Arduino UNO R4 - MQTT
In this tutorial, we will learn how to use the Arduino UNO R4 to send and receive data to an MQTT broker using the MQTT protocol. We'll cover the following details:
- How to connect Arduino UNO R4 with an MQTT broker
- How to program Arduino UNO R4 to send data to an MQTT broker by publishing to an MQTT topic
- How to program Arduino UNO R4 to get data by subscribing to an MQTT topic.
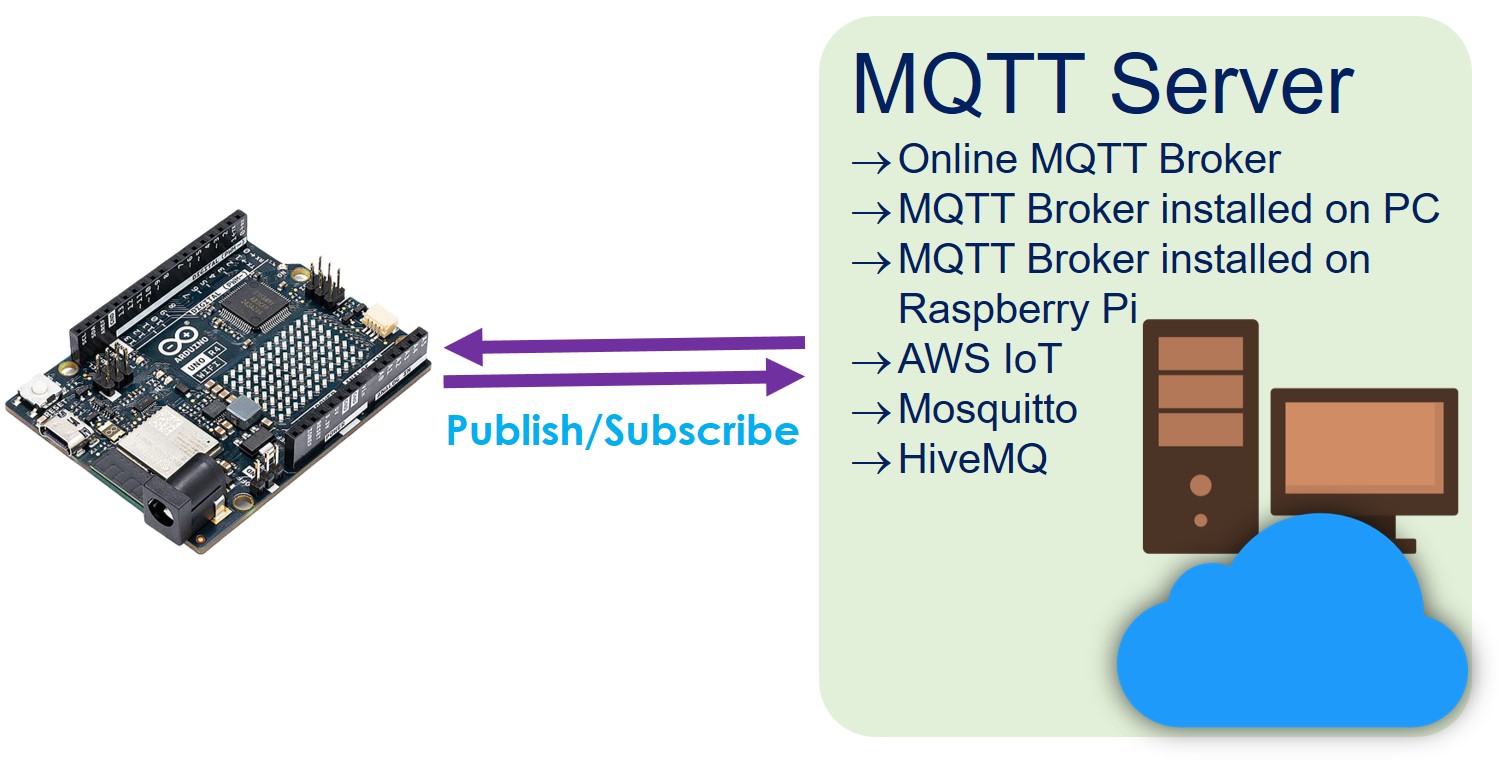
We will look into two various examples:
- Using Arduino UNO R4 with an internet MQTT broker.
- Using Arduino UNO R4 with the MQTT broker installed on your computer.
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Arduino UNO R4 and MQTT
We will learn how to program an Arduino UNO R4 to send and receive data through MQTT broker (alse called MQTT server). MQTT broker can be:
- MQTT broker on the Internet, such as Mosquitto or AWS IoT.
- MQTT broker set up on your computer, like Mosquitto or HiveMQ.
- MQTT broker running on your Raspberry Pi, for example, Mosquitto.
- MQTT broker on the cloud, such as Mosquitto or HiveMQ on AWS EC2.
In this guide, we will start by checking if Arduino UNO R4 can connect to an online Mosquitto broker. Arduino UNO R4 will send and receive messages to and from this broker over the internet.
Next, we will install the Mosquitto broker on our computer. After that, we will connect the Arduino UNO R4 to the MQTT broker on our computer. We will use it to send and receive data through this local broker.
Connect Arduino UNO R4 to an online MQTT broker
In this section, we'll understand how to link Arduino UNO R4 with test.mosquitto.org, an online MQTT service provided by Mosquitto. Remember, this service is meant only for testing.
Arduino UNO R4 Code
This Arduino UNO R4 code performs the following tasks:
- Connect to the MQTT broker
- Subscribe to a topic
- Regularly send messages to the same topic you subscribed to
Detailed Instructions
Follow these instructions step by step:
- If this is your first time using the Arduino Uno R4 WiFi/Minima, refer to the tutorial on setting up the environment for Arduino Uno R4 WiFi/Minima in the Arduino IDE.
- Connect the Arduino Uno R4 board to your computer using a USB cable.
- Launch the Arduino IDE on your computer.
- Select the appropriate Arduino Uno R4 board (e.g., Arduino Uno R4 WiFi) and COM port.
- Click on the "Library Manager" icon found on the left side of Arduino IDE to open it.
- In the search box, enter MQTT and search for the MQTT library by Joel Gaehwiler.
- Click the Install button to add the MQTT library.
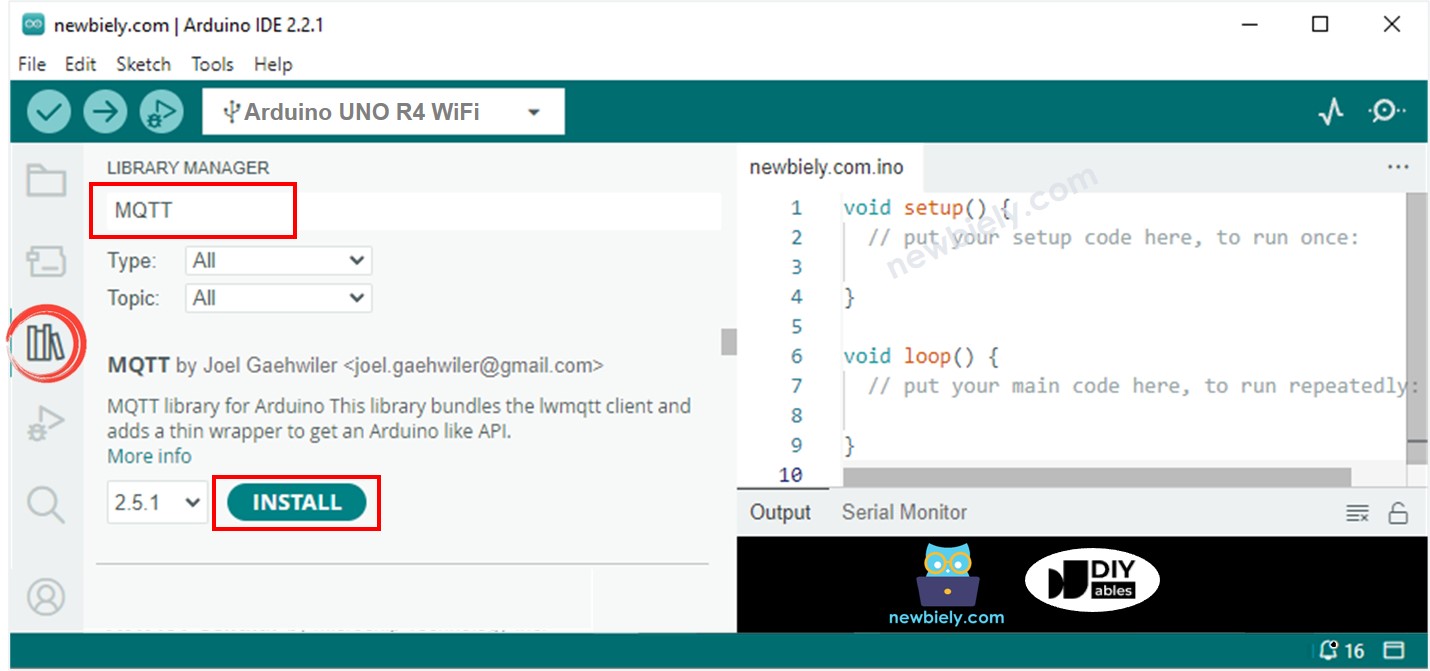
- Search for ArduinoJson in the search box and find the ArduinoJson library by Benoit Blanchon. Then, click on the Install button to install the library.
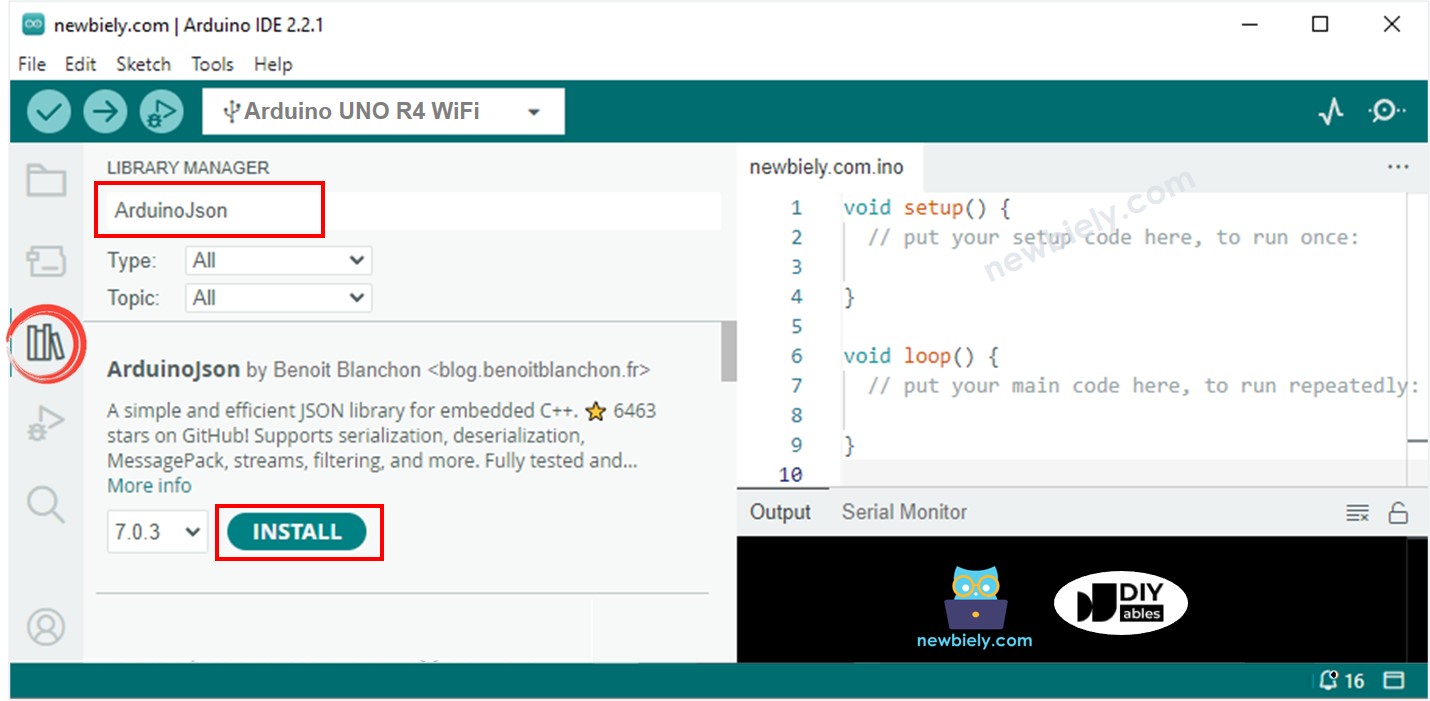
- Copy the code and open it in the Arduino IDE.
- Change the WiFi details (SSID and password) in the code to yours.
- You'll find 'YOUR-NAME' three times in the code. Replace it with your name or any letters (no spaces). This change is important to avoid conflicts from multiple users running the same code.
- Click the Upload button in Arduino IDE to upload the code to your Arduino UNO R4.
- Open the Serial Monitor.
- Check the results on the Serial Monitor.
The Arduino UNO R4 sends messages to the MQTT broker and then gets the same message back. This happens because it is set to receive messages from the same topic it sends them to. If you do not want the Arduino UNO R4 to receive its own messages, just use different topics for sending and receiving.
Connect Arduino UNO R4 to the MQTT broker installed on your PC
Installing Mosquitto MQTT Broker
- Download the Mosquitto MQTT Broker
- Install it on the D: drive, not the C: drive. This helps avoid possible problems.
Run Mosquitto MQTT broker
Now, let's see if the MQTT broker is working correctly by taking these steps:
- Navigate to the folder where you installed Mosquitto, such as D:\Draft\mosquitto. Create a file called test.conf, paste the following details into it, and save the file in this folder.
- Open a Command Prompt as Administrator on your computer. Refer to it as "Broker Window." Remember not to close this window until you finish the tutorial.
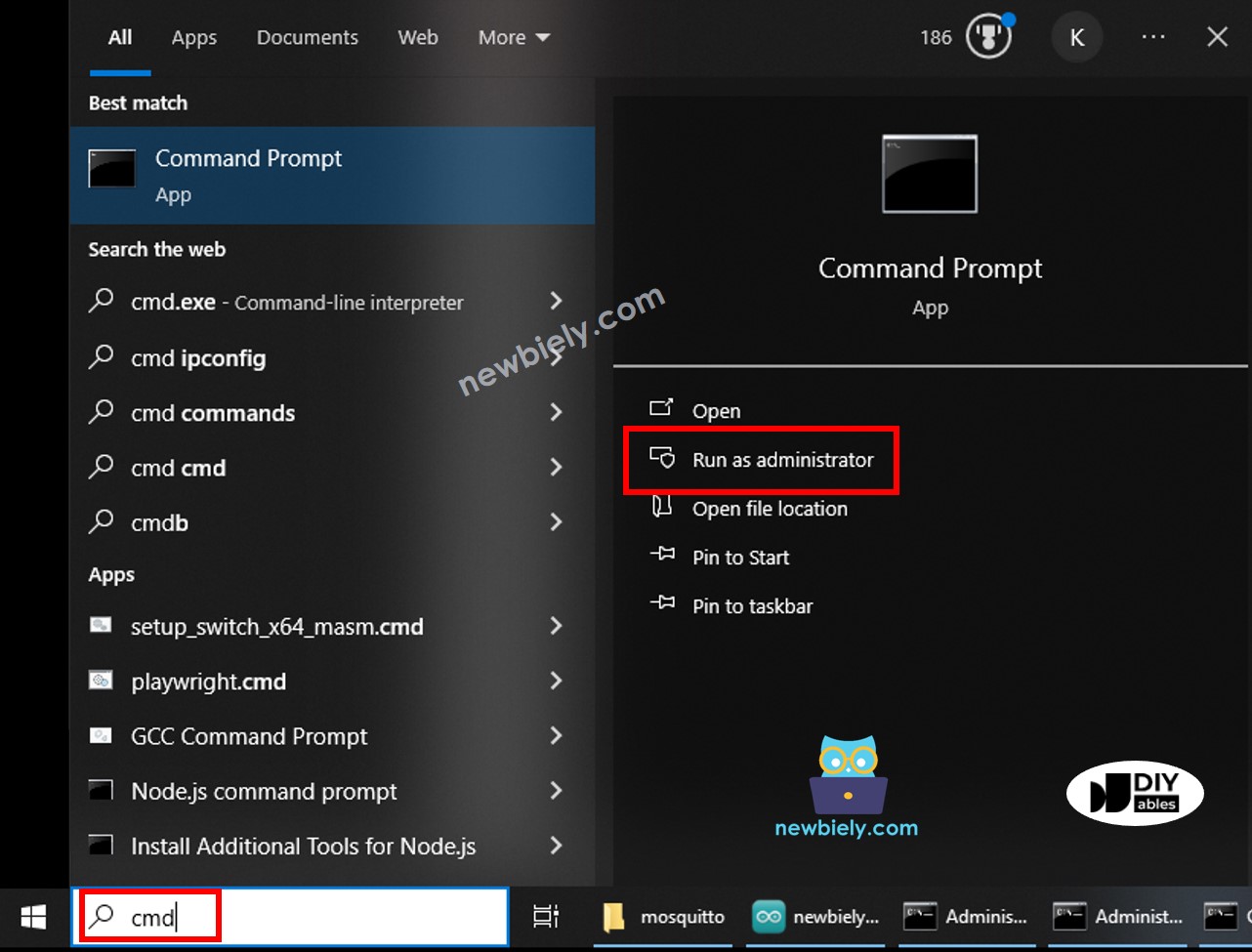
- Execute the following commands sequentially:
- You will notice:
- Open a new Command Prompt as Administrator on your computer.
- To find your computer's IP address, run this command:
- Please note the IP address for future reference. In the example provided: 192.168.0.11.
Test if the Mosquitto Broker works
- Open another Command Prompt as Administrator on your computer. We will refer to it as "Subscriber Window."
- To subscribe to a topic, enter the following commands one at a time, replacing "your IP address" with your actual IP address:
- Open an additional Command Prompt with Administrator rights on your computer. Name it Publisher Window.
- To publish a message to the same topic, execute the following commands one after another (substitute with your IP address):
- You will notice:
The message is sent to the Subscriber Window like this:
You have successfully installed the Mosquitto MQTT broker on your PC. Please do not close three windows: Broker Window, Subscriber Window, and Publisher Window. We will use them next.
Arduino UNO R4 Code
The following code is for Arduino UNO R4 and it does the following:
- Connect to the MQTT broker
- Subscribe to a topic
- Regularly send messages to a different topic
Detailed Instructions
- Copy the code above and open it using Arduino IDE
- Change the WiFi details (SSID and password) in the code to yours.
- Change the MQTT broker address in the code (either domain name or IP address).
- Click the Upload button in Arduino IDE to send the code to your Arduino UNO R4.
Send message from Arduino UNO R4 to PC via MQTT
The Arduino UNO R4 sends information to the MQTT topic named arduino-uno-r4/send. On a computer, the Subscriber Window subscribes to that topic to receive the data.
- Open the Serial Monitor to see that the Arduino UNO R4 regularly sends a message to a topic.
- Look at the Subscriber Window and you'll notice it shows the message sent by the Arduino UNO R4 like this:
Send message from PC to Arduino UNO R4 via MQTT
The Arduino UNO R4 is set to receive messages from the topic arduino-uno-r4/receive. The Publisher Window on the PC sends messages to this topic to communicate with the Arduino UNO R4.
- Send a message to the topic subscribed by Arduino UNO R4 using this command in the Publisher Window:
- You will notice that Arduino UNO R4 receives this message, as shown on the Serial Monitor: