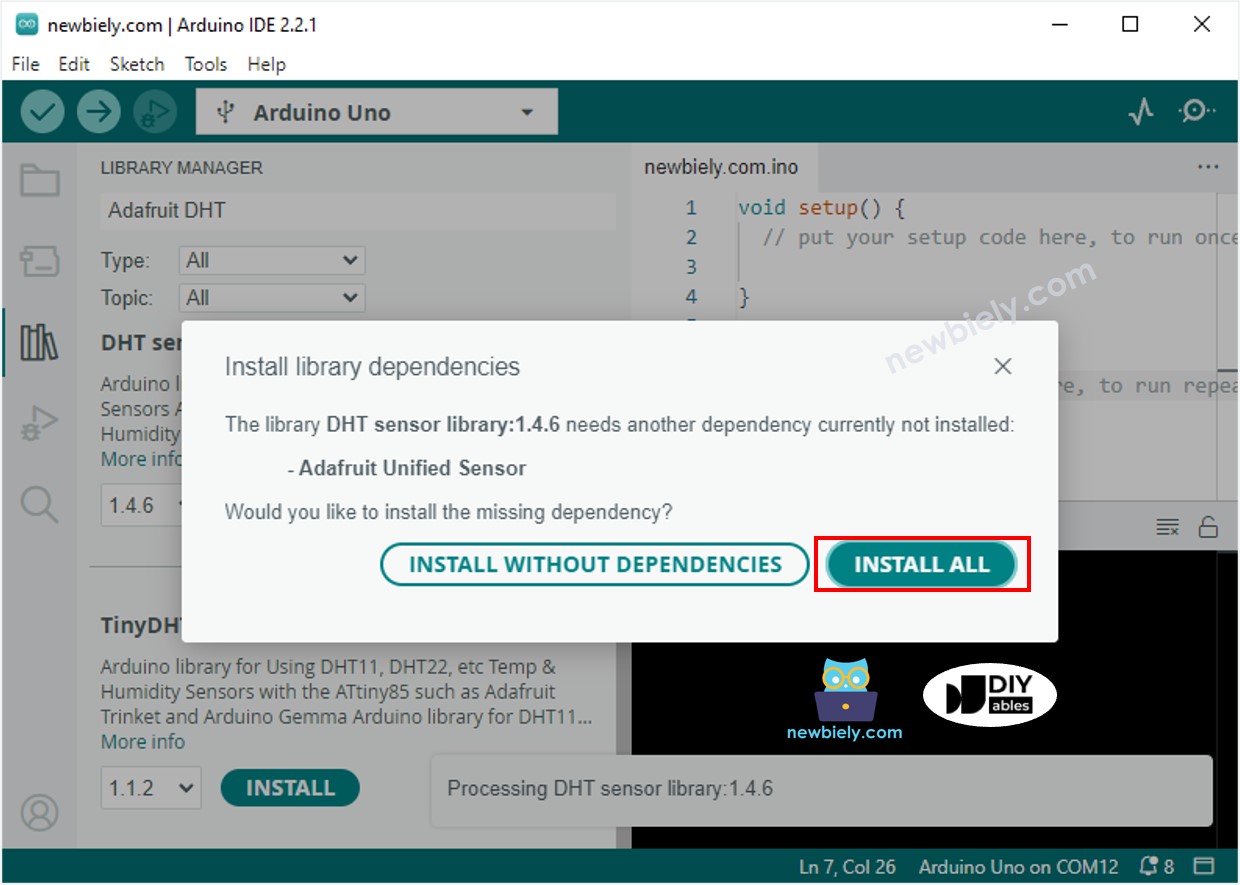
- Look for "LiquidCrystal I2C," then choose the LiquidCrystal_I2C library by Frank de Brabander.
- Click the Install button to add the LiquidCrystal_I2C library.
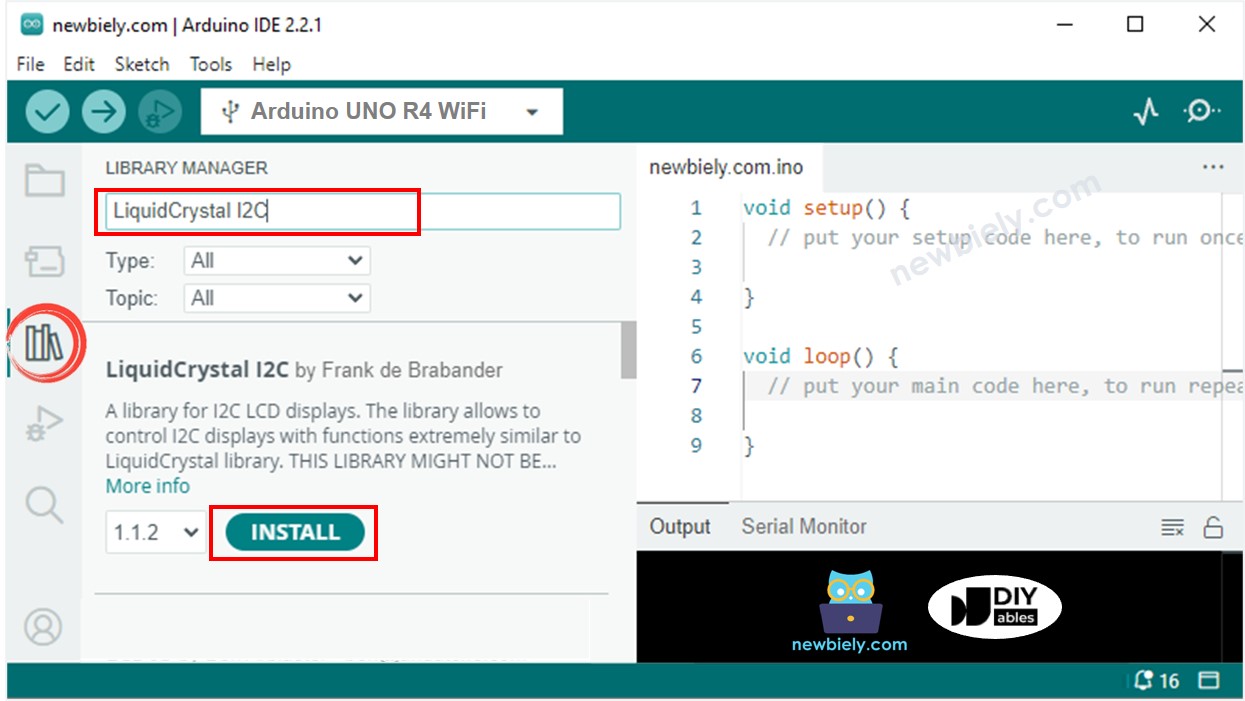
- Copy the code above and open it with Arduino IDE
- Click the Upload button in Arduino IDE to send the code to Arduino UNO R4
- Change the temperature around the sensor to be hotter or colder
- Check the result on the LCD screen
If the LCD shows nothing, please check Troubleshooting on LCD I2C.