Arduino UNO R4 - Relay
This tutorial instructs you on how to use an Arduino UNO R4 and a relay to control the on and off states of devices that use high-voltage power, such as light bulbs, fans, electromagnetic locks, and linear actuators. In detail, we will learn:
- How a relay works.
- How to connect the relay to a high-voltage device.
- How to connect the Arduino UNO R4 to the relay.
- How to program the Arduino UNO R4 to control the relay to turn high-voltage devices on and off.
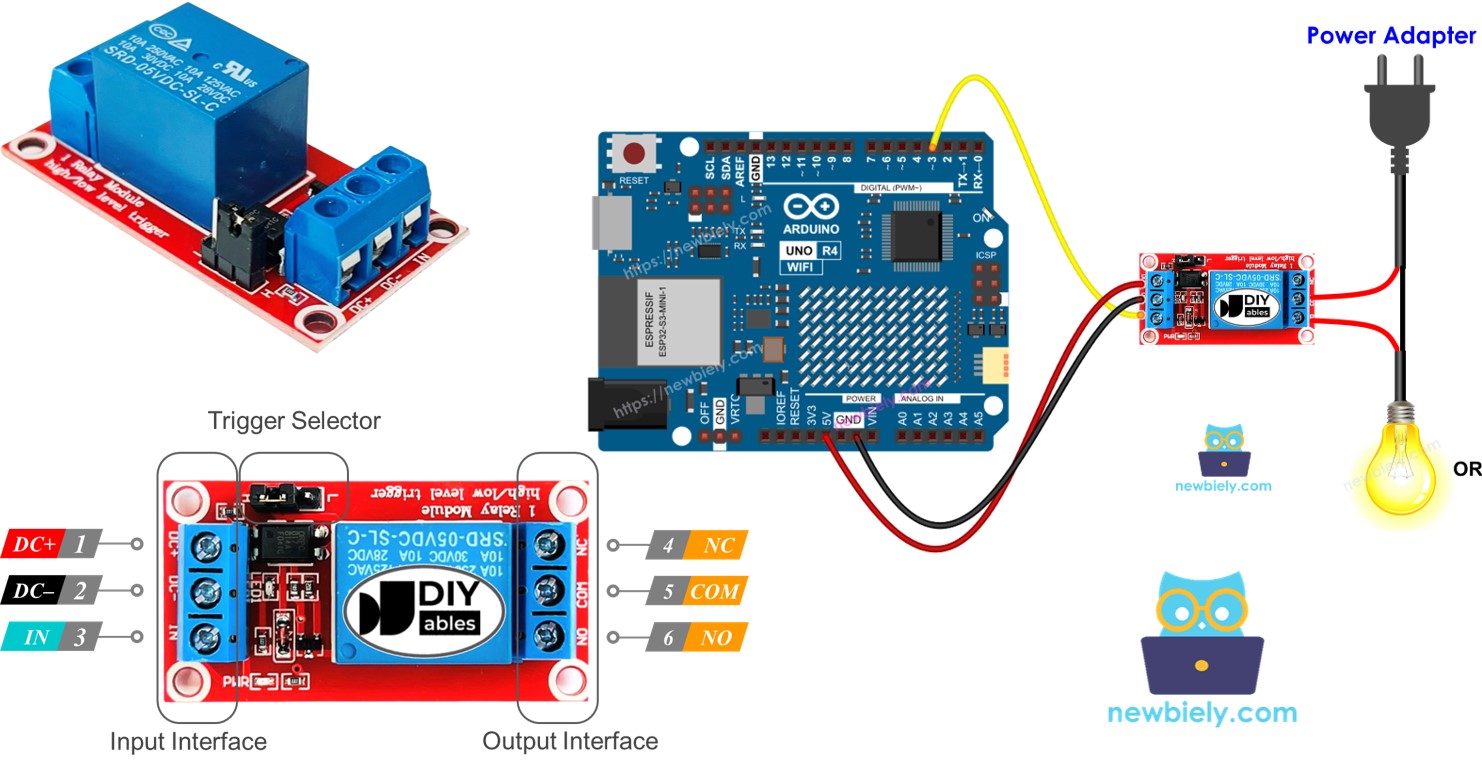
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Relay
A relay is a switch that you can program, controlled by devices like Arduino UNO R4 or other micro-controllers. It lets you turn devices on or off automatically, especially those that use high voltage or high current.
The relay is a bridge between Arduino UNO R4 and high-voltage devices.
WARNING
When working on projects connected to main electricity, it is critical to have proper knowledge to avoid shocking yourself. Safety is very important. If you are not completely sure about what you are doing, please do not attempt to handle anything. Instead, seek help from someone experienced.
We recommend using a DC device (up to 24V) for testing, even though some relays can work with both DC and AC devices.
Relay Pinout
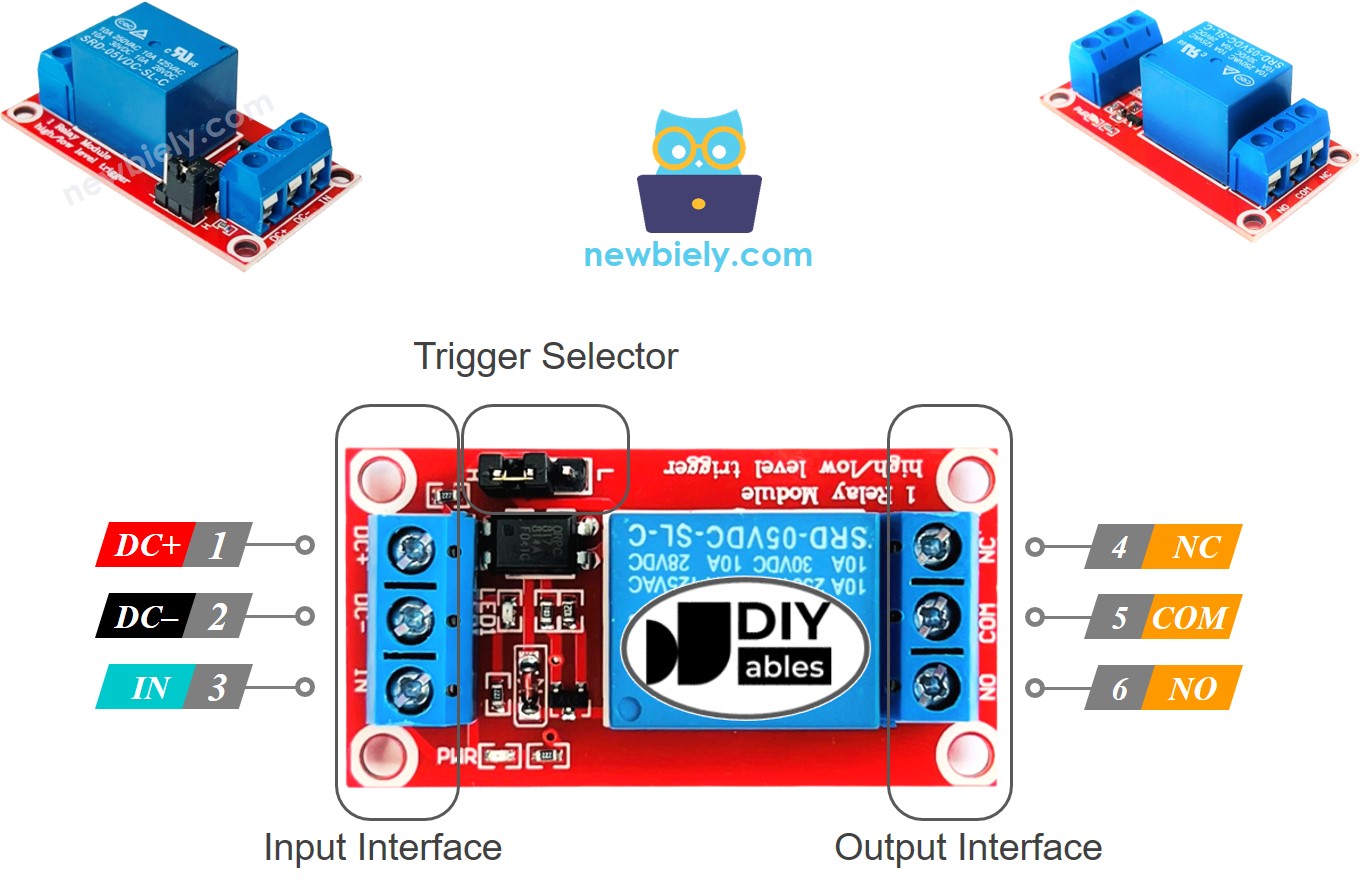
A relay consists of two sets of pins: the input pins, which use low voltage, and the output pins which use high voltage.
- The pins in the input group are connected to the Arduino UNO R4 and include three pins:
- DC- pin: connect this to GND (0V).
- DC+ pin: connect this to VCC (5V).
- IN pin: this accepts the control signal from the Arduino UNO R4.
- The pins in the output group connect to the high-voltage device and also have three pins (usually found in a screw terminal):
- COM pin: this is the common pin used in both the normally open mode and the normally closed mode.
- NO pin: this is the normally open pin. It is used in the normally open mode.
- NC in: this is the normally closed pin. It is used in the normally closed mode.
- For normally open mode, we use only the COM pin and the NO pin.
- For normally closed mode, we use only the COM pin and the NC pin.
- LOW level trigger mode
- HIGH level trigger mode
- normally open mode
- normally closed mode.
- The normally open mode and normally closed mode work in opposite ways.
- Most relay modules support both normally open and normally closed modes.
- The LOW level trigger and HIGH level trigger modes work in opposite ways.
- Not every relay module supports both LOW level trigger and HIGH level trigger modes.
- At any given time, the relay module can operate in only one mode, either LOW level trigger or "HIGH level trigger."
- When the IN pin is connected to LOW (0V), the switch is open and the device is OFF.
- When the IN pin is connected to HIGH (5V), the switch is closed and the device is ON.
- When the IN pin is connected to LOW (0V), the switch is closed. This means the device is ON.
- When the IN pin is connected to HIGH (5V), the switch is open. This means the device is OFF.
- Attach the pin from the Arduino UNO R4 to the IN pin on the relay.
- Program the pin to be LOW or HIGH to operate the relay.
In practice, we usually do not use all the pins in the high voltage group. We only use two of them.
Also, if the relay can use both LOW and HIGH level triggers, there is typically a jumper to choose either LOW level trigger or HIGH level trigger.
※ NOTE THAT:
Different manufacturers may arrange the pins on the relay module in different orders. Always check and follow the labels on the relay to connect it correctly. Make sure to look carefully!
How to Connect the High Voltage Device to Relay
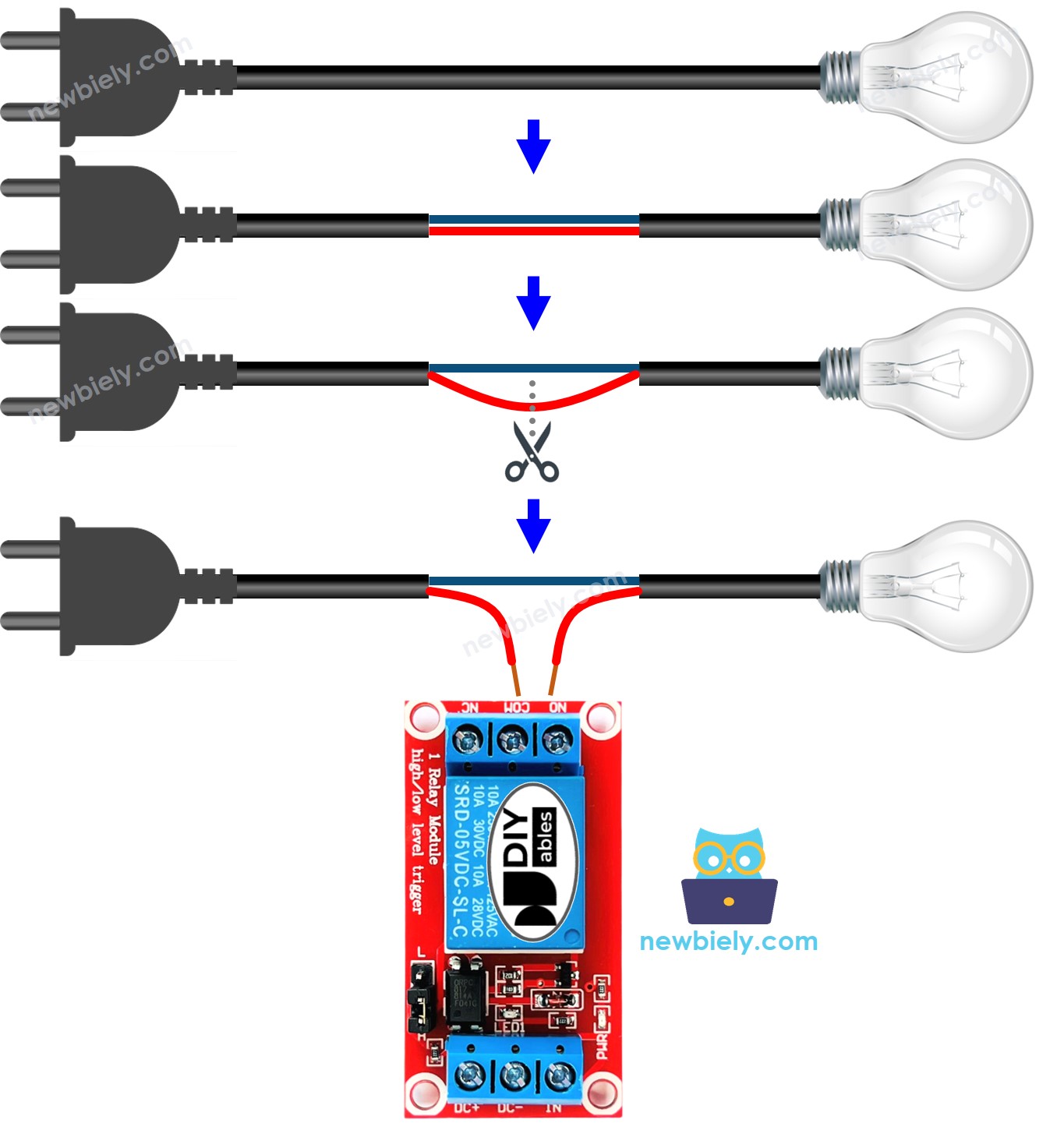
How It Works
A relay might works in different ways depending on the manufacturer and how the user installs it.
The input mode: There are two input modes that make the relay function oppositely:
The output mode: There are two output modes that make the relay work oppositely:
The term “normally” refers to the condition when the "IN pin" is connected to "LOW (0V)". Let's start with some quick information:
The combination of input modes and output modes leads to many use cases. For beginners, we suggest choosing HIGH level trigger mode and normally open mode.
The LOW level trigger and HIGH level trigger modes function in opposite ways. Next, we will describe the HIGH level trigger mode in detail. The LOW level trigger operates in the opposite manner.
HIGH Level Trigger - Normally Open Mode
To set this mode, connect the high voltage device to both the COM pin and the NO pin.
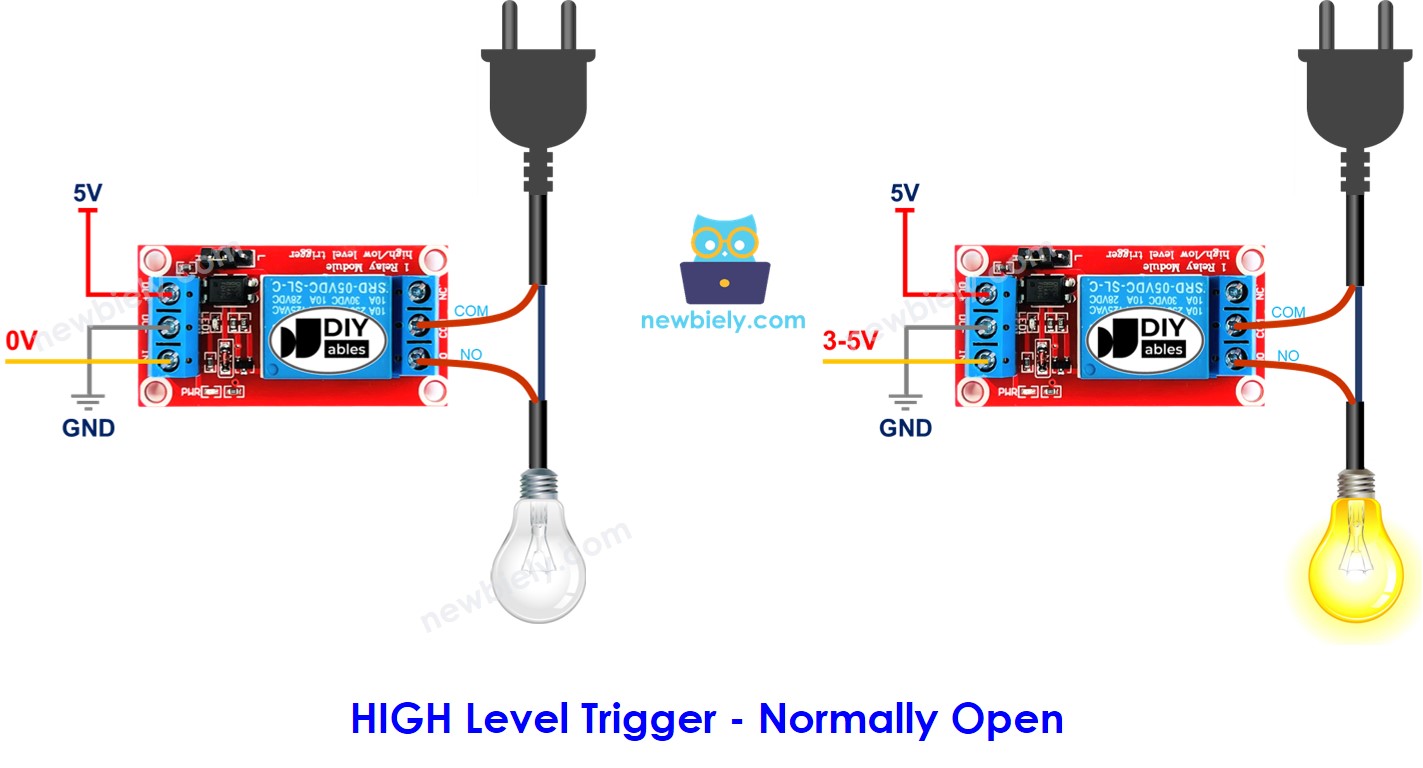
HIGH Level Trigger - Normally Closed Mode
To use this mode, connect the device with high voltage to the COM pin and the NC pin.
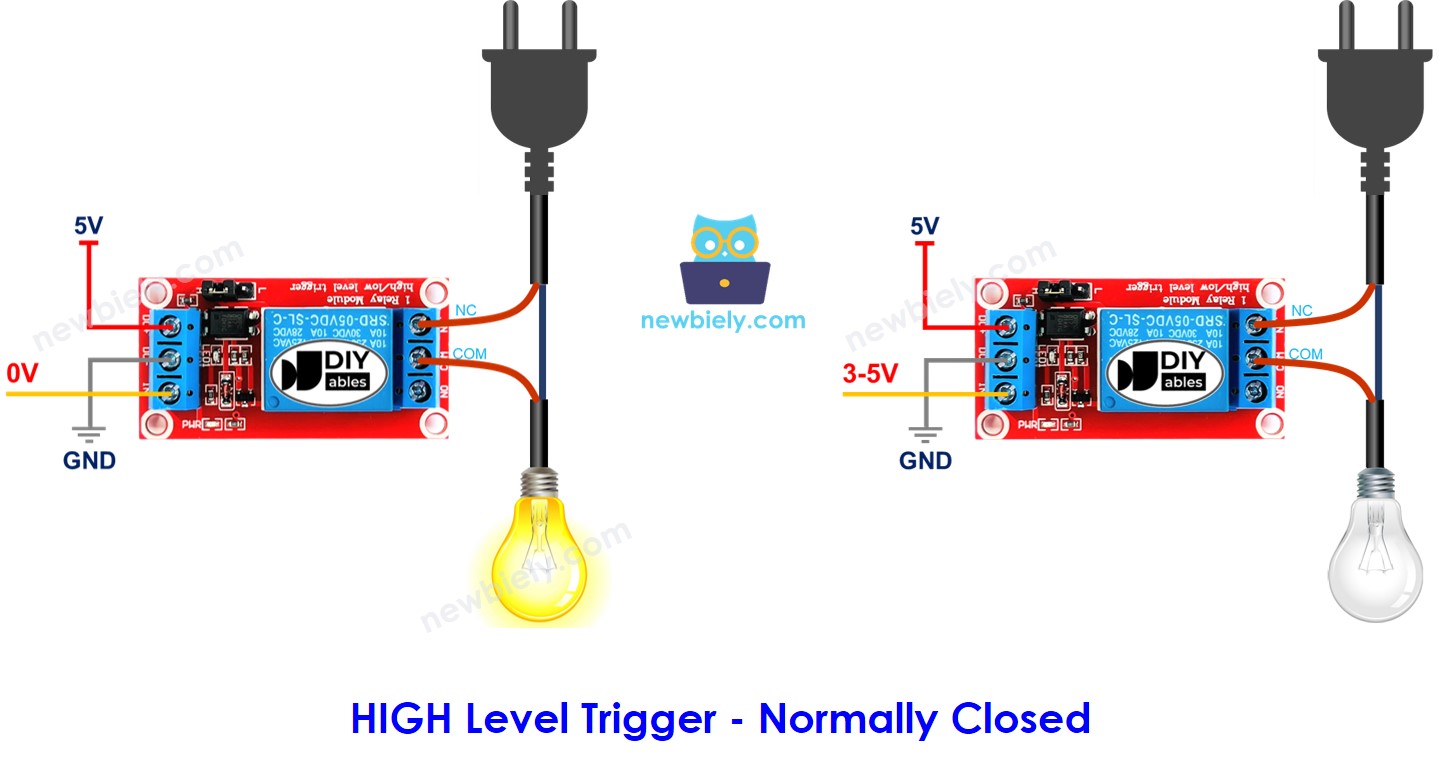
Summary
Input modes | Output Modes | IN pin (programmable) | Output pins | Relay state | Device state |
---|---|---|---|---|---|
HIGH Trigger | Normally Open | LOW | COM and NO pin | ⇒ open | ⇒ OFF |
HIGH Trigger | Normally Open | HIGH | COM and NO pin | ⇒ closed | ⇒ ON |
HIGH Trigger | Normally Closed | LOW | COM and NC pin | ⇒ closed | ⇒ ON |
HIGH Trigger | Normally Closed | HIGH | COM and NC pin | ⇒ open | ⇒ OFF |
LOW Trigger | Normally Open | LOW | COM and NO pin | ⇒ closed | ⇒ ON |
LOW Trigger | Normally Open | HIGH | COM and NO pin | ⇒ open | ⇒ OFF |
LOW Trigger | Normally Closed | LOW | COM and NC pin | ⇒ open | ⇒ OFF |
LOW Trigger | Normally Closed | HIGH | COM and NC pin | ⇒ closed | ⇒ ON |
There can be as many as 8 use cases. This might seem overwhelming. But if you are a beginner, you only need to focus on the first two cases. These involve the HIGH level trigger and normally open settings. This tutorial will mainly cover these two cases.
Arduino UNO R4 - Relay
The Arduino UNO R4 uses a relay to control a device that operates at high voltage.
To control a relay is easy. We just need:
Wiring Diagram
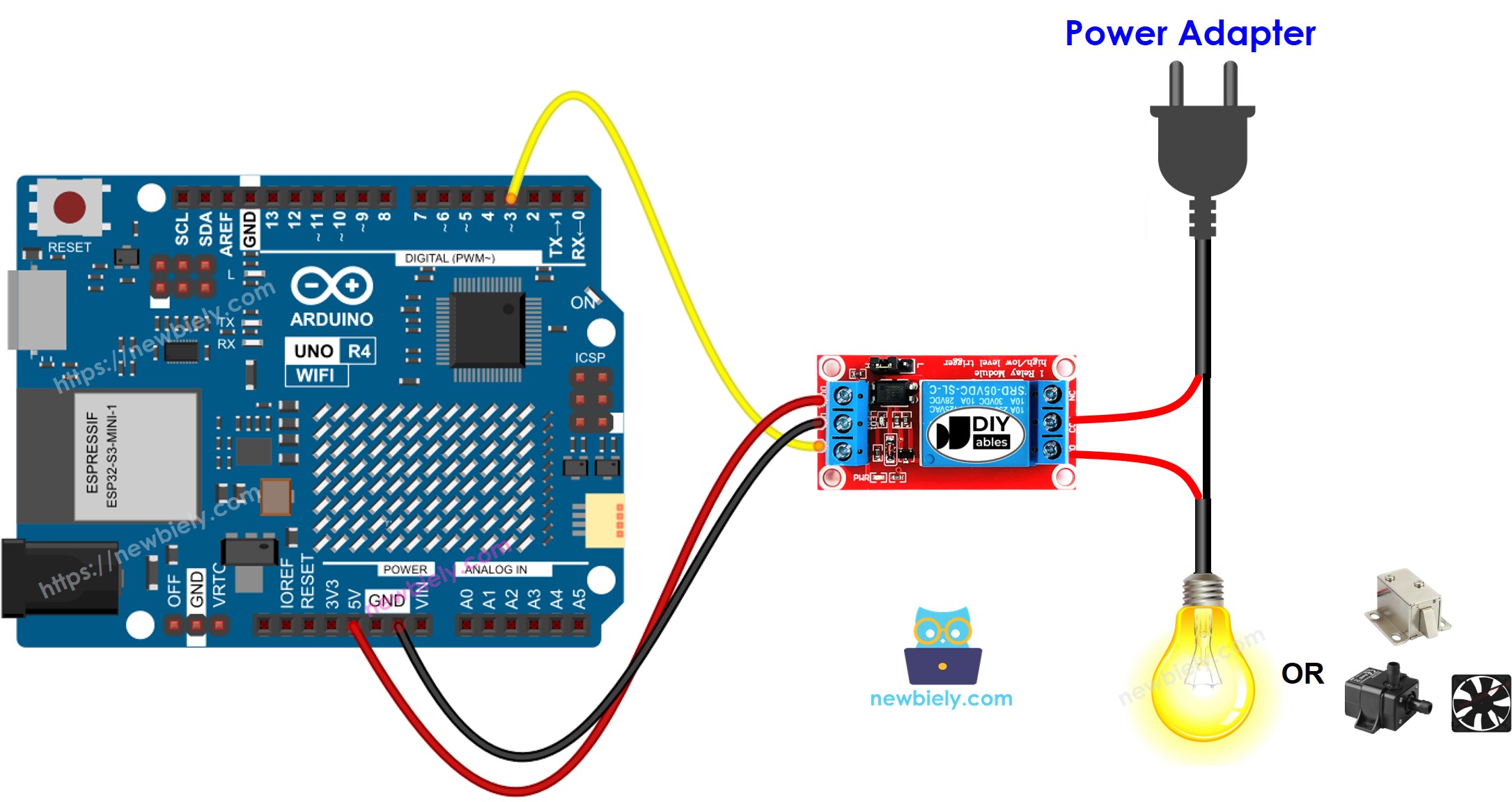
This image is created using Fritzing. Click to enlarge image
See The best way to supply power to the Arduino Uno R4 and other components.
How To Program For Relay
- Set up the digital output mode for an Arduino UNO R4 pin using the pinMode() function. For instance, for pin 3:
- Set the pin to LOW (0 volts) using the digitalWrite() function.
- Set the pin to HIGH (5V) using the digitalWrite() function.
Arduino UNO R4 Code
Detailed Instructions
Follow these instructions step by step:
- If this is your first time using the Arduino Uno R4 WiFi/Minima, refer to the tutorial on setting up the environment for Arduino Uno R4 WiFi/Minima in the Arduino IDE.
- Wire the components according to the provided diagram.
- Connect the Arduino Uno R4 board to your computer using a USB cable.
- Launch the Arduino IDE on your computer.
- Select the appropriate Arduino Uno R4 board (e.g., Arduino Uno R4 WiFi) and COM port.
- Copy the code and open it in Arduino IDE
- Click the Upload button in Arduino IDE to send the code to Arduino UNO R4
- Check the LED strip: it should be blinking