Arduino UNO R4 - Keypad 1x4
In this guide, we will learn how to use a 1x4 keypad with an Arduino UNO R4. We will cover:
- How to link a 1x4 keypad to an Arduino UNO R4.
- How to set up an Arduino UNO R4 to detect keys pressed on a 1x4 keypad.
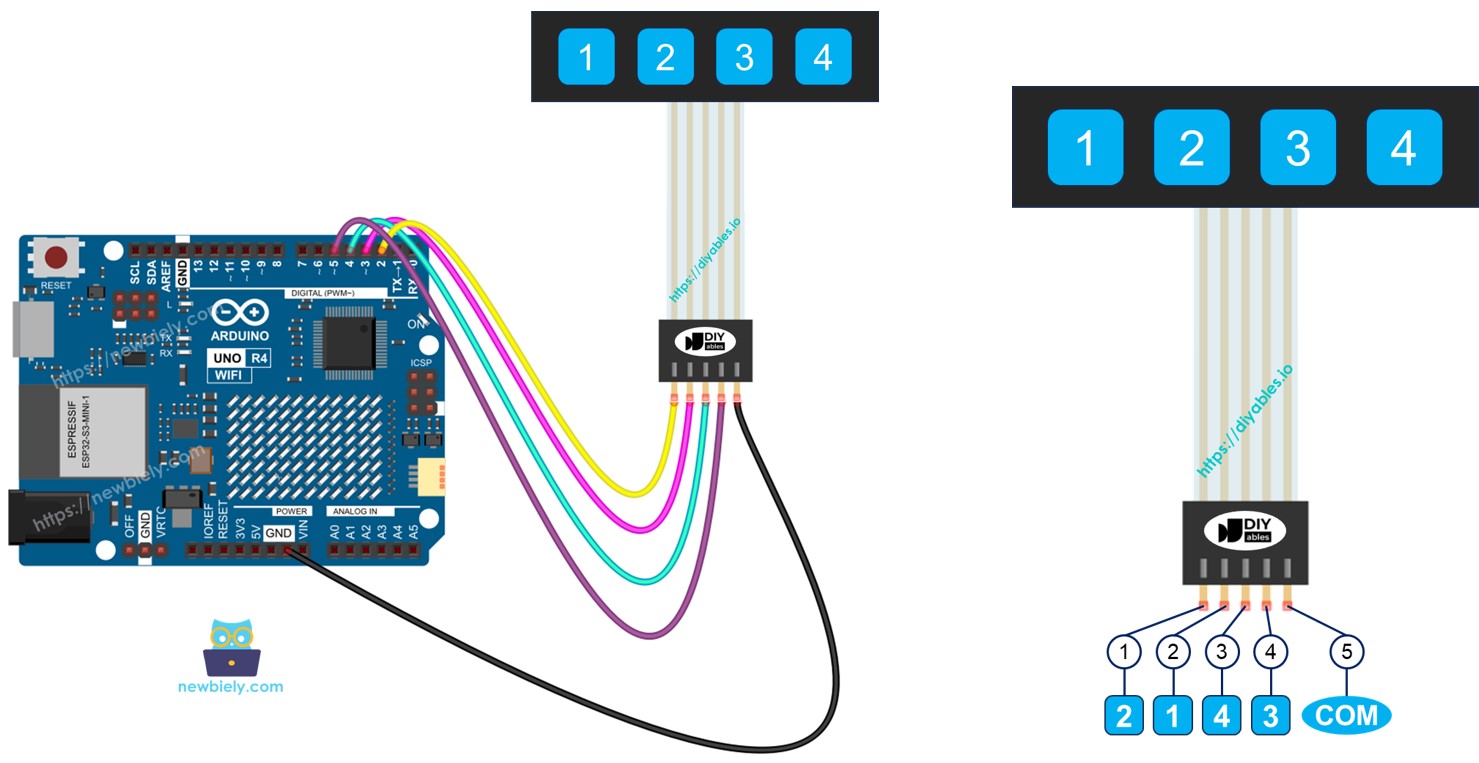
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Keypad 1x4
A 1x4 keypad has four buttons in a row. It is used for entering codes, moving through menus, or controlling interfaces in different projects.
Pinout
The 1x4 keypad has 5 pins. These pins are not arranged in the same order as the labels on the keys.
- Pin 1 links to key 2.
- Pin 2 links to key 1.
- Pin 3 links to key 4.
- Pin 4 links to key 3.
- Pin 5 connects commonly to all keys.
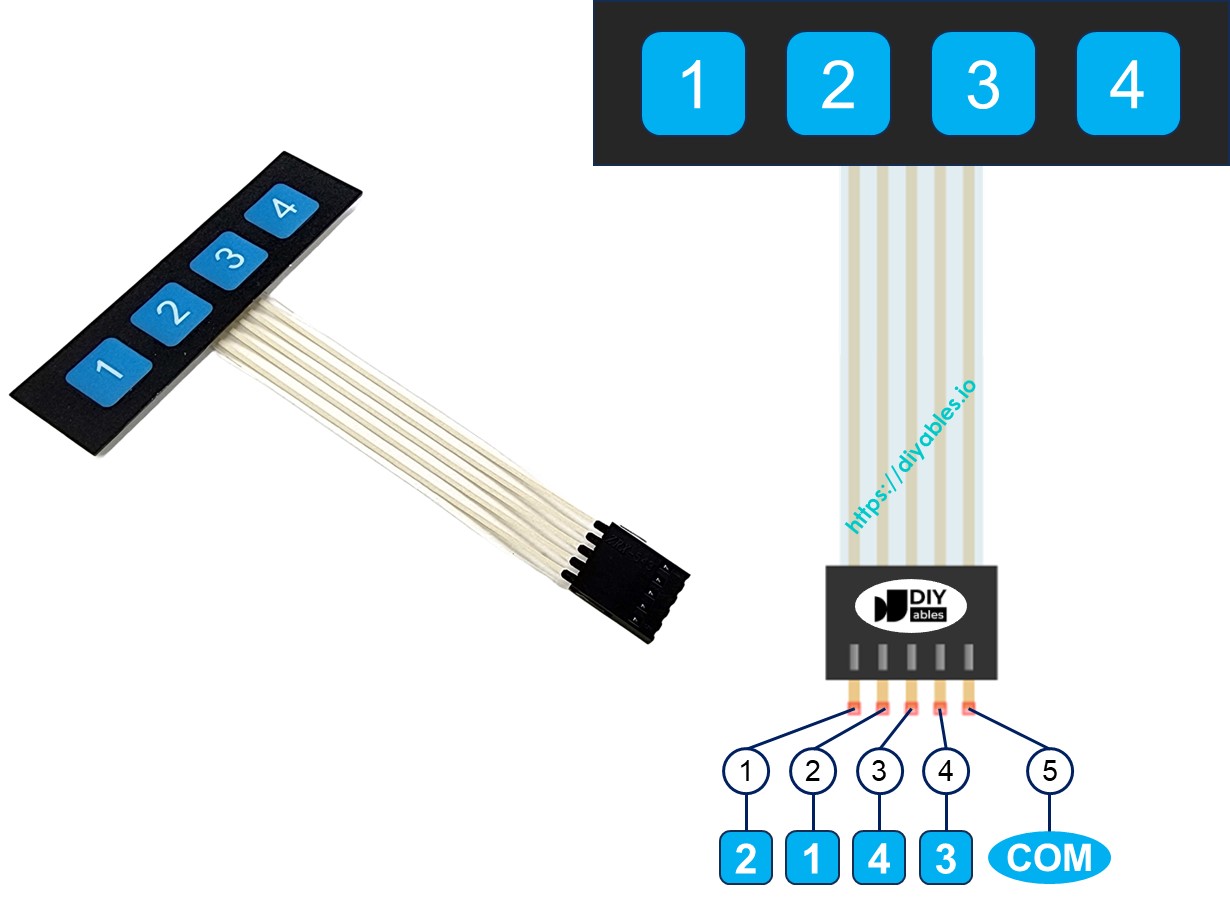
Wiring Diagram
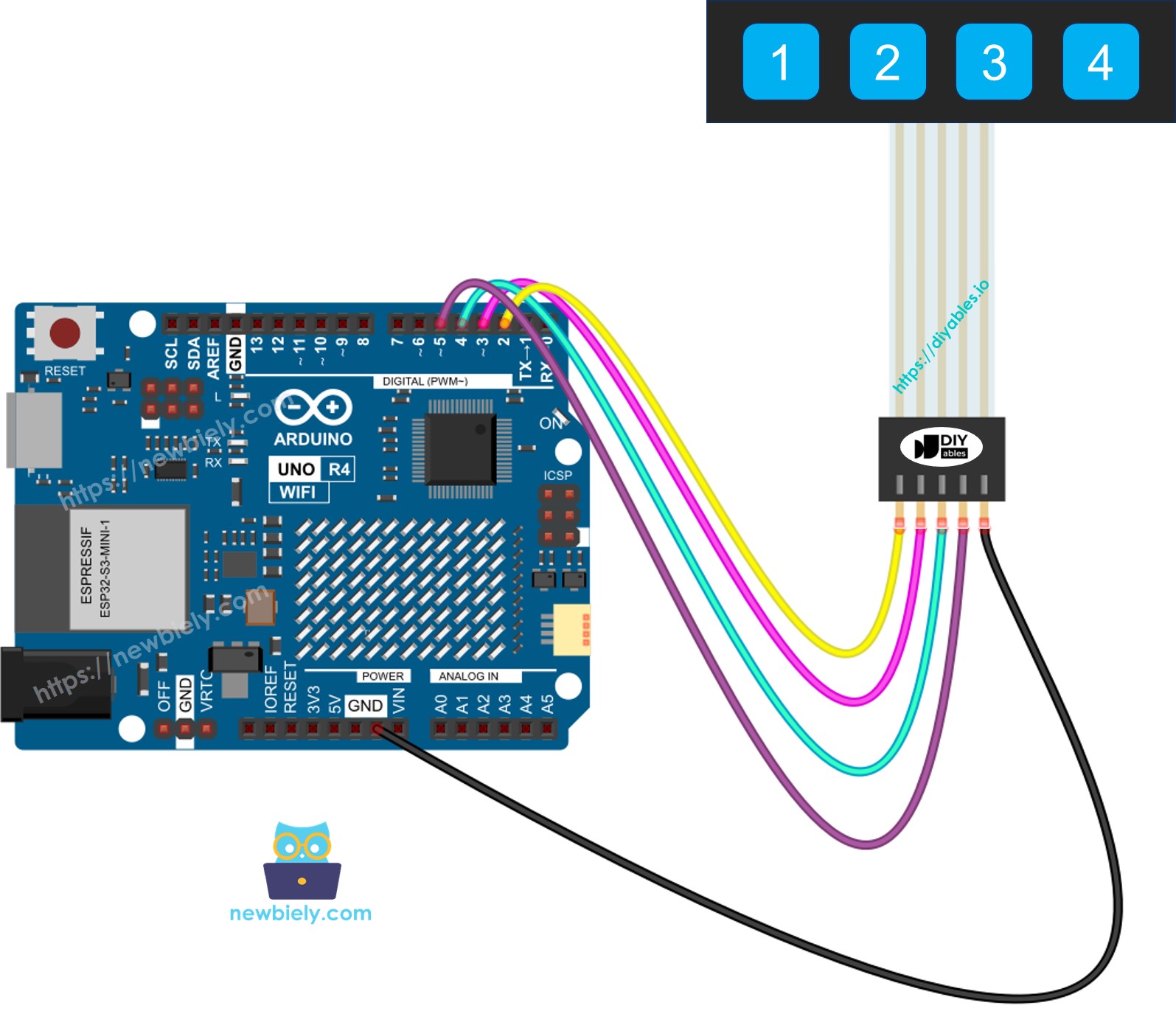
This image is created using Fritzing. Click to enlarge image
See The best way to supply power to the Arduino Uno R4 and other components.
Arduino UNO R4 Code
Each key on the 1x4 keypad works like a button. We can use the digitalRead() function to check how each key is pressed. But like with any button, we face the problem of bouncing - where a single press might look like many presses. To solve this, we must debounce each key. Debouncing four keys without stopping other parts of the code can be hard. Luckily, the ezLink library makes it easier.
Detailed Instructions
Follow these instructions step by step:
- If this is your first time using the Arduino Uno R4 WiFi/Minima, refer to the tutorial on setting up the environment for Arduino Uno R4 WiFi/Minima in the Arduino IDE.
- Connect Arduino UNO R4 to a 1x4 keypad according to the provided diagram.
- Connect the Arduino Uno R4 board to your computer using a USB cable.
- Launch the Arduino IDE on your computer.
- Select the appropriate Arduino Uno R4 board (e.g., Arduino Uno R4 WiFi) and COM port..
- Click on the Libraries icon found on the left side of the Arduino IDE.
- Type "ezButton" in the search box, and locate the button library provided by "ArduinoGetStarted.com".
- Press the Install button to add the ezButton library.
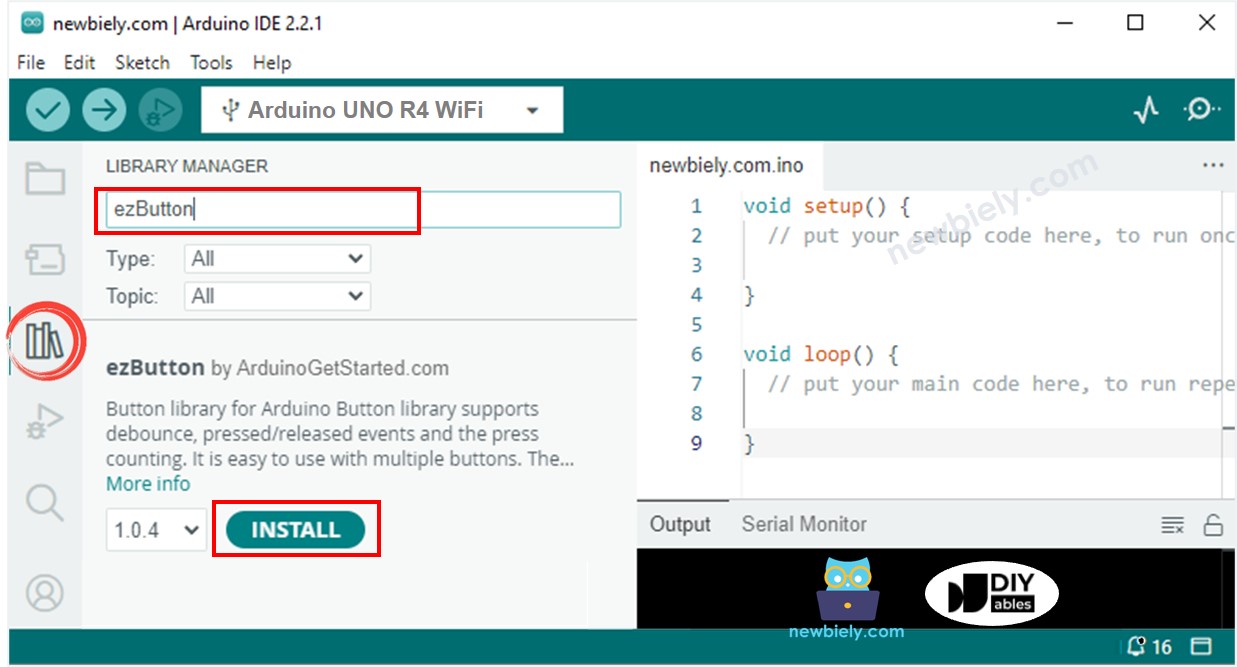
- Copy the code and open it using Arduino IDE
- Click the Upload button in Arduino IDE to send the code to Arduino UNO R4
- Open Serial Monitor
- Press each key on the 1x4 keypad
- Check the results on Serial Monitor