Arduino UNO R4 - Soil Moisture Sensor
In this tutorial, we will learn how to use a moisture sensor with the Arduino UNO R4. Specifically, we will cover:
- Comparison between resistive and capacitive moisture sensors
- How to program Arduino UNO R4 to read values from capacitive moisture sensor
- How to calibrate a capacitive moisture sensor
- How to check if the soil is moist or dry
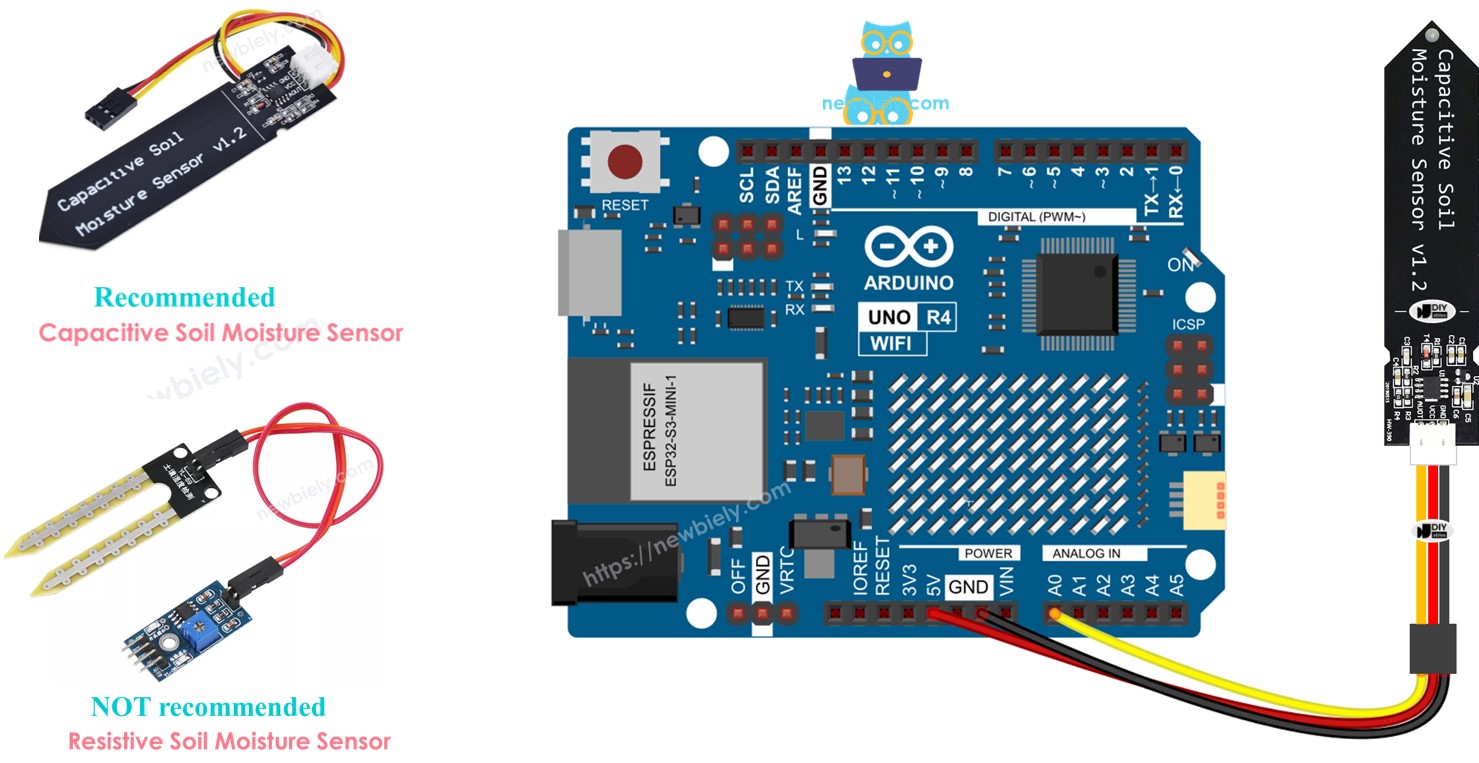
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables.
Overview of Soil Moisture Sensor Sensor
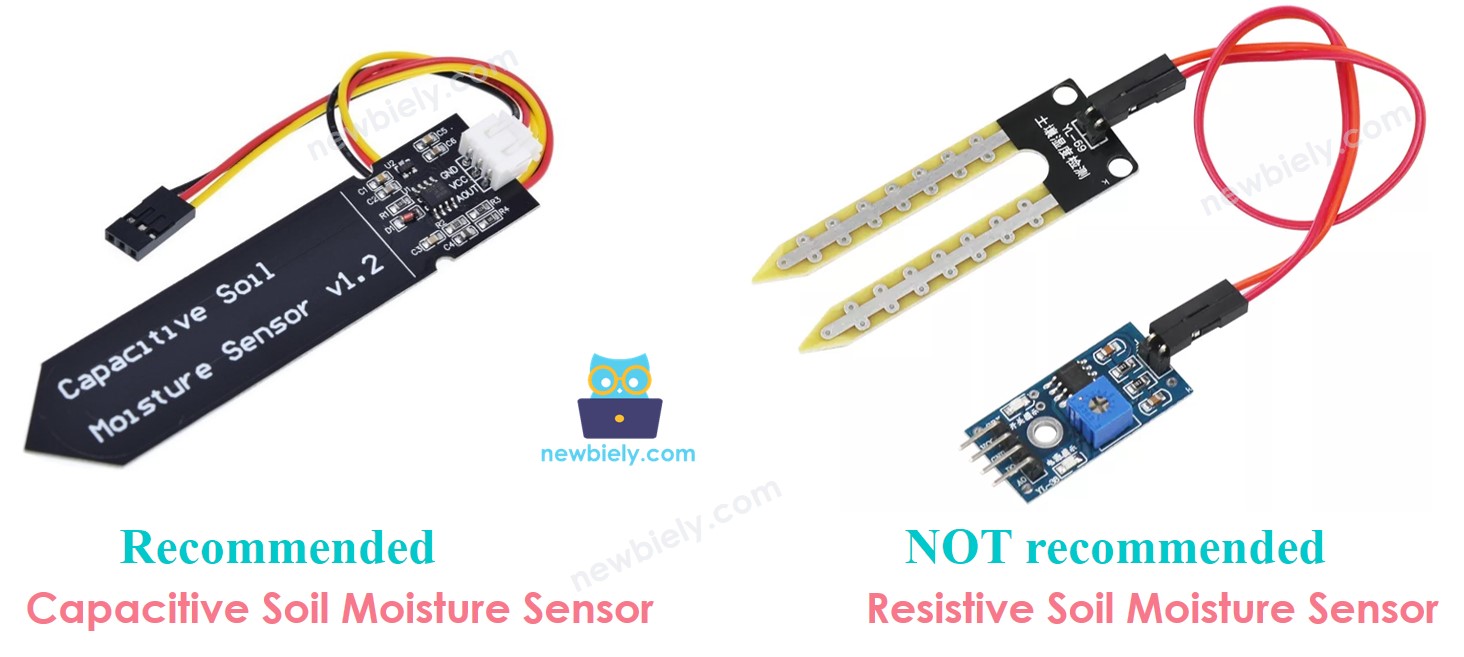
There are two types of moisture sensors:
- Capacitive moisture sensor
- Resistive moisture sensor
Both sensors give information about soil moisture, but they work in different ways. We strongly suggest using the capacitive moisture sensor for this reason:
- The resistive soil moisture sensor gets damaged gradually. This happens because there is an electrical current running between its probes, leading to a type of damage called electrochemical corrosion.
- The capacitive soil moisture sensor does not get damaged over time. The reason is that its electrodes are covered and do not corrode easily.
The image below displays a soil moisture sensor made of resistive material that has corroded over time.
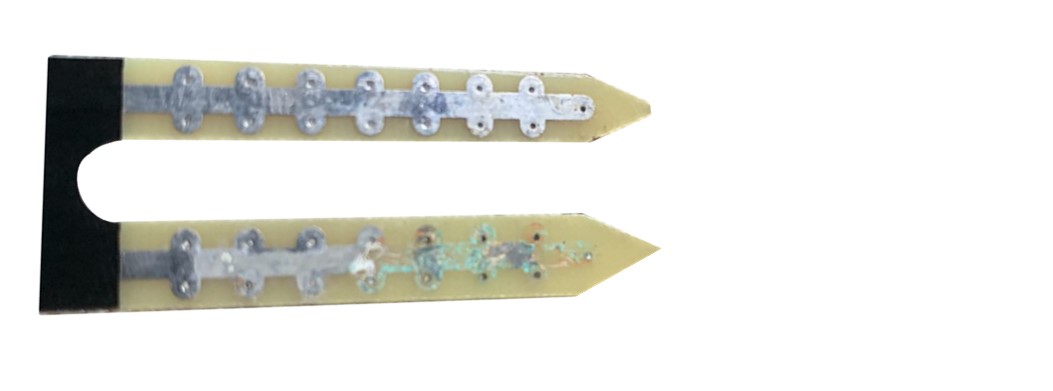
The remaining part of this tutorial will focus on using the capacitive soil moisture sensor.
Capacitive Soil Moisture Sensor Pinout
A capacitive soil moisture sensor comes with three pins:
- GND pin: connect to GND (0V)
- VCC pin: connect to VCC (5V or 3.3V)
- AOUT pin: sends an analog signal which changes with soil moisture. Connect to the analog input pin on an Arduino UNO R4.
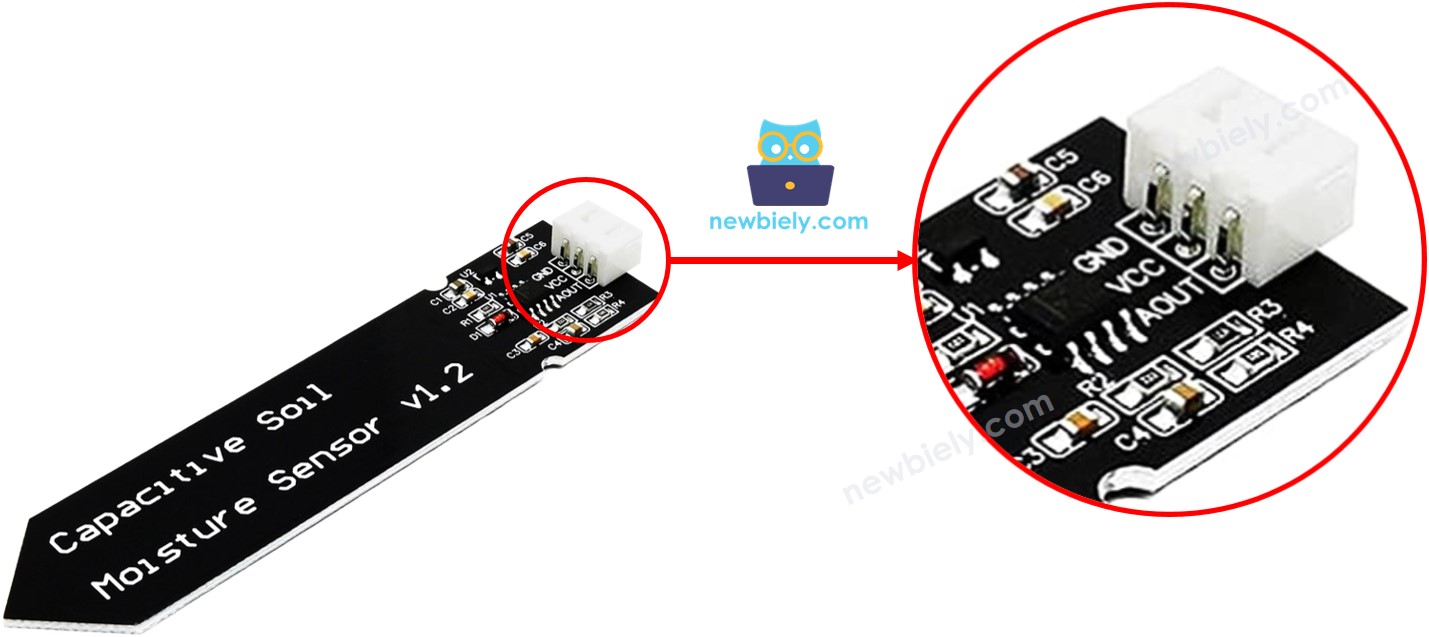
How It Works
The higher the water content in the soil, the lower the voltage at the AOUT pin.
Wiring Diagram
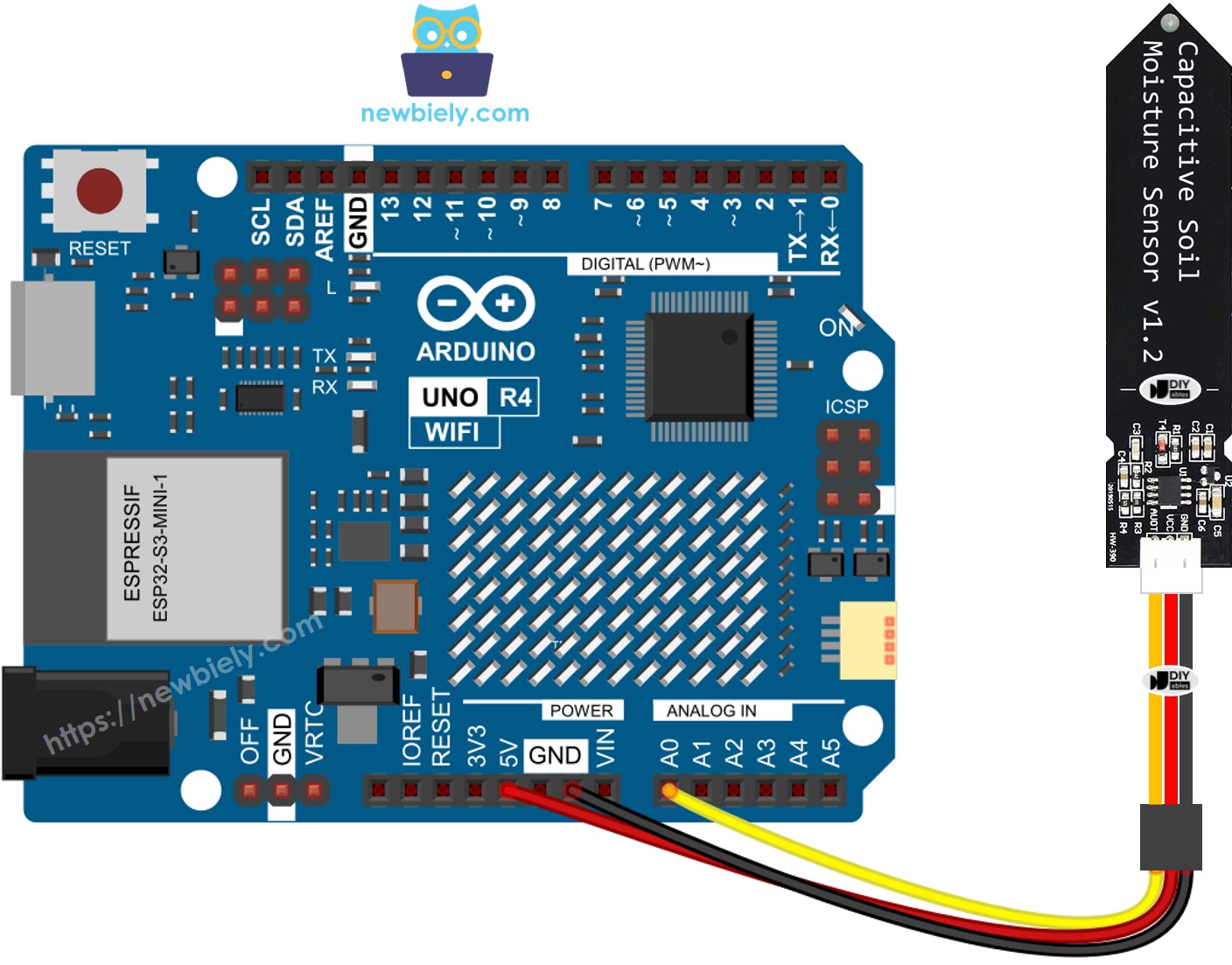
This image is created using Fritzing. Click to enlarge image
Arduino UNO R4 Code
Detailed Instructions
Follow these instructions step by step:
- If this is your first time using the Arduino Uno R4 WiFi/Minima, refer to the tutorial on setting up the environment for Arduino Uno R4 WiFi/Minima in the Arduino IDE.
- Connect the soil moisture sensor to the Arduino Uno R4 according to the provided diagram.
- Connect the Arduino Uno R4 board to your computer using a USB cable.
- Launch the Arduino IDE on your computer.
- Select the appropriate Arduino Uno R4 board (e.g., Arduino Uno R4 WiFi) and COM port.
- Copy the code above and open it in Arduino IDE.
- Click the Upload button in Arduino IDE to upload the code to Arduino UNO R4.
- Place the sensor in the soil, then add water to the soil. Alternatively, you can gently place it into a cup of salt water.
- Check the results on the Serial Monitor. It will display as follows:
※ NOTE THAT:
- Don't use pure water for testing because it doesn't conduct electricity, so it won't affect the sensor readings.
- Normally, the sensor readings don't fall to zero. They usually stay between 500 and 600. However, this can vary depending on how deep the sensor is in the soil or water, the type of soil or water, and the power supply voltage.
- Do not put the circuit part of the sensor (located at the top) into the soil or water as it can damage the sensor.
Calibration for Capacitive Soil Moisture Sensor
The reading from the moisture sensor is not fixed; it varies with the type of soil and its water content. To use it correctly, we must calibrate it to find a cutoff point that tells us when the soil is wet or dry.
How to Perform Calibration:
- Use the Arduino UNO R4 to execute the provided code.
- Insert the moisture sensor into the soil.
- Gradually add water to the soil.
- Observe the Serial Monitor.
- Record the value when you think the soil shift from dry to wet. This value is known as THRESHOLD.
Determine if the soil is wet or dry
After you calibrate, change the THRESHOLD value you noted to the following code. This code checks if the soil is wet or dry.
The outcome as seen on the Serial Monitor.