Arduino UNO R4 - Temperature Sensor
This tutorial instructs you how to use the DS18B20 1-wire temperature sensor with the Arduino UNO R4. In detail, we will learn:
- How to connect the DS18B20 temperature sensor to Arduino UNO R4.
- How to connect the DS18B20 temperature sensor to Arduino UNO R4 using adapter.
- How to program Arduino UNO R4 to read the temperature value from the DS18B20 sensor.
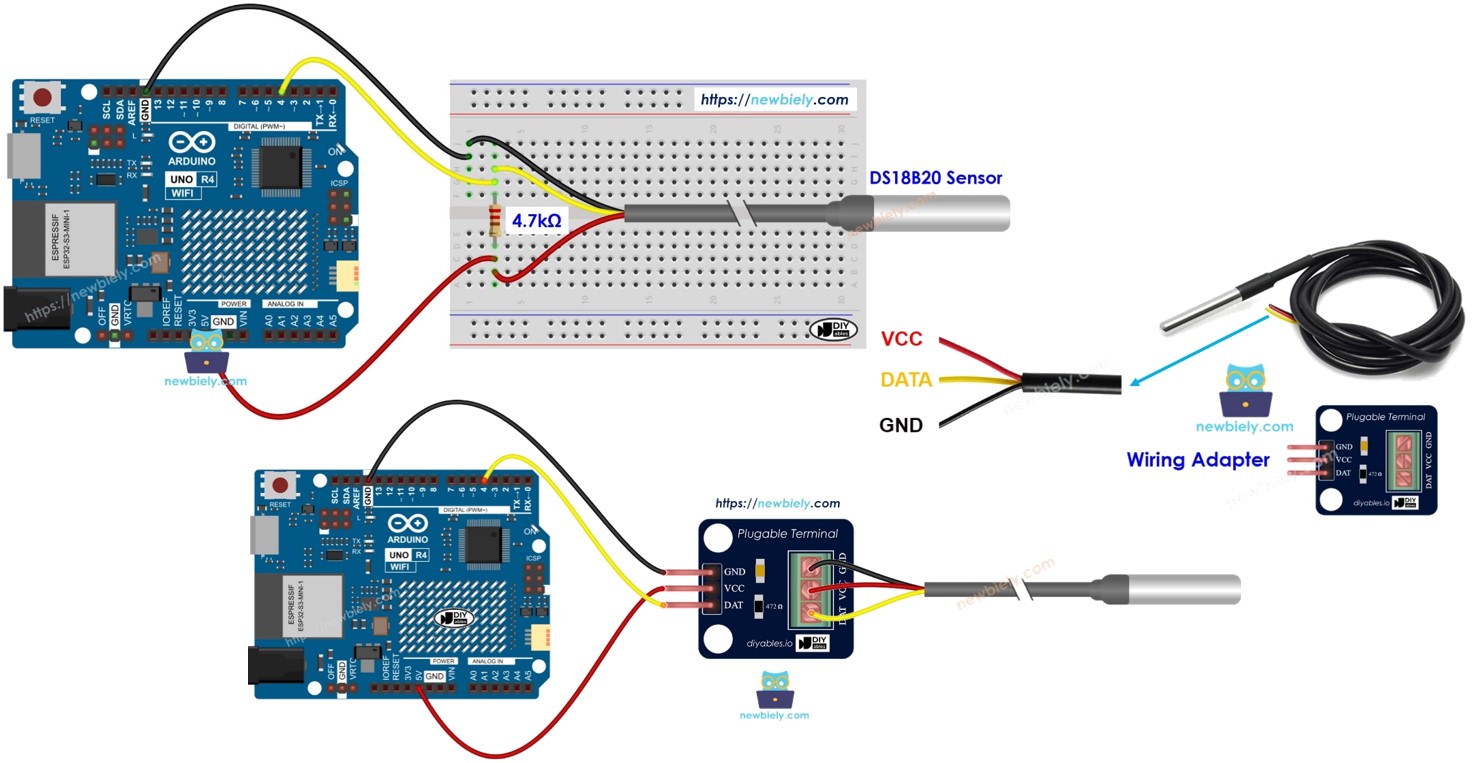
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Buy Note: Many DS18B20 sensors available in the market are unreliable. We strongly recommend buying the sensor from the DIYables brand using the link provided above. We tested it, and it worked reliably.
Overview of One Wire Temperature Sensor DS18B20
Pinout
The DS18B20 temperature sensor comes with three pins.
- GND pin: Connect this to GND (0V).
- VCC pin: Connect this to VCC (5V or 3.3V).
- DATA pin: This is the 1-Wire Data bus. Connect it to a digital pin on the Arduino UNO R4.
The sensor comes in two types: the TO-92 package, which looks like a transistor, and the waterproof probe. In this tutorial, we will use the waterproof probe type.
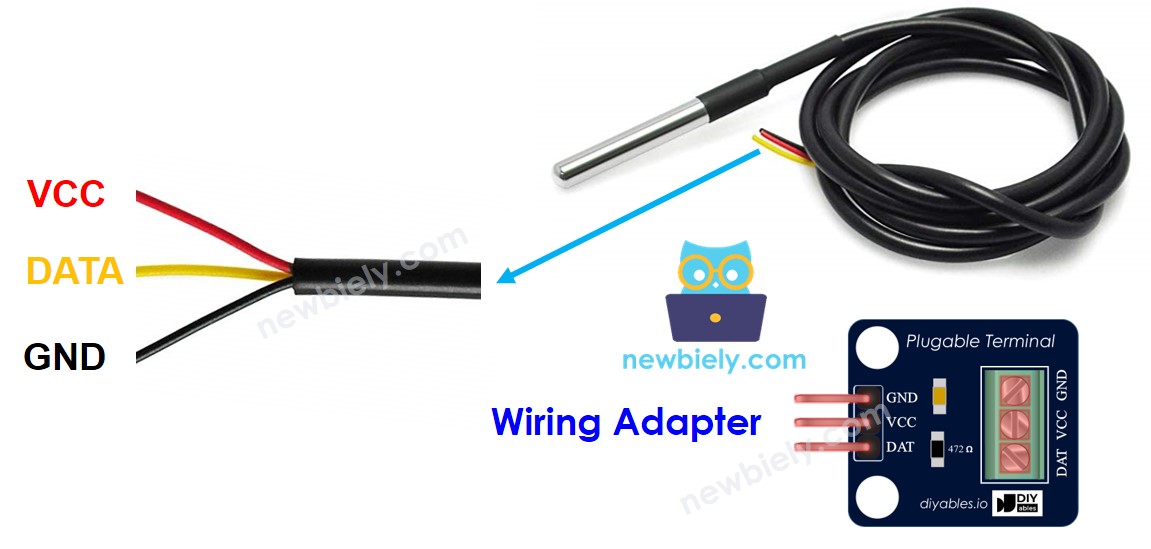
To connect a DS18B20 temperature sensor to an Arduino UNO R4, you need a pull-up resistor, which can be complicated. Some manufacturers provide a wiring adapter with a pull-up resistor and a screw terminal block already included, which simplifies the setup.
Wiring Diagram
- Breadboard wiring diagram
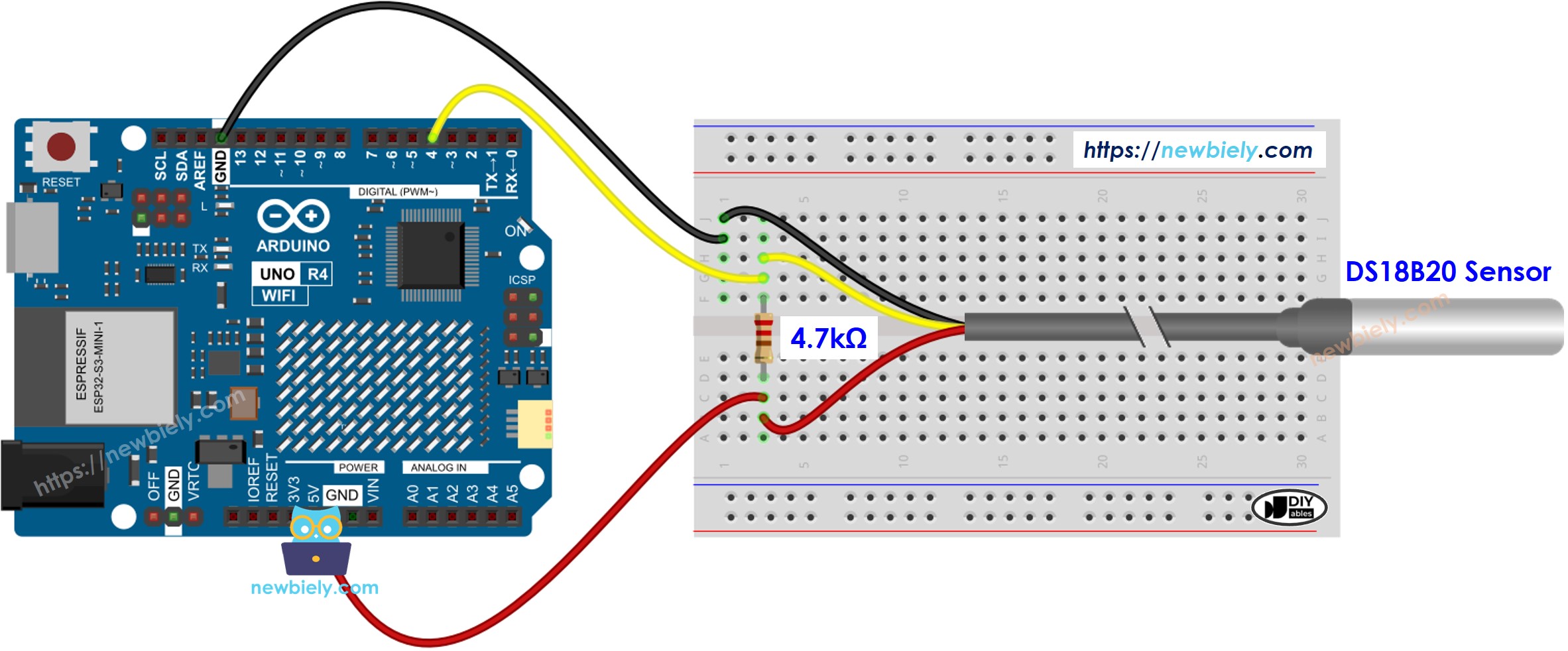
This image is created using Fritzing. Click to enlarge image
- Wiring diagram with the wiring adapter
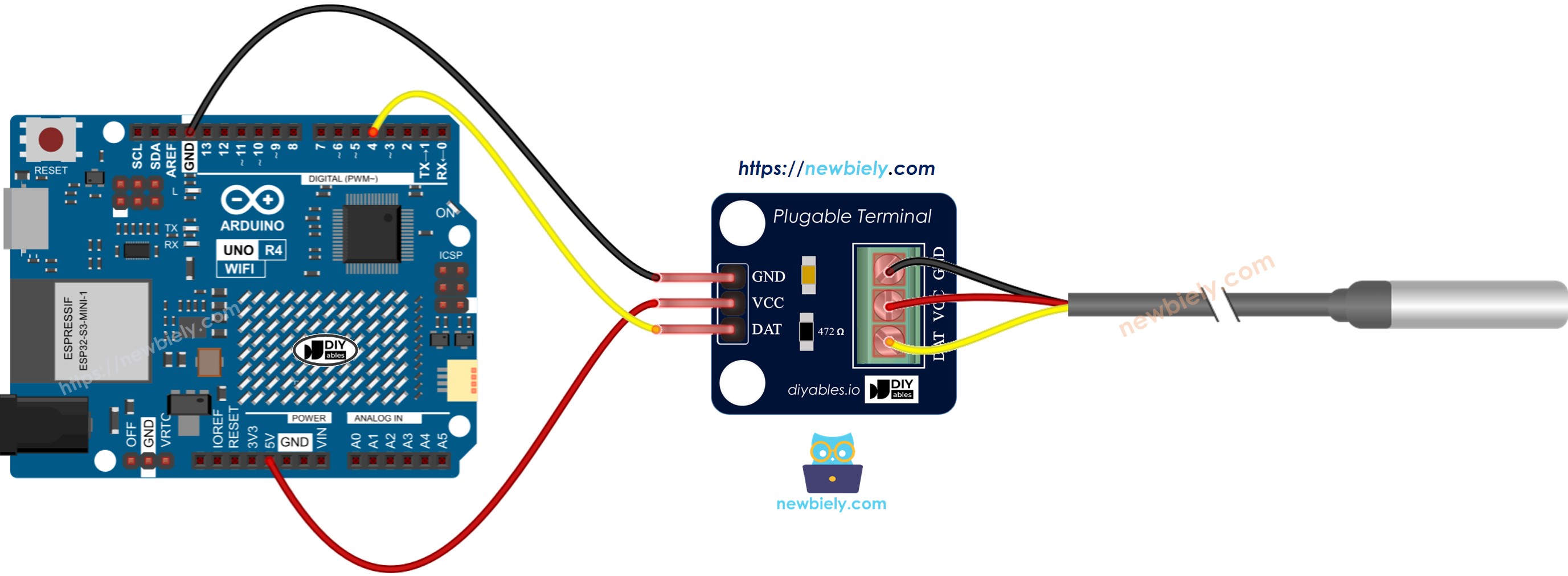
This image is created using Fritzing. Click to enlarge image
See The best way to supply power to the Arduino Uno R4 and other components.
We recommend buying a DS18B20 sensor with a wiring adapter. This adapter makes it simple to connect because it includes a resistor, so you don't need an extra one.
How To Program For DS18B20 Temperature Sensor
- Include the library:
- Create a OneWire object and a DallasTemperature object for the pin connected to the sensor's DATA pin.
- Set up the sensor:
- Send the command to check temperatures:
- Check the temperature in Celsius.
- (Optional) Change Celsius temperature to Fahrenheit.
Arduino UNO R4 Code
Detailed Instructions
Follow these instructions step by step:
- If this is your first time using the Arduino Uno R4 WiFi/Minima, refer to the tutorial on setting up the environment for Arduino Uno R4 WiFi/Minima in the Arduino IDE.
- Connect the DS18B20 1-wire temperature sensor to the Arduino Uno R4 according to the provided diagram.
- Connect the Arduino Uno R4 board to your computer using a USB cable.
- Launch the Arduino IDE on your computer.
- Select the appropriate Arduino Uno R4 board (e.g., Arduino Uno R4 WiFi) and COM port.
- Click on the Libraries icon on the left side of the Arduino IDE.
- Search for DallasTemperature and find the library by Miles Burton.
- Click the Install button to add the DallasTemperature library.
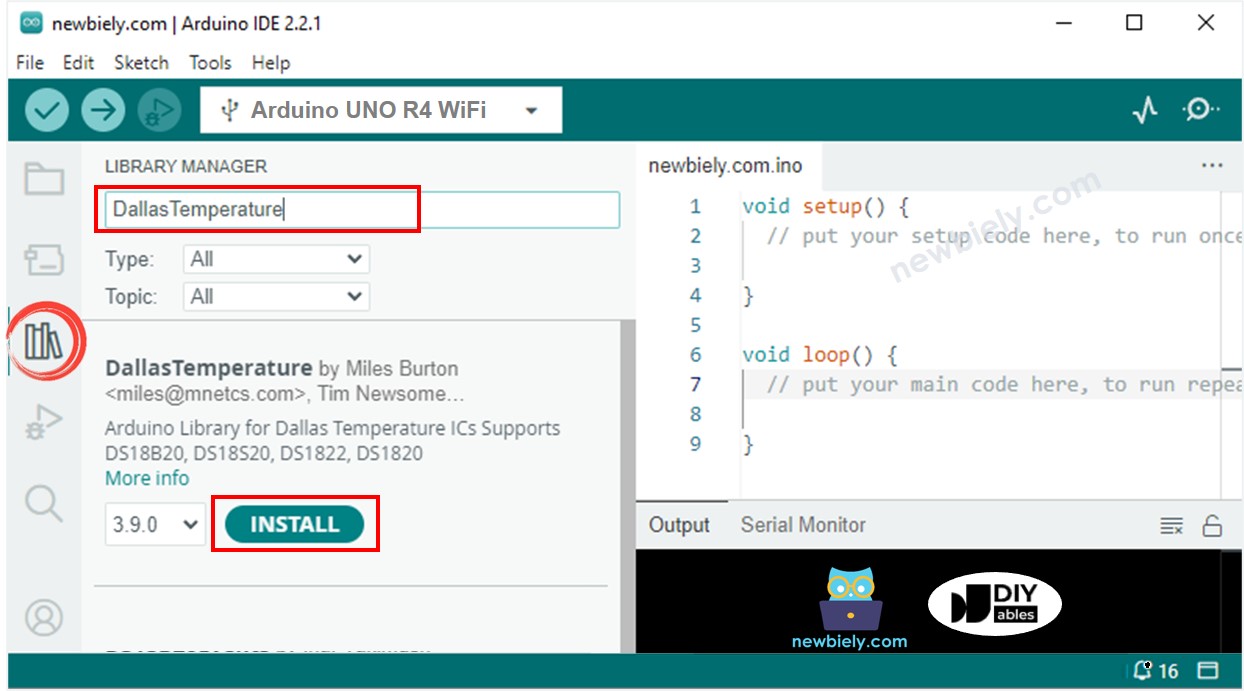
- You need to install a library dependency
- Click the Install All button to install the OneWire library.
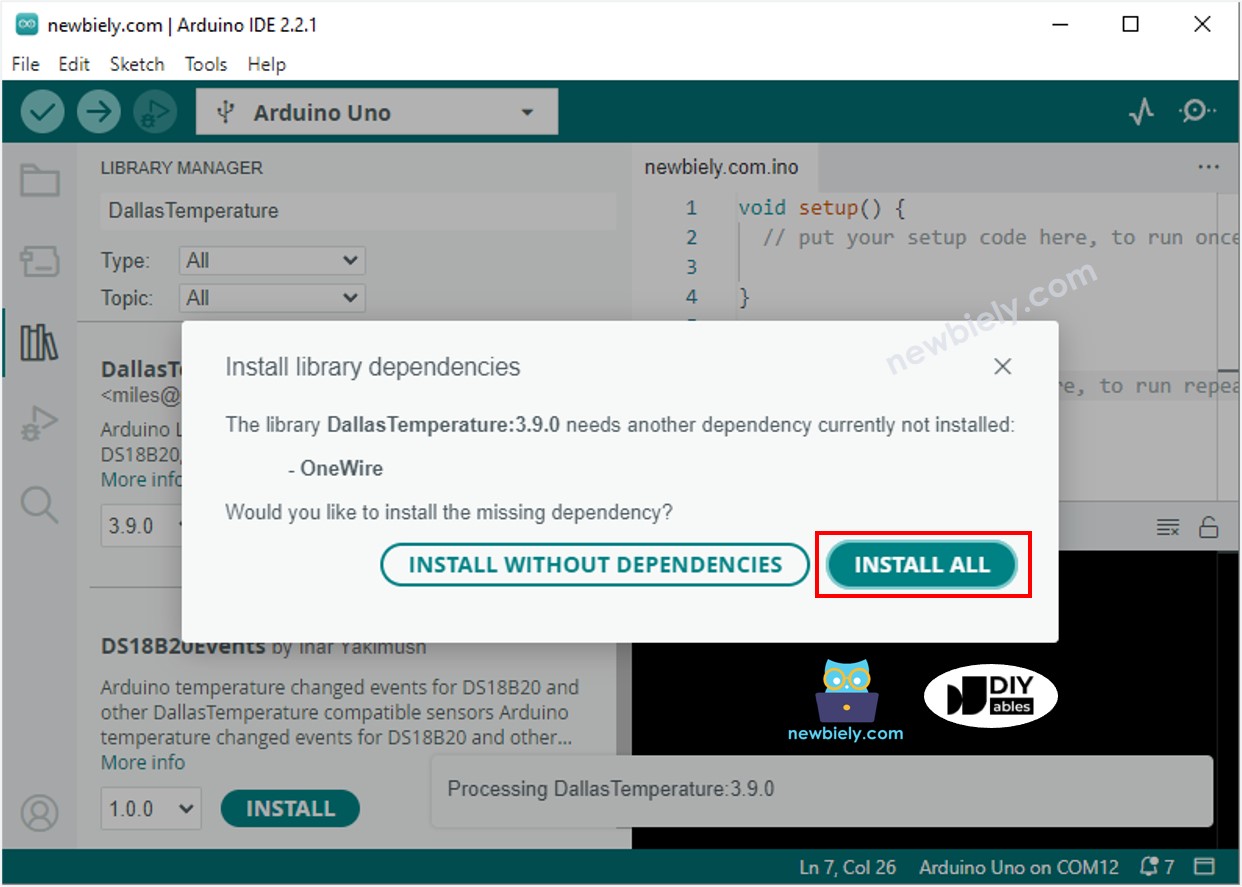
- Copy the code and open it in Arduino IDE.
- Click the Upload button in Arduino IDE to send the code to Arduino UNO R4.
- Place the sensor in hot and cold water, or hold it in your hand.
- Check the results on the Serial Monitor.